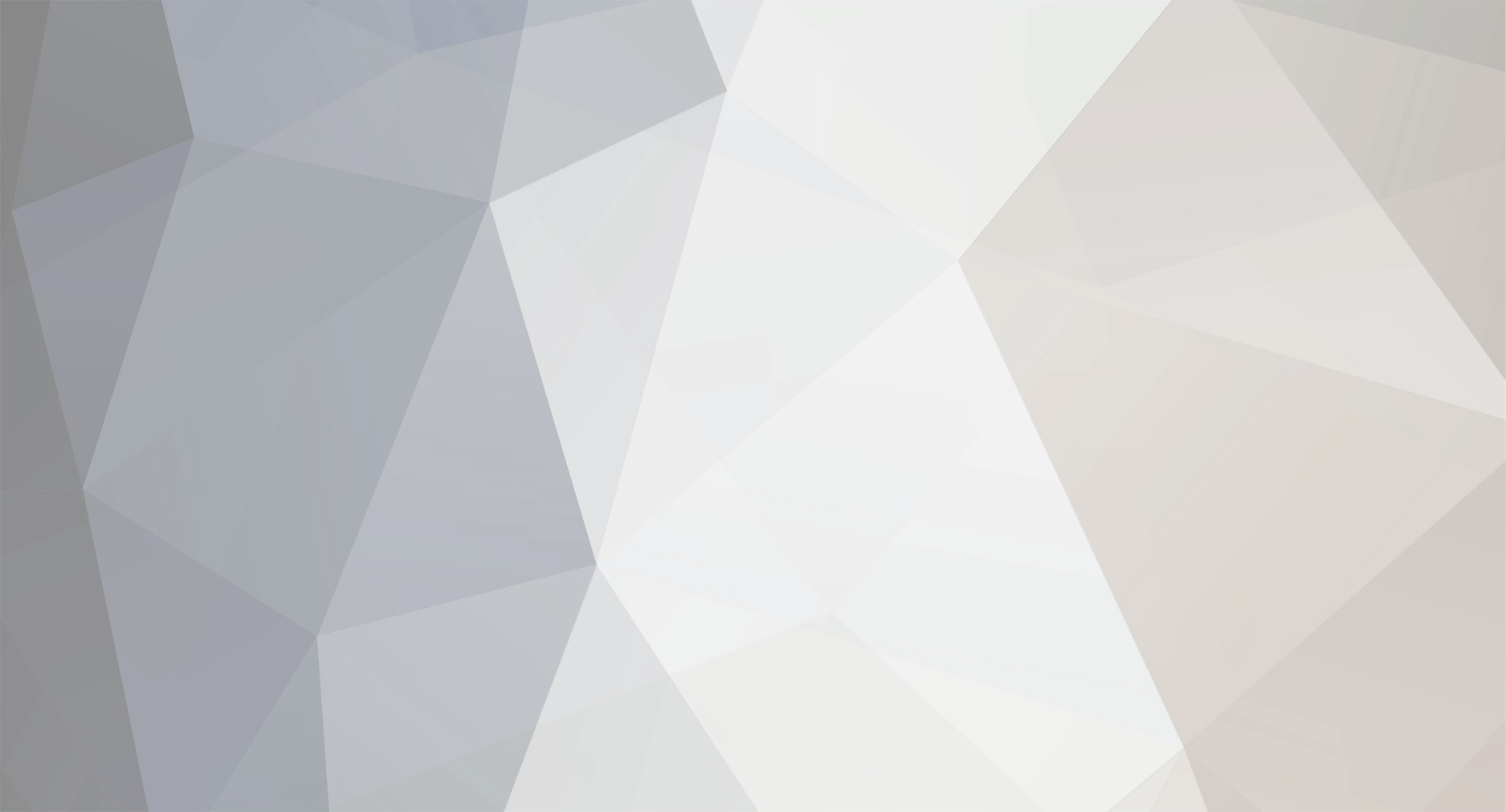
jhonny97
-
Posts
32 -
Joined
-
Last visited
Posts posted by jhonny97
-
-
So instead when I reach a specified age, I should place another crop block on top but with another texture?
-
Hi,
I'm testing a mod idea and I want to create a 3 or 4 block tall crop.
I'm able to create the crop with the collision box of the size that I want, but I didn't find a way to use a taller texture at some stage of growth.
Thanks
-
11 hours ago, diesieben07 said:
ServerPlayerEntity#openSignEditor does one crucial thing: It calls signTile.setPlayer(this) - you don't do that.
I don't know where to place that code, the only place that possibly can go is the DistExecutor, but not work.
I updated the repo to reflect this change.
-
7 minutes ago, diesieben07 said:
Please update your Git repo.
I updated it
-
6 minutes ago, diesieben07 said:
Your edit sign screen sends CUpdateSignPacket to the server when its done. This packet is ignored for anything that is not a SignTileEntity.
I tried also extending the SignTileEntity instead of extending TileEntity but not work.
-
11 minutes ago, diesieben07 said:
The code you posted for your sign TileEntity is not the same as the code in your repository. The code in your repository uses the TileEntityType for the vanilla sign, so your tile entity gets saved as a vanilla sign.
I was doing some testing and i accidentally pushed the changes.
I replaced TileEntityType.SIGN with my custom type, but the result is the same
-
18 minutes ago, diesieben07 said:
This is something that would need to be checked in the debugger. There are so many things that could be wrong here, it is nearly impossible to tell by just staring at incomplete code snippets.
Please post a Git repo of your mod that shows this problem.
This is the Git repo
https://github.com/michele-grifa/MoreStuffMod.git
The branch is the 1.16.1
-
13 minutes ago, ChampionAsh5357 said:
We've already went over that why it doesn't work.
Then you're not saving the information in the TE and/or syncing it to the client on chunk load.
My tile entity extend the SignTileEntity
class CustomSignTileEntity : SignTileEntity() { override fun getType(): TileEntityType<*> { return TileEntityInit.signTileEntity } }
val signTileEntity: TileEntityType<CustomSignTileEntity> by tileEntities.register("my_sign") { TileEntityType.Builder.create({ CustomSignTileEntity() }, BlockInit.mySign, BlockInit.myWallSign).build(null) }
If i extend SignTileEntity, the TE sync shouldn't happen by itself?
-
I used the DistExecutor and the code is now the following
val flag = super.onBlockPlaced(pos, worldIn, player, stack, state) if (worldIn.isRemote && !flag && player != null) { DistExecutor.safeRunWhenOn(Dist.CLIENT) { DistExecutor.SafeRunnable { Minecraft.getInstance().displayGuiScreen(CustomEditSignScreen(worldIn.getTileEntity(pos) as CustomSignTileEntity)) } } } return flag
Now my custom sign screen is opened and i can edit it, but when i exit and re-enter the world, the sign is blank.
If i use
!worldIn.isRemote
instead of
worldIn.isRemote
the game crash even if i use the DistExecutor
-
16 hours ago, ChampionAsh5357 said:
Oh, Kotlin. Haven't seen that here before.
That's quite a simple answer, you're checking to display the screen on the logical server instead of the logical client. You need to check if you're on the logical client and not logical server before calling that method. Also, you shouldn't call the method so directly and instead leave it in some sort of object reference as that piece of code cannot guarantee that it will be executed not on a physical server. Isolate your client only code somewhere else and reference via a DistExecutor.
I tried checking for logical client, but by calling it directly, Minecraft stuck after i place the sign.
I will try with DistExecutor.
-
On 10/18/2020 at 6:08 AM, ChampionAsh5357 said:
Not true, no client synchronization nonsense is going on here. It's the same reason as it was last time when you replaced getSignMaterial within the TER. You are calling EditSignScreen which grabs the material via SignTileEntityRenderer::getMaterial which hardcodes to the vanilla asset library. So, create a custom screen that uses your entry instead of vanilla's.
I had the same problem a while ago, i created the custom sign screen but i don't know how to open the custom screen instead of the vanilla one.
In the ClientPlayerEntity class, the EditSignScreen is hardcodedthis.mc.displayGuiScreen(new EditSignScreen(signTile));
So in a custom SignItem, i cannot use:
player.openSignEditor(worldIn.getTileEntity(pos) as CustomSignTileEntity)
I also tried using this code, but the game crash after placing the sign.
class CustomSignItem(propertiesIn: Properties?, floorBlockIn: Block?, wallBlockIn: Block?) : WallOrFloorItem(floorBlockIn, wallBlockIn, propertiesIn) { override fun onBlockPlaced( pos: BlockPos, worldIn: World, player: PlayerEntity?, stack: ItemStack, state: BlockState ): Boolean { val flag = super.onBlockPlaced(pos, worldIn, player, stack, state) if (!worldIn.isRemote && !flag && player != null) { Minecraft.getInstance().displayGuiScreen(CustomEditSignScreen(worldIn.getTileEntity(pos) as CustomSignTileEntity)) } return flag } }
How i can override them?
-
1 hour ago, ChampionAsh5357 said:
I'm pretty sure it has. That would be due to the placements. To make placements more versatile, they have been separated and allowed to chain on one another. This means that one placement might add more trees to generate, the other may spread them out, and another could change their height coordinate. Take a look at a tree ConfiguredFeature within Features to see what I'm talking about and replicate the chained placements. Note that their order does matter in how they decide to generate.
Your hint about the chaining placement was helpful, using this two placement i'm able to achieve the previous behavior
.withPlacement(Features.Placements.HEIGHTMAP_PLACEMENT) .withPlacement(Placement.field_242902_f.configure(AtSurfaceWithExtraConfig(5, 0.1f, 1))
-
2 hours ago, ChampionAsh5357 said:
Yes, that is true. All entries have a registry key of some kind which can be obtained from the specific registry it's within. They are all singletons, so it doesn't particularly matter that the biome entry is referenced as a key. You can still parse the name of the biome into a registry key with some parent.
The biome would still spawn. This is evident by their implementations across the versions. This can also be seen via Lex's comment on a post to reintroduce the BiomeManager. The only difference is that spawn biomes are specified in the spawn info of each biome itself instead in some list. So, this claim needs some base to be reviewed.
Once again, read what I wrote above. You would need to create a json biome and probably attach the biome via it's registry key to the layer on server start and remove on server stop. A registry key is just a concatenation of the registry and the object registered. You can grab both of these.
Thanks for the explanation.
After a bit of testing, i managed to register and spawn the biome without using json, but now the problem is another.
In this biome i spawn a custom tree that worked until now. Instead of randomly place tree, like vanilla ones, they are placed in a grid (i attached a screenshot).
Nothing has changed in my code since 1.16.1.
This is the code that i use to add the tree to my biome
withFeature( GenerationStage.Decoration.VEGETAL_DECORATION, Feature.TREE.withConfiguration(MyTree.treeConfig) .withPlacement(Placement.field_242902_f.configure(AtSurfaceWithExtraConfig(9, 0.1f, 1))) )
And this is the tree code:
val treeConfig: BaseTreeFeatureConfig = BaseTreeFeatureConfig.Builder( SimpleBlockStateProvider(MyBlocks.log.defaultState), SimpleBlockStateProvider(MyBlocks.leaves.defaultState), BlobFoliagePlacer(FeatureSpread.func_242252_a(2), FeatureSpread.func_242252_a(0), 3), StraightTrunkPlacer(4, 2, 0), TwoLayerFeature(1, 0, 1)) .setIgnoreVines() .build()
This happen with Forge 34.1.17, if i update to the latest Forge version they don't spawn at all.
-
On 10/11/2020 at 6:36 PM, ChampionAsh5357 said:
You answered your own question. As for making it present in an existing dimension, I believe that's still done through BiomeManager. You would probably need to add it when server is about to start as the json will not be populated in the dynamic registries until then.
In 1.16.1 i used to add the biome using this code:
BiomeManager.addBiome(BiomeManager.BiomeType.WARM, BiomeManager.BiomeEntry(biome, 10))
but now BiomeEntry require RegistryKey instead of biome itself and in 1.16.3 don't spawn anymore.
-
Hi, i created added a custom biome in minecraft 1.16.1 and works perfectly, but now updating to 1.16.3, all of the biome code is not working, like BiomeDictionary that is not present anymore. I know of the change made by Mojang in 1.16.3 for the data driven biomes, but how i can convert this biome to the new version?
-
19 minutes ago, killerjdog51 said:
How did you add to the sign atlas? I'm experiencing the same issue.
You have to add sprite in TextureStitchEvent.Pre Event
-
Thanks, it worked perfectly
-
On 8/20/2020 at 1:50 AM, ChampionAsh5357 said:
From there, you can figure it out.
Learn 3d dimensional rotation, translation, and scaling based around a specific point. From there, take a look at how stacks work. For mc in particular, take a look at how they compress textures into an atlas (the sign type points to a location holding the sign textures). Now you have a decent understanding on how rendering works without understanding the complications of rendering types, culling, raytracing, overlays, etc. You probably won't need to know this anyways, but its always helpful.
Ok thanks, i resolved using only using a custom Renderer for the sign and adding the sprite to the atlas and it works. the only problem is the EditSignScreen that show the default Oak WoodType instead of my texture because the screen, call "SignTileEntity.getMaterial" that search for the texture in the minecraft namespace and not my mod namespace. I tried creating a new EditSignScreen, but unfortunately when i try to display the screen, Minecraft crash without error. I checked the original call to display the gui and is done Server side, maybe there is some sort of synchronization between server and client that i have to implement myself.
-
21 hours ago, ChampionAsh5357 said:
Look into IFluidHandler::fill more, it should jump at you in the form of replicating ItemStacks.
i checked more the IFluidHandler::fill but i can't figure out what to do. I the fill method i have only the fluidstack to insert and the action for the fill. If you were a little more specific, I would appreciate it.
-
Have you tried in IntelliJ IDEA to do the Invalidate Caches / Restart under the File menu?
Could you also share the build.gradle file content?
-
1.8 is no longer supported on this forum.
Update to a newer version
-
-
I created a custom block with a TileEntity that implements IFluidHandler and it works good, but i want to know if there is a way to tell the input handler to only accept a specific amount of fluid per tick
-
1 hour ago, ChampionAsh5357 said:
Besides the abysmal programming + copy-paste, it's probably because your texture doesn't exist in the specified Atlas location. I believe it even tells you that in the stacktrace/log when it tries to find it. Read over it again and fix. If you're lucky with this very cutout code, you won't even need to use TextureStitchEvent$Pre.
I copy-pasted the renderer from the original sign renderer, because afaik I cannot instantiate a new woodtype, that has a protected constructor. So i overrided the getRenderMaterial to find my texture and there isn't a error in the log.
How can I add that texture to the Atlas? There are some resources where I can learn this type of things about rendering and so on?
[1.19] Create 3 or 4 block tall crop
in Modder Support
Posted
Ok, thanks for the hint. I will look into it.