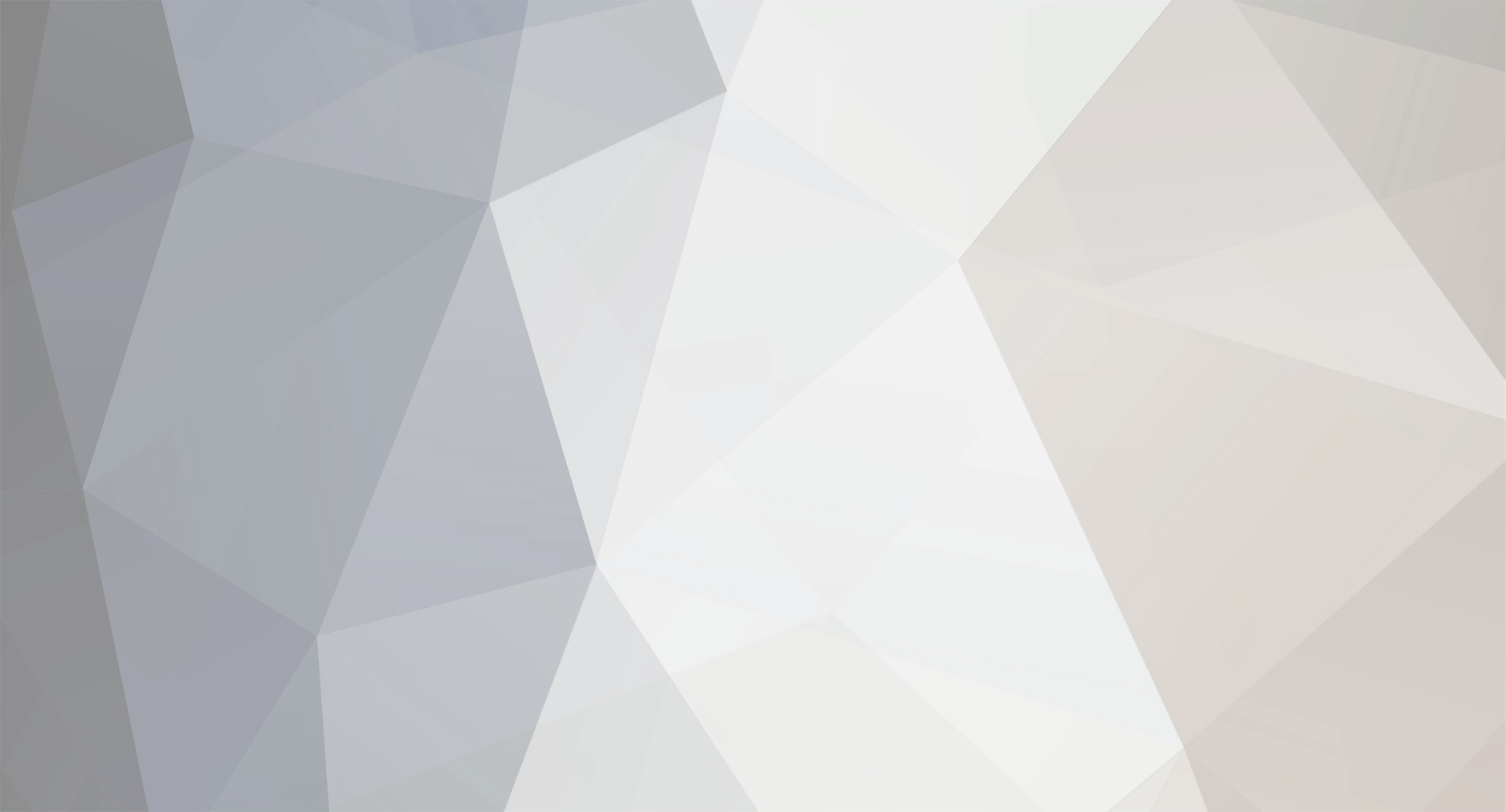
spongieDev01
Members-
Posts
13 -
Joined
-
Last visited
Everything posted by spongieDev01
-
Hi, I am trying to create a mod which has trains, that follow tracks. I have tried doing some movement with trigonometry, but I don't seem to get a nice result as compared to minecarts (It lags a lot and sometimes the trains derail). I have looked at the minecart class and I find it really confusing. I am not asking for code, but more like help on how minecarts work. Thanks!
-
Bump.
-
Anyone? I don't have a lot of experience with server/client stuff.
-
I would say, buy Minecraft and playing around with Forge. Even if you have programming experience, it might still be complicated to do that as your first project. But that's just my opinion.
-
Game Crashes when crafting custom item
spongieDev01 replied to XxZHALO13Xx's topic in Modder Support
What is the method called in which you register you item (CommonProxy)? EDIT: It might be that you are overriding the same method you use to register the item. -
Entity class: public abstract class EntityTrainBase extends Entity { public float speed = 0; public int connectedTrain = -1; public EntityTrainBase(World world) { super(world); } @Override public void entityInit() { this.preventEntitySpawning = false; this.setSize(2F, 2F); DataWatcher dw = this.getDataWatcher(); dw.addObject(20, speed); dw.addObject(21, connectedTrain); } @Override public abstract double getMountedYOffset(); public abstract float getCartLength(); public abstract float getMaxSpeed(); public abstract boolean isDriver(); @Override public boolean canBeCollidedWith() { return true; } @Override public void onEntityUpdate() { super.onEntityUpdate(); //if(worldObj.isRemote) { if(riddenByEntity != null && riddenByEntity instanceof EntityPlayer) { EntityPlayer player = (EntityPlayer) riddenByEntity; this.speed = this.getDataWatcher().getWatchableObjectFloat(20) + player.moveForward * .01f; this.speed = Math.min(this.speed, this.getMaxSpeed()); this.speed = Math.max(this.speed, 0); this.getDataWatcher().updateObject(20, this.speed); } //} moveCart(speed, this); } public void moveCart(float sp, EntityTrainBase cart) { if(!(worldObj.getBlockState(cart.getPosition()).getBlock() instanceof BlockRail)) {return;} EnumRailDirection rd = (EnumRailDirection) worldObj.getBlockState(cart.getPosition()).getValue(BlockRail.SHAPE); float yaw = cart.rotationYaw; int y1 = (int) (RailHelper.getYaw1(rd)); int y2 = (int) (RailHelper.getYaw2(rd)); if(MathHelper.angleDistance(yaw, y1) < MathHelper.angleDistance(yaw, y2)) yaw = y1; else yaw = y2; if(riddenByEntity != null && riddenByEntity instanceof EntityPlayer) cart.riddenByEntity.rotationYaw += net.minecraft.util.MathHelper.wrapAngleTo180_float(yaw)-cart.rotationYaw; cart.rotationYaw = net.minecraft.util.MathHelper.wrapAngleTo180_float(yaw); cart.speed = cart.getDataWatcher().getWatchableObjectFloat(20); cart.moveEntity(Math.sin(Math.toRadians(yaw))*-cart.speed, 0, Math.cos(Math.toRadians(yaw))*cart.speed); //cart.setPositionAndUpdate(cart.posX + Math.sin(Math.toRadians(yaw))*-cart.speed, cart.posY, cart.posZ + Math.cos(Math.toRadians(yaw))*cart.speed); } @Override public boolean interactFirst(EntityPlayer player) { if(player.inventory.getCurrentItem() != null && player.inventory.getCurrentItem().isItemEqual(new ItemStack(ModItems.coupler))) { ((ItemCoupler) ModItems.coupler).interactTrain(player.inventory.getCurrentItem(), player, this); } else if(!this.worldObj.isRemote) { if(this.riddenByEntity == null || player.ridingEntity == null) { player.mountEntity(this); } } return true; } } I looked at both the horse and minecart classes and it looks like they use motionX / motionY / motionZ variables. I tried setting those variables instead already but the entity didn't move.
-
Hello, I am trying to create a vehicle entity. I am not sure what the best way to do the movement is though. Right now I'm using trigonometry to move it based on its yaw. I am then multiplying it by a speed variable, so that the speed can be controlled: cart.moveEntity(Math.sin(Math.toRadians(yaw))*-this.speed, 0, Math.cos(Math.toRadians(yaw))*this.speed); This works great... on singleplayer. For it to work on multiplayer, I started programming packets and setup a Data Watcher to sync the speed variable. I was then thinking, now that all the clients have the speed variable (and of course the yaw as well), the clients should calculate the movement themselves so that there's less lag. I removed the if(!worldObj.isRemote) check so that it would also be run on the client, except that now it's lagging even more. It seems like the problem is that the server still keeps sending position updates to the client. The client receives these a bit later though. The result: The vehicle keeps getting teleported back to where it was a moment ago. -> More Lag! It would be great if anyone could help me solve this. Thank you in advance!
-
[1.8] [SOLVED] Interact event not being called for entities
spongieDev01 replied to spongieDev01's topic in Modder Support
Solved! It turns out I didn't need all the bounding box stuff! Only setSize(). After adding: @Override public boolean canBeCollidedWith() { return true; } to the entity class, I could interact with it! -
[1.8] [SOLVED] Interact event not being called for entities
spongieDev01 replied to spongieDev01's topic in Modder Support
Anyone? This makes no sense! I have looked at some other entity classes, and I don't see anything that could explain this. Maybe this has something do to with Forge not calling the interact method. I would really appreciate any help! Also, I'm using the summon command. Not that I see how that could influence it. -
[1.8] [SOLVED] Interact event not being called for entities
spongieDev01 replied to spongieDev01's topic in Modder Support
I added: @Override public AxisAlignedBB getCollisionBox(Entity entityIn) { return new AxisAlignedBB(posX-1, posY-1, posZ-1, posX+1, posY+1, posZ+1); } to my entity class. I can now collide with the entity, but right clicking it still doesn't do anything. Btw, I am using this to get interacts: @Override public boolean interactFirst(EntityPlayer player) { System.out.println("INTERACTTT!!!"); return true; } -
[1.8] [SOLVED] Interact event not being called for entities
spongieDev01 replied to spongieDev01's topic in Modder Support
It might have something to do with getBoundingBox() or getCollisionBox(). I saw minecarts used them and I'm a bit confused what the difference is to setSize(). I also noticed that attackEntityFrom() isn't being called either. -
[1.8] [SOLVED] Interact event not being called for entities
spongieDev01 replied to spongieDev01's topic in Modder Support
I have tried it both with and without setEntityBoundingBox(). Neither works. -
Hello, I am trying to create a non-living entity. I would like the player to be mounted onto this entity after the mod checks some permission stuff. I have overridden the interactFirst(EntityPlayer player) method, but it seems like it is not being called. I have noticed that when I press F3+B (to show hitboxes of entities), the hitbox seems to be correct, but I can interact with the blocks behind it; not with the actual entity. Code: http://pastebin.com/JjAJgR9f I'd appreciate any help, Thanks!