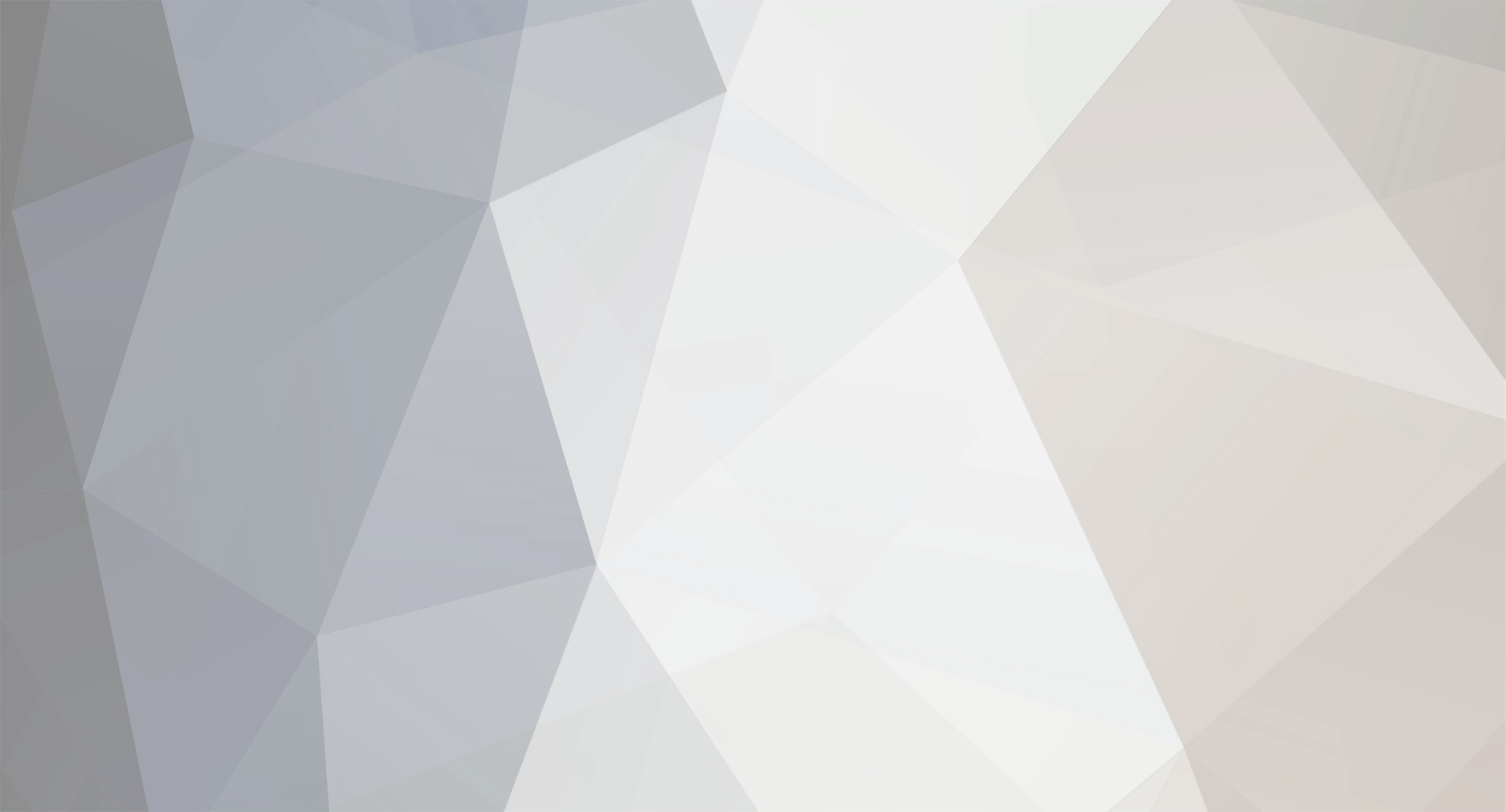
OrangeVillager61
Members-
Posts
339 -
Joined
-
Last visited
Everything posted by OrangeVillager61
-
[1.12] Butcher Harvesting Meat Doesn't do Anything
OrangeVillager61 replied to OrangeVillager61's topic in Modder Support
Hmm, I changed the MutexBits and it didn't do anything. Also, the AI is crashing the game when ever I relog at the updateTask at this line: if (this.villagerObj.getDistanceSqToEntity(this.animal) > 2.25D) However, it is not giving me the println that I put at the start of updateTask, it doesn't make sense. -
[1.12] Butcher Harvesting Meat Doesn't do Anything
OrangeVillager61 replied to OrangeVillager61's topic in Modder Support
What are the mutex bits? -
[1.12] Butcher Harvesting Meat Doesn't do Anything
OrangeVillager61 replied to OrangeVillager61's topic in Modder Support
Github link: https://github.com/Orange1861/Improved-Villagers/tree/Orange1861-1.12_Version -
[1.12] Butcher Harvesting Meat Doesn't do Anything
OrangeVillager61 replied to OrangeVillager61's topic in Modder Support
Forgot to put where it was being called: protected void setAdditionalAItasks() { if (!this.areAdditionalTasksSet) { this.areAdditionalTasksSet = true; if (this.isChild()) { this.tasks.addTask(8, new EntityAIPlay(this, 0.32D)); } else if (this.getProfessionForge() == PROFESSION_FARMER || this.getProfession() == 0) { this.tasks.addTask(6, new EntityAIHarvestFarmland(this, 0.6D)); } else if (this.getProfession() == 4 || this.getProfessionForge() == PROFESSION_BUTCHER) { this.tasks.addTask(6, new VillagerAIHarvestMeat(this)); } if (this.getHired()) { this.tasks.addTask(6, new VillagerFollowOwner(this, 0.9D, 6.0F, 1.5F)); } } } -
[1.12] Butcher Harvesting Meat Doesn't do Anything
OrangeVillager61 replied to OrangeVillager61's topic in Modder Support
Alright, I added printlns throughout my code and fixed many issues but I'm reaching a major issue where shouldExecute doesn't start at all according to my printlns. public boolean shouldExecute() { System.out.println("Should Execute started."); //Doesn't fire if (this.villagerObj.getGrowingAge() != 0) { System.out.println("Isn't adult"); return false; } else if (this.villagerObj.wantsMoreFood() == false) { System.out.println("Doesn't want food"); return false; } else if (this.villagerObj.getProfession() != 4) { System.out.println("Is not a butcher"); return false; } else { EntityAnimal entity = (EntityAnimal) this.world.findNearestEntityWithinAABB(EntityAnimal.class, this.villagerObj.getEntityBoundingBox().expand(100.0D, 7.0D, 100.0D), this.villagerObj); if (entity == null) { System.out.println("Entity is null"); return false; } if (this.villagerObj.world.getWorldTime() - entity.getCapability(HarvestTimeProvider.CAPABILITY_HARVEST_ANIMAL_TIME, null).get_time() < 24000 && entity.getCapability(HarvestTimeProvider.CAPABILITY_HARVEST_ANIMAL_TIME, null).get_time() != 0) { System.out.println("Entity has been harvested"); return false; } if (entity.getGrowingAge() < 0) { System.out.println("Entity is not adult"); return false; } if (entity instanceof EntityCow) { this.animal_type = "Cow"; return true; } else if (entity instanceof EntityPig) { this.animal_type = "Pig"; return true; } else if (entity instanceof EntitySheep) { this.animal_type = "Sheep"; return true; } else if (entity instanceof EntityChicken) { this.animal_type = "Chicken"; return true; } else { System.out.println("Entity is not harvestable"); return false; } } } -
I seem to be having an issue with the capability. I can't find said issue though. public class HarvestTimeProvider implements ICapabilitySerializable<NBTBase>{ @CapabilityInject(IHarvestTime.class) public static final Capability<IHarvestTime> CAPABILITY_HARVEST_ANIMAL_TIME = null; private IHarvestTime instance = CAPABILITY_HARVEST_ANIMAL_TIME.getDefaultInstance(); @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing) { return capability == CAPABILITY_HARVEST_ANIMAL_TIME; } @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { return capability == CAPABILITY_HARVEST_ANIMAL_TIME ? CAPABILITY_HARVEST_ANIMAL_TIME.<T> cast(this.instance) : null; } @Override public NBTBase serializeNBT() { return CAPABILITY_HARVEST_ANIMAL_TIME.getStorage().writeNBT(CAPABILITY_HARVEST_ANIMAL_TIME, this.instance, null); } @Override public void deserializeNBT(NBTBase nbt) { CAPABILITY_HARVEST_ANIMAL_TIME.getStorage().readNBT(CAPABILITY_HARVEST_ANIMAL_TIME, this.instance, null, nbt); } } public class HarvestTimeUser implements IHarvestTime{ private long time = 0; @Override public void setTime(long l) { this.time = l; } @Override public long get_time() { return time; } } public interface IHarvestTime { void setTime(long l); long get_time(); } public class IvCapabilities { public static void regsiterCapabilties() { CapabilityManager.INSTANCE.register(IHarvestTime.class, new IHarvestTimeStorage(), HarvestTimeUser.class); } public static class IHarvestTimeStorage implements IStorage<IHarvestTime> { @Override public NBTBase writeNBT(Capability<IHarvestTime> capability, IHarvestTime instance, EnumFacing side) { return new NBTTagLong (instance.get_time()); } @Override public void readNBT(Capability<IHarvestTime> capability, IHarvestTime instance, EnumFacing side, NBTBase nbt) { instance.setTime(((NBTPrimitive) nbt).getLong()); } } } public class AttachCapabilties { public static final ResourceLocation HARVEST_TIME_CAP = new ResourceLocation(Reference.MOD_ID, "Harvest_Time"); @SubscribeEvent public void AttachCapToEntity (AttachCapabilitiesEvent<Entity> event) { if (event.getObject() instanceof EntityAnimal) { event.addCapability(HARVEST_TIME_CAP, new HarvestTimeProvider()); } } } When I'm using it: if (this.villagerObj.world.getWorldTime() - entity.getCapability(HarvestTimeProvider.CAPABILITY_HARVEST_ANIMAL_TIME, null).get_time() < 24000 || entity.getCapability(HarvestTimeProvider.CAPABILITY_HARVEST_ANIMAL_TIME, null).get_time() == 0) { System.out.println("Entity has been harvested"); return false; }
-
[1.12] Butcher Harvesting Meat Doesn't do Anything
OrangeVillager61 posted a topic in Modder Support
Hello! I am trying to get a butcher to go to mobs and "harvest" a meat out of them. More specifically, the butcher goes up to the mob, gets one meat, type depending on the mob and then goes to another after 10 secs. However, my butcher does nothing and the first println doesn't go off. public class VillagerAIHarvestMeat extends EntityAIBase { public final double VilPerDoor = Config.VilPerDoor; private final IvVillager villagerObj; private EntityAnimal animal; int mateAgain; protected Random rand = new Random(); private World world; String animal_type; int delayCounter; public VillagerAIHarvestMeat(IvVillager villagerIn) { this.villagerObj = villagerIn; this.world = villagerIn.world; this.setMutexBits(3); } /** * Returns whether the EntityAIBase should begin execution. */ public boolean shouldExecute() { if (this.villagerObj.getWorkTicks() > 0) { return false; } if (this.villagerObj.getGrowingAge() != 0) { return false; } if (this.villagerObj.wantsMoreFood() == false) { return false; } if (this.villagerObj.getProfession() != 4) { return false; } else { EntityAnimal entity = (EntityAnimal) this.world.findNearestEntityWithinAABB(EntityAnimal.class, this.villagerObj.getEntityBoundingBox().expand(100.0D, 7.0D, 100.0D), this.villagerObj); if (entity == null) { return false; } if (this.villagerObj.world.getWorldTime() - entity.getCapability(HarvestTimeProvider.CAPABILITY_HARVEST_ANIMAL_TIME, null).get_time() < 24000 || entity.getCapability(HarvestTimeProvider.CAPABILITY_HARVEST_ANIMAL_TIME, null).get_time() == 0) { return false; } if (entity.getGrowingAge() < 0) { return false; } if (entity instanceof EntityCow) { this.animal_type = "Cow"; return true; } else if (entity instanceof EntityPig) { this.animal_type = "Pig"; return true; } else if (entity instanceof EntitySheep) { this.animal_type = "Sheep"; return true; } else if (entity instanceof EntityChicken) { this.animal_type = "Chicken"; return true; } else { return false; } } } /** * Execute a one shot task or start executing a continuous task */ public void startExecuting() { System.out.println("Butcher task approved."); this.delayCounter = 0; } /** * Returns whether an in-progress EntityAIBase should continue executing */ public boolean continueExecuting() { if (this.animal.getGrowingAge() < 0) { return false; } else if (!this.animal.isEntityAlive()) { return false; } else { System.out.println("Butcher task allowed to continue."); return true; } } /** * Resets the task */ public void resetTask() { this.animal = null; } /** * Updates the task */ public void updateTask() { System.out.println("Butcher to animal."); if (this.villagerObj.getDistanceSqToEntity(this.animal) > 2.25D) { if (--this.delayCounter <= 0) { this.delayCounter = 10; this.villagerObj.getNavigator().tryMoveToEntityLiving(this.animal, 0.53D); } } else { this.getMeat(); } } private void getMeat() { if (this.animal_type == "Cow") { this.animal.dropItem(Items.COOKED_BEEF, 1); } else if (this.animal_type == "Pig") { this.animal.dropItem(Items.COOKED_PORKCHOP, 1); } else if (this.animal_type == "Sheep") { this.animal.dropItem(Items.COOKED_MUTTON, 1); } else if (this.animal_type == "Chicken") { this.animal.dropItem(Items.COOKED_CHICKEN, 1); } System.out.println("Butcher got the meat."); this.villagerObj.setWorkTicks(200); this.animal.getCapability(HarvestTimeProvider.CAPABILITY_HARVEST_ANIMAL_TIME, null).setTime(this.animal.world.getWorldTime()); } } public class HarvestTimeUser implements IHarvestTime{ private long time = 0; @Override public void setTime(long l) { this.time = l; } @Override public long get_time() { return time; } } -
Hello! I am getting butchers to harvest meat from animals and I need said animals to be unharvestable to villagers for 24000 ticks. My issue is that I want the butchers to harvest from other mobs in that time so I can't just disallow the butchers from harvesting mobs for that period. How do I this without overriding said mob and creating a new entity since I want compatibility with other mods?
-
Hello! I am allowing butchers to harvest meat from animals for compatibility and to reduce lag instead of straight up killing them. My issue is that MC doesn't want me to get animals out of an EntityAnimal bounding box. public boolean shouldExecute() { if (this.villagerObj.getGrowingAge() != 0) { return false; } else { EntityAnimal entity = (EntityAnimal) this.world.findNearestEntityWithinAABB(EntityAnimal.class, this.villagerObj.getEntityBoundingBox().expand(8.0D, 3.0D, 8.0D), this.villagerObj); if (entity == null) { return false; } if (entity.getGrowingAge() < 0) { return false; } if (entity == EntityCow) { //Doesn't work } }
-
[1.12] Porting from 1.11.2 registry assistance needed
OrangeVillager61 replied to OrangeVillager61's topic in Modder Support
Alright, that fixed the issue. Thanks for the help! -
[1.12] Porting from 1.11.2 registry assistance needed
OrangeVillager61 replied to OrangeVillager61's topic in Modder Support
That's just how I posted it, not my actual files. -
[1.12] Porting from 1.11.2 registry assistance needed
OrangeVillager61 replied to OrangeVillager61's topic in Modder Support
public class ClientProxy extends CommonProxy{ @Override public void preInit(FMLPreInitializationEvent e) { super.preInit(e); MinecraftForge.EVENT_BUS.register(new RegisterModels()); } @Override public void init(FMLInitializationEvent e) { super.init(e); } @Override public void postInit(FMLPostInitializationEvent e) { super.postInit(e); } } public class RegisterModels { @SubscribeEvent public static void registerModels(ModelRegistryEvent event) { IvBlocks.initModels(); } } I fear that the issue is with my client proxy. -
[1.12] Porting from 1.11.2 registry assistance needed
OrangeVillager61 replied to OrangeVillager61's topic in Modder Support
Fixing the mod_id fixed most of it but the block doesn't have a texture in the inventory. @Mod.EventBusSubscriber public final class IvBlocks { @GameRegistry.ObjectHolder("cs:light_blue_stairs") public static final LightBlueStairs light_blue_stairs = null; @SubscribeEvent public static void registerBlocks(RegistryEvent.Register<Block> event) { event.getRegistry().register(new LightBlueStairs()); } @SubscribeEvent public static void registerItems(RegistryEvent.Register<Item> event) { event.getRegistry().register(new ItemBlock(light_blue_stairs).setRegistryName(light_blue_stairs.getRegistryName())); } @SideOnly(Side.CLIENT) public static void initModels() { light_blue_stairs.initModel(); } } public class LightBlueStairs extends BlockStairs{ public LightBlueStairs() { super(Blocks.STAINED_HARDENED_CLAY.getStateFromMeta(3)); this.setCreativeTab(CreativeTabs.MATERIALS); setRegistryName("light_blue_stairs"); setUnlocalizedName(Reference.MOD_ID + ":" + "light_blue_stairs"); } @SideOnly(Side.CLIENT) public void initModel() { ModelLoader.setCustomModelResourceLocation(Item.getItemFromBlock(this), 0, new ModelResourceLocation(getRegistryName(), "inventory")); } } -
[1.12] Porting from 1.11.2 registry assistance needed
OrangeVillager61 replied to OrangeVillager61's topic in Modder Support
public class LightBlueStairs extends BlockStairs{ public LightBlueStairs() { super(Blocks.STAINED_HARDENED_CLAY.getStateFromMeta(3)); this.setCreativeTab(CreativeTabs.MATERIALS); setRegistryName(Reference.MOD_ID + ":" + "light_blue_stairs"); setUnlocalizedName(Reference.MOD_ID + ":" + "light_blue_stairs"); } @SideOnly(Side.CLIENT) public void initModel() { ModelLoader.setCustomModelResourceLocation(Item.getItemFromBlock(this), 0, new ModelResourceLocation(getRegistryName(), "inventory")); } } Okay, I'll try that. Oh, nvm. I've been using the wrong mod_id.