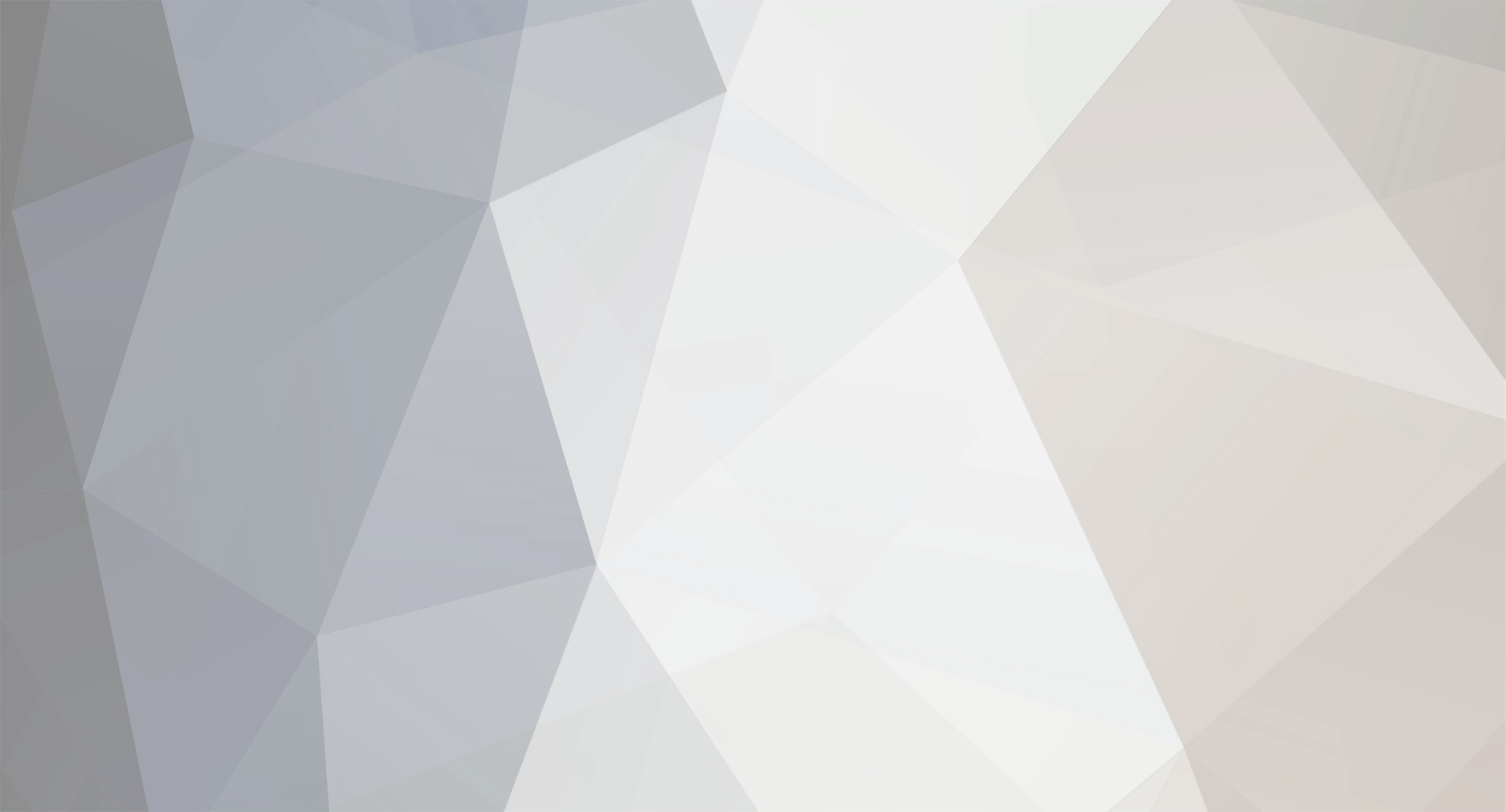
Bystander
Members-
Posts
6 -
Joined
-
Last visited
Everything posted by Bystander
-
On the original question, A trick often used in games is to use additive blending rather than src (1-dest). Use GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE); rather than GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA); Standard src (1-dest) isn't commutative meaning it has to be done in the correct order whereas additive can be done in any order - it also has the nice side effect of saturating the colours quite quickly the more that are overlaid. Though from looking at the first image it looks like z writes are enabled on the beams - which is another issue. Particles or anything transparent should be rendered with z tests on and z writes off unless you sort them. Sorting large transparent objects is quite complex and needs some kind of separating axis test - hence the prevalent use of additive alpha and no z writes.
-
Try @Instance("MyModName")
-
[1.7.10] Tile Entity Owned Resources and shouldRefresh
Bystander replied to Bystander's topic in Modder Support
Oh, by the way for anyone else doing this (looping audio), I solved the resource retention issue the simpler way in the end. A keyed registry seemed like overkill and there's already a Minecraft ITickableSound interface the sound manager checks for. You just need to implement isDonePlaying on the PositionedSound object and in that check back with the parent TileEntity. The only thing that annoys me about the solution is the call to GetTileEntity() each sound manager tick - seems like overkill. A quick guide to looping audio in 1.7.10 - in so far as it works for me. Define this and Implement it on your Tile Entity. public interface IShouldLoop { boolean continueLoopingAudio(); } Create this class. public class LoopingSound extends PositionedSound implements ITickableSound { private int x,y,z; private boolean shouldBePlaying = true; public LoopingSound(ResourceLocation resource,TileEntity parent) { super(resource); repeat = true; xPosF = parent.xCoord+0.5f; yPosF = parent.yCoord+0.5f; zPosF = parent.zCoord+0.5f; x = parent.xCoord; y = parent.yCoord; z = parent.zCoord; } @Override public void update() { } @Override public boolean isDonePlaying() { if (shouldBePlaying) { // we should only be playing if parent still exists and says we are playing - assume not shouldBePlaying = false; // should never be here on the server, but just in case World world = Minecraft.getMinecraft().theWorld; if (world.isRemote) { TileEntity parent = world.getTileEntity(x, y, z); if (parent != null) { if (parent instanceof IShouldLoop) { IShouldLoop iShouldLoop = (IShouldLoop)parent; shouldBePlaying = iShouldLoop.continueLoopingAudio(); } } } } return !shouldBePlaying; } } You kick the loop off like this (has to be client side obviously). ResourceLocation audioLoop = new ResourceLocation("mymod","loopname"); LoopingSound myLoop = new LoopingSound(audioLoop,this); Minecraft.getMinecraft().getSoundHandler().playSound(myLoop); The sound files are specified the same way you'd do normal one shot audio, i.e. they go in assets/mymod/sounds/ (use .ogg format) Create a sounds.json in assets/mymod - use the format of the minecraft one as a template or use something like this. { "loopname": { "category": "master", "sounds": [ "loopname" ] }, "bigswitch": { "category": "master", "sounds": [ "bigswitch" ] } } -
[1.7.10] Tile Entity Owned Resources and shouldRefresh
Bystander replied to Bystander's topic in Modder Support
Thanks, the delegation in your diagram makes the relationship a lot clearer. -
[1.7.10] Tile Entity Owned Resources and shouldRefresh
Bystander replied to Bystander's topic in Modder Support
One more bit of info for anyone else who ends up in this thread as it was confusing me why this behaviour didn't cause all kinds of other problems in mods. Specifically when this refresh happens the orphaned TE appears to get no warning, no chance to flush or store state and there's no copy constructor call on the new TE to transfer state. It seemed to me like any mod that stores inventory on a TE for instance would be screwed. The reality is the block is only orphaned/removed on the client side, and then only if something changes the block meta data, writing to it or even marking the block for update isn't enough to trigger the tile entity refresh. I can still imagine it causing all kinds of client side hazards for any TE using metadata to transfer data to the client - I might try writing an evil block that forces fake block meta data updates on neighbouring blocks. -
This has probably been solved a million times so hopefully somebody can point me the right way. One of my Tile Entities has a looping sound - as in a proper looping ISound, not one I restart after some ticks. I'm using a custom PositionedSound class to do this and setting repeat from within it. I have logic to start and stop the looping audio using the SndManager which all works fine. I have to create an instance of the PositionedSound on the TileEntity and hang onto it in order to be able to stop it. The problem comes when my tile entity gets a block update. This triggers a call to shouldRefresh() which by default returns true for all non vanilla tile entities. What happens then is that the tile entity is refreshed - meaning a new one is made and the old one is orphaned - meaning the reference to the owned resource goes with it. I guess most people probably don't notice this happening if all their state is in NBT, however this tile entity needs to hang on to the owned PositionedSound resource. Without it there is no way to stop the sound looping. I could force shouldRefresh to return false like a vanilla TE, but it's decorated with plenty of warnings about not doing this. Plus I'm guessing this isn't the only way TEs are refreshed so it may not even be a solution. Is the only solution here to make my own registry or is there an accepted way of passing owned resources to your predecessor on refresh?