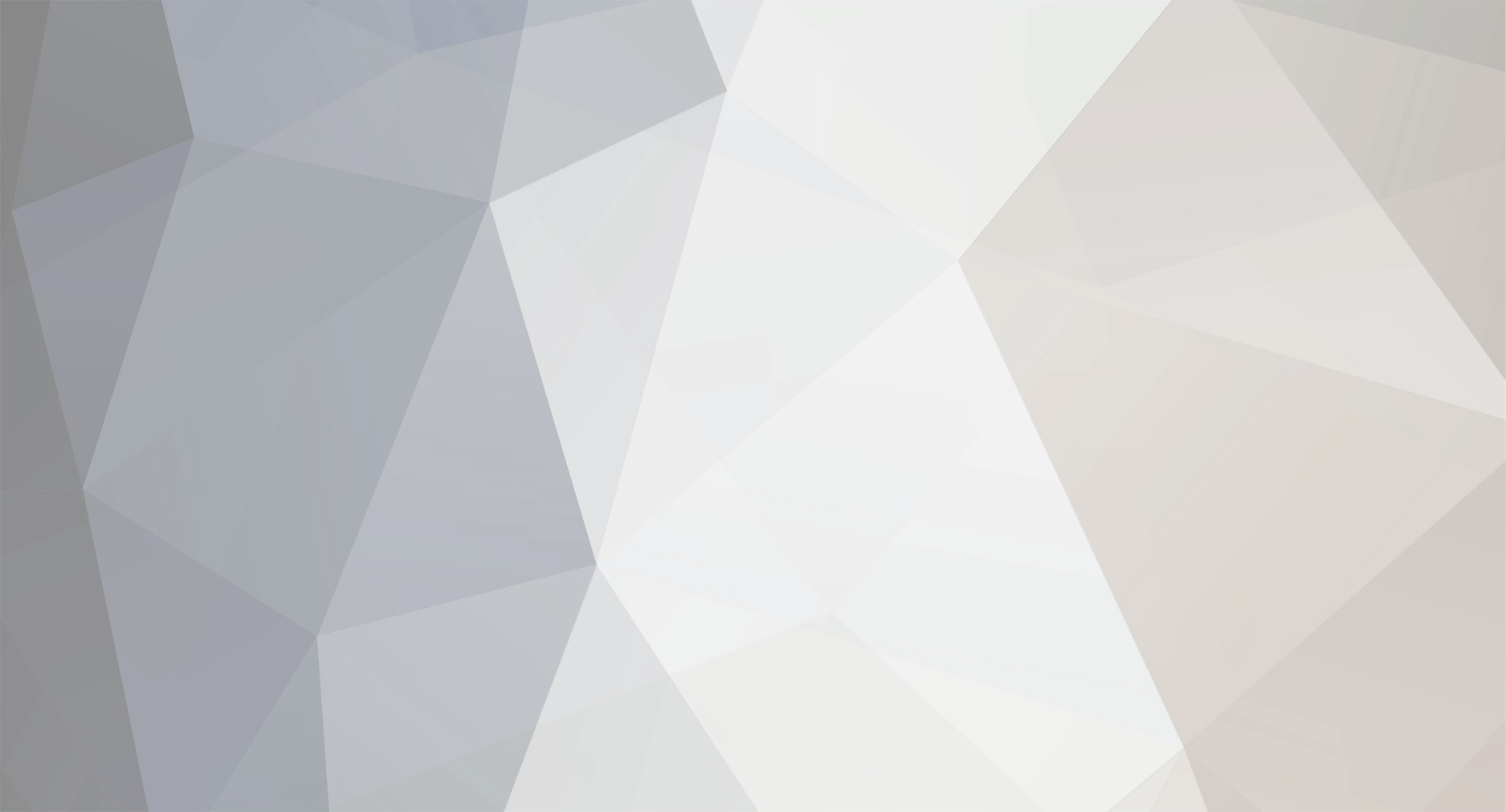
daruskiy
Members-
Posts
30 -
Joined
-
Last visited
Everything posted by daruskiy
-
I have, and as I said above the action that I need are a combination of the log and of the piston/dispenser. The BlockLog, BlockRotatedPillar, BlockHay all used the same logic where when you place it rotates the texture in the same way so if you had half a block blue and half a block red, regardless the direction NSEW you place it, the colors will be in the same order. I am trying to figure out how to make my code work for example: the bottom texture is solid blue, when I place it on the groudn regardless of direction facing, the the blue side is on the ground. when I place it on the ceiling, the blue texture is on the top. When i place on the wall, the blue texture needs to be facing the wall. At the moment with the code I got from the dispenser, what is happening even if i place the block on the ground, the blue side will rotate to match the axis that I am facing, i.e. like the dispenser facing the direction you placed it. Hopefully this is clear now. Any suggestinos appreciated
-
Copy of the dispenser { "variants": { "facing=down": { "model": "respublica:freize0", "x": 180 }, "facing=up": { "model": "respublica:freize0" }, "facing=north": { "model": "respublica:freize0" }, "facing=south": { "model": "respublica:freize0", "y": 180 }, "facing=west": { "model": "respublica:freize0", "y": 270 }, "facing=east": { "model": "respublica:freize0", "y": 90 } } } However it is irrelevant as what is wrong is the actual action that being triggered in game. When i place anything on the ground, I want it to always be right side up. When I place anything on the ceiling or underneath a block, it needs to upside down. In the other cases wen placing on walls it needs to use the directional north east south west rules. At the moment what is happening is like piston placing and dispenser facing, when i place the block on the ground, it starts to follow the NSEW rules and rotates the block to look at me
-
I am trying to achieve blocking placement that when placed on the ground and ceiling, only flips its sides textures 180 degrees, kind of like a log. But then when placing it on a wall, it rotates the side texture 90 degrees with each direction. At the moment I have copied dispenser code to handle the rotation, but what is happening is that if I am placing the block on the ground, it will still rotate 90 degrees based on the direction instead of only doing so when placing it on a wall. Essentially what I want is rotation to act like pistons/dispensers when on walls, and to only invert the texture if its placed on the floor or ceiling. Any suggestions appreciated. public class blockFreize extends blockBase { //public static final PropertyDirection FACING = PropertyDirection.create("facing", EnumFacing.Plane.HORIZONTAL); public static final PropertyDirection FACING = BlockDirectional.FACING; public blockFreize(String name, Material material) { super(name, Material.IRON); this.setHardness(3f); this.setResistance(5f); this.setCreativeTab(menuTab.resBlocks); this.blockSoundType = SoundType.METAL; this.setSoundType(blockSoundType); this.setDefaultState(this.blockState.getBaseState().withProperty(FACING, EnumFacing.NORTH)); } @Override public blockFreize setCreativeTab(CreativeTabs tab) { super.setCreativeTab(tab); return this; } /** * Called after the block is set in the Chunk data, but before the Tile Entity is set */ public void onBlockAdded(World worldIn, BlockPos pos, IBlockState state) { super.onBlockAdded(worldIn, pos, state); this.setDefaultDirection(worldIn, pos, state); } private void setDefaultDirection(World worldIn, BlockPos pos, IBlockState state) { if (!worldIn.isRemote) { EnumFacing enumfacing = (EnumFacing)state.getValue(FACING); boolean flag = worldIn.getBlockState(pos.north()).isFullBlock(); boolean flag1 = worldIn.getBlockState(pos.south()).isFullBlock(); if (enumfacing == EnumFacing.NORTH && flag && !flag1) { enumfacing = EnumFacing.SOUTH; } else if (enumfacing == EnumFacing.SOUTH && flag1 && !flag) { enumfacing = EnumFacing.NORTH; } else { boolean flag2 = worldIn.getBlockState(pos.west()).isFullBlock(); boolean flag3 = worldIn.getBlockState(pos.east()).isFullBlock(); if (enumfacing == EnumFacing.WEST && flag2 && !flag3) { enumfacing = EnumFacing.EAST; } else if (enumfacing == EnumFacing.EAST && flag3 && !flag2) { enumfacing = EnumFacing.WEST; } } worldIn.setBlockState(pos, state.withProperty(FACING, enumfacing), 2); } } protected BlockStateContainer createBlockState() { return new BlockStateContainer(this, new IProperty[] {FACING}); } /** * Returns the blockstate with the given rotation from the passed blockstate. If inapplicable, returns the passed * blockstate. */ public IBlockState withRotation(IBlockState state, Rotation rot) { return state.withProperty(FACING, rot.rotate((EnumFacing)state.getValue(FACING))); } /** * Returns the blockstate with the given mirror of the passed blockstate. If inapplicable, returns the passed * blockstate. */ public IBlockState withMirror(IBlockState state, Mirror mirrorIn) { return state.withRotation(mirrorIn.toRotation((EnumFacing)state.getValue(FACING))); } /** * Called by ItemBlocks just before a block is actually set in the world, to allow for adjustments to the * IBlockstate */ public IBlockState getStateForPlacement(World worldIn, BlockPos pos, EnumFacing facing, float hitX, float hitY, float hitZ, int meta, EntityLivingBase placer) { return this.getDefaultState().withProperty(FACING, EnumFacing.getDirectionFromEntityLiving(pos, placer));//e.withProperty(AXIS, facing.getAxis()); } /** * Convert the BlockState into the correct metadata value */ public int getMetaFromState(IBlockState state) { int i = 0; i = i | ((EnumFacing)state.getValue(FACING)).getIndex(); return i; } /** * Convert the given metadata into a BlockState for this Block */ public IBlockState getStateFromMeta(int meta) { //return this.getDefaultState().withProperty(FACING, EnumFacing.getHorizontal(meta)); return this.getDefaultState().withProperty(FACING, EnumFacing.getFront(meta & 7)); } }
-
[SOLVED] Armor item texture not changing color (Inventory Item) [1.11]
daruskiy replied to daruskiy's topic in Modder Support
Thank you!! I implemented it and it worked immediately! -
Hello, I have my code all set to create custom armor from my bronze ingots that is dyeable like vanilla leather armor. The dying itself works, the nbt tags gets applied and the armor model itself gets properly colored. The only thing that doesnt get colored is the actual inventory sprite. Kind of ironic considering for most solutions ive been searching for have the inverse problem. My code as follows: Colorable Armor Class: protected String name; public static int DEFAULT_COLOR = 14277081;// white // = 8388608; //6684672 // white = 14277081 //public static final int DEFAULT_COLOR = 11324652; public ItemModArmor(ArmorMaterial materialIn, int renderIndexIn, EntityEquipmentSlot equipmentSlotIn, String name) { super(materialIn, renderIndexIn, equipmentSlotIn); //this.DEFAULT_COLOR = BASE_COLOR; this.name = name; setUnlocalizedName(name); setRegistryName(name); setCreativeTab(resTab.resItems); } @Override public void registerItemModel(Item item) { respublica.proxy.registerItemRenderer(this, 0, name); } @Override public ItemModArmor setCreativeTab(CreativeTabs tab) { super.setCreativeTab(tab); return this; } /* If to use overlay */ public boolean hasOverlay(ItemStack stack) { return true; } @Override public boolean hasColor(ItemStack stack) { return !stack.hasTagCompound() ? false : (!stack.getTagCompound().hasKey("display", 10) ? false : stack.getTagCompound().getCompoundTag("display").hasKey("color", 3)); } @Override public int getColor(ItemStack stack) { NBTTagCompound nbttagcompound = stack.getTagCompound(); if (nbttagcompound != null) { NBTTagCompound nbttagcompound1 = nbttagcompound.getCompoundTag("display"); if (nbttagcompound1 != null && nbttagcompound1.hasKey("color", 3)){ return nbttagcompound1.getInteger("color"); } } return DEFAULT_COLOR; } public void removeColor(ItemStack stack) { NBTTagCompound nbttagcompound = stack.getTagCompound(); if (nbttagcompound != null) { NBTTagCompound nbttagcompound1 = nbttagcompound.getCompoundTag("display"); if (nbttagcompound1.hasKey("color")) { nbttagcompound1.removeTag("color"); } } } public void setColor(ItemStack stack, int color) { NBTTagCompound nbttagcompound = stack.getTagCompound(); if (nbttagcompound == null) { nbttagcompound = new NBTTagCompound(); stack.setTagCompound(nbttagcompound); } NBTTagCompound nbttagcompound1 = nbttagcompound.getCompoundTag("display"); if (!nbttagcompound.hasKey("display", 10)){ nbttagcompound.setTag("display", nbttagcompound1); } nbttagcompound1.setInteger("color", color); } Armor dying color: /* Copied and Modified MineCraft->Item->Crafting->RecipesArmorDyes */ public class armorDyes implements IRecipe { /* Receives Item Stack of the armor */ public boolean matches(InventoryCrafting inv, World worldIn) { ItemStack itemstack = ItemStack.EMPTY; List<ItemStack> list = Lists.<ItemStack>newArrayList(); for (int i = 0; i < inv.getSizeInventory(); ++i) { ItemStack itemstack1 = inv.getStackInSlot(i); if (!itemstack1.isEmpty()) { if (itemstack1.getItem() instanceof ItemModArmor) { ItemModArmor itemarmor = (ItemModArmor)itemstack1.getItem(); itemstack = itemstack1; } else { if (itemstack1.getItem() != Items.DYE) { return false; } list.add(itemstack1); } } } return !itemstack.isEmpty() && !list.isEmpty(); } /* Returns an Item that is the result of this recipe */ public ItemStack getCraftingResult(InventoryCrafting inv) { ItemStack itemstack = ItemStack.EMPTY; int[] aint = new int[3]; int i = 0; int j = 0; ItemModArmor itemarmor = null; for (int k = 0; k < inv.getSizeInventory(); ++k) { ItemStack itemstack1 = inv.getStackInSlot(k); if (!itemstack1.isEmpty()) { if (itemstack1.getItem() instanceof ItemModArmor) { itemarmor = (ItemModArmor)itemstack1.getItem(); itemstack = itemstack1.copy(); itemstack.setCount(1); if (itemarmor.hasColor(itemstack1)) { int l = itemarmor.getColor(itemstack); float f = (float)(l >> 16 & 255) / 255.0F; float f1 = (float)(l >> 8 & 255) / 255.0F; float f2 = (float)(l & 255) / 255.0F; i = (int)((float)i + Math.max(f, Math.max(f1, f2)) * 255.0F); aint[0] = (int)((float)aint[0] + f * 255.0F); aint[1] = (int)((float)aint[1] + f1 * 255.0F); aint[2] = (int)((float)aint[2] + f2 * 255.0F); ++j; } } else { if (itemstack1.getItem() != Items.DYE) { return ItemStack.EMPTY; } float[] afloat = EntitySheep.getDyeRgb(EnumDyeColor.byDyeDamage(itemstack1.getMetadata())); int l1 = (int)(afloat[0] * 255.0F); int i2 = (int)(afloat[1] * 255.0F); int j2 = (int)(afloat[2] * 255.0F); i += Math.max(l1, Math.max(i2, j2)); aint[0] += l1; aint[1] += i2; aint[2] += j2; ++j; } } } if (itemarmor == null) { return ItemStack.EMPTY; } else { int i1 = aint[0] / j; int j1 = aint[1] / j; int k1 = aint[2] / j; float f3 = (float)i / (float)j; float f4 = (float)Math.max(i1, Math.max(j1, k1)); i1 = (int)((float)i1 * f3 / f4); j1 = (int)((float)j1 * f3 / f4); k1 = (int)((float)k1 * f3 / f4); int lvt_12_3_ = (i1 << 8) + j1; lvt_12_3_ = (lvt_12_3_ << 8) + k1; itemarmor.setColor(itemstack, lvt_12_3_); return itemstack; } } /* Returns the size of the recipe area */ public int getRecipeSize() { return 10; } public ItemStack getRecipeOutput() { return ItemStack.EMPTY; } public NonNullList<ItemStack> getRemainingItems(InventoryCrafting inv) { NonNullList<ItemStack> nonnulllist = NonNullList.<ItemStack>withSize(inv.getSizeInventory(), ItemStack.EMPTY); for (int i = 0; i < nonnulllist.size(); ++i) { ItemStack itemstack = inv.getStackInSlot(i); nonnulllist.set(i, net.minecraftforge.common.ForgeHooks.getContainerItem(itemstack)); } return nonnulllist; } }