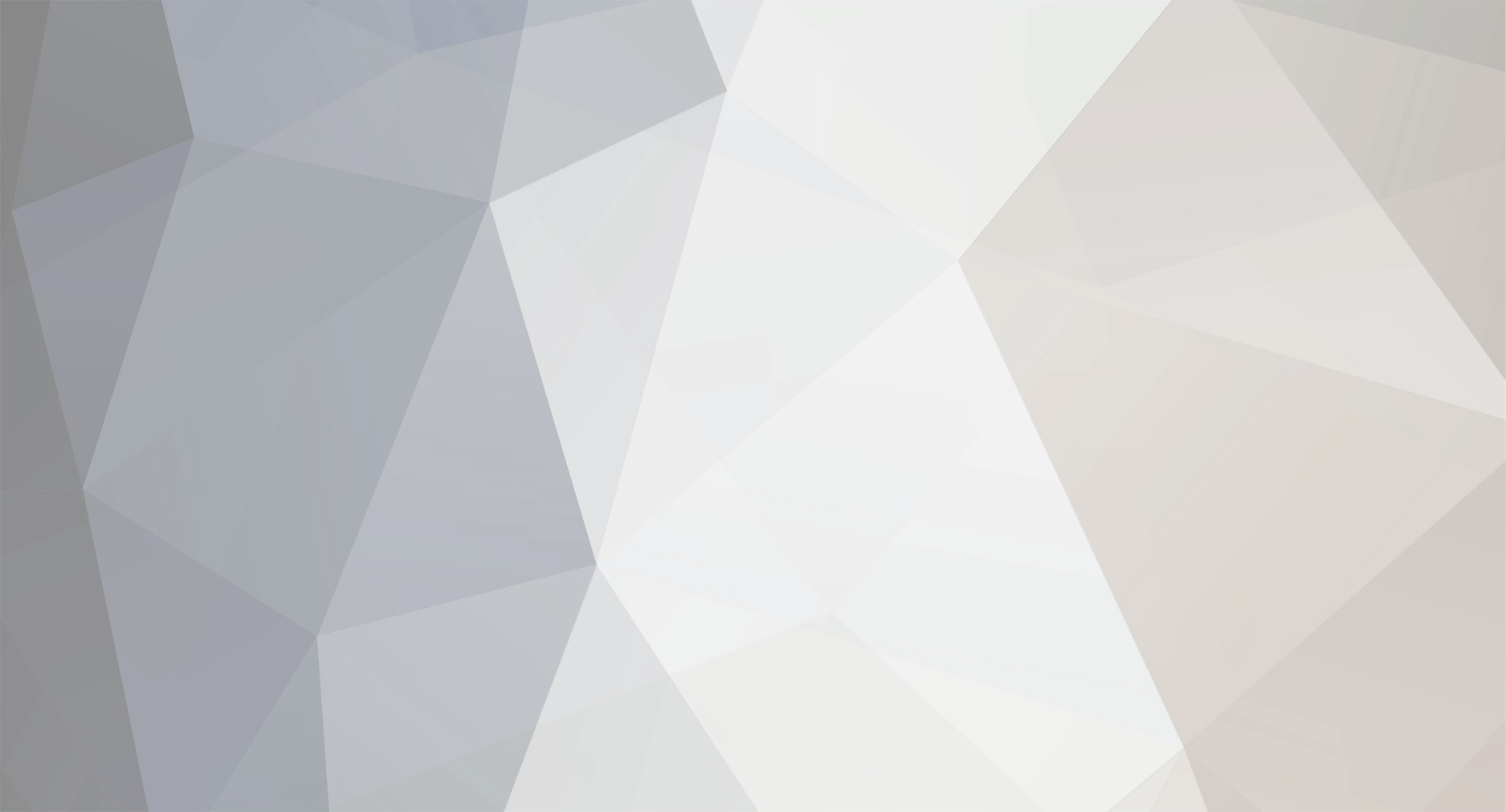
awger
Members-
Posts
10 -
Joined
-
Last visited
Everything posted by awger
-
@Override public void applyEntityCollision(Entity par1Entity) { if (par1Entity != riddenByEntity && par1Entity.canBePushed()) applyEntityCollision(par1Entity); }
-
Is it possible to render techne model with ISimpleBlockRenderingHandler?
awger replied to Naiten's topic in Modder Support
I've seen that error before, not sure what the problem was. Check your tessellator.startDrawing() and tessellator.draw() calls, they should match up like GL11.glPushMatrix() and GL11.glPopMatrix(). -
Proper way to read/write multi-part entity to/from NBT? [SOLVED]
awger replied to awger's topic in Modder Support
Had to do a very lazy late linkage in both directions. SPAWN ORDER IS NOT DEFINED. When restoring from NBT, objects are spawned in random order. parent will spawn before, in the middle of, or after all the children. getUniqueID() in parent extended constructor. UUID is passed in writeSpawnData and written to NBT children track parent by parent UUID (and of course, written to NBT) parent.getParts() has to check for all children loaded and linked, return null if some are missing chlid.onUpdate checks for linkage to parent, attempts to link, bails out if not linked Additional parent/child null checks, in places you wouldn't otherwise need them, will be required. Under normal circumstances (children spawned by parent) the parent will be known when the child instantiates, but not after an NBT load. Same for parent iterating through its child array -- until all children are relinked there will be uninitialized members. Note that external code doesn't null check array members before trying to access them. In order to avoid throw/crash you'll have to trick them into not running (getParts() returns null until all children are linked). -
Proper way to read/write multi-part entity to/from NBT? [SOLVED]
awger replied to awger's topic in Modder Support
You would think... but, no. The EnderDragon takes many shortcuts, not the least of which is the parent recreates its children in the constructor, and the children written to NBT are orphaned. Not a huge problem when you only have one dragon with half a dozen children, and the dragon only gets instantiated when the end loads, but it quickly becomes a problem when you're dealing with dozens of parents that have hundreds of children each. The more I look through the vanilla code, the more I'm convinced that the child entitiy handling was an afterthought. -
I'm trying to work through a chicken-and-egg problem, and I'm not sure what the "proper" method is supposed to be. I have a parent entity that instantiates multiple child entities. Parent has an array for keeping track of children (accessed via getParts) and each child has a pointer to the Parent. Everything works fine when a player builds the item (spawned on server, writeSpawnData sends all the necessary data over, and everything is instantiated and reconnected on the client). The problem occurs when the parent+children is written to NBT and then read back out. All the data is there but, because the children already exist and sometimes get spawned on the client before the parent, reconnection is all out of whack -- MC is trying to update and render the various bits before all the linkage is complete, and it keeps hitting null objects. I've added copious null checks (in the MC code as well) and rigged my children to do lazy linkage back to the parent, but I'm stuck on the parent's child array. It's an MC-defined interface (an array of known size), and it has to exist before the children start showing up, but in between the parent instantiation (and allocation of the array) and the registration of all the children, MC comes along and tries to start loading skins, hits a null, and throws. I tried killing all the children (chunk remove and setDead) when the parent is written to NBT, and then creating new children when the parent is read back out, but that didn't seem to get me much further (caused different problems, like occasional concurrent access throws). Is anyone else using multipart entities? How should the parent+child NBT writing/reading be handled?
-
** WORKAROUND / SOLUTION ** I updated to the most recent recommend release (6.4.1.401) and added a data watcher and a custom packet handler. The basic approach is: spawn parent entity on client side, parent spawns child entities client sends spawn packet to server server spawns parent entity and children server sets parent entityId to match client, screws up child entityId's child entities on server watch for change of entityId, update counter in parent when entityId changes when all children on server have updated entityId, server sets flag in data watcher that syncs to client when flag is set on client, client sends custom packet to update entityId's of children on server server receives packet, updates entityId's of children While testing I was seeing some weird things in my logs. First problem: None of the data watchers appear to be sync'ing with the other end. I don't know if they're supposed to be unidirectional or bidirectional, but they don't seem to be working in either respect. I need to do some more research on this but, for now, I have a work-around (ugly kludge, but it works). Second problem: When trying to update the client entityId's on the server side, I kept retrieving entity data from the client side. I got utterly confused, and started drinking scotch. Which seems to have done the trick. At a minimum it didn't hurt and, in any event, I didn't stumble upon the solution until after I started drinking. I'm using getEntityByID (with the entityId passed in the packet) to find the parent entity and retrieve the child entity list. For some reason unknown to me, within the confines of my PacketHandler, "WorldServer" returns entityId's from the client side, and "WorldClient" returns entityId's from the server side. Code for PacketHandler follows. Note usage of getEntityByID, which is easily switched between wc (WorldClient) and ws (WorldServer). Here's log from a run calling getEntityId from WorldServer: ...and here's the excerpt from calling getEntityId from WorldClient: A couple items to note: the server/client portion of the child entity name is set at instantiation, so there's no doubt that they're associated with one side or the other log messages are comingled from multiple threads, illustrating the base problem and underscoring the need for a "real" fix (although I'm not sure there is one, and this approach may be the best option) when SIDE == SERVER, isRemote is reversed from the client perspective -- ie, when you're on the server, "remote" is the client side WorldClient and WorldServer appear to be backwards, at least from the perspective of getEntityByID Verbosity for posterity, in the hopes that it may be useful for some other poor schmuck.
-
** UPDATE ** The problem appears to be slightly more complicated than I originally thought... seems that child entities aren't necessarily spawned starting with id's equal to parent.entityId+1. In testing I've seen several instances of the child entities starting at +2 or +3, suggesting that some other entity spawned after the parent but before the children. Assuming that another entity can be spawned at any time (ie in between a parent and its children, or in between children) the only complete solution is to pass the entityId's of all the children and sync them directly. Not sure about the best way to do this. I started looking into IEntityAdditionalSpawnData and it looks like it's only one-way (server to client?). This presents a conundrum, as I was using the vanilla boat code that spawns client-side, and it doesn't work properly when spawned server-side (no instantiation on the client side). Looks like I'll be using a custom packet to set the child id's... unless someone has a better suggestion?
-
In FMLClientHandler.java, function spawnEntityIntoClientWorld, the entityId of the instantiated entity is set to the entityId passed in the packet. Further down, the new entityId (not the original) is used to calculate the offset for the child entities, resulting in incorrect entityId's that don't match those on the other end. My quick fix was to calculate the offset before altering the entityId of the parent: int ofs = 0; ... ofs = packet.entityId - entity.entityId; ... parts[j].entityId += ofs;