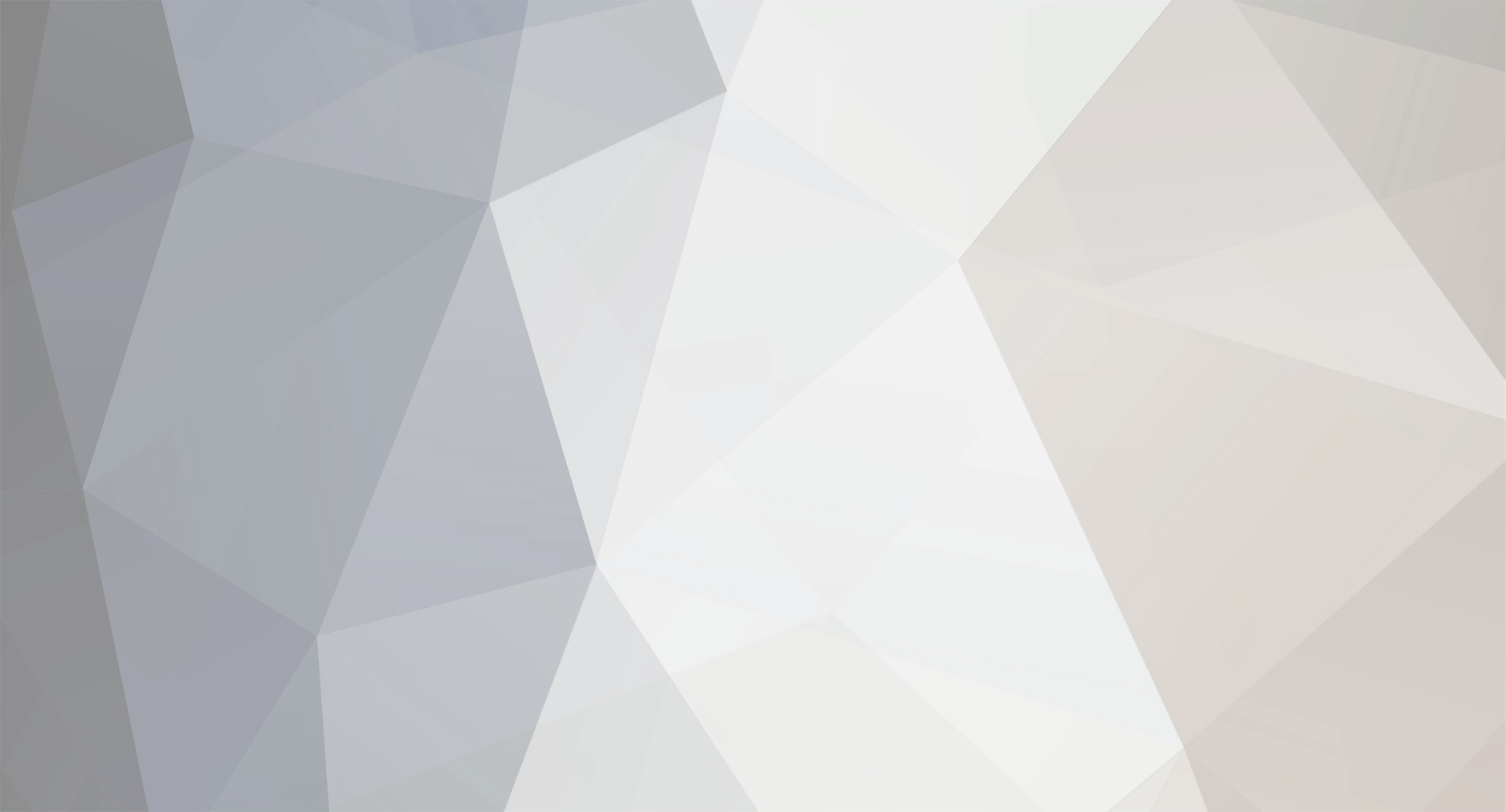
TheDogePwner
Members-
Posts
88 -
Joined
-
Last visited
Everything posted by TheDogePwner
-
Hi, How do I create a custom liquid? Thanks, TDP
-
Hi, How do I have it so when a block is broken, it doesn't drop itself (but, for example, a diamond)? Thanks!
-
[1.8] [SOLVED] Ore Generation Questions
TheDogePwner replied to TheDogePwner's topic in Modder Support
Here's my OreGenerator class: public class OreGenerator implements IWorldGenerator { @Override public void generate(Random random, int chunkX, int chunkZ, World world, IChunkProvider chunkGenerator, IChunkProvider chunkProvider) { generateSurface(world, random, chunkX, chunkZ); } private void generateSurface(World world, Random random, int chunkX, int chunkZ) { System.out.println(); if (random.nextInt(2) == 1) { int x = (chunkX * 16) + random.nextInt(16); int z = (chunkZ * 16) + random.nextInt(16); // Will be found between y = 0 and y = 30 int y = random.nextInt(35); BlockPos blockPos = new BlockPos(x, y, z); // The 3 is max vein size (new WorldGenMinable(NuclearCraft.uraniumOre.getDefaultState(), 1000)) .generate(world, random, blockPos); } } } Registered in the init method: GameRegistry.registerWorldGenerator(new OreGenerator(), 0); -
[1.8] [SOLVED] Ore Generation Questions
TheDogePwner replied to TheDogePwner's topic in Modder Support
Oh, I forgot to register it! What should I put for modGenerationWeight when I register it? -
[1.8] [SOLVED] Ore Generation Questions
TheDogePwner replied to TheDogePwner's topic in Modder Support
So I should have 1 class that implements IWorldGenerator, and one only? And where do I call the generate() method? -
[1.7.10][unsolved] Armor That Changes Skin
TheDogePwner replied to Jetfan16ladd's topic in Modder Support
Okay, thanks Shadow. You might be able to use Java's ImageIO to download a skin and put it inside the directory that the user's skin is in. Not sure if this is possible, but it's an idea. -
[1.8] [SOLVED] Ore Generation Questions
TheDogePwner replied to TheDogePwner's topic in Modder Support
Here is ALL of my code for my block: package net.nc.block; import java.util.Random; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.util.BlockPos; import net.minecraft.world.World; import net.minecraft.world.chunk.IChunkProvider; import net.minecraft.world.gen.feature.WorldGenMinable; import net.minecraftforge.fml.common.IWorldGenerator; import net.nc.NuclearCraft; public class UraniumOre extends Block implements IWorldGenerator { public UraniumOre(Material material) { super(material); setUnlocalizedName("UraniumOre"); setCreativeTab(CreativeTabs.tabBlock); } public void generate(Random random, int chunkX, int chunkZ, World world, IChunkProvider chunkGenerator, IChunkProvider chunkProvider) { switch (world.provider.getDimensionId()) { case 0: generateSurface(world, random, chunkX * 16, chunkZ * 16); break; } } private void generateSurface(World world, Random random, int chunkX, int chunkZ) { if (random.nextInt(2) == 1) { int x = (chunkX * 16) + random.nextInt(16); int z = (chunkZ * 16) + random.nextInt(16); // Will be found between y = 0 and y = 30 int y = random.nextInt(35); BlockPos blockPos = new BlockPos(x, y, z); // The 3 is max vein size (new WorldGenMinable(NuclearCraft.uraniumOre.getDefaultState(), 3)) .generate(world, random, blockPos); } } } -
[1.7.10][unsolved] Armor That Changes Skin
TheDogePwner replied to Jetfan16ladd's topic in Modder Support
Well, to change someone's skin, you need their Minecraft password, so you'd need a GUI to prompt for their Minecraft password. That's a start. -
[1.8] [SOLVED] Ore Generation Questions
TheDogePwner replied to TheDogePwner's topic in Modder Support
Okay, but how do I set the rarity? If I want it as rare as diamonds, what section of code would be modified? -
[1.8] [SOLVED] Ore Generation Questions
TheDogePwner replied to TheDogePwner's topic in Modder Support
Also, here is my new modified code: private void generateSurface(World world, Random random, int chunkX, int chunkZ) { int firstBlockXCoord = chunkX * 16; int firstBlockZCoord = chunkZ * 16; // Will be found between y = 0 and y = 30 int quisqueY = random.nextInt(35); BlockPos blockPos = new BlockPos(firstBlockXCoord, quisqueY, firstBlockZCoord); // The 3 is max vein size (new WorldGenMinable(NuclearCraft.uraniumOre.getDefaultState(), 3)) .generate(world, random, blockPos); } -
[1.8] [SOLVED] Ore Generation Questions
TheDogePwner replied to TheDogePwner's topic in Modder Support
Well, I found this code off of a Reddit post, so it probably has some errors in it (like a for loop). Also, what would the random statement look like to limit that? Thanks! -
As diesieben07 said, your ModID must be lowercase. Also, remember that item.getUnlocalizedName() returns item.[the unlocalizedname]. So, Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(item, 0, new ModelResourceLocation(Reference.MODID+":"+itemname, "inventory")); SHOULD LOOK LIKE THIS: Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(item, 0, new ModelResourceLocation(Reference.MODID+":"+ item.getUnlocalizedName().substring(5), "inventory")) If that doesn't work, then something is wrong with your .json file.
-
Hi, I have a couple questions about ore generation. 1. Would this code work? private void generateSurface(World world, Random random, int chunkX, int chunkZ) { for (int i = 0; i < 16; i++) { int firstBlockXCoord = chunkX + random.nextInt(16); int firstBlockZCoord = chunkZ + random.nextInt(16); // Will be found between y = 0 and y = 30 int quisqueY = random.nextInt(35); BlockPos quisquePos = new BlockPos(firstBlockXCoord, quisqueY, firstBlockZCoord); // The 3 is max vein size (new WorldGenMinable(NuclearCraft.uraniumOre.getDefaultState(), 3)) .generate(world, random, quisquePos); } } 2. If so, about how rare would it be? As rare as diamonds? Iron? Emeralds? Coal? Thanks for any help.
-
Hi, I want to be able to do this: 1. Have a GUI opened when I right-click on a block. 2. Have the GUI have slots (like a furnace) How do I do this in 1.8? Thanks!
-
Hi, I was curious of what the .json format for armor texture are. Please give me a working example. Thanks!
-
[1.8] [UNSOLVED] Creating wearable armor?
TheDogePwner replied to TheDogePwner's topic in Modder Support
1. You can't super() if the method you're using isn't the constructor, and that would be the incorrect constructor parameters. 2. Where is getArmorTexture ran? Does the game run it itself, like an init method? Or do I need to call it myself? 3: What should an armor .json file look like? -
[1.8] [SOLVED] Certain items loading, certain not?
TheDogePwner replied to TheDogePwner's topic in Modder Support
Welp! Nevermind. It just felt like working... -
[1.8] [SOLVED] Certain items loading, certain not?
TheDogePwner replied to TheDogePwner's topic in Modder Support
Remember, all of those .json's work except EmeraldPickaxe and MachineCasing. And the en_US.lang doesn't work for those two. -
[1.8] [SOLVED] Certain items loading, certain not?
TheDogePwner replied to TheDogePwner's topic in Modder Support
Here is a link to all of my assets: https://www.dropbox.com/s/fn2mo3c8zo8epm9/ultracraft.zip?dl=0 -
[1.8] [SOLVED] Certain items loading, certain not?
TheDogePwner replied to TheDogePwner's topic in Modder Support
1. Sir, I apologize for pwning you (lol). 2. HEY LOOK! YAY! [15:51:26] [Client thread/ERROR] [FML]: Model definition for location ultracraft:MachineCasing#inventory not found Strange, considering I have MachineCasing.json in ultracraft/models/item/MachineCasing.json... and a texture in ultracraft/textures/items/MachineCasing.png... Also remember the name isn't loading from en_US.lang. -
Hi, This is the most bewildering thing that has ever happened to me during programming. Most of my items load, but some don't. The ones that do have working names (from my .lang file), working texture, etc. They all have pretty much identical code. Here is my main class: Here is the class of an item that has no texture, and it's name is item.MachineCasing.name: Here is the texturer for the MachineCasing: What on earth is the problem???
-
Um, back on the subject of what to do once you've made your MainMod file... Go to wuppy29's site: http://www.wuppy29.com/minecraft/modding-tutorials/forge-modding-1-8/#sthash.GUAStUe5.dpbs His tutorials are really useful and really accurate. Also, please keep in mind: getUnlocalizedName() returns name.[the unlocalizedname you set it to] And when you call GameRegistry.registerItem(), register your item with its getUnlocalizedName().substring(5). I took me something like 8 posts to figure this out- if you don't do this, your textures won't load.
-
[1.8] [UNSOLVED] Creating wearable armor?
TheDogePwner replied to TheDogePwner's topic in Modder Support
Also, where do I call the constructor for EmeraldArmor? What should I give for its parameters? -
Hi, I was wondering how to create an Item so that it can be used as a piece of wearable armor. Here is my armor class: package uc.armor; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Items; import net.minecraft.item.ItemArmor; import net.minecraft.item.ItemStack; import net.minecraft.util.DamageSource; import net.minecraftforge.common.ISpecialArmor.ArmorProperties; import uc.mainmod.UltraCraft; public class EmeraldArmor extends ItemArmor { public EmeraldArmor(ArmorMaterial p_i45325_1_, int p_i45325_2_, int p_i45325_3_) { super(p_i45325_1_, p_i45325_2_, p_i45325_3_); } public ArmorProperties getProperties(EntityLivingBase player, ItemStack armor, DamageSource source, double damage, int slot) { return new ArmorProperties(0, 0, 0); } public int getArmorDisplay(EntityPlayer player, ItemStack armor, int slot) { return 4; } public void damageArmor(EntityLivingBase entity, ItemStack stack, DamageSource source, int damage, int slot) { stack.damageItem(damage * 2, entity); } public String getArmorTexture(ItemStack armor, Entity entity, int slot, String type) { if (armor.getItem() == UltraCraft.emeraldLeggings) { return "UltraCraft:textures/armor/emerald_layer_2.png"; } else { return "UltraCraft:textures/armor/emerald_layer_1.png"; } } public CreativeTabs[] getCreativeTabs() { return new CreativeTabs[] { CreativeTabs.tabCombat }; } public boolean getIsRepairable(ItemStack armor, ItemStack stack) { return stack.getItem() == Items.emerald; } } I also have: public static Item emeraldHelmet; public static Item emeraldChestplate; public static Item emeraldLeggings; public static Item emeraldBoots; But how do I make those items pieces of wearable armor?
-
[1.8] [UNSOLVED] Shapeless Recipes Problem?
TheDogePwner replied to TheDogePwner's topic in Modder Support
Never mind! I just had to do this: GameRegistry.addShapelessRecipe(new ItemStack(UltraCraft.steelDust), Items.coal, Items.coal, Items.coal, Items.iron_ingot); Thanks though!