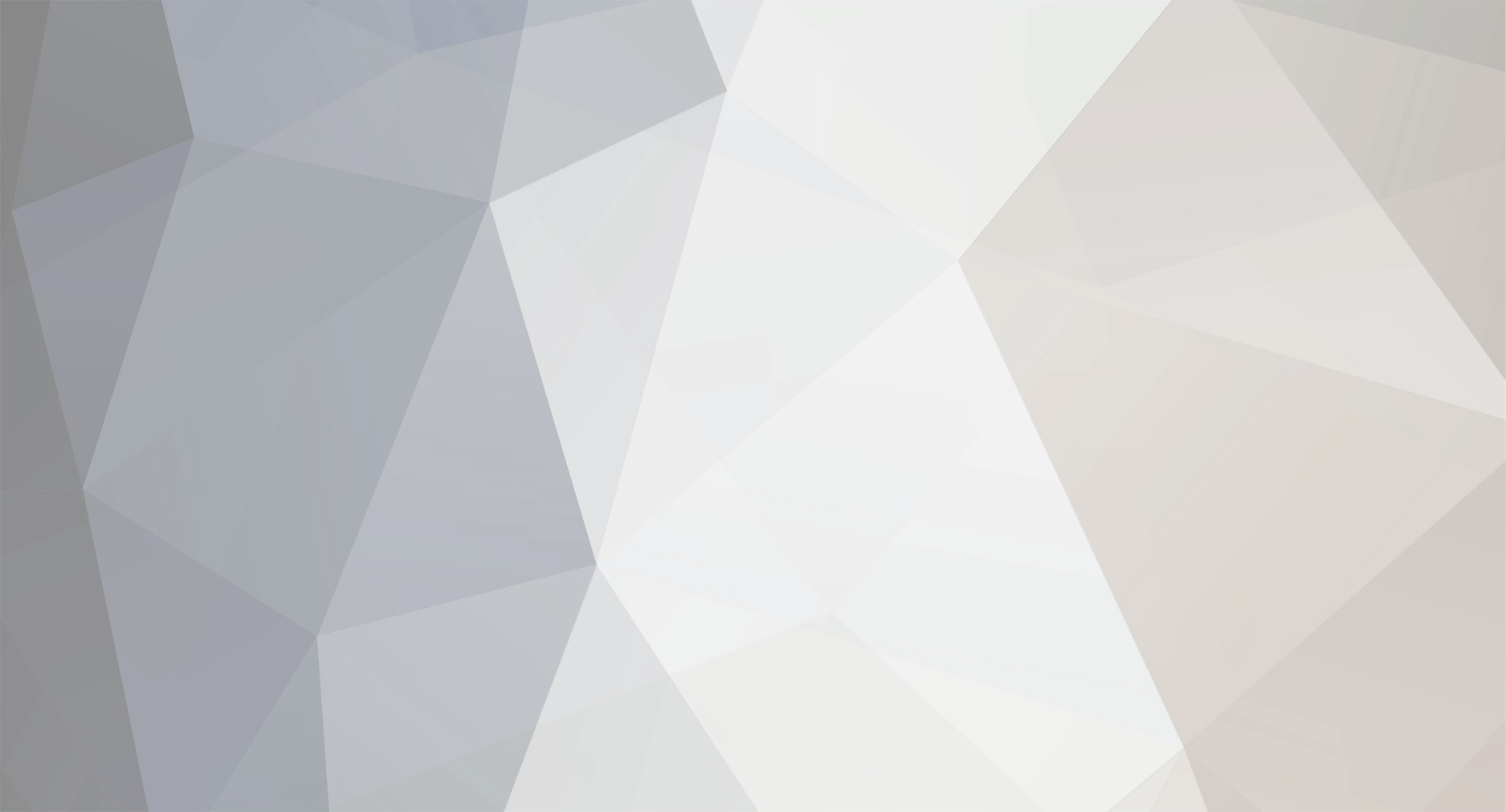
blowuptheocean
Members-
Posts
1 -
Joined
-
Last visited
Converted
-
Gender
Undisclosed
-
Personal Text
I am new!
blowuptheocean's Achievements

Tree Puncher (2/8)
0
Reputation
-
So let me preface this by saying that I'm a bit new to modding in Minecraft and I've been slowly learning Java on the side. I'm currently working on a mod that goes through just about everything there is to do with coding a mod (bit by bit!) and I've become stuck with custom entity models. I followed several tutorials and tried to use all the info I found to get this right but I keep getting the same error and I'm not entirely sure why. I'm sorry I don't have a github repository but if anyone could help me figure out what's going on it'd be greatly appreciated! The error pops up as soon as I spawn my custom entity, the renderer has an issue stating that it can't find the entity texture. at com.blowuptheocean.rpgworld.entities.RenderRpgGoblin.getEntityTexture(RenderRpgGoblin.java:22) followed by: Stacktrace: at net.minecraft.client.renderer.entity.RenderManager.func_147939_a(RenderManager.java:339) at net.minecraft.client.renderer.entity.RenderManager.renderEntityStatic(RenderManager.java:278) at net.minecraft.client.renderer.entity.RenderManager.renderEntitySimple(RenderManager.java:251) at net.minecraft.client.renderer.RenderGlobal.renderEntities(RenderGlobal.java:527) at net.minecraft.client.renderer.EntityRenderer.renderWorld(EntityRenderer.java:1300) at net.minecraft.client.renderer.EntityRenderer.updateCameraAndRender(EntityRenderer.java:1087) at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1056) at net.minecraft.client.Minecraft.run(Minecraft.java:951) at net.minecraft.client.main.Main.main(Main.java:164) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:78) at GradleStart.main(GradleStart.java:45) Now, I've placed the texture where it should be and with the correct name I believe. I've tried changing things around a bunch with no luck...Here are my files if anyone has a second to look over them! Client Proxy: package com.blowuptheocean.rpgworld; import net.minecraft.client.Minecraft; import com.blowuptheocean.rpgworld.entities.EntityRpgGoblin; import com.blowuptheocean.rpgworld.entities.RenderRpgGoblin; import com.blowuptheocean.rpgworld.entities.RpgGoblin; import cpw.mods.fml.client.registry.RenderingRegistry; import cpw.mods.fml.common.FMLCommonHandler; public class ClientProxy extends ServerProxy{ public void registerRenderThings(){ RenderingRegistry.registerEntityRenderingHandler(EntityRpgGoblin.class, new RenderRpgGoblin(new RpgGoblin(), 0)); FMLCommonHandler.instance().bus().register(new ServerTickHandler(Minecraft.getMinecraft())); } } Main File: package com.blowuptheocean.rpgworld; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; import com.blowuptheocean.rpgworld.blocks.RpgBlocks; import com.blowuptheocean.rpgworld.entities.RpgEntity; import com.blowuptheocean.rpgworld.handler.EventManager; import com.blowuptheocean.rpgworld.handler.RpgRecipes; import com.blowuptheocean.rpgworld.help.Reference; import com.blowuptheocean.rpgworld.items.RpgItems; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.EventHandler; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.registry.GameRegistry; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; @Mod(modid = Reference.MODID, version = Reference.VERSION) public class RPGWorld { //Establish Proxies// @SidedProxy(clientSide = "com.blowuptheocean.rpgworld.ClientProxy", serverSide = "com.blowuptheocean.rpgworld.ServerProxy") public static ServerProxy proxy; //Establish Entities// //Establish World Generators// public static EventManager rpgcopperGen = new EventManager(); //Establish Creative Tabs// public static CreativeTabs rpgItemTab = new CreativeTabs(CreativeTabs.getNextID(), "rpg_items") { @SideOnly(Side.CLIENT) public Item getTabIconItem() { return RpgItems.copperIngot; } }; public static final CreativeTabs rpgBlockTab = new CreativeTabs(CreativeTabs.getNextID(), "rpg_blocks") { @SideOnly(Side.CLIENT) public Item getTabIconItem() { return RpgItems.copperDust; } }; public static final CreativeTabs rpgGearTab = new CreativeTabs(CreativeTabs.getNextID(), "rpg_gear") { @SideOnly(Side.CLIENT) public Item getTabIconItem() { return RpgItems.copperPickaxe; } }; //EVENT HANDLERS// @EventHandler public void preInit(FMLPreInitializationEvent event) { //Register Renderer// proxy.registerRenderThings(); //Register Entities? // RpgEntity.loadEntities(); //Block handlers, handles all blocks RpgBlocks.loadBlocks(); //Item handlers, handles all items RpgItems.loadItems(); //Register handler, adds all the recipes RpgRecipes.addRecipes(); //World Gen Registry // GameRegistry.registerWorldGenerator(rpgcopperGen, 1); } } My EntityGoblin code: package com.blowuptheocean.rpgworld.entities; import net.minecraft.entity.EntityAgeable; import net.minecraft.entity.SharedMonsterAttributes; import net.minecraft.entity.ai.EntityAIPanic; import net.minecraft.entity.ai.EntityAITempt; import net.minecraft.entity.ai.EntityAIWander; import net.minecraft.entity.monster.EntityMob; import net.minecraft.entity.passive.EntityAnimal; import net.minecraft.init.Items; import net.minecraft.world.World; public class EntityRpgGoblin extends EntityMob{ public EntityRpgGoblin(World par1World) { super(par1World); this.setSize(0.7F, 0.4F); this.tasks.addTask(0, new EntityAIWander(this, 0.5D)); this.tasks.addTask(1, new EntityAIPanic(this, 1.D)); this.tasks.addTask(2, new EntityAITempt(this, 0.8D, Items.apple, false)); } public boolean isAIEnabled(){ return true; } protected void applyEntityAttributes(){ super.applyEntityAttributes(); this.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(26); this.getEntityAttribute(SharedMonsterAttributes.movementSpeed).setBaseValue(0.5D); } } RenderGoblin code: package com.blowuptheocean.rpgworld.entities; import com.blowuptheocean.rpgworld.help.Reference; import net.minecraft.client.model.ModelBase; import net.minecraft.client.renderer.entity.RenderLiving; import net.minecraft.entity.Entity; import net.minecraft.util.ResourceLocation; public class RenderRpgGoblin extends RenderLiving{ private static final ResourceLocation mobTextures = new ResourceLocation(Reference.MODID + "textures/entity/EntityRpgGoblin.png"); public RenderRpgGoblin(ModelBase par1ModelBase, float parShadowSize) { super(par1ModelBase, parShadowSize); } protected ResourceLocation getEntityTextures(EntityRpgGoblin entity){ return mobTextures; } protected ResourceLocation getEntityTexture(Entity entity){ return this.getEntityTexture((EntityRpgGoblin)entity); } } Entity Registration code: package com.blowuptheocean.rpgworld.entities; import java.util.Random; import net.minecraft.entity.EntityList; import net.minecraft.entity.EnumCreatureType; import net.minecraft.world.biome.BiomeGenBase; import com.blowuptheocean.rpgworld.help.Reference; import com.blowuptheocean.rpgworld.help.RegisterHelper; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.registry.EntityRegistry; public class RpgEntity { public static void loadEntities(){ registerEntity(); } public static void registerEntity(){ createEntity(EntityRpgGoblin.class, "Goblin", 0x91C4FF, 0xFFCC91); } public static void createEntity(Class entityClass, String entityName, int solidColor, int spotColor){ int randomId = EntityRegistry.findGlobalUniqueEntityId(); EntityRegistry.registerGlobalEntityID(entityClass, entityName, randomId); EntityRegistry.registerModEntity(entityClass, entityName, randomId, Reference.MODID, 64, 1, true); EntityRegistry.addSpawn(entityClass, 2, 0, 1, EnumCreatureType.monster, BiomeGenBase.forest); createEgg(randomId, solidColor, spotColor); } private static void createEgg(int randomId, int solidColor, int spotColor){ EntityList.entityEggs.put(Integer.valueOf(randomId), new EntityList.EntityEggInfo(randomId, solidColor,spotColor)); } } And then I have the actually goblin java model code from Techne! The texture is located in main/resources/assets/rpgworld/textures/entity/ and I've changed the name of the file around just to make sure it's not something weird with the name, though it could be part of the issue. (right now it's set to EntityRpgGoblin.png) Sorry for the lengthy post, just hoping someone can help me out! Also if there is a better way to organize a post like this, feel free to let me know!