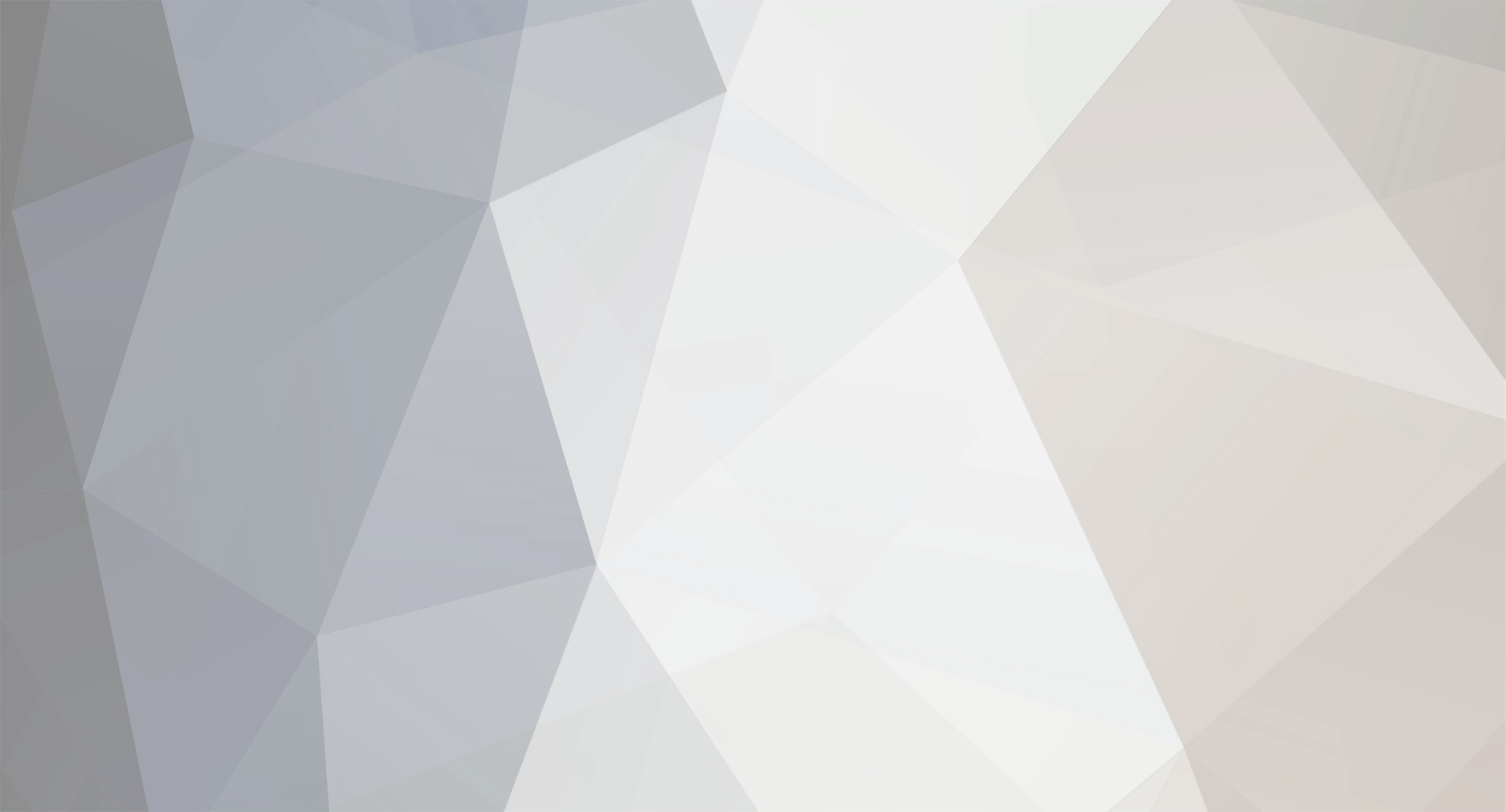
rypofalem
Members-
Posts
20 -
Joined
-
Last visited
Everything posted by rypofalem
-
Use the debugger to see how and where they are set to zero or where they might be set higher but were not.
-
You don't want to delete the constructor Try printing moveStrafing and moveForward, I suspect those values are zero. Better yet, use the debugger which should show you how the values are returning whatever.
-
[solved][1.7.10] Some explosions not animating
rypofalem replied to rypofalem's topic in Modder Support
Thanks for the reply! It makes me feel great seeing someone trying to help and I really appreciate it. You are right about the packet sending thing. It happens when when you use World.createExplosion(), though it doesn't happen if I copy the same code. Really strange but I do notice something I didn't notice before in teh source code Maybe that's part of what is unfinished? I guess if I am going to do custom explosions I will have to add it myself. Now I know what I need to learn. This is progress! Keeping each players egg's separate is certainly on my todo list but thanks for catching that! Edit: to clarify, this works as intended. public void explode(){ if(!this.worldObj.isRemote && !isDead){ worldObj.createExplosion(this, posX, posY, posZ, explosionSize, true); this.setDead(); } } but this does not send packets to client. public void explode(){ if(!this.worldObj.isRemote && !isDead){ Explosion explosion = new Explosion(this.worldObj, this, posX, posY, posZ, explosionSize); explosion.isFlaming = false; explosion.isSmoking = true; explosion.doExplosionA(); explosion.doExplosionB(true); this.setDead(); } } -
[solved][1.7.10] Some explosions not animating
rypofalem replied to rypofalem's topic in Modder Support
This is wearing on me. I just can't leave it alone! I hate that half-assed solution I posted (in any case it doesn't work in multiplayer, it spawns the explosion particles at the egg's initial position, right in the player's head). Any ideas on how to reference the most recent projectile that is relevant for client/server? lastEgg, as it stands, always references an EntityThrowable with a serverside World object even if on the client version of things. -
Yeah, it's not an issue with isMovementBlocked, I think. As far as I can tell that has nothing to do with the player controling the horse, but with the AI controlling the horse. Since you don't want the bicycle to control itself ever (I assume) you should just have it control true.
-
Container item doesn't display 'lore' after being crafted with
rypofalem replied to ilan321's topic in Modder Support
Everything's possible. I'll look for a simple solution when I get home again though. -
Giving a player a diamond if they eat a food?
rypofalem replied to KingYoshiYT's topic in Modder Support
If you can't tell that by glancing at that I would advise that you search online for java tutorials. There's lots of free resources available. -
Giving a player a diamond if they eat a food?
rypofalem replied to KingYoshiYT's topic in Modder Support
@Override protected void onFoodEaten(ItemStack item, World world, EntityPlayer player) { super.onFoodEaten(item, world, player); if(!world.isRemote){ player.dropItem(Items.diamond, 1); MinecraftServer.getServer().getConfigurationManager().sendChatMsg(new ChatComponentText(player.getDisplayName() + "Successfully did the thing!")); } } -
Container item doesn't display 'lore' after being crafted with
rypofalem replied to ilan321's topic in Modder Support
I see, so you're telling it function like a bucket. But doesn't a bucket full of milk in a crafting recipe return an empty bucket? I think that is what is happening to your stone, you change the lore in the crafting table but since it's a container the crafting thing returns a new instance of the stone instead of one with lore. Maybe instead of having the stone be a container item, check to see if the stone was used in the recipe and if it was insert a new stone into the player's inventory with with one less use. I tried that myself with your code and it seemed to work fine. edit: I wanted to avoid posting code but I may as well before I remove it. It's better if you try to follow the description and try to implement it yourself but if you're stumped on that: @SubscribeEvent public void onItemCraftEvent(ItemCraftedEvent e) { for (int i = 0; i < e.craftMatrix.getSizeInventory(); i++) { if (e.craftMatrix.getStackInSlot(i) != null) { if (e.craftMatrix.getStackInSlot(i).getItem() == SuperCool.superItem) //my item { if (e.craftMatrix.getStackInSlot(i).stackTagCompound != null) { e.craftMatrix.getStackInSlot(i).stackTagCompound.setInteger("uses", (e.craftMatrix.getStackInSlot(i).stackTagCompound.getInteger("uses") - 1)); ItemStack superItem = e.craftMatrix.getStackInSlot(i); //stores the item with lore for future use if (e.craftMatrix.getStackInSlot(i).stackTagCompound.getInteger("uses") < 1) { e.craftMatrix.setInventorySlotContents(i, null); }else{ InventoryPlayer inv = e.player.inventory; //no need for this, just wanted code to be more readable boolean placedItem = false; //finds first empty slot, inserts the item with lore. for (int j = 0; j < inv.getSizeInventory() && !placedItem; j++){ if(inv.getStackInSlot(j) == null){ inv.addItemStackToInventory(superItem); placedItem = true; e.player.inventory = inv; } } if(!placedItem) e.player.dropItem(superItem.getItem(), 1); //if all slots were full, drops item with lore on the ground } } } } } } -
Giving a player a diamond if they eat a food?
rypofalem replied to KingYoshiYT's topic in Modder Support
Have poop extend ItemFood. Override OnFoodEaten and put these two lines somewhere in that method. Remember that both should only be called serverside. //Drops a diamond at player's position player.dropItem(Items.diamond, 1); //Broadcasts the message MinecraftServer.getServer().getConfigurationManager().sendChatMsg(new ChatComponentText(player.getDisplayName() + " successfully completed the poop eating challenge")); -
[solved][1.7.10] Some explosions not animating
rypofalem replied to rypofalem's topic in Modder Support
Alright I fixed it. For those wondering since the client was getting the server version of lastEgg (the EntityItem that explodes), when I told it to explode like this Explosion explosion = new Explosion(lastEgg.worldObj, lastEgg, x, y, z, lastEgg.explosionSize); it would always use the server version of worldObj. Instead I made a new explosion with the player's world object and it worked fine. I need to make a note that the player and entity could unexpectedly be in different worlds and account for that but for now it works. Explosion explosion = new Expolosion(living.worldObj, lastEgg, x, y, z, lastEgg.explosionSize); -
[solved][1.7.10] Some explosions not animating
rypofalem replied to rypofalem's topic in Modder Support
After more debugging I think I am finally getting close to the answer. When I take the if statement out of onEntity swing and replace it with a print statement to see which side is calling it like so. @Override public boolean onEntitySwing(EntityLivingBase living, ItemStack stack) { super.onEntitySwing(living, stack); String side = living.worldObj != null && living.worldObj.isRemote ? "Client": "Server"; System.out.println("exploding" + " on " + side); lastEgg.explode(); return false; } And then insert a similar print statement my slightly altered explosion I get: It seems to tell the server version of lastEgg to explode, regardless of if the client or server is saying it. The lastEgg reference is being updated to the server version I guess. How might I reference the client version of the entity so I can explode that for the clientside? I recognize, of course, at this point I could get the coordinates of lastEgg and have the client spawn the particles I want it to but I am curious if it is possible to get the client version of the entity. -
Container item doesn't display 'lore' after being crafted with
rypofalem replied to ilan321's topic in Modder Support
You are using the stone to craft an item, how are you getting the stone back? -
You print it just before the return statement. Also, I think you need to change != to ==. this.riddenByEntity != null would return true when you tried to ride it. edit: nevermind, I think that method pertains to the horse AI movement being blocked. It is good that you got rid of the need for a saddle in moveEntityWithHeading though.
-
Did you put a saddle on the bicycle? Print the result of isMovementBlocked to make sure none of those conditions are impairing movement
-
As someone new to moding and programming in general I'd be interested in hearing about your solution.
-
Making my entity drop an item every 10 minutes?
rypofalem replied to KingYoshiYT's topic in Modder Support
You can override onUpdate in your entity class. It's called once per tick while your entity exists so just count the ticks that way. When the counter reaches enough ticks spawn an item at his position. Edit: I will demonstrate when I get home if someone else has not already helped. I'm on my phone atm though. -
Hi. I'm working on an item that shoots projectiles. The projectiles explode when they hit a block/entity, when a timer expires, or when the player swings his held item. The issue I am having is that although all three call the same explosion method, the explosion doesn't render when the player swings the held item. It does, however, destroy blocks, damage entities and play sound as intended. Youtube video to demonstrate: Here are the item and throwable entity classes. It normally calls a custom explosion method but I've replaced it with vanillaExplode for debugging purposes. Sorry in advance that this isn't in a code block, the code button wasn't working and I don't know what the tag is. public class SuperItem extends Item { @SideOnly(Side.CLIENT) protected IIcon itemIcon; private EntitySuperEgg lastEgg; int i= 0; public SuperItem() { this.setCreativeTab(SuperCool.tabSuperCool); setMaxStackSize(1); } @Override public ItemStack onItemRightClick(ItemStack held, World world, EntityPlayer player) { if(player.capabilities.isCreativeMode||player.inventory.consumeInventoryItem(SuperCool.superEgg)) { world.playSoundAtEntity(player, "random.bow", 0.5F, 0.4F / (itemRand.nextFloat() * 0.4F + 0.8F)); lastEgg = new EntitySuperEgg(world, player); if (!world.isRemote) { world.spawnEntityInWorld(lastEgg); } } return held; } @Override public boolean onEntitySwing(EntityLivingBase living, ItemStack stack) { super.onEntitySwing(living, stack); if( living instanceof EntityPlayer && lastEgg!= null && !lastEgg.isDead){ lastEgg.vanillaExplode(); } return false; } } public class EntitySuperEgg extends EntityThrowable { private float explosionSize = 1.5F; private float explosionDamage = 25.0F; private float explosionDamageSize = 8.0F; private int ticks =0; public EntitySuperEgg(World world) { super(world); } public EntitySuperEgg(World world, EntityLivingBase thrower) { super(world, thrower); } public EntitySuperEgg(World world, double par2, double par4, double par6) { super(world, par2, par4, par6); } @Override protected void onImpact(MovingObjectPosition pos){ explode(); } public void explode(){ double x = this.posX; double y = this.posY; double z = this.posZ; SuperEggExplosion explosion = new SuperEggExplosion(this.worldObj, this, x, y, z, explosionSize, explosionDamage, explosionDamageSize ); explosion.isFlaming = false; explosion.isSmoking = true; if(!this.worldObj.isRemote){ explosion.doExplosionA(); } explosion.doExplosionB(true); this.setDead(); } public void vanillaExplode(){ double x = this.posX; double y = this.posY; double z = this.posZ; Explosion explosion = new Explosion(this.worldObj, this, x, y, z, explosionSize); explosion.isFlaming = false; explosion.isSmoking = true; if(!this.worldObj.isRemote){ explosion.doExplosionA(); } explosion.doExplosionB(true); this.setDead(); } @Override public void onUpdate(){ super.onUpdate(); if(++ticks > 100){ vanillaExplode(); } } @Override protected float getGravityVelocity() { return 0.02F; } }
-
if(!this.worldObj.isRemote){ this.setDead(); if(!this.worldObj.isRemote){ this.worldObj.createExplosion((Entity) null, this.posX, this.posY, this.posZ, 4.5F, true); } } I ran that code and it produced perfectly valid explosions that damaged the player. So I think something else is preventing your character from taking damage. This may sound silly but I must ask. Are you testing this in creative mode? If not I would start looking at things like blast resistance or other parts of your mod.