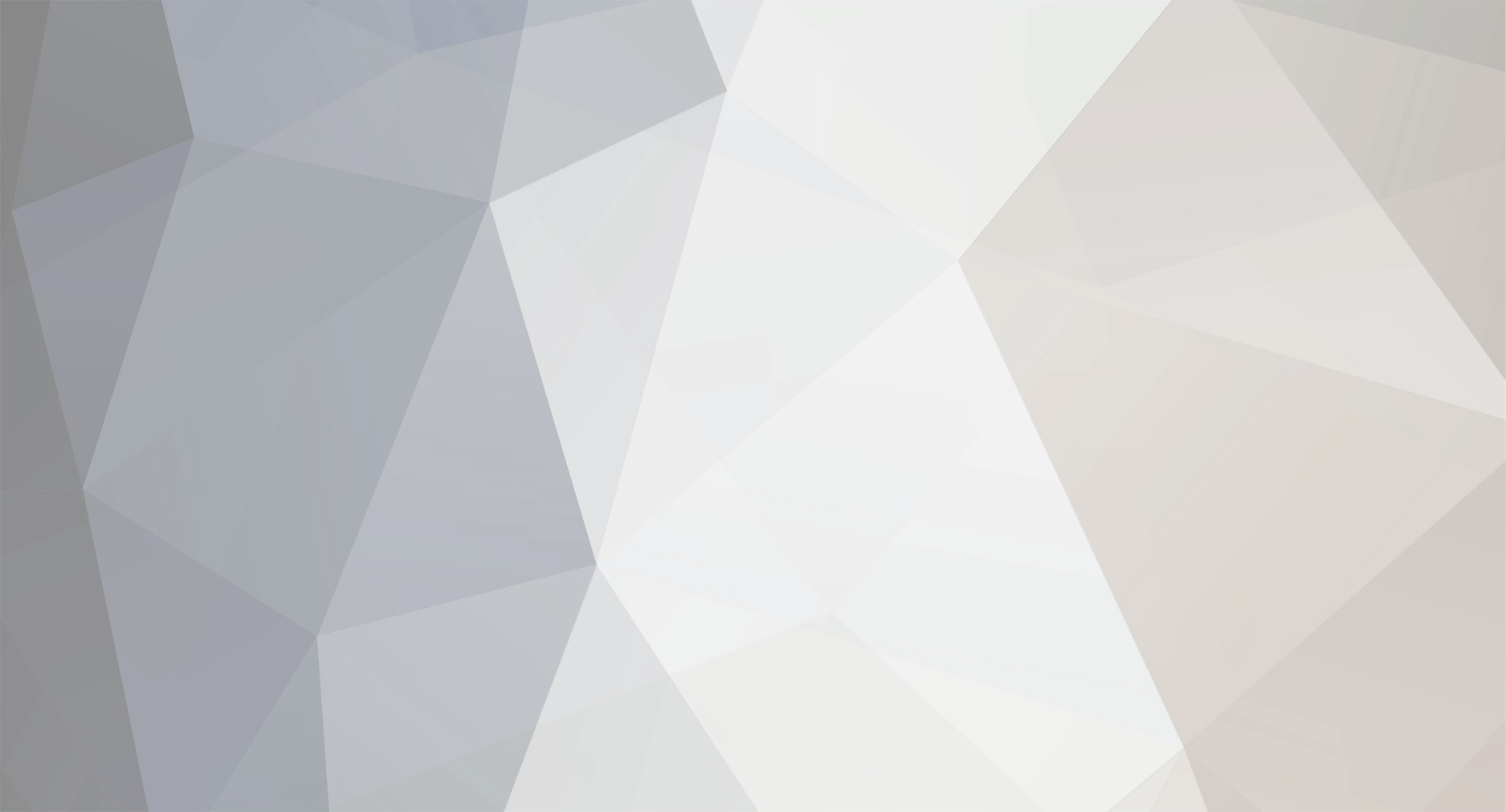
shadowking97
Members-
Posts
4 -
Joined
-
Last visited
Everything posted by shadowking97
-
A long while ago, I created an addon for Mystcraft that allowed for the loading and viewing of a secondary dimension. It was a fun challenge, and I learned a lot since then, so I decided I wanted to touch back with it and re-purpose and improve it for the sake of challenging myself. I am attempting to create the long-requested, yet untouched, seamless portals mod. This would require loading way more than the one extra dimension, and it would be a lot pickier about loading/unloading dimensions on the client side, but all of these are things I can handle and optimize. But I need to be able to view live entities in these other worlds, and changes to blocks. While I found entry points I could use for vanilla packets, in the interest of supporting most mods, I will have to create what is probably one of the greatest modding sins of all time: Coremodding forge. I want to be able to capture all packets that are sent close to one of these portals, or across a dimension, regardless of what mod it is, add dimensional data, repackage them, and send them to the additional clients, or server, that would need them. This would not affect the original packets, but merely copy them. Then objects would be swapped in and out of place while that specific packet is being handled, which in my experience on the first mod I had made, had been mostly lag-free and reliable. In order to allow a greater number of mods compatible, I plan on releasing a backwards-compatible API that would detect the presence of my mod and be able to pull the correct information so they would be able to pull any extra-world data they may save themselves. Not the most ideal of things, but possibly the only way to pull it off. But back to my query: My hopes here would be that someone who is experienced with the internals of forge could point me into the right direction as to what classes/functions I might need to add my own code to capture these packets. Thank you in advance for your time, and have a great day!
-
[1.7.2-1083] Netty Truncating Packets?
shadowking97 replied to shadowking97's topic in Modder Support
Mrph, thanks. I hadn't tried running optimizations on code I got from vanilla yet.... renamed variables only, basically. But anyway, now I have to track down what's causing the memory leak in my new packet pipeline and fix it. Then I should be good... -
[1.7.2-1083] Netty Truncating Packets?
shadowking97 replied to shadowking97's topic in Modder Support
They're not odd computations, they're how vanilla minecraft compresses the chunk data that I'm sending to my second dimension renderer. This is a converted vanilla minecraft packet so I can redirected to my instance of theWorld instead of the normal one. Here's my problem: I'm writing to the packet, both the length of the data and the data itself: buffer.writeInt(this.compressedChunkData.length); int i = buffer.writableBytes(); buffer.ensureWritable(this.compressedChunkData.length); buffer.writeBytes(this.compressedChunkData); And then I'm attempting to read it: int len = in.readInt(); if (field_149286_i.length < len) { field_149286_i = new byte[len]; } in.readBytes(field_149286_i, 0, len); But when I try to read it: Caused by: java.lang.IndexOutOfBoundsException: readerIndex(21) + length(2879) exceeds writerIndex(36): SlicedByteBuf(ridx: 21, widx: 36, cap: 36/36, unwrapped: UnpooledHeapByteBuf(ridx: 1, widx: 37, cap: 37/37)) So it SHOULD be sending a packet with the size of 21+2879, but it's only receiving a packet with the size of 36. The server isn't giving me any warnings or errors about the packet being too big when I try to send it (using simpleimpl), but the client isn't receiving it. In other notes: I have just switched my packet pipeline to one of the tutorial ones that doesn't use simpleimpl, editing it so I didn't have to mess with my packets (other than switching which classes everything extends), and it started working fine. Other than the fact that the tutorial I used was marked as having horrible memory leaks. But at least now I know it's not anything wrong with my code, but some error or limitation within simpleimpl. At least in 1083. -
Upon upgrading my dynamic link panels mod to 1.7.2, it appears my packets are being truncated by netty. I am using slightly modified versions of the simpleimpl packet handlers, only modified gain access to playerentity. This is the code I'm using in my packet: public void toBytes(ByteBuf buffer, ChannelHandlerContext ctx) { if (this.compressedChunkData == null) { deflateGate.acquireUninterruptibly(); if (this.compressedChunkData == null) { deflate(); } deflateGate.release(); } buffer.writeInt(dimension); buffer.writeInt(this.xPos); buffer.writeInt(this.zPos); buffer.writeBoolean(this.includeInitialize); buffer.writeShort((short)(this.yPos & 65535)); buffer.writeShort((short)(this.yMSBPos & 65535)); buffer.writeInt(this.compressedChunkData.length); int i = buffer.writableBytes(); buffer.ensureWritable(this.compressedChunkData.length); buffer.writeBytes(this.compressedChunkData); } @Override public void fromBytes(ByteBuf in, ChannelHandlerContext ctx) { this.dimension = in.readInt(); this.xPos = in.readInt(); this.zPos = in.readInt(); this.includeInitialize = in.readBoolean(); this.yPos = in.readShort(); this.yMSBPos = in.readShort(); int len = in.readInt(); if (field_149286_i.length < len) { field_149286_i = new byte[len]; } in.readBytes(field_149286_i, 0, len); int i = 0; int j; int msb = 0; //BugFix: MC does not read the MSB array from the packet properly, causing issues for servers that use blocks > 256 for (j = 0; j < 16; ++j) { i += this.yPos >> j & 1; msb += this.yPos >> j & 1; } j = 12288 * i; j += 2048 * msb; if (this.includeInitialize) { j += 256; } this.chunkData = new byte[j]; Inflater inflater = new Inflater(); inflater.setInput(field_149286_i, 0, len); try { inflater.inflate(this.chunkData); } catch (DataFormatException dataformatexception) { error = true; } finally { inflater.end(); } } And this is the crash I'm getting on the client side: Caused by: java.lang.IndexOutOfBoundsException: readerIndex(21) + length(2879) exceeds writerIndex(36): SlicedByteBuf(ridx: 21, widx: 36, cap: 36/36, unwrapped: UnpooledHeapByteBuf(ridx: 1, widx: 37, cap: 37/37)) at io.netty.buffer.AbstractByteBuf.checkReadableBytes(AbstractByteBuf.java:1160) ~[AbstractByteBuf.class:?] at io.netty.buffer.AbstractByteBuf.readBytes(AbstractByteBuf.java:668) ~[AbstractByteBuf.class:?] at com.shadowking97.mystcraftplugin.dynamicLinkPanels.network.packets.ChunkInfoPacket.fromBytes(ChunkInfoPacket.java:131) ~[ChunkInfoPacket.class:?] at com.shadowking97.mystcraftplugin.dynamicLinkPanels.network.impl.DLPIndexedCodec.decodeInto(DLPIndexedCodec.java:17) ~[DLPIndexedCodec.class:?] at com.shadowking97.mystcraftplugin.dynamicLinkPanels.network.impl.DLPIndexedCodec.decodeInto(DLPIndexedCodec.java:1) ~[DLPIndexedCodec.class:?] at cpw.mods.fml.common.network.FMLIndexedMessageToMessageCodec.decode(FMLIndexedMessageToMessageCodec.java:77) ~[FMLIndexedMessageToMessageCodec.class:?] at cpw.mods.fml.common.network.FMLIndexedMessageToMessageCodec.decode(FMLIndexedMessageToMessageCodec.java:17) ~[FMLIndexedMessageToMessageCodec.class:?] at io.netty.handler.codec.MessageToMessageCodec$2.decode(MessageToMessageCodec.java:81) ~[MessageToMessageCodec$2.class:?] at io.netty.handler.codec.MessageToMessageDecoder.channelRead(MessageToMessageDecoder.java:89) ~[MessageToMessageDecoder.class:?] ... 18 more The server isn't reporting any errors, so it *appears* buffer.ensureWritable is passing, allowing the packet to send the full chunkData... but the client isn't receiving the 2879 bytes that it's supposed to. If I can just be pointed in the right direction to ensure my packets are being sent and received completely, that would be lovely. Thank you!