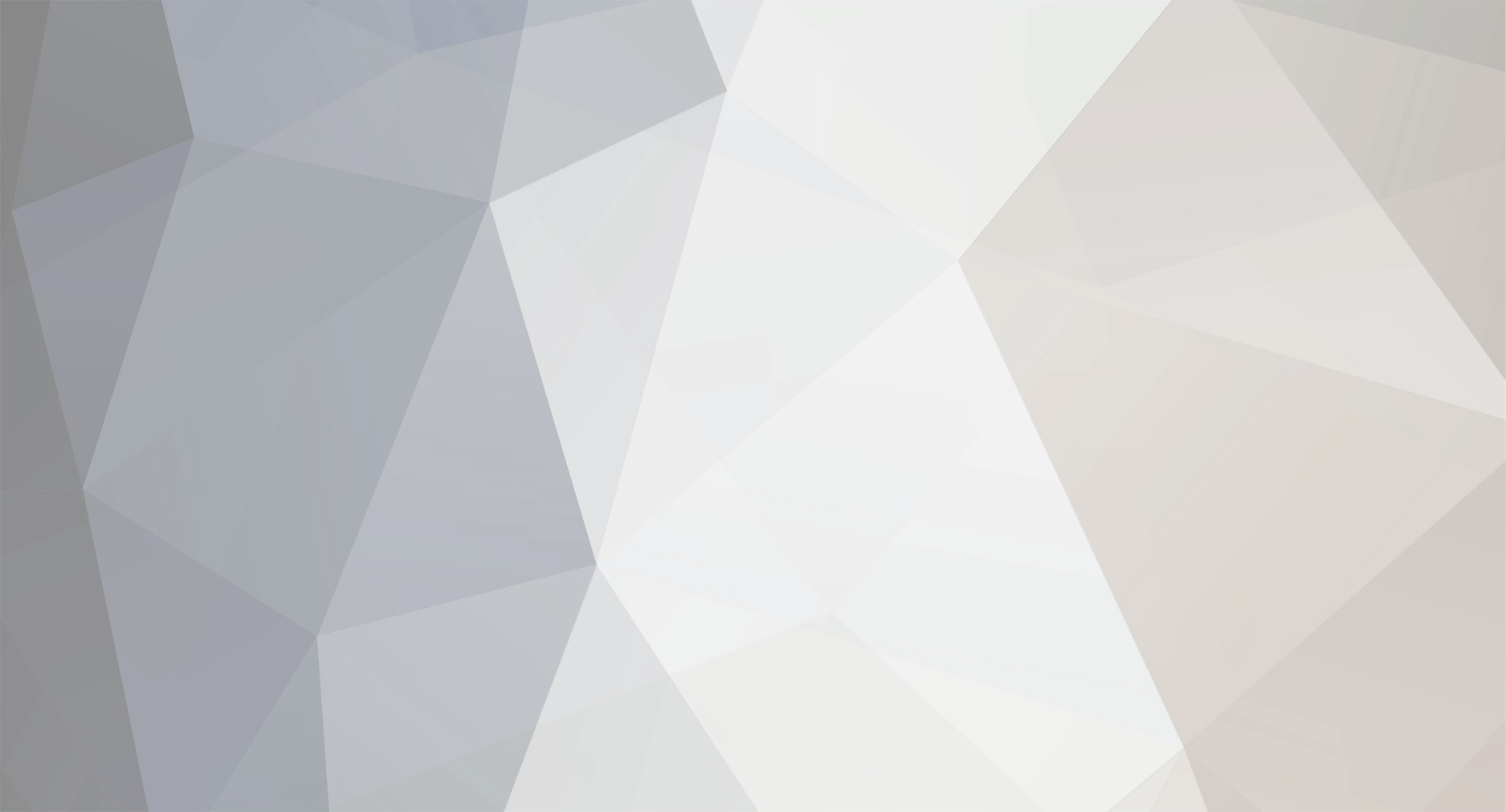
Black
Members-
Posts
103 -
Joined
-
Last visited
Everything posted by Black
-
[1.7.10][Connection] How to connect to an online radio and listen to it
Black replied to _gjkf_'s topic in Modder Support
Well I really don't have time to write a long reply as I only came to check something here but let me warn you this isn't something you should do if you need to ask the question on a board unrelated to it. And yes, this is advanced base java, not minecraft forge code. And sure, it is doable, afterall it is just a matter of connecting to the url, getting the stream and putting it through to the desired output(and a lot of other bits and pieces). Something which is easily explained however far less fun to do without a decent bit of experience in that particular field of programming. I'll point you in the right direction but you'll need to do the rest mostly yourself. First of all you have a perfect, well not perfect but decent enough to know how to do it, with that openblocks code. 'It didn't help me' is well... good luck getting any further with programming this radio. Secondly, look into connecting to an url, and look into andriod based radios as those applications do that often. As for ITzZ, you can set the stations in the config files of openblocks. Secondly, the openblocks code uses a open source android class to do it so you can easily use it. -
Disclaimer: I haven't been modding minecraft for very long TheGreyGhost gave a typical Java answer. And that's also something you have to keep in mind, minecraft is written in java. It's not in C++ with its handy destructors, pointers, cpu & memory control Instead it is in java which is a generalized language with less possibilities towards your particular application & optimalization but easier to code in e.g. it is meant for not worrying about cpu or ram. So in a sense it suffices to simply follow normal procedure of only saving necessary things and calculating the rest, avoiding calculations in for loops or in much triggered methods etc. The way I generally approach my minecraft methods is if I see they'll be called a lot without needing it is by simply throwing in an if statement first. Other than that, there really isn't a need to worry about cpu or memory unless you really see it. Just write your classes and after you're done, just go over them and improve where possible.
-
In the block class there is a function getHarvestLevel(metadata) Just return the harvestlevel according to the metadata you get and don't set it in the constructor.
-
Well, nbt does seem the way to go. However, if I were you I'd store that on the player instead. Afterall, it is the player who can't access it, not the block, e.g. it is information about the player. Thus according to propper programming ethique it is data that should belong in the player. Furthermore, let us consider a server with 100 players. If the 100th player click on the block it has to iterate through a list of 99 players, check if the player is in the list and if not hand the item. Now if that information was in the player, then it would have to iterate through only 5 blocks, namely the 5 possible blocks he can click on. And this can even be solved with a simple array of booleans. Also worth to note is that the amount of data that has to be saved is about the same while the data it needs to load at one time is significantly less, just 5 booleans for the player. I think this is a decent start for a suggestion. Consider it.
-
I gave it a quick test since I wanted to see whether this code was still viable in 1.7.10. I hadn't gotten around to fixing a syringe in my own mod so this was an excellent opportunity. This animates the provided textures. @Override @SideOnly(Side.CLIENT) public IIcon getIcon(ItemStack stack, int renderPass, EntityPlayer player, ItemStack usingItem, int useRemaining) { if (usingItem == null) { return itemIcon; } int ticksInUse = stack.getMaxItemUseDuration() - useRemaining; if (ticksInUse > 18) { return iconArray[2]; } else if (ticksInUse > 14) { return iconArray[1]; } else if (ticksInUse > 0) { return iconArray[0]; } else { return itemIcon; } } As coolAlias said, set this.setFull3D() in constructor and provide the texture with public static final String[] SyringePullIconNameArray = new String[] {"pulling_0", "pulling_1", "pulling_2"}; @SideOnly(Side.CLIENT) private IIcon[] iconArray; and this @SideOnly(Side.CLIENT) @Override public void registerIcons(IIconRegister parIconRegister) { this.itemIcon = parIconRegister.registerIcon(MODID + ":" + "basesyringe"); this.iconArray = new IIcon[syringePullIconNameArray.length]; for (int i = 0; i < this.iconArray.length; ++i) { this.iconArray[i] = parIconRegister.registerIcon(MODID + ":" + "basesyringe" + "_" + SyringePullIconNameArray[i]); } } I gave you a lot, which is not my habit unless I see that the person knows java/modding/does effort, but at least I now know where to refer to once there is a similar question asked here.
-
I'll be just as concise as you are. Use an event for your binded key. Set a value with that. Take that value with a switch and bind the texture you want. Just make sure you open & close your matrix/tessellator/... in each statement.
-
That's because the normal bow animation is a hardcoded one only useable for the vanilla code. In this topic a solution is offered: http://www.minecraftforum.net/forums/mapping-and-modding/minecraft-mods/modification-development/1437164-1-6-4-forge-bow-texture-not-working And it might just solve your rotation problem, which is probably caused by the animation not working thus rendering a standard item.
-
Well, first of all add a system.out.println("test"); just inside the function (before the if statement). That way, if it is triggered you'll see it in the console printing test. Or it might just be that this isn't the correct event you need, in which case you can also make sure the event triggers by opening a chest, which is a container. However, I've told you before how to find the list of events and it is up to you to look through them and find the event that fits your needs perfectly. You'll learn more that way than if I spoon feed you everything. Secondly, have you registered your event in your main class? It won't trigger if isn't registered correctly. And if you don't know how, look up minecraft forge events in google, I'm sure you'll find the correct line of code for it.
-
The parameter is your event, and I would create a new event handler class for it(don't forget to register it as you normally would). (no idea if it'll trigger with yours but it just might and otherwise there are plenty of other options, look through them) Then check if the container is your ship inside this method, get the player and check his inventory etc. Never use a null parameter, it serves no other purpose than to crash the game. And don't call that event, it'll trigger once the player opens a container. That's how events work, you don't have to call them at a particular point, they trigger when an event happens.
-
The problem with declaring an EntityPlayer at the top is that it is empty so even if you add an achievement to that player object it won't show up in the game(if it doesn't crashes because of it, as that also would be a nullpointer because you didn't create a new player) because simply, that player object doesn't contain the current player, it is as simple as that. If I were you I'd set up my event again in my custom eventHandler, choose the event correctly and register it. If you want to know what all the events are, go to the event class (F3 on any type of event class and then F3 on the eventclass itself) and type ctrl+T for the type hierarchy. For instance, there is a playercontainer event, which is called when a player opens a container. And secondly, don't call the method yourself.
-
I take it that with the textures not working you mean the animation isn't being done? This thread offers a perfect solution: http://www.minecraftforum.net/forums/mapping-and-modding/minecraft-mods/modification-development/1438011-1-7-2-custom-bow-not-using-animation-textures And if you have trouble with the texture itself, it might solve that as well.
-
Alright, so now you know which line is returning null. What you have to do now is deduce what is returning a null. Let us analyse the different possibilities. event.player.addStat(Skyline.achievementCivilianCaptain, 1) We clearly only have 2. The addStat and the achievement itself. One of them is null, why? Looking at addStat we only have 3 possibilities: Either you didn't register it correctly, you're calling the method from somewhere which shouldn't be done(the game calls the method itself when something is crafted, that's what the event is for), or the event doesn't return the player. Of the three, the most likely are 1 & 2. Looking at the achievement itself, well as long as the achievement was created and registered correctly it should be work. So have a look at those two different things. I personally believe you are calling the method instead of letting the event handle it. Or as a last option, I might be wrong as well since I haven't implemented an achievement yet(although I am going to create one now).
-
Well, reading your errorlog always helps: java.lang.NullPointerException: Rendering screen at esvdefcon.skyline.chunk.AssembleResult.getShipType(AssembleResult.java:244) Have a look at the line 244 of the file. It should be within the method getShipType. One of variables or methods you call there return a null. E.g. what they contain or return is empty. Check which one it is (by substituting the others until you find which one crashes for instance) and then look at what that method or variable actually does and why it returns null. And you weren't really wrong with ItemCraftEvent. It's just that it's only going to trigger when you have crafted something.
-
No I'm pretty sure he typed this according to his log: gradlew gradlew setupDecompWorkspace --refresh-dependencies So gradlew was looking inside its folders for a task it could execute called gradlew which it obviously doesn't have since that's itself.
-
Decomp is still functional, I used it two days ago on the latest forge snapshot. Generally all you really have to do is download the src version of the latest forge, unzip it in the folder your project goes in(make sure it is an empty folder and that the foldername doesn't contain weird signs or symbols), shift right click and click on 'open console here' type in gradlew setupDecompWorkspace, let it run until complete and run gradlew eclipse as many times said before. And if it doesn't work no matter what, give the 1.6.4 935 I think it was a go and see if that works, if neither of them does its obvious the problem is on your side.
-
Allright, First of all the error log (reading that thing actually helps, who knew) Caused by: java.lang.NullPointerException at net.theepictekkit.generator.common.tileentity.TileEntityDistillationChamberFrame.checkMultiBlockForm(TileEntityDistillationChamberFrame.java:53) This line tells you that at line 53 of the file and in the method checkMultiBlockForm a nullpointerexception is occuring. E.g. You're asking an object and a null is returned. Go to that line and find out why you're not getting what you're asking. Secondly: The line I gave you puts the TileEntity in a variable of type TileEntity. It's quite obvious it can't call methods that are only known by its subclass. A solution is a simple reflection. Replace TileEntity with the class you want to access (granted it inherits the Tileentity class some way or another) and place that same class in round brackets just after the '='. Example: TileEntityDistillationChamberFrame Tile = (TileEntityDistillationChamberFrame) parWorld.getTileEntity(parCoordinateX, parCoordinateY, parCoordinateZ); Thirdly: I didn't notice this before but this might possibly be the longest line of closing brackets I have seen that are actually useful, and I have seen quite a bit of code already.
-
Well, I'm not going to write this mod for you know, you'll have to do your own research. There are several methods but the best way I believe is that you can increment a counter if you've found the correct liquid and deleted it(whether as item or block). Then if you need to make the liquid come out again you decrement the counter and spawn a liquid block at the correct coordinates. Since the core block is obviously a tile entity, simply save the counter in nbt.
-
Well, to start of with, replace about 300 of those if statements with a few nested for loops. It'll clean your code up a lot. Secondly, there are several methods to do the things you asked. One way is all but one block are dummy blocks and simply reference the main block and its inventory, right click etc. Second way, make them all real blocks but each has a reference of the original block as to interact with it. So basically you always need one main block and every other block interacts with it. This doesn't mean those blocks can't do anything but the things that have to be the same everywhere will use that. However do NOT attempt the following: Make all blocks identical, and constantly update each other, you're wasting too much cpu that way. You can use the same block though, just make a block and if it is in the correct position(for instance in the bottom middle if it is a 3x3 at the bottom(it can detect another block there and has the same block around it) and simply let it behave as the core block, if isn't in that spot let it behave as a fake block.
-
Well, most of this based on how you design it really. First, I want you to understand this is simply how I would do something. This doesn't mean this is the only correct way. And actually, this should be your homework instead of mine. It seems you do not understand what a tile entity (you should if want to add a complicated thing to minecraft). A tile entity creates an object for each separate block. This is opposed to a normal block class which uses the same object for each block. This means a tile entity is more flexible but also takes more memory and cpu. You can also save data particular to that block in the nbt of a tile entity. And each tile entity has a loop built in (a method called updateEntity) which is called every 1/20th of a second. //Just a note, I changed something from my previous comment, this way is better. //And I am also not accounting for the physical blocks, I assume you have a way of dealing with those. We have(or I assume you have) at least 1 tileentity. The ship. Its update method handles everything that happens automatically such as movement by wind for instance. It also has a keydown event method. This method only fires if the player controlling the ship(once again, look at horses, minecarts etc) pressed a key. The ship also has an inventory which can be loaded by, for instance placing bombs in slots in a gui, right clicking certain spots in the ship etc. The ship also has a custom render class to render its object. In there you render the ship and you also check how many bombs there are in the inventory of the ship. If the ship has at least 1 bomb it will render a big one above the hatch to drop bombs, else, for every bomb added it will render extras in shelves. (And if you want to be really fancy you even let the big bomb disappear for a while after you've dropped the bomb to simulate the reloading). So, the player controls the ship and presses a key. Our previously created function checks for the correct one and if correct will look into the inventory of the ship(a byte for instance) and if it more than 0 it will spawn above the hatch an explosive and decrement our byte(So the ship has one bomb less in the inventory and thus renders one less). From this point onwards the bomb exists as a separate entity with its own loop. In that loop it decrements a value which renders the bomb on the y axis. Either look at the item or sand class or do some trickery with a loop that counts down the y value of a block(not the coordinate Y but the one that places the block points minY - maxY etc) and if it hits the bottom of the block you go to the next one and do the same. Then simply use that value in your render class to translate this together with the Y coordinate. It's a bit tricky though so make sure to have a good look at the sand class if it doesn't use a better method (I didn't yet so I can't tell you how it works) It falls until you tell it to stop(for instance if it collides with something). At that point you tell it to explode and you delete the tileentity(and block). Case Closed. The bomb is dropped and high above in the ship another one is ready to be dropped. And if you want to drop several behind each other simply use an if statement and counter in the updateEntity method of the ship that will drop the bomb. Check if it has bombs, the counter is dividable (% in java) by a certain number (such as 100. E.g. every 100 1/20th seconds it will drop one), and if the playerdrop boolean is true(you'll need the gap between your key event method and the update method). If it is true, set counter to 0 and start counting. Etc. But honestly, you should be able to come up with this easily if you want to make objects that can travel large distances without lagging in minecraft. Now, why did I even bother writing this all out? EDIT: you can obviously substitute the ships inventory with that of the controlling player. Simply use that to check how many bombs need to be rendered, dropped etc.
-
It's really simple actually. First of all, make your bomb a tileentity, I think that's a given. In the update method just check a boolean if it should fall or not. Then set a control panel(for instance, the helm) from where the player can operate the bomb. I also take it the ship itself is a tileentity and the helm is a part of it. Check in the update method of the ship if the player operating it(like in a minecart) is pressing a particular key, or you can write a custom method outside of the tileupdate. This is generally better since there is more code optimalization. In any case, check in the method if your particular key(or key bind) is down. If it is down set the boolean of the bomb to true. How you do that will be most likely with reflection but I can't tell you how to get the bomb as I don't know if you have one or multiple bombs placed. It will most likely be a case of scanning the area for one. Once the boolean has been set to true, simply start decreasing the y axis value. However you'll need to get it smooth. Have a look at the code of an item that drops to the ground. And the cannons aren't any more difficult. The only difference is calculating the rotation and trajectory of the cannon and bullet. The rotation is the same as if you would animate a mob. Simply seperate it from the shipmodel, rotate it with opengl and translate the xyz coordinates to where you want it on the ship. The most difficult part is the arc. You'll have to fiddle around a bit with that but all you really have to do is look up how to calculate trajectories of objects. There are plenty of tutorials out there for all sorts of games.
-
I don't know if this is the right kind of mod to write if you still have to find out how you detect a keypress. Nonetheless, plenty of info on all sort of sides: Opengl: http://www.swiftless.com/tutorials/opengl/keyboard.html Basic Java: http://docs.oracle.com/javase/tutorial/uiswing/events/keylistener.html A lwjgl example: http://ninjacave.com/lwjglbasics2
-
SOLVED - Machine model wont face player [1.7.10]
Black replied to iLegendx98's topic in Modder Support
Hmm, I did not think of that, but a very good point. Nonetheless, the solution is still equally as simple. Simply check if the metadata is 0. And if it is, set the default position. If it isn't, that means the player has put the block down and the metadata has been filled, if at least, you didn't set a side except the default position to metadata 0. -
My apologies, it seems I misread your problem. Another solution is a custom craftingHandler. It does check everytime something is crafted but the impact is really negligible. It suffices that everytime something is crafted you check if the first slot contains a plank with an if and then look at the other matrixslots inside. Once you have checked that the planks are indeed the same, pass the metadata to the crafted item or if isn't the same, cancel the crafting.