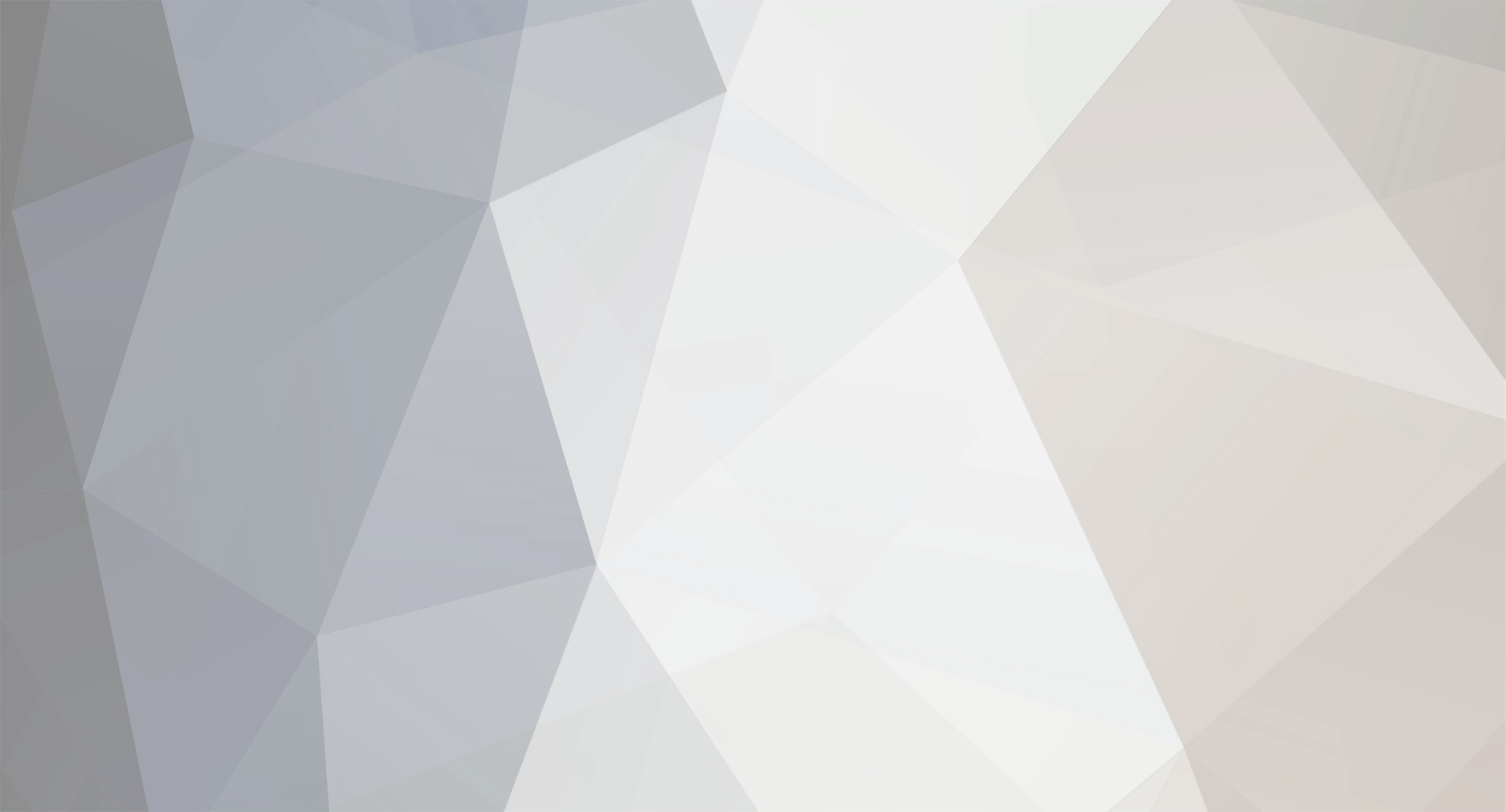
Atomos
Members-
Posts
10 -
Joined
-
Last visited
Everything posted by Atomos
-
[1.8] world.setBlockState not actually creating blocks
Atomos replied to Atomos's topic in Modder Support
And now it works, huzzah! -
[1.8] world.setBlockState not actually creating blocks
Atomos replied to Atomos's topic in Modder Support
Ok that explains it then. I call minecraft.getminecraft.theWorld from my setblock method. -
[1.8] world.setBlockState not actually creating blocks
Atomos replied to Atomos's topic in Modder Support
My updated on right click: public ItemStack onItemRightClick(ItemStack Item, World world, EntityPlayer player) { double x = player.posX; double y = player.posY; double z = player.posZ; BlockPos playerLoc = new BlockPos(x,y,z); if (player.inventory.hasItem(Items.diamond)&&player.inventory.hasItem(Items.ghast_tear)) { if (!world.isRemote) { RoomData room = new RoomData(); room.width = 10; room.height = 4; room.length = 10; room.isIlluminated=true; room.roomValues.add(0); // room.roomValues.add(1); // room.roomValues.add(2); // room.roomValues.add(3); room.lightSpacing=5; room.center = player.getPosition(); StoneDungeon.Generate(StoneDungeon.CreateData(room,5)); } } else { if (!world.isRemote) { player.addChatComponentMessage(new ChatComponentTranslation("You do not have a catalyst or a flow source!",new Object[0])); } } return Item; } and my stoneDungeon class is in an earleir reply -
Are you creating a surface ore? If you are than perhaps you could create a method for your y coordinate that creates a blockPos list of all the blocks in the x z area that is randomized by your code, then iterate through it till the block type is grass, then add 1, and you should have it. Just a suggestion. Also, please know what you are saying before you post, it really helps everyone else.
-
[1.8] world.setBlockState not actually creating blocks
Atomos replied to Atomos's topic in Modder Support
Ok, it is no longer crashing, but when i run it server side using !world.isRemote, the same thing happens as before. The blocks are not actually being created (it did however fix one of my other problems) -
what exactly would the negative signal do?
-
[1.8] world.setBlockState not actually creating blocks
Atomos replied to Atomos's topic in Modder Support
And using if (!world.isRemote) just causes a crash -
[1.8] world.setBlockState not actually creating blocks
Atomos replied to Atomos's topic in Modder Support
I already know that I am running the method client side, but how am I supposed to run it server side? Here is my onItemRightClick method: public ItemStack onItemRightClick(ItemStack Item, World world, EntityPlayer player) { double x = player.posX; double y = player.posY; double z = player.posZ; BlockPos playerLoc = new BlockPos(x,y,z); if (player.inventory.hasItem(Items.diamond)&&player.inventory.hasItem(Items.ghast_tear)) { RoomData room = new RoomData(); room.width = 10; room.height = 4; room.length = 10; room.isIlluminated=true; room.roomValues.add(0); // room.roomValues.add(1); // room.roomValues.add(2); // room.roomValues.add(3); room.lightSpacing=5; room.center = player.getPosition(); StoneDungeon.Generate(StoneDungeon.CreateData(room,5)); } else { player.addChatComponentMessage(new ChatComponentTranslation("You do not have a catalyst or a flow source!",new Object[0])); } return Item; } And here is the stoneDungeon class: package dungeonGenerator.algorithms; import net.minecraft.block.Block; import net.minecraft.block.state.IBlockState; import net.minecraft.client.Minecraft; import net.minecraft.init.Blocks; import net.minecraft.util.BlockPos; import net.minecraft.util.ChatComponentTranslation; import java.util.*; import net.minecraft.world.World; import net.minecraftforge.event.world.WorldEvent; import net.minecraftforge.event.world.WorldEvent.*; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; import java.util.*; public class StoneDungeon { public static int roomCount = 0; public static List<RoomData> CreateData(RoomData base, int roomsCount) { List<RoomData> output = new ArrayList<RoomData>(); output.add(base); BlockPos previousPos = new BlockPos(base.center); List<RoomData> previousRooms = new ArrayList<RoomData>(); List<RoomData> tempPreviousRooms = new ArrayList<RoomData>(); previousRooms.add(base); for (int i = 0;i<roomsCount;i++) { for (RoomData previousRoom : previousRooms) { for (int location : previousRoom.roomValues) { RoomData room = new RoomData(); room.height = base.height; room.width = base.width; room.length = base.length; room.isIlluminated = true; room.lightSpacing = base.lightSpacing; double x = previousRoom.center.getX(); double y = previousRoom.center.getY(); double z = previousRoom.center.getZ(); if (location == 0) { room.center = new BlockPos(x,y,z+previousRoom.length-2); room.roomValues.add(1); room.roomValues.add(random()); room.roomValues.add(random()); room.roomValues.add(random()); output.add(room); tempPreviousRooms.add(room); } if (location == 1) { room.center = new BlockPos(x,y,z-previousRoom.length+2); room.roomValues.add(0); room.roomValues.add(random()); room.roomValues.add(random()); room.roomValues.add(random()); output.add(room); tempPreviousRooms.add(room); } if (location == 2) { room.center = new BlockPos(x+previousRoom.width-2,y,z); room.roomValues.add(3); room.roomValues.add(random()); room.roomValues.add(random()); room.roomValues.add(random()); output.add(room); tempPreviousRooms.add(room); } if (location == 3) { room.center = new BlockPos(x-previousRoom.width+2,y,z); room.roomValues.add(2); room.roomValues.add(random()); room.roomValues.add(random()); room.roomValues.add(random()); output.add(room); tempPreviousRooms.add(room); } } } previousRooms.addAll(tempPreviousRooms); tempPreviousRooms.clear(); } Minecraft.getMinecraft().thePlayer.addChatComponentMessage(new ChatComponentTranslation("Creating "+output.size()+" Rooms")); return output; } public static void Generate(List<RoomData> rooms) { //Define Variables for (RoomData room : rooms) { double x = room.center.getX(); double y = room.center.getY()-1; double z = room.center.getZ(); int dirUp = room.length/2; int dirSide = room.width/2; int height = room.height; int lightSpacing = room.lightSpacing; //Generate the floor for (int i = 0;i < dirSide;i++){ for (int j = 0;j < dirUp;j++) { setBlock (Blocks.stonebrick,new BlockPos(x+i,y,z+j)); setBlock (Blocks.stonebrick,new BlockPos(x-i,y,z+j)); setBlock (Blocks.stonebrick,new BlockPos(x+i,y,z-j)); setBlock (Blocks.stonebrick, new BlockPos(x-i,y,z-j)); //Set illumination Blocks if (room.isIlluminated == true) { setBlock (Blocks.glowstone,new BlockPos(x,y,z)); if (i%lightSpacing==0) { setBlock(Blocks.glowstone,new BlockPos(x+i,y,z)); setBlock(Blocks.glowstone,new BlockPos(x-i,y,z)); } if (j%lightSpacing==0) { setBlock(Blocks.glowstone,new BlockPos(x,y,z+j)); setBlock(Blocks.glowstone,new BlockPos(x,y,z-j)); } if (i%lightSpacing==0&&j%lightSpacing==0) { setBlock(Blocks.glowstone,new BlockPos(x+i,y,z+j)); setBlock(Blocks.glowstone,new BlockPos(x+i,y,z-j)); setBlock(Blocks.glowstone,new BlockPos(x-i,y,z+j)); setBlock(Blocks.glowstone,new BlockPos(x-i,y,z-j)); } } } } //set ceiling for (int i = 0;i < dirSide;i++){ for (int j = 0;j < dirUp;j++) { setBlock (Blocks.stonebrick,new BlockPos(x+i,y+height,z+j)); setBlock (Blocks.stonebrick,new BlockPos(x-i,y+height,z+j)); setBlock (Blocks.stonebrick,new BlockPos(x+i,y+height,z-j)); setBlock (Blocks.stonebrick, new BlockPos(x-i,y+height,z-j)); } } //Break out internals for (int i = 0;i<dirSide;i++) { for (int j = 0;j<dirUp;j++) { for (int k = 1;k<height;k++) { deleteBlock (new BlockPos(x+i-1,y+k,z+j-1)); deleteBlock (new BlockPos(x-i+1,y+k,z+j-1)); deleteBlock (new BlockPos(x+i-1,y+k,z-j+1)); deleteBlock (new BlockPos(x-i+1,y+k,z-j+1)); } } } //set left & right walls for (int k = 0;k < height;k++) { for (int j = 0;j < dirUp;j++) { setBlock (Blocks.stonebrick, new BlockPos(x+dirSide-1,y+k,z+j)); setBlock(Blocks.stonebrick, new BlockPos(x-dirSide+1,y+k,z+j)); setBlock (Blocks.stonebrick, new BlockPos(x+dirSide-1,y+k,z-j)); setBlock(Blocks.stonebrick, new BlockPos(x-dirSide+1,y+k,z-j)); } } //set up & down walls for (int k = 0;k < height;k++) { for (int i = 0;i < dirUp;i++) { setBlock (Blocks.stonebrick, new BlockPos(x+i,y+k,z+dirUp-1)); setBlock(Blocks.stonebrick, new BlockPos(x+i,y+k,z-dirUp+1)); setBlock (Blocks.stonebrick, new BlockPos(x-i,y+k,z+dirUp-1)); setBlock(Blocks.stonebrick, new BlockPos(x-i,y+k,z-dirUp+1)); } } //set doors if (room.roomValues.contains(1)==true) { deleteBlock(new BlockPos(x,y+1,z-dirUp+1)); deleteBlock(new BlockPos(x,y+2,z-dirUp+1)); } if (room.roomValues.contains(0)==true) { deleteBlock(new BlockPos(x,y+1,z+dirUp-1)); deleteBlock(new BlockPos(x,y+2,z+dirUp-1)); } if (room.roomValues.contains(3)==true) { deleteBlock(new BlockPos(x+dirSide-1,y+1,z)); deleteBlock(new BlockPos(x+dirSide-1,y+2,z)); } if (room.roomValues.contains(2)==true) { deleteBlock(new BlockPos(x-dirSide+1,y+1,z)); deleteBlock(new BlockPos(x-dirSide+1,y+2,z)); } } } public static void setBlock(Block type,BlockPos location) { World world = Minecraft.getMinecraft().theWorld; IBlockState blockState = type.getDefaultState(); world.setBlockState(location, blockState); } public static void deleteBlock(BlockPos location) { World world = Minecraft.getMinecraft().theWorld; world.setBlockToAir(location); } public static int random() { Random rand = new Random(); return rand.nextInt(4); } } -
[1.8] world.setBlockState not actually creating blocks
Atomos replied to Atomos's topic in Modder Support
Yes, I am calling it on client from my onItemRightClick function on a custom item. How do I call it from server side? (sorry if this is a noob question, i am more apt to c# then java) -
In my mod, I set a lot of blocks. However when I use the world.setBlockState(location, blockstate) command, the block is created, but is then deleted once i reload that world. When I delete blocks, a similar thing happens. That is, I cannot walk through the generated air, and the old blocks reappear on reload. My setBlock method is as follows: public static void setBlock(Block type,BlockPos location) { World world = Minecraft.getMinecraft().theWorld; IBlockState blockState = type.getDefaultState(); world.setBlockState(location, blockState); } and my deleteBlock method public static void deleteBlock(BlockPos location) { World world = Minecraft.getMinecraft().theWorld; world.setBlockToAir(location); }