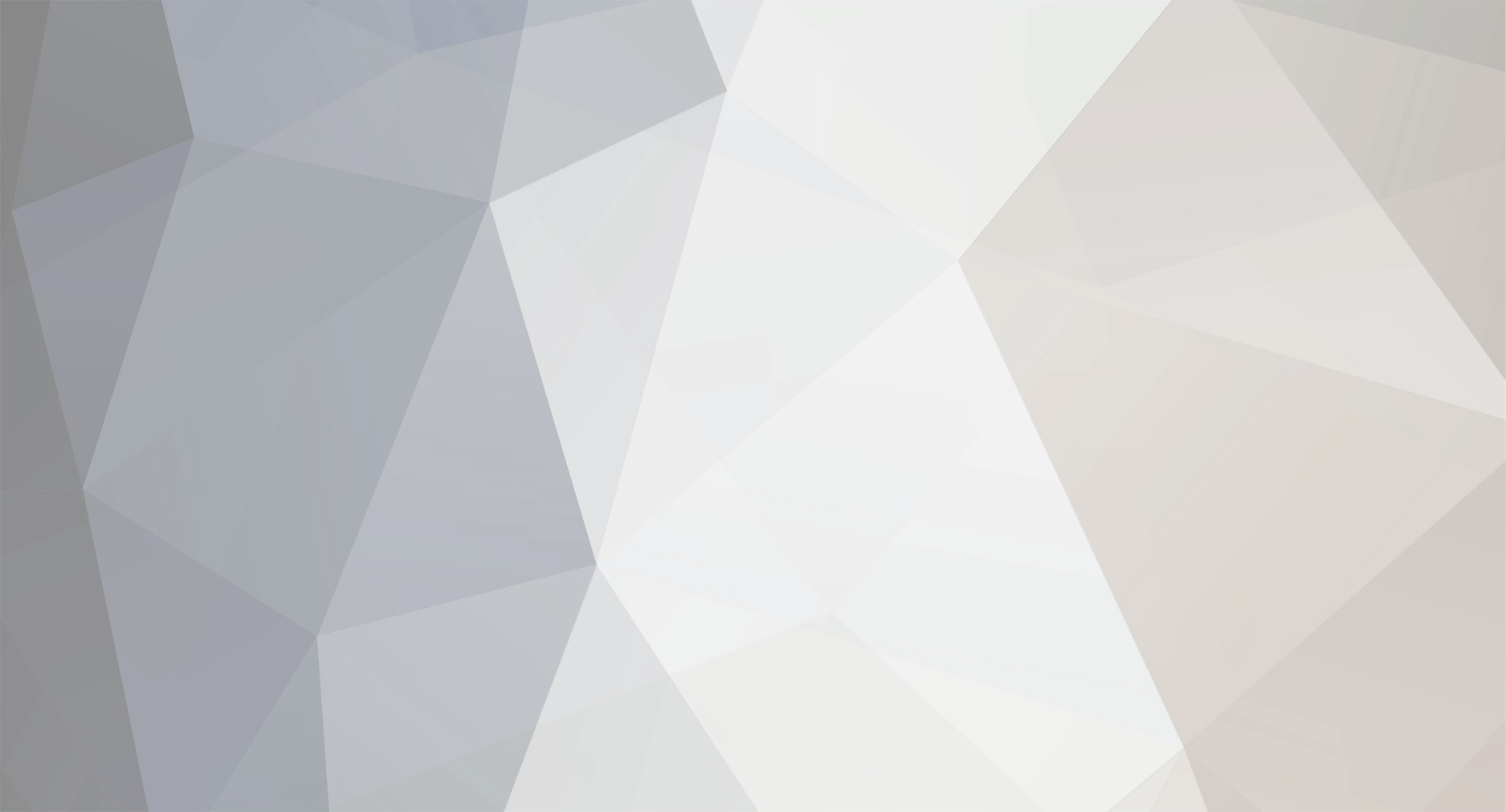
jhonny97
-
Posts
32 -
Joined
-
Last visited
Posts posted by jhonny97
-
-
Thanks it worked
-
I have a block with a different front texture.
When i place down this block, the front is facing me. The left side for me, is the left side of the front.
I don't know if I've made myself clear
-
Hi, i have a problem with the getCapability method. I created a custom tile entity with a FluidHandler and an ItemStackHandler capability and i want to restrict fluid only on the left side of the block.
The problem is that the "side" parameter that is present, is not the side of the block, instead is the global facing.
This is my code
@Nonnull @Override public <T> LazyOptional<T> getCapability(@Nonnull Capability<T> cap, Direction side) { if(cap == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) { if(side == Direction.UP || side == Direction.NORTH) { return inputHandler.cast(); } else if(side == Direction.DOWN || side == Direction.EAST) { return outputHandler.cast(); } } if(cap == CapabilityFluidHandler.FLUID_HANDLER_CAPABILITY) { if(side == Direction.WEST) { return fluidHandler.cast(); } } return LazyOptional.empty(); }
How i can check the real side of the block?
-
You can find the code at this link: https://github.com/michele-grifa/MoreStuffMod
The branch is the 1.16.1
Another problem is that in the TileEntityRender, my custom texture for the Sign don't get loaded.
Thanks
-
Hi, i'm new to modding and i created a new different tree type. Now i created all the associated blocks, but i have some trouble creating a new Sign.
I extended the SignBlock class and the SignTileEntity and binded the TileEntityRenderer and all works good, but when i place down the sign the GUI for the text not show.
I'm missing something?
I'm using Minecraft 1.16.1 with Forge 32.0.98
-
Hello guys I installed FML and Car Mod, but when I start Minecraft the game freezes and I view this error:
3 mods loaded
Minecraft Forge 3.3.7.135
FML v2.2.48.135
Forge Mod Loader version 2.2.48.135 for Minecraft 1.2.5
mod_MinecraftForge : Pre-initialized (minecraft.jar)
mod_ModLoaderMp : Pre-initialized (minecraft.jar)
mod_cars : Loaded (1.2.4 carmod v2.2.zip)
Minecraft has crashed!
----------------------
Minecraft has stopped running because it encountered a problem.
--- BEGIN ERROR REPORT 69360557 --------
Generated 11/09/12 17.15
Minecraft: Minecraft 1.2.5
OS: Windows 7 (x86) version 6.1
Java: 1.7.0_05, Oracle Corporation
VM: Java HotSpot Client VM (mixed mode), Oracle Corporation
LWJGL: 2.4.2
OpenGL: Mobile Intel® HD Graphics version 3.0.0 - Build 8.15.10.2279, Intel
cpw.mods.fml.common.LoaderException: java.lang.IllegalAccessException: Class cpw.mods.fml.common.modloader.ModLoaderModContainer can not access a member of class mod_cars with modifiers "public"
at cpw.mods.fml.common.modloader.ModLoaderModContainer.preInit(ModLoaderModContainer.java:114)
at cpw.mods.fml.common.Loader.preModInit(Loader.java:235)
at cpw.mods.fml.common.Loader.loadMods(Loader.java:593)
at cpw.mods.fml.client.FMLClientHandler.onPreLoad(FMLClientHandler.java:193)
at net.minecraft.client.Minecraft.a(Minecraft.java:383)
at net.minecraft.client.Minecraft.run(Minecraft.java:735)
at java.lang.Thread.run(Unknown Source)
Caused by: java.lang.IllegalAccessException: Class cpw.mods.fml.common.modloader.ModLoaderModContainer can not access a member of class mod_cars with modifiers "public"
at sun.reflect.Reflection.ensureMemberAccess(Unknown Source)
at java.lang.Class.newInstance0(Unknown Source)
at java.lang.Class.newInstance(Unknown Source)
at cpw.mods.fml.common.modloader.ModLoaderModContainer.preInit(ModLoaderModContainer.java:107)
... 6 more
--- END ERROR REPORT 4eb853a7 ----------
I commend to you the attached file ForgeModLoader-0.
Problem creating custom sign type
in Modder Support
Posted · Edited by jhonny97
Hi,
in my mod in Minecraft 1.15.2, i added a new type of wood and i want to create the sign for it, but when i place down the block, it has no texture and the GUI don't open.
I'm missing something that i don't know?
Here is the TileEntityClass
The Block initialization
The tile entities initialization
And the TileEntityRenderer
And the setup event
private void setup(final FMLCommonSetupEvent event) { ClientRegistry.bindTileEntityRenderer(TileEntityInit.japCherrySignTileEntity.get(), JapaneseCherrySignTileEntityRenderer::new); }