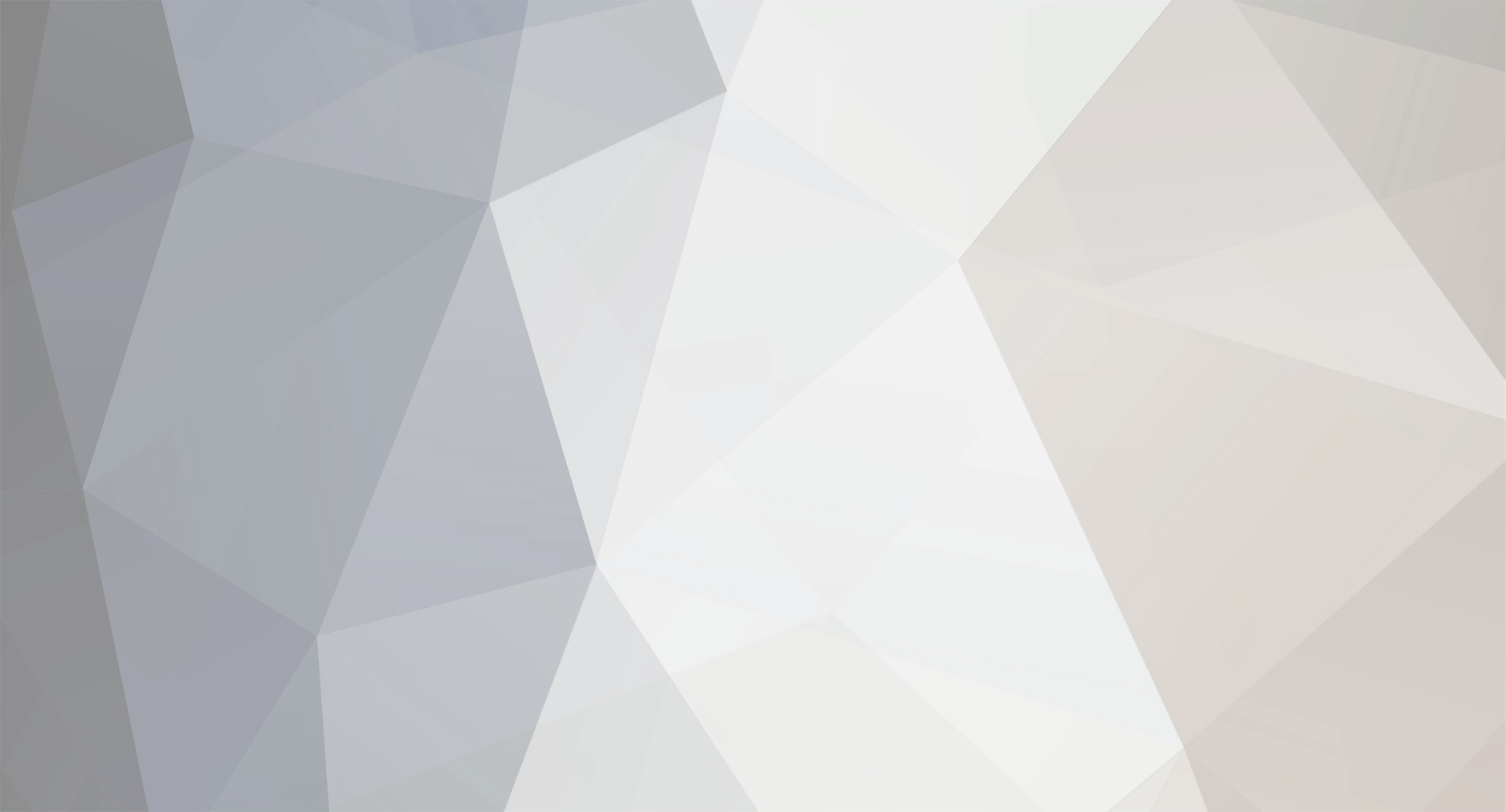
AshIndigo
Members-
Posts
40 -
Joined
-
Last visited
Everything posted by AshIndigo
-
In my mod I have a furnace which will a base item and an additional item but when I try to change the resulting nbt of the item I cant becuase one of the methods is void and my method for recipes isnt void. Is there another way to change the nbt data? Here is my code ForgeRecipes package com.ashindigo.alloycraft.lib; import com.ashindigo.alloycraft.AlloycraftItems; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; public class ForgeRecipes { public ForgeRecipes() { } public static ItemStack getSmeltingResult(Item item, Item item2) { return getOutput(item, item2); } public static ItemStack getOutput(Item item, Item item2) { // TODO get nbt setup if (item == Items.iron_ingot && item2 == AlloycraftItems.alloy || item == AlloycraftItems.alloy && item2 == Items.iron_ingot) { return new ItemStack(AlloycraftItems.alloy, 2); } return null; } } ForgeTileEntity package com.ashindigo.alloycraft.tileentites; import com.ashindigo.alloycraft.blocks.ForgeBlock; import com.ashindigo.alloycraft.lib.ForgeRecipes; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.inventory.ISidedInventory; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.nbt.NBTTagList; import net.minecraft.tileentity.TileEntity; public class ForgeTileEntity extends TileEntity implements ISidedInventory { private ItemStack slots[]; public int dualPower; public int dualCookTime; public static final int maxPower = 10000; public static final int smeltingSpeed = 100; private static final int[] slots_top = new int[] {0, 1}; private static final int[] slots_bottom = new int[] {3}; private static final int[] slots_side = new int[] {2}; private String customName; public ForgeTileEntity() { slots = new ItemStack[4]; } @Override public int getSizeInventory() { return slots.length; } @Override public ItemStack getStackInSlot(int i) { return slots[i]; } @Override public ItemStack getStackInSlotOnClosing(int i) { if (slots[i] != null) { ItemStack itemstack = slots[i]; slots[i] = null; return itemstack; }else{ return null; } } @Override public void setInventorySlotContents(int i, ItemStack itemstack) { slots[i] = itemstack; if (itemstack != null && itemstack.stackSize > getInventoryStackLimit()) { itemstack.stackSize = getInventoryStackLimit(); } } @Override public int[] getAccessibleSlotsFromSide(int i) { return i == 0 ? slots_bottom : (i == 1 ? slots_top : slots_side); } @Override public int getInventoryStackLimit() { return 64; } @Override public boolean isUseableByPlayer(EntityPlayer player) { if (worldObj.getTileEntity(xCoord, yCoord, zCoord) != this) { return false; }else{ return player.getDistanceSq((double)xCoord + 0.5D, (double)yCoord + 0.5D, (double)zCoord + 0.5D) <= 64; } } public void openInventory() {} public void closeInventory() {} @Override public boolean isItemValidForSlot(int i, ItemStack itemstack) { return i == 2 ? false : (i == 1 ? hasItemPower(itemstack) : true); } public boolean hasItemPower(ItemStack itemstack) { return getItemPower(itemstack) > 0; } private static int getItemPower (ItemStack itemstack) { if (itemstack == null) { return 0; }else{ Item item = itemstack.getItem(); if (item == Items.coal) return 50; if (item == Item.getItemFromBlock(Blocks.coal_block)) return 450; return 0; } } public ItemStack decrStackSize(int i, int j) { if (slots[i] != null) { if (slots[i].stackSize <= j) { ItemStack itemstack = slots[i]; slots[i] = null; return itemstack; } ItemStack itemstack1 = slots[i].splitStack(j); if (slots[i].stackSize == 0) { slots[i] = null; } return itemstack1; }else{ return null; } } public void readFromNBT (NBTTagCompound nbt) { super.readFromNBT(nbt); NBTTagList list = nbt.getTagList("Items", 10); slots = new ItemStack[getSizeInventory()]; for (int i = 0; i < list.tagCount(); i++) { NBTTagCompound nbt1 = (NBTTagCompound)list.getCompoundTagAt(i); byte b0 = nbt1.getByte("Slot"); if (b0 >= 0 && b0 < slots.length) { slots[b0] = ItemStack.loadItemStackFromNBT(nbt1); } } dualPower = nbt.getShort("PowerTime"); dualCookTime = nbt.getShort("CookTime"); } public void writeToNBT(NBTTagCompound nbt) { super.writeToNBT(nbt); nbt.setShort("PowerTime", (short)dualPower); nbt.setShort("CookTime", (short)dualCookTime); NBTTagList list = new NBTTagList(); for (int i = 0; i < slots.length; i++) { if (slots[i] != null) { NBTTagCompound nbt1 = new NBTTagCompound(); nbt1.setByte("Slot", (byte)i); slots[i].writeToNBT(nbt1); list.appendTag(nbt1); } } nbt.setTag("Items", list); } @Override public String getInventoryName() { return "Alloy Furnace"; } @Override public boolean canInsertItem(int var1, ItemStack itemstack, int var3) { return this.isItemValidForSlot(var1, itemstack); } @Override public boolean canExtractItem(int i, ItemStack itemstack, int j) { return j != 0 || i != 1 || itemstack.getItem() == Items.bucket; } @Override public boolean hasCustomInventoryName() { return this.customName != null && this.customName.length() > 0; } public int getSmeltingProgressScaled(int i) { return (dualCookTime * i) / this.smeltingSpeed; } public int getPowerRemainingScaled(int i) { return (dualPower * i) / maxPower; } private boolean canSmelt() { if (slots[0] == null || slots[1] == null) { return false; } ItemStack itemstack = ForgeRecipes.getSmeltingResult(slots[0].getItem(), slots[1].getItem()); if (itemstack == null) { return false; } if (slots[3] == null) { return true; } if (!slots[3].isItemEqual(itemstack)) { return false; } if (slots[3].stackSize < getInventoryStackLimit() && slots[3].stackSize < slots[3].getMaxStackSize()) { return true; }else{ return slots[3].stackSize < itemstack.getMaxStackSize(); } } private void mashItem() { if (canSmelt()) { ItemStack itemstack = ForgeRecipes.getSmeltingResult(slots[0].getItem(), slots[1].getItem()); if (slots[3] == null) { slots[3] = itemstack.copy(); }else if (slots[3].isItemEqual(itemstack)) { slots[3].stackSize += itemstack.stackSize; } for (int i = 0; i < 2; i++) { if (slots[i].stackSize <= 0) { slots[i] = new ItemStack(slots[i].getItem().setFull3D()); }else{ slots[i].stackSize--; } if (slots[i].stackSize <= 0){ slots[i] = null; } } } } public boolean hasPower() { return dualPower > 0; } public boolean isSmelting() { return this.dualCookTime > 0; } public void updateEntity() { boolean flag = this.hasPower(); boolean flag1= false; if(hasPower() && this.isSmelting()) { this.dualPower--; } if(!worldObj.isRemote) { if (this.hasItemPower(this.slots[2]) && this.dualPower < (this.maxPower - this.getItemPower(this.slots[2]))) { this.dualPower += getItemPower(this.slots[2]); if(this.slots[2] != null) { flag1 = true; this.slots[2].stackSize--; if(this.slots[2].stackSize == 0) { this.slots[2] = this.slots[2].getItem().getContainerItem(this.slots[2]); } } } if (hasPower() && canSmelt()) { dualCookTime++; if (this.dualCookTime == this.smeltingSpeed) { this.dualCookTime = 0; this.mashItem(); flag1 = true; } }else{ dualCookTime = 0; } if (flag != this.isSmelting()) { flag1 = true; ForgeBlock.updateBlockState(this.isSmelting(), this.worldObj, this.xCoord, this.yCoord, this.zCoord); } } if (flag1) { this.markDirty(); } } }
-
[1.7.10] Configuration Comments not working
AshIndigo replied to Elrol_Arrowsend's topic in Modder Support
Tip for future posts: Post your complete code But the way has changed config's are now declared as variables. hardcoremode = config.getBoolean("Is Hardcore Mode enabled?", "general", false, "Enables Hardcore mode if true Default: False"); Here is an example of a boolean config. The first parameter is the name, the second is category I would just stick with general, the third is the default value, and the fourth is the comment. If you know some java then you should know how to make this work. Feel free to correct if I'm wrong on any parts of this. -
I would have never noticed that! Thank you so much!
-
The tutorials are for 1.6.2 but with some work you could get them working on 1.7.10. But like Jedispencer21 said take a look at those classes.
-
So I have modified my code now with the changes you described but now I can't open the Gui anymore because the game crashes New crash log: http://pastebin.com/aVXYtuJS
-
I've been working on a custom machine for my mod but when I try to insert items into the slots the game crashes. I have been unable to figure out why the game is crashing. I posted a link to my github for the classes that may be needed. Crashlog: http://pastebin.com/dyhENRsK Github Link: https://github.com/AshIndigo/Alloycraft-Reborn/tree/master/src/main/java/com/ashindigo/alloycraft https://github.com/AshIndigo/Alloycraft-Reborn/tree/master/src/main/java/com/ashindigo/utils
-
Alright I'll start looking into those classes. Thank you so much though for helping me with this.
-
Alright sweet so I got the class made now do I use a if statement to get if the items are there and then replace it with the other item?
-
No not yet Here I made an example I have a diamond sword with 5 damage and then if I craft it with lapis then I get an iron sword with the same damage as the diamond sword.
-
Thanks for responding but I just forgot to change the resulting item to a different one.
-
For my mod I have tools which can have different looks but I need to keep the damage in the resulting item when I craft the new tool. GameRegistry.addRecipe(new ItemStack(AlloycraftItems.aluminumtooltest, 1, 1), new Object[]{ " A ", " B ", " ", 'B', Items.dye, 'A', new ItemStack(AlloycraftItems.aluminumtooltest, WILDCARD_VALUE, WILDCARD_VALUE) });
-
i used the solution but now it crashes whenever i open a gui Error log:
-
It said it cant find the files but i find the folder in a new location and i try copying it it still wont work Below is my client proxy code and i even tried a tutorial please reply if you the fix using eclipse
-
Crash When trying to connect to a forge server (Solved)
AshIndigo replied to AshIndigo's topic in Support & Bug Reports
Tried it without portal gun server and client Minecraft Freezes and my server said i was disconnnected and here is the server log i know it said no class found but it still loaded