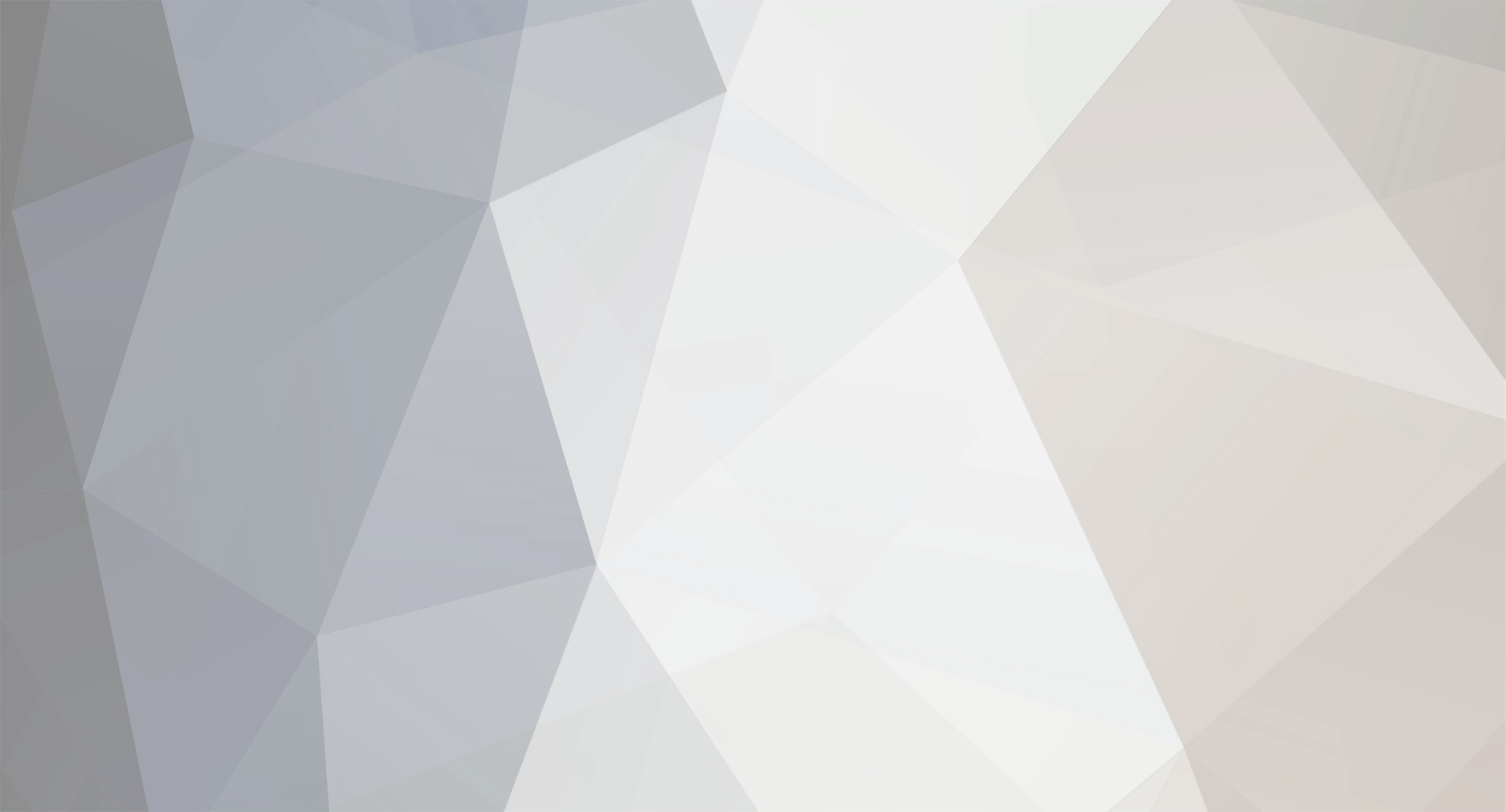
DJD
Members-
Posts
64 -
Joined
-
Last visited
Everything posted by DJD
-
Thanks all... Its called Brix and its (at the moment) a creative mode tool for design purposes (think diablo3 and how it uses a few parts to randomize dungeons in various ways). I'm a few weeks away from any kind of release anyway- got to clean up the code, remove some debug stuff etc etc)...
-
I've got (or almost got) a workable mod I'd like to get out for others to kick around, get some feedback etc etc. What is the best way to go about this ?
-
Its been challenging, to be sure, (cutting a section from world and putting in text file that is easily editable, getting the placement so I can rotate stuff properly in the 4 directions and change the origin if need be) but well worth it so far I think (from a Java knowledge standpoint)
-
I'm generating some stuff with my mod and I was wondering if I should get the map seed and ensure that any random generation is used off of that or whether I should embrace another (self generated) seed..... Or to put it more succinctly: // Walk through rules starting at last and determine if that rule is to be used instead private int useRule(Bricks rules) { int ret = 0; Random rnd = new Random(); for (int num = rules.bricks.size() -1; num > 0; num--) { Brick brick = new Brick(rules.getBrick(num)); if (rnd.nextInt(100)+1 <= brick.chance) { ret = num; break; } } return ret; } If I should use new Random or is there a minecraft generated random instead ?
-
I have a few quick questions about random number generation inside minecraft itself: How do I ensure I am using the same seed that minecraft generates for my random numbers, as if I use my own external Random Number Generator it won't tie in with the minecraft seed so that with the same seed I get totally different results from stuff generated my way. I guess I'd like to know what is the proper way to use random numbers for generation in my mod (same seed as minecraft, or new seed or whatever).
-
I was wondering if there a way to execute a registered server command by keystroke instead of having to type "/" followed by the command ?
-
Sorry that you think this is a double post- I think I can use THREE passes to totally remove all blocks. I'll update the other post with the solution I found as well. 1st- from bottom of selected cube to top- only blocks that are one blocklayer CUTOUT_MIPPED, or CUTOUT. 2nd- from top down to bottom of cube- blocks that are not FullCube 3rd- any left over. This seems to clean it up (does not drop doors all over the place). The reason I ask is that it seems strange that it appears you have to manipulate the blocks one at a time (in loops) and there is no batch processor where you can do them all then have the physics update after you have done what you want to do to those blocks. Of course, for some strange reason Minecraft uses the Y axis as the 3rd dimension too, so I guess its par the course
-
Is there a way to prevent a cluster of blocks to not update physics on the regular tick until I "release" them ? The problem I am having is that I can cut portions of the map (set the block one at a time to "Air"), but there are a few caveats: 1. If I cut from the lowest to highest level (one block at a time), things that are supported (e.g. pressure plates on fences to look like tables) fall when their block below them is removed. 2. If I cut from highest to lowest, doors drop (they look like they have to be removed from the bottom or they drop onto the map). Right now I use 2 passes to successfully remove things- 1st where not(isSolidCube) then anything else on the 2nd pass. The problem child is doors- they are not(isFullCube) so get removed top down in pass 1 (which does not destroy it but harvest it and leaves it on the ground). It would optimally be great to not allow the blocks to be updated until I have run through the delete loop. Not sure this is doable however.....
-
I'm using block getExtendedState to return the properties of a block that I save to file as text. It gets the proper properties (or so I thought) until I ran into blue wool (it works fine for all the other wools and so far most of the other blocks (still testing on some of the buttons etc). the enumeration for that is BLUE_WOOL, but the valid value you get for the IBlockState.getAllowedValues is "lightBlue". So when you go to replicate that saved block in the world it cannot find "lightBlue" value because it is looking for "LIGHT_BLUE" instead. Its probably something easy but for some reason I can't seem to figure it out Solved ! In my comparator I was using (E iterator): if (prop.getName(E).equalsIgnoreCase(value)) instead of if (E.toString().equalsIgnoreCase(value)) Beware the pitfalls for the unwary ! Thankfully Eclipse has that integrated debugging tool with it
-
[1.8] [RESOLVED] return blockPos of clicked spot- even if it is air
DJD replied to DJD's topic in Modder Support
It's a shame something that is kind of basic requires so much to achieve -
[1.8] [RESOLVED] return blockPos of clicked spot- even if it is air
DJD replied to DJD's topic in Modder Support
Experimenting atm... Too bad they've don't have a left click air like RIGHT_CLICK_AIR.. Suppose I could just use right click instead (along with LeftControl of course).... -
[1.8] [Resolved] perform event on MouseClick only when Control key down
DJD replied to DJD's topic in Modder Support
Thanks ! I was looking at tutorials and they all had parts of what I needed but then again it seems things changed in 1.7 so I was a bit confused and some of the tutorials are for an earlier version.... -
Thanks ! I was on the right track and looking at looking at some nifty code from: Irtimaled: https://github.com/irtimaled BoundingBoxReloaded... Got to reading about the tessalotor thing on the minecraft.net page and figured it was the way to go... j ust couldn't figure where (which event) to fire it (still rudamentary knowledge of all the proper events at this point)....
-
What is the right way to render a bounding box for a selection of points on screen (with the blocks in the bounding box highlighted in some fashion (like transluscent red) ? There appear to be some functions way down in the source code for handling bounding boxes and was wondering how to maybe use those to achieve the effect (e.g. area selected by the player is highlighted).....
-
Thank you ! I figured that have to be an easier way than the byzantine ways I was hoping not to have to implement For any future readers, flag value 2 fit the bill... Also- had to place the blocks in 2 passes through the placement loops for things like torches etc to spawn properly.. 1st pass by property isFullCube() 2nd pass by property !isFullCube() Note: actually doors fail when you do the above. This seems to work: 1st- from bottom of selected cube to top- only blocks that are one blocklayer CUTOUT_MIPPED, or CUTOUT. 2nd- from top down to bottom of cube- blocks that are not FullCube 3rd- any left over (Top to Bottom)
-
Is there a way to force a block placement (and not process any of those blocks) until all blocks have been placed ? For example, if you are placing a crop down and there is no block under it (yet), is there a way to keep the engine from making it a harvested crop immediately until I tell it to process that block ? Currently I can cut/paste chunks of map, but if the grabbed area contains something that requires another block next to it that hasn't been placed yet, the engine processes it immediately instead of when all the blocks around it have been put in place... I would guess I would have to interrupt some event with a flag then reset the flag when the pasting is done.....
-
Using IExtendedEntityProperties for custom (non-simple) data types
DJD replied to DJD's topic in Modder Support
That points me in the right direction, thanks ! But is that the only way to handle keeping data on an entity ? -
Using IExtendedEntityProperties for custom (non-simple) data types
DJD replied to DJD's topic in Modder Support
I was under the impression that you get/set NBTTagCompound variables with methods like getDouble(value) and setDouble(value). How does one get/set the NBTTagCompount properties with a collection (or a complex data object) ? -
I've got an class that extends ExtendedEntityProperties that handles simple data (doubles, strings etc) for a player.... How does one go about implementing something more complex (like an array of doubles, or another custom object ?). I am looking for a way to properly handle something relatively simple (a collection of doubles) to something more complex (saving a buffer of data which contains collections of multiple objects that form a data structure)....
-
Suppose I could do that after I re-create the block. I would hope that any attachments (fences etc) are updated when the block is rotated as well. If not then it could get VERY ugly code It does not seem to work- I set the BlockProperties correctly and it places the block, but when I attempt the rotate after that (passing block.rotateBlock(((EntityPlayer) sender).worldObj, pos, rot) it returns true but doesn't seem to update the world. If its an older part of Forge, maybe its not working correctly since 1.8 ? Or is there another process to correctly using rotateBlock ? For the record for now I've settled on a direction re-map function (I know the direction the block was "grabbed" and the player direction) and just re-map the grabbed direction based on the player direction (simple enough but it would be nice not to have to manipulate the facing property directly)....
-
Thanks, I'll give it a look see I've got a cut/paste thing (multiple blocks) going for my mod and would prefer a better way to perform the paste portion with a rotation without having to directly manipulate the properties (which is messy).... I did something similiar to your class (although not as well thought out as yours for facing, but it seems messy.... I'm looking for a way to maybe use the block.rotateBlock instead as this will handle any other changes besides facing that have to be handled....
-
I need to be able to 1) get a block from the world (done). 2) rotate that block to the player's direction (done- but messy atm). 3) place the block back in the world (done). I was wondering what the best way to rotate a block in this instance (right now I am manipulating the property "FACING" (if it exists) in its IBlockState) to face the correct direction. Is there a way to change ANY block's facing without having to manipulate the properties directly ? Let me add a little more info as well: a) I get a block from the world, save all its data to a file and bring it back. (this works) b) I have to be able to bring the block back into existence and using the properties I've saved (this works) c) Its when bringing back the block into the world and having to change the facing (because its not facing the original direction that it was selected from) that I am dealing with (works, but messy and has to deal directly with the FACING property).
-
Thanks... so far this mod has pushed my knowledge of Java to new levels.... Gotta do some reading up on a Collection<? extends Comparable<?>>.....