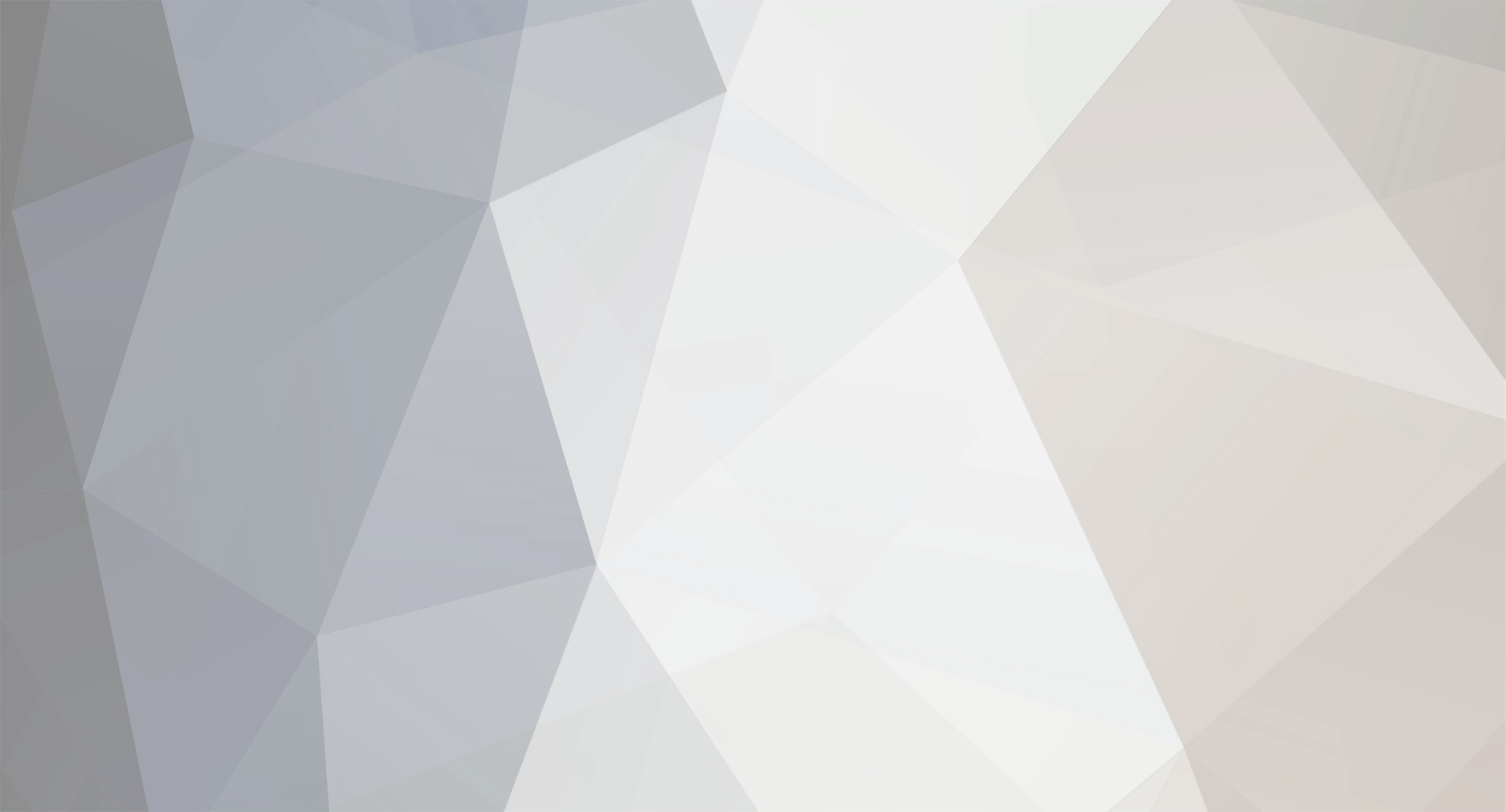
robmart
Members-
Posts
56 -
Joined
-
Last visited
Everything posted by robmart
-
[1.12.2] Problems with overriding vanilla potions
robmart replied to robmart's topic in Modder Support
Is it maybe because PotionUtils#addPotionToItemStack tries to get the name of the potion from the registry and since it isn't in the registry (overridden) it crashes? E: And it never checks if it's null because that'd be a smart thing to do -
[1.12.2] Problems with overriding vanilla potions
robmart replied to robmart's topic in Modder Support
Alright, I'll get rid of the static initializer. Here is the code for the register thingy public static <T extends IForgeRegistryEntry<T>> T getRegistryEntry( final IForgeRegistry<T> registry, final String name) { final ResourceLocation key = new ResourceLocation(name); final T registryEntry = registry.getValue(key); return Preconditions.checkNotNull(registryEntry, "%s doesn't exist in registry %s", key, RegistryManager.ACTIVE.getName(registry)); } Basically just a slightly better IForgeRegistry#getValue The code I use to register the potion types is the same as normal @SubscribeEvent public static void registerPotionTypes(final RegistryEvent.Register<PotionType> event) { event.getRegistry().registerAll( STRENGTH, LONG_STRENGTH, STRONG_STRENGTH ); -
[1.12.2] Problems with overriding vanilla potions
robmart replied to robmart's topic in Modder Support
Isn't what I'm doing the same as what vanilla does? registerPotionType("strength", new PotionType(new PotionEffect[] {new PotionEffect(MobEffects.STRENGTH, 3600)})); That's from vanilla. I can see 3 differences: 1. I don't use an array. 2. I don't hardcode the names, I get them from the potion 3. I get the potions from the registry instead of getting them directly What am I doing wrong? -
[1.12.2] Problems with overriding vanilla potions
robmart replied to robmart's topic in Modder Support
What isn't it's intended purpose? Please elaborate. The RegistryHelper thing gets the potion from the registry. -
[1.12.2] Problems with overriding vanilla potions
robmart replied to robmart's topic in Modder Support
Registered a normal potion with this public PotionBase(final boolean isBadEffect, final int liquidColor, final String name, boolean useGlint) { super(isBadEffect, liquidColor); setPotionName(name); } public Potion setPotionName(final String potionName) { this.setRegistryName(potionName); final ResourceLocation registryName = Objects.requireNonNull(this.getRegistryName()); super.setPotionName("effect." + registryName.toString()); return this; } Except instead of using my mod id I used minecraft Then I registered the potion types like this public static final PotionType STRENGTH; public static final PotionType LONG_STRENGTH; public static final PotionType STRONG_STRENGTH; static { final String LONG_PREFIX = "long_"; final String STRONG_PREFIX = "strong_"; final int HELPFUL_DURATION_STANDARD = 3600; final int HELPFUL_DURATION_LONG = 9600; final int HELPFUL_DURATION_STRONG = 1800; final IForgeRegistry<Potion> potionRegistry = ForgeRegistries.POTIONS; final Potion strength = RegistryHelper.getRegistryEntry(potionRegistry, RefPotionNames.STRENGTH); STRENGTH = createPotionType(new PotionEffect(strength, HELPFUL_DURATION_STANDARD)); LONG_STRENGTH = createPotionType(new PotionEffect(strength, HELPFUL_DURATION_LONG), LONG_PREFIX); STRONG_STRENGTH = createPotionType(new PotionEffect(strength, HELPFUL_DURATION_STRONG, 1), STRONG_PREFIX); } private static PotionType createPotionType(final PotionEffect effect) { return createPotionType(effect, null); } private static PotionType createPotionType(final PotionEffect effect, @Nullable final String namePrefix) { final ResourceLocation potionName = effect.getPotion().getRegistryName(); final ResourceLocation potionTypeName; if (namePrefix != null) { assert potionName != null; potionTypeName = new ResourceLocation( potionName.getResourceDomain(), namePrefix + potionName.getResourcePath()); } else potionTypeName = potionName; assert potionName != null; return new PotionType(potionName.toString(), effect).setRegistryName(potionTypeName); } I of course registered both all of these using the RegistryEvent.Register event to register these. -
So I've overridden the vanilla strength potion and potion types however when I try to brew the potion the game crashes. It's obviously because Minecraft is trying to add the brewing recipe for the normal strength potion, but the potion types are not in the registry. How can I fix this?
-
[Solved] [1.12.2] Generating blocks on soul sand in the nether
robmart replied to robmart's topic in Modder Support
Yup I just somehow missed that. Thanks for the help! -
[Solved] [1.12.2] Generating blocks on soul sand in the nether
robmart replied to robmart's topic in Modder Support
Alright, got it! Would me replacing the decorator on all biomes with the type nether not potentially fuck up other mods' generation though? -
[Solved] [1.12.2] Generating blocks on soul sand in the nether
robmart replied to robmart's topic in Modder Support
I pretty sure I understand, but where would I do the iterating and replacing? In the event? -
I need to generate my block (a flower) on soul sand in the nether. The actual generation I have no problem with. What I need help with is actually doing it *on* the soul sand. This is my current code: @SubscribeEvent(priority = EventPriority.LOWEST) public static void onWorldDecoration(DecorateBiomeEvent.Decorate event){ if((event.getResult() == Event.Result.ALLOW || event.getResult() == Event.Result.DEFAULT) && event.getType() == DecorateBiomeEvent.Decorate.EventType.SHROOM) { if (event.getWorld().provider.isNether()){ int dist = Math.min(8, Math.max(1, 4)); for(int i = 0; i < 1; i++) { if(event.getRand().nextInt(8) == 0) { int x = event.getPos().getX() + event.getRand().nextInt(16) + 8; int z = event.getPos().getZ() + event.getRand().nextInt(16) + 8; int y = event.getWorld().getTopSolidOrLiquidBlock(event.getPos()).getY(); for (int j = 0; j < 1 * 8; j++) { int x1 = x + event.getRand().nextInt(dist * 2) - dist; int y1 = y + event.getRand().nextInt(4) - event.getRand().nextInt(4); int z1 = z + event.getRand().nextInt(dist * 2) - dist; BlockPos pos2 = new BlockPos(x1, y1, z1); if (event.getWorld().isAirBlock(pos2) && InitBlocks.HELL_FLOWER.canPlaceBlockAt(event.getWorld(), pos2)) { event.getWorld().setBlockState(pos2, InitBlocks.HELL_FLOWER.getDefaultState()); System.out.println("Placed flower " + pos2); } } } } } } } I obviously cannot use EventType.FLOWERS since that doesn't trigger in the nether, and I can't keep using EventType.SHROOM either since that doesn't accomplish what I want. Oh and I need a replacement for getWorld().getTopSolidOrLiquidBlock since that'll give me the bedrock. Any help?
-
Right so I have my custom creative tab GUI and I've replaced the vanilla one with it. My only problem is that I can't seem to figure out *where* to filter the stuff out. In fact, I can't find where or how stuff is added to the tab in the first place. Would you mind pointing me in the right direction?
-
@jabelar For some reason the GuiOpenEvent doesn't seem to be doing anything. I have this code as a test @GameRegistry.ObjectHolder(Reference.MOD_ID) @Mod.EventBusSubscriber(modid = Reference.MOD_ID) @SuppressWarnings("unused") public class CreativeTabHandler { @SubscribeEvent public void onGuiOpen(GuiOpenEvent event){ event.setGui(new GuiChat("Test")); } } but it doesn't do anything. I've tried other things but I just can't seem to get the event to work. Am I doing something wrong? P.S: Sorry for the constant barrage of messages.
-
I have a potion base class: https://github.com/robmart/RPG-Mode/blob/master/src/main/java/robmart/rpgmode/common/potion/PotionBase.java And the potion class: https://github.com/robmart/RPG-Mode/blob/master/src/main/java/robmart/rpgmode/common/potion/PotionStrength.java Now my problem is that the code in PotionBase.renderHUDEffect doesn't run, the picture doesn't render and the print message isn't printed. The rest of the potion works fine.
-
[1.8.9] Multiple item textures in one file (image)
robmart replied to robmart's topic in Modder Support
So how would I do this? EDIT: Also, why do you not recommend it? -
[1.8.9] Multiple item textures in one file (image)
robmart replied to robmart's topic in Modder Support
Because I have a lot of items that change texture depending on the metadata. I figured it would be a lot cleaner to have it this way then to have 17 different image files. -
I have a file that is 32 x 16 pixel with two textures in it. I want to specify that the texture is from pixel 17 to pixel 32. Is this possible, and if so how? This is my current code: public static void registerItemRenderer(){ registerItemModel(InitItems.berylloniteGem); } public static void init(Item item, int meta){ RenderItem renderItem = Minecraft.getMinecraft().getRenderItem(); renderItem.getItemModelMesher().register(item, meta, new ModelResourceLocation(item.getUnlocalizedName().substring(5), "inventory")); }
-
[1.8.9] RuntimeException on saving tile entity NBT data
robmart replied to robmart's topic in Modder Support
I do register the tile entity, but my tile entity extends BlockContainer so i don't use registerTileEntity EDIT: The tile entity works like normal but when saving the world the error is printed in the log -
This is my code @Override public void writeToNBT(NBTTagCompound nbt) { super.writeToNBT(nbt); NBTTagList list = new NBTTagList(); for (int i = 0; i < this.getSizeInventory(); ++i) { if (this.getStackInSlot(i) != null) { System.out.println(i); NBTTagCompound stackTag = new NBTTagCompound(); stackTag.setByte("Slot", (byte) i); this.getStackInSlot(i).writeToNBT(stackTag); list.appendTag(stackTag); } } nbt.setTag("Items", list); if (this.hasCustomName()) { nbt.setString("CustomName", this.getCustomName()); } } The line super.writeToNBT(nbt); gives me this error [23:21:52] [server thread/ERROR]: A TileEntity type robmart.rpgmode.entity.tileentity.ContainerTileEntity has throw an exception trying to write state. It will not persist. Report this to the mod author java.lang.RuntimeException: class robmart.rpgmode.entity.tileentity.ContainerTileEntity is missing a mapping! This is a bug! at net.minecraft.tileentity.TileEntity.writeToNBT(TileEntity.java:86) ~[TileEntity.class:?] at robmart.rpgmode.entity.tileentity.ContainerTileEntity.writeToNBT(ContainerTileEntity.java:200) ~[ContainerTileEntity.class:?] at net.minecraft.world.chunk.storage.AnvilChunkLoader.writeChunkToNBT(AnvilChunkLoader.java:393) [AnvilChunkLoader.class:?] at net.minecraft.world.chunk.storage.AnvilChunkLoader.saveChunk(AnvilChunkLoader.java:172) [AnvilChunkLoader.class:?] at net.minecraft.world.gen.ChunkProviderServer.saveChunkData(ChunkProviderServer.java:257) [ChunkProviderServer.class:?] at net.minecraft.world.gen.ChunkProviderServer.saveChunks(ChunkProviderServer.java:324) [ChunkProviderServer.class:?] at net.minecraft.world.WorldServer.saveAllChunks(WorldServer.java:945) [WorldServer.class:?] at net.minecraft.server.MinecraftServer.saveAllWorlds(MinecraftServer.java:427) [MinecraftServer.class:?] at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:144) [integratedServer.class:?] at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:548) [MinecraftServer.class:?] at java.lang.Thread.run(Thread.java:745) [?:1.8.0_51]
-
So i fixed it, but it still crashes. New log https://gist.github.com/robmart/5f5fe85573fb54b2a077
-
Forge version = 1506 I'm getting a nullpointer, but i can not find where it is. The game is not crashing, but it stops loading the game at "Loading - Initializing mods Phase 1". This is the log: https://gist.github.com/robmart/490b7910c0ae10c457c2 InitItems class ItemTextures