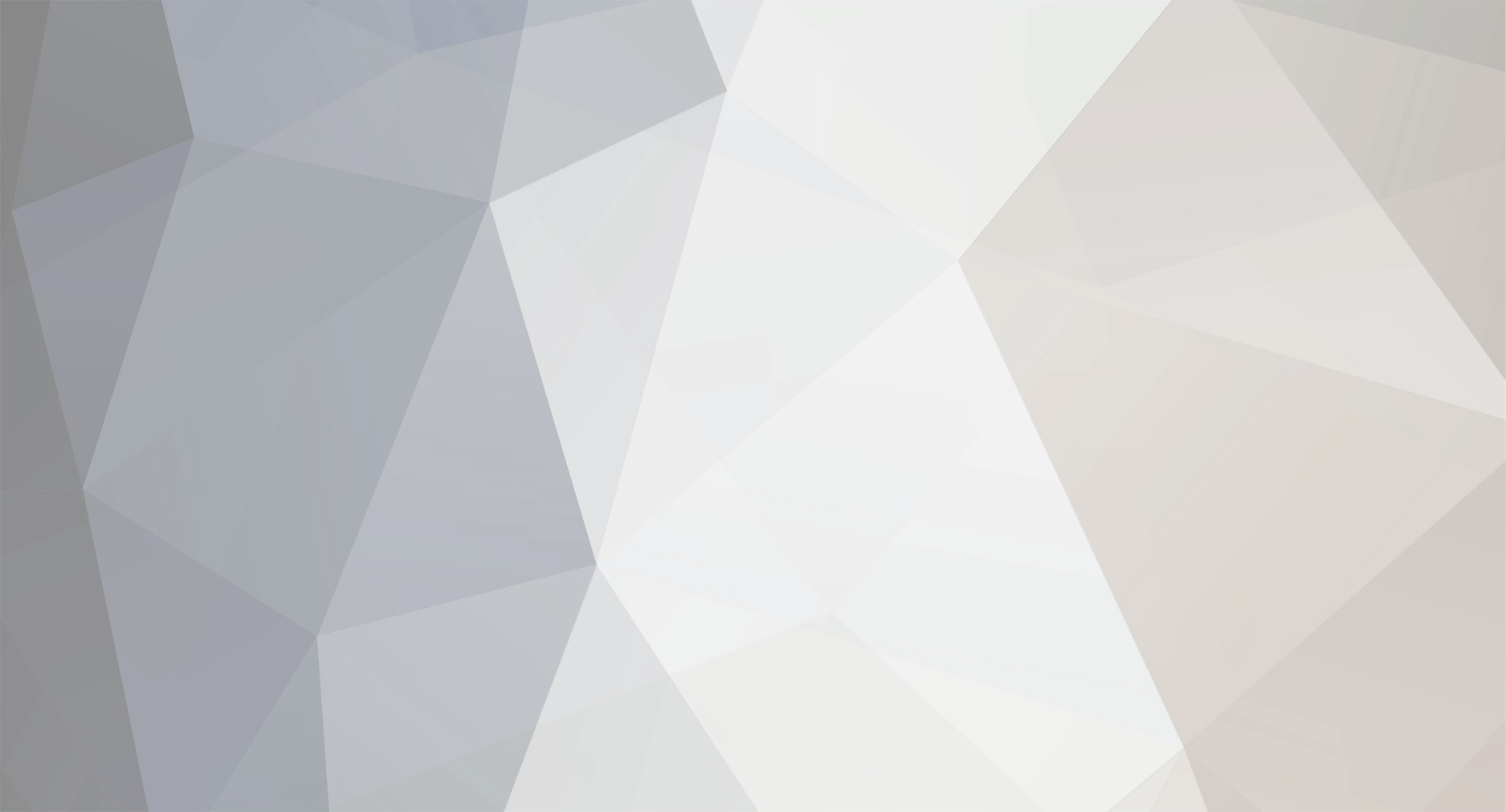
OnePoundPP
-
Posts
64 -
Joined
-
Last visited
Posts posted by OnePoundPP
-
-
Intro:
Minecraft runs on 2 (+2 network) threads (http://mcforge.readthedocs.org/en/latest/concepts/sides/), you want to do stuff on client (bot?).
Each thread tick 20 times, 1tick = 50ms.
Aside from partialTicks (rendering only), this is "planck time" (smallest) in Minecraft world.
What to do?
Make scheduler.
1. @SubscribeEvent to ClientTickEvent.
- Check for event.phase (pick one).
2. Create static Queue or List or whatever (its important it's elements are ordered by inserting).
- You will be throwing "commands" to that list.
- Those "commands" will be calls to execute specific key.
3. Make ClientTickEvent execute one command at time from top of ordered list above every few ticks.
- 10 ticks = 0.5sec like you want.
- You want to do:
int ticks; Queue commands; ClientTickEvent { if (ticks % 10 && !commands.isEmpty) { commands.getFromTop().call(); commands.removeFromTop(); } ++ticks; }
4. Throw "commands" from wherever you want (client thread) into queue.
Right, this is starting to make sense... I add commands to a queue which is handled by another class on each client tick, the commands i'd add in order would be like press key D, followed by release key D and one line would be executed for each client tick, right?
I added this code to a separate class but its coming up with errors, possibly due to a different version?
int ticks;
Queue commands;
@SubscribeEvent
public void onClientTick(TickEvent.ClientTickEvent event){
if(ticks % 10 && !commands.isEmpty()){ *Line doesn't work*
commands.getFromTop().call; *GET FROM and REMOVE FROM top not recognized*
commands.removeFromTop();
}
++ticks;
}
-
That's not at all how Thread.sleep works. Thread.sleep, as the name implies blocks the entire thread for the duration.
As diesieben07 said, you have to use a tick handler and count the number of ticks that have passed to determine the amount of time that has passed.
But I don't need to determine the amount of time that's passed? I just need to insert a delay between two commands running which doesn't pause the entire game, I don't really understand how a tick handler will help, don't really understand how ticks work, all I know is minecraft tick's 20 times per second, I'm guessing its obvious that i'm quite new but what you're saying makes little sense to me. How would i code a delay without pausing minecraft?
-
Right, I've tried the idea of creating a separate class to run the delay off of, got it all working, however the delay still causes the game to freeze..
-
Yes, you can, but only if you understand multithreading well. For example you cannot interact with Minecraft at all from this separate thread, for the most part.
I did not know that. You can't, for example, interact with a TileEntity from a separate thread?
TileEntities exist in the world, so no.
Ok, how would I go about exiting the method and then returning at the same point?It's called "two separate functions."
I'm pretty sure I have an idea of what I'm meant to do, just not how to do it...
In simple terms, in class 1 I would have:
KeyPresser.keyPress(KeyEvent.VK_W);
RUN METHOD IN CLASS 2
KeyPresser.keyRelease(KeyEvent.VK_W);
...
And the method in Class 2:
thread.sleep(20) (FOR ONE SECOND)
Is this how I would do it? and how would this look in code, I'm new to JAVA, appologies. If this is anything like Python, i'd make a function containing code and then call that function but its not as simple as that in java I'm gathering...
-
You have to exit out of the method, count ticks in a tick handler and then when you have counted the right amount of ticks (20 ticks = 1 second) you run the next part of the code.
Ok, how would I go about exiting the method and then returning at the same point?
I'm guessing i'd have to go into my TickHandlerClass and then run a command to exit the EventHandlerClass(where I'm using the Robot KeyPresser), and then run a command to re enter at the same point?
-
Got a quick question. I know using Thread.Sleep or anything as such causes minecraft to pause aswell as it is single threaded, which is obviously a nuisance...
Is there anyway to insert a delay between executing lines of code WITHOUT pausing minecraft?
For example:
KeyPresser.KeyPress(KeyEvent.VK_W);
//WAIT 1 SECOND WITHOUT PAUSING MINECRAFT
KeyPresser.KeyRelease(KeyEvent.VK_W);
I do have a tick handler class, but not really sure how this would help in this case...
Thanks in advance!
-
Basically whenever I press the keybind linked to the following code, the game crashes... There are no errors in the code (I believe) so I cannot really figure whats going on maybe I'm just doing something that I cannot do and being plain stupid
Thanks in advance to any helpers!
---- Minecraft Crash Report ----
// My bad.
Time: 22/08/16 18:24
Description: Unexpected error
java.lang.IllegalMonitorStateException
at java.lang.Object.wait(Native Method)
at me.OnePoundPiglet.SugarBot.EventHandlerClass.onKeyPress(EventHandlerClass.java:48)
at cpw.mods.fml.common.eventhandler.ASMEventHandler_6_EventHandlerClass_onKeyPress_KeyInputEvent.invoke(.dynamic)
at cpw.mods.fml.common.eventhandler.ASMEventHandler.invoke(ASMEventHandler.java:54)
at cpw.mods.fml.common.eventhandler.EventBus.post(EventBus.java:140)
at cpw.mods.fml.common.FMLCommonHandler.fireKeyInput(FMLCommonHandler.java:540)
at net.minecraft.client.Minecraft.runTick(Minecraft.java:1964)
at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1039)
at net.minecraft.client.Minecraft.run(Minecraft.java:962)
at net.minecraft.client.main.Main.main(Main.java:164)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source)
at java.lang.reflect.Method.invoke(Unknown Source)
at net.minecraft.launchwrapper.Launch.launch(Launch.java:135)
at net.minecraft.launchwrapper.Launch.main(Launch.java:28)
at net.minecraftforge.gradle.GradleStartCommon.launch(Unknown Source)
at GradleStart.main(Unknown Source)
A detailed walkthrough of the error, its code path and all known details is as follows:
---------------------------------------------------------------------------------------
-- Head --
Stacktrace:
at java.lang.Object.wait(Native Method)
at me.OnePoundPiglet.SugarBot.EventHandlerClass.onKeyPress(EventHandlerClass.java:48)
at cpw.mods.fml.common.eventhandler.ASMEventHandler_6_EventHandlerClass_onKeyPress_KeyInputEvent.invoke(.dynamic)
at cpw.mods.fml.common.eventhandler.ASMEventHandler.invoke(ASMEventHandler.java:54)
at cpw.mods.fml.common.eventhandler.EventBus.post(EventBus.java:140)
at cpw.mods.fml.common.FMLCommonHandler.fireKeyInput(FMLCommonHandler.java:540)
-- Affected level --
Details:
Level name: MpServer
All players: 1 total; [EntityClientPlayerMP['Player930'/309, l='MpServer', x=-77.63, y=65.62, z=265.60]]
Chunk stats: MultiplayerChunkCache: 460, 460
Level seed: 0
Level generator: ID 00 - default, ver 1. Features enabled: false
Level generator options:
Level spawn location: World: (-76,64,252), Chunk: (at 4,4,12 in -5,15; contains blocks -80,0,240 to -65,255,255), Region: (-1,0; contains chunks -32,0 to -1,31, blocks -512,0,0 to -1,255,511)
Level time: 7701 game time, 7701 day time
Level dimension: 0
Level storage version: 0x00000 - Unknown?
Level weather: Rain time: 0 (now: false), thunder time: 0 (now: false)
Level game mode: Game mode: survival (ID 0). Hardcore: false. Cheats: false
Forced entities: 75 total; [EntityCreeper['Creeper'/259, l='MpServer', x=-8.44, y=36.00, z=265.88], EntityCreeper['Creeper'/133, l='MpServer', x=-112.00, y=10.00, z=235.63], EntityWolf['Wolf'/134, l='MpServer', x=-124.91, y=72.00, z=247.50], EntityBat['Bat'/135, l='MpServer', x=-119.03, y=20.10, z=284.75], EntityChicken['Chicken'/136, l='MpServer', x=-129.60, y=78.40, z=275.51], EntityZombie['Zombie'/137, l='MpServer', x=-123.69, y=15.00, z=303.59], EntityZombie['Zombie'/164, l='MpServer', x=-110.28, y=55.00, z=189.16], EntityWolf['Wolf'/165, l='MpServer', x=-103.22, y=72.00, z=268.56], EntitySkeleton['Skeleton'/166, l='MpServer', x=-97.50, y=12.00, z=304.91], EntitySkeleton['Skeleton'/167, l='MpServer', x=-101.50, y=64.00, z=325.13], EntityZombie['Zombie'/183, l='MpServer', x=-84.72, y=14.00, z=202.31], EntitySpider['Spider'/184, l='MpServer', x=-88.34, y=14.00, z=203.97], EntityHorse['Horse'/185, l='MpServer', x=-80.88, y=64.00, z=195.97], EntityHorse['Horse'/186, l='MpServer', x=-84.06, y=64.00, z=195.97], EntityZombie['Zombie'/187, l='MpServer', x=-93.41, y=13.00, z=213.09], EntityZombie['Zombie'/188, l='MpServer', x=-93.47, y=14.00, z=208.50], EntitySkeleton['Skeleton'/189, l='MpServer', x=-80.88, y=14.00, z=261.59], EntityWolf['Wolf'/190, l='MpServer', x=-82.56, y=64.00, z=271.16], EntityHorse['Horse'/197, l='MpServer', x=-71.00, y=63.00, z=191.00], EntityCreeper['Creeper'/198, l='MpServer', x=-77.50, y=55.00, z=194.94], EntityHorse['Horse'/199, l='MpServer', x=-72.87, y=64.00, z=197.50], EntityItem['item.item.seeds'/200, l='MpServer', x=-69.88, y=66.13, z=230.81], EntityItem['item.tile.dirt.default'/201, l='MpServer', x=-75.59, y=64.13, z=224.81], EntityZombie['Zombie'/202, l='MpServer', x=-68.53, y=13.00, z=251.54], EntityClientPlayerMP['Player930'/309, l='MpServer', x=-77.63, y=65.62, z=265.60], EntityCreeper['Creeper'/203, l='MpServer', x=-66.91, y=14.00, z=248.75], EntityCreeper['Creeper'/204, l='MpServer', x=-63.53, y=17.00, z=243.58], EntityCreeper['Creeper'/205, l='MpServer', x=-66.50, y=14.00, z=247.50], EntityWolf['Wolf'/206, l='MpServer', x=-80.00, y=64.00, z=246.25], EntityCreeper['Creeper'/207, l='MpServer', x=-66.91, y=12.00, z=259.66], EntitySkeleton['Skeleton'/208, l='MpServer', x=-72.56, y=13.00, z=275.50], EntitySkeleton['Skeleton'/209, l='MpServer', x=-70.56, y=20.00, z=274.03], EntitySkeleton['Skeleton'/210, l='MpServer', x=-79.03, y=46.00, z=284.59], EntitySkeleton['Skeleton'/211, l='MpServer', x=-77.56, y=44.00, z=296.16], EntitySquid['Squid'/212, l='MpServer', x=-62.37, y=48.44, z=308.50], EntityZombie['Zombie'/213, l='MpServer', x=-72.81, y=36.00, z=334.34], EntityHorse['Horse'/222, l='MpServer', x=-61.06, y=65.00, z=208.25], EntitySkeleton['Skeleton'/223, l='MpServer', x=-55.06, y=13.00, z=283.50], EntitySkeleton['Skeleton'/224, l='MpServer', x=-60.50, y=16.00, z=285.50], EntitySquid['Squid'/225, l='MpServer', x=-55.50, y=47.40, z=302.93], EntityCreeper['Creeper'/226, l='MpServer', x=-60.97, y=30.00, z=313.50], EntitySquid['Squid'/227, l='MpServer', x=-64.56, y=48.00, z=309.41], EntityChicken['Chicken'/99, l='MpServer', x=-151.72, y=66.44, z=194.44], EntitySquid['Squid'/228, l='MpServer', x=-64.53, y=48.41, z=312.53], EntityChicken['Chicken'/100, l='MpServer', x=-152.53, y=70.00, z=228.53], EntitySquid['Squid'/229, l='MpServer', x=-49.11, y=48.33, z=316.68], EntityBat['Bat'/101, l='MpServer', x=-147.25, y=24.10, z=245.94], EntityBat['Bat'/230, l='MpServer', x=-63.22, y=19.10, z=340.78], EntitySkeleton['Skeleton'/102, l='MpServer', x=-147.50, y=23.00, z=289.10], EntitySkeleton['Skeleton'/103, l='MpServer', x=-145.06, y=23.00, z=289.53], EntitySquid['Squid'/232, l='MpServer', x=-43.43, y=46.37, z=225.80], EntityChicken['Chicken'/104, l='MpServer', x=-158.59, y=69.00, z=289.19], EntitySquid['Squid'/233, l='MpServer', x=-42.47, y=46.15, z=297.53], EntityChicken['Chicken'/105, l='MpServer', x=-146.53, y=64.00, z=286.10], EntitySquid['Squid'/234, l='MpServer', x=-43.44, y=46.37, z=292.47], EntityChicken['Chicken'/106, l='MpServer', x=-146.66, y=67.00, z=290.47], EntitySquid['Squid'/235, l='MpServer', x=-40.76, y=48.00, z=311.61], EntitySquid['Squid'/237, l='MpServer', x=-24.31, y=47.17, z=213.24], EntitySquid['Squid'/238, l='MpServer', x=-30.39, y=46.00, z=229.58], EntitySquid['Squid'/239, l='MpServer', x=-30.60, y=47.38, z=270.11], EntitySquid['Squid'/240, l='MpServer', x=-26.40, y=47.34, z=281.51], EntityChicken['Chicken'/112, l='MpServer', x=-133.31, y=66.40, z=207.81], EntityBat['Bat'/113, l='MpServer', x=-135.79, y=17.00, z=257.50], EntitySkeleton['Skeleton'/242, l='MpServer', x=-18.28, y=23.00, z=298.50], EntityBat['Bat'/114, l='MpServer', x=-139.77, y=19.30, z=270.41], EntityCreeper['Creeper'/243, l='MpServer', x=-16.59, y=35.00, z=324.00], EntityZombie['Zombie'/115, l='MpServer', x=-136.00, y=12.00, z=302.44], EntitySkeleton['Skeleton'/244, l='MpServer', x=-18.69, y=35.00, z=336.31], EntitySkeleton['Skeleton'/245, l='MpServer', x=-17.53, y=35.00, z=340.00], EntitySpider['Spider'/250, l='MpServer', x=-9.09, y=18.00, z=206.75], EntityCreeper['Creeper'/251, l='MpServer', x=-0.94, y=15.00, z=212.97], EntityZombie['Zombie'/252, l='MpServer', x=-12.03, y=19.00, z=222.53], EntityZombie['Zombie'/253, l='MpServer', x=-8.38, y=20.00, z=210.50], EntityEnderman['Enderman'/254, l='MpServer', x=-4.66, y=17.00, z=222.00], EntityEnderman['Enderman'/255, l='MpServer', x=-3.60, y=16.00, z=220.53]]
Retry entities: 0 total; []
Server brand: fml,forge
Server type: Integrated singleplayer server
Stacktrace:
at net.minecraft.client.multiplayer.WorldClient.addWorldInfoToCrashReport(WorldClient.java:415)
at net.minecraft.client.Minecraft.addGraphicsAndWorldToCrashReport(Minecraft.java:2566)
at net.minecraft.client.Minecraft.run(Minecraft.java:991)
at net.minecraft.client.main.Main.main(Main.java:164)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source)
at java.lang.reflect.Method.invoke(Unknown Source)
at net.minecraft.launchwrapper.Launch.launch(Launch.java:135)
at net.minecraft.launchwrapper.Launch.main(Launch.java:28)
at net.minecraftforge.gradle.GradleStartCommon.launch(Unknown Source)
at GradleStart.main(Unknown Source)
-- System Details --
Details:
Minecraft Version: 1.7.10
Operating System: Windows 10 (amd64) version 10.0
Java Version: 1.8.0_101, Oracle Corporation
Java VM Version: Java HotSpot 64-Bit Server VM (mixed mode), Oracle Corporation
Memory: 656920912 bytes (626 MB) / 1038876672 bytes (990 MB) up to 1038876672 bytes (990 MB)
JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M
AABB Pool Size: 0 (0 bytes; 0 MB) allocated, 0 (0 bytes; 0 MB) used
IntCache: cache: 0, tcache: 0, allocated: 12, tallocated: 94
FML: MCP v9.05 FML v7.10.99.99 Minecraft Forge 10.13.4.1614 4 mods loaded, 4 mods active
States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored
UCHIJAAAA mcp{9.05} [Minecraft Coder Pack] (minecraft.jar)
UCHIJAAAA FML{7.10.99.99} [Forge Mod Loader] (forgeSrc-1.7.10-10.13.4.1614-1.7.10.jar)
UCHIJAAAA Forge{10.13.4.1614} [Minecraft Forge] (forgeSrc-1.7.10-10.13.4.1614-1.7.10.jar)
UCHIJAAAA SugarBot{1.0} [sugarBot] (bin)
GL info: ' Vendor: 'NVIDIA Corporation' Version: '4.5.0 NVIDIA 368.69' Renderer: 'GeForce GTX 970/PCIe/SSE2'
Launched Version: 1.7.10
LWJGL: 2.9.1
OpenGL: GeForce GTX 970/PCIe/SSE2 GL version 4.5.0 NVIDIA 368.69, NVIDIA Corporation
GL Caps: Using GL 1.3 multitexturing.
Using framebuffer objects because OpenGL 3.0 is supported and separate blending is supported.
Anisotropic filtering is supported and maximum anisotropy is 16.
Shaders are available because OpenGL 2.1 is supported.
Is Modded: Definitely; Client brand changed to 'fml,forge'
Type: Client (map_client.txt)
Resource Packs: []
Current Language: English (US)
Profiler Position: N/A (disabled)
Vec3 Pool Size: 0 (0 bytes; 0 MB) allocated, 0 (0 bytes; 0 MB) used
Anisotropic Filtering: Off (1)
CODE:
@SubscribeEvent
public void onKeyPress(InputEvent.KeyInputEvent keyin) throws AWTException, InterruptedException {
EntityPlayer player = Minecraft.getMinecraft().thePlayer;
if(SugarBot.start.isPressed()){
int posx = player.getPlayerCoordinates().posX;
int posxupdate = player.getPlayerCoordinates().posX;
int posz = player.getPlayerCoordinates().posZ;
int poszupdate= player.getPlayerCoordinates().posZ;
Robot keypresser = new Robot();
player.addChatMessage(new ChatComponentText("Harvest Started"));
player.addChatMessage(new ChatComponentText("Press 'C' to cancel"));
boolean harvest = true;
boolean rightharvest = true;
boolean leftharvest = false;
KeyEvent nexthome;
int num = 0;
while(harvest == true){
while(rightharvest = true){
keypresser.keyPress(KeyEvent.VK_D);
this.wait(1);
posxupdate = player.getPlayerCoordinates().posX;
if(posx == posxupdate){
keypresser.keyRelease(KeyEvent.VK_D);
posz = player.getPlayerCoordinates().posZ;
keypresser.keyPress(KeyEvent.VK_W);
this.wait(1);
keypresser.keyRelease(KeyEvent.VK_W);
poszupdate = player.getPlayerCoordinates().posZ;
if(posz == poszupdate){
player.addChatMessage(new ChatComponentText(EnumChatFormatting.GREEN + "Teleporting..."));
keypresser.keyPress(KeyEvent.VK_SLASH);
keypresser.keyPress(KeyEvent.VK_SLASH);
keypresser.keyPress(KeyEvent.VK_H);
keypresser.keyPress(KeyEvent.VK_O);
keypresser.keyPress(KeyEvent.VK_M);
keypresser.keyPress(KeyEvent.VK_E);
keypresser.keyPress(KeyEvent.VK_SPACE);
keypresser.keyPress(KeyEvent.VK_F);
keypresser.keyPress(KeyEvent.VK_A);
keypresser.keyPress(KeyEvent.VK_R);
keypresser.keyPress(KeyEvent.VK_M);
}else{
rightharvest = false;
leftharvest = true;
}
} //POS Z UPDATE
}//RIGHT HARVEST IS TRUE
while(leftharvest == true){
keypresser.keyPress(KeyEvent.VK_A);
this.wait(1);
posxupdate = player.getPlayerCoordinates().posX;
if(posx == posxupdate){
keypresser.keyRelease(KeyEvent.VK_A);
posz = player.getPlayerCoordinates().posZ;
keypresser.keyPress(KeyEvent.VK_W);
this.wait(1);
keypresser.keyRelease(KeyEvent.VK_W);
poszupdate = player.getPlayerCoordinates().posZ;
if(posz == poszupdate){
player.addChatMessage(new ChatComponentText(EnumChatFormatting.GREEN + "Teleporting..."));
keypresser.keyPress(KeyEvent.VK_SLASH);
keypresser.keyPress(KeyEvent.VK_SLASH);
keypresser.keyPress(KeyEvent.VK_H);
keypresser.keyPress(KeyEvent.VK_O);
keypresser.keyPress(KeyEvent.VK_M);
keypresser.keyPress(KeyEvent.VK_E);
keypresser.keyPress(KeyEvent.VK_SPACE);
keypresser.keyPress(KeyEvent.VK_F);
keypresser.keyPress(KeyEvent.VK_A);
keypresser.keyPress(KeyEvent.VK_R);
keypresser.keyPress(KeyEvent.VK_M);
keypresser.keyPress(KeyEvent.VK_ENTER);
}else{
rightharvest = true;
leftharvest = false;
}
}//POSXUPDATE
}//LEFT HARVEST IS TRUE
}//HARVEST IS TRUE
}//if key H is pressed
}//onKeyPress void
[SOLVED] Wait (x) seconds *WITHOUT PAUSING GAME*
in Modder Support
Posted
...