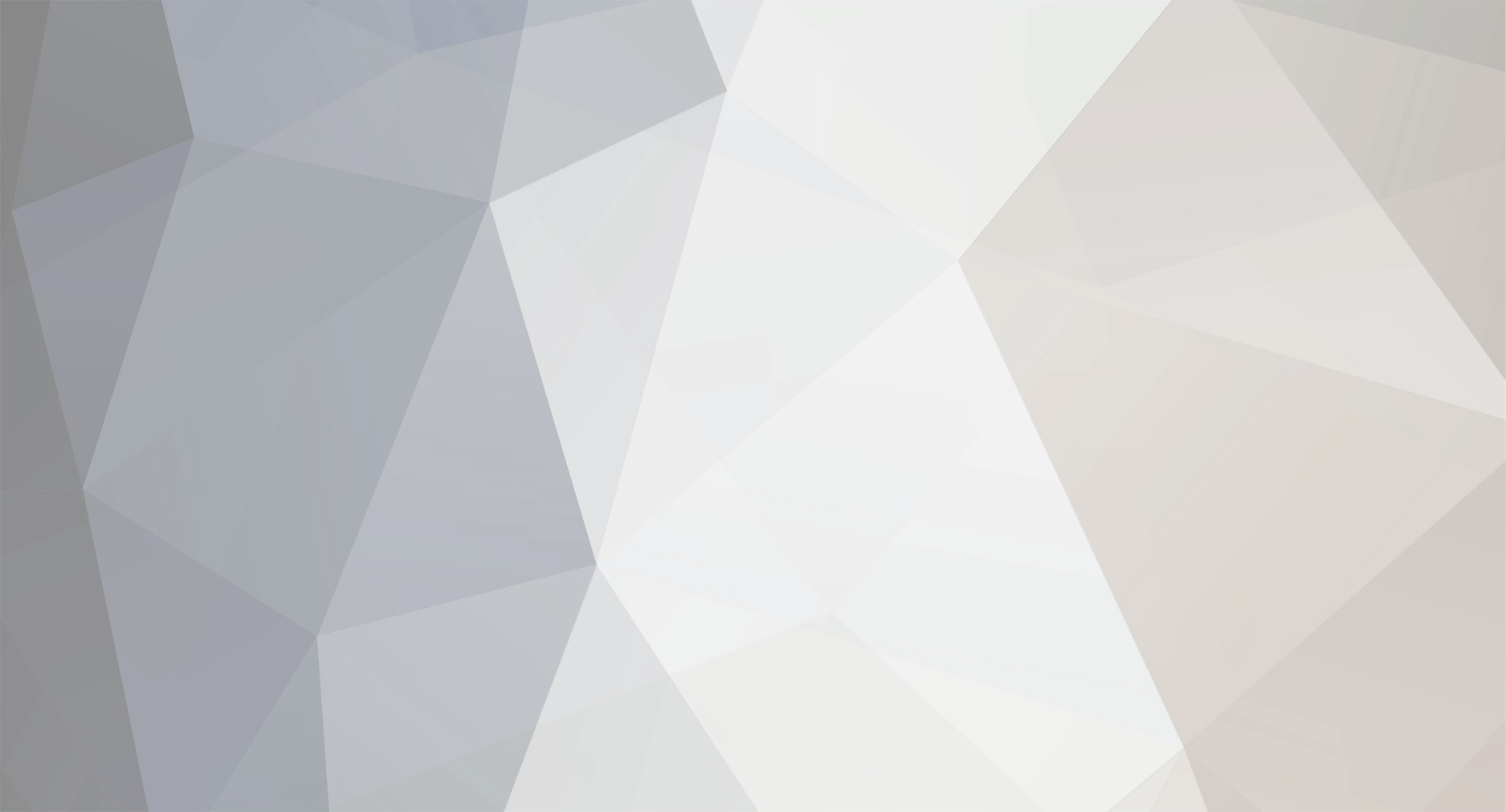
TheDav1311
Members-
Posts
73 -
Joined
-
Last visited
Everything posted by TheDav1311
-
I'm done so far, but 2 Problems: 1. Some Texture are wrong like gray grass and the different wood has always the oak texture 2. After a reload the textures are away public class VariableBlock extends BlockContainer implements ITileEntityProvider { public boolean onBlockActivated(World world, int x, int y, int z, EntityPlayer player, int meta, float f1, float f2, float f3) { ItemStack stack = player.getCurrentEquippedItem(); TileEntityVariableBlock te = (TileEntityVariableBlock) world.getTileEntity(x, y, z); if(stack != null && Block.getBlockFromItem(stack.getItem()) != null) { te.setIconStack(stack); return true; } return false; } @Override public TileEntity createNewTileEntity(World var1, int var2) { return new TileEntityVariableBlock(); } @SideOnly(Side.CLIENT) public IIcon getIcon(IBlockAccess ba, int x, int y, int z, int side) { TileEntityVariableBlock te = (TileEntityVariableBlock) ba.getTileEntity(x, y, z); return (te != null && te.getIcon(side) != null) ? te.getIcon(side) : this.blockIcon; } public class TileEntityVariableBlock extends TileEntity { private ItemStack iconStack; public void setIconStack(ItemStack stack) { iconStack = stack; } public IIcon getIcon(int side) { return iconStack != null ? Block.getBlockFromItem(iconStack.getItem()).getIcon(side, 0) : null; } @Override public void readFromNBT(NBTTagCompound nbt) { super.readFromNBT(nbt); iconStack = ItemStack.loadItemStackFromNBT(nbt); } @Override public void writeToNBT(NBTTagCompound nbt) { super.writeToNBT(nbt); iconStack.writeToNBT(nbt); } } public static Block VariableBlock= new VariableBlock().setBlockName("VariableBlock"); @EventHandler private void FMLInit(FMLInitializationEvent event) { GameRegistry.registerBlock(VariableBlock, "VariableBlock"); GameRegistry.registerTileEntity(TileEntityVariableBlock.class, "TileEntityVariableBlock"); }
-
With a TileEntity I need them to save in the NBT-Tag right? But how i should save it in there? And how I should get the Texture from there in the getIcon Method?
-
With metadata i can only have 4 (?) different textures in one world. And one mistake by myself: I mean: You can click with a cobble on one of this blocks, at another with a sandstone , ... And then they look like the block. 3. Problem after reload they have the first texture
-
I want that u can change the texture of the block by doing right-click with another Block. public class VariableBlock extends BlockContainer implements ITileEntityProvider { @SideOnly(Side.CLIENT) private IIcon topIcon; public VariableBlock() { super(Material.ground); this.setCreativeTab(CreativeTabs.tabBlock); } @Override public boolean isOpaqueCube() { return false; } public boolean onBlockActivated(World world, int x, int y, int z, EntityPlayer player, int meta, float f1, float f2, float f3) { ItemStack stack = player.getCurrentEquippedItem(); if(stack != null && Block.getBlockFromItem(stack.getItem()) != null) { Block block = Block.getBlockFromItem(stack.getItem()); topIcon = block.getIcon(1, 0); blockIcon = block.getIcon(3, 0); return true; } return false; } @Override public TileEntity createNewTileEntity(World var1, int var2) { return new TileEntityVariableBlock(); } @SideOnly(Side.CLIENT) public IIcon getIcon(int side, int meta) { return side == 1 ? this.topIcon : (side == 0 ? this.topIcon : this.blockIcon); } @SideOnly(Side.CLIENT) public void registerBlockIcons(IIconRegister ir) { this.blockIcon = ir.registerIcon("furnace_side"); this.topIcon = ir.registerIcon("furnace_top"); } } With this code i can change the texture, but all change the texutre, not only one. Second problem: The grass is gray.
-
What you mean with this?
-
Forgot this: @SubscribeEvent public void startTimer(WorldTickEvent event) { if(event.world.isRemote) return; if(event.side == Side.SERVER) { World world = event.world; if(RoundHandler.Preparing) { RoundHandler.processCountdown(); if(RoundHandler.Countdown == 0) { RoundHandler.Preparing = false; RoundHandler.starteRunde(world); RoundHandler.setCountdown(120 * 8 * 60); } } //more stuff here } }
-
The Start-Command @Override public void processCommand(ICommandSender var1, String[] var2) { World world = var1.getEntityWorld(); if(RoundHandler.getFahne(world) != null) { RoundHandler.tileEntityLeeren(world); TileEntityFahne fahne = ((TileEntityFahne) RoundHandler.getFahne(world)); RoundHandler.setCountdown(120 * 60); for(int m = 0; m < world.playerEntities.size(); m++) { EntityPlayerMP playerMP = (EntityPlayerMP) world.playerEntities.get(m); ChatComponentTranslation dc = new ChatComponentTranslation("Es geht LOS"); playerMP.addChatComponentMessage(dc); } } } public static void starteRunde(World world) { Scoreboard sc = world.getScoreboard(); if(sc.getTeamNames().contains(traitor)) sc.removeTeam(sc.getTeam(traitor)); if(sc.getTeamNames().contains(inno)) sc.removeTeam(sc.getTeam(inno)); if(sc.getTeamNames().contains(detec)) sc.removeTeam(sc.getTeam(detec)); if(sc.getTeamNames().contains(tot)) sc.removeTeam(sc.getTeam(tot)); innoList.clear(); traitorList.clear(); detecList.clear(); sc.createTeam(traitor); sc.createTeam(inno); sc.createTeam(detec); sc.createTeam(tot); sc.getTeam(inno).setSeeFriendlyInvisiblesEnabled(false); int traitorZahlfehl = world.playerEntities.size() == 5 ? 2 : world.playerEntities.size()/3; int detectivZahlfehl = world.playerEntities.size() >= 5 ? 1: 0; for(int s = 0; s < world.playerEntities.size(); s++) { EntityPlayerMP playerMP = (EntityPlayerMP) world.playerEntities.get(s); playerMP.setHealth(20); innoList.add(playerMP); } for(int s = 0; s < traitorZahlfehl; s++) { int random = rand.nextInt(innoList.size()-1); traitorList.add(innoList.get(random)); innoList.remove(random); } for(int s = 0; s < detectivZahlfehl; s++) { int random = rand.nextInt(innoList.size()-1); detecList.add(innoList.get(random)); innoList.remove(random); } for(int s = 0; s < traitorList.size(); s++) { EntityPlayerMP player = (EntityPlayerMP) traitorList.get(s); sc.func_151392_a(player.getCommandSenderName(), traitor); } for(int s = 0; s < detecList.size(); s++) { EntityPlayerMP player = (EntityPlayerMP) detecList.get(s); sc.func_151392_a(player.getCommandSenderName(), detec); } for(int s = 0; s < innoList.size(); s++) { EntityPlayerMP player = (EntityPlayerMP) innoList.get(s); sc.func_151392_a(player.getCommandSenderName(), inno); } } } @SubscribeEvent public void spielerTot(LivingDeathEvent event) { Entity entityP = event.entity; double x = event.entity.posX; double y = event.entity.posY; double z = event.entity.posZ; World world = event.entity.worldObj; if(!world.isRemote && entityP instanceof EntityPlayer && Roundaktiv && !Preparing && ((EntityPlayer) entityP).getTeam() != null) { EntityPlayer player = (EntityPlayer) event.entity; if(!((EntityPlayer) player).getTeam().isSameTeam(world.getScoreboard().getTeam(traitor))) spielerTotTimeAdd(); EntityLeiche entity = new EntityLeiche(world, x, y, z); entity.setPlayer(((EntityPlayer) player).getCommandSenderName()); entity.setTeam(((EntityPlayer) player).getTeam().getRegisteredName()); world.spawnEntityInWorld(entity); world.getScoreboard().removePlayerFromTeam(player.getCommandSenderName(), (ScorePlayerTeam) player.getTeam()); player.setGameType(GameType.CREATIVE); } }
-
I use this to add them to a Team. And I use it since a few weeks, first time that it makes problems.
-
But the thing is: I never do something like this in my whole code.
-
I don't know why only this works. I tried just all and this was the only one which works.
-
That's wrong. It only mean the server side. If you play in singleplayer, you have too a server and a client.
-
Try this one: player.setPositionAndUpdate(x, y, z);
-
---- Minecraft Crash Report ---- // I bet Cylons wouldn't have this problem. Time: 01.09.14 18:37 Description: Unexpected error java.lang.IllegalStateException: Player is either on another team or not on any team. Cannot remove from team 'Innocent'. at net.minecraft.scoreboard.Scoreboard.func_96512_b(SourceFile:206) at net.minecraft.client.network.NetHandlerPlayClient.func_147247_a(NetHandlerPlayClient.java:1556) at net.minecraft.network.play.server.S3EPacketTeams.func_148833_a(SourceFile:99) at net.minecraft.network.play.server.S3EPacketTeams.func_148833_a(SourceFile:13) at net.minecraft.network.NetworkManager.func_74428_b(NetworkManager.java:197) at net.minecraft.client.multiplayer.PlayerControllerMP.func_78765_e(PlayerControllerMP.java:273) at net.minecraft.client.Minecraft.func_71407_l(Minecraft.java:1541) at net.minecraft.client.Minecraft.func_71411_J(Minecraft.java:916) at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:835) at net.minecraft.client.main.Main.main(SourceFile:103) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Stacktrace: at net.minecraft.scoreboard.Scoreboard.func_96512_b(SourceFile:206) at net.minecraft.client.network.NetHandlerPlayClient.func_147247_a(NetHandlerPlayClient.java:1556) at net.minecraft.network.play.server.S3EPacketTeams.func_148833_a(SourceFile:99) at net.minecraft.network.play.server.S3EPacketTeams.func_148833_a(SourceFile:13) at net.minecraft.network.NetworkManager.func_74428_b(NetworkManager.java:197) at net.minecraft.client.multiplayer.PlayerControllerMP.func_78765_e(PlayerControllerMP.java:273) -- Affected level -- Details: Level name: MpServer All players: 5 total; [EntityClientPlayerMP['TheDav'/188, l='MpServer', x=-402,62, y=70,73, z=156,69], EntityOtherPlayerMP['Luca'/187, l='MpServer', x=-397,97, y=69,00, z=165,75], EntityOtherPlayerMP['AlessB'/228, l='MpServer', x=-396,97, y=69,00, z=165,06], EntityOtherPlayerMP['Luca'/187, l='MpServer', x=-399,72, y=68,50, z=165,38], EntityOtherPlayerMP['AlessB'/228, l='MpServer', x=-402,13, y=68,00, z=165,91]] Chunk stats: MultiplayerChunkCache: 222, 222 Level seed: 0 Level generator: ID 00 - default, ver 1. Features enabled: false Level generator options: Level spawn location: World: (-284,64,194), Chunk: (at 4,4,2 in -18,12; contains blocks -288,0,192 to -273,255,207), Region: (-1,0; contains chunks -32,0 to -1,31, blocks -512,0,0 to -1,255,511) Level time: 2086732 game time, 1146 day time Level dimension: 0 Level storage version: 0x00000 - Unknown? Level weather: Rain time: 0 (now: false), thunder time: 0 (now: false) Level game mode: Game mode: creative (ID 1). Hardcore: false. Cheats: false Forced entities: 10 total; [EntityClientPlayerMP['TheDav'/188, l='MpServer', x=-400,11, y=70,12, z=165,75], EntityOtherPlayerMP['Luca'/187, l='MpServer', x=-397,97, y=69,00, z=165,75], EntityMinecartChest['entity.MinecartChest.name'/8, l='MpServer', x=-462,50, y=7,50, z=168,50], EntityHorse['Paul Hesse'/40, l='MpServer', x=-370,66, y=63,00, z=156,34], EntityClientPlayerMP['TheDav'/188, l='MpServer', x=-402,62, y=70,73, z=156,69], EntityLeiche['Leiche'/267, l='MpServer', x=-401,91, y=68,00, z=165,91], EntityPig['Kevin'/24, l='MpServer', x=-404,25, y=74,00, z=126,47], EntityOtherPlayerMP['Luca'/187, l='MpServer', x=-399,72, y=68,50, z=165,38], EntityOtherPlayerMP['AlessB'/228, l='MpServer', x=-402,13, y=68,00, z=165,91], EntityOtherPlayerMP['AlessB'/228, l='MpServer', x=-396,97, y=69,00, z=165,06]] Retry entities: 0 total; [] Server brand: mcpc,craftbukkit,fml,forge Server type: Non-integrated multiplayer server Stacktrace: at net.minecraft.client.multiplayer.WorldClient.func_72914_a(WorldClient.java:368) at net.minecraft.client.Minecraft.func_71396_d(Minecraft.java:2382) at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:864) at net.minecraft.client.main.Main.main(SourceFile:103) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) -- System Details -- Details: Minecraft Version: 1.7.2 Operating System: Windows 7 (amd64) version 6.1 Java Version: 1.7.0_51, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 100379168 bytes (95 MB) / 451936256 bytes (431 MB) up to 954728448 bytes (910 MB) JVM Flags: 2 total; -XX:HeapDumpPath=MojangTricksIntelDriversForPerformance_javaw.exe_minecraft.exe.heapdump -Xmx1G AABB Pool Size: 4053 (226968 bytes; 0 MB) allocated, 2 (112 bytes; 0 MB) used IntCache: cache: 0, tcache: 0, allocated: 13, tallocated: 95 FML: MCP v9.03 FML v7.2.193.1078 Minecraft Forge 10.12.1.1078 4 mods loaded, 4 mods active mcp{9.03} [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available FML{7.2.193.1078} [Forge Mod Loader] (forge-1.7.2-10.12.1.1078.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available Forge{10.12.1.1078} [Minecraft Forge] (forge-1.7.2-10.12.1.1078.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available minecrafttrouble{1.6.2} [Trouble in Steve's Town] (modid-1.0.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available Launched Version: 1.7.2-Forge10.12.1.1078 LWJGL: 2.9.0 OpenGL: AMD Radeon(TM) HD 6520G GL version 4.1.11156 Compatibility Profile Context, ATI Technologies Inc. Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: [] Current Language: Deutsch (Deutschland) Profiler Position: N/A (disabled) Vec3 Pool Size: 3078 (172368 bytes; 0 MB) allocated, 22 (1232 bytes; 0 MB) used Anisotropic Filtering: Off (1) The person which does nothing by the kill get this Crash-Report Code: @SubscribeEvent public void spielerTot(LivingDeathEvent event) { Entity entityP = event.entity; World world = event.entity.worldObj; if(entityP instanceof EntityPlayer && Roundaktiv && !Preparing && ((EntityPlayer) entityP).getTeam() != null) { EntityPlayer player = (EntityPlayer) event.entity; world.getScoreboard().func_151392_a(player.getCommandSenderName(), tot); player.setGameType(GameType.CREATIVE); }
-
Oh, I not saw this with all x. But the event not start. I never got the System.out .println();
-
Why does this not work? @SubscribeEvent public void fernbedienung(PlayerInteractEvent event) { if(event.action == event.action.RIGHT_CLICK_BLOCK && event.entityPlayer.getCurrentEquippedItem() != null && event.entityPlayer.getCurrentEquippedItem().getItem() == main.Fernbedienung) { System.out.println("asd"); EntityPlayer player = event.entityPlayer; ItemStack stack = event.entityPlayer.getCurrentEquippedItem(); if(player.isSneaking()) { if(!stack.hasTagCompound()) stack.setTagCompound(new NBTTagCompound()); stack.stackTagCompound.setInteger("x", event.x); stack.stackTagCompound.setInteger("y", event.x); stack.stackTagCompound.setInteger("z", event.x); stack.stackTagCompound.setBoolean("rdy", true); stack.damageItem(5, player); } } } @EventHandler private void FMLPreInit(FMLPreInitializationEvent event) { FMLCommonHandler.instance().bus().register(new ItemHandler()); }
-
Is there a way to reduce damage of a player? i tried something like this: @SubscribeEvent public void damageEvent(AttackEntityEvent event) { if(event.target instanceof EntityPlayer) { if(somethinghere()) { //damage reduction } } }
-
I try now something like this: @SubscribeEvent public void useWeapon(RenderPlayerEvent.Post e) { if(e.entityPlayer.getHeldItem() != null && e.entityPlayer.getHeldItem().getItem() == myWeapon) { ItemStack stack= e.entityPlayer.getHeldItem(); ModelBiped model = e.renderer.modelBipedMain; int count = stack.stackTagCompound.getInteger("count"); model.bipedRightArm.rotateAngleY = 0.0F; model.bipedRightArm.rotationPointZ = MathHelper.sin(model.bipedBody.rotateAngleY) * 5.0F; model.bipedRightArm.rotationPointX = -MathHelper.cos(model.bipedBody.rotateAngleY) * 5.0F; model.bipedBody.rotateAngleX = (float) count; } } In the weapon class @Override public void onUsingTick(ItemStack stack, EntityPlayer player, int count) { if(!stack.hasTagCompound()) stack.setTagCompound(new NBTTagCompound()); stack.stackTagCompound.setInteger("count", count); } But the arm never rotate
-
Is there a way to rotate something of the playermodel like the arm? Example: I have a custom crossbow, but in the 3. personview it looks ugly because he hold it like everything else.
-
I tried a few new thing but nothing helped? Someone an idea?
-
My Game crashed last time random with this Error and I can't fix it. The new class since it crash: public class EntityCorpus extends EntityAmbientCreature { public String player; public String team; public EntityCorpus(World par1World) { super(par1World); player = "Steve"; //Just fillers team = "NoTeam"; } public boolean interact(EntityPlayer entityplayer) { if(entityplayer.capabilities.isCreativeMode) { return false; } if(!entityplayer.worldObj.isRemote) { for(int m = 0; m < entityplayer.worldObj.playerEntities.size(); m++) { ChatComponentTranslation cc = new ChatComponentTranslation(team); EntityPlayerMP playerMP = (EntityPlayerMP) entityplayer.worldObj.playerEntities.get(m); ChatComponentTranslation cd = new ChatComponentTranslation(player + " was a "); cd.appendSibling(cc); playerMP.addChatComponentMessage(cd); } } return true; } public EntityCorpus(World par1World, double x ,double y, double z) { super(par1World); player = "Steve"; //Just fillers team = "NoTeam"; this.setPosition(x, y, z); } protected void applyEntityAttributes() { super.applyEntityAttributes(); this.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(100.0D); } @Override public void readEntityFromNBT(NBTTagCompound par1NBTTagCompound) { player = par1NBTTagCompound.getString("player"); team = par1NBTTagCompound.getString("team"); super.readFromNBT(par1NBTTagCompound); } @Override public void writeEntityToNBT(NBTTagCompound par1NBTTagCompound) { if(player != null) par1NBTTagCompound.setString("player", player); if(team != null) par1NBTTagCompound.setString("team", team); super.writeToNBT(par1NBTTagCompound); } public void setPlayer(String player) { this.player = player; } public void setTeam(String team) { this.team = team; } }
-
@SubscribeEvent public void renderTeamBar(RenderGameOverlayEvent event) { if(event.isCancelable() || event.type != ElementType.EXPERIENCE) { return; } String team = this.mc.thePlayer.getTeam().getRegisteredName(); if(RoundHandler.isRoundaktiv) //that is a static boolean { FontRenderer fontrenderer = mc.fontRenderer; mc.getTextureManager().bindTexture(new ResourceLocation("minecrafttrouble:textures/gui/teambar.png")); GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F) GL11.glEnable(GL11.GL_BLEND); OpenGlHelper.glBlendFunc(770, 771, 1, 0); GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA); this.drawTexturedModalRect(this.xPosition, this.yPosition, 0, 0, 100, 20); this.drawCenteredString(fontrenderer, team, this.xPosition + 100 / 2, this.yPosition + (20 - / 2, 14737632); } } The Problem is: In a Lan-World only the Host see the Gui, noone else. How I can fix that?
-
I have figured out by myself this code was the Button code from MC just a little bit changed from me. And this two textured reacts were my problem. And my Solution was this: public class TradingButton extends GuiButton { public ResourceLocation texture; public TradingButton(int id, int x, int y, ResourceLocation texture) { super(id, x, y, 20, 20, ""); this.texture = texture; this.width = 20; this.height = 20; } public void drawButton(Minecraft mc, int x, int y) { if (this.visible) { FontRenderer fontrenderer = mc.fontRenderer; mc.getTextureManager().bindTexture(texture); GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); this.field_146123_n = x >= this.xPosition && y >= this.yPosition && x < this.xPosition + this.width && y < this.yPosition + this.height; int k = this.getHoverState(this.field_146123_n); GL11.glEnable(GL11.GL_BLEND); OpenGlHelper.glBlendFunc(770, 771, 1, 0); GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA); this.drawTexturedModalRect(this.xPosition, this.yPosition, 0, k * 20, this.width + 1, this.height); this.mouseDragged(mc, x, y); } } } It should be like a normal Button. What it is now. ----> Meaning: In a Gui as a normal Button The texture is the normal MC-Button texture only now with pictures what i like. And the button was only the half. Next time i will write more that everyone should understand
-
I have done this so far but the image never load right. public class TradingButton extends GuiButton { public ResourceLocation texture; public TradingButton(int id, int x, int y, int width, int height, ResourceLocation texture) { super(id, x, y, width, height, ""); this.texture = texture; } public void drawButton(Minecraft p_146112_1_, int p_146112_2_, int p_146112_3_) { if (this.visible) { FontRenderer fontrenderer = p_146112_1_.fontRenderer; p_146112_1_.getTextureManager().bindTexture(texture); GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); this.field_146123_n = p_146112_2_ >= this.xPosition && p_146112_3_ >= this.yPosition && p_146112_2_ < this.xPosition + this.width && p_146112_3_ < this.yPosition + this.height; int k = this.getHoverState(this.field_146123_n); GL11.glEnable(GL11.GL_BLEND); OpenGlHelper.glBlendFunc(770, 771, 1, 0); GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA); this.drawTexturedModalRect(this.xPosition, this.yPosition, 0, 46 + k * 20, this.width / 2, this.height); this.drawTexturedModalRect(this.xPosition + this.width / 2, this.yPosition, 200 - this.width / 2, 46 + k * 20, this.width / 2, this.height); this.mouseDragged(p_146112_1_, p_146112_2_, p_146112_3_); } } }
-
Write this in your class, that's the simple way public void setMeta(World world, int x, int y, int z, int meta) { world.setBlockMetadataWithNotify(x, y, z, MathHelper.clamp_int(meta, 0, 3), 2); world.func_147453_f(x, y, z, this); }
-
My projectiles are invisible, how I can render them right? The tutorial is on 1.6.4 and not work anylonger The Render class public class BolzenRender extends Render { @Override public void doRender(Entity entity, double par2, double par4, double par6,float par8, float par9) { } Main class public main() { [...] RenderingRegistry.registerEntityRenderingHandler(EntityBolzen.class, new BolzenRender()); } The EntityBolzen class is more or less the same like the arrow class