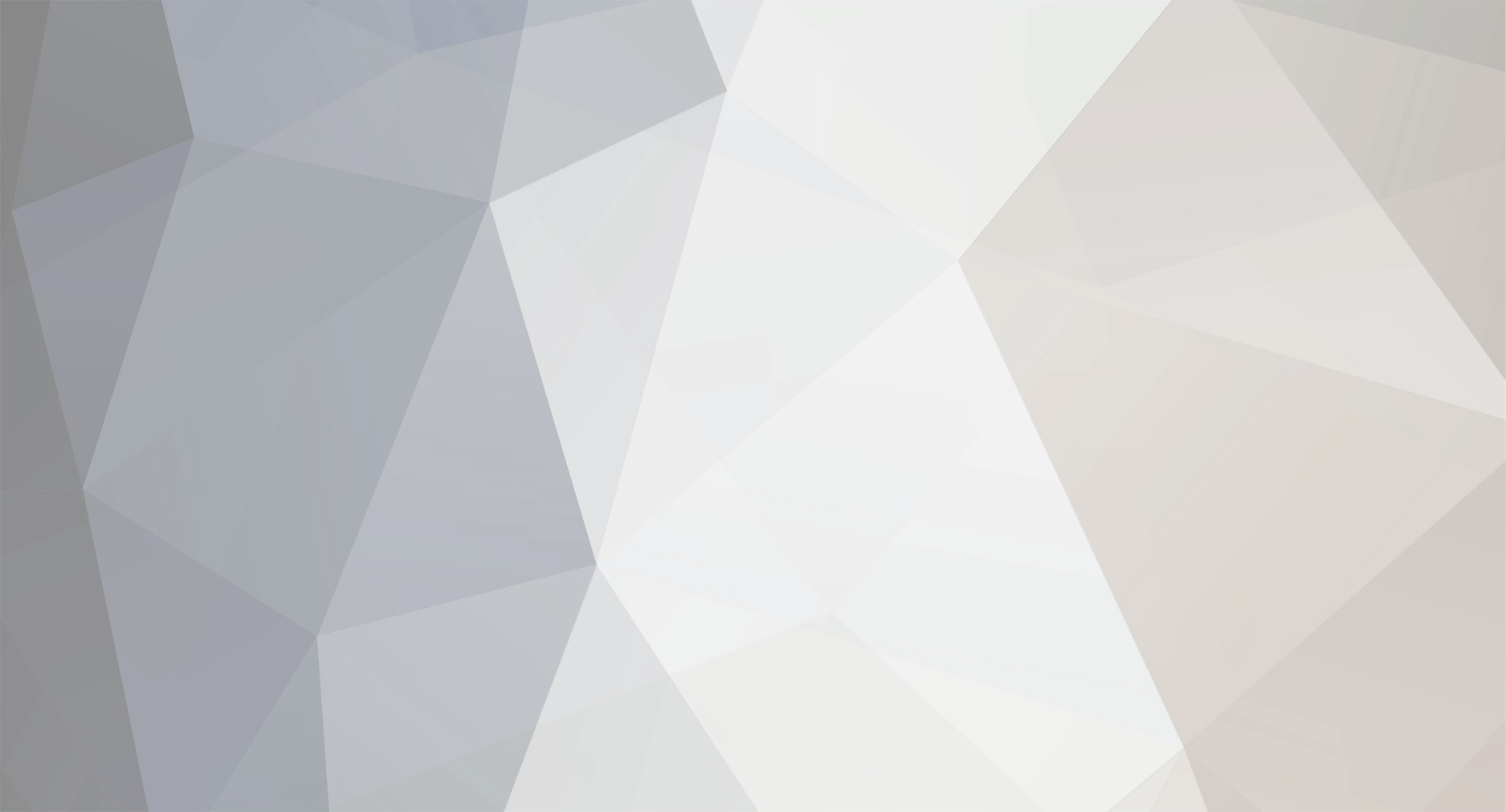
WaffleMan0310
Members-
Posts
35 -
Joined
-
Last visited
Everything posted by WaffleMan0310
-
Now is that IManaHandler you posted what you would initiate in the capability on? Example: public static final Capability<IManaHandler> name = null; Or is it it's own entity?
-
Ok, I get the structure of a capability now, but now say I want to get data from this capability, (disregard packets, I'm talking just getting the capability itself from the player) would I just call getCapability() on the player object and pass in the capability and the default facing objects? What about modifying variables from the capability? Edit: One implementation I have of this capability is getting some values from it to use in a book gui, and I ran getCapability() from the onItemRightClick() as I stated above and it did retrieve the correct value, however to my understanding gui's only operate on the client and information about the player is stored on the client. So I am slightly confused here... Few more questions: - I assume that the class the implements IStorage is where I would put all of the variables pertaining to the capability? - Having studied java syntax and the language itself, but not having a ton of experience, I have an event handler class and a method as follows: public static class EventHandler { @SubscribeEvent public void attachCapabilityEntity(AttachCapabilitiesEvent.Entity e) { if (e.getEntity() instanceof EntityPlayer) { e.addCapability(ID, new Provider()); } } } My IDE is telling me that attachCapabilityEntity() is never used, shouldn't it be called on the attach capabilities event? Or can the IDE not assume an event is called? - In the storage class, when are readNBT and writeNBT called? More will probably arise but that's it for now. I apologize for any stupidity in the questions I ask, I am just trying to learn forge. I am also prone to over-thinking things. Thanks for the help!
-
I looked at your code and read the forge documentation on capabilities and am curious about a couple things... I fully understand the interface that tells the capability all of the data it holds and the storage class that goes with it. What I don't understand is implementing ICapabilityProvider, and if I don't implement it what do I pass into addCapability? I would be nice if someone could run through this in a bit more depth. The forge documentation spoke of a factory as a third parameter to the register() method, and I don't quite see what this does. In addition, what exactly does the CapabilityInject annotation do?
-
When trying to add some properties to the player, I noticed that there is no registerExtenedProperties. Is this just not implemented into 1.9 at this point in time? Or is there a new way to do this?
-
Thanks, I thought that might be it but I wasn't sure so I figured I'd confirm.
-
Hello, So I've been working on a gui that is like the achievement gui and have been quite successful in my venture. I managed to setup boundaries and whatnot. I have one question through, I didn't base it off of the achievement gui class, rather I pawned it up myself and the only real discrepancy that I can notice is that the achievement panel seems to drag smoother. The only thing that I can think of is that the achievement pane is updated at the speed of the fps, yet mine is updated at tick speed. I just curious if this is true or not, and weather or not I can fix this. //Reasource locations for the resources being used in this gui. (guidebook_frame.png, guidebook_background.png) private static final ResourceLocation background = new ResourceLocation(Reference.MODID, ResourceHelper.GUIDEBOOK_BACKGROUND); private static final ResourceLocation frame = new ResourceLocation(Reference.MODID, ResourceHelper.GUIDEBOOK_FRAME); //Width and Height of the background image itself. private float backgroundWidth = 521; private float backgroundHeight = 389; //Size of border of the image on the respective sides. private int borderTopSize = 45; private int borderBottomSize = 41; //Scaled width and height of the frame containing the book gui. private int frameWidth; private int frameHeight; //Variables used to store the x and y of the mouse upon clicking. private int mouseStartX; private int mouseStartY; //Variables used to store the displacement vectors of the mouse x and y, while the mouse is held. private int mouseDisplacementX; private int mouseDisplacementY; //Current u and v coordinates of the background image. private float scrollXDisplacement; private float scrollYDisplacement; //Non-formatted version of the current image location. private int backgroundX; private int backgroundY; //Coordinates of which to start drawing at. private int startX; private int startY; //Modified versions of the startX and startY variables to compensate for the border in the frame texture. private int backgroundStartX; private int backgroundStartY; //Offset vector in pixels of both the frame and background. private int verticalOffset; private int horizontalOffset; public GuiGuideBook() { } @Override public void initGui() { frameWidth = (int)(width * 0.; frameHeight = (int)(height * 1.00); startX = Math.round(((float)width / 2) - ((float) frameWidth / 2)); startY = Math.round(((float)height / 2) - ((float) frameHeight / 2)); verticalOffset = 0; horizontalOffset = -(int)(height * 0.065); backgroundStartX = startX; backgroundStartY = startY + (int)(frameHeight * ((float)(borderTopSize) / (float)backgroundHeight)); } @Override public void updateScreen() { } @Override protected void mouseClicked(int mouseX, int mouseY, int mouseButton) { if (mouseButton == 0) { mouseStartX = mouseX; mouseStartY = mouseY; } } @Override protected void mouseReleased(int mouseX, int mouseY, int mouseButton) { if (mouseButton == 0) { backgroundX += mouseDisplacementX; backgroundY += mouseDisplacementY; mouseDisplacementX = 0; mouseDisplacementY = 0; } } @Override protected void mouseClickMove(int mouseX, int mouseY, int clickedMouseButton, long timeSinceLastClick) { if (clickedMouseButton == 0) { mouseDisplacementX = mouseX - mouseStartX; mouseDisplacementY = mouseY - mouseStartY; scrollXDisplacement = (float) (backgroundX + mouseDisplacementX); scrollYDisplacement = (float) (backgroundY + mouseDisplacementY); if (scrollXDisplacement > 0) { scrollXDisplacement = 0; backgroundX = 0; } if (scrollYDisplacement > 0) { scrollYDisplacement = 0; backgroundY = 0; } if (scrollXDisplacement <= -backgroundWidth) { scrollXDisplacement = -backgroundWidth; backgroundX = -(int) backgroundWidth; } if (scrollYDisplacement <= -backgroundHeight) { scrollYDisplacement = -backgroundHeight; backgroundY = -(int) backgroundHeight; } } } @Override public void drawScreen(int mouseX, int mouseY, float partialTicks) { drawSchoolPane(mouseX, mouseY, partialTicks); drawFrame(); } @Override public void onGuiClosed() { } @Override public boolean doesGuiPauseGame() { return false; } public void drawSchoolPane(int mouseX, int mouseY, float partialTicks) { if (Mouse.isButtonDown(0)) { } GlStateManager.disableFog(); GlStateManager.disableLighting(); GlStateManager.color(1.0F, 1.0F, 1.0F, 1.0F); mc.getTextureManager().bindTexture(background); drawModalRectWithCustomSizedTexture(backgroundStartX + verticalOffset, backgroundStartY + horizontalOffset, -scrollXDisplacement, -scrollYDisplacement, frameWidth, (frameHeight - (int) (frameHeight * ((float) (borderTopSize + borderBottomSize) / backgroundHeight))) - 5, backgroundWidth * 2, backgroundHeight * 2); } public void drawFrame() { GlStateManager.disableFog(); GlStateManager.disableLighting(); GlStateManager.color(1.0F, 1.0F, 1.0F, 1.0F); mc.getTextureManager().bindTexture(frame); drawScaledCustomSizeModalRect(startX + verticalOffset, startY + horizontalOffset, 0, 0, 768, 768, frameWidth, frameHeight, 768, 768); } } Thanks in advanced, WaffleMan0310
-
[1.9] drawTexturedModalRect and other such draw commands
WaffleMan0310 replied to WaffleMan0310's topic in Modder Support
As another follow-up, I got to the point in that I am rendering the frame correctly and got a system to cause the texture to move when the mouse is held down and moved. Here's the code: public class GuiGuideBook extends GuiScreen{ private static final ResourceLocation background = new ResourceLocation(Reference.MODID, ResourceHelper.GUIDEBOOK_BACKGROUND); private static final ResourceLocation frame = new ResourceLocation(Reference.MODID, ResourceHelper.GUIDEBOOK_FRAME); private int frameWidth; private int frameHeight; private int mouseStartX; private int mouseStartY; private int mouseDisplacementX; private int mouseDisplacementY; private int backgroundX; private int backgroundY; public GuiGuideBook() { setGuiSize(512, 512); } @Override public void initGui() { this.frameWidth = (int)(width * 1.1); this.frameHeight = (int)(height * 1.92); } @Override public void updateScreen() { } @Override protected void mouseClicked(int mouseX, int mouseY, int mouseButton) { this.mouseStartX = mouseX; this.mouseStartY = mouseY; } @Override protected void mouseReleased(int mouseX, int mouseY, int mouseButton) { backgroundX += mouseDisplacementX; backgroundY += mouseDisplacementY; mouseDisplacementX = 0; mouseDisplacementY = 0; } @Override protected void mouseClickMove(int mouseX, int mouseY, int clickedMouseButton, long timeSinceLastClick) { this.mouseDisplacementX = mouseX - mouseStartX; this.mouseDisplacementY = mouseY - mouseStartY; } @Override public void drawScreen(int mouseX, int mouseY, float partialTicks) { drawMovingBackground(); drawFrame(); } @Override public void onGuiClosed() { } @Override public boolean doesGuiPauseGame() { return false; } public void drawMovingBackground() { GlStateManager.disableFog(); GlStateManager.disableLighting(); GlStateManager.color(1.0F, 1.0F, 1.0F, 1.0F); mc.getTextureManager().bindTexture(background); drawModalRectWithCustomSizedTexture(0, 0, 1.0F - (float) (backgroundX + mouseDisplacementX), 1.0F - (float) (backgroundY + mouseDisplacementY), frameWidth, frameHeight, 1280, 720); } public void drawFrame() { int startX = Math.round(((float)width / 2) - ((float) frameWidth / 2)); int startY = Math.round(((float)height / 2) - ((float) frameHeight / 2)); GlStateManager.disableFog(); GlStateManager.disableLighting(); GlStateManager.color(1.0F, 1.0F, 1.0F, 1.0F); mc.getTextureManager().bindTexture(frame); drawScaledCustomSizeModalRect(startX, startY - 5, 0, 0, 768, 768, frameWidth, frameHeight, 768, 768); } } The only issue is making the moving background texture contained in the frame. Currently the frame is rendered over the background and the background takes up the whole screen. I tried looking at the achievement gui class and found that achievement was readable, but it seemed to only be for adding the achievements themselves. While GuiAchievements appears to be the class that generates the gui itself, and is full of a bunch of "field_146567_u" type variables. Here is an example of what it looks like in game: http://imgur.com/qqmLnv4 The space background scrolls just like the achievement panel does, I just need some education on how exactly I would make a "window" to see said background. Any help would be most appreciated! -
[1.9] drawTexturedModalRect and other such draw commands
WaffleMan0310 replied to WaffleMan0310's topic in Modder Support
Oh...wow. Thanks! I guess a good follow-up would be: Is there a built-in or conventional way for centering a drawn texture? -
Hello, So I'll start off by saying hello to the forge community as I am new to modding. (I have tried in the past but it didn't stick) I have a solid foundation in Java, have done a lot of reading and what not on it, I feel as if I know enough to fluently program, it's just a matter of learning forge and rendering and such. My issue is as follows, I am trying to render a texture that is 768 * 768 as the background for a gui (I know your supposed to have 256*256) so I have to rewrite drawTexturedModalRect to adapt to this. But being new to all of this rendering stuff such as U, V coordinates; which seem simple. Here's my code: double startX = (double)((float)mc.displayWidth / 2) - (((float)texWidth / 2)); double startY = (double)((float)mc.displayHeight / 2) + (((float)texHeight / 2)); Tessellator tessellator = Tessellator.getInstance(); VertexBuffer vertexBuffer = tessellator.getBuffer(); GlStateManager.disableFog(); GlStateManager.disableLighting(); GlStateManager.color(1.0F, 1.0F, 1.0F, 1.0F); mc.getTextureManager().bindTexture(background); vertexBuffer.begin(7, DefaultVertexFormats.POSITION_TEX); vertexBuffer.pos(startX, startY, 0).tex(0, 0).endVertex(); vertexBuffer.pos(startX + texWidth, startY, 0).tex(1, 0).endVertex(); vertexBuffer.pos(startX, startY + texHeight, 0).tex(0, 1).endVertex(); vertexBuffer.pos(startX + texWidth, startY + texHeight, 0).tex(1, 1).endVertex(); tessellator.draw(); What I am trying to: Well end of the day, I am trying to make a panel like the achievement panel in which you can click and drag through a larger texture to find information and I am currently rendering the frame this will be inside. I want it to be in the center of the screen reliably which is what I assume I will get if I divide the display width and height by 2 (+/- 1 of course) and then set that as the point to start drawing at. Then just telling it to go the texture width and height away from that point to render the rest of the texture. This is all just what I derived from looking at the built-in drawTexturedModalRect, however I find it kind of hard to read because of all of the casting. Questions & Clarifications: So to my interpretation vertexBuffer.pos() takes an X and a Y, these being the location on the screen. vertexBuffer.tex() takes a U and a V of which is a value from 0 - 1 with 1 being the full texture. And vertexBuffer.endVertex() simply ending the declaration of a vertex. However in game when I right click the item this gui is bound to, nothing shows up and I can for the life of me figure out why. It's probably something huge that just hasn't hit me yet due my my ineptness in rendering. Again, I know this is probably a noob question, I'm just looking to further educate myself on rendering and what not. So if someone would run through rendering in a bit of detail, not from just a code perspective like "Call this function and it takes these parameters, this is what these parameters are..." instead; this is what this does in-game and here's why. Or a place where I can find this information would be most appreciated. Thanks, WaffleMan0310
-
So I recently started to learn how to mod and was progressing doing that when I had this idea to create blocks that create their own textures, overlay different stuff based off of their metadata. This lead me to the "ISimpleBlockRenderHandler" I was doing some playing around with it and came about some problems... For one I had this working, then I added a new class that takes some parameters and does the drawing for the tessellator. ("addBlockVerticies") and changed some other stuff that I can't remember, and now I get a priority queue crash upon entering the world. Is probably something stupid that I'm not seeing, here is the class: http://pastebin.com/psWcKV6r Onto the real problem, when it was working I was having a problem with placing more than one of the custom rendered blocks and placing the block below or at ground level. Whenever I placed the block at ground level or below, I world in roughly the chunk around the block would go transparent and nothing but the one face of the block I had alpha pass code for was rendering. Additionally whenever I placed two of more of the blocks together the same effect would happen just alpha pass texture would pick a random block. Again I am new to modding and am probably doing something stupid that you experienced people would smack me for. So be gentle. Onto some concept questions: Binding textures to the tessellator, is there an easier way than I am currently doing it? (like fetching an icon texture, scaling it down and rendering it onto the block face) I am currently registering the item and overlay textures in the block class, then in the render handler I am overriding the renderer with the default coal block texture, and using getIcon from the block class to get the other stuff. I tried using getIcon from a item class but it wouldn't, it have this weird blue tinted texture with a small texture in the corner. For reference: The next question, is there a method (maybe in GL11?) that would scale a texture, and translate it? I tried "GL11.glScaleF()" and I actaully shrunk the chunk that the block was in which I am assuming correlates to the original problem Thanks for your time and tolerance for my noob questions. EDIT: I fixed the issue, it turns out that using GL11.glPushMatrix()/glPopMatrix(), then just changing the tessellator vertex methods to go based off of the block location is better than using tessellator.getTranslated() then operating in terms of 1. My question still remains about scaling and moving a texture on a block face.