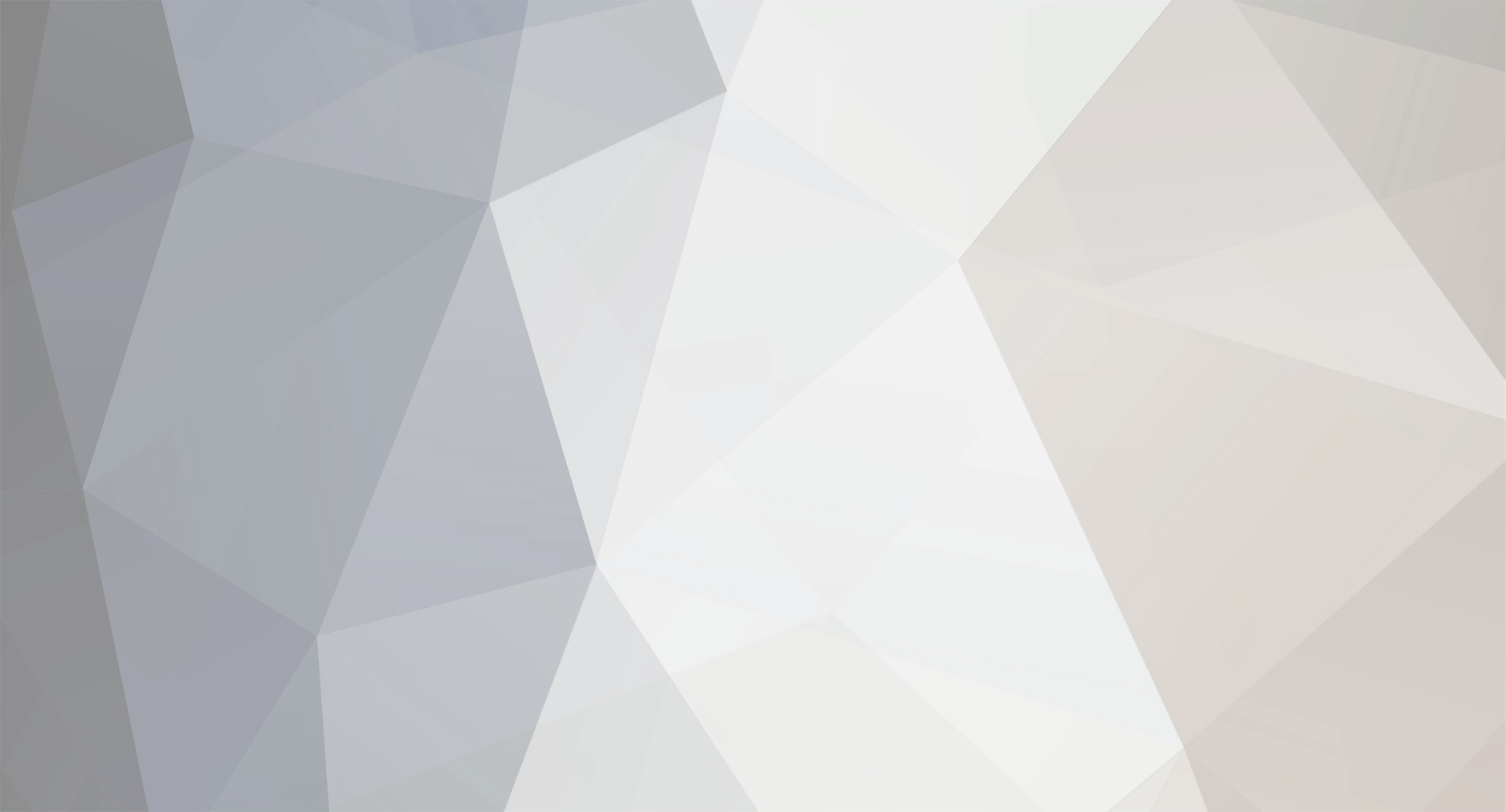
Trese759
Members-
Posts
16 -
Joined
-
Last visited
Everything posted by Trese759
-
Any idea?
-
Grazie, ho rimosso quella stringa, riposto il TileEntity completo e la Gui e Contaire class. TileEntity public class TileEntitySuperGlueFurnace extends TileEntity implements IInventory { private ItemStack slots[]; public int dualPower; public int dualCookTime; public static final int maxPower = 10000; public static final int mashingSpeed = 100; private static final int[] slots_top = new int[] {0, 1}; private static final int[] slots_bottom = new int[] {3}; private static final int[] slots_side = new int[] {2}; private String customName; public TileEntitySuperGlueFurnace() { slots = new ItemStack[4]; } @Override public int getSizeInventory() { return slots.length; } @Override public ItemStack getStackInSlot(int i) { return slots[i]; } @Override public ItemStack getStackInSlotOnClosing(int i) { if (slots[i] != null) { ItemStack itemstack = slots[i]; slots[i] = null; return itemstack; }else{ return null; } } @Override public void setInventorySlotContents(int i, ItemStack itemstack) { slots[i] = itemstack; if (itemstack != null && itemstack.stackSize > getInventoryStackLimit()) { itemstack.stackSize = getInventoryStackLimit(); } } public int[] getAccessibleSlotsFromSide(int i) { return i == 0 ? slots_bottom : (i == 1 ? slots_top : slots_side); } @Override public int getInventoryStackLimit() { return 64; } @Override public boolean isUseableByPlayer(EntityPlayer player) { return player.getDistanceSq((double)xCoord + 0.5D, (double)yCoord + 0.5D, (double)zCoord + 0.5D) <= 64; } public void openInventory() {} public void closeInventory() {} @Override public boolean isItemValidForSlot(int i, ItemStack itemstack) { return i == 2 ? false : (i == 1 ? hasItemPower(itemstack) : true); } public boolean hasItemPower(ItemStack itemstack) { return getItemPower(itemstack) > 0; } private static int getItemPower (ItemStack itemstack) { if (itemstack == null) { return 0; }else{ Item item = itemstack.getItem(); if (item == Megakron.tackyTyre) return 500; return 0; } } @Override public ItemStack decrStackSize(int i, int j) { if (this.slots[i] != null) { ItemStack itemstack; if (this.slots[i].stackSize <= j) { itemstack = this.slots[i]; this.slots[i] = null; return itemstack; } else { itemstack = this.slots[i].splitStack(j); if (this.slots[i].stackSize == 0) { this.slots[i] = null; } return itemstack; } } else { return null; } } public void readFromNBT (NBTTagCompound nbt) { super.readFromNBT(nbt); NBTTagList list = nbt.getTagList("Items", 10); slots = new ItemStack[getSizeInventory()]; for (int i = 0; i < list.tagCount(); i++) { NBTTagCompound nbt1 = (NBTTagCompound)list.getCompoundTagAt(i); byte b0 = nbt1.getByte("Slot"); if (b0 >= 0 && b0 < slots.length) { slots[b0] = ItemStack.loadItemStackFromNBT(nbt1); } } dualPower = nbt.getShort("PowerTime"); dualCookTime = nbt.getShort("CookTime"); } public void writeToNBT(NBTTagCompound nbt) { super.writeToNBT(nbt); nbt.setShort("PowerTime", (short)dualPower); nbt.setShort("CookTime", (short)dualCookTime); NBTTagList list = new NBTTagList(); for (int i = 0; i < slots.length; i++) { if (slots[i] != null) { NBTTagCompound nbt1 = new NBTTagCompound(); nbt1.setByte("Slot", (byte)i); slots[i].writeToNBT(nbt1); list.appendTag(nbt1); } } nbt.setTag("Items", list); } @Override public String getInventoryName() { return ""; } public boolean canInsertItem(int var1, ItemStack itemstack, int var3) { return this.isItemValidForSlot(var1, itemstack); } public boolean canExtractItem(int i, ItemStack itemstack, int j) { return j != 0 || i != 1 || itemstack.getItem() == Items.bucket; } @Override public boolean hasCustomInventoryName() { return this.customName != null && this.customName.length() > 0; } public int getMasherProgressScaled(int i) { return (dualCookTime * i) / this.mashingSpeed; } public int getPowerRemainingScaled(int i) { return (dualPower * i) / maxPower; } private boolean canMash() { if (slots[0] == null || slots[1] == null) { return false; } ItemStack itemstack = SuperGlueFurnaceRecipes.getMashingResult(slots[0].getItem(), slots[1].getItem()); if (itemstack == null) { return false; } if (slots[3] == null) { return true; } if (!slots[3].isItemEqual(itemstack)) { return false; } if (slots[3].stackSize < getInventoryStackLimit() && slots[3].stackSize < slots[3].getMaxStackSize()) { return true; }else{ return slots[3].stackSize < itemstack.getMaxStackSize(); } } private void mashItem() { if (canMash()) { ItemStack itemstack = SuperGlueFurnaceRecipes.getMashingResult(slots[0].getItem(), slots[1].getItem()); if (slots[3] == null) { slots[3] = itemstack.copy(); }else if (slots[3].isItemEqual(itemstack)) { slots[3].stackSize += itemstack.stackSize; } for (int i = 0; i < 2; i++) { if (slots[i].stackSize <= 0) { slots[i] = new ItemStack(slots[i].getItem().setFull3D()); }else{ slots[i].stackSize--; } if (slots[i].stackSize <= 0){ slots[i] = null; } } } } public boolean hasPower() { return dualPower > 0; } public boolean isMashing() { return this.dualCookTime > 0; } public void updateEntity() { boolean flag = this.hasPower(); boolean flag1= false; if(hasPower() && this.isMashing()) { this.dualPower--; } if(!worldObj.isRemote) { if (this.hasItemPower(this.slots[2]) && this.dualPower < (this.maxPower - this.getItemPower(this.slots[2]))) { this.dualPower += getItemPower(this.slots[2]); if(this.slots[2] != null) { flag1 = true; this.slots[2].stackSize--; if(this.slots[2].stackSize == 0) { this.slots[2] = this.slots[2].getItem().getContainerItem(this.slots[2]); } } } if (hasPower() && canMash()) { dualCookTime++; if (this.dualCookTime == this.mashingSpeed) { this.dualCookTime = 0; this.mashItem(); flag1 = true; } }else{ dualCookTime = 0; } if (flag != this.isMashing()) { flag1 = true; SuperGlueFurnace.updateBlockState(this.isMashing(), this.worldObj, this.xCoord, this.yCoord, this.zCoord); } } if (flag1) { this.markDirty(); } } } Gui public class GuiSuperGlueFurnace extends GuiContainer { private ResourceLocation texture = new ResourceLocation("megakron:textures/gui/superGlueFurnace.png"); private TileEntitySuperGlueFurnace superGlueFurnace; public GuiSuperGlueFurnace(InventoryPlayer invPlayer, TileEntitySuperGlueFurnace teSuperGlueFurnace) { super(new ContainerSuperGlueFurnace(invPlayer, teSuperGlueFurnace)); superGlueFurnace = teSuperGlueFurnace; } protected void drawGuiContainerForegroundLayer(int i, int j) { String name = this.superGlueFurnace.hasCustomInventoryName() ? this.superGlueFurnace.getInventoryName() : I18n.format(this.superGlueFurnace.getInventoryName()); this.fontRendererObj.drawString(name, this.xSize / 2 - this.fontRendererObj.getStringWidth(name) / 2, 6, 4210752); this.fontRendererObj.drawString(I18n.format(""), 8, this.ySize - 96 + 5, 4210752); } @Override protected void drawGuiContainerBackgroundLayer(float var1, int var2, int var3) { GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); Minecraft.getMinecraft().getTextureManager().bindTexture(texture); drawTexturedModalRect(guiLeft, guiTop, 0, 0, xSize, ySize); if (superGlueFurnace.hasPower()) { int i1 = superGlueFurnace.getPowerRemainingScaled(45); drawTexturedModalRect(guiLeft + 8, guiTop + 56 - i1, 176, 50 - i1, 2, i1); } int i1 = superGlueFurnace.getMasherProgressScaled(24); drawTexturedModalRect(guiLeft + 77, guiTop + 16, 176, 52, i1 + 1, 54); } } ontainer public class ContainerSuperGlueFurnace extends Container { private TileEntitySuperGlueFurnace superGlue; private int dualCookTime; private int dualPower; private int lastItemBurn; public ContainerSuperGlueFurnace(InventoryPlayer invPlayer, TileEntitySuperGlueFurnace teSuperGlueFurnace) { dualCookTime = 0; dualPower = 0; lastItemBurn = 0; superGlue = teSuperGlueFurnace; this.addSlotToContainer(new Slot(teSuperGlueFurnace, 0, 56, 17)); this.addSlotToContainer(new Slot(teSuperGlueFurnace, 1, 56, 53)); this.addSlotToContainer(new Slot(teSuperGlueFurnace, 2, 8, 61)); this.addSlotToContainer(new SlotSuperGlueFurnace(invPlayer.player, teSuperGlueFurnace, 3, 116, 35)); for(int i = 0; i < 3; i++) { for(int j = 0; j < 9; j++) { this.addSlotToContainer(new Slot(invPlayer, j + i * 9 + 9, 8 + j * 18, 84 + i * 18)); } } for(int i = 0; i < 9; i++) { this.addSlotToContainer(new Slot(invPlayer, i, 8 + i * 18, 142)); } } public void addCraftingToCrafters(ICrafting crafting) { super.addCraftingToCrafters(crafting); crafting.sendProgressBarUpdate(this, 0, this.superGlue.dualCookTime); crafting.sendProgressBarUpdate(this, 1, this.superGlue.dualPower); } public ItemStack transferStackInSlot(EntityPlayer par1EntityPlayer, int par2) { ItemStack itemstack = null; Slot slot = (Slot)this.inventorySlots.get(par2); if (slot != null && slot.getHasStack()) { ItemStack itemstack1 = slot.getStack(); itemstack = itemstack1.copy(); if (par2 == 2) { if (!this.mergeItemStack(itemstack1, 3, 39, true)) { return null; } slot.onSlotChange(itemstack1, itemstack); } else if (par2 != 1 && par2 != 0) { if (FurnaceRecipes.smelting().getSmeltingResult(itemstack1) != null) { if (!this.mergeItemStack(itemstack1, 0, 1, false)) { return null; } } else if (TileEntityFurnace.isItemFuel(itemstack1)) { if (!this.mergeItemStack(itemstack1, 1, 2, false)) { return null; } } else if (par2 >= 3 && par2 < 30) { if (!this.mergeItemStack(itemstack1, 30, 39, false)) { return null; } } else if (par2 >= 30 && par2 < 39 && !this.mergeItemStack(itemstack1, 3, 30, false)) { return null; } } else if (!this.mergeItemStack(itemstack1, 3, 39, false)) { return null; } if (itemstack1.stackSize == 0) { slot.putStack((ItemStack)null); } else { slot.onSlotChanged(); } if (itemstack1.stackSize == itemstack.stackSize) { return null; } slot.onPickupFromSlot(par1EntityPlayer, itemstack1); } return itemstack; } @Override public boolean canInteractWith(EntityPlayer player) { // TODO Auto-generated method stub return superGlue.isUseableByPlayer(player); } public void detectAndSendChanges() { super.detectAndSendChanges(); for (int i = 0; i < this.crafters.size(); ++i) { ICrafting icrafting = (ICrafting)this.crafters.get(i); if (this.dualCookTime != this.superGlue.dualCookTime) { icrafting.sendProgressBarUpdate(this, 0, this.superGlue.dualCookTime); } if (this.dualPower != this.superGlue.dualPower) { icrafting.sendProgressBarUpdate(this, 1, this.superGlue.dualPower); } } this.dualCookTime = this.superGlue.dualCookTime; this.dualPower = this.superGlue.dualPower; } @SideOnly(Side.CLIENT) public void updateProgressBar(int par1, int par2) { if (par1 == 0) { this.superGlue.dualCookTime = par2; } if (par1 == 1) { this.superGlue.dualPower = par2; } } }
-
Hi, when I try to take an item from inventory repositions it in the same slot not let me move. Where did I go wrong? I think there is a mistake in decrStackSize but can not find it. public class TileEntitySuperGlueFurnace extends TileEntity implements IInventory { private ItemStack slots[]; public int dualPower; public int dualCookTime; public static final int maxPower = 10000; public static final int mashingSpeed = 100; private static final int[] slots_top = new int[] {0, 1}; private static final int[] slots_bottom = new int[] {3}; private static final int[] slots_side = new int[] {2}; private String customName; public TileEntitySuperGlueFurnace() { slots = new ItemStack[4]; } @Override public int getSizeInventory() { return slots.length; } @Override public ItemStack getStackInSlot(int i) { return slots[i]; } @Override public ItemStack getStackInSlotOnClosing(int i) { if (slots[i] != null) { ItemStack itemstack = slots[i]; slots[i] = null; return itemstack; }else{ return null; } } @Override public void setInventorySlotContents(int i, ItemStack itemstack) { slots[i] = itemstack; if (itemstack != null && itemstack.stackSize > getInventoryStackLimit()) { itemstack.stackSize = getInventoryStackLimit(); } } public int[] getAccessibleSlotsFromSide(int i) { return i == 0 ? slots_bottom : (i == 1 ? slots_top : slots_side); } @Override public int getInventoryStackLimit() { return 64; } @Override public boolean isUseableByPlayer(EntityPlayer player) { if (worldObj.getTileEntity(xCoord, yCoord, zCoord) != this) { return false; }else{ return player.getDistanceSq((double)xCoord + 0.5D, (double)yCoord + 0.5D, (double)zCoord + 0.5D) <= 64; } } public void openInventory() {} public void closeInventory() {} @Override public boolean isItemValidForSlot(int i, ItemStack itemstack) { return i == 2 ? false : (i == 1 ? hasItemPower(itemstack) : true); } public boolean hasItemPower(ItemStack itemstack) { return getItemPower(itemstack) > 0; } private static int getItemPower (ItemStack itemstack) { if (itemstack == null) { return 0; }else{ Item item = itemstack.getItem(); if (item == Megakron.tackyTyre) return 500; return 0; } } @Override public ItemStack decrStackSize(int i, int j) { if (this.slots[i] != null) { ItemStack itemstack; if (this.slots[i].stackSize <= j) { itemstack = this.slots[i]; this.slots[i] = null; return itemstack; } else { itemstack = this.slots[i].splitStack(j); if (this.slots[i].stackSize == 0) { this.slots[i] = null; } return itemstack; } } else { return null; } } }
-
[1.7.2] ResourceLocation Problem (Techne Model)
Trese759 replied to Trese759's topic in Modder Support
I'm so sorry. package megakron.render; import org.lwjgl.opengl.GL11; import megakron.Megakron; import net.minecraft.client.renderer.tileentity.TileEntitySpecialRenderer; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.tileentity.TileEntity; import net.minecraftforge.client.IItemRenderer; import net.minecraftforge.client.MinecraftForgeClient; public class ItemRenderSuperWorkbench implements IItemRenderer { TileEntitySpecialRenderer render; private TileEntity entity; public ItemRenderSuperWorkbench(TileEntitySpecialRenderer render, TileEntity entity) { this.entity = entity; this.render = render; } @Override public boolean handleRenderType(ItemStack item, ItemRenderType type) { // TODO Auto-generated method stub return type != ItemRenderType.INVENTORY; } @Override public boolean shouldUseRenderHelper(ItemRenderType type, ItemStack item, ItemRendererHelper helper) { // TODO Auto-generated method stub return true; } @Override public void renderItem(ItemRenderType type, ItemStack item, Object... data) { if(type == IItemRenderer.ItemRenderType.ENTITY) { GL11.glTranslatef(-0.5F, 0.0F, -0.5F); this.render.renderTileEntityAt(this.entity, 0.0D, 0.0D, 0.0D, 0.0F); } } } -
[1.7.2] ResourceLocation Problem (Techne Model)
Trese759 replied to Trese759's topic in Modder Support
I already registered the render within the client proxy -
Thank you so much. Solved
-
This is the Main class: @Mod(modid = Megakron.MODID, name = Megakron.NAME, version = Megakron.VERSION) public class Megakron { //Name public static final String MODID = "megakron"; public static final String NAME = "MegaKron"; public static final String VERSION = "1.0"; public static final String CLIENT_PROXY = "megakron.ClientProxy"; public static final String COMMON_PROXY = "megakron.CommonProxy"; @Instance("Megakron") public static Megakron instance; @SidedProxy(clientSide = Megakron.CLIENT_PROXY, serverSide = Megakron.COMMON_PROXY) public static CommonProxy proxy; //Models public static Block superWorkbench; public static final int guiIDSuperWorkbench = 0; @EventHandler public void preInit(FMLPreInitializationEvent event) { //Models superWorkbench = new SuperWorkbench(Material.rock).setBlockName("SuperWorkbench").setCreativeTab(tabMK).setBlockTextureName(""); LanguageRegistry.addName(superWorkbench, "Super Workbench"); GameRegistry.registerBlock(superWorkbench, "SuperWorkbench"); } @EventHandler public void load(FMLInitializationEvent event) { //GuiHandller NetworkRegistry.INSTANCE.registerGuiHandler(instance, new GuiHandler()); }
-
[1.7.2] ResourceLocation Problem (Techne Model)
Trese759 replied to Trese759's topic in Modder Support
I forgot the "s" for texture. As for the model in the inventory how do I fix? -
[1.7.2] ResourceLocation Problem (Techne Model)
Trese759 replied to Trese759's topic in Modder Support
I tried without success, any other ideas? -
Hi, I have a problem with the ResourceLocation for a model created on techne. The model of Minecraft exists but can not recognize the texture, I also tried to make sure that the object is three-dimensional but also inventory them is completely invisible. How can I fix? Main @EventHandler public void preInit(FMLPreInitializationEvent event) { //Models superWorkbench = new SuperWorkbench(Material.rock).setBlockName("SuperWorkbench").setCreativeTab(tabMK).setBlockTextureName(""); LanguageRegistry.addName(superWorkbench, "Super Workbench"); GameRegistry.registerBlock(superWorkbench, "SuperWorkbench"); @EventHandler public void load(FMLInitializationEvent event) { //Renders proxy.registerRenderThing(); } SuperWorkbench package megakron.blocks; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import megakron.tileentity.TileEntitySuperWorkbench; import net.minecraft.block.BlockContainer; import net.minecraft.block.material.Material; import net.minecraft.client.renderer.texture.IIconRegister; import net.minecraft.tileentity.TileEntity; import net.minecraft.world.World; public class SuperWorkbench extends BlockContainer { public SuperWorkbench(Material material) { super(material); this.setHardness((float) 2.0); this.setResistance((float) 4.0); } public int getRenderType() { return -1; } public boolean isOpaqueCube() { return false; } public boolean renderAsNormalBlock() { return false; } @Override public TileEntity createNewTileEntity(World var1, int var2) { // TODO Auto-generated method stub return new TileEntitySuperWorkbench(); } @SideOnly(Side.CLIENT) public void registerBlockIcons(IIconRegister iconRegister) { this.blockIcon = iconRegister.registerIcon("megakron:SuperWorkbench"); } } ModelSuperWorkbench package megakron.models; import net.minecraft.client.model.ModelBase; import net.minecraft.client.model.ModelRenderer; import net.minecraft.entity.Entity; public class ModelSuperWorkbench extends ModelBase { //fields ModelRenderer Shape1; ModelRenderer Shape2; ModelRenderer Shape3; ModelRenderer Shape4; ModelRenderer Shape5; ModelRenderer Shape6; ModelRenderer Shape7; ModelRenderer Shape8; ModelRenderer Shape9; ModelRenderer Shape10; ModelRenderer Shape11; ModelRenderer Shape12; ModelRenderer Shape13; ModelRenderer Shape14; ModelRenderer Shape15; public ModelSuperWorkbench() { textureWidth = 64; textureHeight = 64; Shape1 = new ModelRenderer(this, 0, 0); Shape1.addBox(0F, 0F, 0F, 16, 7, 16); Shape1.setRotationPoint(-8F, 17F, -8F); Shape1.setTextureSize(64, 64); Shape1.mirror = true; setRotation(Shape1, 0F, 0F, 0F); Shape2 = new ModelRenderer(this, 0, 23); Shape2.addBox(0F, 0F, 0F, 14, 2, 14); Shape2.setRotationPoint(-7F, 15F, -7F); Shape2.setTextureSize(64, 64); Shape2.mirror = true; setRotation(Shape2, 0F, 0F, 0F); Shape3 = new ModelRenderer(this, 0, 0); Shape3.addBox(0F, 0F, 0F, 16, 7, 16); Shape3.setRotationPoint(-8F, 8F, -8F); Shape3.setTextureSize(64, 64); Shape3.mirror = true; setRotation(Shape3, 0F, 0F, 0F); Shape4 = new ModelRenderer(this, 0, 0); Shape4.addBox(0F, 0F, 0F, 2, 2, 2); Shape4.setRotationPoint(-8F, 15F, -8F); Shape4.setTextureSize(64, 64); Shape4.mirror = true; setRotation(Shape4, 0F, 0F, 0F); Shape5 = new ModelRenderer(this, 0, 0); Shape5.addBox(0F, 0F, 0F, 2, 2, 2); Shape5.setRotationPoint(-8F, 15F, 6F); Shape5.setTextureSize(64, 64); Shape5.mirror = true; setRotation(Shape5, 0F, 0F, 0F); Shape6 = new ModelRenderer(this, 0, 0); Shape6.addBox(0F, 0F, 0F, 2, 2, 2); Shape6.setRotationPoint(6F, 15F, -8F); Shape6.setTextureSize(64, 64); Shape6.mirror = true; setRotation(Shape6, 0F, 0F, 0F); Shape7 = new ModelRenderer(this, 0, 0); Shape7.addBox(0F, 0F, 0F, 2, 2, 2); Shape7.setRotationPoint(6F, 15F, 6F); Shape7.setTextureSize(64, 64); Shape7.mirror = true; setRotation(Shape7, 0F, 0F, 0F); Shape8 = new ModelRenderer(this, 0, 39); Shape8.addBox(0F, 0F, 0F, 16, 1, 1); Shape8.setRotationPoint(-8F, 7.5F, -8F); Shape8.setTextureSize(64, 64); Shape8.mirror = true; setRotation(Shape8, 0F, 0F, 0F); Shape9 = new ModelRenderer(this, 0, 39); Shape9.addBox(0F, 0F, 0F, 16, 1, 1); Shape9.setRotationPoint(-8F, 7.5F, -3F); Shape9.setTextureSize(64, 64); Shape9.mirror = true; setRotation(Shape9, 0F, 0F, 0F); Shape10 = new ModelRenderer(this, 0, 39); Shape10.addBox(0F, 0F, 0F, 16, 1, 1); Shape10.setRotationPoint(-8F, 7.5F, 2F); Shape10.setTextureSize(64, 64); Shape10.mirror = true; setRotation(Shape10, 0F, 0F, 0F); Shape11 = new ModelRenderer(this, 0, 39); Shape11.addBox(0F, 0F, 0F, 16, 1, 1); Shape11.setRotationPoint(-8F, 7.5F, 7F); Shape11.setTextureSize(64, 64); Shape11.mirror = true; setRotation(Shape11, 0F, 0F, 0F); Shape12 = new ModelRenderer(this, 0, 41); Shape12.addBox(0F, 0F, 0F, 1, 1, 16); Shape12.setRotationPoint(-8F, 7.5F, -8F); Shape12.setTextureSize(64, 64); Shape12.mirror = true; setRotation(Shape12, 0F, 0F, 0F); Shape13 = new ModelRenderer(this, 0, 41); Shape13.addBox(0F, 0F, 0F, 1, 1, 16); Shape13.setRotationPoint(-3F, 7.5F, -8F); Shape13.setTextureSize(64, 64); Shape13.mirror = true; setRotation(Shape13, 0F, 0F, 0F); Shape14 = new ModelRenderer(this, 0, 41); Shape14.addBox(0F, 0F, 0F, 1, 1, 16); Shape14.setRotationPoint(2F, 7.5F, -8F); Shape14.setTextureSize(64, 64); Shape14.mirror = true; setRotation(Shape14, 0F, 0F, 0F); Shape15 = new ModelRenderer(this, 0, 41); Shape15.addBox(0F, 0F, 0F, 1, 1, 16); Shape15.setRotationPoint(7F, 7.5F, -8F); Shape15.setTextureSize(64, 64); Shape15.mirror = true; setRotation(Shape15, 0F, 0F, 0F); } public void render(Entity entity, float f, float f1, float f2, float f3, float f4, float f5) { super.render(entity, f, f1, f2, f3, f4, f5); setRotationAngles(f, f1, f2, f3, f4, f5, entity); Shape1.render(f5); Shape2.render(f5); Shape3.render(f5); Shape4.render(f5); Shape5.render(f5); Shape6.render(f5); Shape7.render(f5); Shape8.render(f5); Shape9.render(f5); Shape10.render(f5); Shape11.render(f5); Shape12.render(f5); Shape13.render(f5); Shape14.render(f5); Shape15.render(f5); } public void renderModel(float f) { Shape1.render(f); Shape2.render(f); Shape3.render(f); Shape4.render(f); Shape5.render(f); Shape6.render(f); Shape7.render(f); Shape8.render(f); Shape9.render(f); Shape10.render(f); Shape11.render(f); Shape12.render(f); Shape13.render(f); Shape14.render(f); Shape15.render(f); } private void setRotation(ModelRenderer model, float x, float y, float z) { model.rotateAngleX = x; model.rotateAngleY = y; model.rotateAngleZ = z; } public void setRotationAngles(float f, float f1, float f2, float f3, float f4, float f5, Entity entity) { super.setRotationAngles(f, f1, f2, f3, f4, f5, entity); } } TileEntitySuperWorkbench package megakron.tileentity; import net.minecraft.tileentity.TileEntity; public class TileEntitySuperWorkbench extends TileEntity { } RenderSuperWorkbench package megakron.render; import org.lwjgl.opengl.GL11; import scala.tools.nsc.Phases.Model; import megakron.models.ModelSuperWorkbench; import net.minecraft.client.renderer.tileentity.TileEntitySpecialRenderer; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.ResourceLocation; public class RenderSuperWorkbench extends TileEntitySpecialRenderer { private static final ResourceLocation texture = new ResourceLocation("megakron:/texture/models/SuperWorkbench.png"); private ModelSuperWorkbench model; public RenderSuperWorkbench() { this.model = new ModelSuperWorkbench(); } @Override public void renderTileEntityAt(TileEntity tileentity, double x, double y, double z, float f) { GL11.glPushMatrix(); GL11.glTranslatef((float) x + 0.5F, (float) y + 1.5F, (float) z + 0.5F); GL11.glRotatef(180, (float) 0.0, (float) 0.0, (float) 1.0); this.bindTexture(texture); GL11.glPushMatrix(); this.model.renderModel((float) 0.0625F); GL11.glPopMatrix(); GL11.glPopMatrix(); } } ClientProxy package megakron; import cpw.mods.fml.client.registry.ClientRegistry; import net.minecraft.client.renderer.tileentity.TileEntitySpecialRenderer; import net.minecraft.item.Item; import net.minecraftforge.client.MinecraftForgeClient; import megakron.render.ItemRenderSuperWorkbench; import megakron.render.RenderSuperWorkbench; import megakron.tileentity.TileEntitySuperWorkbench; public class ClientProxy extends CommonProxy { public void registerRenderThing() { //SuperWorkbench TileEntitySpecialRenderer render = new RenderSuperWorkbench(); ClientRegistry.bindTileEntitySpecialRenderer(TileEntitySuperWorkbench.class, new RenderSuperWorkbench()); MinecraftForgeClient.registerItemRenderer(Item.getItemFromBlock(Megakron.superWorkbench), new ItemRenderSuperWorkbench(render, new TileEntitySuperWorkbench ())); } public void registerTileEntitySpescialRenderer() { } } Report [15:37:42] [main/INFO] [LaunchWrapper]: Loading tweak class name cpw.mods.fml.common.launcher.FMLTweaker [15:37:42] [main/INFO] [LaunchWrapper]: Using primary tweak class name cpw.mods.fml.common.launcher.FMLTweaker [15:37:42] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.common.launcher.FMLTweaker [15:37:42] [main/INFO] [FML]: Forge Mod Loader version 7.2.217.1147 for Minecraft 1.7.2 loading [15:37:42] [main/INFO] [FML]: Java is Java HotSpot(TM) 64-Bit Server VM, version 1.7.0, running on Windows 7:amd64:6.1, installed at C:\Program Files\Java\jre1.7.0 [15:37:42] [main/INFO] [FML]: Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation [15:37:42] [main/INFO] [LaunchWrapper]: Loading tweak class name cpw.mods.fml.common.launcher.FMLInjectionAndSortingTweaker [15:37:42] [main/INFO] [LaunchWrapper]: Loading tweak class name cpw.mods.fml.common.launcher.FMLDeobfTweaker [15:37:42] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.common.launcher.FMLInjectionAndSortingTweaker [15:37:42] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.common.launcher.FMLInjectionAndSortingTweaker [15:37:42] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.relauncher.CoreModManager$FMLPluginWrapper [15:37:42] [main/ERROR] [FML]: The binary patch set is missing. Either you are in a development environment, or things are not going to work! [15:37:44] [main/ERROR] [FML]: The minecraft jar file:/C:/Users/PC/.gradle/caches/minecraft/net/minecraftforge/forge/1.7.2-10.12.2.1147/forgeSrc-1.7.2- 10.12.2.1147.jar!/net/minecraft/client/ClientBrandRetriever.class appears to be corrupt! There has been CRITICAL TAMPERING WITH MINECRAFT, it is highly unlikely minecraft will work! STOP NOW, get a clean copy and try again! [15:37:44] [main/ERROR] [FML]: FML has been ordered to ignore the invalid or missing minecraft certificate. This is very likely to cause a problem! [15:37:44] [main/ERROR] [FML]: Technical information: ClientBrandRetriever was at jar:file:/C:/Users/PC/.gradle/caches/minecraft/net/minecraftforge/forge/1.7.2- 10.12.2.1147/forgeSrc-1.7.2-10.12.2.1147.jar!/net/minecraft/client/ClientBrandRetriever.class, there were 0 certificates for it [15:37:44] [main/ERROR] [FML]: FML appears to be missing any signature data. This is not a good thing [15:37:44] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.relauncher.CoreModManager$FMLPluginWrapper [15:37:44] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.common.launcher.FMLDeobfTweaker [15:37:44] [main/INFO] [LaunchWrapper]: Launching wrapped minecraft {net.minecraft.client.main.Main} [15:37:45] [main/INFO]: Setting user: Player216 [15:37:46] [Client thread/INFO]: LWJGL Version: 2.9.0 [15:37:46] [Client thread/ERROR]: Couldn't set icon javax.imageio.IIOException: Can't read input file! at javax.imageio.ImageIO.read(Unknown Source) ~[?:1.7.0] at net.minecraft.client.Minecraft.readImage(Minecraft.java:629) ~[Minecraft.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:458) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:880) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:112) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0] at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) [launchwrapper-1.9.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.9.jar:?] [15:37:46] [Client thread/INFO] [MinecraftForge]: Attempting early MinecraftForge initialization [15:37:46] [Client thread/INFO] [FML]: MinecraftForge v10.12.2.1147 Initialized [15:37:46] [Client thread/INFO] [FML]: Replaced 182 ore recipies [15:37:46] [Client thread/INFO] [MinecraftForge]: Completed early MinecraftForge initialization [15:37:47] [Client thread/INFO] [FML]: Searching C:\Users\PC\Desktop\Minecraft mods\1.7.10 MegaKron\eclipse\mods for mods [15:37:47] [Client thread/ERROR] [FML]: FML has detected a mod that is using a package name based on 'net.minecraft.src' : net.minecraft.src.FMLRenderAccessLibrary. This is generally a severe programming error. There should be no mod code in the minecraft namespace. MOVE YOUR MOD! If you're in eclipse, select your source code and 'refactor' it into a new package. Go on. DO IT NOW! [15:37:49] [Client thread/INFO] [FML]: Forge Mod Loader has identified 4 mods to load [15:37:49] [Client thread/INFO]: Reloading ResourceManager: Default, FMLFileResourcePack:Forge Mod Loader, FMLFileResourcePack:Minecraft Forge, FMLFileResourcePack:MegaKron [15:37:49] [Client thread/INFO] [FML]: Processing ObjectHolder annotations [15:37:49] [Client thread/INFO] [FML]: Found 341 ObjectHolder annotations [15:37:49] [Client thread/INFO] [FML]: Configured a dormant chunk cache size of 0 [15:37:49] [Client thread/INFO] [FML]: Applying holder lookups [15:37:50] [Client thread/INFO] [FML]: Holder lookups applied Starting up SoundSystem... Initializing LWJGL OpenAL (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org) OpenAL initialized. [15:37:50] [sound Library Loader/INFO]: Sound engine started [15:37:50] [Client thread/ERROR]: Using missing texture, unable to load megakron:textures/blocks/SuperWorkbench.png java.io.FileNotFoundException: megakron:textures/blocks/SuperWorkbench.png at net.minecraft.client.resources.FallbackResourceManager.getResource(FallbackResourceManager.java:65) ~[FallbackResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:67) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.texture.TextureMap.loadTextureAtlas(TextureMap.java:126) [TextureMap.class:?] at net.minecraft.client.renderer.texture.TextureMap.loadTexture(TextureMap.java:91) [TextureMap.class:?] at net.minecraft.client.renderer.texture.TextureManager.loadTexture(TextureManager.java:89) [TextureManager.class:?] at net.minecraft.client.renderer.texture.TextureManager.loadTickableTexture(TextureManager.java:71) [TextureManager.class:?] at net.minecraft.client.renderer.texture.TextureManager.loadTextureMap(TextureManager.java:58) [TextureManager.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:569) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:880) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:112) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0] at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) [launchwrapper-1.9.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.9.jar:?] [15:37:51] [Client thread/INFO]: Created: 1024x1024 textures/blocks-atlas [15:37:52] [Client thread/INFO]: Created: 512x512 textures/items-atlas [15:37:52] [Client thread/INFO] [FML]: Forge Mod Loader has successfully loaded 4 mods [15:37:52] [Client thread/INFO]: Reloading ResourceManager: Default, FMLFileResourcePack:Forge Mod Loader, FMLFileResourcePack:Minecraft Forge, FMLFileResourcePack:MegaKron [15:37:52] [Client thread/INFO]: Created: 512x512 textures/items-atlas [15:37:52] [Client thread/ERROR]: Using missing texture, unable to load megakron:textures/blocks/SuperWorkbench.png java.io.FileNotFoundException: megakron:textures/blocks/SuperWorkbench.png at net.minecraft.client.resources.FallbackResourceManager.getResource(FallbackResourceManager.java:65) ~[FallbackResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:67) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.texture.TextureMap.loadTextureAtlas(TextureMap.java:126) [TextureMap.class:?] at net.minecraft.client.renderer.texture.TextureMap.loadTexture(TextureMap.java:91) [TextureMap.class:?] at net.minecraft.client.renderer.texture.TextureManager.loadTexture(TextureManager.java:89) [TextureManager.class:?] at net.minecraft.client.renderer.texture.TextureManager.onResourceManagerReload(TextureManager.java:170) [TextureManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:134) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:118) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:614) [Minecraft.class:?] at cpw.mods.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:303) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:573) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:880) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:112) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0] at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) [launchwrapper-1.9.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.9.jar:?] [15:37:53] [Client thread/INFO]: Created: 1024x1024 textures/blocks-atlas SoundSystem shutting down... Author: Paul Lamb, www.paulscode.com Starting up SoundSystem... Initializing LWJGL OpenAL (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org) OpenAL initialized. [15:37:54] [sound Library Loader/INFO]: Sound engine started [15:37:54] [MCO Availability Checker #1/ERROR]: Couldn't connect to Realms [15:37:56] [Client thread/WARN]: Unable to play unknown soundEvent: minecraft:gui.button.press [15:37:58] [Client thread/WARN]: Unable to play unknown soundEvent: minecraft:gui.button.press [15:37:58] [server thread/INFO]: Starting integrated minecraft server version 1.7.2 [15:37:58] [server thread/INFO]: Generating keypair [15:37:58] [server thread/INFO] [FML]: Injecting existing block and item data into this server instance [15:37:59] [server thread/INFO] [FML]: Applying holder lookups [15:37:59] [server thread/INFO] [FML]: Holder lookups applied [15:37:59] [server thread/INFO] [FML]: Loading dimension 0 (Copy of New World) (net.minecraft.server.integrated.IntegratedServer@db53b7d) [15:37:59] [server thread/INFO] [FML]: Loading dimension 1 (Copy of New World) (net.minecraft.server.integrated.IntegratedServer@db53b7d) [15:37:59] [server thread/INFO] [FML]: Loading dimension -1 (Copy of New World) (net.minecraft.server.integrated.IntegratedServer@db53b7d) [15:37:59] [server thread/INFO]: Preparing start region for level 0 [15:38:00] [server thread/INFO]: Preparing spawn area: 33% [15:38:01] [Netty Client IO #0/INFO] [FML]: Server protocol version 1 [15:38:01] [Netty IO #1/INFO] [FML]: Client protocol version 1 [15:38:01] [Netty IO #1/INFO] [FML]: Client attempting to join with 4 mods : [email protected],[email protected],[email protected],[email protected] [15:38:01] [Netty IO #1/INFO] [FML]: Attempting connection with missing mods [] at CLIENT [15:38:01] [Netty Client IO #0/INFO] [FML]: Attempting connection with missing mods [] at SERVER [15:38:01] [server thread/INFO] [FML]: [server thread] Server side modded connection established [15:38:01] [Client thread/INFO] [FML]: [Client thread] Client side modded connection established [15:38:01] [server thread/INFO]: Player216[local:E:d4cf89e2] logged in with entity id 316 at (-244.37276383032616, 68.0, 167.11981303878963) [15:38:01] [server thread/INFO]: Player216 joined the game [15:38:02] [Client thread/WARN]: Failed to load texture: megakron:/texture/models/SuperWorkbench.png java.io.FileNotFoundException: megakron:/texture/models/SuperWorkbench.png at net.minecraft.client.resources.FallbackResourceManager.getResource(FallbackResourceManager.java:65) ~[FallbackResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:67) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.texture.SimpleTexture.loadTexture(SimpleTexture.java:35) ~[simpleTexture.class:?] at net.minecraft.client.renderer.texture.TextureManager.loadTexture(TextureManager.java:89) [TextureManager.class:?] at net.minecraft.client.renderer.texture.TextureManager.bindTexture(TextureManager.java:45) [TextureManager.class:?] at net.minecraft.client.renderer.tileentity.TileEntitySpecialRenderer.bindTexture(TileEntitySpecialRenderer.java:25) [TileEntitySpecialRenderer.class:?] at megakron.render.RenderSuperWorkbench.renderTileEntityAt(RenderSuperWorkbench.java:28) [RenderSuperWorkbench.class:?] at net.minecraft.client.renderer.tileentity.TileEntityRendererDispatcher.renderTileEntityAt(TileEntityRendererDispatcher.java:141) [TileEntityRendererDispatcher.class:?] at net.minecraft.client.renderer.tileentity.TileEntityRendererDispatcher.renderTileEntity(TileEntityRendererDispatcher.java:126) [TileEntityRendererDispatcher.class:?] at net.minecraft.client.renderer.RenderGlobal.renderEntities(RenderGlobal.java:538) [RenderGlobal.class:?] at net.minecraft.client.renderer.EntityRenderer.renderWorld(EntityRenderer.java:1290) [EntityRenderer.class:?] at net.minecraft.client.renderer.EntityRenderer.updateCameraAndRender(EntityRenderer.java:1087) [EntityRenderer.class:?] at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1012) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:900) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:112) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0] at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) [launchwrapper-1.9.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.9.jar:?]
-
I managed to solve the problem of the textures, the crash persists.
-
The class GuiHandler is already registered and the @Override tells me to remove it. MainClass for the GuiHandler: NetworkRegistry.INSTANCE.registerGuiHandler(this, new GuiHandler());
-
Hi, I'm trying to make a custom workbench and I encountered two problems: the first is that it fails to take the textures and the second is that when I right click on the workbench minecraft crash. How can I fix these two problems? Workbench import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import megakron.Megakron; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.client.renderer.texture.IIconRegister; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Blocks; import net.minecraft.util.IIcon; import net.minecraft.world.World; public class WorkSurface extends Block { @SideOnly(Side.CLIENT) private IIcon workSurfaceTop; private IIcon workSurfaceFront; public WorkSurface() { super(Material.wood); this.setHardness((float) 3.0); this.setResistance((float) 5.0); this.setCreativeTab(Megakron.tabMK); } @SideOnly(Side.CLIENT) public IIcon getIcon(int side, int meta) { return side == 1 ? this.workSurfaceTop : (side == 0 ? Blocks.obsidian.getBlockTextureFromSide(side) : (side != 2 && side != 4 ? this.blockIcon : this.workSurfaceFront)); } @SideOnly(Side.CLIENT) public void registerBlockIcon(IIconRegister iconRegister) { this.blockIcon = iconRegister.registerIcon("megakron:workSurfaceSide"); this.workSurfaceTop = iconRegister.registerIcon("megakron:workSurfaceTap"); this.workSurfaceFront = iconRegister.registerIcon("megakron:workSurfaceFront"); } public boolean onBlockActivated(World world, int x, int y, int z, EntityPlayer player, int q, float a, float b, float c) { if(!player.isSneaking()) { player.openGui(Megakron.instance, Megakron.guiIDWorkSurface, world, x, y, z); return true; } else { return false; } } } GuiHandler import megakron.Megakron; import megakron.container.ContainerSuperGlueFurnace; import megakron.container.ContainerWorkSurface; import megakron.gui.GuiSuperGlueFurnace; import megakron.tileentity.TileEntitySuperGlueFurnace; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.tileentity.TileEntity; import net.minecraft.world.World; import cpw.mods.fml.common.network.IGuiHandler; public class GuiHandler implements IGuiHandler { @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { TileEntity entity = world.getTileEntity(x, y, z); if(entity != null) { switch(ID) { case Megakron.guiIDSuperGlueFurnace: if(entity instanceof TileEntitySuperGlueFurnace) { return new ContainerSuperGlueFurnace(player.inventory, (TileEntitySuperGlueFurnace) entity); } } } if(ID == Megakron.guiIDWorkSurface) { return ID == Megakron.guiIDWorkSurface && world.getBlock(x, y, z) == Megakron.blockWorkSurface ? new ContainerWorkSurface(player.inventory, world, x, y, z) : null; } return null; } @Override public Object getClientGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { TileEntity entity = world.getTileEntity(x, y, z); if(entity != null) { switch(ID) { case Megakron.guiIDSuperGlueFurnace: if(entity instanceof TileEntitySuperGlueFurnace) { return new GuiSuperGlueFurnace(player.inventory, (TileEntitySuperGlueFurnace) entity); } } } return null; } } WorkbenchContainer import megakron.Megakron; import megakron.craftign.WorkSurfaceCraftingManager; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.player.InventoryPlayer; import net.minecraft.inventory.Container; import net.minecraft.inventory.IInventory; import net.minecraft.inventory.InventoryCraftResult; import net.minecraft.inventory.InventoryCrafting; import net.minecraft.inventory.Slot; import net.minecraft.inventory.SlotCrafting; import net.minecraft.item.ItemStack; import net.minecraft.item.crafting.CraftingManager; import net.minecraft.world.World; public class ContainerWorkSurface extends Container { public InventoryCrafting craftMatrix; public IInventory craftResult; private World worldObj; private int posX; private int posY; private int posZ; public ContainerWorkSurface(InventoryPlayer invPlayer, World world, int x, int y, int z) { craftMatrix = new InventoryCrafting(this, 5, 5); craftResult = new InventoryCraftResult(); worldObj = world; posX = x; posY = y; posZ = z; this.addSlotToContainer(new SlotCrafting(invPlayer.player, craftMatrix, craftResult, 0, 131, 36)); for(int i = 0; i < 5; i++) { for(int k = 0; k < 5; k++) { this.addSlotToContainer(new Slot(craftMatrix, k + i * 5, 4 + k * 18, 3 + i * 18)); } } for(int i = 0; i < 3; i++) { for(int k = 0; k < 9; k++) { this.addSlotToContainer(new Slot(invPlayer, k + i * 9 + 9, 8 + k * 18, 94 + i * 18)); } } for(int i = 0; i < 9; i++) { this.addSlotToContainer(new Slot(invPlayer, i, 8 + i * 18, 148)); } onCraftMatrixChanged(craftMatrix); } public void onCraftMatrixChanged(IInventory iInventory) { this.craftResult.setInventorySlotContents(0, WorkSurfaceCraftingManager.getInstance().findMatchingRecipe(this.craftMatrix, this.worldObj)); } @Override public boolean canInteractWith(EntityPlayer player) { if(worldObj.getBlock(posX, posY, posZ) != Megakron.blockWorkSurface) { return false; } else { return player.getDistanceSq((double) posX + 0.5, (double) posY + 0.5, (double) posZ + 0.5) <= 64.0D; } } public void onContainerClosed(EntityPlayer par1EntityPlayer) { super.onContainerClosed(par1EntityPlayer); if (!this.worldObj.isRemote) { for (int var2 = 0; var2 < 29; ++var2) { ItemStack var3 = this.craftMatrix.getStackInSlotOnClosing(var2); if (var3 != null) { par1EntityPlayer.dropPlayerItemWithRandomChoice(var3, false); } } } } public ItemStack transferStackInSlot(EntityPlayer p_82846_1_, int p_82846_2_) { ItemStack var3 = null; Slot var4 = (Slot)this.inventorySlots.get(p_82846_2_); if (var4 != null && var4.getHasStack()) { ItemStack var5 = var4.getStack(); var3 = var5.copy(); if (p_82846_2_ == 0) { if (!this.mergeItemStack(var5, 10, 46, true)) { return null; } var4.onSlotChange(var5, var3); } else if (p_82846_2_ >= 10 && p_82846_2_ < 37) { if (!this.mergeItemStack(var5, 37, 46, false)) { return null; } } else if (p_82846_2_ >= 37 && p_82846_2_ < 46) { if (!this.mergeItemStack(var5, 10, 37, false)) { return null; } } else if (!this.mergeItemStack(var5, 10, 46, false)) { return null; } if (var5.stackSize == 0) { var4.putStack((ItemStack)null); } else { var4.onSlotChanged(); } if (var5.stackSize == var3.stackSize) { return null; } var4.onPickupFromSlot(p_82846_1_, var5); } return var3; } } Crash Report textures [14:40:24] [Client thread/ERROR]: Using missing texture, unable to load minecraft:textures/blocks/MISSING_ICON_BLOCK_184_WorkSurface.png java.io.FileNotFoundException: minecraft:textures/blocks/MISSING_ICON_BLOCK_184_WorkSurface.png at net.minecraft.client.resources.FallbackResourceManager.getResource(FallbackResourceManager.java:65) ~[FallbackResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:67) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.texture.TextureMap.loadTextureAtlas(TextureMap.java:126) [TextureMap.class:?] at net.minecraft.client.renderer.texture.TextureMap.loadTexture(TextureMap.java:91) [TextureMap.class:?] at net.minecraft.client.renderer.texture.TextureManager.loadTexture(TextureManager.java:89) [TextureManager.class:?] at net.minecraft.client.renderer.texture.TextureManager.onResourceManagerReload(TextureManager.java:170) [TextureManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:134) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:118) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:614) [Minecraft.class:?] at cpw.mods.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:303) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:573) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:880) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:112) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0] at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) [launchwrapper-1.9.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.9.jar:?] [14:40:25] [Client thread/INFO]: Created: 1024x1024 textures/blocks-atlas Crash Report right click [14:40:36] [Client thread/FATAL]: Unreported exception thrown! java.lang.NullPointerException at cpw.mods.fml.common.network.NetworkRegistry.getLocalGuiContainer(NetworkRegistry.java:263) ~[NetworkRegistry.class:?] at cpw.mods.fml.common.network.internal.FMLNetworkHandler.openGui(FMLNetworkHandler.java:93) ~[FMLNetworkHandler.class:?] at net.minecraft.entity.player.EntityPlayer.openGui(EntityPlayer.java:2501) ~[EntityPlayer.class:?] at megakron.blocks.WorkSurface.onBlockActivated(WorkSurface.java:43) ~[WorkSurface.class:?] at net.minecraft.client.multiplayer.PlayerControllerMP.onPlayerRightClick(PlayerControllerMP.java:376) ~[PlayerControllerMP.class:?] at net.minecraft.client.Minecraft.func_147121_ag(Minecraft.java:1486) ~[Minecraft.class:?] at net.minecraft.client.Minecraft.runTick(Minecraft.java:1999) ~[Minecraft.class:?] at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:984) ~[Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:900) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:112) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0] at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) [launchwrapper-1.9.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.9.jar:?] ---- Minecraft Crash Report ---- // Why is it breaking Time: 07/12/14 14.40 Description: Unexpected error java.lang.NullPointerException: Unexpected error at cpw.mods.fml.common.network.NetworkRegistry.getLocalGuiContainer(NetworkRegistry.java:263) at cpw.mods.fml.common.network.internal.FMLNetworkHandler.openGui(FMLNetworkHandler.java:93) at net.minecraft.entity.player.EntityPlayer.openGui(EntityPlayer.java:2501) at megakron.blocks.WorkSurface.onBlockActivated(WorkSurface.java:43) at net.minecraft.client.multiplayer.PlayerControllerMP.onPlayerRightClick(PlayerControllerMP.java:376) at net.minecraft.client.Minecraft.func_147121_ag(Minecraft.java:1486) at net.minecraft.client.Minecraft.runTick(Minecraft.java:1999) at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:984) at net.minecraft.client.Minecraft.run(Minecraft.java:900) at net.minecraft.client.main.Main.main(Main.java:112) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Stacktrace: at cpw.mods.fml.common.network.NetworkRegistry.getLocalGuiContainer(NetworkRegistry.java:263) at cpw.mods.fml.common.network.internal.FMLNetworkHandler.openGui(FMLNetworkHandler.java:93) at net.minecraft.entity.player.EntityPlayer.openGui(EntityPlayer.java:2501) at megakron.blocks.WorkSurface.onBlockActivated(WorkSurface.java:43) at net.minecraft.client.multiplayer.PlayerControllerMP.onPlayerRightClick(PlayerControllerMP.java:376) at net.minecraft.client.Minecraft.func_147121_ag(Minecraft.java:1486) -- Affected level -- Details: Level name: MpServer All players: 1 total; [EntityClientPlayerMP['Player64'/341, l='MpServer', x=145,73, y=65,62, z=277,96]] Chunk stats: MultiplayerChunkCache: 225, 225 Level seed: 0 Level generator: ID 00 - default, ver 1. Features enabled: false Level generator options: Level spawn location: World: (-4,64,256), Chunk: (at 12,4,0 in -1,16; contains blocks -16,0,256 to -1,255,271), Region: (-1,0; contains chunks -32,0 to -1,31, blocks -512,0,0 to -1,255,511) Level time: 41664 game time, 12469 day time Level dimension: 0 Level storage version: 0x00000 - Unknown? Level weather: Rain time: 0 (now: true), thunder time: 0 (now: false) Level game mode: Game mode: creative (ID 1). Hardcore: false. Cheats: false Forced entities: 108 total; [EntityCreeper['Creeper'/275, l='MpServer', x=149,94, y=32,00, z=286,75], EntityCreeper['Creeper'/274, l='MpServer', x=159,65, y=56,00, z=234,70], EntityCreeper['Creeper'/273, l='MpServer', x=158,59, y=56,00, z=233,96], EntityWitch['Witch'/272, l='MpServer', x=155,50, y=56,00, z=234,50], EntitySkeleton['Skeleton'/279, l='MpServer', x=153,56, y=19,00, z=302,09], EntitySkeleton['Skeleton'/278, l='MpServer', x=159,16, y=60,00, z=287,59], EntityBat['Bat'/277, l='MpServer', x=152,47, y=61,10, z=287,75], EntityBat['Bat'/276, l='MpServer', x=152,25, y=63,10, z=285,75], EntityBat['Bat'/283, l='MpServer', x=147,34, y=60,10, z=306,25], EntityBat['Bat'/282, l='MpServer', x=155,53, y=52,10, z=301,38], EntityBat['Bat'/281, l='MpServer', x=155,75, y=52,10, z=297,41], EntitySkeleton['Skeleton'/280, l='MpServer', x=148,91, y=32,00, z=288,50], EntitySkeleton['Skeleton'/287, l='MpServer', x=150,50, y=44,00, z=342,88], EntityZombie['Zombie'/286, l='MpServer', x=151,41, y=49,00, z=310,41], EntityZombie['Zombie'/285, l='MpServer', x=151,50, y=49,00, z=314,50], EntitySpider['Spider'/284, l='MpServer', x=156,59, y=49,00, z=312,44], EntitySquid['Squid'/258, l='MpServer', x=143,50, y=49,00, z=262,50], EntityZombie['Zombie'/259, l='MpServer', x=133,31, y=46,00, z=296,03], EntitySquid['Squid'/256, l='MpServer', x=141,96, y=55,27, z=244,50], EntitySkeleton['Skeleton'/257, l='MpServer', x=130,38, y=25,00, z=261,30], EntitySpider['Spider'/262, l='MpServer', x=140,00, y=47,00, z=293,25], EntitySkeleton['Skeleton'/263, l='MpServer', x=132,16, y=46,00, z=295,50], EntitySkeleton['Skeleton'/260, l='MpServer', x=136,47, y=46,00, z=296,16], EntityCreeper['Creeper'/261, l='MpServer', x=131,31, y=46,00, z=295,50], EntitySkeleton['Skeleton'/264, l='MpServer', x=128,50, y=48,00, z=299,09], EntitySkeleton['Skeleton'/265, l='MpServer', x=134,88, y=14,00, z=319,47], EntityCreeper['Creeper'/270, l='MpServer', x=153,31, y=17,00, z=225,84], EntitySkeleton['Skeleton'/271, l='MpServer', x=150,50, y=55,00, z=234,91], EntityChicken['Chicken'/269, l='MpServer', x=156,28, y=80,00, z=200,66], EntitySlime['Slime'/305, l='MpServer', x=164,69, y=37,00, z=284,69], EntityCreeper['Creeper'/304, l='MpServer', x=164,25, y=52,00, z=263,84], EntityBat['Bat'/307, l='MpServer', x=175,72, y=42,10, z=280,28], EntitySkeleton['Skeleton'/306, l='MpServer', x=160,94, y=47,00, z=285,47], EntityBat['Bat'/309, l='MpServer', x=162,69, y=59,10, z=295,31], EntityBat['Bat'/308, l='MpServer', x=177,03, y=40,32, z=284,44], EntityZombie['Zombie'/311, l='MpServer', x=174,09, y=20,00, z=314,50], EntityZombie['Zombie'/310, l='MpServer', x=170,59, y=20,00, z=314,06], EntitySkeleton['Skeleton'/313, l='MpServer', x=174,69, y=20,00, z=311,38], EntityCreeper['Creeper'/312, l='MpServer', x=175,16, y=20,00, z=305,78], EntityBat['Bat'/315, l='MpServer', x=171,25, y=26,10, z=356,75], EntityZombie['Zombie'/314, l='MpServer', x=160,59, y=45,00, z=351,00], EntityZombie['Zombie'/317, l='MpServer', x=162,50, y=45,00, z=353,22], EntityZombie['Zombie'/318, l='MpServer', x=164,13, y=52,00, z=356,50], EntityZombie['Zombie'/288, l='MpServer', x=146,50, y=43,00, z=341,50], EntityBat['Bat'/289, l='MpServer', x=142,56, y=42,00, z=343,03], EntityZombie['Zombie'/290, l='MpServer', x=153,56, y=53,00, z=341,00], EntityChicken['Chicken'/298, l='MpServer', x=170,50, y=92,00, z=204,50], EntityChicken['Chicken'/299, l='MpServer', x=161,29, y=84,00, z=197,94], EntityChicken['Chicken'/300, l='MpServer', x=171,53, y=66,00, z=219,47], EntityZombie['Zombie'/301, l='MpServer', x=162,47, y=58,00, z=234,88], EntityChicken['Chicken'/302, l='MpServer', x=169,13, y=68,00, z=227,59], EntityZombie['Zombie'/303, l='MpServer', x=164,97, y=41,00, z=252,47], EntityPig['Pig'/343, l='MpServer', x=216,56, y=67,00, z=276,75], EntitySkeleton['Skeleton'/342, l='MpServer', x=193,50, y=25,00, z=292,88], EntityPig['Pig'/351, l='MpServer', x=213,14, y=68,00, z=329,60], EntityPig['Pig'/348, l='MpServer', x=199,06, y=70,00, z=217,91], EntityPig['Pig'/347, l='MpServer', x=216,56, y=68,00, z=248,38], EntityPig['Pig'/346, l='MpServer', x=201,33, y=71,00, z=225,38], EntityCreeper['Creeper'/345, l='MpServer', x=217,84, y=17,00, z=291,35], EntityPig['Pig'/344, l='MpServer', x=220,69, y=65,00, z=257,50], EntityZombie['Zombie'/326, l='MpServer', x=181,13, y=52,00, z=263,56], EntityBat['Bat'/327, l='MpServer', x=181,53, y=24,07, z=297,53], EntityCreeper['Creeper'/324, l='MpServer', x=176,00, y=42,00, z=255,59], EntityCreeper['Creeper'/325, l='MpServer', x=176,50, y=40,00, z=260,50], EntityPig['Pig'/322, l='MpServer', x=187,91, y=75,00, z=222,13], EntityChicken['Chicken'/323, l='MpServer', x=176,84, y=65,00, z=235,91], EntityChicken['Chicken'/321, l='MpServer', x=177,50, y=69,00, z=218,50], EntitySkeleton['Skeleton'/330, l='MpServer', x=179,09, y=20,00, z=307,56], EntityCreeper['Creeper'/328, l='MpServer', x=182,50, y=32,08, z=286,48], EntitySkeleton['Skeleton'/329, l='MpServer', x=185,09, y=19,00, z=316,63], EntityPig['Pig'/352, l='MpServer', x=215,94, y=66,00, z=327,84], EntityPig['Pig'/353, l='MpServer', x=216,19, y=67,00, z=322,56], EntityChicken['Chicken'/366, l='MpServer', x=213,53, y=72,00, z=206,63], EntityPig['Pig'/360, l='MpServer', x=209,50, y=69,00, z=338,69], EntitySkeleton['Skeleton'/362, l='MpServer', x=192,94, y=38,00, z=356,50], EntitySkeleton['Skeleton'/363, l='MpServer', x=195,32, y=42,00, z=356,69], EntityClientPlayerMP['Player64'/341, l='MpServer', x=145,73, y=65,62, z=277,96], EntityChicken['Chicken'/205, l='MpServer', x=69,59, y=69,00, z=259,38], EntitySkeleton['Skeleton'/204, l='MpServer', x=73,28, y=47,00, z=229,50], EntityCreeper['Creeper'/207, l='MpServer', x=68,50, y=31,00, z=275,00], EntityChicken['Chicken'/206, l='MpServer', x=72,19, y=68,00, z=265,69], EntityPig['Pig'/216, l='MpServer', x=84,72, y=64,00, z=334,66], EntityPig['Pig'/217, l='MpServer', x=92,70, y=66,00, z=353,13], EntityBat['Bat'/212, l='MpServer', x=90,50, y=48,10, z=233,53], EntityCreeper['Creeper'/213, l='MpServer', x=92,09, y=26,00, z=241,25], EntitySkeleton['Skeleton'/214, l='MpServer', x=90,84, y=31,00, z=278,41], EntitySquid['Squid'/215, l='MpServer', x=89,33, y=56,34, z=321,82], EntityChicken['Chicken'/209, l='MpServer', x=67,19, y=67,92, z=323,53], EntityChicken['Chicken'/210, l='MpServer', x=66,47, y=64,00, z=329,47], EntityPig['Pig'/239, l='MpServer', x=98,81, y=64,00, z=340,91], EntityPig['Pig'/238, l='MpServer', x=97,38, y=64,00, z=344,59], EntityPig['Pig'/237, l='MpServer', x=109,44, y=64,00, z=334,53], EntitySquid['Squid'/236, l='MpServer', x=95,96, y=54,28, z=309,63], EntitySquid['Squid'/235, l='MpServer', x=101,50, y=53,00, z=304,50], EntitySquid['Squid'/234, l='MpServer', x=96,50, y=53,21, z=307,60], EntityCreeper['Creeper'/233, l='MpServer', x=110,72, y=17,00, z=282,06], EntityCreeper['Creeper'/232, l='MpServer', x=107,78, y=17,00, z=285,16], EntitySkeleton['Skeleton'/231, l='MpServer', x=97,59, y=15,00, z=266,30], EntityZombie['Zombie'/230, l='MpServer', x=102,09, y=46,00, z=255,38], EntityCreeper['Creeper'/229, l='MpServer', x=97,00, y=46,00, z=254,56], EntityZombie['Zombie'/228, l='MpServer', x=103,01, y=66,00, z=236,56], EntitySkeleton['Skeleton'/254, l='MpServer', x=145,39, y=52,00, z=235,23], EntitySquid['Squid'/255, l='MpServer', x=135,00, y=56,00, z=244,51], EntityZombie['Zombie'/253, l='MpServer', x=128,50, y=16,00, z=213,50], EntitySkeleton['Skeleton'/250, l='MpServer', x=118,13, y=19,00, z=276,44], EntitySlime['Slime'/248, l='MpServer', x=124,31, y=11,57, z=208,31], EntitySkeleton['Skeleton'/249, l='MpServer', x=122,41, y=14,00, z=214,00], EntityPig['Pig'/240, l='MpServer', x=107,13, y=64,00, z=336,19]] Retry entities: 0 total; [] Server brand: fml,forge Server type: Integrated singleplayer server Stacktrace: at net.minecraft.client.multiplayer.WorldClient.addWorldInfoToCrashReport(WorldClient.java:410) at net.minecraft.client.Minecraft.addGraphicsAndWorldToCrashReport(Minecraft.java:2509) at net.minecraft.client.Minecraft.run(Minecraft.java:929) at net.minecraft.client.main.Main.main(Main.java:112) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) -- System Details -- Details: Minecraft Version: 1.7.2 Operating System: Windows 7 (amd64) version 6.1 Java Version: 1.7.0, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 869837744 bytes (829 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M AABB Pool Size: 15110 (846160 bytes; 0 MB) allocated, 2 (112 bytes; 0 MB) used IntCache: cache: 0, tcache: 0, allocated: 13, tallocated: 95 FML: MCP v9.03 FML v7.2.217.1147 Minecraft Forge 10.12.2.1147 4 mods loaded, 4 mods active mcp{9.03} [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available FML{7.2.217.1147} [Forge Mod Loader] (forgeSrc-1.7.2-10.12.2.1147.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available Forge{10.12.2.1147} [Minecraft Forge] (forgeSrc-1.7.2-10.12.2.1147.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available megakron{1.0} [MegaKron] (bin) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available Launched Version: 1.6 LWJGL: 2.9.0 OpenGL: GeForce GTX 760/PCIe/SSE2 GL version 4.4.0 NVIDIA 344.60, NVIDIA Corporation Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: [] Current Language: ~~ERROR~~ NullPointerException: null Profiler Position: N/A (disabled) Vec3 Pool Size: 5202 (291312 bytes; 0 MB) allocated, 19 (1064 bytes; 0 MB) used Anisotropic Filtering: Off (1) #@!@# Game crashed! Crash report saved to: #@!@# C:\Users\PC\Desktop\Minecraft mods\1.7.10 MegaKron\eclipse\.\crash-reports\crash-2014-12-07_14.40.36-client.txt AL lib: (EE) alc_cleanup: 1 device not closed
-
Thank you, it works ^^
-
I am sorry, crash when I try to grow the tree. Now place the crash report: [Client thread/FATAL]: Unreported exception thrown! java.lang.ArrayIndexOutOfBoundsException: 1 at megakron.trees.TyreLeaf.getIcon(TyreLeaf.java:58) ~[TyreLeaf.class:?] at net.minecraft.block.Block.getIcon(Block.java:643) ~[block.class:?] at net.minecraft.client.renderer.RenderBlocks.getBlockIcon(RenderBlocks.java:8417) ~[RenderBlocks.class:?] at net.minecraft.client.renderer.RenderBlocks.renderStandardBlockWithAmbientOcclusion(RenderBlocks.java:4614) ~[RenderBlocks.class:?] at net.minecraft.client.renderer.RenderBlocks.renderStandardBlock(RenderBlocks.java:4416) ~[RenderBlocks.class:?] at net.minecraft.client.renderer.RenderBlocks.renderBlockByRenderType(RenderBlocks.java:345) ~[RenderBlocks.class:?] at net.minecraft.client.renderer.WorldRenderer.updateRenderer(WorldRenderer.java:207) ~[WorldRenderer.class:?] at net.minecraft.client.renderer.RenderGlobal.updateRenderers(RenderGlobal.java:1610) ~[RenderGlobal.class:?] at net.minecraft.client.renderer.EntityRenderer.renderWorld(EntityRenderer.java:1254) ~[EntityRenderer.class:?] at net.minecraft.client.renderer.EntityRenderer.updateCameraAndRender(EntityRenderer.java:1087) ~[EntityRenderer.class:?] at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1012) ~[Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:900) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:112) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0] at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) [launchwrapper-1.9.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.9.jar:?] ---- Minecraft Crash Report ---- // I feel sad now Time: 04/12/14 16.06 Description: Unexpected error java.lang.ArrayIndexOutOfBoundsException: 1 at megakron.trees.TyreLeaf.getIcon(TyreLeaf.java:58) at net.minecraft.block.Block.getIcon(Block.java:643) at net.minecraft.client.renderer.RenderBlocks.getBlockIcon(RenderBlocks.java:8417) at net.minecraft.client.renderer.RenderBlocks.renderStandardBlockWithAmbientOcclusion(RenderBlocks.java:4614) at net.minecraft.client.renderer.RenderBlocks.renderStandardBlock(RenderBlocks.java:4416) at net.minecraft.client.renderer.RenderBlocks.renderBlockByRenderType(RenderBlocks.java:345) at net.minecraft.client.renderer.WorldRenderer.updateRenderer(WorldRenderer.java:207) at net.minecraft.client.renderer.RenderGlobal.updateRenderers(RenderGlobal.java:1610) at net.minecraft.client.renderer.EntityRenderer.renderWorld(EntityRenderer.java:1254) at net.minecraft.client.renderer.EntityRenderer.updateCameraAndRender(EntityRenderer.java:1087) at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1012) at net.minecraft.client.Minecraft.run(Minecraft.java:900) at net.minecraft.client.main.Main.main(Main.java:112) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Stacktrace: at megakron.trees.TyreLeaf.getIcon(TyreLeaf.java:58) at net.minecraft.block.Block.getIcon(Block.java:643) at net.minecraft.client.renderer.RenderBlocks.getBlockIcon(RenderBlocks.java:8417) at net.minecraft.client.renderer.RenderBlocks.renderStandardBlockWithAmbientOcclusion(RenderBlocks.java:4614) at net.minecraft.client.renderer.RenderBlocks.renderStandardBlock(RenderBlocks.java:4416) at net.minecraft.client.renderer.RenderBlocks.renderBlockByRenderType(RenderBlocks.java:345) at net.minecraft.client.renderer.WorldRenderer.updateRenderer(WorldRenderer.java:207) at net.minecraft.client.renderer.RenderGlobal.updateRenderers(RenderGlobal.java:1610) at net.minecraft.client.renderer.EntityRenderer.renderWorld(EntityRenderer.java:1254) -- Affected level -- Details: Level name: MpServer All players: 1 total; [EntityClientPlayerMP['Player686'/106, l='MpServer', x=163,50, y=67,62, z=246,50]] Chunk stats: MultiplayerChunkCache: 225, 225 Level seed: 0 Level generator: ID 00 - default, ver 1. Features enabled: false Level generator options: Level spawn location: World: (160,64,256), Chunk: (at 0,4,0 in 10,16; contains blocks 160,0,256 to 175,255,271), Region: (0,0; contains chunks 0,0 to 31,31, blocks 0,0,0 to 511,255,511) Level time: 292 game time, 292 day time Level dimension: 0 Level storage version: 0x00000 - Unknown? Level weather: Rain time: 0 (now: false), thunder time: 0 (now: false) Level game mode: Game mode: creative (ID 1). Hardcore: false. Cheats: false Forced entities: 90 total; [EntityHorse['Horse'/6, l='MpServer', x=92,50, y=67,00, z=263,50], EntityHorse['Horse'/7, l='MpServer', x=94,13, y=67,00, z=262,13], EntityHorse['Horse'/8, l='MpServer', x=139,28, y=65,00, z=178,72], EntityHorse['Horse'/9, l='MpServer', x=141,13, y=65,00, z=180,00], EntityHorse['Horse'/10, l='MpServer', x=143,53, y=64,00, z=180,00], EntityHorse['Horse'/11, l='MpServer', x=135,99, y=66,00, z=177,00], EntitySheep['Sheep'/12, l='MpServer', x=140,50, y=65,00, z=168,50], EntitySheep['Sheep'/13, l='MpServer', x=142,50, y=67,00, z=171,50], EntitySheep['Sheep'/14, l='MpServer', x=144,50, y=67,00, z=169,50], EntitySheep['Sheep'/15, l='MpServer', x=144,50, y=67,00, z=171,50], EntityHorse['Horse'/17, l='MpServer', x=144,50, y=68,00, z=282,50], EntityHorse['Horse'/16, l='MpServer', x=140,50, y=69,00, z=283,50], EntityHorse['Horse'/19, l='MpServer', x=148,50, y=67,00, z=299,50], EntityHorse['Horse'/18, l='MpServer', x=146,13, y=67,00, z=300,13], EntityHorse['Horse'/29, l='MpServer', x=176,14, y=68,00, z=260,02], EntityHorse['Horse'/28, l='MpServer', x=172,56, y=68,00, z=262,22], EntityHorse['Horse'/31, l='MpServer', x=167,81, y=67,00, z=258,81], EntityHorse['Horse'/30, l='MpServer', x=176,13, y=67,00, z=257,13], EntityHorse['Horse'/34, l='MpServer', x=213,84, y=65,00, z=309,04], EntityHorse['Horse'/35, l='MpServer', x=205,75, y=64,00, z=298,25], EntityHorse['Horse'/32, l='MpServer', x=176,13, y=67,00, z=258,13], EntityHorse['Horse'/33, l='MpServer', x=177,83, y=68,00, z=260,71], EntityHorse['Horse'/38, l='MpServer', x=209,50, y=67,00, z=297,50], EntityHorse['Horse'/39, l='MpServer', x=212,13, y=68,00, z=296,13], EntityHorse['Horse'/36, l='MpServer', x=202,72, y=64,00, z=299,13], EntityHorse['Horse'/37, l='MpServer', x=207,88, y=64,00, z=297,72], EntityCreeper['Creeper'/112, l='MpServer', x=91,50, y=14,00, z=167,50], EntityCreeper['Creeper'/2099, l='MpServer', x=124,50, y=13,00, z=265,50], EntitySquid['Squid'/220, l='MpServer', x=104,50, y=60,16, z=192,50], EntitySquid['Squid'/222, l='MpServer', x=108,63, y=58,38, z=186,34], EntitySquid['Squid'/239, l='MpServer', x=133,28, y=55,31, z=202,03], EntitySquid['Squid'/238, l='MpServer', x=132,41, y=54,69, z=196,06], EntitySquid['Squid'/237, l='MpServer', x=131,25, y=55,34, z=207,50], EntitySquid['Squid'/236, l='MpServer', x=129,72, y=53,84, z=202,97], EntitySquid['Squid'/235, l='MpServer', x=136,50, y=54,00, z=199,63], EntitySquid['Squid'/234, l='MpServer', x=129,94, y=55,03, z=200,13], EntitySquid['Squid'/233, l='MpServer', x=136,50, y=54,72, z=200,78], EntitySquid['Squid'/232, l='MpServer', x=135,09, y=53,28, z=202,50], EntitySquid['Squid'/231, l='MpServer', x=109,50, y=59,06, z=188,88], EntitySquid['Squid'/230, l='MpServer', x=112,28, y=60,22, z=188,06], EntitySquid['Squid'/229, l='MpServer', x=114,50, y=57,78, z=183,78], EntitySquid['Squid'/227, l='MpServer', x=114,72, y=57,34, z=197,09], EntitySquid['Squid'/226, l='MpServer', x=114,63, y=57,22, z=190,25], EntitySquid['Squid'/225, l='MpServer', x=113,81, y=59,03, z=193,34], EntitySquid['Squid'/240, l='MpServer', x=135,50, y=54,00, z=198,50], EntityClientPlayerMP['Player686'/106, l='MpServer', x=163,50, y=67,62, z=246,50], EntitySpider['Spider'/312, l='MpServer', x=170,44, y=17,00, z=184,13], EntitySpider['Spider'/315, l='MpServer', x=161,91, y=16,00, z=185,78], EntityCreeper['Creeper'/322, l='MpServer', x=117,50, y=15,00, z=252,50], EntityCreeper['Creeper'/357, l='MpServer', x=106,50, y=30,00, z=229,50], EntityCreeper['Creeper'/358, l='MpServer', x=105,50, y=30,00, z=232,50], EntityBat['Bat'/444, l='MpServer', x=159,66, y=23,07, z=207,96], EntityCreeper['Creeper'/458, l='MpServer', x=156,72, y=21,00, z=217,13], EntityWitch['Witch'/510, l='MpServer', x=110,50, y=54,00, z=210,50], EntityWitch['Witch'/511, l='MpServer', x=110,50, y=54,00, z=209,50], EntityBat['Bat'/561, l='MpServer', x=159,53, y=50,96, z=314,50], EntityZombie['Zombie'/2810, l='MpServer', x=102,50, y=31,00, z=186,50], EntitySpider['Spider'/2814, l='MpServer', x=101,34, y=31,00, z=190,56], EntityZombie['Zombie'/2812, l='MpServer', x=100,31, y=31,00, z=188,88], EntityItem['item.tile.gravel'/2795, l='MpServer', x=190,81, y=23,13, z=200,78], EntitySkeleton['Skeleton'/638, l='MpServer', x=109,44, y=22,00, z=210,16], EntityBat['Bat'/751, l='MpServer', x=223,28, y=34,00, z=246,40], EntityBat['Bat'/830, l='MpServer', x=162,75, y=26,10, z=192,28], EntitySpider['Spider'/800, l='MpServer', x=179,50, y=41,00, z=324,50], EntityBat['Bat'/782, l='MpServer', x=111,94, y=46,00, z=213,51], EntityCreeper['Creeper'/832, l='MpServer', x=239,50, y=41,00, z=176,50], EntityCreeper['Creeper'/833, l='MpServer', x=241,50, y=41,00, z=173,50], EntityZombie['Zombie'/2818, l='MpServer', x=102,84, y=31,00, z=189,28], EntityZombie['Zombie'/2819, l='MpServer', x=104,50, y=31,00, z=188,50], EntitySpider['Spider'/1104, l='MpServer', x=104,30, y=28,00, z=241,70], EntitySkeleton['Skeleton'/1038, l='MpServer', x=117,50, y=47,00, z=234,50], EntityZombie['Zombie'/1175, l='MpServer', x=116,38, y=48,00, z=185,63], EntityZombie['Zombie'/1176, l='MpServer', x=116,00, y=49,00, z=184,22], EntityZombie['Zombie'/1177, l='MpServer', x=113,88, y=48,00, z=184,44], EntitySkeleton['Skeleton'/1206, l='MpServer', x=162,94, y=15,00, z=191,47], EntityZombie['Zombie'/1207, l='MpServer', x=226,50, y=49,00, z=187,50], EntitySkeleton['Skeleton'/1205, l='MpServer', x=167,50, y=17,00, z=183,50], EntityCreeper['Creeper'/1309, l='MpServer', x=164,50, y=26,00, z=184,50], EntityCreeper['Creeper'/1322, l='MpServer', x=113,50, y=43,00, z=175,50], EntitySlime['Slime'/1524, l='MpServer', x=108,78, y=28,18, z=170,78], EntityCreeper['Creeper'/1470, l='MpServer', x=108,50, y=19,00, z=202,50], EntityZombie['Zombie'/1600, l='MpServer', x=196,66, y=43,00, z=199,97], EntityZombie['Zombie'/1599, l='MpServer', x=195,13, y=44,00, z=201,53], EntityZombie['Zombie'/1592, l='MpServer', x=115,50, y=44,00, z=208,50], EntitySpider['Spider'/2017, l='MpServer', x=192,13, y=29,00, z=194,38], EntitySpider['Spider'/2016, l='MpServer', x=191,19, y=28,00, z=194,41], EntityZombie['Zombie'/2019, l='MpServer', x=191,34, y=25,00, z=197,68], EntityZombie['Zombie'/2020, l='MpServer', x=192,47, y=27,00, z=193,44], EntityZombie['Zombie'/2000, l='MpServer', x=105,50, y=31,00, z=187,50], EntityZombie['Zombie'/1999, l='MpServer', x=105,50, y=31,00, z=184,50]] Retry entities: 0 total; [] Server brand: fml,forge Server type: Integrated singleplayer server Stacktrace: at net.minecraft.client.multiplayer.WorldClient.addWorldInfoToCrashReport(WorldClient.java:410) at net.minecraft.client.Minecraft.addGraphicsAndWorldToCrashReport(Minecraft.java:2509) at net.minecraft.client.Minecraft.run(Minecraft.java:929) at net.minecraft.client.main.Main.main(Main.java:112) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) -- System Details -- Details: Minecraft Version: 1.7.2 Operating System: Windows 7 (amd64) version 6.1 Java Version: 1.7.0, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 791221688 bytes (754 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M AABB Pool Size: 9907 (554792 bytes; 0 MB) allocated, 1337 (74872 bytes; 0 MB) used IntCache: cache: 0, tcache: 0, allocated: 13, tallocated: 95 FML: MCP v9.03 FML v7.2.217.1147 Minecraft Forge 10.12.2.1147 4 mods loaded, 4 mods active mcp{9.03} [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available FML{7.2.217.1147} [Forge Mod Loader] (forgeSrc-1.7.2-10.12.2.1147.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available Forge{10.12.2.1147} [Minecraft Forge] (forgeSrc-1.7.2-10.12.2.1147.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available megakron{1.0} [MegaKron] (bin) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available Launched Version: 1.6 LWJGL: 2.9.0 OpenGL: GeForce GTX 760/PCIe/SSE2 GL version 4.4.0 NVIDIA 344.60, NVIDIA Corporation Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: [] Current Language: ~~ERROR~~ NullPointerException: null Profiler Position: N/A (disabled) Vec3 Pool Size: 3246 (181776 bytes; 0 MB) allocated, 1457 (81592 bytes; 0 MB) used Anisotropic Filtering: Off (1) #@!@# Game crashed! Crash report saved to: #@!@# C:\Users\PC\Desktop\Minecraft mods\1.7.10 MegaKron\eclipse\.\crash-reports\crash-2014-12-04_16.06.48-client.txt 2014-12-04 16:06:48,382 WARN Unable to register shutdown hook due to JVM state AL lib: (EE) alc_cleanup: 1 device not closed[\code]
-
Hi, I'm new to the forum and I am Italian so sorry for my English. I'm working on a mod for Minecraft 1.7.2 and I have to insert a custom tree. The problem is that as soon as I make the tree grow with bonemeal minecraft crash. The error brings me back to the line 58 of the code (the return of the IIcon function) , what's wrong with that line? package megakron.trees; import java.util.List; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import megakron.Megakron; import net.minecraft.block.BlockLeaves; import net.minecraft.client.renderer.texture.IIconRegister; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.IIcon; import net.minecraft.world.World; public class TyreLeaf extends BlockLeaves { public static final String[][] leaftypes = new String[][] {{"leaf_TyreOpaque"},{"leaf_Tyre"}}; public static final String[] leaves = new String[] {"Tyre"}; protected void func_150124_c(World world, int x, int y, int z, int side, int meta) { if((side & 3) == 1 && world.rand.nextInt(meta) == 0) { this.dropBlockAsItem(world, x, y, z, new ItemStack(Megakron.tyreSapling, 1, 0)); } } public int damageDropped(int i) { return super.damageDropped(i) + 4; } public int getDamageValue(World world, int x, int y, int z) { return world.getBlockMetadata(x, y, z) & 3; } @SideOnly(Side.CLIENT) public void getSubBlocks(Item item, CreativeTabs tab, List list) { for(int i = 0; i < leaves.length; i++) { list.add(new ItemStack(item, 1, i)); } } @SideOnly(Side.CLIENT) public void registerBlockIcons(IIconRegister iconRegister) { for(int i = 0; i < leaftypes.length; ++i) { this.field_150129_M[i] = new IIcon[leaftypes[i].length]; for(int j = 0; j < leaftypes[i].length; ++j) { this.field_150129_M[i][j] = iconRegister.registerIcon("megakron:" + leaftypes[i][j]); } } } @Override public IIcon getIcon(int side, int meta) { return (meta & 3) == 1 ? this.field_150129_M[this.field_150127_b][1] : this.field_150129_M[this.field_150127_b][0]; } @Override public String[] func_150125_e() { return leaves; } }