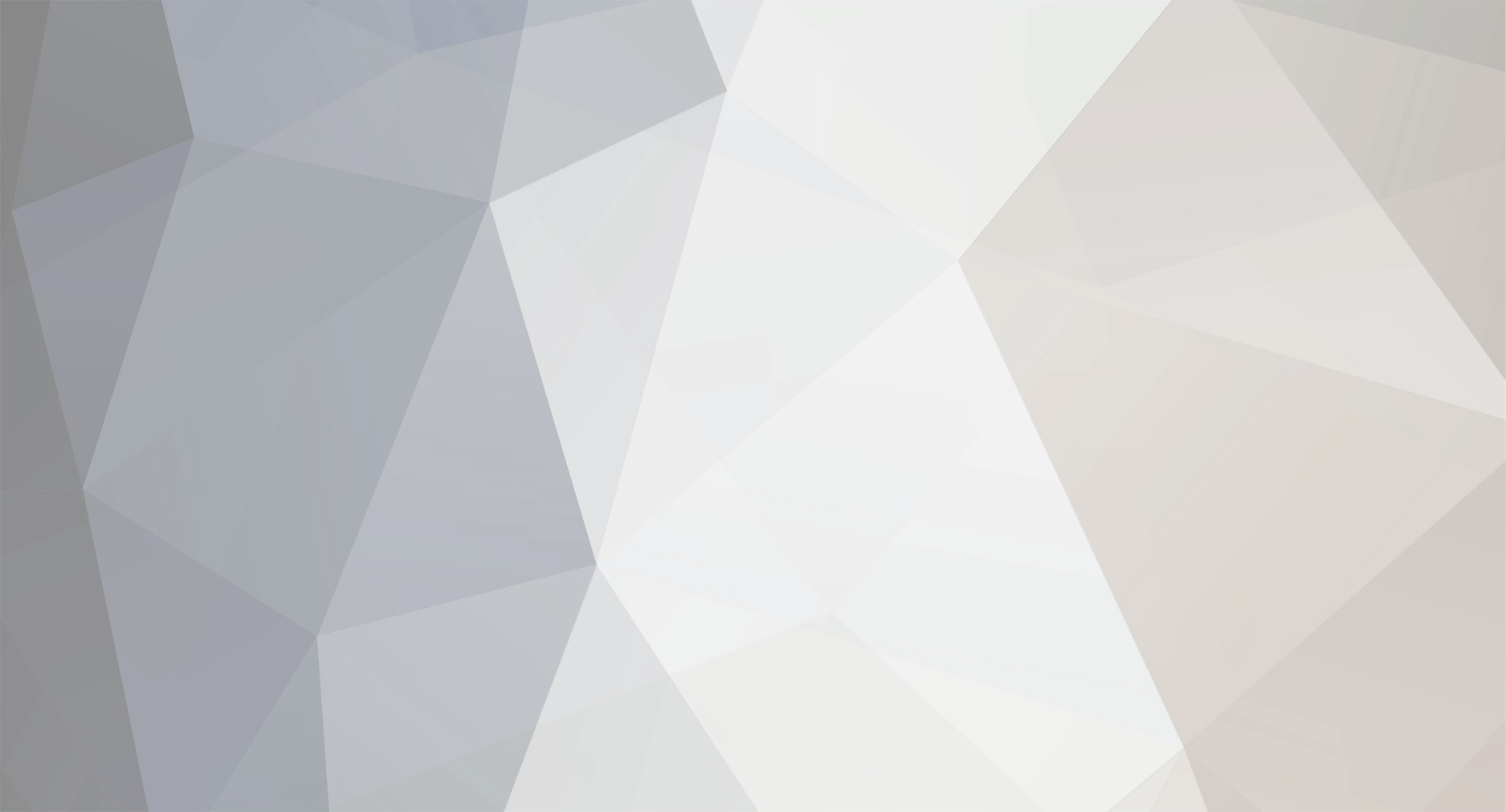
DF_Waffle
-
Posts
7 -
Joined
-
Last visited
Posts posted by DF_Waffle
-
-
Ok so I added 2 lines to print both the reading and writing and its printing both but not sure if its actually doing it.
[00:27:16] [server thread/INFO]: [com.waffle.to.tileentity.TOTileEntity:writeToNBT:208]: WRITING NBT DATA
[00:27:10] [server thread/INFO]: [com.waffle.to.tileentity.TOTileEntity:readFromNBT:185]: READING NBT DATA
-
Ok thanks, going to try and figure it out but not really sure because this is my first tile entity not really sure how to call these
-
My tileenetity is not saving its items. I can place items in the slots but once I leave and save the world and go back in the items are deleted.
TileEntity:
package com.waffle.to.tileentity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.IInventory; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.nbt.NBTTagList; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.text.ITextComponent; import java.util.ArrayList; public class TOTileEntity extends TileEntity implements IInventory{ private ItemStack[] stacks = new ItemStack[9]; private boolean aBoolean; private byte aByte; private short aShort; private int anInt; private long aLong; private float aFloat; private double aDouble; private String aString; private byte[] aByteArray; private int[] anIntArray; private ItemStack anItemStack; private ArrayList aList = new ArrayList(); private ItemStack[] inventory; private String customName; public TOTileEntity(){ this.inventory = new ItemStack[this.getSizeInventory()]; } public String getCustomName(){ return this.customName; } public void setCustomName(String customName){ this.customName = customName; } @Override public String getName(){ return this.hasCustomName() ? this.customName : "container.to_tile_entity"; } @Override public boolean hasCustomName(){ return this.customName != null && !this.customName.equals(""); } @Override public ITextComponent getDisplayName() { return null; } @Override //How many slots public int getSizeInventory(){ return 9; } @Override public ItemStack getStackInSlot(int index) { if (index < 0 || index >= this.getSizeInventory()) return null; return this.inventory[index]; } @Override public ItemStack decrStackSize(int index, int count) { if (this.getStackInSlot(index) != null) { ItemStack itemstack; if (this.getStackInSlot(index).stackSize <= count) { itemstack = this.getStackInSlot(index); this.setInventorySlotContents(index, null); this.markDirty(); return itemstack; } else { itemstack = this.getStackInSlot(index).splitStack(count); if (this.getStackInSlot(index).stackSize <= 0) { this.setInventorySlotContents(index, null); } else { //Just to show that changes happened this.setInventorySlotContents(index, this.getStackInSlot(index)); } this.markDirty(); return itemstack; } } else { return null; } } @Override public ItemStack removeStackFromSlot(int index) { return null; } //@Override public ItemStack getStackInSlotOnClosing(int index) { ItemStack stack = this.getStackInSlot(index); this.setInventorySlotContents(index, null); return stack; } @Override public void setInventorySlotContents(int index, ItemStack stack) { if (index < 0 || index >= this.getSizeInventory()) return; if (stack != null && stack.stackSize > this.getInventoryStackLimit()) stack.stackSize = this.getInventoryStackLimit(); if (stack != null && stack.stackSize == 0) stack = null; this.inventory[index] = stack; this.markDirty(); } @Override //Stack Limit per slot public int getInventoryStackLimit(){ return 64; } @Override public boolean isUseableByPlayer(EntityPlayer player) { return false; } //@Override public boolean isUsableByPlayer(EntityPlayer player){ return this.worldObj.getTileEntity(this.getPos()) == this && player.getDistanceSq(this.pos.add(0.5, 0.5, 0.5)) <= 64; } @Override public void openInventory(EntityPlayer player) { } @Override public void closeInventory(EntityPlayer player) { } @Override public boolean isItemValidForSlot(int index, ItemStack stack){ return true; } @Override public int getField(int id) { return 0; } @Override public void setField(int id, int value) { } @Override public int getFieldCount() { return 0; } @Override public void clear(){ for (int i = 0; i < this.getSizeInventory(); i++) this.setInventorySlotContents(i, null); } @Override public void writeToNBT(NBTTagCompound nbt) { super.writeToNBT(nbt); NBTTagList list = new NBTTagList(); for (int i = 0; i < this.getSizeInventory(); ++i) { if (this.getStackInSlot(i) != null) { NBTTagCompound stackTag = new NBTTagCompound(); stackTag.setByte("Slot", (byte) i); this.getStackInSlot(i).writeToNBT(stackTag); list.appendTag(stackTag); } nbt.setTag("Items", list); if (this.hasCustomName()) { nbt.setString("CustomName", this.getCustomName()); } } } @Override public void readFromNBT(NBTTagCompound nbt){ super.readFromNBT(nbt); NBTTagList list = nbt.getTagList("Items", 10); for (int i = 0; i < list.tagCount(); ++i) { NBTTagCompound stackTag = list.getCompoundTagAt(i); int slot = stackTag.getByte("Slot") & 255; this.setInventorySlotContents(slot, ItemStack.loadItemStackFromNBT(stackTag)); } if (nbt.hasKey("CustomName", ){ this.setCustomName(nbt.getString("CustomName")); } } //Implement ITickable for this to print /*@Override public void update(){ if (!this.worldObj.isRemote) System.out.println("TestBlock TileEntity"); }*/ }
-
I want to make a recipe for a certain player like my self that I can craft a Dev item. like if playername = waffle then recipe is.....
-
How can I give only me a Dev item. How can I call it so its only searching for my name and no one else unless specified.
-
Is there any way to give my character a item when I'm in game? like if player .... then give item ....
[SOLVED][1.9] TileEntity not saving Data
in Modder Support
Posted
I think I implemented the getDescriptionPacket and onDataPacket but nothing happened the same thing is still happening.