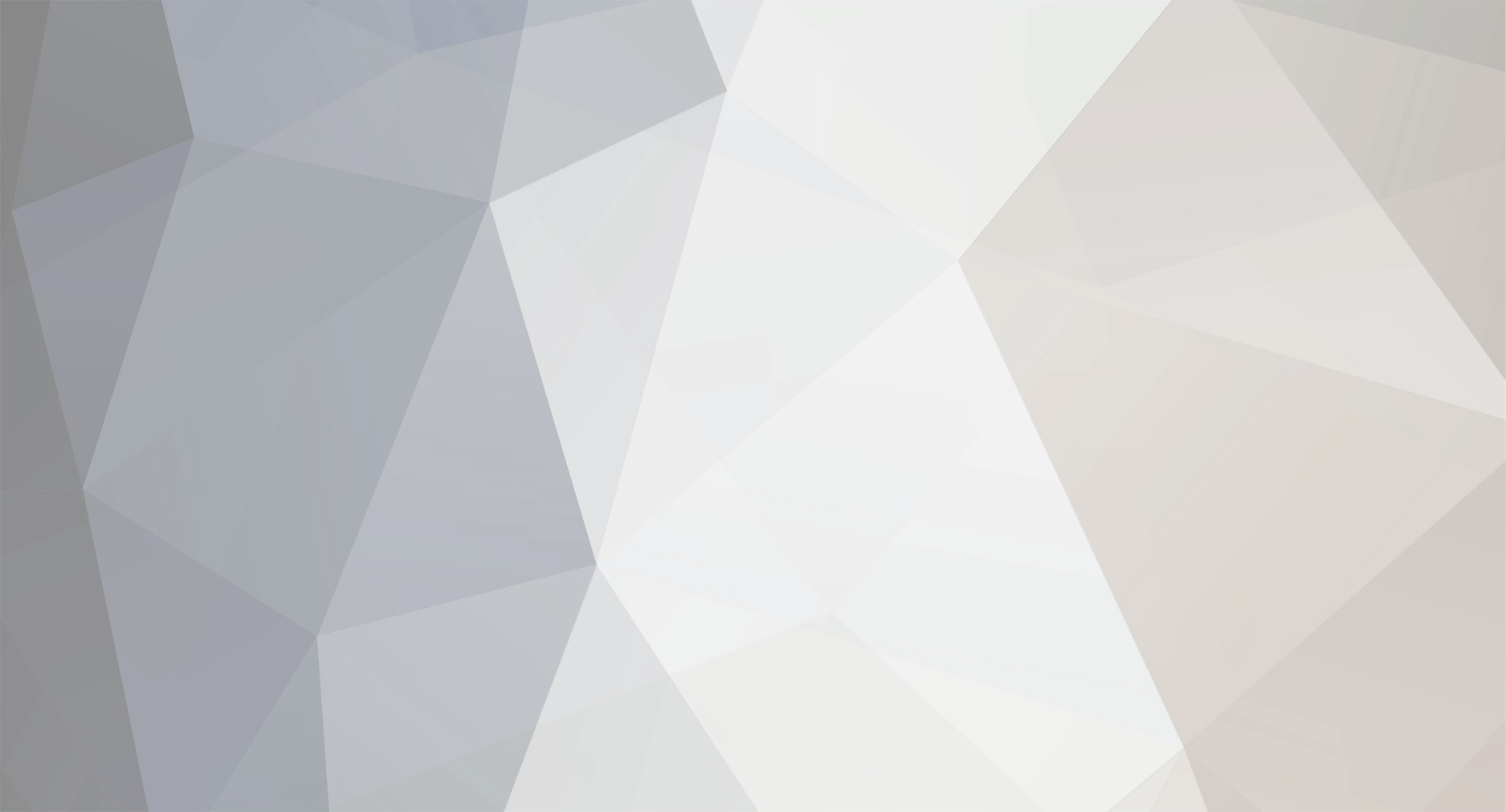
strumshot
Members-
Posts
133 -
Joined
-
Last visited
Everything posted by strumshot
-
[SOLVED] Change NBT tag of ItemStack in container from GUI
strumshot replied to xilef11's topic in Modder Support
return new SendCost((short) container.tileEntity.Cost); In this scenario, SendCost is just another packet, registered on the client side, not the server. So just like any other packet. network.registerMessage(PacketToServer.Handler.class, PacketToServer.class, 0, Side.SERVER); network.registerMessage(ResponseToClient.Handler.class, ResponseToClient.class, 1, Side.CLIENT); @Override public IMessage onMessage(PacketToServer message, MessageContext ctx) { return new ResponseToClient(); } @Override public IMessage onMessage(ResponseToClient message, MessageContext ctx) { return null; } -
Preventing an item from being placed in a container?
strumshot replied to ianc1215's topic in Modder Support
This is just a method I chose to use to override vanilla tnt - doesn't mean its the best idea - but I subscribed to PlayerInteract event and intercepted a player placing the block on the ground. public class BuildEventHook { @SubscribeEvent public void onClick(PlayerInteractEvent event) { if (event.action == event.action.RIGHT_CLICK_BLOCK) { if(!event.world.isRemote){ if(event.entityPlayer.inventory.mainInventory[event.entityPlayer.inventory.currentItem] != null && event.entityPlayer.inventory.mainInventory[event.entityPlayer.inventory.currentItem].getItem() == Item.getItemFromBlock(Blocks.tnt)){ int i = event.face; int x = event.x; int y = event.y; int z = event.z; boolean can = false; // bottom top north south west east if(i == 0){ y--; } else if(i == 1){ y++; } else if(i == 2){ z--; } else if(i == 3){ z++; } else if(i == 4){ x--; } else if(i == 5){ x++; } if(event.world.isAirBlock(x, y, z)) can = true; List l = event.world.getEntitiesWithinAABB( Entity.class, AxisAlignedBB.getBoundingBox( (double) x, (double) y, (double) z, (double) x + 1.0d, (double) y + 1.0d, (double) z + 1.0d)); if(l.size() > 0) can = false; if(can){ event.world.setBlock(x, y, z, IFaction.NewTNT); event.world.notifyBlockOfNeighborChange(x, y, z, IFaction.NewTNT); } event.setCanceled(true); } } } } } -
[Solved] Packets not updating GUI in published mode only
strumshot replied to strumshot's topic in Modder Support
So wait... basically IsRemote is relative to each thread? Meaning the server is remote from client, and the client is remote from server? If so, that seems problematic to me and I should be using SideOnly whenever possible... -
Preventing an item from being placed in a container?
strumshot replied to ianc1215's topic in Modder Support
A few things... custom container/slot classes would be easiest, via Is ItemValidForSlot, for example. As for replacing vanilla blocks, its a bit more difficult, but you are on the right track. There are few containers automatically placed in the world, I find it easier to hijack a Block placing event and substitute the vanilla block being placed with a custom class. Check the vanilla classes, like maybe furnace, as it display ISidedInventory and some of the functions you would override to filter items. -
Update: 1.6.2.1 (check the changelog!)
-
[Solved] Packets not updating GUI in published mode only
strumshot replied to strumshot's topic in Modder Support
I had if(world.isRemote == false) IShop.network.sendToServer(new RequestCost()); and it didn't fix it. Am I dyslexic and looking at this backwards? That is seriously possible, lol. -
[Solved] Packets not updating GUI in published mode only
strumshot replied to strumshot's topic in Modder Support
I'm moving the RequestCost into the gui initialization to see if that clears it up. I'll edit this post with results... That solved it. Even after doing an explicit remote check. Odd. I'd sure like to know how that happened, but all is well that ends well. -
[Solved] Packets not updating GUI in published mode only
strumshot replied to strumshot's topic in Modder Support
Actually I did paste that part, so you say that with that in mind.. I should do a more explicit check at the time of sending I guess? How did it get past my if/else? -
[Solved] Packets not updating GUI in published mode only
strumshot replied to strumshot's topic in Modder Support
Actually I just checked and I didn't paste that part in here, I do indeed do a check... public boolean onBlockActivated(World world, int x, int y, int z, EntityPlayer player, int p_149727_6_, float p_149727_7_, float p_149727_8_, float p_149727_9_) { if (world.isRemote) { return true; } else { IInventory iinventory = (TileEntityShopChest)world.getTileEntity(x, y, z); TileEntity tileEntity = world.getTileEntity(x, y, z); if (tileEntity == null || player.isSneaking()|| !(tileEntity instanceof TileEntityShopChest)) { return false; } TileEntityShopChest chest = (TileEntityShopChest) tileEntity; if (iinventory != null) { //if owner, open owner gui, id = 0? if (chest.isOwnedBy(player)) { FMLNetworkHandler.openGui(player, IShop.INSTANCE, 0, world, x, y, z); IShop.network.sendToServer(new RequestCost()); } else { FMLNetworkHandler.openGui(player, IShop.INSTANCE, 1, world, x, y, z); IShop.network.sendToServer(new RequestCost()); } //else open shopper gui, id = 1? } return true; } } -
[Solved] Packets not updating GUI in published mode only
strumshot replied to strumshot's topic in Modder Support
After enlisting a friend to assist me in debugging, I have found that RequestCost() ONLY is throwing exception: Every other packet I registered is executing perfectly. -
[Solved] Packets not updating GUI in published mode only
strumshot replied to strumshot's topic in Modder Support
Am I registering these correctly? Are the index numbers supposed to be unique per side or something? network = NetworkRegistry.INSTANCE.newSimpleChannel("shopChannel"); network.registerMessage(ChangeCostUp.Handler.class, ChangeCostUp.class, 0, Side.SERVER); network.registerMessage(ChangeCostDown.Handler.class, ChangeCostDown.class, 3, Side.SERVER); network.registerMessage(RequestCost.Handler.class, RequestCost.class, 2, Side.SERVER); network.registerMessage(SendCost.Handler.class, SendCost.class, 1, Side.CLIENT); -
I've written a set of guis that display a value stored in the container. While running from eclipse, every test I can do comes back successful. However after publishing to a full server-client setup (meaning not debug-able) ALMOST all of it works. The order of operation is as follows: Player opens gui -> request packet is sent -> server receives request -> server responds with packet containing value -> player receives packet and adjusts local value to be displayed in the gui. Again, while in testing, everything works perfectly. In full publish, I have evidence that the value is indeed stored properly on the server, and I have a way of basically forcing an update as an OP - for lack of better words. (There is another gui in which I can CHANGE the value, and after doing so, it all displays properly.) But any other player - or by logging out then back in - will display 0 in the client gui, despite the value on the server. I don't personally have a way to connect a debug client to a full release server, so I don't know how to find the problem! OnBlockActivate RequestCost packet (handled on server) SendCost packet (handled on client) What am I missing?
-
[SOLVED] Change NBT tag of ItemStack in container from GUI
strumshot replied to xilef11's topic in Modder Support
..to clarify my example, the client has a gui open, presses a button, and then this packet is sent to the server for processing, where the value is changed. I returned a packet to update the client from this, where you are only returning null. Guis sit on the client alone, and so will not be updating automatically. Again, my first question would be where are you watching the value to which nothing is happening? -
[SOLVED] Change NBT tag of ItemStack in container from GUI
strumshot replied to xilef11's topic in Modder Support
When you say nothing happens, are you logging the expected changes on the server or client? In my example, I send the updated info back to the client as the return to the Imessage (another packet) @Override public IMessage onMessage(ChangeCostUp message, MessageContext ctx) { EntityPlayer player = ctx.getServerHandler().playerEntity; ContainerShop container = (ContainerShop)player.openContainer; container.tileEntity.Cost++; if(container.tileEntity.Cost > 144) container.tileEntity.Cost = 144; player.worldObj.markBlockForUpdate(container.tileEntity.xCoord, container.tileEntity.yCoord, container.tileEntity.zCoord); container.tileEntity.markDirty(); return new SendCost((short) container.tileEntity.Cost); } whether you are updating a field (in my case) or NBT (yours) the situation should be the same. -
[Solved][1.7.10] Server and client gets out of sync
strumshot replied to EmperorZelos's topic in Modder Support
How important is it for them to be in sync? It sounds to me like the steam is something you render on the client but hold the data for on the server? Is it affecting gameplay? If its just rendering and has no effect on gameplay, I would basically expect them to be out of sync and you as the debugger are most likely the only one who will ever even realize they are out of sync, and only from watching so closely in your debugging. If it DOES effect gameplay and needs to be the same across all clients then you may have an issue and need to send a LOT of packets or follow the advice from above, but you may be able to at least make the logic make sense per player by handling as much as you can on the client, like you would particles or a gui, and most clients wouldn't know the difference. Maybe a more in depth description of what this "steam" is doing in your game and post some more code? -
Its a big city! (Sorry for not helping bit at the end of the day its the truth. Different buses for different destinations!)
-
KOTR Modpack 1.6.2 Entirely original map locking, non-op teleporting, fair tnt, and player gui-based shops. I present to you the first public publish of KOTR modpack! A very vanilla-like experience, simply extended for a more rewarding and in-depth SMP experience. IChunkLock Players can lock up to 4 64x64-block chunks and add other players to the approved list. Players not approved can not break or build in these areas. Players can toggle monster spawns in locked lands. OP's can lock lands, which are locked only to other creative-enabled players. Players are notified when entering and leaving different lands, denoted by "wilderness" (traditional map rules) "foreign lands" (locked lands that the player does not have access to) "safe" (lands with monster spawns turned off) Foreign players can not activate items or redstone by hand, but will trigger floor plates and trip-wires, for example. Chunklock commands /chunklock (/cl) /chunklock off /chunklock add <player> /chunklock remove <player> /chunkinfo /spawnlock (/sl) Fair TNT TNT will only explode in locked lands when manually lit by an approved player. TNT exploding in the wilderness will destroy blocks in locked lands if the blast radius reaches, so be careful building at locked-land perimeters. ITeleLink Players can craft "Mark Books" with a book and an eye of ender. "Using" a mark book will mark the book with coordinates of the player at the time of marking, making a "Marked Book" and the player will be prompted to name this location. Marked books can be placed in a valid "teleporter structure" for permanent usage by any player, even in foreign lands. "Using" a marked book will remove one (of ten) "Mark Papers" which can be used for immediate personal teleportation from anywhere, and the paper is consumed upon use. Teleporter structures consist of two empty blocks the player can walk into (surrounded by solid blocks on the left, right, back, and top) with a "teleporter core" below them that the player can stand on, as well as an item frame on the left-front face of the structure at eye-height, where the Marked Book can be placed. (a link to a simple visual guide is provided with the download) a "Teleporter Core" is crafted using 8 emeralds surrounding one redstone dust. These teleport options in conjunction with ChunkLock provide many options for player travel, such as pre-building teleporter banks in OP-locked lands, placing teleporter structures in player-locked areas providing simple, permanent travel to favorite locations, and using/giving Mark Papers to other players for single-sue teleportation to player bases, shops, etc. *Marking is not possible in foreign lands IShop IShop is operated by a "Shop Chest" block, which is crafted like a normal chest, but with one "Coin" in the center. Coins are dropped randomly from monsters when killed by a player, and are occasionally offered as trade for gold bars by villagers at a varying rate. Shop Chests can be used by any shopper, even in foreign lands. Each shop chest has one price (in coin) for all items sold within, which can be set only by the owner, via the GUI opened by using the block. Each shop chest has 12 shop slots and 27 bank slots which hold the payments received. Shop chests can be fully automated via hoppers/carts. The top and sides will fill the shop slots, while the bottom will extract the coin in the bank. *There are great opportunities for vibrant player economies via the marriage of these three mods. Lock lands, build a store-front, stock the shop and manage your bank automatically, and give away books or papers marked to other players. (Shop blocks can have a 0-price for free items.) *There are also great server-design/OP build opportunities through locking the lands to OP and building public teleporter structures. *There are also great PvP opportunities as TNT can breach a perimeter but not grief, and redstone traps and contraptions actually work! (Also, you may notice Factions are under development!) The modpack, changelog, and simple visual guide can be downloaded here. *It is highly recommended to read the changelog, view the guide, and only use the latest version. Please post any bugs/issues/questions/comments here!
-
Dynamically-created items: item graphics and names
strumshot replied to Feldherren's topic in Modder Support
I'm not entirely sure your approach, but you may be able to utilize @Override public String getItemStackDisplayName(ItemStack stack){ return "whatever""; } Or an equivalent... -
What version of Forge are you using? Also, don't be afraid to use the code tag on your posts... its the # button above. code here
-
Also I believe the files themselves have to be "powers of 2", and are default 256x256. Yours is wider than this, did you try 512x512?
-
You are extending GuiContainer, set it to your size in your initialization. public FCGuidePage5(World world, int i, int j, int k, EntityPlayer entity) { super(new FCGuiContainer((EntityPlayer)entity)); this.xSize=315; this.ySize=195; }
-
Manipulating TNT Explosions, Explosion Events
strumshot replied to strumshot's topic in Modder Support
Edit: Sorry, be sure to put if(!event.world.isRemote){ in your PlayerInteractEvent check. The check should actually look as follows. if(!event.world.isRemote){ if(event.entityPlayer.inventory.mainInventory[event.entityPlayer.inventory.currentItem].getItem() == Item.getItemFromBlock(Blocks.tnt)){ int i = event.face; int x = event.x; int y = event.y; int z = event.z; boolean can = false; // bottom top north south west east if(i == 0){ y--; } else if(i == 1){ y++; } else if(i == 2){ z--; } else if(i == 3){ z++; } else if(i == 4){ x--; } else if(i == 5){ x++; } if(event.world.isAirBlock(x, y, z)) can = true; List l = event.world.getEntitiesWithinAABB( Entity.class, AxisAlignedBB.getBoundingBox( (double) x, (double) y, (double) z, (double) x + 1.0d, (double) y + 1.0d, (double) z + 1.0d)); if(l.size() > 0) can = false; if(can){ event.world.setBlock(x, y, z, IFaction.NewTNT); event.world.notifyBlockOfNeighborChange(x, y, z, IFaction.NewTNT); } event.setCanceled(true); } -
Manipulating TNT Explosions, Explosion Events
strumshot replied to strumshot's topic in Modder Support
Note: to use a clone of vanilla tnt, you will have to create and register a new entity for the tnt, which can be cloned from EntityTNTPrimed (notice it extends the vanilla): public class NewTNTPrimed extends EntityTNTPrimed { /** How long the fuse is */ public int fuse; private EntityLivingBase tntPlacedBy; public NewTNTPrimed(World p_i1729_1_) { super(p_i1729_1_); this.preventEntitySpawning = true; this.setSize(0.98F, 0.98F); this.yOffset = this.height / 2.0F; this.fuse = 80; } public NewTNTPrimed(World p_i1730_1_, double p_i1730_2_, double p_i1730_4_, double p_i1730_6_, EntityLivingBase p_i1730_8_) { this(p_i1730_1_); this.setPosition(p_i1730_2_, p_i1730_4_, p_i1730_6_); float f = (float)(Math.random() * Math.PI * 2.0D); this.motionX = (double)(-((float)Math.sin((double)f)) * 0.02F); this.motionY = 0.20000000298023224D; this.motionZ = (double)(-((float)Math.cos((double)f)) * 0.02F); this.fuse = 80; this.prevPosX = p_i1730_2_; this.prevPosY = p_i1730_4_; this.prevPosZ = p_i1730_6_; this.tntPlacedBy = p_i1730_8_; } protected void entityInit() {} /** * returns if this entity triggers Block.onEntityWalking on the blocks they walk on. used for spiders and wolves to * prevent them from trampling crops */ protected boolean canTriggerWalking() { return false; } /** * Returns true if other Entities should be prevented from moving through this Entity. */ public boolean canBeCollidedWith() { return !this.isDead; } /** * Called to update the entity's position/logic. */ public void onUpdate() { this.prevPosX = this.posX; this.prevPosY = this.posY; this.prevPosZ = this.posZ; this.motionY -= 0.03999999910593033D; this.moveEntity(this.motionX, this.motionY, this.motionZ); this.motionX *= 0.9800000190734863D; this.motionY *= 0.9800000190734863D; this.motionZ *= 0.9800000190734863D; if (this.onGround) { this.motionX *= 0.699999988079071D; this.motionZ *= 0.699999988079071D; this.motionY *= -0.5D; } if (this.fuse-- <= 0) { this.setDead(); if (!this.worldObj.isRemote) { if(Events.onTNTExplode(this, (EntityPlayer)this.tntPlacedBy)) this.explode(); } } else { this.worldObj.spawnParticle("smoke", this.posX, this.posY + 0.5D, this.posZ, 0.0D, 0.0D, 0.0D); } } private void explode() { float f = 4.0F; this.worldObj.createExplosion(this, this.posX, this.posY, this.posZ, f, true); } /** * (abstract) Protected helper method to write subclass entity data to NBT. */ protected void writeEntityToNBT(NBTTagCompound p_70014_1_) { p_70014_1_.setByte("NFuse", (byte)this.fuse); } /** * (abstract) Protected helper method to read subclass entity data from NBT. */ protected void readEntityFromNBT(NBTTagCompound p_70037_1_) { this.fuse = p_70037_1_.getByte("NFuse"); } @SideOnly(Side.CLIENT) public float getShadowSize() { return 0.0F; } /** * returns null or the entityliving it was placed or ignited by */ public EntityLivingBase getTntPlacedBy() { return this.tntPlacedBy; } } take note of this line: if(Events.onTNTExplode(this, (EntityPlayer)this.tntPlacedBy)) this.explode(); ...it will let you create an explosion event handler as such: /** * Fired just before a tnt explosion * @param tnt * @param player = null often! be careful! * @return false will cancel the explosion */ public static boolean onTNTExplode(NewTNTPrimed tnt, EntityPlayer player) { return true; } Also, your tnt may not render correctly after lighting it. Be sure to register the renderer in your proxy! I suggest using the same renderer as vanilla tnt. RenderingRegistry.registerEntityRenderingHandler(NewTNTPrimed.class, new RenderTNTPrimed()); -
Due to the popularity of the question on how to stop or manipulate explosions without needing a core mod, I decided to tackle/post the following. What I've illustrated here is how to silently replace tnt with custom tnt, with the players being none-the-wiser. This allows you to write a custom tnt that looks/feels just like tnt, but you can override methods and add custom fields/methods/etc, giving you complete manipulation of tnt on your server. Just replace bedrock in the following example with your custom tnt and voila! import java.util.List; import net.minecraft.block.Block; import net.minecraft.entity.Entity; import net.minecraft.entity.item.EntityItemFrame; import net.minecraft.init.Blocks; import net.minecraft.item.Item; import net.minecraft.item.ItemBlock; import net.minecraft.util.AxisAlignedBB; import net.minecraftforge.common.util.ForgeDirection; import net.minecraftforge.event.entity.player.PlayerInteractEvent; import cpw.mods.fml.common.eventhandler.SubscribeEvent; public class BuildEventHook { @SubscribeEvent public void onClick(PlayerInteractEvent event) { if (event.action == event.action.RIGHT_CLICK_BLOCK) { if(event.entityPlayer.inventory.mainInventory[event.entityPlayer.inventory.currentItem].getItem() == Item.getItemFromBlock(Blocks.tnt)){ int i = event.face; int x = event.x; int y = event.y; int z = event.z; boolean can = false; // bottom top north south west east if(i == 0){ y--; } else if(i == 1){ y++; } else if(i == 2){ z--; } else if(i == 3){ z++; } else if(i == 4){ x--; } else if(i == 5){ x++; } if(event.world.isAirBlock(x, y, z)) can = true; List l = event.world.getEntitiesWithinAABB( Entity.class, AxisAlignedBB.getBoundingBox( (double) x, (double) y, (double) z, (double) x + 1.0d, (double) y + 1.0d, (double) z + 1.0d)); if(l.size() > 0) can = false; if(can){ event.world.setBlock(x, y, z, Blocks.bedrock); } event.setCanceled(true); } } } }
-
You'll have to make a method in your proxy for every specific screen to make this work. If you already have a GuiHandler it's much easier to just reuse that. I just did this, and found it easiest to make a GuiScreen class, borrow some methods from GuiContainer, and make an empty container class for the server side, so it all handles well and I can still send packets and whatnot back and forth easily. *code not meant for direct copy-paste @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { return new FactionContainer(); } @Override public Object getClientGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { return new GuiFaction(); } @SideOnly(Side.CLIENT) public class GuiFaction extends GuiScreen { protected void drawGuiContainerBackgroundLayer(float p_146976_1_, int p_146976_2_, int p_146976_3_) { mc.renderEngine.bindTexture(field_147085_u); GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); int x = (width - xSize) / 2; int y = (height - ySize) / 2; this.drawTexturedModalRect(x, y, 0, 0, xSize, ySize); } @Override /** * Draws the screen and all the components in it. */ public void drawScreen(int p_73863_1_, int p_73863_2_, float p_73863_3_) { this.drawDefaultBackground(); int k = this.guiLeft; int l = this.guiTop; this.drawGuiContainerBackgroundLayer(p_73863_3_, p_73863_1_, p_73863_2_); GL11.glDisable(GL12.GL_RESCALE_NORMAL); RenderHelper.disableStandardItemLighting(); GL11.glDisable(GL11.GL_LIGHTING); GL11.glDisable(GL11.GL_DEPTH_TEST); super.drawScreen(p_73863_1_, p_73863_2_, p_73863_3_); } public class FactionContainer extends Container { @Override public boolean canInteractWith(EntityPlayer p_75145_1_) { // TODO Auto-generated method stub return true; } } Edit: forgot these: FMLNetworkHandler.openGui(player, IFaction.INSTANCE, 0, world, x, y, z); NetworkRegistry.INSTANCE.registerGuiHandler(IFaction.INSTANCE, new FactionGuiHandler());