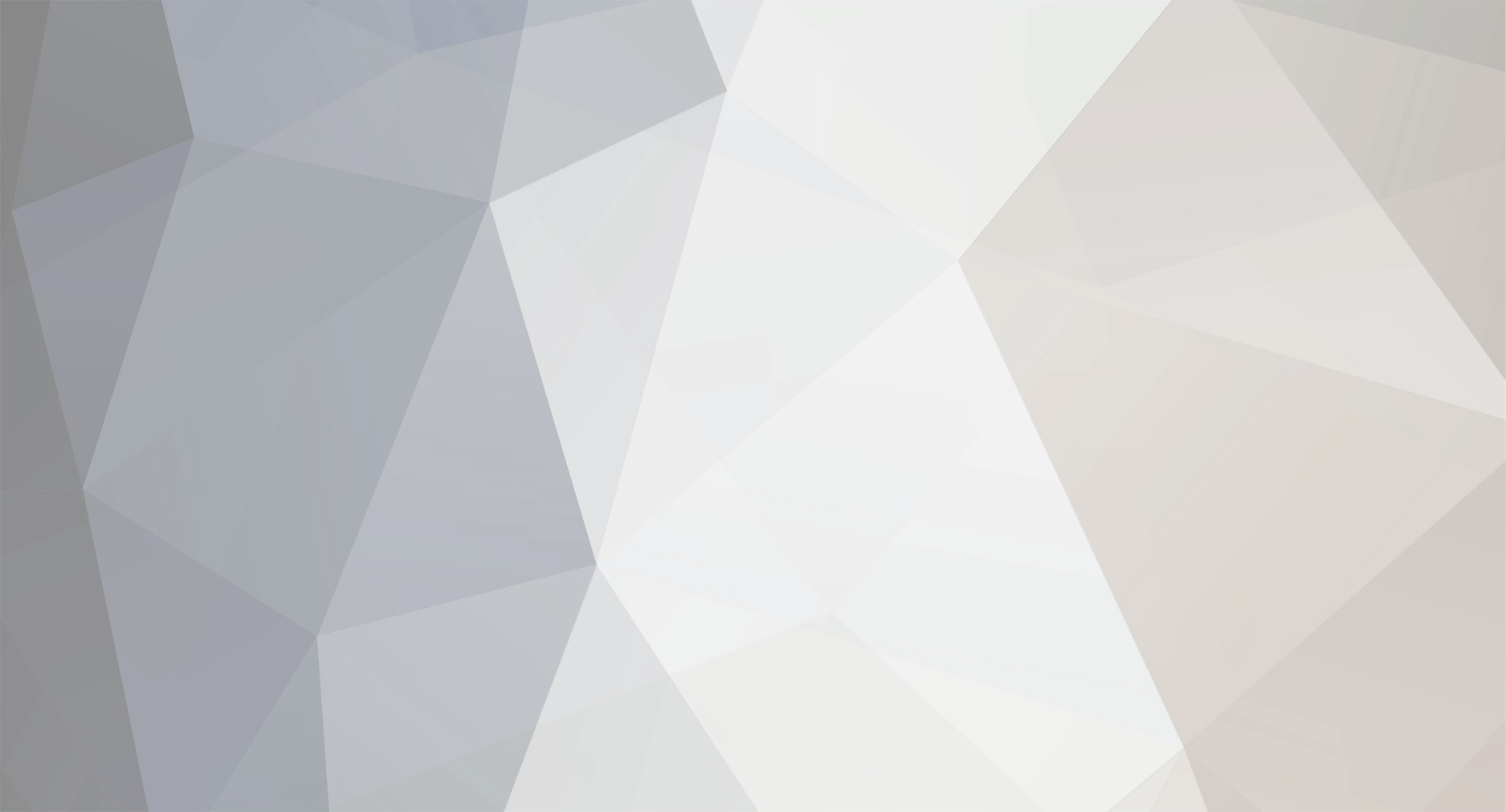
lynchiem
Members-
Posts
10 -
Joined
-
Last visited
Everything posted by lynchiem
-
Ew. There is no reason to combine them. Thank you. As I have mentioned, I am very new to modding, and was simply sharing thoughts based on the difference I could identify between my working code and the problematic code. Combined is probably an overstatement, given the GUI related functions are the only ones I am currently handling over riding in the "proxy". I am still struggling to get my head around how the proxy system works. I need to explicitly tell the mod which class to use as the client and server proxies. @SidedProxy(clientSide = "net.clockworkrocketry.clockworkadventure.network.ClientProxy", serverSide = "net.clockworkrocketry.clockworkadventure.network.CommonProxy") If I get rid of this, my GUI stops working. And my proxy is nothing more than in implementation of IGuiHandler at this stage, containing pretty much only the getClientGuiElement and getServerGuiElement functions. So I thought it was worth exploring as a possible breakage point, as I thought maybe the getClientGuiElement and getServerGuiElement functions needed to be "wired" up to the server/client workflow. But obviously this is incorrect.
-
Sorry, I haven't had a huge amount of time today, but had a quick look over now and my first thoughts are: Your proxy and guihandler are separate. When I implemented mine, based on examples I had seen, my proxy is also the guihandler. I am not experienced enough with modding to know if this would cause a problem, but figured I would mention it, because perhaps there is a conflict happening between server code and client code. If you look at my code (linked in a post above) you will note that my CommonProxy, used by the server, returns null for the getClientGuiElement, but my ClientProxy overrides that behaviour. I will have more time tomorrow after work, so if you haven't resolved by then, I will take a more thorough look at your code.
-
If you are still having trouble and can post your updated code (is it in github or similar where it can easily be browsed by chance?), I would be happy to help you debug.
-
Hello DuckCreeper, I am fairly new to modding, so feel free to beheckle me if my observation is born of ignorance, but I noticed in your GUI handler, you have overridden the drawGuiContainerBackgroundLayer, but you are not doing anything in the body. Which, if I understand things correctly, would mean that the UI would be effectively invisible. If it is any help at all, I have recently been learning in this area too, and my source repository has code that does not much more than enable a block inventory / gui... so it may be of some help as cross-reference in resolving your issues. (Though, as stated, I am very new, so there maybe many glaring errors and bad practices in play in my code.) https://github.com/lynchiem/ClockworkAdventure/blob/master/src/main/java/net/clockworkrocketry/clockworkadventure/gui/GUIPortableChest.java I also noticed that when you register your guiHandler, you are passing in "this" where all the examples I have seen pass in "instance"... though I am not sure this will make any difference, as assuming the instantiation is done without voodoo they are probably equivalent. Sorry I could not be of more help, and best of luck resolving your issue.
-
[1.8.9] [Solved] Correct way to store non-item/non-entity data?
lynchiem replied to lynchiem's topic in Modder Support
Thank you very much for the response and the example -
I am currently writing my first mod, and having a blast doing so, but I have a question I am hoping one of you kind folk might be able to help me out with. I am quite familiar with NBT and saving data against items, but I am reaching a point where I need to start saving data about the world itself, not relating to any given item or entity. Now I could obviously just use standard I/O libraries to serialize the data to disk (and read on load), but I am assuming there are cleaner more standard ways to handle data within the Minecraft/Forge framework. As an example of the type of data I would like to store: I generate dungeons, and would like to maintain a list of known dungeon coordinates so that I can tightly control the distribution and progression of the dungeons. I also plan to introduce boss monsters, and would like to store a "last killed" time to prevent over farming. I would also like to introduce various world "achievements" where if anyone on a server completes a certain task, it unlocks new adventures for everyone on the server. So I need to store which tasks have been completed. Hope this makes sense. I don't need specifics on how to pull of each of the listed items, just a general guideline on how best to store world-level data.
-
[1.8.9] Inventory Persistence after Breaking Block [Solved]
lynchiem replied to lynchiem's topic in Modder Support
Thank you I presumed it would be something like this, though I hadn't yet thought of a way to tackle it. Handy to know, though in this case I do have some data that is required for rendering as I have a orientation based texture. -
[1.8.9] Inventory Persistence after Breaking Block [Solved]
lynchiem replied to lynchiem's topic in Modder Support
Thank you again coolAlias, your advice has put me well and truly on the path to a solution. I am still having a few quirks (it seems temperamental moving to a new location), but I am fairly certain this will have something to do with copying more NBT than I need to, or maybe just a weird synchronicity bug... ...but it is definitely a massive step forward, and I am confident I can nut out the rest. I have provided the solution so far below for the future curious (sans the bulk of some safety and sanity checks for brevity). I did have some difficulty accessing the TileEntity from the dropBlockAsItemWithChance function, which I will continue to investigate, but I was able to take your advice more broadly to arrive at this solution (after learning a little about EntityItems). Firstly, I disabled the default drop behaviour: @Override public void dropBlockAsItemWithChance(World worldIn, BlockPos pos, IBlockState blockState, float chance, int fortune) { // Disable super to prevent standard drop. // super.dropBlockAsItemWithChance(worldIn, pos, blockState, chance, fortune); } Then I captured the NBT data as suggested, but in the breakBlock function, which seems to have cleaner access to the TileEntity. @Override public void breakBlock(World world, BlockPos pos, IBlockState blockState) { NBTTagCompound tag = new NBTTagCompound(); TileEntity blockTileEntity = world.getTileEntity(pos); blockTileEntity.writeToNBT(tag); ItemStack blockItem = new ItemStack(Item.getItemFromBlock(this), 1, this.damageDropped(blockState)); EntityItem entityitem = new EntityItem(world, pos.getX(), pos.getY(), pos.getZ(), blockItem); entityitem.getEntityItem().setTagCompound(tag); world.spawnEntityInWorld(entityitem); super.breakBlock(world, pos, blockState); } And lastly I write the NBT back to the chest as I place it (this is where the quirks currently reside - but quirks aside it works). @Override public void onBlockPlacedBy(World world, BlockPos pos, IBlockState blockState, EntityLivingBase entityliving, ItemStack itemStack) { TileEntity blockTileEntity = world.getTileEntity(pos); if (blockTileEntity != null && blockTileEntity instanceof TileEntityPortableChest) { TileEntityPortableChest chestTileEntity = (TileEntityPortableChest)blockTileEntity; chestTileEntity.wasPlaced(entityliving, itemStack); if(itemStack.hasTagCompound()) { NBTTagCompound tag = itemStack.getTagCompound(); chestTileEntity.readFromNBT(tag); } world.markBlockForUpdate(pos); } } -
[1.8.9] Inventory Persistence after Breaking Block [Solved]
lynchiem replied to lynchiem's topic in Modder Support
Thank you very much coolAlias. I will give it a shot. -
Hi All, I am an experience developer, but very new to mod development (enjoying it a lot though!), so please bear with me. Any help you can offer would be extremely appreciated. I am trying to build a portable chest, and item that you can place in the world and use like a chest, but that you can also "pick up" (return to player inventory) and move around without the chest losing its inventory. I have the item working as a chest perfectly. When I put items in the chest, they stay there. Even after quitting. (At least in single player, I haven't got around to server testing yet). In other words, I have figured out enough to know to override [readFromNBT] and [writeToNBT] on my [IInventory]. What I am struggling with is how to "pick up" the block and have the inventory retained. I have been able to figure out that if I override [onBlockPlacedBy] and [breakBlock] on my [Block], I have an opportunity access and play around with the [TileEntity] before it is added to/removed from world completely... ...but I am really struggling to figure out how to transfer inventory in to an item I can pick up (and that can persist NBT data). I am sure I will figure it out with enough persistence, but figured it would be a good question to jump in to these forums and engage with more experienced folk.