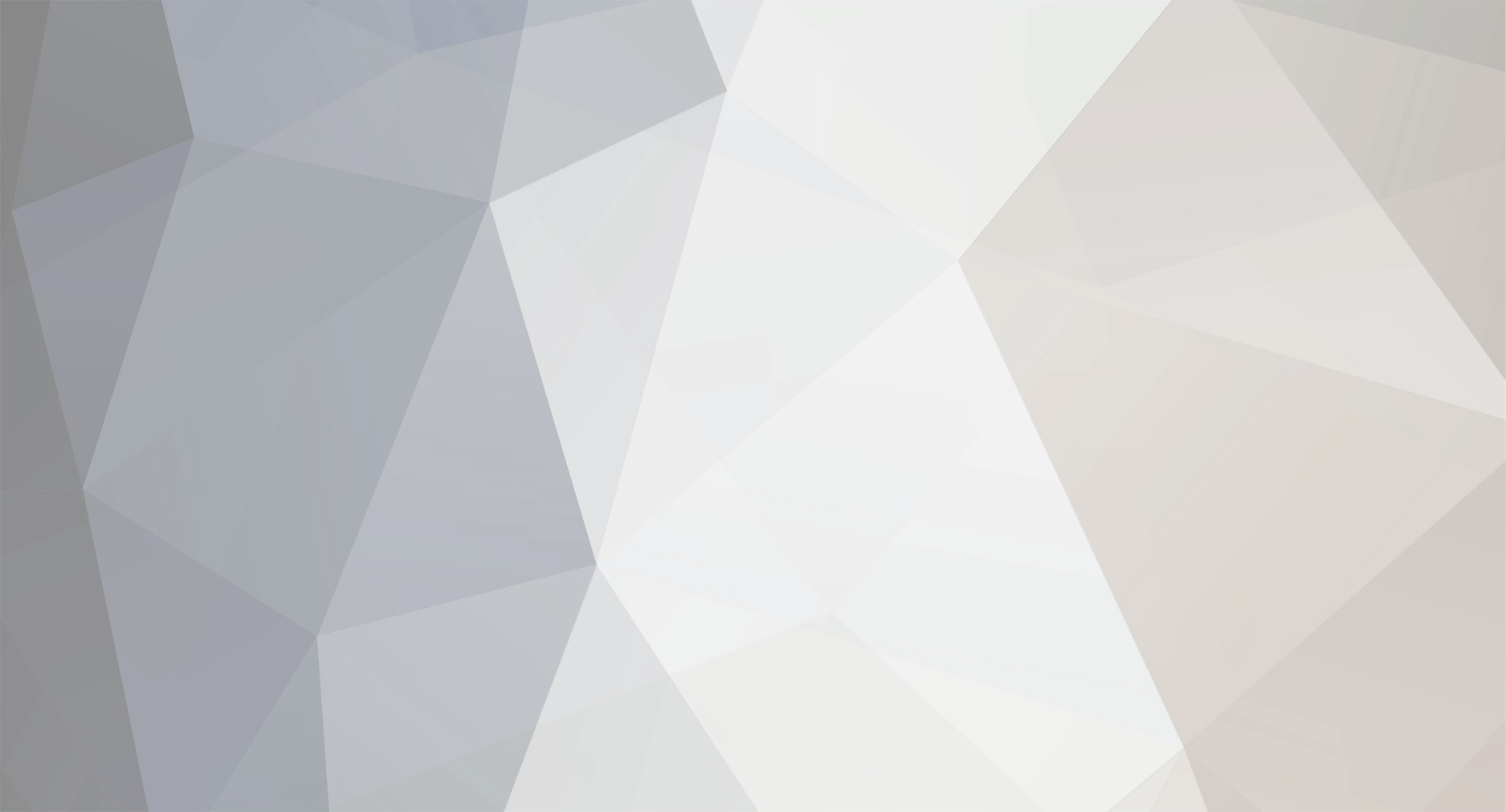
robertcars
Members-
Posts
38 -
Joined
-
Last visited
Everything posted by robertcars
-
Well I guess I will have to rethink it because it would happen when a player wanted it to so if hey where on a server they could just spam it. To make my own data structure seems a little out of my reach. Thanks for the advice
-
So I need my tile entity to have a method on finding all the tile entity's in the world of the same type. Also my tile entity has a id variable in it and the tile entities it finds has to have the same id as the one that's looking for the others and then I need the first tile entity to get the coords of the one it found. How would I go about doing this and what is the best way?
-
[1.7.10][Solved] Packet not called or not sending correctly?
robertcars replied to robertcars's topic in Modder Support
I actually did use the initialize and post initialize for the pipeline however i didn't register the packet im going to try that now. I know about the old code thing but I just couldn't get the simple network wrapper to work so I figured I would get it working the old way then later down the road change to the simple network wrapper. Thanks for the help. -
I am trying to set a integer in my tile entity from my GUI so I tried to make a packet system. As far as I can tell my packet isn't being called. GUI package com.robert.instaport.gui; import java.io.ByteArrayOutputStream; import java.io.DataOutputStream; import net.minecraft.client.gui.GuiButton; import net.minecraft.client.gui.GuiTextField; import net.minecraft.client.gui.inventory.GuiContainer; import net.minecraft.entity.player.InventoryPlayer; import net.minecraft.util.ResourceLocation; import org.lwjgl.opengl.GL11; import com.robert.instaport.Instaport; import com.robert.instaport.common.container.ContainerTeleporter; import com.robert.instaport.common.tileentity.TileEntityTeleporter; import com.robert.instaport.lib.Reference; import com.robert.instaport.packet.TeleporterPacket; public class GuiTeleporter extends GuiContainer { public final int xSizeOfTexture = 176; public final int ySizeOfTexture = 228; private GuiTextField idField; public int id; @SuppressWarnings("unused") private TileEntityTeleporter tileEntityKeyPad; private TileEntityTeleporter tileEntityTeleporter = new TileEntityTeleporter(); public GuiTeleporter (InventoryPlayer inventoryPlayer, TileEntityTeleporter tileEntity) { //the container is instanciated and passed to the superclass for handling super(new ContainerTeleporter(inventoryPlayer, tileEntity)); tileEntityTeleporter = tileEntity; this.xSize = xSizeOfTexture; this.ySize = ySizeOfTexture; TileEntityTeleporter tile = (TileEntityTeleporter) tileEntityTeleporter.getWorldObj().getTileEntity(tileEntityTeleporter.xCoord, tileEntityTeleporter.yCoord, tileEntityTeleporter.zCoord); id = tile.getId(); } @Override protected void drawGuiContainerForegroundLayer(int param1, int param2) { String title = "Teleporter"; fontRendererObj.drawString(title, 7, ySize - 220, 4210752); fontRendererObj.drawString("Security Card:", 7, ySize - 118, 4210752); } @SuppressWarnings("unchecked") @Override public void initGui() { super.initGui(); //make buttons buttonList.add(new GuiButton(1, width / 2 - 20 / 2 - 60, this.height / 2 - 65, 20, 20, "+")); buttonList.add(new GuiButton(2, width / 2 - 20 / 2 + 60, this.height / 2 - 65, 20, 20, "-")); buttonList.add(new GuiButton(3, width / 2 - 100 / 2, this.height / 2 - 35, 100, 20, "Save")); idField = new GuiTextField(fontRendererObj, this.width / 2 - 70 / 2, this.height / 2 - 65, 70, 20); idField.setFocused(false); idField.setMaxStringLength(10); } public void updateScreen() { idField.setText(Integer.toString(id)); } public void drawScreen(int i, int j, float f) { super.drawScreen(i, j, f); idField.drawTextBox(); } ByteArrayOutputStream bos = new ByteArrayOutputStream(; DataOutputStream outputStream = new DataOutputStream(bos); protected void actionPerformed(GuiButton guibutton) { switch(guibutton.id) { case 1://add id += 1; break; case 2:// subtract if(!(id <= 0)) id -= 1; break; case 3://save Instaport.packetPipeline.sendToServer(new TeleporterPacket(tileEntityTeleporter.xCoord, tileEntityTeleporter.yCoord, tileEntityTeleporter.zCoord, id)); } //Packet code here //PacketDispatcher.sendPacketToServer(packet); //send packet } ResourceLocation texture = new ResourceLocation(Reference.MOD_ID.toLowerCase() + ":" + "textures/gui/Teleporter.png"); @Override protected void drawGuiContainerBackgroundLayer(float par1, int par2, int par3) { GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); //this.mc.renderEngine.bindTexture(texture); this.mc.getTextureManager().bindTexture(texture); int x = (width - xSizeOfTexture) / 2; int y = (height - ySizeOfTexture) / 2; this.drawTexturedModalRect(x, y, 0, 0, xSizeOfTexture, ySizeOfTexture); } } TileEntity package com.robert.instaport.common.tileentity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.IInventory; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.nbt.NBTTagList; import net.minecraft.network.NetworkManager; import net.minecraft.network.Packet; import net.minecraft.network.play.server.S35PacketUpdateTileEntity; import net.minecraft.tileentity.TileEntity; public class TileEntityTeleporter extends TileEntity implements IInventory { private ItemStack[] inv; public int detectionRange; public static int id; public TileEntityTeleporter(){ inv = new ItemStack[1]; } public void setInvalid() { worldObj.setBlockMetadataWithNotify(xCoord, yCoord, zCoord, 0, 2); } @Override public int getSizeInventory() { return inv.length; } @Override public ItemStack getStackInSlot(int slot) { return inv[slot]; } @Override public Packet getDescriptionPacket() { NBTTagCompound tag = new NBTTagCompound(); this.writeToNBT(tag); return new S35PacketUpdateTileEntity(xCoord, yCoord, zCoord, 1, tag); } @Override public void onDataPacket(NetworkManager net, S35PacketUpdateTileEntity packet) { readFromNBT(packet.func_148857_g()); } @Override public void setInventorySlotContents(int slot, ItemStack stack) { inv[slot] = stack; if (stack != null && stack.stackSize > getInventoryStackLimit()) { stack.stackSize = getInventoryStackLimit(); } } @Override public ItemStack decrStackSize(int slot, int amt) { ItemStack stack = getStackInSlot(slot); if (stack != null) { if (stack.stackSize <= amt) { setInventorySlotContents(slot, null); } else { stack = stack.splitStack(amt); if (stack.stackSize == 0) { setInventorySlotContents(slot, null); } } } return stack; } @Override public ItemStack getStackInSlotOnClosing(int slot) { ItemStack stack = getStackInSlot(slot); if (stack != null) { setInventorySlotContents(slot, null); } return stack; } @Override public int getInventoryStackLimit() { return 1; } @Override public boolean isUseableByPlayer(EntityPlayer player) { return worldObj.getTileEntity(xCoord, yCoord, zCoord) == this && player.getDistanceSq(xCoord + 0.5, yCoord + 0.5, zCoord + 0.5) < 64; } @Override public void readFromNBT(NBTTagCompound tagCompound) { super.readFromNBT(tagCompound); NBTTagList tagList = tagCompound.getTagList("Inventory", 10); for (int i = 0; i < tagList.tagCount(); i++) { NBTTagCompound tag = (NBTTagCompound) tagList.getCompoundTagAt(i); byte slot = tag.getByte("Slot"); if (slot >= 0 && slot < inv.length) { inv[slot] = ItemStack.loadItemStackFromNBT(tag); } } } @Override public void writeToNBT(NBTTagCompound tagCompound) { super.writeToNBT(tagCompound); NBTTagList itemList = new NBTTagList(); for (int i = 0; i < inv.length; i++) { ItemStack stack = inv[i]; if (stack != null) { NBTTagCompound tag = new NBTTagCompound(); tag.setByte("Slot", (byte) i); stack.writeToNBT(tag); itemList.appendTag(tag); } } tagCompound.setTag("Inventory", itemList); } public int getId() { return id; } public void setId(int id) { this.id = id; } @Override public String getInventoryName() { return null; } @Override public boolean hasCustomInventoryName() { return false; } @Override public void openInventory() { } @Override public void closeInventory() { } @Override public boolean isItemValidForSlot(int p_94041_1_, ItemStack p_94041_2_) { return false; } public void setDetectionRange(int _range) { } } PacketPipeline(From NettyPacket Handler tutorial) package com.robert.instaport.packet; import java.util.*; import io.netty.buffer.ByteBuf; import io.netty.buffer.Unpooled; import io.netty.channel.ChannelHandler; import io.netty.channel.ChannelHandlerContext; import io.netty.handler.codec.MessageToMessageCodec; import net.minecraft.client.Minecraft; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.player.EntityPlayerMP; import net.minecraft.network.INetHandler; import net.minecraft.network.NetHandlerPlayServer; import cpw.mods.fml.common.FMLCommonHandler; import cpw.mods.fml.common.network.FMLEmbeddedChannel; import cpw.mods.fml.common.network.FMLOutboundHandler; import cpw.mods.fml.common.network.NetworkRegistry; import cpw.mods.fml.common.network.internal.FMLProxyPacket; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; /** * Packet pipeline class. Directs all registered packet data to be handled by the packets themselves. * @author sirgingalot * some code from: cpw */ @ChannelHandler.Sharable public class PacketPipeline extends MessageToMessageCodec<FMLProxyPacket, AbstractPacket> { private EnumMap<Side, FMLEmbeddedChannel> channels; private LinkedList<Class<? extends AbstractPacket>> packets = new LinkedList<Class<? extends AbstractPacket>>(); private boolean isPostInitialised = false; /** * Register your packet with the pipeline. Discriminators are automatically set. * * @param clazz the class to register * * @return whether registration was successful. Failure may occur if 256 packets have been registered or if the registry already contains this packet */ public boolean registerPacket(Class<? extends AbstractPacket> clazz) { if (this.packets.size() > 256) { // You should log here!! return false; } if (this.packets.contains(clazz)) { // You should log here!! return false; } if (this.isPostInitialised) { // You should log here!! return false; } this.packets.add(clazz); return true; } // In line encoding of the packet, including discriminator setting @Override protected void encode(ChannelHandlerContext ctx, AbstractPacket msg, List<Object> out) throws Exception { ByteBuf buffer = Unpooled.buffer(); Class<? extends AbstractPacket> clazz = msg.getClass(); if (!this.packets.contains(msg.getClass())) { throw new NullPointerException("No Packet Registered for: " + msg.getClass().getCanonicalName()); } byte discriminator = (byte) this.packets.indexOf(clazz); buffer.writeByte(discriminator); msg.encodeInto(ctx, buffer); FMLProxyPacket proxyPacket = new FMLProxyPacket(buffer.copy(), ctx.channel().attr(NetworkRegistry.FML_CHANNEL).get()); out.add(proxyPacket); } // In line decoding and handling of the packet @Override protected void decode(ChannelHandlerContext ctx, FMLProxyPacket msg, List<Object> out) throws Exception { ByteBuf payload = msg.payload(); byte discriminator = payload.readByte(); Class<? extends AbstractPacket> clazz = this.packets.get(discriminator); if (clazz == null) { throw new NullPointerException("No packet registered for discriminator: " + discriminator); } AbstractPacket pkt = clazz.newInstance(); pkt.decodeInto(ctx, payload.slice()); EntityPlayer player; switch (FMLCommonHandler.instance().getEffectiveSide()) { case CLIENT: player = this.getClientPlayer(); pkt.handleClientSide(player); break; case SERVER: INetHandler netHandler = ctx.channel().attr(NetworkRegistry.NET_HANDLER).get(); player = ((NetHandlerPlayServer) netHandler).playerEntity; pkt.handleServerSide(player); break; default: } out.add(pkt); } // Method to call from FMLInitializationEvent public void initialise() { this.channels = NetworkRegistry.INSTANCE.newChannel("INSTAPORT", this); } // Method to call from FMLPostInitializationEvent // Ensures that packet discriminators are common between server and client by using logical sorting public void postInitialise() { if (this.isPostInitialised) { return; } this.isPostInitialised = true; Collections.sort(this.packets, new Comparator<Class<? extends AbstractPacket>>() { @Override public int compare(Class<? extends AbstractPacket> clazz1, Class<? extends AbstractPacket> clazz2) { int com = String.CASE_INSENSITIVE_ORDER.compare(clazz1.getCanonicalName(), clazz2.getCanonicalName()); if (com == 0) { com = clazz1.getCanonicalName().compareTo(clazz2.getCanonicalName()); } return com; } }); } @SideOnly(Side.CLIENT) private EntityPlayer getClientPlayer() { return Minecraft.getMinecraft().thePlayer; } /** * Send this message to everyone. * <p/> * Adapted from CPW's code in cpw.mods.fml.common.network.simpleimpl.SimpleNetworkWrapper * * @param message The message to send */ public void sendToAll(AbstractPacket message) { this.channels.get(Side.SERVER).attr(FMLOutboundHandler.FML_MESSAGETARGET).set(FMLOutboundHandler.OutboundTarget.ALL); this.channels.get(Side.SERVER).writeAndFlush(message); } /** * Send this message to the specified player. * <p/> * Adapted from CPW's code in cpw.mods.fml.common.network.simpleimpl.SimpleNetworkWrapper * * @param message The message to send * @param player The player to send it to */ public void sendTo(AbstractPacket message, EntityPlayerMP player) { this.channels.get(Side.SERVER).attr(FMLOutboundHandler.FML_MESSAGETARGET).set(FMLOutboundHandler.OutboundTarget.PLAYER); this.channels.get(Side.SERVER).attr(FMLOutboundHandler.FML_MESSAGETARGETARGS).set(player); this.channels.get(Side.SERVER).writeAndFlush(message); } /** * Send this message to everyone within a certain range of a point. * <p/> * Adapted from CPW's code in cpw.mods.fml.common.network.simpleimpl.SimpleNetworkWrapper * * @param message The message to send * @param point The {@link cpw.mods.fml.common.network.NetworkRegistry.TargetPoint} around which to send */ public void sendToAllAround(AbstractPacket message, NetworkRegistry.TargetPoint point) { this.channels.get(Side.SERVER).attr(FMLOutboundHandler.FML_MESSAGETARGET).set(FMLOutboundHandler.OutboundTarget.ALLAROUNDPOINT); this.channels.get(Side.SERVER).attr(FMLOutboundHandler.FML_MESSAGETARGETARGS).set(point); this.channels.get(Side.SERVER).writeAndFlush(message); } /** * Send this message to everyone within the supplied dimension. * <p/> * Adapted from CPW's code in cpw.mods.fml.common.network.simpleimpl.SimpleNetworkWrapper * * @param message The message to send * @param dimensionId The dimension id to target */ public void sendToDimension(AbstractPacket message, int dimensionId) { this.channels.get(Side.SERVER).attr(FMLOutboundHandler.FML_MESSAGETARGET).set(FMLOutboundHandler.OutboundTarget.DIMENSION); this.channels.get(Side.SERVER).attr(FMLOutboundHandler.FML_MESSAGETARGETARGS).set(dimensionId); this.channels.get(Side.SERVER).writeAndFlush(message); } /** * Send this message to the server. * <p/> * Adapted from CPW's code in cpw.mods.fml.common.network.simpleimpl.SimpleNetworkWrapper * * @param message The message to send */ public void sendToServer(AbstractPacket message) { this.channels.get(Side.CLIENT).attr(FMLOutboundHandler.FML_MESSAGETARGET).set(FMLOutboundHandler.OutboundTarget.TOSERVER); this.channels.get(Side.CLIENT).writeAndFlush(message); } } Packet package com.robert.instaport.packet; import com.robert.instaport.common.tileentity.TileEntityTeleporter; import io.netty.buffer.ByteBuf; import io.netty.channel.ChannelHandlerContext; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.tileentity.TileEntity; import net.minecraft.world.World; import cpw.mods.fml.common.network.ByteBufUtils; public class TeleporterPacket extends AbstractPacket { private int x, y, z; private int id; public TeleporterPacket() { } public TeleporterPacket(int x, int y, int z, int ids) { this.x = x; this.y = y; this.z = z; this.id = ids; } @Override public void encodeInto (ChannelHandlerContext ctx, ByteBuf buffer) { buffer.writeInt(x); buffer.writeInt(y); buffer.writeInt(z); ByteBufUtils.writeUTF8String(buffer, Integer.toString(id)); } @Override public void decodeInto (ChannelHandlerContext ctx, ByteBuf buffer) { x = buffer.readInt(); y = buffer.readInt(); z = buffer.readInt(); id = Integer.parseInt(ByteBufUtils.readUTF8String(buffer)); } @Override public void handleClientSide (EntityPlayer player) { } @Override public void handleServerSide (EntityPlayer player) { World world = player.worldObj; TileEntity te = world.getTileEntity(x, y, z); if (te instanceof TileEntityTeleporter) { ((TileEntityTeleporter) te).setId(id); System.out.println("Set id"); } if (te instanceof TileEntityTeleporter) { ((TileEntityTeleporter) te).setId(id); System.out.println("Set id"); } } }
-
[1.7.10] Gui setting variable in Tile Entity?
robertcars replied to robertcars's topic in Modder Support
I'm not exactly sure how to receive my packet... -
[1.7.10] Gui setting variable in Tile Entity?
robertcars replied to robertcars's topic in Modder Support
Would I have to add some type of class for the server or would that go in the gui or tile entity? -
So I am trying to have my GUI set a integer in the tile entity. I was told I would have to do it with packets so that's what im trying to do however i'm new to packets. I do not know how to get the packet to the tile entity I am pretty sure I made the packet and am sending the integer from the GUI to it correctly. Some help would be much appreciated. Thanks in advance. The integer i'm trying to set is id. Tileentity package com.robert.instaport.common.tileentity; import com.robert.instaport.Instaport; import com.robert.instaport.packet.TutorialMessage; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.IInventory; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.nbt.NBTTagList; import net.minecraft.network.NetworkManager; import net.minecraft.network.Packet; import net.minecraft.network.play.server.S35PacketUpdateTileEntity; import net.minecraft.tileentity.TileEntity; public class TileEntityTeleporter extends TileEntity implements IInventory { private ItemStack[] inv; public int id; public TileEntityTeleporter(){ inv = new ItemStack[1]; } @Override public int getSizeInventory() { return inv.length; } @Override public ItemStack getStackInSlot(int slot) { return inv[slot]; } @Override public Packet getDescriptionPacket() { NBTTagCompound tagCompound = new NBTTagCompound(); this.writeToNBT(tagCompound); return new S35PacketUpdateTileEntity(this.xCoord, this.yCoord, this.zCoord, 1, tagCompound); } @Override public void onDataPacket(NetworkManager net, S35PacketUpdateTileEntity pkt) { readFromNBT(pkt.func_148857_g()); worldObj.markBlockForUpdate(xCoord, yCoord, zCoord); } @Override public void setInventorySlotContents(int slot, ItemStack stack) { inv[slot] = stack; if (stack != null && stack.stackSize > getInventoryStackLimit()) { stack.stackSize = getInventoryStackLimit(); } } @Override public ItemStack decrStackSize(int slot, int amt) { ItemStack stack = getStackInSlot(slot); if (stack != null) { if (stack.stackSize <= amt) { setInventorySlotContents(slot, null); } else { stack = stack.splitStack(amt); if (stack.stackSize == 0) { setInventorySlotContents(slot, null); } } } return stack; } @Override public ItemStack getStackInSlotOnClosing(int slot) { ItemStack stack = getStackInSlot(slot); if (stack != null) { setInventorySlotContents(slot, null); } return stack; } @Override public int getInventoryStackLimit() { return 1; } @Override public boolean isUseableByPlayer(EntityPlayer player) { return worldObj.getTileEntity(xCoord, yCoord, zCoord) == this && player.getDistanceSq(xCoord + 0.5, yCoord + 0.5, zCoord + 0.5) < 64; } @Override public void readFromNBT(NBTTagCompound tagCompound) { super.readFromNBT(tagCompound); NBTTagList tagList = tagCompound.getTagList("Inventory", 10); for (int i = 0; i < tagList.tagCount(); i++) { NBTTagCompound tag = (NBTTagCompound) tagList.getCompoundTagAt(i); byte slot = tag.getByte("Slot"); if (slot >= 0 && slot < inv.length) { inv[slot] = ItemStack.loadItemStackFromNBT(tag); } } setId(tagCompound.getInteger("ids")); } TutorialMessage mes; @Override public void writeToNBT(NBTTagCompound tagCompound) { super.writeToNBT(tagCompound); NBTTagList itemList = new NBTTagList(); for (int i = 0; i < inv.length; i++) { ItemStack stack = inv[i]; if (stack != null) { NBTTagCompound tag = new NBTTagCompound(); tag.setByte("Slot", (byte) i); stack.writeToNBT(tag); itemList.appendTag(tag); } } tagCompound.setTag("Inventory", itemList); tagCompound.setInteger("ids", getId()); System.out.println("ID: " + Instaport.snw.getPacketFrom(new TutorialMessage())); } public int getId() { return id; } public void setId(int id) { this.id = id; } @Override public String getInventoryName() { return null; } @Override public boolean hasCustomInventoryName() { return false; } @Override public void openInventory() { } @Override public void closeInventory() { } @Override public boolean isItemValidForSlot(int p_94041_1_, ItemStack p_94041_2_) { return false; } } Gui package com.robert.instaport.gui; import io.netty.buffer.ByteBuf; import java.io.ByteArrayOutputStream; import java.io.DataOutputStream; import java.io.IOException; import net.minecraft.client.gui.GuiButton; import net.minecraft.client.gui.GuiTextField; import net.minecraft.client.gui.inventory.GuiContainer; import net.minecraft.entity.player.InventoryPlayer; import net.minecraft.network.play.server.S35PacketUpdateTileEntity; import net.minecraft.util.ResourceLocation; import org.lwjgl.opengl.GL11; import com.robert.instaport.Instaport; import com.robert.instaport.common.container.ContainerTeleporter; import com.robert.instaport.common.tileentity.TileEntityTeleporter; import com.robert.instaport.lib.Reference; import com.robert.instaport.packet.TutorialMessage; public class GuiTeleporter extends GuiContainer { public final int xSizeOfTexture = 176; public final int ySizeOfTexture = 228; private GuiTextField idField; public int id; @SuppressWarnings("unused") private TileEntityTeleporter tileEntityKeyPad; private TileEntityTeleporter tileEntityTeleporter = new TileEntityTeleporter(); public GuiTeleporter (InventoryPlayer inventoryPlayer, TileEntityTeleporter tileEntity) { //the container is instanciated and passed to the superclass for handling super(new ContainerTeleporter(inventoryPlayer, tileEntity)); tileEntityTeleporter = tileEntity; this.xSize = xSizeOfTexture; this.ySize = ySizeOfTexture; TileEntityTeleporter tile = (TileEntityTeleporter) tileEntityTeleporter.getWorldObj().getTileEntity(tileEntityTeleporter.xCoord, tileEntityTeleporter.yCoord, tileEntityTeleporter.zCoord); id = tile.getId(); } @Override protected void drawGuiContainerForegroundLayer(int param1, int param2) { String title = "Teleporter"; fontRendererObj.drawString(title, 7, ySize - 220, 4210752); fontRendererObj.drawString("Security Card:", 7, ySize - 118, 4210752); } @SuppressWarnings("unchecked") @Override public void initGui() { super.initGui(); //make buttons buttonList.add(new GuiButton(1, width / 2 - 20 / 2 - 60, this.height / 2 - 65, 20, 20, "+")); buttonList.add(new GuiButton(2, width / 2 - 20 / 2 + 60, this.height / 2 - 65, 20, 20, "-")); buttonList.add(new GuiButton(3, width / 2 - 100 / 2, this.height / 2 - 35, 100, 20, "Save")); idField = new GuiTextField(fontRendererObj, this.width / 2 - 70 / 2, this.height / 2 - 65, 70, 20); idField.setFocused(false); idField.setMaxStringLength(10); } public void updateScreen() { idField.setText(Integer.toString(id)); } public void drawScreen(int i, int j, float f) { super.drawScreen(i, j, f); idField.drawTextBox(); } ByteArrayOutputStream bos = new ByteArrayOutputStream(; DataOutputStream outputStream = new DataOutputStream(bos); protected void actionPerformed(GuiButton guibutton) { TileEntityTeleporter tile = (TileEntityTeleporter) tileEntityTeleporter.getWorldObj().getTileEntity(tileEntityTeleporter.xCoord, tileEntityTeleporter.yCoord, tileEntityTeleporter.zCoord); switch(guibutton.id) { case 1://add id += 1; break; case 2:// subtract if(!(id <= 0)) id -= 1; break; case 3://save Instaport.snw.sendToServer(new TutorialMessage(id)); } //Packet code here //PacketDispatcher.sendPacketToServer(packet); //send packet } ResourceLocation texture = new ResourceLocation(Reference.MOD_ID.toLowerCase() + ":" + "textures/gui/Teleporter.png"); @Override protected void drawGuiContainerBackgroundLayer(float par1, int par2, int par3) { GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); //this.mc.renderEngine.bindTexture(texture); this.mc.getTextureManager().bindTexture(texture); int x = (width - xSizeOfTexture) / 2; int y = (height - ySizeOfTexture) / 2; this.drawTexturedModalRect(x, y, 0, 0, xSizeOfTexture, ySizeOfTexture); } } PacketHandler package com.robert.instaport.packet; import cpw.mods.fml.common.network.simpleimpl.IMessage; import cpw.mods.fml.common.network.simpleimpl.IMessageHandler; import cpw.mods.fml.common.network.simpleimpl.MessageContext; public class TutorialMessageHandler implements IMessageHandler<TutorialMessage, IMessage> { @Override public IMessage onMessage(TutorialMessage message, MessageContext ctx) { System.out.println(message.extremelyImportantInteger); return null; } } Packet package com.robert.instaport.packet; import io.netty.buffer.ByteBuf; import cpw.mods.fml.common.network.simpleimpl.IMessage; public class TutorialMessage implements IMessage{ public int extremelyImportantInteger; public TutorialMessage() {} public TutorialMessage(int a) { this.extremelyImportantInteger = a; } @Override public void toBytes(ByteBuf buf) { buf.writeInt(extremelyImportantInteger); } @Override public void fromBytes(ByteBuf buf) { this.extremelyImportantInteger = buf.readInt(); } }
-
Well I think I got the packet tutorial that you sent working but I had to change a couple things. Im also pretty sure im sending my id from the gui to it but how do I get it to the tile entity?
-
Well it was more of syntax errors. Ill put the code im using now below. main package com.robert.instaport; import net.minecraft.creativetab.CreativeTabs; import com.robert.instaport.common.blocks.ModBlocks; import com.robert.instaport.common.crafting.Crafting; import com.robert.instaport.common.creativetabs.InstaportTab; import com.robert.instaport.common.items.ModItems; import com.robert.instaport.gui.GuiHandler; import com.robert.instaport.lib.Reference; import com.robert.instaport.packet.MyMessage; import com.robert.instaport.proxy.CommonProxy; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.EventHandler; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPostInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.network.NetworkRegistry; import cpw.mods.fml.common.network.simpleimpl.SimpleNetworkWrapper; import cpw.mods.fml.relauncher.Side; @Mod(modid = Reference.MOD_ID, name = Reference.MOD_NAME, version = Reference.MOD_VER) public class Instaport { @Instance(value = Reference.MOD_ID) public static Instaport instance; @SidedProxy(clientSide="com.robert.instaport.proxy.ClientProxy", serverSide="com.robert.instaport.proxy.CommonProxy") public static CommonProxy proxy; public static CreativeTabs tabInstaport = new InstaportTab("Instaport"); public static SimpleNetworkWrapper network; @EventHandler public void preInit(FMLPreInitializationEvent event) { ModBlocks.init(); ModItems.init(); Crafting.init(); NetworkRegistry.INSTANCE.registerGuiHandler(this, new GuiHandler()); network = NetworkRegistry.INSTANCE.newSimpleChannel("MyChannel"); network.registerMessage(MyMessage.Handler.class, MyMessage.class, 0, Side.SERVER); } @EventHandler public void load(FMLInitializationEvent event) { proxy.registerRenderers(); } @EventHandler public void postInit(FMLPostInitializationEvent event) { } } packet package com.robert.instaport.packet; import com.robert.instaport.common.tileentity.TileEntityTeleporter; import io.netty.buffer.ByteBuf; import cpw.mods.fml.common.network.ByteBufUtils; import cpw.mods.fml.common.network.simpleimpl.IMessage; import cpw.mods.fml.common.network.simpleimpl.IMessageHandler; import cpw.mods.fml.common.network.simpleimpl.MessageContext; public class MyMessage implements IMessage { private String text; public MyMessage() { } public MyMessage(String text) { this.text = text; } @Override public void fromBytes(ByteBuf buf) { text = ByteBufUtils.readUTF8String(buf); // this class is very useful in general for writing more complex objects } @Override public void toBytes(ByteBuf buf) { ByteBufUtils.writeUTF8String(buf, text); } public static class Handler implements IMessageHandler<MyMessage, IMessage> { @Override public IMessage onMessage(MyMessage message, MessageContext ctx) { System.out.println(String.format("Received %s from %s", message.text, ctx.getServerHandler().playerEntity.getDisplayName())); return null; // no response in this case } } } gui package com.robert.instaport.gui; import java.util.EnumMap; import net.minecraft.client.gui.GuiButton; import net.minecraft.client.gui.GuiTextField; import net.minecraft.client.gui.inventory.GuiContainer; import net.minecraft.entity.player.InventoryPlayer; import net.minecraft.util.ResourceLocation; import org.lwjgl.opengl.GL11; import com.robert.instaport.Instaport; import com.robert.instaport.common.container.ContainerTeleporter; import com.robert.instaport.common.tileentity.TileEntityTeleporter; import com.robert.instaport.lib.Reference; import com.robert.instaport.packet.MyMessage; import cpw.mods.fml.common.network.FMLEmbeddedChannel; import cpw.mods.fml.common.network.NetworkRegistry; import cpw.mods.fml.relauncher.Side; public class GuiTeleporter extends GuiContainer { public final int xSizeOfTexture = 176; public final int ySizeOfTexture = 228; private GuiTextField idField; public int id; @SuppressWarnings("unused") private TileEntityTeleporter tileEntityKeyPad; private TileEntityTeleporter tileEntityTeleporter = new TileEntityTeleporter(); public GuiTeleporter (InventoryPlayer inventoryPlayer, TileEntityTeleporter tileEntity) { //the container is instanciated and passed to the superclass for handling super(new ContainerTeleporter(inventoryPlayer, tileEntity)); tileEntityTeleporter = tileEntity; this.xSize = xSizeOfTexture; this.ySize = ySizeOfTexture; TileEntityTeleporter tile = (TileEntityTeleporter) tileEntityTeleporter.getWorldObj().getTileEntity(tileEntityTeleporter.xCoord, tileEntityTeleporter.yCoord, tileEntityTeleporter.zCoord); id = tile.getId(); } @Override protected void drawGuiContainerForegroundLayer(int param1, int param2) { String title = "Teleporter"; fontRendererObj.drawString(title, 7, ySize - 220, 4210752); fontRendererObj.drawString("Security Card:", 7, ySize - 118, 4210752); } @SuppressWarnings("unchecked") @Override public void initGui() { super.initGui(); //make buttons buttonList.add(new GuiButton(1, width / 2 - 20 / 2 - 60, this.height / 2 - 65, 20, 20, "+")); buttonList.add(new GuiButton(2, width / 2 - 20 / 2 + 60, this.height / 2 - 65, 20, 20, "-")); buttonList.add(new GuiButton(3, width / 2 - 100 / 2, this.height / 2 - 35, 100, 20, "Save")); idField = new GuiTextField(fontRendererObj, this.width / 2 - 70 / 2, this.height / 2 - 65, 70, 20); idField.setFocused(false); idField.setMaxStringLength(10); } public void updateScreen() { idField.setText(Integer.toString(id)); } public void drawScreen(int i, int j, float f) { super.drawScreen(i, j, f); idField.drawTextBox(); } protected void actionPerformed(GuiButton guibutton) { TileEntityTeleporter tile = (TileEntityTeleporter) tileEntityTeleporter.getWorldObj().getTileEntity(tileEntityTeleporter.xCoord, tileEntityTeleporter.yCoord, tileEntityTeleporter.zCoord); switch(guibutton.id) { case 1://add id += 1; break; case 2:// subtract if(!(id <= 0)) id -= 1; break; case 3://save Instaport.network.sendToServer(new MyMessage(Integer.toString(id))); } //Packet code here //PacketDispatcher.sendPacketToServer(packet); //send packet } ResourceLocation texture = new ResourceLocation(Reference.MOD_ID.toLowerCase() + ":" + "textures/gui/Teleporter.png"); @Override protected void drawGuiContainerBackgroundLayer(float par1, int par2, int par3) { GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); //this.mc.renderEngine.bindTexture(texture); this.mc.getTextureManager().bindTexture(texture); int x = (width - xSizeOfTexture) / 2; int y = (height - ySizeOfTexture) / 2; this.drawTexturedModalRect(x, y, 0, 0, xSizeOfTexture, ySizeOfTexture); } }
-
How would I send it to the client I ended up using a slightly different tutorial because the one you gave me kept giving me errors but I never figured out how to set the variable in my tile entity from it. Do i do it from the onMessage method and set it like i did from the gui or do I have to have something in the tileentity setting it?
-
Oh that makes sense thank you for explaing
-
Ok thank you I will try that. But you said it sends to the server will that still work in single player?
-
So I am trying to get an integer from my gui class when someone hits the save button to my tile entity class and save it with nbt however it always equals 0 or nothing. Tile Entity package com.robert.instaport.common.tileentity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.IInventory; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.nbt.NBTTagList; import net.minecraft.tileentity.TileEntity; import com.robert.instaport.gui.GuiTeleporter; public class TileEntityTeleporter extends TileEntity implements IInventory { private ItemStack[] inv; public int id; public TileEntityTeleporter(){ inv = new ItemStack[1]; } @Override public int getSizeInventory() { return inv.length; } @Override public ItemStack getStackInSlot(int slot) { return inv[slot]; } @Override public void setInventorySlotContents(int slot, ItemStack stack) { inv[slot] = stack; if (stack != null && stack.stackSize > getInventoryStackLimit()) { stack.stackSize = getInventoryStackLimit(); } } @Override public ItemStack decrStackSize(int slot, int amt) { ItemStack stack = getStackInSlot(slot); if (stack != null) { if (stack.stackSize <= amt) { setInventorySlotContents(slot, null); } else { stack = stack.splitStack(amt); if (stack.stackSize == 0) { setInventorySlotContents(slot, null); } } } return stack; } @Override public ItemStack getStackInSlotOnClosing(int slot) { ItemStack stack = getStackInSlot(slot); if (stack != null) { setInventorySlotContents(slot, null); } return stack; } @Override public int getInventoryStackLimit() { return 1; } @Override public boolean isUseableByPlayer(EntityPlayer player) { return worldObj.getTileEntity(xCoord, yCoord, zCoord) == this && player.getDistanceSq(xCoord + 0.5, yCoord + 0.5, zCoord + 0.5) < 64; } @Override public void readFromNBT(NBTTagCompound tagCompound) { super.readFromNBT(tagCompound); NBTTagList tagList = tagCompound.getTagList("Inventory", 10); for (int i = 0; i < tagList.tagCount(); i++) { NBTTagCompound tag = (NBTTagCompound) tagList.getCompoundTagAt(i); byte slot = tag.getByte("Slot"); if (slot >= 0 && slot < inv.length) { inv[slot] = ItemStack.loadItemStackFromNBT(tag); } } id = tagCompound.getInteger("ids"); } GuiTeleporter gui; @Override public void writeToNBT(NBTTagCompound tagCompound) { super.writeToNBT(tagCompound); NBTTagList itemList = new NBTTagList(); for (int i = 0; i < inv.length; i++) { ItemStack stack = inv[i]; if (stack != null) { NBTTagCompound tag = new NBTTagCompound(); tag.setByte("Slot", (byte) i); stack.writeToNBT(tag); itemList.appendTag(tag); } } tagCompound.setTag("Inventory", itemList); tagCompound.setInteger("ids", id); System.out.println("ID: " + id); } @Override public String getInventoryName() { return null; } @Override public boolean hasCustomInventoryName() { return false; } @Override public void openInventory() { } @Override public void closeInventory() { } @Override public boolean isItemValidForSlot(int p_94041_1_, ItemStack p_94041_2_) { return false; } } Gui package com.robert.instaport.gui; import net.minecraft.client.gui.GuiButton; import net.minecraft.client.gui.GuiTextField; import net.minecraft.client.gui.inventory.GuiContainer; import net.minecraft.entity.player.InventoryPlayer; import net.minecraft.util.ResourceLocation; import org.lwjgl.opengl.GL11; import com.robert.instaport.common.container.ContainerTeleporter; import com.robert.instaport.common.tileentity.TileEntityTeleporter; import com.robert.instaport.lib.Reference; public class GuiTeleporter extends GuiContainer { public final int xSizeOfTexture = 176; public final int ySizeOfTexture = 228; private GuiTextField idField; public int id; @SuppressWarnings("unused") private TileEntityTeleporter tileEntityKeyPad; private TileEntityTeleporter tileEntityTeleporter = new TileEntityTeleporter(); public GuiTeleporter (InventoryPlayer inventoryPlayer, TileEntityTeleporter tileEntity) { //the container is instanciated and passed to the superclass for handling super(new ContainerTeleporter(inventoryPlayer, tileEntity)); tileEntityTeleporter = tileEntity; this.xSize = xSizeOfTexture; this.ySize = ySizeOfTexture; TileEntityTeleporter tile = (TileEntityTeleporter) tileEntityTeleporter.getWorldObj().getTileEntity(tileEntityTeleporter.xCoord, tileEntityTeleporter.yCoord, tileEntityTeleporter.zCoord); id = new TileEntityTeleporter().id; } @Override protected void drawGuiContainerForegroundLayer(int param1, int param2) { String title = "Teleporter"; fontRendererObj.drawString(title, 7, ySize - 220, 4210752); fontRendererObj.drawString("Security Card:", 7, ySize - 118, 4210752); } @SuppressWarnings("unchecked") @Override public void initGui() { super.initGui(); //make buttons buttonList.add(new GuiButton(1, width / 2 - 20 / 2 - 60, this.height / 2 - 65, 20, 20, "+")); buttonList.add(new GuiButton(2, width / 2 - 20 / 2 + 60, this.height / 2 - 65, 20, 20, "-")); buttonList.add(new GuiButton(3, width / 2 - 100 / 2, this.height / 2 - 35, 100, 20, "Save")); idField = new GuiTextField(fontRendererObj, this.width / 2 - 70 / 2, this.height / 2 - 65, 70, 20); idField.setFocused(false); idField.setMaxStringLength(10); } public void updateScreen() { idField.setText(Integer.toString(id)); } public void drawScreen(int i, int j, float f) { super.drawScreen(i, j, f); idField.drawTextBox(); } protected void actionPerformed(GuiButton guibutton) { TileEntityTeleporter tile = (TileEntityTeleporter) tileEntityTeleporter.getWorldObj().getTileEntity(tileEntityTeleporter.xCoord, tileEntityTeleporter.yCoord, tileEntityTeleporter.zCoord); switch(guibutton.id) { case 1://add id += 1; break; case 2:// subtract if(!(id <= 0)) id -= 1; break; case 3://save new TileEntityTeleporter().id = id; } //Packet code here //PacketDispatcher.sendPacketToServer(packet); //send packet } ResourceLocation texture = new ResourceLocation(Reference.MOD_ID.toLowerCase() + ":" + "textures/gui/Teleporter.png"); @Override protected void drawGuiContainerBackgroundLayer(float par1, int par2, int par3) { GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); //this.mc.renderEngine.bindTexture(texture); this.mc.getTextureManager().bindTexture(texture); int x = (width - xSizeOfTexture) / 2; int y = (height - ySizeOfTexture) / 2; this.drawTexturedModalRect(x, y, 0, 0, xSizeOfTexture, ySizeOfTexture); } }
-
It was my bad I forgot to set the xSize and ySize in the gui class
-
I am making a Gui and I made it taller then a standard one and i have the players inventory in it and I noticed that if you click items in the hot bar it spits them out like you are throwing them from your inventory I think it is because if you through a item there in a standard Gui it drops. If i move my hot bar up it doesn't do that. Here is the code used for my hot bar slots. protected void bindPlayerInventory(InventoryPlayer inventoryPlayer) { for (int i = 0; i < 3; i++) { for (int j = 0; j < 9; j++) { addSlotToContainer(new Slot(inventoryPlayer, j + i * 9 + 9, 8 + j * 18, 114 + i * 18)); } } for (int i = 0; i < 9; i++) { addSlotToContainer(new Slot(inventoryPlayer, i, 8 + i * 18, 172)); } }
-
It looks like I messed up something. I got it working now. Thank you so much for your help.
-
Yes line 64 is the if statement but the extra check didnt work it still crashed. It crashes as soon as the item goes into the hot bar. net.minecraft.util.ReportedException: Ticking entity at net.minecraft.world.World.updateEntities(World.java:2102) ~[World.class:?] at net.minecraft.client.Minecraft.runTick(Minecraft.java:2096) ~[Minecraft.class:?] at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1038) ~[Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:961) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:164) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0_45] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_45] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_45] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0_45] at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) [launchwrapper-1.9.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.9.jar:?] Caused by: java.lang.NullPointerException at com.robert.modulartools.items.ModAxe.onUpdate(ModAxe.java:62) ~[ModAxe.class:?] at net.minecraft.item.ItemStack.updateAnimation(ItemStack.java:478) ~[itemStack.class:?] at net.minecraft.entity.player.InventoryPlayer.decrementAnimations(InventoryPlayer.java:349) ~[inventoryPlayer.class:?] at net.minecraft.entity.player.EntityPlayer.onLivingUpdate(EntityPlayer.java:624) ~[EntityPlayer.class:?] at net.minecraft.client.entity.EntityPlayerSP.onLivingUpdate(EntityPlayerSP.java:299) ~[EntityPlayerSP.class:?] at net.minecraft.entity.EntityLivingBase.onUpdate(EntityLivingBase.java:1826) ~[EntityLivingBase.class:?] at net.minecraft.entity.player.EntityPlayer.onUpdate(EntityPlayer.java:341) ~[EntityPlayer.class:?] at net.minecraft.client.entity.EntityClientPlayerMP.onUpdate(EntityClientPlayerMP.java:100) ~[EntityClientPlayerMP.class:?] at net.minecraft.world.World.updateEntityWithOptionalForce(World.java:2277) ~[World.class:?] at net.minecraft.world.World.updateEntity(World.java:2237) ~[World.class:?] at net.minecraft.world.World.updateEntities(World.java:2087) ~[World.class:?] ... 10 more ---- Minecraft Crash Report ---- // There are four lights! Time: 8/16/14 3:05 PM Description: Ticking entity java.lang.NullPointerException: Ticking entity at com.robert.modulartools.items.ModAxe.onUpdate(ModAxe.java:62) at net.minecraft.item.ItemStack.updateAnimation(ItemStack.java:478) at net.minecraft.entity.player.InventoryPlayer.decrementAnimations(InventoryPlayer.java:349) at net.minecraft.entity.player.EntityPlayer.onLivingUpdate(EntityPlayer.java:624) at net.minecraft.client.entity.EntityPlayerSP.onLivingUpdate(EntityPlayerSP.java:299) at net.minecraft.entity.EntityLivingBase.onUpdate(EntityLivingBase.java:1826) at net.minecraft.entity.player.EntityPlayer.onUpdate(EntityPlayer.java:341) at net.minecraft.client.entity.EntityClientPlayerMP.onUpdate(EntityClientPlayerMP.java:100) at net.minecraft.world.World.updateEntityWithOptionalForce(World.java:2277) at net.minecraft.world.World.updateEntity(World.java:2237) at net.minecraft.world.World.updateEntities(World.java:2087) at net.minecraft.client.Minecraft.runTick(Minecraft.java:2096) at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1038) at net.minecraft.client.Minecraft.run(Minecraft.java:961) at net.minecraft.client.main.Main.main(Main.java:164) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Stacktrace: at com.robert.modulartools.items.ModAxe.onUpdate(ModAxe.java:62) at net.minecraft.item.ItemStack.updateAnimation(ItemStack.java:478) at net.minecraft.entity.player.InventoryPlayer.decrementAnimations(InventoryPlayer.java:349) at net.minecraft.entity.player.EntityPlayer.onLivingUpdate(EntityPlayer.java:624) at net.minecraft.client.entity.EntityPlayerSP.onLivingUpdate(EntityPlayerSP.java:299) at net.minecraft.entity.EntityLivingBase.onUpdate(EntityLivingBase.java:1826) at net.minecraft.entity.player.EntityPlayer.onUpdate(EntityPlayer.java:341) at net.minecraft.client.entity.EntityClientPlayerMP.onUpdate(EntityClientPlayerMP.java:100) at net.minecraft.world.World.updateEntityWithOptionalForce(World.java:2277) at net.minecraft.world.World.updateEntity(World.java:2237) -- Entity being ticked -- Details: Entity Type: null (net.minecraft.client.entity.EntityClientPlayerMP) Entity ID: 224 Entity Name: Player937 Entity's Exact location: -247.50, 63.62, 214.50 Entity's Block location: World: (-248,63,214), Chunk: (at 8,3,6 in -16,13; contains blocks -256,0,208 to -241,255,223), Region: (-1,0; contains chunks -32,0 to -1,31, blocks -512,0,0 to -1,255,511) Entity's Momentum: 0.00, -0.02, 0.00 Stacktrace: at net.minecraft.world.World.updateEntities(World.java:2087) -- Affected level -- Details: Level name: MpServer All players: 1 total; [EntityClientPlayerMP['Player937'/224, l='MpServer', x=-247.50, y=63.62, z=214.50]] Chunk stats: MultiplayerChunkCache: 265, 265 Level seed: 0 Level generator: ID 00 - default, ver 1. Features enabled: false Level generator options: Level spawn location: World: (-256,64,224), Chunk: (at 0,4,0 in -16,14; contains blocks -256,0,224 to -241,255,239), Region: (-1,0; contains chunks -32,0 to -1,31, blocks -512,0,0 to -1,255,511) Level time: 61 game time, 61 day time Level dimension: 0 Level storage version: 0x00000 - Unknown? Level weather: Rain time: 0 (now: false), thunder time: 0 (now: false) Level game mode: Game mode: creative (ID 1). Hardcore: false. Cheats: false Forced entities: 74 total; [EntityFallingBlock['Falling Block'/1363, l='MpServer', x=-333.50, y=26.49, z=322.50], EntityFallingBlock['Falling Block'/1479, l='MpServer', x=-303.50, y=60.38, z=126.50], EntityFallingBlock['Falling Block'/1478, l='MpServer', x=-311.50, y=3.38, z=118.50], EntityFallingBlock['Falling Block'/1477, l='MpServer', x=-308.50, y=4.38, z=121.50], EntityFallingBlock['Falling Block'/1476, l='MpServer', x=-309.50, y=3.38, z=121.50], EntityFallingBlock['Falling Block'/1475, l='MpServer', x=-307.50, y=4.38, z=121.50], EntityFallingBlock['Falling Block'/1474, l='MpServer', x=-311.50, y=65.38, z=100.50], EntityFallingBlock['Falling Block'/1473, l='MpServer', x=-309.50, y=65.38, z=99.50], EntityFallingBlock['Falling Block'/1472, l='MpServer', x=-310.50, y=65.38, z=100.50], EntityFallingBlock['Falling Block'/1484, l='MpServer', x=-304.50, y=60.38, z=126.50], EntityFallingBlock['Falling Block'/1483, l='MpServer', x=-305.50, y=61.38, z=125.50], EntityBat['Bat'/1346, l='MpServer', x=-208.78, y=37.28, z=181.46], EntityFallingBlock['Falling Block'/1482, l='MpServer', x=-305.50, y=61.38, z=124.50], EntityFallingBlock['Falling Block'/1481, l='MpServer', x=-306.50, y=61.38, z=124.50], EntityFallingBlock['Falling Block'/1480, l='MpServer', x=-304.50, y=60.38, z=124.50], EntityBat['Bat'/1345, l='MpServer', x=-201.66, y=37.10, z=178.93], EntitySquid['Squid'/305, l='MpServer', x=-299.69, y=59.13, z=228.92], EntitySquid['Squid'/304, l='MpServer', x=-299.73, y=58.22, z=230.01], EntitySquid['Squid'/307, l='MpServer', x=-292.50, y=57.85, z=235.50], EntitySquid['Squid'/306, l='MpServer', x=-300.54, y=59.38, z=232.28], EntitySquid['Squid'/308, l='MpServer', x=-295.59, y=59.28, z=232.80], EntityBat['Bat'/1264, l='MpServer', x=-265.06, y=23.14, z=243.76], EntityFallingBlock['Falling Block'/1513, l='MpServer', x=-304.50, y=61.50, z=124.50], EntityChicken['Chicken'/63, l='MpServer', x=-307.50, y=63.00, z=161.50], EntityFallingBlock['Falling Block'/1512, l='MpServer', x=-304.50, y=61.50, z=123.50], EntityChicken['Chicken'/62, l='MpServer', x=-310.50, y=63.00, z=161.50], EntityBat['Bat'/897, l='MpServer', x=-210.50, y=54.90, z=240.34], EntityFallingBlock['Falling Block'/1514, l='MpServer', x=-304.50, y=61.50, z=125.50], EntitySquid['Squid'/303, l='MpServer', x=-297.66, y=57.31, z=229.85], EntityChicken['Chicken'/68, l='MpServer', x=-303.50, y=68.00, z=208.50], EntityChicken['Chicken'/69, l='MpServer', x=-302.50, y=68.00, z=209.50], EntityChicken['Chicken'/70, l='MpServer', x=-291.50, y=64.00, z=142.50], EntityChicken['Chicken'/71, l='MpServer', x=-293.50, y=68.00, z=146.50], EntityChicken['Chicken'/64, l='MpServer', x=-306.50, y=63.00, z=158.50], EntityChicken['Chicken'/65, l='MpServer', x=-306.50, y=63.00, z=158.50], EntityChicken['Chicken'/66, l='MpServer', x=-306.50, y=65.00, z=212.50], EntityChicken['Chicken'/67, l='MpServer', x=-305.50, y=67.00, z=209.50], EntityChicken['Chicken'/76, l='MpServer', x=-282.50, y=66.00, z=201.50], EntityChicken['Chicken'/77, l='MpServer', x=-281.50, y=66.00, z=201.50], EntityChicken['Chicken'/72, l='MpServer', x=-293.50, y=63.00, z=145.50], EntityBat['Bat'/893, l='MpServer', x=-211.72, y=55.25, z=243.09], EntityChicken['Chicken'/73, l='MpServer', x=-290.50, y=63.00, z=148.50], EntityChicken['Chicken'/74, l='MpServer', x=-281.79, y=67.00, z=204.16], EntityChicken['Chicken'/75, l='MpServer', x=-282.50, y=66.00, z=203.50], EntityChicken['Chicken'/85, l='MpServer', x=-270.50, y=71.00, z=229.50], EntityChicken['Chicken'/84, l='MpServer', x=-270.50, y=71.00, z=229.50], EntityFallingBlock['Falling Block'/1288, l='MpServer', x=-362.50, y=16.49, z=178.50], EntityFallingBlock['Falling Block'/1289, l='MpServer', x=-362.50, y=17.49, z=179.50], EntityChicken['Chicken'/83, l='MpServer', x=-275.74, y=67.00, z=229.72], EntityChicken['Chicken'/82, l='MpServer', x=-270.50, y=70.00, z=227.50], EntityChicken['Chicken'/93, l='MpServer', x=-241.50, y=63.00, z=164.50], EntityChicken['Chicken'/95, l='MpServer', x=-245.50, y=64.00, z=158.50], EntityChicken['Chicken'/94, l='MpServer', x=-245.50, y=64.00, z=160.50], EntitySheep['Sheep'/102, l='MpServer', x=-208.35, y=72.00, z=231.55], EntitySheep['Sheep'/103, l='MpServer', x=-207.94, y=72.00, z=230.46], EntitySheep['Sheep'/101, l='MpServer', x=-209.44, y=72.00, z=231.50], EntityBat['Bat'/374, l='MpServer', x=-237.21, y=36.29, z=180.39], EntityFallingBlock['Falling Block'/1462, l='MpServer', x=-306.50, y=64.38, z=100.50], EntityChicken['Chicken'/96, l='MpServer', x=-244.50, y=64.00, z=158.50], EntityFallingBlock['Falling Block'/1463, l='MpServer', x=-307.50, y=64.38, z=100.50], EntityFallingBlock['Falling Block'/1464, l='MpServer', x=-307.50, y=64.38, z=101.50], EntityFallingBlock['Falling Block'/1465, l='MpServer', x=-306.50, y=64.38, z=99.50], EntityFallingBlock['Falling Block'/1466, l='MpServer', x=-306.50, y=64.38, z=101.50], EntityClientPlayerMP['Player937'/224, l='MpServer', x=-247.50, y=63.62, z=214.50], EntityFallingBlock['Falling Block'/1467, l='MpServer', x=-308.50, y=65.38, z=100.50], EntityFallingBlock['Falling Block'/1468, l='MpServer', x=-307.50, y=65.38, z=99.50], EntityFallingBlock['Falling Block'/1469, l='MpServer', x=-308.50, y=64.38, z=101.50], EntityFallingBlock['Falling Block'/1470, l='MpServer', x=-308.50, y=65.38, z=99.50], EntitySheep['Sheep'/104, l='MpServer', x=-205.69, y=72.00, z=230.41], EntityFallingBlock['Falling Block'/1471, l='MpServer', x=-309.50, y=65.38, z=100.50], EntitySheep['Sheep'/116, l='MpServer', x=-173.50, y=71.00, z=273.50], EntitySheep['Sheep'/115, l='MpServer', x=-170.50, y=71.00, z=273.97], EntitySheep['Sheep'/114, l='MpServer', x=-171.81, y=72.00, z=271.19], EntitySheep['Sheep'/113, l='MpServer', x=-170.22, y=72.00, z=272.47]] Retry entities: 0 total; [] Server brand: fml,forge Server type: Integrated singleplayer server Stacktrace: at net.minecraft.client.multiplayer.WorldClient.addWorldInfoToCrashReport(WorldClient.java:417) at net.minecraft.client.Minecraft.addGraphicsAndWorldToCrashReport(Minecraft.java:2567) at net.minecraft.client.Minecraft.run(Minecraft.java:983) at net.minecraft.client.main.Main.main(Main.java:164) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28)
-
net.minecraft.util.ReportedException: Ticking entity at net.minecraft.world.World.updateEntities(World.java:2102) ~[World.class:?] at net.minecraft.client.Minecraft.runTick(Minecraft.java:2096) ~[Minecraft.class:?] at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1038) ~[Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:961) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:164) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0_45] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_45] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_45] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0_45] at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) [launchwrapper-1.9.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.9.jar:?] Caused by: java.lang.NullPointerException at com.robert.modulartools.items.ModAxe.onUpdate(ModAxe.java:64) ~[ModAxe.class:?] at net.minecraft.item.ItemStack.updateAnimation(ItemStack.java:478) ~[itemStack.class:?] at net.minecraft.entity.player.InventoryPlayer.decrementAnimations(InventoryPlayer.java:349) ~[inventoryPlayer.class:?] at net.minecraft.entity.player.EntityPlayer.onLivingUpdate(EntityPlayer.java:624) ~[EntityPlayer.class:?] at net.minecraft.client.entity.EntityPlayerSP.onLivingUpdate(EntityPlayerSP.java:299) ~[EntityPlayerSP.class:?] at net.minecraft.entity.EntityLivingBase.onUpdate(EntityLivingBase.java:1826) ~[EntityLivingBase.class:?] at net.minecraft.entity.player.EntityPlayer.onUpdate(EntityPlayer.java:341) ~[EntityPlayer.class:?] at net.minecraft.client.entity.EntityClientPlayerMP.onUpdate(EntityClientPlayerMP.java:100) ~[EntityClientPlayerMP.class:?] at net.minecraft.world.World.updateEntityWithOptionalForce(World.java:2277) ~[World.class:?] at net.minecraft.world.World.updateEntity(World.java:2237) ~[World.class:?] at net.minecraft.world.World.updateEntities(World.java:2087) ~[World.class:?] ... 10 more ---- Minecraft Crash Report ---- // But it works on my machine. Time: 8/16/14 2:56 PM Description: Ticking entity java.lang.NullPointerException: Ticking entity at com.robert.modulartools.items.ModAxe.onUpdate(ModAxe.java:64) at net.minecraft.item.ItemStack.updateAnimation(ItemStack.java:478) at net.minecraft.entity.player.InventoryPlayer.decrementAnimations(InventoryPlayer.java:349) at net.minecraft.entity.player.EntityPlayer.onLivingUpdate(EntityPlayer.java:624) at net.minecraft.client.entity.EntityPlayerSP.onLivingUpdate(EntityPlayerSP.java:299) at net.minecraft.entity.EntityLivingBase.onUpdate(EntityLivingBase.java:1826) at net.minecraft.entity.player.EntityPlayer.onUpdate(EntityPlayer.java:341) at net.minecraft.client.entity.EntityClientPlayerMP.onUpdate(EntityClientPlayerMP.java:100) at net.minecraft.world.World.updateEntityWithOptionalForce(World.java:2277) at net.minecraft.world.World.updateEntity(World.java:2237) at net.minecraft.world.World.updateEntities(World.java:2087) at net.minecraft.client.Minecraft.runTick(Minecraft.java:2096) at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1038) at net.minecraft.client.Minecraft.run(Minecraft.java:961) at net.minecraft.client.main.Main.main(Main.java:164) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Stacktrace: at com.robert.modulartools.items.ModAxe.onUpdate(ModAxe.java:64) at net.minecraft.item.ItemStack.updateAnimation(ItemStack.java:478) at net.minecraft.entity.player.InventoryPlayer.decrementAnimations(InventoryPlayer.java:349) at net.minecraft.entity.player.EntityPlayer.onLivingUpdate(EntityPlayer.java:624) at net.minecraft.client.entity.EntityPlayerSP.onLivingUpdate(EntityPlayerSP.java:299) at net.minecraft.entity.EntityLivingBase.onUpdate(EntityLivingBase.java:1826) at net.minecraft.entity.player.EntityPlayer.onUpdate(EntityPlayer.java:341) at net.minecraft.client.entity.EntityClientPlayerMP.onUpdate(EntityClientPlayerMP.java:100) at net.minecraft.world.World.updateEntityWithOptionalForce(World.java:2277) at net.minecraft.world.World.updateEntity(World.java:2237) -- Entity being ticked -- Details: Entity Type: null (net.minecraft.client.entity.EntityClientPlayerMP) Entity ID: 275 Entity Name: Player250 Entity's Exact location: 238.10, 73.62, 265.17 Entity's Block location: World: (238,73,265), Chunk: (at 14,4,9 in 14,16; contains blocks 224,0,256 to 239,255,271), Region: (0,0; contains chunks 0,0 to 31,31, blocks 0,0,0 to 511,255,511) Entity's Momentum: 0.00, -0.08, 0.00 Stacktrace: at net.minecraft.world.World.updateEntities(World.java:2087) -- Affected level -- Details: Level name: MpServer All players: 1 total; [EntityClientPlayerMP['Player250'/275, l='MpServer', x=238.10, y=73.62, z=265.17]] Chunk stats: MultiplayerChunkCache: 110, 110 Level seed: 0 Level generator: ID 00 - default, ver 1. Features enabled: false Level generator options: Level spawn location: World: (200,64,248), Chunk: (at 8,4,8 in 12,15; contains blocks 192,0,240 to 207,255,255), Region: (0,0; contains chunks 0,0 to 31,31, blocks 0,0,0 to 511,255,511) Level time: 1344 game time, 1344 day time Level dimension: 0 Level storage version: 0x00000 - Unknown? Level weather: Rain time: 0 (now: false), thunder time: 0 (now: false) Level game mode: Game mode: creative (ID 1). Hardcore: false. Cheats: false Forced entities: 52 total; [EntityChicken['Chicken'/143, l='MpServer', x=160.50, y=68.00, z=253.50], EntityChicken['Chicken'/142, l='MpServer', x=161.50, y=69.00, z=249.50], EntityChicken['Chicken'/152, l='MpServer', x=175.46, y=69.00, z=216.54], EntityItem['item.item.seeds'/153, l='MpServer', x=182.22, y=68.13, z=236.31], EntityChicken['Chicken'/154, l='MpServer', x=188.44, y=66.00, z=252.56], EntityChicken['Chicken'/155, l='MpServer', x=176.53, y=65.00, z=255.47], EntityChicken['Chicken'/156, l='MpServer', x=185.53, y=65.00, z=264.41], EntityBat['Bat'/157, l='MpServer', x=204.62, y=33.72, z=219.20], EntityBat['Bat'/158, l='MpServer', x=200.86, y=33.01, z=221.79], EntityChicken['Chicken'/159, l='MpServer', x=200.44, y=70.00, z=319.59], EntityChicken['Chicken'/144, l='MpServer', x=171.41, y=67.00, z=251.66], EntityChicken['Chicken'/149, l='MpServer', x=181.50, y=70.00, z=211.50], EntityChicken['Chicken'/150, l='MpServer', x=181.50, y=70.00, z=213.50], EntityChicken['Chicken'/151, l='MpServer', x=187.47, y=70.00, z=216.47], EntityChicken['Chicken'/170, l='MpServer', x=211.56, y=69.00, z=324.47], EntityChicken['Chicken'/169, l='MpServer', x=209.53, y=69.00, z=323.53], EntityBat['Bat'/168, l='MpServer', x=213.14, y=18.04, z=327.59], EntityChicken['Chicken'/160, l='MpServer', x=194.56, y=69.00, z=321.59], EntityChicken['Chicken'/167, l='MpServer', x=222.44, y=66.00, z=279.47], EntityChicken['Chicken'/166, l='MpServer', x=222.34, y=65.00, z=278.38], EntityChicken['Chicken'/165, l='MpServer', x=211.56, y=65.00, z=279.47], EntityChicken['Chicken'/186, l='MpServer', x=236.88, y=64.00, z=279.81], EntityBat['Bat'/187, l='MpServer', x=240.70, y=30.00, z=334.12], EntityCow['Cow'/185, l='MpServer', x=239.63, y=60.00, z=287.38], EntityClientPlayerMP['Player250'/275, l='MpServer', x=238.10, y=73.62, z=265.17], EntityBat['Bat'/205, l='MpServer', x=246.54, y=26.81, z=298.92], EntityCow['Cow'/204, l='MpServer', x=247.47, y=68.00, z=277.47], EntityBat['Bat'/207, l='MpServer', x=248.92, y=21.00, z=314.43], EntityBat['Bat'/206, l='MpServer', x=246.42, y=27.12, z=300.01], EntityCow['Cow'/201, l='MpServer', x=252.47, y=63.00, z=245.75], EntityCow['Cow'/200, l='MpServer', x=246.53, y=63.00, z=247.50], EntityCow['Cow'/203, l='MpServer', x=242.50, y=67.00, z=276.38], EntityCow['Cow'/202, l='MpServer', x=250.69, y=64.00, z=285.53], EntityChicken['Chicken'/197, l='MpServer', x=246.50, y=64.00, z=233.50], EntityChicken['Chicken'/196, l='MpServer', x=255.59, y=65.00, z=232.53], EntityCow['Cow'/199, l='MpServer', x=246.53, y=63.00, z=247.50], EntityCow['Cow'/198, l='MpServer', x=245.47, y=63.00, z=247.50], EntityChicken['Chicken'/195, l='MpServer', x=246.50, y=64.00, z=234.19], EntityChicken['Chicken'/194, l='MpServer', x=245.50, y=64.00, z=233.50], EntityBat['Bat'/223, l='MpServer', x=281.75, y=22.10, z=231.25], EntityBat['Bat'/216, l='MpServer', x=274.01, y=51.66, z=312.15], EntityBat['Bat'/212, l='MpServer', x=265.21, y=37.30, z=299.28], EntityBat['Bat'/213, l='MpServer', x=266.52, y=56.24, z=314.18], EntityBat['Bat'/214, l='MpServer', x=264.56, y=60.10, z=306.38], EntityBat['Bat'/215, l='MpServer', x=268.92, y=56.67, z=315.09], EntityBat['Bat'/211, l='MpServer', x=269.50, y=48.10, z=267.25], EntityBat['Bat'/227, l='MpServer', x=286.47, y=39.05, z=281.83], EntityBat['Bat'/226, l='MpServer', x=277.38, y=47.10, z=272.50], EntityChicken['Chicken'/225, l='MpServer', x=276.47, y=62.23, z=262.53], EntityChicken['Chicken'/224, l='MpServer', x=276.41, y=62.30, z=267.41], EntityBat['Bat'/248, l='MpServer', x=297.25, y=47.45, z=294.33], EntityItem['item.tile.mushroom'/247, l='MpServer', x=296.13, y=22.13, z=241.88]] Retry entities: 0 total; [] Server brand: fml,forge Server type: Integrated singleplayer server Stacktrace: at net.minecraft.client.multiplayer.WorldClient.addWorldInfoToCrashReport(WorldClient.java:417) at net.minecraft.client.Minecraft.addGraphicsAndWorldToCrashReport(Minecraft.java:2567) at net.minecraft.client.Minecraft.run(Minecraft.java:983) at net.minecraft.client.main.Main.main(Main.java:164) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28)
-
When ever I put the item in the hot bar it crashes. I did try changing one thing because I want it to run that code everytime the item is pulled out not just in slot 2. @Override public void onUpdate(ItemStack par1ItemStack, World par2World, Entity par3Entity, int par4, boolean par5) { super.onUpdate(par1ItemStack, par2World, par3Entity, par4, par5); if (par3Entity instanceof EntityPlayer) { EntityPlayer player = (EntityPlayer)par3Entity; if (player.getHeldItem().equals(par1ItemStack)) { System.out.println("Test"); } } }
-
I am trying to run a method when someone pulls out the item on the hot bar. So if the item is in the third slot and the player switches to that I need it to run a method. How would I do that?
-
So I have a custom tool and I want it to just stop working when durability runs out. I figured I could do this by changing the tool material. How would I change the tool material without changing all the tools of the same type and change it while the player still has it. I already stop it from breaking but it still works. Can somebody explain how I would do that or a better way.
-
Thanks alot it is getDisplayName() just in a different location it was Minecraft.getMinecraft().thePlayer.getDisplayName();
-
How would I get the player name I cant seem to find anything on it because there all for the older versions of minecraft. I'm trying to accomplish getting the player name so I can have blocks have owners. Thanks for your help.
-
You are correct! Thank you very much for your help
-
it should work it is usually just very hard to see in the console Well it doesnt atleast not here