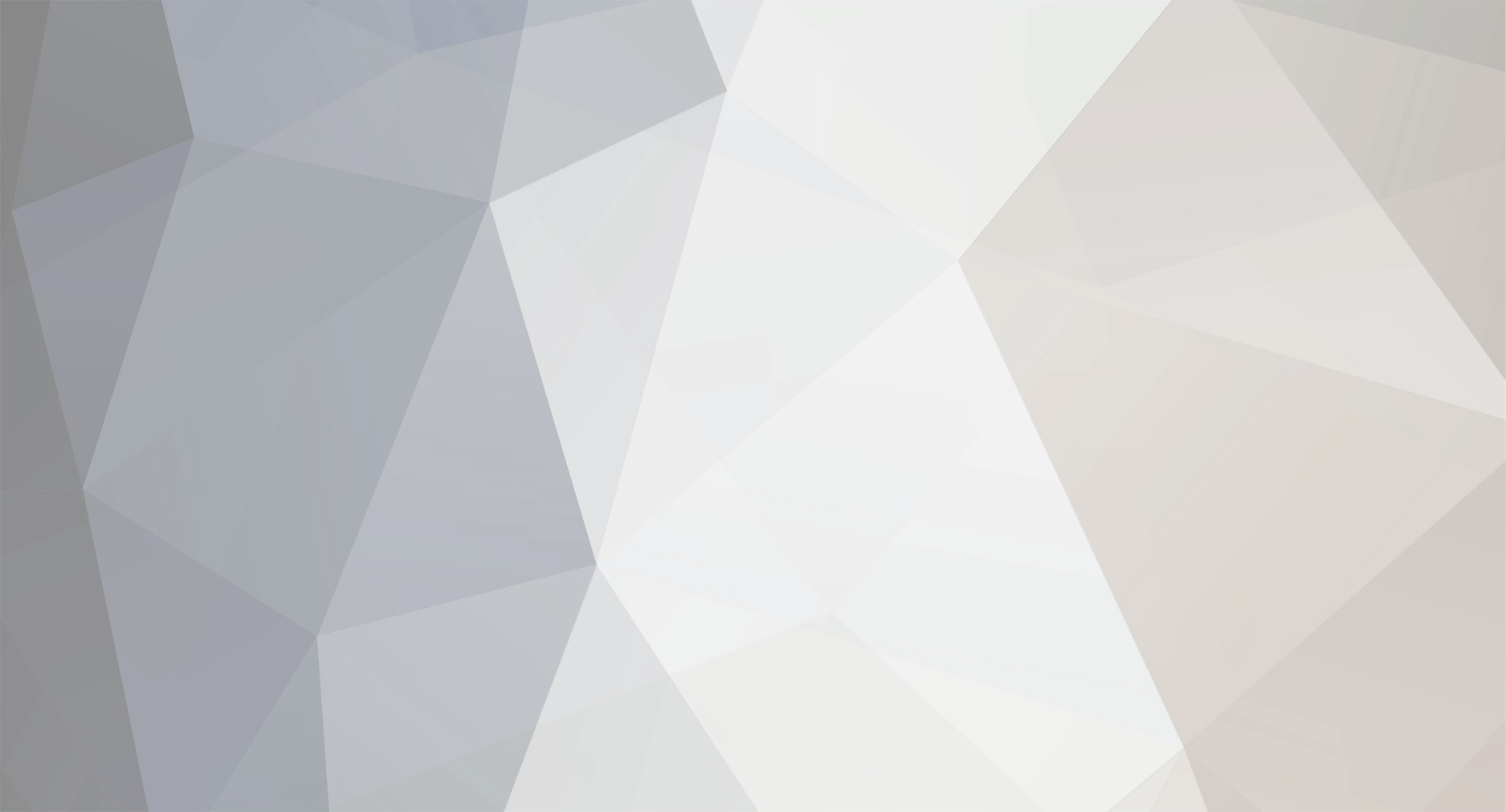
Born2Code
Members-
Posts
57 -
Joined
-
Last visited
Everything posted by Born2Code
-
[1.8] Custom entity attacking when attacked
Born2Code replied to expert700's topic in Modder Support
Oh wow, I didn't know that. Thanks =D -
[1.8] Custom entity attacking when attacked
Born2Code replied to expert700's topic in Modder Support
Override this method below to do whatever you want when the entity is attacked. The way the wolf does it for making it attack the player, (I'm assuming you want it to keep attacking after it's hit rather than hit for it) is using data watchers. Method to override... /** * Called when the entity is attacked. */ public boolean attackEntityFrom(DamageSource source, float amount) { if (entity != null && entity instanceof EntityPlayer) { //do stuff here } return super.attackEntityFrom(source, amount); } } -
[1.8][SOLVED]Issues with item that spawns entities
Born2Code replied to The_Fireplace's topic in Modder Support
Okay. As for the problem of not properly consuming the item, is that part is outside of the if(!world.isRemote) checker. As for the entity you might not have registered the entity properly (or at all), or maybe the applied the rendering wrong and it is spawning. -
[1.8] Dimensions, Generating A Structure Once (SOLVED)
Born2Code replied to Born2Code's topic in Modder Support
Finally, got it solved. Updating OP for anyone else that has the problem. -
The material. Block block = (new Block(Material.rock)).setHardness(2.0F).setResistance(10.0F).setStepSound(soundTypePiston).setUnlocalizedName("stonebrick").setCreativeTab(CreativeTabs.tabBlock); public BlockPlanks() { super(Material.wood); }
-
[1.8] Dimensions, Generating A Structure Once (SOLVED)
Born2Code replied to Born2Code's topic in Modder Support
1. You should post the full code, not just a method. 2. Post the crash report together. All I need, is an empty dimension, with one structure generated inside, that way when teleported there (working), I am teleported into that structure. -
[1.8] Dimensions, Generating A Structure Once (SOLVED)
Born2Code replied to Born2Code's topic in Modder Support
Thanks Nodnarb3! I'll look into that. -
[1.8] Dimensions, Generating A Structure Once (SOLVED)
Born2Code replied to Born2Code's topic in Modder Support
Generating a cube of air and my structure inside forever was just a quick though, I would prefer the OP answered instead. ChunkProvider -
He figured out why it kept disappearing haha, his only problem now is he's using a vanilla renderer, that automatically uses it's own entity.
-
[1.8] Dimensions, Generating A Structure Once (SOLVED)
Born2Code replied to Born2Code's topic in Modder Support
Here.. public void func_180520_a(int p_180520_1_, int p_180520_2_, ChunkPrimer primer) { for(int x = 0; x < 100; x++) { for(int z = 0; z < 100; z++) { primer.setBlockState(x, 100, z, Blocks.air.getDefaultState()); } } primer.setBlockState(0, 100, 0, Blocks.end_stone.getDefaultState()); } -
[1.8] Dimensions, Generating A Structure Once (SOLVED)
Born2Code replied to Born2Code's topic in Modder Support
If it's easier, I could also just have it generate a cube of air and then build the structure inside. But I also couldn't get that working, as I keep getting index out of bound errors. -
Well of course it's not rendering anything. public void doRender(EntityEnderCrystal entity, double x, double y, double z, float p_76986_8_, float partialTicks) { -snip- } The default renderer uses it's own entity (which I thing is true with all vanilla renderers), so you need to make your own renderer, which can do everything the same, except use your own entities and such
-
[1.8] Dimensions, Generating A Structure Once (SOLVED)
Born2Code replied to Born2Code's topic in Modder Support
Oh, yes, sorry. Forgot to include that in last post It doesn't work, it just doesn't add anything, but... I did another test, I had a counter variable, that increased every time that method was called and was printed out. When I first entered my dimension it was being increased without stopping until a certain point.. then it only increased as a moved towards un-generates terrain. -
[1.8] Dimensions, Generating A Structure Once (SOLVED)
Born2Code replied to Born2Code's topic in Modder Support
Don't think it would work. I added a dummy variable to hold whether or not it's been built and based on that add the block. private boolean built = false; if(built == false) { addblockstuffhere built = true; } -
SOLVED ---------- Problem: I had a block where when you bump into it, it takes you inside of it, I added a new dimension for this but couldn't figure out how to generate the interior only once in the dimension. Solution: In whatever your teleporting method is, either an item, colliding with block, etc... whereever you change the player's dimension is where you need to do the generation. Just use entity.getEntityWorld() to get the world, AFTER you change dimensions, then you will have the "dimension's world". Then use that world to do all of the structure creation.
-
Okay, turns out I just needed to make my own Teleporter and use that. Thank you for hinting towards that since you capitalized the name of Teleporter. I can now freely travel to my dimension now without it breaking. I will update the OP for anyone else who might have this problem.
-
Well, I could be wrong, in which case please correct me, but I don't think the chunk provider has anything to do with the problem. Looking at this https://github.com/Eternaldoom/Realms-of-Chaos/blob/master/com/eternaldoom/realmsofchaos/iceruins/gen/ChunkProviderIceRuins.java which is supposedly updated to 1.8 I can tell little to no difference between his chunk provider and the ChunkProviderEnd (which I'm using also). I looked at his world provider as well as mine and I don't think the problem is there either. Thinking it has something to do with how I'm registering (or not registering) it.
-
I have the block for it working fine.. here it is the part that teleports. public void onEntityCollidedWithBlock(World world, BlockPos pos, IBlockState state, Entity entity) { if(!world.isRemote) { entity.travelToDimension(2); System.out.println(entity.dimension); } }
-
Haha, apparently there was more to the worldprovider that I missed and because I won't use the hell chunk manager, I made my own. Further info in the OP.
-
Hello. I'm working on a mod that has a specialthing that you walk into and it teleports you inside of it, well I decided to instead of creating the interior inside of the world somewhere, add a whole new dimension for it. Well, everything else is working. I've done all I've figured out so far of adding a new dimension to add it and then tried to port myself to it, by the way I do not expect (or want) the world to have anything generated in it. The problem is, when I collide with my specialthing (that part triggers fine) and tell the player to travel to my dimension it works, and then fails. I put a print to test if I was actually getting put in my dimension because 1 second later I get kicked into the overworld and it spawns a nether portal (). It does set me in the dimension.. but kicks me out as said above. Any help is appreciated. MainModFile MyWorldProvider BlockClass (aka my portal) MyTeleporter
-
Okay, I've tried doing it that way, but it seems any time I can get it to render without catching at nulls or anything, it simply gets stuck on the loop mentioned in the OP. @SubscribeEvent public void preRenderPlayer(RenderLivingEvent.Pre event) { if (!(event.entity instanceof EntityPlayer)) return; if(Villager.newModel == null) return; if(Villager.manager == null) return; event.setCanceled(true); Villager.newModel.doRender(event.entity, event.x, event.y, event.z, 0f, 0f); }
-
Please be more specific. new RenderPlayer(manager).doRender(event.entity, 0d, 0d, 0d, 0f, 0f); Okay, then I ask how do I change the model.. response: Extend RenderPlayer and alter anything you want, Model, whatever. So.... when do I use my renderer?
-
Okay. @SubscribeEvent public void preRenderPlayer(RenderPlayerEvent.Pre event) { System.out.println("I'm working"); } Doesn't get called? =/ And how would I go about changing the player model then?
-
Hello, what I'm trying to do is change the player model by using forge events to override the players pre-rendering. I have the event handler setup and working, (aka registered correctly, as I tested other things and they work just fine) but I can't seem to figure out how to actually render a new model instead of the normal player. I have one of the things I've tried below, which is what I'm finding everywhere that apparently should work, but instead locks up the game and spams the same crash message. Here is my event handler class... public class PreRenderEvent { RenderManager manager = Minecraft.getMinecraft().getRenderManager(); @SubscribeEvent public void preRenderPlayer(RenderLivingEvent.Pre event) { if (!(event.entity instanceof EntityPlayer)) return; event.setCanceled(true); new RenderPlayer(manager).doRender(event.entity, 0d, 0d, 0d, 0f, 0f); } } And here is the crash message..
-
[1.7.10] Trouble with onImpact for thrown entity
Born2Code replied to Ms_Raven's topic in Modder Support
if(!world.isRemote) { mop.entityHit.setdead(); } use that instead.. otherwise you're telling the knife to kill itself on the client.