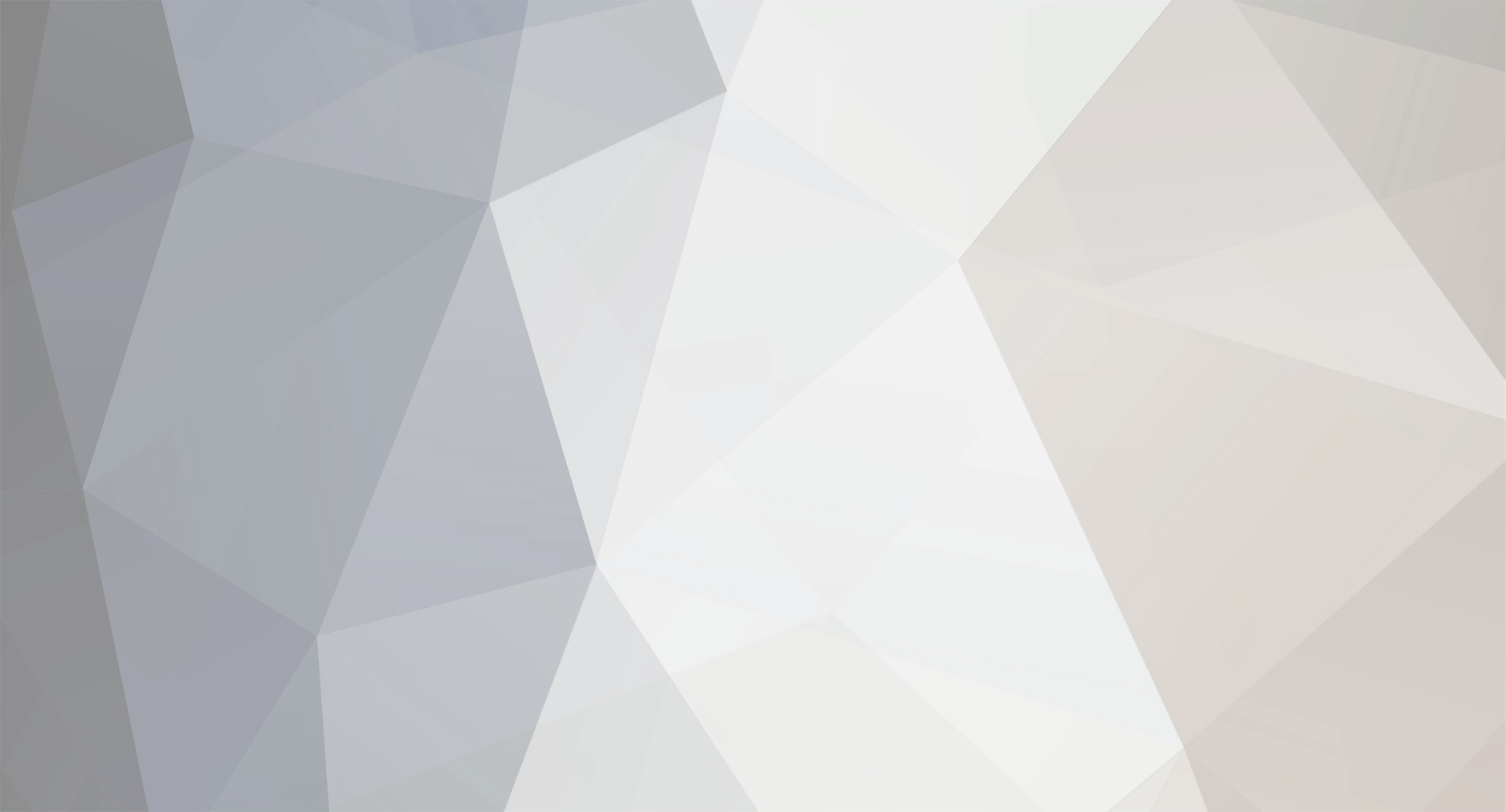
boredherobrine13
-
Posts
86 -
Joined
-
Last visited
Posts posted by boredherobrine13
-
-
1 minute ago, diesieben07 said:
You are not registering your blocks, because your registerBlocks method is not marked as an event handler.
Oh wow. Can't believe I missed that haha. Thanks so much for the help.
-
Hi, I'm having an issue with getting my Block to display in game. The ItemBlock is clearly registered and working correctly as the texture displays in inventory and when dropped. However placing the block gives a no texture purple/black cube at first. Then if you stop looking at it, respawn, or reload the world, the texture becomes invisible. Jumping on top of the block causes a weird glitchy effect, and they don't seem to be able to be broken at this point, but can be mined before the texture disappears. Never experienced anything like this before but I am rewriting my mod for 1.12 following a tutorial because I'm not that familiar with the 1.12 changes and I'm a bit rusty at this. Here are the relevant .java files. Any ideas on what I'm missing here?
MoreFuelsMod.java (Main class)
Spoilerpackage com.bored.morefuelsmod; import com.bored.morefuelsmod.block.ModBlocks; import com.bored.morefuelsmod.item.ModItems; import com.bored.morefuelsmod.proxy.CommonProxy; import net.minecraft.block.Block; import net.minecraft.init.SoundEvents; import net.minecraft.item.Item; import net.minecraft.item.ItemArmor; import net.minecraftforge.client.event.ModelRegistryEvent; import net.minecraftforge.common.util.EnumHelper; import net.minecraftforge.event.RegistryEvent; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.common.eventhandler.SubscribeEvent; import net.minecraftforge.fml.common.network.NetworkRegistry; import net.minecraftforge.fml.common.network.simpleimpl.SimpleNetworkWrapper; import net.minecraftforge.fml.common.registry.GameRegistry; import net.minecraftforge.fml.relauncher.Side; @Mod(modid = MoreFuelsMod.modId, name = MoreFuelsMod.name, version = MoreFuelsMod.version) public class MoreFuelsMod { public static final String modId = "morefuelsmod"; public static final String name = "More Fuels Mod"; public static final String version = "1.6.3"; @Mod.Instance(modId) public static MoreFuelsMod instance; @SidedProxy(serverSide = "com.bored.morefuelsmod.proxy.CommonProxy", clientSide = "com.bored.morefuelsmod.proxy.ClientProxy") public static CommonProxy proxy; @Mod.EventHandler public void preInit(FMLPreInitializationEvent event) { System.out.println(name + " " + version + " is loading!"); } @Mod.EventHandler public void init(FMLInitializationEvent event) { } @Mod.EventHandler public void postInit(FMLPostInitializationEvent event) { } @Mod.EventBusSubscriber public static class RegistrationHandler { @SubscribeEvent public static void registerItems(RegistryEvent.Register<Item> event) { ModItems.register(event.getRegistry()); ModBlocks.registerItemBlocks(event.getRegistry()); } @SubscribeEvent public static void registerItems(ModelRegistryEvent event) { ModItems.registerModels(); ModBlocks.registerModels(); } public static void registerBlocks(RegistryEvent.Register<Block> event) { ModBlocks.register(event.getRegistry()); } } }
BlockBase.java
Spoilerpackage com.bored.morefuelsmod.block; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; import net.minecraft.item.ItemBlock; import com.bored.morefuelsmod.MoreFuelsMod; public class BlockBase extends Block { protected String name; public BlockBase(Material material, String name) { super(material); this.name = name; setUnlocalizedName(name); setRegistryName(name); } public void registerItemModel(Item item) { MoreFuelsMod.proxy.registerItemRenderer(item, 0, name); } public Item createItemBlock() { return new ItemBlock(this).setRegistryName(getRegistryName()); } @Override public BlockBase setCreativeTab(CreativeTabs tab) { super.setCreativeTab(tab); return this; } }
BlockOre.java
Spoilerpackage com.bored.morefuelsmod.block; import net.minecraft.block.material.Material; import net.minecraft.creativetab.CreativeTabs; public class BlockOre extends BlockBase { public BlockOre(String name){ super(Material.ROCK, name); setHardness(3f); setResistance(5f); } @Override public BlockOre setCreativeTab(CreativeTabs tab) { super.setCreativeTab(tab); return this; } }
ModBlocks.java
Spoilerpackage com.bored.morefuelsmod.block; import net.minecraft.block.Block; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; import net.minecraft.item.ItemBlock; import net.minecraftforge.registries.IForgeRegistry; public class ModBlocks { public static BlockOre oreBituminousCoal = new BlockOre("ore_bituminous_coal").setCreativeTab(CreativeTabs.MATERIALS); public static void register(IForgeRegistry<Block> registry) { registry.registerAll( oreBituminousCoal ); } public static void registerItemBlocks(IForgeRegistry<Item> registry) { registry.registerAll( oreBituminousCoal.createItemBlock() ); } public static void registerModels() { oreBituminousCoal.registerItemModel(Item.getItemFromBlock(oreBituminousCoal)); } }
ore_bituminous_coal.json (Blockstates)
Spoiler{ "forge_marker": 1, "defaults": { "textures": { "all": "morefuelsmod:blocks/ore_bituminous_coal" } }, "variants": { "normal": { "model": "cube_all" }, "inventory": { "model": "cube_all" } } }
-
Hi, I have IFuelHandler implemented as an Inferface so I can have fuel handlers individually enabled/disabled rather than having to put each fuel in its own class. I know I could use a map to do this as well, but I'm quite frankly not super knowledgeable about maps in Java or any language. In eclipse, my code works. When i run the project, the fuel handlers work, and when i edit the config file stored in the run directory, I can disable them. However, when I compile this into a mod using the "gradlew build" command, the resulting product has the same code (checked sources.jar) and outputs the config file correctly, but the fuels do NOT work at all and the config switches seem to be dead weight. Of note, I had to change source-compatibility in build.gradle from 1.6 to 1.8 so it would compile correctly as java 1.6 and 1.7 don't allow use of Lambda expressions. Here is my code:
NewFuelSystem.java
package com.bored.morefuelsmod.newfuelsystem; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraftforge.fml.common.IFuelHandler; import net.minecraftforge.fml.common.Optional.Interface; public class TestFuelSystem{ public static IFuelHandler arrow = s -> { if(s.getItem() == Items.ARROW){ return 100; }else{ return 0; } }; public static IFuelHandler apple = s -> { if(s.getItem() == Items.APPLE){ return 100; }else{ return 0; } }; }
Main.java (excerpt)
@Mod.EventHandler public void preInit(FMLPreInitializationEvent event){ //Skip unrelated code Configuration config = new Configuration(event.getSuggestedConfigurationFile()); config.load(); //Skip unrelated code boolean enableTest1 = config.get(Configuration.CATEGORY_GENERAL, "fuelenableTestExperiment1", true).getBoolean(true); if(enableTest1) GameRegistry.registerFuelHandler(TestFuelSystem.arrow); boolean enableTest2 = config.get(Configuration.CATEGORY_GENERAL, "fuelenableTestExperiment2", true).getBoolean(true); if(enableTest2) GameRegistry.registerFuelHandler(TestFuelSystem.apple); //Skip unrelated code config.save(); }
-
22 minutes ago, draganz said:
Then use a boolean
That's what I already do. Here's what I'm using RN.
boolean enableTest1 = config.get(Configuration.CATEGORY_GENERAL, "fuelenableTestExperiment1", true).getBoolean(true); if(enableTest1) GameRegistry.registerFuelHandler(TestFuelSystem.arrow);
Which is moderately concerning because it doesn't really work ATM, And I'm not sure how to register a lambda expression as a fuel handler rather than a class. In fact, the fuel doesn't work at all regardless of what the config flag is after the mod is compiled. That's a problem. Previously each fuel had its own class and they were configured like this for example:
boolean enableDeadbush = config.get(Configuration.CATEGORY_GENERAL, "fuelenableDeadbush", true).getBoolean(true); if(enableDeadbush) GameRegistry.registerFuelHandler(new Deadbush());
-
1 minute ago, draganz said:
why don't you just load values from the config file. Assuming you have a "common" config file (present on both server and client), then the values can be loaded from the file. Just have the file contain (say item unlocalized names) and corresponding integer values for the burn time. Then get the item from the name (Item class has a static method, I believe it is for the unlocalized name) and then save the item and value to your map.
I don't necessarily want the mod to be that user-tweakable just yet. IE, it's partly designed as an experience, and if those values are messed with it would unbalance other parts of the mod. So they can either totally enable or disable, but it would balance the delicate weighting of some other things in the mod if users could just change the values to whatever they want. Not so much like it would break the code, but it would make a very unbalanced experience for players.
-
10 minutes ago, diesieben07 said:
Well, there is no parameter "fuel" in the lambda. You called the parameter "getBurnTime". Why you did that is beyond me, but you did.
I'm not sure why, but I figured since you were using the Interface IFuelHandler, and it had a fuel parameter, I wouldn't need to name one for the lambda expression. I admit my java knowledge is still expanding, but on the bright side this was a good experience and has helped me to understand lambda expressions as a whole better than I previously did.
-
17 minutes ago, draganz said:
What even are you doing?!? Based solely on what you are doing, you should be doing something along the lines of:
GameRegistry.registerFuelHandler(s -> { if(s.getItem() == Items.APPLE){ return 200; }else{ return 0; } }); /////////or///////////// IFuelHandler fuelHandler = s -> { if(s.getItem() == Items.ARROW){ return 100; } }; GameRegistry.registerFuelHandler(fuelHandler); ////////or as jeffryfisher suggested (you could also do a .contains if statement then get a value if true, but what have you)////// private static final Map<Item, Integer> fuelMap = new HashMap(); fuelMap.put(Items.APPLE, 200); GameRegistry.registerFuelHandler(s -> { final Integer i = fuelMap.get(s.getItem()); if(i != null){ return i; }else{ return 0; } });
Any of these ways should work. But, you seem to just be bull-crapping you way through this. Learn the language first, then try to solve the problem at hand.
I am learning the language. This is basically my practice as I learn more. I admit I need to learn more about maps and some other concepts. I do have one question though. Assuming I go with a HashMap, I would need to edit how my config system works. At present, I have an (absolutely atrocious system, made by me when I had almost no java knowledge) where each fuel has its own class and the config switch is required to register the FuelHandler. If I use a Map, what would be the best way to edit the map and change values to 0 from the main class in accordance with my config stuff.
Edit: I also see how my previous lambda statements were severely flawed. For some reason, I thought I needed to use getBurnTime, rather than just assign the int I wanted to return a name. *facepalm*
-
20 minutes ago, diesieben07 said:20 minutes ago, diesieben07 said:
You still have to return something in the lambda even if the if-statement's condition does not evaluate to true.
14 minutes ago, Draco18s said:(Hint: that value would be 0)
You still have to return something in the lambda even if the if-statement's condition does not evaluate to true.
1If I use an else and return 0, it still gives me an error in the actual statement. The big issue here is that I cannot use "fuel".getItem because it does not know what fuel is. This code does not work. However based on some other comments I may investigate maps instead, as they seem simpler than all this.
IFuelHandler test = (getBurnTime) -> { if(fuel.getItem == Items.ARROW) return 100; else return 0; };
-
On 5/27/2017 at 10:54 AM, diesieben07 said:
If I understand you correctly you basically want many fuel handlers without the overhead of having a class for every one of them.
Thankfully IFuelHandler is a functional interface and as such can be implemented using a lambda.
Hi, I will admit that I wasn't fully aware of how Lamba expressions work. I spent some time advancing my java knowledge and now I have code that ALMOST checks out. This is probably a dumb java mistake, but would you mind pointing out to me why the third part of the lambda expression (code executed) doesn't work. Specifically why I can't use fuel.getItem and what I should use instead. Here is the current code:
package com.bored.morefuelsmod.newfuelsystem; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraftforge.fml.common.IFuelHandler; import net.minecraftforge.fml.common.Optional.Interface; public class TestFuelSystem{ IFuelHandler test = (getBurnTime) -> { if(fuel.getItem == Items.ARROW) return 100; }; IFuelHandler testtwo = (getBurnTime) -> { if(fuel.getiItem == Items.APPLE) return 100; }; }
-
Warning: My java knowledge level is intermediate but I am still learning.
Hi, my mod, More Fuels Mod, which is a total rehaul of the Minecraft fuel timings to generally be more realistic as well as adding certain items, needed to be configurable. In order to have a config flag for each of over 100 custom timings formerly in one class that implemented IFuelHandler, I ended up having to move each fuel to its own class. I hate this, but I have been thus far unable to find a way to individually call one if(fuel.getItem == Item.ITEM) statement from within the main method of a class implementing IFuelHandler. I recognize this is probably due to those methods not working with IFuelHandler even if they are just used to call each if statement individually from one class rather than having one class for each if statement. I am unsure if it is at all possible to implement IFuelHandler as a method rather than a class or using an Interface, and if so how. Any ideas how? Or am I missing some obvious other easy methods to containerize all these if statements in one class and still be able to individually enable and disable them using config flags. Sorry If I am missing something obvious due to my intermediate java knowledge.
Example of the rough gist of what I'd like to be able to do if at all possible (this code doesn't work)
public class TestFuelSystem implements IFuelHandler{ @Override public int getBurnTime(ItemStack fuel) { public static int AppleFuelSwitch{ if(fuel.getItem() == Items.APPLE) return 100; } public static int ArrowFuelSwitch{ if(fuel.getItem() == Items.ARROW) return 200; } return 0; } }
The theoretical methods AppleFuelSwitch and ArrowFuelSwitch could then be used to individually enable or disable the fuels then from my main class, rather than lumping them all in one class and having no ability to config which ones are on, or putting each if statement in its OWN class implementing IFuelHandler and making way too many classes...
-
Hi, I am making some very basic tweaks to Biomes O' Plenty. The tweak is using IFuelHandler to make certain items usable as a furnace fuel, which currently works without errors. And I know this is probably a noob question, but I get a compiler error when trying to build the mod. In order to be able to import stuff from this (non-developer since the mod doesn't really offer that) class, I added the universal.jar of the mod and added it to the "Referenced Libraries" section of Eclipse. I can use the mod in eclipse, It doesn't get errors or anything when I try to use the blocks, and it all works logically. I just have 0 experience building on top of another mod and or trying to use its class files as an API. No dev jar is available for BOP to my knowledge, but some of the package names have ...API... in them.
-
I've never done a liquid or made anything but ores spawn, good places to start/tutorials to look at for this? Texturing guides?
-
addCustomCategoryComment
Its in the Configuration class.
EDIT: NVM, I TRIED TO INIT ORE SPAWNING BEFORE LOADING THE ACTUAL ORE. BAD IDEA
Hi, here is my code but it crashes:
@Mod.EventHandler public void preInit(FMLPreInitializationEvent event) { System.out.println(name + " is making smelting better for you!"); Configuration config = new Configuration(event.getSuggestedConfigurationFile()); config.load(); boolean enableRFtLrecipe = config.get(Configuration.CATEGORY_GENERAL, "enableRFtLrecipe", true).getBoolean(true); if(enableRFtLrecipe) RFtL.init(); boolean enableCustomFuels = config.get(Configuration.CATEGORY_GENERAL, "enableCustomFuels", true).getBoolean(true); if(enableCustomFuels) GameRegistry.registerFuelHandler(new Fuels()); [b]config.addCustomCategoryComment(Configuration.CATEGORY_GENERAL, "TEST");[/b] boolean enableCustomOreSpawn = config.get(Configuration.CATEGORY_GENERAL, "enableCustomOreSpawn", true).getBoolean(true); if(enableCustomOreSpawn) GameRegistry.registerWorldGenerator(new ModWorldGen(), 1); config.save(); ModItems.init(); ModBlocks.init(); ModCrafting.init(); ModSmelting.init(); }
[22:45:56] [Client thread/ERROR] [FML]: The following problems were captured during this phase [22:45:56] [Client thread/ERROR] [FML]: Caught exception from §4More Fuels Mod (morefuelsmod-bleeding) java.lang.NullPointerException at com.bored.morefuelsmod.ModWorldGen.<init>(ModWorldGen.java:20) ~[bin/:?] at com.bored.morefuelsmod.Main.preInit(Main.java:41) ~[bin/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_112] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_112] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_112] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_112] at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:639) ~[forgeSrc-1.11.2-13.20.0.2201.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_112] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_112] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_112] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_112] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) ~[guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) ~[guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:243) ~[forgeSrc-1.11.2-13.20.0.2201.jar:?] at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:221) ~[forgeSrc-1.11.2-13.20.0.2201.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_112] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_112] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_112] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_112] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) ~[guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) ~[guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:145) [LoadController.class:?] at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:626) [Loader.class:?] at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:266) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:478) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:387) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_112] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_112] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_112] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_112] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_112] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_112] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_112] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_112] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] [22:45:56] [Client thread/INFO] [sTDOUT]: [net.minecraft.init.Bootstrap:printToSYSOUT:600]: ---- Minecraft Crash Report ---- // Surprise! Haha. Well, this is awkward.
-
Hi, I am experimenting with configuration files and currently I have all of my config flags in CATEGORY_GENERAL. There is also CATEGORY_CLIENT, but I'd like to make my own "category". Any recommendations on how to go about doing this?
-
Alright thanks. I have basic blocks down and basic java down. I made a small tweak mod that plays with fuels mostly on my own. I just kinda lost understanding for some reason when it got to more complicated stuff. I'm studying java a bit more closely. I think my issue is I lack proper understanding of certain conventions and it hinders me from understanding some of the code.
-
Hi, I am newer to java and I technically "have" a mod, but I feel that it too heavily abuses large chunks of tutorial code that..is often quite limiting and inefficient to begin with. I'm just wondering what the best way to "learn" to mod is. It seems like there is no best path or really centralized way to learn. Lots of semi-incomplete tutorials.
-
Mojang has changed and enforced that every resource filename has to be lowercase.
This helped me
-
this works for me
return new ItemStack(ModItems.INGOT_BRASS ) //yea i use same class as you for items
This worked, thanks.
-
Just tried that, now the only working texture is the coke item
-
Hi, I'm updating my relatively simple mod to 1.11 and so far all of the 1.10 code just works with minor tweaks. Which is great, but i have one issue. Here is the class for my creative tab:
package com.bored.morefuelsmod.client; import com.bored.morefuelsmod.Main; import com.bored.morefuelsmod.item.ModItems; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; public class MoreFuelsTab extends CreativeTabs { public MoreFuelsTab() { super(Main.modid); } @Override public Item getTabIconItem() { return ModItems.coke; } }
On the override, i am now told that "The return type is incompatible with CreativeTabs.getTabIconItem()." Code correction suggests that ItemStack is what it wants, however that simply does not work with my code. Is there a workaround for this? When i try to launch minecraft, it will launch and load in a world, however if you go to the creative tab it will crash before even displaying the tab.
-
Hi, as stated. I am wondering if there is a simple equivalent to IChunkGenerator for 1.8. I am doing a backport of some code for my mod's users. Mainly because 1.8 is still semi-popular for modpacks. Here is my code, problem line in bold:
package com.bored.morefuelsmod;
import java.util.Random;
import com.bored.morefuelsmod.block.ModBlocks;
import net.minecraft.util.BlockPos;
import net.minecraft.world.World;
import net.minecraft.world.chunk.IChunkProvider;
import net.minecraft.world.chunk.IChunkGenerator;
import net.minecraft.world.gen.feature.WorldGenMinable;
import net.minecraft.world.gen.feature.WorldGenerator;
import net.minecraftforge.fml.common.IWorldGenerator;
public class ModWorldGen implements IWorldGenerator{
private WorldGenerator bituminousCoalOre;
public ModWorldGen(){
this.bituminousCoalOre = new WorldGenMinable(ModBlocks.bituminousCoalOre.getDefaultState(), 4);
}
private void runGenerator(WorldGenerator generator, World world, Random rand, int chunk_X, int chunk_Z, int chancesToSpawn, int minHeight, int maxHeight) {
if (minHeight < 0 || maxHeight > 256 || minHeight > maxHeight)
throw new IllegalArgumentException("Illegal Height Arguments for WorldGenerator");
int heightDiff = maxHeight - minHeight + 1;
for (int i = 0; i < chancesToSpawn; i ++) {
int x = chunk_X * 16 + rand.nextInt(16);
int y = minHeight + rand.nextInt(heightDiff);
int z = chunk_Z * 16 + rand.nextInt(16);
generator.generate(world, rand, new BlockPos(x, y, z));
}
}
@Override
public void generate(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator,
IChunkProvider chunkProvider) {
switch (world.provider.getDimensionId()){
case 0://Over_World
//this.runGenerator(generator, world, random, chunkX, chunkZ, chancesToSpawn, minHeight, maxHeight);//
this.runGenerator(this.bituminousCoalOre, world, random, chunkX, chunkZ, 10, 0, 60);
break;
case -1://Nether
break;
case 1://End
break;
}
}
@Override
public void generate(Random random, int chunkX, int chunkZ, World world, IChunkProvider chunkGenerator,
IChunkProvider chunkProvider) {
// TODO Auto-generated method stub
}
}
-
Thank you so much! That fixed the issue!
-
Hi...so I use IfuelHandler to make custom fuels and a few of my mod items have very high burn times. Someof these items/blocks, code show belong only burn for the length of time of one wood block, when in reality they should burn for over an hour. Any ideas what's up? Is their some tick cap that I'm unaware of? Any ideas for workarounds? Code below:
if (fuel.getItem() == Item.getItemFromBlock(ModBlocks.pelletBlock)){ return 8100; //9 times the value of fuel pellets } if (fuel.getItem() == ModItems.concentratedPelletsFuel){ return 30000; //4 times the value of pellet blocks } if (fuel.getItem() == Item.getItemFromBlock(ModBlocks.concentratedPelletBlock)){ return 291600; //9 times the value of concentrated pellet fuel }
-
Thank you SO much man.
[Forge 1.12.2] Issues applying .getItemDropped
in Modder Support
Posted
Hi, I am recoding my mod for 1.12.2 and in the past I have made a new "BlockX" glass for each new ore so that I can define what item the ore will drop when mined using a method like the following.
However as I continue to expand my mod this is clearly inefficient because I will have a ton of redundant class files. So I'd like to define this when declaring the blocks in my ModBlocks class. However everything I've tried so far has had issues. Ex:
In the case of .getItemDropped(state, rand, fortune) I'm not sure what to input for the state, rand, and fortune. I recognize I need to change my method to something more generic and have it return something else but I'm not sure exactly how to restructure it and if i should mess with the "IBlockState state, Random rand, int fortune)" in the original method from above or if I even can remove things. Is this even possible? Am I overlooking something simple?