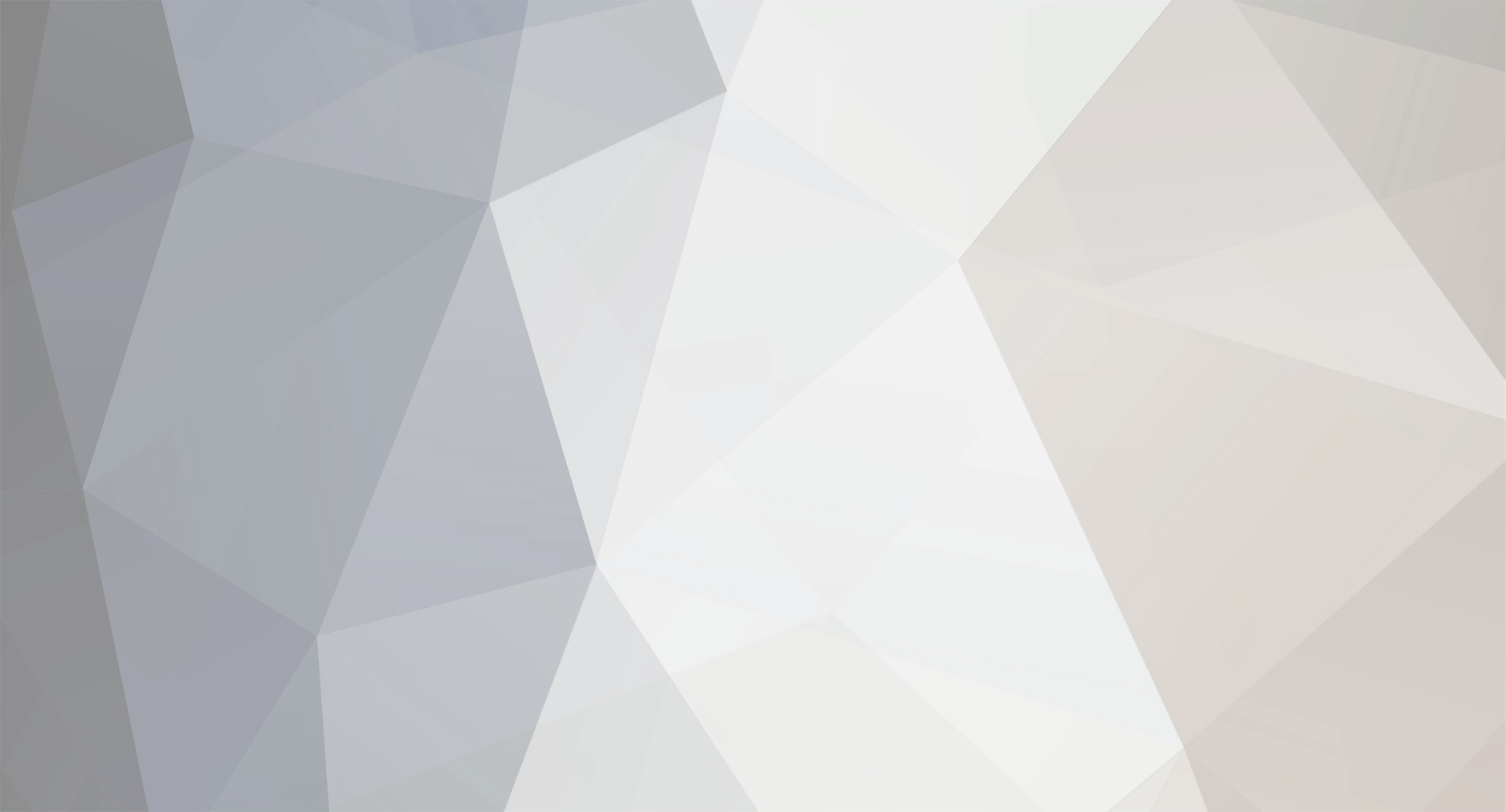
BinaryCrafter
Members-
Posts
8 -
Joined
-
Last visited
Everything posted by BinaryCrafter
-
Thanks. I did spot the AIs, but wasn't expecting to find anything there and focused on other class members. I'll take another look. Many thanks for the suggestion.
-
I'm iterating through mobs that are spawned within a specific range of each player, and am trying to identify which of the mobs are neutral mobs (i.e. those will that are normally passive, but will turn aggressive towards a player if provoked, e.g. Wolf, Zombie Pigman). I've looked at the class definitions of those mobs hoping to find an interface or property that I could check for, but haven't managed to find a fool-proof way of identifying neutral mobs. Can anyone offer any advice? I'll resort to hard coding if I really must, but I'd much prefer to do it properly.
-
Storage block loses contents when block moved
BinaryCrafter replied to BinaryCrafter's topic in Modder Support
For anyone else having a similar problem, I've managed to resolve this. I've added the following line to my onBlockPlacedMethod world.setTileEntity(pos, siloTileEntity); So it now looks like this: @Override public void onBlockPlacedBy(World world, BlockPos pos, IBlockState blockState, EntityLivingBase entityPlayer, ItemStack itemStack) { super.onBlockPlacedBy(world, pos, blockState, entityPlayer, itemStack); TileEntity blockTileEntity = world.getTileEntity(pos); if (blockTileEntity != null && blockTileEntity instanceof SiloTileEntity) { SiloTileEntity siloTileEntity = (SiloTileEntity) blockTileEntity; if (itemStack.hasTagCompound()) { NBTTagCompound tag = itemStack.getTagCompound(); siloTileEntity.readFromNBT(tag); siloTileEntity.markDirty(); world.setTileEntity(pos, siloTileEntity); } } } -
I have a simple block used as item storage in game, and I've added methods to the block to enable the TileEntity to be broken with a pick and placed elsewhere in the world, preserving the inventory within the storage block. All works fine, however there is a problem that can cause the inventory of the block to be cleared, the steps to reproduce this are as follows: 1. add item(s) to the inventory of the storage block 2. break the storage block 3. place the storage block back in the world (in a different location) 4. Inventory in the storage block has moved with the block correctly 5. quit the game and re-launch 6. Inventory in the storage block is lost Mitigating actions: there are no save issues unless the storage block is moved if the block is broken and placed in the exact same location, then the inventory seems to persist between saves fine if the player continues to play for an indeterminate amount of time (i.e. doesn't quit immediately), the inventory of the moved storage block will persist between saves It's the first time I've tried to have a block with a TileInventory persist after the block is broken using NBT, so I'm sure I'm getting something wrong, though I have used this post to guide me. Any help understand what I'm doing wrong would be appreciated. Relevant code: @Override public void breakBlock(World world, BlockPos blockPos, IBlockState blockState) { NBTTagCompound tag = new NBTTagCompound(); TileEntity blockTileEntity = world.getTileEntity(blockPos); blockTileEntity.writeToNBT(tag); ItemStack blockItem = new ItemStack(Item.getItemFromBlock(this), 1, this.damageDropped(blockState)); EntityItem entityitem = new EntityItem(world, blockPos.getX(), blockPos.getY(), blockPos.getZ(), blockItem); entityitem.getEntityItem().setTagCompound(tag); world.spawnEntityInWorld(entityitem); } @Override public void onBlockPlacedBy(World world, BlockPos pos, IBlockState blockState, EntityLivingBase entityPlayer, ItemStack itemStack) { super.onBlockPlacedBy(world, pos, blockState, entityPlayer, itemStack); TileEntity blockTileEntity = world.getTileEntity(pos); if (blockTileEntity != null && blockTileEntity instanceof SiloTileEntity) { SiloTileEntity siloTileEntity = (SiloTileEntity) blockTileEntity; if (itemStack.hasTagCompound()) { NBTTagCompound tag = itemStack.getTagCompound(); siloTileEntity.readFromNBT(tag); siloTileEntity.markDirty(); } } }
-
Just wondering why the instance method block.isAir (as an example from many methods) takes parameters in the method call, when the instance of the class already has the required properties. I've just looked at the de-obfuscated source code of net.minecraft.block.Block and as I suspected the parameters are unused. There appear to be other examples in this class, and I'm sure I've seen it in other classes too. It would make sense to require the parameters if it were a static method, but myself being an experienced software developer that's fairly new to Minecraft Forge modding, I'm just trying to understand if there's some history or a reason why it's like this?
-
I'm developing what will hopefully be a simple mod, which is a simple item storage based mod, that I'm calling DeepStorageChest. It's essentially intended to be a mash up of a chest and a DeepStorageUnit from the mod MineFactory Reloaded. As I'm sure you know, DeepStorageUnit allows for lots of storage of one particular item type, whereas, I want to allow lots of storage of multiple item types. Essentially, I'm creating a chest with 27 / 54 slots, but slots aren't limited by the item's maximum stack size. e.g. Cobblestone has a MaxStackSize of 64, EnderPearls 16, etc, but I want my storage to exceed this limit. I've created my own TileEntity and Container classes for the storage block, and overriden methods such that I am able to shift click items to my storage block and exceed the normal MaxStackSize limit. The problem I'm finding, is that the only way I can allow a slot to contain more than MaxStackSize items per slot is by shift clicking items in to the inventory, if I click drag to move them or use a hopper to place items into my container, then the MaxStackSize for the item is honoured, which is not what I want. Can anybody explain how I might be able to accomplish this, as I can't find any methods I can override might allow me to be able to do this? I'm building the mod for Forge 1.7.10, if that's relevant, source code so far is on GitHub (https://github.com/WalterWeight/DeepStorageChest)