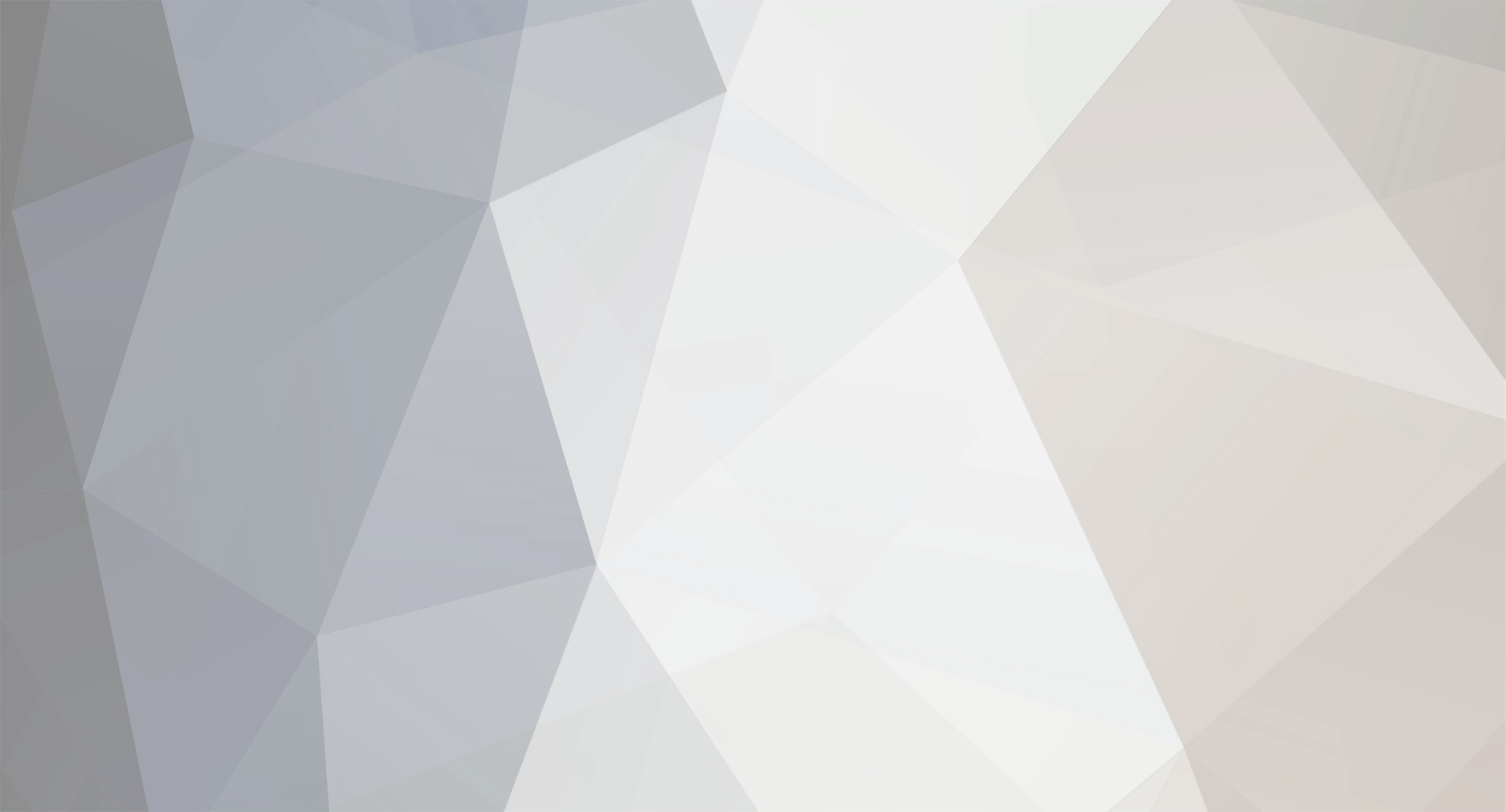
T-10a
Members-
Posts
59 -
Joined
-
Last visited
Everything posted by T-10a
-
Okay, got something basic set up. It's returning a NullPointerException on first tool that it loads. Here's the init class: [spoiler=ModItems.class] package com.t10a.minedran.init; import com.t10a.minedran.item.material.ItemTutorial; import com.t10a.minedran.item.tools.*; import com.t10a.minedran.item.weapons.ItemCustomSword; import com.t10a.minedran.item.weapons.ItemMace; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.block.model.ModelResourceLocation; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraftforge.common.util.EnumHelper; import net.minecraftforge.fml.common.registry.GameRegistry; import net.minecraftforge.oredict.OreDictionary; public class ModItems { public static Item mace_wood; public static Item mace_stone; public static Item mace_iron; public static Item mace_gold; public static Item mace_diamond; public static Item mace_tutorial; public static Item axe_tutorial; public static Item sword_tutorial; public static Item pickaxe_tutorial; public static Item shovel_tutorial; public static Item hoe_tutorial; public static Item item_tutorial; public static Item shears_gold; public static Item shears_diamond; public static Item shears_tutorial; public static Item.ToolMaterial TUTORIAL; public static void init() { /*<unlocalized name>=new Item<name>(); * NOTICE: Because of how Mojang coded axes, any future item that extends ItemAxe HAS to be registered as follows: *<unlocalized name>=new Item<name>(MATERIAL,DAMAGE_FLOAT,ATTACKSPEED_FLOAT); */ mace_wood=new ItemMace(Item.ToolMaterial.WOOD); mace_stone=new ItemMace(Item.ToolMaterial.STONE); mace_iron=new ItemMace(Item.ToolMaterial.IRON); mace_diamond=new ItemMace(Item.ToolMaterial.DIAMOND); mace_gold=new ItemMace(Item.ToolMaterial.GOLD); mace_tutorial=new ItemMace(TUTORIAL); axe_tutorial=new ItemCustomAxe(TUTORIAL, 9F, -3.0F); sword_tutorial=new ItemCustomSword(TUTORIAL); pickaxe_tutorial=new ItemCustomPickaxe(TUTORIAL); shovel_tutorial=new ItemCustomShovel(TUTORIAL); hoe_tutorial=new ItemCustomHoe(TUTORIAL); item_tutorial=new ItemTutorial(); shears_gold=new ItemCustomShears(Item.ToolMaterial.GOLD); shears_diamond=new ItemCustomShears(Item.ToolMaterial.DIAMOND); shears_tutorial=new ItemCustomShears(TUTORIAL); Item.ToolMaterial TUTORIAL = EnumHelper.addToolMaterial("TUTORIAL", 3, 1000, 15.0F, 4.0F, 30); TUTORIAL.setRepairItem(new ItemStack(ModItems.item_tutorial)); } public static void register() { //GameRegistry.register(<unlocalized name>); //NOTICE: THIS IS VERY LIKELY TO CHANGE ONCE I FIGURE OUT HOW TO REGISTER STUFF PROPERLY GameRegistry.register(mace_wood); GameRegistry.register(mace_stone); GameRegistry.register(mace_iron); GameRegistry.register(mace_diamond); GameRegistry.register(mace_gold); GameRegistry.register(mace_tutorial); GameRegistry.register(axe_tutorial); GameRegistry.register(sword_tutorial); GameRegistry.register(pickaxe_tutorial); GameRegistry.register(shovel_tutorial); GameRegistry.register(hoe_tutorial); GameRegistry.register(item_tutorial); GameRegistry.register(shears_diamond); GameRegistry.register(shears_gold); GameRegistry.register(shears_tutorial); } public static void registerRenders() { //registerRender(<unlocalized name>); registerRender(mace_wood); registerRender(mace_stone); registerRender(mace_iron); registerRender(mace_diamond); registerRender(mace_gold); registerRender(mace_tutorial); registerRender(axe_tutorial); registerRender(sword_tutorial); registerRender(pickaxe_tutorial); registerRender(shovel_tutorial); registerRender(hoe_tutorial); registerRender(item_tutorial); registerRender(shears_diamond); registerRender(shears_gold); registerRender(shears_tutorial); } public static void registerOreDictionary() { OreDictionary.registerOre("tutorial", new ItemStack(ModItems.item_tutorial)); } private static void registerRender(Item item) { //Is likely to change. It'll be a pain in the ass to fix though. Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(item, 0, new ModelResourceLocation(item.getRegistryName(),"inventory")); } } And here's the item that it crashes on: [spoiler=ItemMace.class] package com.t10a.minedran.item.weapons; import com.google.common.collect.Sets; import com.t10a.minedran.item.MaterialEffects; import com.t10a.minedran.reference.Reference; import net.minecraft.block.Block; import net.minecraft.block.state.IBlockState; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Blocks; import net.minecraft.init.MobEffects; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.item.ItemTool; import net.minecraft.potion.PotionEffect; import net.minecraft.util.math.BlockPos; import net.minecraft.world.World; import java.util.List; import java.util.Set; public class ItemMace extends ItemTool { //Dummy set to get the tool class to shut up. Also, stops the crashing. I know, I could set a custom item like the sword. private static final Set<Block> MaceBlocks = Sets.newHashSet(new Block[] {Blocks.BEDROCK}); public final Item.ToolMaterial material; public ItemMace(ToolMaterial material) { super(1.0F, -2.8F, material, MaceBlocks); setMaxStackSize(1); this.material = material; this.efficiencyOnProperMaterial = 0.0F; setCreativeTab(CreativeTabs.COMBAT); //It's a good idea to put the mod id in front of the unlocalised name. Otherwise, naming conflicts may arise. setUnlocalizedName(Reference.MOD_ID + "." + Reference.ItemBase.MACE.getUnlocalizedName() + "_" + getToolMaterialName().toLowerCase()); setRegistryName(Reference.ItemBase.MACE.getRegistryName() + "_" + getToolMaterialName().toLowerCase()); } @Override public void addInformation(ItemStack stack, EntityPlayer player, List<String> list, boolean par4) { list.add("Hit enemies for a chance to inflict Slowness!"); } @Override public boolean hitEntity(ItemStack stack, EntityLivingBase target, EntityLivingBase attacker) { MaterialEffects.effectsOnAttack(material, target, attacker); int pow=(int)(Math.random()*4+1); if (pow == 1) target.addPotionEffect(new PotionEffect(MobEffects.SLOWNESS, 80)); stack.damageItem(1, attacker); return super.hitEntity(stack, target, attacker); } @Override public boolean onBlockDestroyed(ItemStack stack, World worldIn, IBlockState state, BlockPos pos, EntityLivingBase entityLiving) { if ((double)state.getBlockHardness(worldIn, pos) != 0.0D) { stack.damageItem(2, entityLiving); } return true; } }
-
Hello, After creating a custom item, as well as a custom tool material & custom tools, I realised I can't repair them, as they have no defined repair material. How would I set the repair material? I have the tools to be repaired, and the item I would like to set as the repair material already there.
-
Good. Thanks for the answers everyone!
-
Okay, so a good way is to register unlocalised names is as "item.modid.item.name"?
-
Ah, so I don't need a short word in front of my item name in the unlocalised name, to differentiate between similar items from different mods?
-
Hello, I'm just wondering what'll happen if 2 mods somehow register items with the same registry name & unlocalised name. As in, say Mod A registers an item with the registry name "cheese" and unlocalised name "item.cheese.name", and Mod B registers an item with the same registry and unlocalised name. What'll happen then? I'm just asking so I can prevent any potential conflicts.
-
Yup, just had to change the classes to remove the abstract. It loads up fine now \o/
-
Ok, here they are: [spoiler=Client Proxy] package com.t10a.minedran.proxy; public abstract class ClientProxy implements ICommonProxy { @Override public void init() { } } [spoiler=Server Proxy] package com.t10a.minedran.proxy; public abstract class ServerProxy implements ICommonProxy { @Override public void init() { } } And my ICommonProxy [spoiler=ICommonProxy] package com.t10a.minedran.proxy; public interface ICommonProxy { public void init(); }
-
they exist, here's a snippet of my IDEA workspace:
-
Hello, After trying to rewrite my mod because of render issues from my updating attempt, I ended up with an error regarding the proxies: (I know, it's saying my Proxies aren't there. However, they do exist). [spoiler=Log] /usr/lib/jvm/java-8-openjdk/bin/java -Didea.launcher.port=7532 -Didea.launcher.bin.path=/usr/share/intellijidea-ce/bin -Dfile.encoding=UTF-8 -classpath /usr/lib/jvm/java-8-openjdk/jre/lib/charsets.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/cldrdata.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/dnsns.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/jaccess.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/localedata.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/nashorn.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/sunec.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/sunjce_provider.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/sunpkcs11.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/zipfs.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/jce.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/jsse.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/management-agent.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/resources.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/rt.jar:/drv/Data/Content/Minecraft/Mods/1.10.2/Minedran/out/production/Minedran_main:/home/thomas/.gradle/caches/minecraft/deobfedDeps/compileDummy.jar:/home/thomas/.gradle/caches/minecraft/deobfedDeps/providedDummy.jar:/home/thomas/.gradle/caches/minecraft/net/minecraftforge/forge/1.10.2-12.18.1.2011/snapshot/20160518/forgeSrc-1.10.2-12.18.1.2011.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.google.code.findbugs/jsr305/3.0.1/f7be08ec23c21485b9b5a1cf1654c2ec8c58168d/jsr305-3.0.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.mojang/netty/1.6/4b75825a06139752bd800d9e29c5fd55b8b1b1e4/netty-1.6.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/oshi-project/oshi-core/1.1/9ddf7b048a8d701be231c0f4f95fd986198fd2d8/oshi-core-1.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.java.dev.jna/jna/3.4.0/803ff252fedbd395baffd43b37341dc4a150a554/jna-3.4.0.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.java.dev.jna/platform/3.4.0/e3f70017be8100d3d6923f50b3d2ee17714e9c13/platform-3.4.0.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.ibm.icu/icu4j-core-mojang/51.2/63d216a9311cca6be337c1e458e587f99d382b84/icu4j-core-mojang-51.2.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.sf.jopt-simple/jopt-simple/4.6/306816fb57cf94f108a43c95731b08934dcae15c/jopt-simple-4.6.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/io.netty/netty-all/4.0.23.Final/294104aaf1781d6a56a07d561e792c5d0c95f45/netty-all-4.0.23.Final.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.google.guava/guava/17.0/9c6ef172e8de35fd8d4d8783e4821e57cdef7445/guava-17.0.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.apache.commons/commons-lang3/3.3.2/90a3822c38ec8c996e84c16a3477ef632cbc87a3/commons-lang3-3.3.2.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/commons-io/commons-io/2.4/b1b6ea3b7e4aa4f492509a4952029cd8e48019ad/commons-io-2.4.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/commons-codec/commons-codec/1.9/9ce04e34240f674bc72680f8b843b1457383161a/commons-codec-1.9.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.java.jutils/jutils/1.0.0/e12fe1fda814bd348c1579329c86943d2cd3c6a6/jutils-1.0.0.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.google.code.gson/gson/2.2.4/a60a5e993c98c864010053cb901b7eab25306568/gson-2.2.4.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.mojang/authlib/1.5.22/afaa8f6df976fcb5520e76ef1d5798c9e6b5c0b2/authlib-1.5.22.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.mojang/realms/1.9.3/b291425bf7ef763452eaa894575018706339f72b/realms-1.9.3.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.apache.commons/commons-compress/1.8.1/a698750c16740fd5b3871425f4cb3bbaa87f529d/commons-compress-1.8.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.apache.httpcomponents/httpclient/4.3.3/18f4247ff4572a074444572cee34647c43e7c9c7/httpclient-4.3.3.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/commons-logging/commons-logging/1.1.3/f6f66e966c70a83ffbdb6f17a0919eaf7c8aca7f/commons-logging-1.1.3.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.apache.httpcomponents/httpcore/4.3.2/31fbbff1ddbf98f3aa7377c94d33b0447c646b6e/httpcore-4.3.2.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/it.unimi.dsi/fastutil/7.0.12_mojang/ba787e741efdc425fc5d2ea654b57c15fba27efa/fastutil-7.0.12_mojang.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.apache.logging.log4j/log4j-api/2.0-beta9/1dd66e68cccd907880229f9e2de1314bd13ff785/log4j-api-2.0-beta9.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.apache.logging.log4j/log4j-core/2.0-beta9/678861ba1b2e1fccb594bb0ca03114bb05da9695/log4j-core-2.0-beta9.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.minecraft/launchwrapper/1.12/111e7bea9c968cdb3d06ef4632bf7ff0824d0f36/launchwrapper-1.12.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/jline/jline/2.13/2d9530d0a25daffaffda7c35037b046b627bb171/jline-2.13.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.ow2.asm/asm-debug-all/5.0.3/f9e364ae2a66ce2a543012a4668856e84e5dab74/asm-debug-all-5.0.3.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.typesafe.akka/akka-actor_2.11/2.3.3/ed62e9fc709ca0f2ff1a3220daa8b70a2870078e/akka-actor_2.11-2.3.3.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.typesafe/config/1.2.1/f771f71fdae3df231bcd54d5ca2d57f0bf93f467/config-1.2.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang/scala-actors-migration_2.11/1.1.0/dfa8bc42b181d5b9f1a5dd147f8ae308b893eb6f/scala-actors-migration_2.11-1.1.0.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang/scala-compiler/2.11.1/56ea2e6c025e0821f28d73ca271218b8dd04926a/scala-compiler-2.11.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang.plugins/scala-continuations-library_2.11/1.0.2/e517c53a7e9acd6b1668c5a35eccbaa3bab9aac/scala-continuations-library_2.11-1.0.2.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang.plugins/scala-continuations-plugin_2.11.1/1.0.2/f361a3283452c57fa30c1ee69448995de23c60f7/scala-continuations-plugin_2.11.1-1.0.2.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang/scala-library/2.11.1/e11da23da3eabab9f4777b9220e60d44c1aab6a/scala-library-2.11.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang.modules/scala-parser-combinators_2.11/1.0.1/f05d7345bf5a58924f2837c6c1f4d73a938e1ff0/scala-parser-combinators_2.11-1.0.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang/scala-reflect/2.11.1/6580347e61cc7f8e802941e7fde40fa83b8badeb/scala-reflect-2.11.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang.modules/scala-swing_2.11/1.0.1/b1cdd92bd47b1e1837139c1c53020e86bb9112ae/scala-swing_2.11-1.0.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang.modules/scala-xml_2.11/1.0.2/820fbca7e524b530fdadc594c39d49a21ea0337e/scala-xml_2.11-1.0.2.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/lzma/lzma/0.0.1/521616dc7487b42bef0e803bd2fa3faf668101d7/lzma-0.0.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.sf.trove4j/trove4j/3.0.3/42ccaf4761f0dfdfa805c9e340d99a755907e2dd/trove4j-3.0.3.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.paulscode/codecjorbis/20101023/c73b5636faf089d9f00e8732a829577de25237ee/codecjorbis-20101023.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.paulscode/codecwav/20101023/12f031cfe88fef5c1dd36c563c0a3a69bd7261da/codecwav-20101023.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.paulscode/libraryjavasound/20101123/5c5e304366f75f9eaa2e8cca546a1fb6109348b3/libraryjavasound-20101123.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.paulscode/librarylwjglopenal/20100824/73e80d0794c39665aec3f62eee88ca91676674ef/librarylwjglopenal-20100824.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.paulscode/soundsystem/20120107/419c05fe9be71f792b2d76cfc9b67f1ed0fec7f6/soundsystem-20120107.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.java.jinput/jinput/2.0.5/39c7796b469a600f72380316f6b1f11db6c2c7c4/jinput-2.0.5.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.lwjgl.lwjgl/lwjgl/2.9.4-nightly-20150209/697517568c68e78ae0b4544145af031c81082dfe/lwjgl-2.9.4-nightly-20150209.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.lwjgl.lwjgl/lwjgl_util/2.9.4-nightly-20150209/d51a7c040a721d13efdfbd34f8b257b2df882ad0/lwjgl_util-2.9.4-nightly-20150209.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/java3d/vecmath/1.5.2/79846ba34cbd89e2422d74d53752f993dcc2ccaf/vecmath-1.5.2.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.fusesource.jansi/jansi/1.11/655c643309c2f45a56a747fda70e3fadf57e9f11/jansi-1.11.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang/scala-actors/2.11.0/8ccfb6541de179bb1c4d45cf414acee069b7f78b/scala-actors-2.11.0.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.java.jinput/jinput-platform/2.0.5/7ff832a6eb9ab6a767f1ade2b548092d0fa64795/jinput-platform-2.0.5-natives-linux.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.java.jinput/jinput-platform/2.0.5/385ee093e01f587f30ee1c8a2ee7d408fd732e16/jinput-platform-2.0.5-natives-windows.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.java.jinput/jinput-platform/2.0.5/53f9c919f34d2ca9de8c51fc4e1e8282029a9232/jinput-platform-2.0.5-natives-osx.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.lwjgl.lwjgl/lwjgl-platform/2.9.4-nightly-20150209/b84d5102b9dbfabfeb5e43c7e2828d98a7fc80e0/lwjgl-platform-2.9.4-nightly-20150209-natives-windows.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.lwjgl.lwjgl/lwjgl-platform/2.9.4-nightly-20150209/931074f46c795d2f7b30ed6395df5715cfd7675b/lwjgl-platform-2.9.4-nightly-20150209-natives-linux.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.lwjgl.lwjgl/lwjgl-platform/2.9.4-nightly-20150209/bcab850f8f487c3f4c4dbabde778bb82bd1a40ed/lwjgl-platform-2.9.4-nightly-20150209-natives-osx.jar:/home/thomas/.gradle/caches/minecraft/net/minecraftforge/forge/1.10.2-12.18.1.2011/start:/usr/share/intellijidea-ce/lib/idea_rt.jar com.intellij.rt.execution.application.AppMain GradleStart [13:18:34] [main/INFO] [GradleStart]: Extra: [] [13:18:34] [main/INFO] [GradleStart]: Running with arguments: [--userProperties, {}, --assetsDir, /home/thomas/.gradle/caches/minecraft/assets, --assetIndex, 1.10, --accessToken{REDACTED}, --version, 1.10.2, --tweakClass, net.minecraftforge.fml.common.launcher.FMLTweaker, --tweakClass, net.minecraftforge.gradle.tweakers.CoremodTweaker] [13:18:34] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLTweaker [13:18:34] [main/INFO] [LaunchWrapper]: Using primary tweak class name net.minecraftforge.fml.common.launcher.FMLTweaker [13:18:34] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.CoremodTweaker [13:18:34] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLTweaker [13:18:34] [main/INFO] [FML]: Forge Mod Loader version 12.18.1.2011 for Minecraft 1.10.2 loading [13:18:34] [main/INFO] [FML]: Java is OpenJDK 64-Bit Server VM, version 1.8.0_102, running on Linux:amd64:4.6.4-1-ARCH, installed at /usr/lib/jvm/java-8-openjdk/jre [13:18:34] [main/INFO] [FML]: Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation [13:18:34] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.CoremodTweaker [13:18:34] [main/INFO] [GradleStart]: Injecting location in coremod net.minecraftforge.fml.relauncher.FMLCorePlugin [13:18:34] [main/INFO] [GradleStart]: Injecting location in coremod net.minecraftforge.classloading.FMLForgePlugin [13:18:34] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [13:18:34] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLDeobfTweaker [13:18:34] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.AccessTransformerTweaker [13:18:34] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [13:18:34] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [13:18:34] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper [13:18:34] [main/ERROR] [FML]: The binary patch set is missing. Either you are in a development environment, or things are not going to work! [13:18:35] [main/ERROR] [FML]: FML appears to be missing any signature data. This is not a good thing [13:18:35] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper [13:18:35] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLDeobfTweaker [13:18:35] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.AccessTransformerTweaker [13:18:35] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.TerminalTweaker [13:18:35] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.TerminalTweaker [13:18:35] [main/INFO] [LaunchWrapper]: Launching wrapped minecraft {net.minecraft.client.main.Main} [13:18:36] [Client thread/INFO]: Setting user: Player167 [13:18:38] [Client thread/INFO]: LWJGL Version: 2.9.4 ATTENTION: default value of option vblank_mode overridden by environment. [13:18:38] [Client thread/INFO] [sTDOUT]: [net.minecraftforge.fml.client.SplashProgress:start:221]: ---- Minecraft Crash Report ---- // I feel sad now Time: 6/08/16 1:18 PM Description: Loading screen debug info This is just a prompt for computer specs to be printed. THIS IS NOT A ERROR A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- System Details -- Details: Minecraft Version: 1.10.2 Operating System: Linux (amd64) version 4.6.4-1-ARCH Java Version: 1.8.0_102, Oracle Corporation Java VM Version: OpenJDK 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 103857496 bytes (99 MB) / 535298048 bytes (510 MB) up to 1845493760 bytes (1760 MB) JVM Flags: 0 total; IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: Loaded coremods (and transformers): GL info: ' Vendor: 'X.Org' Version: '3.0 Mesa 12.0.1' Renderer: 'Gallium 0.4 on AMD HAWAII (DRM 2.43.0 / 4.6.4-1-ARCH, LLVM 3.8.0)' [13:18:38] [Client thread/INFO] [FML]: MinecraftForge v12.18.1.2011 Initialized [13:18:38] [Client thread/INFO] [FML]: Replaced 233 ore recipes [13:18:38] [Client thread/INFO] [FML]: Found 0 mods from the command line. Injecting into mod discoverer [13:18:38] [Client thread/INFO] [FML]: Searching /drv/Data/Content/Minecraft/Mods/1.10.2/Minedran/run/mods for mods [13:18:40] [Client thread/INFO] [FML]: Forge Mod Loader has identified 4 mods to load [13:18:40] [Client thread/INFO] [FML]: Attempting connection with missing mods [mcp, FML, Forge, minedran] at CLIENT [13:18:40] [Client thread/INFO] [FML]: Attempting connection with missing mods [mcp, FML, Forge, minedran] at SERVER [13:18:40] [Client thread/ERROR] [FML]: An error occurred trying to load a proxy into {serverSide=com.t10a.minedran.proxy.ServerProxy, clientSide=com.t10a.minedran.proxy.ClientProxy}.com.t10a.minedran.Minedran java.lang.InstantiationException at sun.reflect.InstantiationExceptionConstructorAccessorImpl.newInstance(InstantiationExceptionConstructorAccessorImpl.java:48) ~[?:1.8.0_102] at java.lang.reflect.Constructor.newInstance(Constructor.java:423) ~[?:1.8.0_102] at java.lang.Class.newInstance(Class.java:442) ~[?:1.8.0_102] at net.minecraftforge.fml.common.ProxyInjector.inject(ProxyInjector.java:71) [ProxyInjector.class:?] at net.minecraftforge.fml.common.FMLModContainer.constructMod(FMLModContainer.java:559) [FMLModContainer.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_102] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_102] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_102] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_102] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) [guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) [guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:235) [LoadController.class:?] at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:213) [LoadController.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_102] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_102] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_102] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_102] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) [guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) [guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:142) [LoadController.class:?] at net.minecraftforge.fml.common.Loader.loadMods(Loader.java:531) [Loader.class:?] at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:216) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_102] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_102] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_102] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_102] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_102] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_102] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_102] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_102] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_102] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_102] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_102] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_102] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] [13:18:40] [Client thread/ERROR] [FML]: Fatal errors were detected during the transition from CONSTRUCTING to PREINITIALIZATION. Loading cannot continue [13:18:40] [Client thread/ERROR] [FML]: States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored UC mcp{9.19} [Minecraft Coder Pack] (minecraft.jar) UC FML{8.0.99.99} [Forge Mod Loader] (forgeSrc-1.10.2-12.18.1.2011.jar) UC Forge{12.18.1.2011} [Minecraft Forge] (forgeSrc-1.10.2-12.18.1.2011.jar) UE minedran{1.10.2-1.0} [Minedran] (Minedran_main) [13:18:40] [Client thread/ERROR] [FML]: The following problems were captured during this phase [13:18:40] [Client thread/ERROR] [FML]: Caught exception from minedran net.minecraftforge.fml.common.LoaderException: java.lang.InstantiationException at net.minecraftforge.fml.common.ProxyInjector.inject(ProxyInjector.java:88) ~[forgeSrc-1.10.2-12.18.1.2011.jar:?] at net.minecraftforge.fml.common.FMLModContainer.constructMod(FMLModContainer.java:559) ~[forgeSrc-1.10.2-12.18.1.2011.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_102] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_102] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_102] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_102] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) ~[guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) ~[guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:235) ~[forgeSrc-1.10.2-12.18.1.2011.jar:?] at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:213) ~[forgeSrc-1.10.2-12.18.1.2011.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_102] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_102] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_102] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_102] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) ~[guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) ~[guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:142) [LoadController.class:?] at net.minecraftforge.fml.common.Loader.loadMods(Loader.java:531) [Loader.class:?] at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:216) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_102] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_102] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_102] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_102] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_102] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_102] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_102] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_102] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_102] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_102] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_102] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_102] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: java.lang.InstantiationException at sun.reflect.InstantiationExceptionConstructorAccessorImpl.newInstance(InstantiationExceptionConstructorAccessorImpl.java:48) ~[?:1.8.0_102] at java.lang.reflect.Constructor.newInstance(Constructor.java:423) ~[?:1.8.0_102] at java.lang.Class.newInstance(Class.java:442) ~[?:1.8.0_102] at net.minecraftforge.fml.common.ProxyInjector.inject(ProxyInjector.java:71) ~[forgeSrc-1.10.2-12.18.1.2011.jar:?] ... 44 more [13:18:40] [Client thread/INFO] [sTDOUT]: [net.minecraft.init.Bootstrap:printToSYSOUT:649]: ---- Minecraft Crash Report ---- // I blame Dinnerbone. Time: 6/08/16 1:18 PM Description: There was a severe problem during mod loading that has caused the game to fail net.minecraftforge.fml.common.LoaderException: java.lang.InstantiationException at net.minecraftforge.fml.common.ProxyInjector.inject(ProxyInjector.java:88) at net.minecraftforge.fml.common.FMLModContainer.constructMod(FMLModContainer.java:559) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:235) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:213) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:142) at net.minecraftforge.fml.common.Loader.loadMods(Loader.java:531) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:216) at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) at net.minecraft.client.Minecraft.run(Minecraft.java:386) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) Caused by: java.lang.InstantiationException at sun.reflect.InstantiationExceptionConstructorAccessorImpl.newInstance(InstantiationExceptionConstructorAccessorImpl.java:48) at java.lang.reflect.Constructor.newInstance(Constructor.java:423) at java.lang.Class.newInstance(Class.java:442) at net.minecraftforge.fml.common.ProxyInjector.inject(ProxyInjector.java:71) ... 44 more A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- System Details -- Details: Minecraft Version: 1.10.2 Operating System: Linux (amd64) version 4.6.4-1-ARCH Java Version: 1.8.0_102, Oracle Corporation Java VM Version: OpenJDK 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 462126024 bytes (440 MB) / 691535872 bytes (659 MB) up to 1845493760 bytes (1760 MB) JVM Flags: 0 total; IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP 9.32 Powered by Forge 12.18.1.2011 4 mods loaded, 4 mods active States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored UC mcp{9.19} [Minecraft Coder Pack] (minecraft.jar) UC FML{8.0.99.99} [Forge Mod Loader] (forgeSrc-1.10.2-12.18.1.2011.jar) UC Forge{12.18.1.2011} [Minecraft Forge] (forgeSrc-1.10.2-12.18.1.2011.jar) UE minedran{1.10.2-1.0} [Minedran] (Minedran_main) Loaded coremods (and transformers): GL info: ' Vendor: 'X.Org' Version: '3.0 Mesa 12.0.1' Renderer: 'Gallium 0.4 on AMD HAWAII (DRM 2.43.0 / 4.6.4-1-ARCH, LLVM 3.8.0)' [13:18:40] [Client thread/INFO] [sTDOUT]: [net.minecraft.init.Bootstrap:printToSYSOUT:649]: #@!@# Game crashed! Crash report saved to: #@!@# /drv/Data/Content/Minecraft/Mods/1.10.2/Minedran/run/./crash-reports/crash-2016-08-06_13.18.40-client.txt Process finished with exit code 255 Here's my main class: [spoiler=Main Class] package com.t10a.minedran; import com.t10a.minedran.proxy.ICommonProxy; import com.t10a.minedran.reference.Reference; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; @Mod(modid=Reference.MOD_ID, name=Reference.NAME, version=Reference.VERSION, acceptedMinecraftVersions = Reference.ACCEPTED_VERSION) public class Minedran { @Mod.Instance public static Minedran instance; @SidedProxy(clientSide = Reference.CLIENT_PROXY_CLASS,serverSide = Reference.SERVER_PROXY_CLASS) public static ICommonProxy proxy; @Mod.EventHandler public void preInit(FMLPreInitializationEvent event) { System.out.println("Pre Initialisation!"); } @Mod.EventHandler public void Init(FMLInitializationEvent event) { System.out.println("Initialisation!"); proxy.init(); } @Mod.EventHandler public void postInit(FMLPostInitializationEvent event) { System.out.println("Post Initialisation!"); } } Here's my Reference file: [spoiler=Reference] package com.t10a.minedran.reference; public class Reference { public static final String MOD_ID = "minedran"; public static final String NAME = "Minedran"; public static final String VERSION = "1.10.2-1.0"; public static final String ACCEPTED_VERSION = "[1.10.2]"; public static final String CLIENT_PROXY_CLASS = "com.t10a.minedran.proxy.ClientProxy"; public static final String SERVER_PROXY_CLASS = "com.t10a.minedran.proxy.ServerProxy"; } If you could help me with this issue, that would be appreciated.
-
[1.10.2] [Unsolved] Updating mod results in broken models
T-10a replied to T-10a's topic in Modder Support
Bump. Still doesn't seem to work, even when I set all of the blockstate files to the type "inventory", the model doesn't work, and the en_US.lang doesn't work either. They were all valid prior to 1.10.2 -
[1.10.2] [Unsolved] Updating mod results in broken models
T-10a replied to T-10a's topic in Modder Support
I entered those commands, and entered gradle setupDecompWorkspace and gradle idea , and the same error occurs. -
[1.10.2] [Unsolved] Updating mod results in broken models
T-10a replied to T-10a's topic in Modder Support
Okay, I added blockstate files. However, it's still not reading the json models properly. Here's a log example for an item, which is repeated for them all: [spoiler=Item Log Exerpt] [16:58:27] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemMace_gold#inventory for item "minedran:ItemMace_gold", normal location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:item/ItemMace_gold with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:317) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:122) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:540) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: java.io.FileNotFoundException: minedran:models/item/ItemMace_gold.json at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:69) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:311) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$1100(ModelLoader.java:118) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:868) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 25 more [16:58:27] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemMace_gold#inventory for item "minedran:ItemMace_gold", blockstate location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:ItemMace_gold#inventory with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:325) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:122) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:540) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:78) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1183) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 25 more The original Github link still applies, and the init file has not been changed at all, aside from removing a few things from the Ore Dictionary. Also, the en_US.lang no longer works, which is weird. -
Hello, After finally being able to get my mod running in a 1.10.2 environment, I cannot seem to be able to load my models or lang files anymore. They worked perfectly before in 1.9.4, but they don't anymore. Here's my log. [spoiler=Last runtime log] /usr/lib/jvm/java-8-openjdk/bin/java -Didea.launcher.port=7532 -Didea.launcher.bin.path=/usr/share/intellijidea-ce/bin -Dfile.encoding=UTF-8 -classpath /usr/lib/jvm/java-8-openjdk/jre/lib/charsets.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/cldrdata.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/dnsns.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/jaccess.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/localedata.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/nashorn.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/sunec.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/sunjce_provider.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/sunpkcs11.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/ext/zipfs.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/jce.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/jsse.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/management-agent.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/resources.jar:/usr/lib/jvm/java-8-openjdk/jre/lib/rt.jar:/drv/Data/Content/Minecraft/Mods/1.10.2/minedran/build/classes/main:/home/thomas/.gradle/caches/minecraft/deobfedDeps/compileDummy.jar:/home/thomas/.gradle/caches/minecraft/deobfedDeps/providedDummy.jar:/home/thomas/.gradle/caches/minecraft/net/minecraftforge/forge/1.10.2-12.18.1.2014/snapshot/20160518/forgeSrc-1.10.2-12.18.1.2014.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.google.code.findbugs/jsr305/3.0.1/f7be08ec23c21485b9b5a1cf1654c2ec8c58168d/jsr305-3.0.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.mojang/netty/1.6/4b75825a06139752bd800d9e29c5fd55b8b1b1e4/netty-1.6.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/oshi-project/oshi-core/1.1/9ddf7b048a8d701be231c0f4f95fd986198fd2d8/oshi-core-1.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.java.dev.jna/jna/3.4.0/803ff252fedbd395baffd43b37341dc4a150a554/jna-3.4.0.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.java.dev.jna/platform/3.4.0/e3f70017be8100d3d6923f50b3d2ee17714e9c13/platform-3.4.0.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.ibm.icu/icu4j-core-mojang/51.2/63d216a9311cca6be337c1e458e587f99d382b84/icu4j-core-mojang-51.2.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.sf.jopt-simple/jopt-simple/4.6/306816fb57cf94f108a43c95731b08934dcae15c/jopt-simple-4.6.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/io.netty/netty-all/4.0.23.Final/294104aaf1781d6a56a07d561e792c5d0c95f45/netty-all-4.0.23.Final.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.google.guava/guava/17.0/9c6ef172e8de35fd8d4d8783e4821e57cdef7445/guava-17.0.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.apache.commons/commons-lang3/3.3.2/90a3822c38ec8c996e84c16a3477ef632cbc87a3/commons-lang3-3.3.2.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/commons-io/commons-io/2.4/b1b6ea3b7e4aa4f492509a4952029cd8e48019ad/commons-io-2.4.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/commons-codec/commons-codec/1.9/9ce04e34240f674bc72680f8b843b1457383161a/commons-codec-1.9.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.java.jutils/jutils/1.0.0/e12fe1fda814bd348c1579329c86943d2cd3c6a6/jutils-1.0.0.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.google.code.gson/gson/2.2.4/a60a5e993c98c864010053cb901b7eab25306568/gson-2.2.4.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.mojang/authlib/1.5.22/afaa8f6df976fcb5520e76ef1d5798c9e6b5c0b2/authlib-1.5.22.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.mojang/realms/1.9.3/b291425bf7ef763452eaa894575018706339f72b/realms-1.9.3.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.apache.commons/commons-compress/1.8.1/a698750c16740fd5b3871425f4cb3bbaa87f529d/commons-compress-1.8.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.apache.httpcomponents/httpclient/4.3.3/18f4247ff4572a074444572cee34647c43e7c9c7/httpclient-4.3.3.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/commons-logging/commons-logging/1.1.3/f6f66e966c70a83ffbdb6f17a0919eaf7c8aca7f/commons-logging-1.1.3.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.apache.httpcomponents/httpcore/4.3.2/31fbbff1ddbf98f3aa7377c94d33b0447c646b6e/httpcore-4.3.2.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/it.unimi.dsi/fastutil/7.0.12_mojang/ba787e741efdc425fc5d2ea654b57c15fba27efa/fastutil-7.0.12_mojang.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.apache.logging.log4j/log4j-api/2.0-beta9/1dd66e68cccd907880229f9e2de1314bd13ff785/log4j-api-2.0-beta9.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.apache.logging.log4j/log4j-core/2.0-beta9/678861ba1b2e1fccb594bb0ca03114bb05da9695/log4j-core-2.0-beta9.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.minecraft/launchwrapper/1.12/111e7bea9c968cdb3d06ef4632bf7ff0824d0f36/launchwrapper-1.12.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/jline/jline/2.13/2d9530d0a25daffaffda7c35037b046b627bb171/jline-2.13.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.ow2.asm/asm-debug-all/5.0.3/f9e364ae2a66ce2a543012a4668856e84e5dab74/asm-debug-all-5.0.3.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.typesafe.akka/akka-actor_2.11/2.3.3/ed62e9fc709ca0f2ff1a3220daa8b70a2870078e/akka-actor_2.11-2.3.3.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.typesafe/config/1.2.1/f771f71fdae3df231bcd54d5ca2d57f0bf93f467/config-1.2.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang/scala-actors-migration_2.11/1.1.0/dfa8bc42b181d5b9f1a5dd147f8ae308b893eb6f/scala-actors-migration_2.11-1.1.0.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang/scala-compiler/2.11.1/56ea2e6c025e0821f28d73ca271218b8dd04926a/scala-compiler-2.11.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang.plugins/scala-continuations-library_2.11/1.0.2/e517c53a7e9acd6b1668c5a35eccbaa3bab9aac/scala-continuations-library_2.11-1.0.2.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang.plugins/scala-continuations-plugin_2.11.1/1.0.2/f361a3283452c57fa30c1ee69448995de23c60f7/scala-continuations-plugin_2.11.1-1.0.2.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang/scala-library/2.11.1/e11da23da3eabab9f4777b9220e60d44c1aab6a/scala-library-2.11.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang.modules/scala-parser-combinators_2.11/1.0.1/f05d7345bf5a58924f2837c6c1f4d73a938e1ff0/scala-parser-combinators_2.11-1.0.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang/scala-reflect/2.11.1/6580347e61cc7f8e802941e7fde40fa83b8badeb/scala-reflect-2.11.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang.modules/scala-swing_2.11/1.0.1/b1cdd92bd47b1e1837139c1c53020e86bb9112ae/scala-swing_2.11-1.0.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang.modules/scala-xml_2.11/1.0.2/820fbca7e524b530fdadc594c39d49a21ea0337e/scala-xml_2.11-1.0.2.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/lzma/lzma/0.0.1/521616dc7487b42bef0e803bd2fa3faf668101d7/lzma-0.0.1.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.sf.trove4j/trove4j/3.0.3/42ccaf4761f0dfdfa805c9e340d99a755907e2dd/trove4j-3.0.3.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.paulscode/codecjorbis/20101023/c73b5636faf089d9f00e8732a829577de25237ee/codecjorbis-20101023.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.paulscode/codecwav/20101023/12f031cfe88fef5c1dd36c563c0a3a69bd7261da/codecwav-20101023.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.paulscode/libraryjavasound/20101123/5c5e304366f75f9eaa2e8cca546a1fb6109348b3/libraryjavasound-20101123.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.paulscode/librarylwjglopenal/20100824/73e80d0794c39665aec3f62eee88ca91676674ef/librarylwjglopenal-20100824.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/com.paulscode/soundsystem/20120107/419c05fe9be71f792b2d76cfc9b67f1ed0fec7f6/soundsystem-20120107.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.java.jinput/jinput/2.0.5/39c7796b469a600f72380316f6b1f11db6c2c7c4/jinput-2.0.5.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.lwjgl.lwjgl/lwjgl/2.9.4-nightly-20150209/697517568c68e78ae0b4544145af031c81082dfe/lwjgl-2.9.4-nightly-20150209.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.lwjgl.lwjgl/lwjgl_util/2.9.4-nightly-20150209/d51a7c040a721d13efdfbd34f8b257b2df882ad0/lwjgl_util-2.9.4-nightly-20150209.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/java3d/vecmath/1.5.2/79846ba34cbd89e2422d74d53752f993dcc2ccaf/vecmath-1.5.2.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.fusesource.jansi/jansi/1.11/655c643309c2f45a56a747fda70e3fadf57e9f11/jansi-1.11.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.scala-lang/scala-actors/2.11.0/8ccfb6541de179bb1c4d45cf414acee069b7f78b/scala-actors-2.11.0.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.java.jinput/jinput-platform/2.0.5/7ff832a6eb9ab6a767f1ade2b548092d0fa64795/jinput-platform-2.0.5-natives-linux.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.java.jinput/jinput-platform/2.0.5/385ee093e01f587f30ee1c8a2ee7d408fd732e16/jinput-platform-2.0.5-natives-windows.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/net.java.jinput/jinput-platform/2.0.5/53f9c919f34d2ca9de8c51fc4e1e8282029a9232/jinput-platform-2.0.5-natives-osx.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.lwjgl.lwjgl/lwjgl-platform/2.9.4-nightly-20150209/b84d5102b9dbfabfeb5e43c7e2828d98a7fc80e0/lwjgl-platform-2.9.4-nightly-20150209-natives-windows.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.lwjgl.lwjgl/lwjgl-platform/2.9.4-nightly-20150209/931074f46c795d2f7b30ed6395df5715cfd7675b/lwjgl-platform-2.9.4-nightly-20150209-natives-linux.jar:/home/thomas/.gradle/caches/modules-2/files-2.1/org.lwjgl.lwjgl/lwjgl-platform/2.9.4-nightly-20150209/bcab850f8f487c3f4c4dbabde778bb82bd1a40ed/lwjgl-platform-2.9.4-nightly-20150209-natives-osx.jar:/home/thomas/.gradle/caches/minecraft/net/minecraftforge/forge/1.10.2-12.18.1.2014/start:/usr/share/intellijidea-ce/lib/idea_rt.jar com.intellij.rt.execution.application.AppMain GradleStart [16:02:28] [main/INFO] [GradleStart]: Extra: [] [16:02:28] [main/INFO] [GradleStart]: Running with arguments: [--userProperties, {}, --assetsDir, /home/thomas/.gradle/caches/minecraft/assets, --assetIndex, 1.10, --accessToken{REDACTED}, --version, 1.10.2, --tweakClass, net.minecraftforge.fml.common.launcher.FMLTweaker, --tweakClass, net.minecraftforge.gradle.tweakers.CoremodTweaker] [16:02:28] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLTweaker [16:02:28] [main/INFO] [LaunchWrapper]: Using primary tweak class name net.minecraftforge.fml.common.launcher.FMLTweaker [16:02:28] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.CoremodTweaker [16:02:28] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLTweaker [16:02:29] [main/INFO] [FML]: Forge Mod Loader version 12.18.1.2014 for Minecraft 1.10.2 loading [16:02:29] [main/INFO] [FML]: Java is OpenJDK 64-Bit Server VM, version 1.8.0_92, running on Linux:amd64:4.6.4-1-ARCH, installed at /usr/lib/jvm/java-8-openjdk/jre [16:02:29] [main/INFO] [FML]: Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation [16:02:29] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.CoremodTweaker [16:02:29] [main/INFO] [GradleStart]: Injecting location in coremod net.minecraftforge.fml.relauncher.FMLCorePlugin [16:02:29] [main/INFO] [GradleStart]: Injecting location in coremod net.minecraftforge.classloading.FMLForgePlugin [16:02:29] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [16:02:29] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLDeobfTweaker [16:02:29] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.AccessTransformerTweaker [16:02:29] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [16:02:29] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [16:02:29] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper [16:02:29] [main/ERROR] [FML]: The binary patch set is missing. Either you are in a development environment, or things are not going to work! [16:02:29] [main/ERROR] [FML]: FML appears to be missing any signature data. This is not a good thing [16:02:29] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper [16:02:29] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLDeobfTweaker [16:02:29] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.AccessTransformerTweaker [16:02:29] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.TerminalTweaker [16:02:29] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.TerminalTweaker [16:02:29] [main/INFO] [LaunchWrapper]: Launching wrapped minecraft {net.minecraft.client.main.Main} [16:02:30] [Client thread/INFO]: Setting user: Player273 [16:02:32] [Client thread/WARN]: Skipping bad option: lastServer: [16:02:32] [Client thread/INFO]: LWJGL Version: 2.9.4 [16:02:33] [Client thread/INFO] [sTDOUT]: [net.minecraftforge.fml.client.SplashProgress:start:221]: ---- Minecraft Crash Report ---- // You should try our sister game, Minceraft! Time: 24/07/16 4:02 PM Description: Loading screen debug info This is just a prompt for computer specs to be printed. THIS IS NOT A ERROR A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- System Details -- Details: Minecraft Version: 1.10.2 Operating System: Linux (amd64) version 4.6.4-1-ARCH Java Version: 1.8.0_92, Oracle Corporation Java VM Version: OpenJDK 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 248783792 bytes (237 MB) / 584581120 bytes (557 MB) up to 1845493760 bytes (1760 MB) JVM Flags: 0 total; IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: Loaded coremods (and transformers): GL info: ' Vendor: 'X.Org' Version: '3.0 Mesa 12.0.1' Renderer: 'Gallium 0.4 on AMD HAWAII (DRM 2.43.0 / 4.6.4-1-ARCH, LLVM 3.8.0)' [16:02:33] [Client thread/INFO] [FML]: MinecraftForge v12.18.1.2014 Initialized [16:02:33] [Client thread/INFO] [FML]: Replaced 233 ore recipes [16:02:33] [Client thread/INFO] [FML]: Found 0 mods from the command line. Injecting into mod discoverer [16:02:33] [Client thread/INFO] [FML]: Searching /drv/Data/Content/Minecraft/Mods/1.10.2/minedran/mods for mods [16:02:34] [Client thread/INFO] [FML]: Forge Mod Loader has identified 4 mods to load [16:02:34] [Client thread/INFO] [FML]: Attempting connection with missing mods [mcp, FML, Forge, minedran] at CLIENT [16:02:34] [Client thread/INFO] [FML]: Attempting connection with missing mods [mcp, FML, Forge, minedran] at SERVER [16:02:35] [Client thread/INFO]: Reloading ResourceManager: Default, FMLFileResourcePack:Forge Mod Loader, FMLFileResourcePack:Minecraft Forge, FMLFileResourcePack:Minedran [16:02:35] [Client thread/INFO] [FML]: Processing ObjectHolder annotations [16:02:35] [Client thread/INFO] [FML]: Found 423 ObjectHolder annotations [16:02:35] [Client thread/INFO] [FML]: Identifying ItemStackHolder annotations [16:02:35] [Client thread/INFO] [FML]: Found 0 ItemStackHolder annotations [16:02:35] [Client thread/INFO] [FML]: Configured a dormant chunk cache size of 0 [16:02:35] [Forge Version Check/INFO] [ForgeVersionCheck]: [Forge] Starting version check at http://files.minecraftforge.net/maven/net/minecraftforge/forge/promotions_slim.json [16:02:35] [Client thread/INFO] [sTDOUT]: [com.t10a.minedran.Minedran:preInit:30]: Pre Initialisation! [16:02:35] [Client thread/INFO] [FML]: Applying holder lookups [16:02:35] [Client thread/INFO] [FML]: Holder lookups applied [16:02:35] [Client thread/INFO] [FML]: Injecting itemstacks [16:02:35] [Client thread/INFO] [FML]: Itemstack injection complete [16:02:35] [sound Library Loader/INFO]: Starting up SoundSystem... [16:02:35] [Forge Version Check/INFO] [ForgeVersionCheck]: [Forge] Found status: OUTDATED Target: 12.18.1.2020 [16:02:35] [Thread-7/INFO]: Initializing LWJGL OpenAL [16:02:35] [Thread-7/INFO]: (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org) [16:02:35] [Thread-7/INFO]: OpenAL initialized. [16:02:36] [sound Library Loader/INFO]: Sound engine started [16:02:36] [Client thread/INFO] [FML]: Max texture size: 16384 [16:02:36] [Client thread/INFO]: Created: 16x16 textures-atlas [16:02:37] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemMace_gold#inventory for item "minedran:ItemMace_gold", normal location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:item/ItemMace_gold with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:317) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:122) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:540) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: java.io.FileNotFoundException: minedran:models/item/ItemMace_gold.json at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:69) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:311) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$1100(ModelLoader.java:118) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:868) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 25 more [16:02:37] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemMace_gold#inventory for item "minedran:ItemMace_gold", blockstate location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:ItemMace_gold#inventory with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:325) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:122) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:540) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:78) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1183) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 25 more [16:02:37] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemHandsaw_tutorial#inventory for item "minedran:ItemHandsaw_tutorial", normal location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:item/ItemHandsaw_tutorial with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:317) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:122) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:540) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: java.io.FileNotFoundException: minedran:models/item/ItemHandsaw_tutorial.json at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:69) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:311) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$1100(ModelLoader.java:118) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:868) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 25 more [16:02:37] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemHandsaw_tutorial#inventory for item "minedran:ItemHandsaw_tutorial", blockstate location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:ItemHandsaw_tutorial#inventory with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:325) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:122) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:540) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:78) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1183) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 25 more [16:02:37] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemWaraxe_diamond#inventory for item "minedran:ItemWaraxe_diamond", normal location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:item/ItemWaraxe_diamond with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:317) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:122) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:540) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: java.io.FileNotFoundException: minedran:models/item/ItemWaraxe_diamond.json at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:69) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:311) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$1100(ModelLoader.java:118) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:868) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 25 more [16:02:37] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemWaraxe_diamond#inventory for item "minedran:ItemWaraxe_diamond", blockstate location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:ItemWaraxe_diamond#inventory with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:325) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:122) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:540) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:78) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1183) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 25 more [16:02:37] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemModHoe_tutorial#inventory for item "minedran:ItemModHoe_tutorial", normal location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:item/ItemModHoe_tutorial with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:317) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:122) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:540) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: java.io.FileNotFoundException: minedran:models/item/ItemModHoe_tutorial.json at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:69) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:311) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$1100(ModelLoader.java:118) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:868) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 25 more [16:02:37] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemModHoe_tutorial#inventory for item "minedran:ItemModHoe_tutorial", blockstate location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:ItemModHoe_tutorial#inventory with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:325) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:122) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:540) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:78) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1183) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 25 more [16:02:37] [Client thread/ERROR] [FML]: Suppressed additional 26 model loading errors for domain minedran [16:02:37] [Client thread/INFO] [sTDOUT]: [com.t10a.minedran.Minedran:Init:39]: Initialisation! [16:02:37] [Client thread/INFO] [FML]: Injecting itemstacks [16:02:37] [Client thread/INFO] [FML]: Itemstack injection complete [16:02:37] [Client thread/INFO] [sTDOUT]: [com.t10a.minedran.Minedran:postInit:49]: Post Initialisation! [16:02:37] [Client thread/INFO] [FML]: Forge Mod Loader has successfully loaded 4 mods [16:02:37] [Client thread/INFO]: Reloading ResourceManager: Default, FMLFileResourcePack:Forge Mod Loader, FMLFileResourcePack:Minecraft Forge, FMLFileResourcePack:Minedran [16:02:38] [Client thread/INFO]: SoundSystem shutting down... [16:02:38] [Client thread/WARN]: Author: Paul Lamb, www.paulscode.com [16:02:38] [sound Library Loader/INFO]: Starting up SoundSystem... [16:02:38] [Thread-9/INFO]: Initializing LWJGL OpenAL [16:02:38] [Thread-9/INFO]: (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org) [16:02:38] [Thread-9/INFO]: OpenAL initialized. [16:02:38] [sound Library Loader/INFO]: Sound engine started [16:02:39] [Client thread/INFO] [FML]: Max texture size: 16384 [16:02:39] [Client thread/INFO]: Created: 1024x512 textures-atlas [16:02:39] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemMace_gold#inventory for item "minedran:ItemMace_gold", normal location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:item/ItemMace_gold with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:317) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:132) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:113) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:799) [Minecraft.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:338) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:561) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: java.io.FileNotFoundException: minedran:models/item/ItemMace_gold.json at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:69) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:311) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$1100(ModelLoader.java:118) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:868) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 28 more [16:02:39] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemMace_gold#inventory for item "minedran:ItemMace_gold", blockstate location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:ItemMace_gold#inventory with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:325) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:132) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:113) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:799) [Minecraft.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:338) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:561) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:78) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1183) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 28 more [16:02:39] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemHandsaw_tutorial#inventory for item "minedran:ItemHandsaw_tutorial", normal location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:item/ItemHandsaw_tutorial with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:317) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:132) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:113) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:799) [Minecraft.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:338) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:561) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: java.io.FileNotFoundException: minedran:models/item/ItemHandsaw_tutorial.json at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:69) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:311) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$1100(ModelLoader.java:118) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:868) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 28 more [16:02:39] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemHandsaw_tutorial#inventory for item "minedran:ItemHandsaw_tutorial", blockstate location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:ItemHandsaw_tutorial#inventory with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:325) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:132) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:113) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:799) [Minecraft.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:338) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:561) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:78) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1183) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 28 more [16:02:39] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemWaraxe_diamond#inventory for item "minedran:ItemWaraxe_diamond", normal location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:item/ItemWaraxe_diamond with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:317) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:132) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:113) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:799) [Minecraft.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:338) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:561) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: java.io.FileNotFoundException: minedran:models/item/ItemWaraxe_diamond.json at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:69) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:311) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$1100(ModelLoader.java:118) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:868) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 28 more [16:02:39] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemWaraxe_diamond#inventory for item "minedran:ItemWaraxe_diamond", blockstate location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:ItemWaraxe_diamond#inventory with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:325) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:132) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:113) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:799) [Minecraft.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:338) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:561) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:78) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1183) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 28 more [16:02:39] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemModHoe_tutorial#inventory for item "minedran:ItemModHoe_tutorial", normal location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:item/ItemModHoe_tutorial with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:317) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:132) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:113) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:799) [Minecraft.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:338) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:561) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: java.io.FileNotFoundException: minedran:models/item/ItemModHoe_tutorial.json at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:69) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:311) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$1100(ModelLoader.java:118) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:868) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 28 more [16:02:39] [Client thread/ERROR] [FML]: Exception loading model for variant minedran:ItemModHoe_tutorial#inventory for item "minedran:ItemModHoe_tutorial", blockstate location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model minedran:ItemModHoe_tutorial#inventory with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:325) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:132) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:113) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:799) [Minecraft.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:338) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:561) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_92] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_92] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_92] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_92] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:78) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1183) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 28 more [16:02:39] [Client thread/ERROR] [FML]: Suppressed additional 26 model loading errors for domain minedran [16:02:39] [Client thread/WARN]: Skipping bad option: lastServer: [16:02:41] [Realms Notification Availability checker #1/INFO]: Could not authorize you against Realms server: Invalid session id [16:02:49] [server thread/INFO]: Starting integrated minecraft server version 1.10.2 [16:02:49] [server thread/INFO]: Generating keypair [16:02:49] [server thread/INFO] [FML]: Injecting existing block and item data into this server instance [16:02:49] [server thread/INFO] [FML]: Applying holder lookups [16:02:49] [server thread/INFO] [FML]: Holder lookups applied [16:02:50] [server thread/INFO] [FML]: Loading dimension 0 (New World) (net.minecraft.server.integrated.IntegratedServer@53675c7d) [16:02:50] [server thread/INFO] [FML]: Loading dimension 1 (New World) (net.minecraft.server.integrated.IntegratedServer@53675c7d) [16:02:50] [server thread/INFO] [FML]: Loading dimension -1 (New World) (net.minecraft.server.integrated.IntegratedServer@53675c7d) [16:02:50] [server thread/INFO]: Preparing start region for level 0 [16:02:51] [server thread/INFO]: Preparing spawn area: 81% [16:02:51] [server thread/INFO]: Changing view distance to 12, from 10 [16:02:52] [Netty Local Client IO #0/INFO] [FML]: Server protocol version 2 [16:02:52] [Netty Server IO #1/INFO] [FML]: Client protocol version 2 [16:02:52] [Netty Server IO #1/INFO] [FML]: Client attempting to join with 4 mods : [email protected],[email protected],[email protected],[email protected] [16:02:52] [Netty Local Client IO #0/INFO] [FML]: [Netty Local Client IO #0] Client side modded connection established [16:02:52] [server thread/INFO] [FML]: [server thread] Server side modded connection established [16:02:52] [server thread/INFO]: Player273[local:E:e79137e0] logged in with entity id 401 at (-143.71870818168446, 80.02679130334145, 237.66175297429402) [16:02:52] [server thread/INFO]: Player273 joined the game [16:02:53] [server thread/INFO]: Saving and pausing game... [16:02:53] [server thread/INFO]: Saving chunks for level 'New World'/Overworld [16:02:53] [server thread/INFO]: Saving chunks for level 'New World'/Nether [16:02:53] [server thread/INFO]: Saving chunks for level 'New World'/The End [16:02:53] [pool-2-thread-1/WARN]: Couldn't look up profile properties for com.mojang.authlib.GameProfile@791b5072[id=18b3a035-3e67-34ed-889e-932160306e4f,name=Player273,properties={},legacy=false] com.mojang.authlib.exceptions.AuthenticationException: The client has sent too many requests within a certain amount of time at com.mojang.authlib.yggdrasil.YggdrasilAuthenticationService.makeRequest(YggdrasilAuthenticationService.java:65) ~[YggdrasilAuthenticationService.class:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService.fillGameProfile(YggdrasilMinecraftSessionService.java:175) [YggdrasilMinecraftSessionService.class:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService$1.load(YggdrasilMinecraftSessionService.java:59) [YggdrasilMinecraftSessionService$1.class:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService$1.load(YggdrasilMinecraftSessionService.java:56) [YggdrasilMinecraftSessionService$1.class:?] at com.google.common.cache.LocalCache$LoadingValueReference.loadFuture(LocalCache.java:3524) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$Segment.loadSync(LocalCache.java:2317) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$Segment.lockedGetOrLoad(LocalCache.java:2280) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$Segment.get(LocalCache.java:2195) [guava-17.0.jar:?] at com.google.common.cache.LocalCache.get(LocalCache.java:3934) [guava-17.0.jar:?] at com.google.common.cache.LocalCache.getOrLoad(LocalCache.java:3938) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$LocalLoadingCache.get(LocalCache.java:4821) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$LocalLoadingCache.getUnchecked(LocalCache.java:4827) [guava-17.0.jar:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService.fillProfileProperties(YggdrasilMinecraftSessionService.java:165) [YggdrasilMinecraftSessionService.class:?] at net.minecraft.client.Minecraft.getProfileProperties(Minecraft.java:3060) [Minecraft.class:?] at net.minecraft.client.resources.SkinManager$3.run(SkinManager.java:131) [skinManager$3.class:?] at java.util.concurrent.Executors$RunnableAdapter.call(Executors.java:511) [?:1.8.0_92] at java.util.concurrent.FutureTask.run(FutureTask.java:266) [?:1.8.0_92] at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1142) [?:1.8.0_92] at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:617) [?:1.8.0_92] at java.lang.Thread.run(Thread.java:745) [?:1.8.0_92] [16:04:09] [server thread/INFO]: Player273 has just earned the achievement [Taking Inventory] [16:04:09] [Client thread/INFO]: [CHAT] Player273 has just earned the achievement [Taking Inventory] [16:04:31] [server thread/INFO]: Saving and pausing game... [16:04:31] [server thread/INFO]: Saving chunks for level 'New World'/Overworld [16:04:31] [server thread/INFO]: Saving chunks for level 'New World'/Nether [16:04:31] [server thread/INFO]: Saving chunks for level 'New World'/The End [16:04:31] [server thread/INFO]: Stopping server [16:04:31] [server thread/INFO]: Saving players [16:04:31] [server thread/INFO]: Saving worlds [16:04:31] [server thread/INFO]: Saving chunks for level 'New World'/Overworld [16:04:31] [server thread/INFO]: Saving chunks for level 'New World'/Nether [16:04:31] [server thread/INFO]: Saving chunks for level 'New World'/The End [16:04:32] [server thread/INFO] [FML]: Unloading dimension 0 [16:04:32] [server thread/INFO] [FML]: Unloading dimension -1 [16:04:32] [server thread/INFO] [FML]: Unloading dimension 1 [16:04:32] [server thread/INFO] [FML]: Applying holder lookups [16:04:32] [server thread/INFO] [FML]: Holder lookups applied [16:04:33] [Client thread/INFO]: Stopping! [16:04:33] [Client thread/INFO]: SoundSystem shutting down... [16:04:33] [Client thread/WARN]: Author: Paul Lamb, www.paulscode.com Process finished with exit code 0 Here's my item init file: [spoiler=Mod Item init file] package com.t10a.minedran.init; import com.t10a.minedran.item.material.ItemTutorial; import com.t10a.minedran.item.tools.*; import com.t10a.minedran.item.weapons.*; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.block.model.ModelResourceLocation; import net.minecraft.item.Item; import net.minecraft.item.Item.ToolMaterial; import net.minecraft.item.ItemStack; import net.minecraftforge.client.model.ModelLoader; import net.minecraftforge.common.util.EnumHelper; import net.minecraftforge.fml.common.registry.GameRegistry; import net.minecraftforge.oredict.OreDictionary; public class ModItems { // public static Item <unlocalized name>; public static Item waraxe_wood; public static Item waraxe_stone; public static Item waraxe_iron; public static Item waraxe_diamond; public static Item waraxe_gold; public static Item waraxe_tutorial; public static Item mace_wood; public static Item mace_stone; public static Item mace_iron; public static Item mace_diamond; public static Item mace_gold; public static Item mace_tutorial; public static Item tutorial; public static Item sword_tutorial; public static Item axe_tutorial; public static Item pickaxe_tutorial; public static Item shovel_tutorial; public static Item hoe_tutorial; public static Item shears_gold; public static Item shears_tutorial; public static Item shears_diamond; public static Item handsaw_iron; public static Item handsaw_diamond; public static Item handsaw_gold; public static Item handsaw_tutorial; public static Item scythe_wood; public static Item scythe_stone; public static Item scythe_iron; public static Item scythe_diamond; public static Item scythe_gold; public static Item scythe_tutorial; /*does the texture for items made of this obliterate your eyes? Good news! This is a placeholder that will go once I add proper materials. * TODO MATERIALS: COPPER, BRONZE, SILVER, STEEL, maybe others */ public static ToolMaterial TUTORIAL = EnumHelper.addToolMaterial("TUTORIAL", 3, 1000, 15.0F, 4.0F, 30); public static void init() { /*<unlocalized name>=new Item<name>(); * NOTICE: Because of how Mojang coded axes, any future item that extends ItemAxe HAS to be registered as follows: *<unlocalized name>=new Item<name>(MATERIAL,DAMAGE_FLOAT,ATTACKSPEED_FLOAT); */ waraxe_wood=new ItemWaraxe(ToolMaterial.WOOD); waraxe_stone=new ItemWaraxe(ToolMaterial.STONE); waraxe_iron=new ItemWaraxe(ToolMaterial.IRON); waraxe_diamond=new ItemWaraxe(ToolMaterial.DIAMOND); waraxe_gold=new ItemWaraxe(ToolMaterial.GOLD); waraxe_tutorial=new ItemWaraxe(TUTORIAL); mace_wood=new ItemMace(ToolMaterial.WOOD); mace_stone=new ItemMace(ToolMaterial.STONE); mace_iron=new ItemMace(ToolMaterial.IRON); mace_diamond=new ItemMace(ToolMaterial.DIAMOND); mace_gold=new ItemMace(ToolMaterial.GOLD); mace_tutorial=new ItemMace(TUTORIAL); tutorial = new ItemTutorial(); sword_tutorial = new ItemCustomSword(TUTORIAL); axe_tutorial = new ItemCustomAxe(TUTORIAL, 9F, -3.0F); shovel_tutorial = new ItemCustomShovel(TUTORIAL); pickaxe_tutorial = new ItemCustomPickaxe(TUTORIAL); hoe_tutorial = new ItemCustomHoe(TUTORIAL); shears_gold = new ItemCustomShears(ToolMaterial.GOLD); shears_diamond = new ItemCustomShears(ToolMaterial.DIAMOND); shears_tutorial = new ItemCustomShears(TUTORIAL); handsaw_iron = new ItemHandsaw(ToolMaterial.IRON); handsaw_diamond = new ItemHandsaw(ToolMaterial.DIAMOND); handsaw_gold = new ItemHandsaw(ToolMaterial.GOLD); handsaw_tutorial = new ItemHandsaw(TUTORIAL); scythe_wood = new ItemScythe(ToolMaterial.WOOD); scythe_stone = new ItemScythe(ToolMaterial.STONE); scythe_iron = new ItemScythe(ToolMaterial.IRON); scythe_diamond = new ItemScythe(ToolMaterial.DIAMOND); scythe_gold = new ItemScythe(ToolMaterial.GOLD); scythe_tutorial = new ItemScythe(TUTORIAL); } public static void register() { //GameRegistry.register(<unlocalized name>); //NOTICE: THIS IS VERY LIKELY TO CHANGE ONCE I FIGURE OUT HOW TO REGISTER STUFF PROPERLY GameRegistry.register(waraxe_wood); GameRegistry.register(waraxe_stone); GameRegistry.register(waraxe_iron); GameRegistry.register(waraxe_diamond); GameRegistry.register(waraxe_gold); GameRegistry.register(waraxe_tutorial); GameRegistry.register(mace_wood); GameRegistry.register(mace_stone); GameRegistry.register(mace_iron); GameRegistry.register(mace_diamond); GameRegistry.register(mace_gold); GameRegistry.register(mace_tutorial); GameRegistry.register(tutorial); GameRegistry.register(sword_tutorial); GameRegistry.register(shovel_tutorial); GameRegistry.register(axe_tutorial); GameRegistry.register(pickaxe_tutorial); GameRegistry.register(hoe_tutorial); GameRegistry.register(shears_gold); GameRegistry.register(shears_tutorial); GameRegistry.register(shears_diamond); GameRegistry.register(handsaw_iron); GameRegistry.register(handsaw_diamond); GameRegistry.register(handsaw_gold); GameRegistry.register(handsaw_tutorial); GameRegistry.register(scythe_wood); GameRegistry.register(scythe_stone); GameRegistry.register(scythe_iron); GameRegistry.register(scythe_diamond); GameRegistry.register(scythe_gold); GameRegistry.register(scythe_tutorial); } public static void registerRenders() { //registerRender(<unlocalized name>); registerRender(waraxe_wood); registerRender(waraxe_stone); registerRender(waraxe_iron); registerRender(waraxe_diamond); registerRender(waraxe_gold); registerRender(waraxe_tutorial); registerRender(mace_wood); registerRender(mace_stone); registerRender(mace_iron); registerRender(mace_diamond); registerRender(mace_gold); registerRender(mace_tutorial); registerRender(tutorial); registerRender(sword_tutorial); registerRender(axe_tutorial); registerRender(shovel_tutorial); registerRender(pickaxe_tutorial); registerRender(hoe_tutorial); registerRender(shears_gold); registerRender(shears_tutorial); registerRender(shears_diamond); registerRender(handsaw_iron); registerRender(handsaw_diamond); registerRender(handsaw_gold); registerRender(handsaw_tutorial); registerRender(scythe_wood); registerRender(scythe_stone); registerRender(scythe_iron); registerRender(scythe_diamond); registerRender(scythe_gold); registerRender(scythe_tutorial); } public static void registerOreDictionary() { OreDictionary.registerOre("tutorial", new ItemStack(ModItems.tutorial)); OreDictionary.registerOre("handsaw", new ItemStack(ModItems.handsaw_iron)); OreDictionary.registerOre("handsaw", new ItemStack(ModItems.handsaw_diamond)); OreDictionary.registerOre("handsaw", new ItemStack(ModItems.handsaw_gold)); OreDictionary.registerOre("handsaw", new ItemStack(ModItems.handsaw_tutorial)); } private static void registerRender(Item item) { ModelLoader.setCustomModelResourceLocation(item, 0, new ModelResourceLocation(item.getRegistryName(), "inventory")); } } And yes, I know the log says that the files aren't there. They are, here's my Github for how the mod is setup in the workspace.
-
[1.9.4] Trying to make a certain effect happen with a specific material
T-10a replied to T-10a's topic in Modder Support
Oops. Silly me. Thanks -
Hello, I'm trying to make a custom material apply wither, and set the target on fire if the entity it hits is undead. However, it doesn't seem to apply this effect on hit. Here's my code: [spoiler=Sword Class] package com.t10a.minedran.item.weapons; import com.t10a.minedran.reference.Reference; import com.t10a.minedran.init.ModItems; import com.t10a.minedran.item.MaterialEffects; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.EnumCreatureAttribute; import net.minecraft.init.MobEffects; import net.minecraft.item.ItemStack; import net.minecraft.item.ItemSword; import net.minecraft.potion.PotionEffect; import net.minecraft.util.DamageSource; public class ItemCustomSword extends ItemSword { public ItemCustomSword(ToolMaterial material) { super(material); setMaxStackSize(1); setCreativeTab(CreativeTabs.COMBAT); setUnlocalizedName(Reference.ItemBase.MODSWORD.getUnlocalizedName() + "_" + getToolMaterialName().toLowerCase()); setRegistryName(Reference.ItemBase.MODSWORD.getRegistryName() + "_" + getToolMaterialName().toLowerCase()); } public boolean hitEntity(ItemStack stack, EntityLivingBase target, EntityLivingBase attacker, ToolMaterial material) { MaterialEffects.effectsOnAttack(material, stack, target, attacker); stack.damageItem(1, attacker); return true; } } [spoiler=Material Effects Class] package com.t10a.minedran.item; import com.t10a.minedran.init.ModItems; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.EnumCreatureAttribute; import net.minecraft.init.MobEffects; import net.minecraft.item.Item.ToolMaterial; import net.minecraft.potion.PotionEffect; import net.minecraft.item.ItemStack; public abstract class MaterialEffects { public static void effectsOnAttack(final ToolMaterial material, final ItemStack stack, final EntityLivingBase target, final EntityLivingBase attacker) { if (material.equals(ModItems.TUTORIAL)) { if(target.getCreatureAttribute() == EnumCreatureAttribute.UNDEAD) { target.addPotionEffect(new PotionEffect(MobEffects.WITHER, 60, 3)); target.setFire(10); } } } } Thanks if you can help.
-
Hello, I've been making a new item that I'm planning on making to delete log blocks, and replace it with at least 6 plank blocks of that kind of wood. So far, it's got the shovel code (i.e. turn grass into paths) as a placeholder right click event. What could I use to make this item do what I want? Thanks if you can help.
-
[1.9.4] Making a weapon apply a potion effect randomly?
T-10a replied to T-10a's topic in Modder Support
Fixed it by replacing 'pow == 0' with 'pow == 1' and it works flawlessly. Thanks for the help!