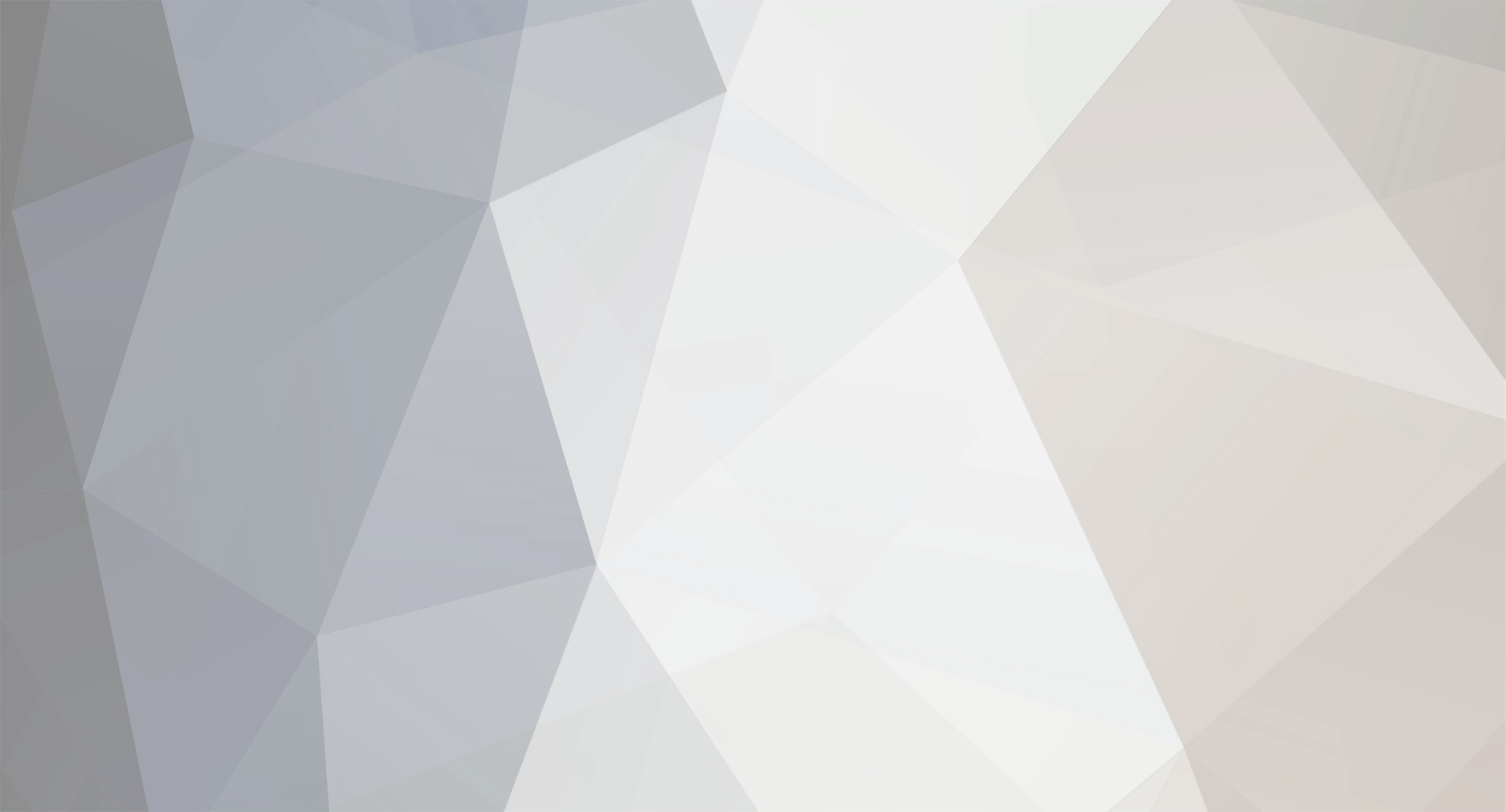
shucke
Members-
Posts
126 -
Joined
-
Last visited
Everything posted by shucke
-
Im trying to run my mod outside of mcp on minecraft with forge installed. but when i run minecraft im getting this error. http://pastebin.com/pfHspcZj# does anyone knows how to fix that?
-
you have to put the sprite in the spritesheet. and load it with the public String getTextureFile(){ return YourTextureFile; } method inside your item/block class
-
here is my world save data: package legendz.common; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.world.World; import net.minecraft.world.WorldSavedData; import net.minecraft.world.storage.MapStorage; public class WorldSaveData extends WorldSavedData { public static boolean[] alreadyGenerated = new boolean[8]; public WorldSaveData(String par1Str) { super(par1Str); } @Override public void readFromNBT(NBTTagCompound nbt) { for(int i = 0; i < alreadyGenerated.length; i++){ alreadyGenerated[i] = nbt.getBoolean("generated"+i); } } @Override public void writeToNBT(NBTTagCompound nbt) { for(int i = 0; i < alreadyGenerated.length; i++){ nbt.setBoolean("generated"+i, alreadyGenerated[i]); } } } i call it in the generate method so structures will only be generated once. here is the code where i call it: if(world.loadItemData(WorldSaveData.class, "LegendzWorldSaveData") == null){ WorldSavedData data = new WorldSaveData("LegendzWorldSaveData"); world.setItemData("LegendzWorldSaveData", data); } worldSaveData = (WorldSaveData) world.loadItemData(WorldSaveData.class, "LegendzWorldSaveData"); hope this makes it a little more clear for you. i had the same trouble figuring out how to store data in the world and is was a pain in the @$$
-
the code i provided is only working for animations which have to rotate only 1 part of the entity leg. im currently trying to figure out how to do these advanced animations by studying the Ender Dragon Model File. the ender dragon does it by adding the wing tip as a child of the main wing. so the wing tip move like the normal wing but has it own animation as wel. you only have to check if the Entity is walking or attacking. you could do that by setting up a variable in your model file and check if the entity is just walking or attacking. if you want to animate the arms differently you could do this. if(isAtacking){ // Setup your arms in the way you like }else{ //Normal arm animation } //Rest of the Animation
-
Animations are done by some calculations inside the SetRatationAngles() method in your model file. this.Head.rotateAngleY = par4 / (180F / (float)Math.PI); this.Head.rotateAngleX = par5 / (180F / (float)Math.PI); this.ArmRight.rotateAngleX = MathHelper.cos(par1 * 0.6662F + (float)Math.PI) * 2.0F * par2 * 0.5F; this.ArmLeeft.rotateAngleX = MathHelper.cos(par1 * 0.6662F) * 2.0F * par2 * 0.5F; this.ArmRight.rotateAngleZ = 0.0F; this.ArmLeeft.rotateAngleZ = 0.0F; this.LegRight.rotateAngleX = MathHelper.cos(par1 * 0.6662F) * 1.4F * par2; this.LegLeft.rotateAngleX = MathHelper.cos(par1 * 0.6662F + (float)Math.PI) * 1.4F * par2; this.LegRight.rotateAngleY = 0.0F; this.LegLeft.rotateAngleY = 0.0F; this lets my model to move exactly like the player in minecraft. i coppied this code from the ModelBiped Class three is an comment above the setRotationAngles of this class which should help you understand the way it works a little more. and setting up the model properly like Mazetar said is also a key thing to animations. otherwise you will get all messed up animattions
-
i tried to add the datawatcher to the player by using the playerLoggedIn event in the my ConnectionHandler. but when i try to get the datawatchers value it still gives me an null pointer exception. this is what i added to the connection handler: @Override public void playerLoggedIn(Player player, NetHandler netHandler, INetworkManager manager) { EntityPlayer play = (EntityPlayer) player; play.getDataWatcher().addObject(25, new Integer(0)); } i also registred it corretly. EDIT: nvm i figgured it out. the playerLoggedIn is only called on the server so i send a packet to the client saying add a datawatcher to the player and the problem was solved. thx for ur help
-
Thanks! it helped me understand datawatchers a whole lot more but i still dont get how i can intialize the datawatcher. you did it in EntityInit(). but since i wont edit the player's base class i cant add any code to the entityInit method. so how can i intilize the new datawatcher?
-
*facepalm* should have checked for a getter EDIT: So i got the NBT working but the DataWatcher still isnt working properly. Because i need to add a new datawatcher but i dont know how to do that properly. I have never worked with Datawatchers before so this is all new to me. So when i want to "Watch" my mana i get the error that it don't exist. So i tried to check if it exists and add a new DataWatcher if it doesnt. But how do i check if a datawatcher exists? i tried: if(player.getDataWatcher().getWatchableObjectInt(25) == 0) but it gave me an error for a nullpointer exception and when i replace == 0 with == null it gives me an error in eclipse that an int cant be null. Is there a way to check if the datawatcher exist and if it doesnt add it to the datawatcher?
-
the dataWatcher field is protected in the Entity class. is there any way to acces it?
-
And how can i add datawatchers to the player without actually editing a base class?
-
I searched a little bit and this is what i found. http://www.minecraftforum.net/topic/1597083-how-to-save-custom-data-with-a-player/ also using NBT. now i want to figure out where i should call this code from. are there any methods in for example my base class to do this kind of stuff?
-
What is the best way to add a new variable to a player on client side and server side? I'm trying to make a mana bar and a new inventory for the player but i can't figure out how to add a variable to the EntityPlayer. So i was wondering if there are any hooks for adding new variables to the player and saving them?
-
only if you want to make a Gui screen. otherwise you cant add slots into your gui. and thats the wole point of a guiContainer
-
i did that but it wont save. i set my variable to true and when i restart the game it is false again. if(world.loadItemData(WorldSaveData.class, "LegendzWorldSaveData") == null){ WorldSavedData data = new WorldSaveData("LegendzWorldSaveData"); world.setItemData("LegendzWorldSaveData", data); } WorldSaveData worldSaveData = (WorldSaveData) world.loadItemData(WorldSaveData.class, "LegendzWorldSaveData"); System.out.println(worldSaveData.alreadyGenerated[0]); i put this in the generate method in my WorldGenerator. and here is my WorldSaveData Class package legendz.common; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.world.World; import net.minecraft.world.WorldSavedData; import net.minecraft.world.storage.MapStorage; public class WorldSaveData extends WorldSavedData { public static boolean[] alreadyGenerated = new boolean[8]; public WorldSaveData(String par1Str) { super(par1Str); } @Override public void readFromNBT(NBTTagCompound nbt) { for(int i = 0; i < alreadyGenerated.length; i++){ alreadyGenerated[i] = nbt.getBoolean("generated"+i); } } @Override public void writeToNBT(NBTTagCompound nbt) { for(int i = 0; i < alreadyGenerated.length; i++){ nbt.setBoolean("generated"+i, alreadyGenerated[i]); } } }
-
the world save data way looks a lot easier to me then making a file. and since it is already implemented into minecraft i think im gonna go with that one. but do i need to register it or something? i cant find anything about it.
-
I tried to make a new WorldSavedData class but i cant firgure out how it works. can someone explain it to me pls? i can't find anything about it on the internet to.
-
you didnt intialized your block. there are a lot of tutorials about this. just search for them.
-
Your problem is the packet handler class. first of all you named you class ClientPacketHandler but in your networkMod annotation you put PacketHandler.class. second you didnt put any code in your packet handler to actually handle the packet. the packet reaches the server but the server doesnt know what to do with it so ignores it. there is an excellent packet hadling tutorial on the forge wiki. http://www.minecraftforge.net/wiki/Packet_Handling i would advice to follow this tutorial and if you got any questions left ask them
-
How can i make a structure generate only once? i want to make a structure which generates only once and not everytime a new chunk is beeing generated. i got the code to work but the problem is i used a variable to check if the structure is already generated. but when i exit the game it resets the variable and the structure is beeing generated again. so can i write the variable to NBT or something? or is there another way to save variables in for example in my baseclass or CommonProxy?
-
Hey, how can i make multipile entities while only using a single class? im trying to make an quest npc and i got a few quests. now i dont want to create a new class for every NPC that holds a quest. so how can i create different types of entity's with only using 1 main class to hold the behaviour. i tried making it about the same as Block's and Item's but i cant get it to work since the entity's require a World variable. can someone pls help me with this?
-
[SOLVED] How do i spawn an enitity on client side?
shucke replied to laifsjo's topic in Modder Support
A packet is used sending informartion from the client to the server or visa versa. so what you need to is send a packet from the client to the server. the tutorial describes how to do that. i dont think its very helpfull to code it for you since you dont understand packets yet. so my advice is to just read to tutorial over and over again and try to experiment some with the code so you can see what it does. if you have any questions about the turorial ask them here instead of saying i dont understand. try to explain what you dont get about it. -
just a tip to get you going in the right direction. look at x and z variable. you defined it to be 0. so it only checks what the biome is at 0,0. hope this helps you. also you removed the ! mark from the if statement and left it at this. if(world.getBiomeGenForCoords(x, z) = BiomeGenBase.ocean){ // NO ! mark when you put only one = it try's to set the left hand variable to the right hand variable. and you dont want that to happen. so if you want to compare those variables you have to put a double = instead of a single one.
-
Hello, actually the title says t all. i want to render a texture above a entity. for example you see in most RPG's when a NPC has a available quest it renders a exclamation mark above its head. i want to do the same thing but i don't have a clue how to do it. some info on how to do this would be much appreciated
-
Hello, i made a new dimension and now im trying to generate ores in that dimension. but somehow it doesnt generate any ores in the dimension. when i start up minecraft it does print the line i setup in the generation method in the eclipse console but just for the normal dimension. anyway here is my code. and i did registered the dimension on id nr 7. public class WorldGenerator implements IWorldGenerator{ private boolean alreadyRendered = false; @Override public void generate(Random random, int chunkX, int chunkZ, World world, IChunkProvider chunkGenerator, IChunkProvider chunkProvider) { int id = world.provider.dimensionId; switch(id){ case 0: //generateWatchtower(world, random, chunkX*16, chunkZ*16); break; case 7: System.out.println("generating 7 called"); generateBeach(world, random, chunkX*16, chunkZ*16); break; } } private void generateBeach(World world, Random random, int i, int j) { generatePalmTree(world, random, i*16, j*16); genrateAquaticOre(world, random, i*16, j*16); } private void genrateAquaticOre(World world, Random random, int i, int j) { int Xcoord = i + random.nextInt(16); int Ycoord = random.nextInt(60); int Zcoord = j + random.nextInt(16); for(int k = 0; k < 50; k++){ (new WorldGenMinable(BaseLegendz.aquaticOre.blockID, 4)).generate(world, random, Xcoord, Ycoord, Zcoord); } } private void generatePalmTree(World world, Random random, int i, int j) { int Xcoord1 = i + random.nextInt(16); int Zcoord1 = j + random.nextInt(16); for(int var1 = 0; var1 < 80; var1++){ if(world.canBlockSeeTheSky(Xcoord1, var1, Zcoord1) && world.getBlockId(Xcoord1, var1, Zcoord1) == Block.sand.blockID){ new WorldGenPalmTree().generate(world, random, Xcoord1, var1+1, Zcoord1); System.out.println("generated a palm tree!"); } } } } the palm tree's arent generating aswell so i think there is something wrong with my switch statement. because they do generate when i just put them in the generate() methoed
-
thank you verry much works like a charm now!