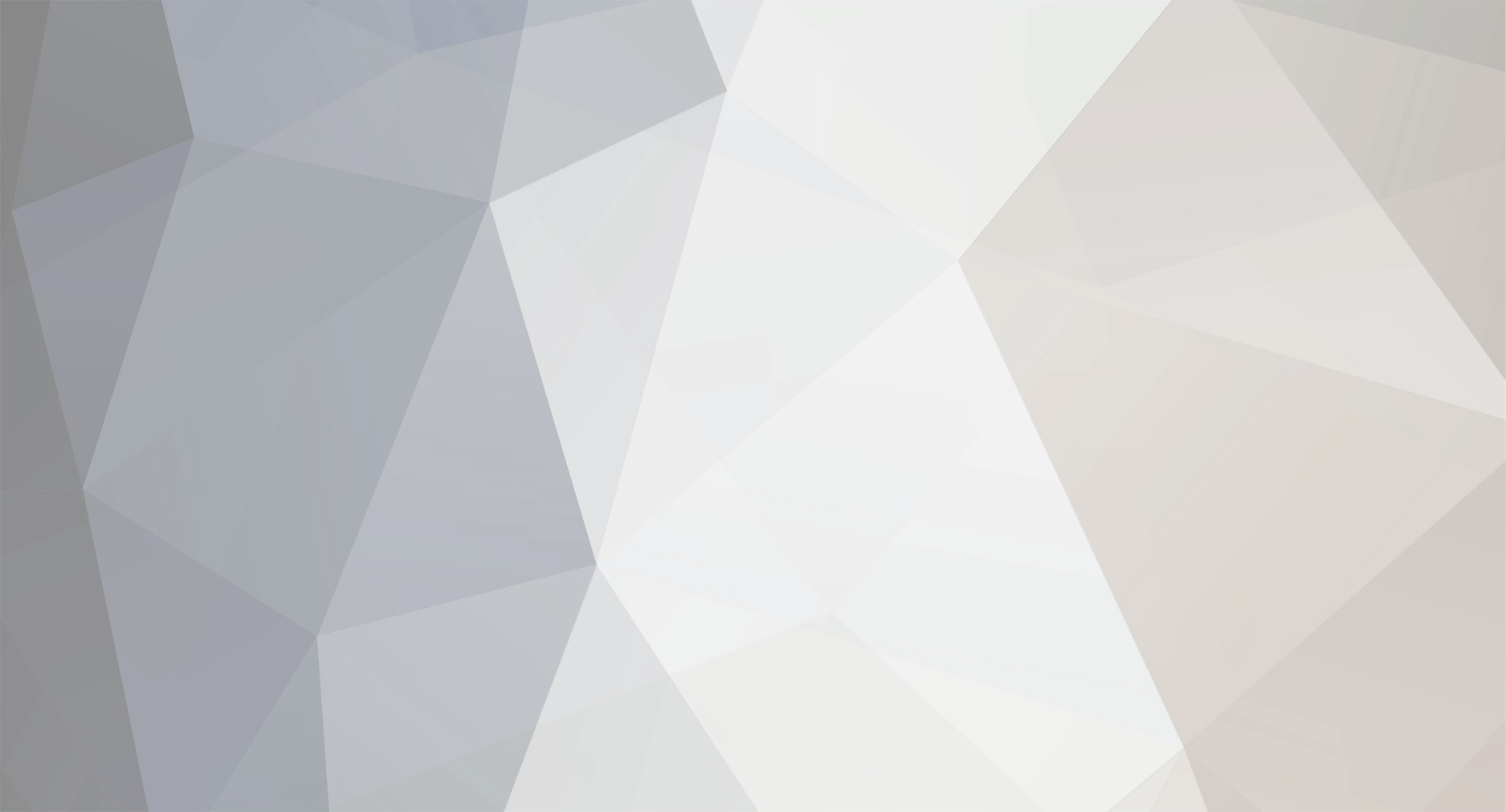
Lambda
Members-
Posts
486 -
Joined
-
Last visited
Everything posted by Lambda
-
Minecraft python api - where is left mouse button
Lambda replied to superbilder's topic in Modder Support
Forge is an API/ML that provides modders with being able to access the Minecraft code and extend upon it.. Yes you can do the things mentioned, however its pretty complicated and I would start with the basics, especially if your new to Java and Forge all together. A great tutorial you could start with is here: https://shadowfacts.net/tutorials/forge-modding-1102/ -
Hey there! So I'm developing a mod on 1.11, however I want to use CompatLayer so that my mod is compatable with 1.10... However, the guide that he provides is upgrading from 1.10 > 1.11, so how would I reverse those roles? Which project would I apply the compatlayer gradle changes? Thanks
-
Hmm after refreshing my gradle project all of my libraries don't exist anymore..? I'm running the setupDecompWorkspace again.. Was this supposed to happen? Edit: After the decomp the libraries are back.. Thanks for your help.
-
Hey there! So I'm trying to decomplie the 'The One Probe' mod so I can add proper intergration for it.. However my build.gradle seems to be throwing this: Error:Could not GET 'https://libraries.minecraft.net/mcjty/theoneprobe/TheOneProbe/1.11-1.3.4-47/TheOneProbe-1.11-1.3.4-47.pom'. Received status code 403 from server: Forbidden Enable Gradle 'offline mode' and sync project Trying to Enable Offline Mode just throws the opposite execption, here is my build.gradle [i have NEVER worked with gradle so, uh I don't know proper syntax. Sorry about that ] buildscript { repositories { jcenter() maven { url = "http://files.minecraftforge.net/maven" } maven { // TOP name 'tterrag maven' url "http://maven.tterrag.com/" } } dependencies { classpath 'net.minecraftforge.gradle:ForgeGradle:2.2-SNAPSHOT' } } apply plugin: 'net.minecraftforge.gradle.forge' //Only edit below this line, the above code adds and enables the necessary things for Forge to be setup. version = "0.0.25" //r-b-a : 25 alpha commits group = "com.lambda.plentifulutilities" // http://maven.apache.org/guides/mini/guide-naming-conventions.html archivesBaseName = "plentifulutilities" sourceCompatibility = targetCompatibility = "1.8" // Need this here so eclipse task generates correctly. compileJava { sourceCompatibility = targetCompatibility = "1.8" } minecraft { version = "1.11.2-13.20.0.2201" runDir = "run" // the mappings can be changed at any time, and must be in the following format. // snapshot_YYYYMMDD snapshot are built nightly. // stable_# stables are built at the discretion of the MCP team. // Use non-default mappings at your own risk. they may not always work. // simply re-run your setup task after changing the mappings to update your workspace. mappings = "snapshot_20161220" // makeObfSourceJar = false // an Srg named sources jar is made by default. uncomment this to disable. } dependencies { deobfCompile "mcjty.theoneprobe:TheOneProbe:1.11-1.3.4-47" // you may put jars on which you depend on in ./libs // or you may define them like so.. //compile "some.group:artifact:version:classifier" //compile "some.group:artifact:version" // real examples //compile 'com.mod-buildcraft:buildcraft:6.0.8:dev' // adds buildcraft to the dev env //compile 'com.googlecode.efficient-java-matrix-library:ejml:0.24' // adds ejml to the dev env // the 'provided' configuration is for optional dependencies that exist at compile-time but might not at runtime. //provided 'com.mod-buildcraft:buildcraft:6.0.8:dev' // the deobf configurations: 'deobfCompile' and 'deobfProvided' are the same as the normal compile and provided, // except that these dependencies get remapped to your current MCP mappings //deobfCompile 'com.mod-buildcraft:buildcraft:6.0.8:dev' //deobfProvided 'com.mod-buildcraft:buildcraft:6.0.8:dev' // for more info... // http://www.gradle.org/docs/current/userguide/artifact_dependencies_tutorial.html // http://www.gradle.org/docs/current/userguide/dependency_management.html } processResources { // this will ensure that this task is redone when the versions change. inputs.property "version", project.version inputs.property "mcversion", project.minecraft.version // replace stuff in mcmod.info, nothing else from(sourceSets.main.resources.srcDirs) { include 'mcmod.info' // replace version and mcversion expand 'version':project.version, 'mcversion':project.minecraft.version } // copy everything else except the mcmod.info from(sourceSets.main.resources.srcDirs) { exclude 'mcmod.info' } } Thanks!
-
Thanks for your help! If anybody is searching for this here is the completed code:
-
Here is what I have so far: @Override public ArrayList<ItemStack> getDrops(IBlockAccess world, BlockPos pos, IBlockState state, int fortune) { TileEntityVoidCrystal te = (TileEntityVoidCrystal)world.getTileEntity(pos); ArrayList<ItemStack> stack = new ArrayList<>(); if(te != null) { NBTTagCompound compound = new NBTTagCompound(); compound.setFloat("Decay", te.decay); compound.setFloat("Purity", te.purity); compound.setFloat("Charge", te.charge); stack.add(new ItemStack()) } } @Override public boolean removedByPlayer(IBlockState state, World world, BlockPos pos, EntityPlayer player, boolean willHarvest) { if(willHarvest) return true; return super.removedByPlayer(state, world, pos, player, willHarvest); } @Override public void harvestBlock(World world, EntityPlayer player, BlockPos pos, IBlockState state, TileEntity te, ItemStack tool) { super.harvestBlock(world, player, pos, state, te, tool); world.setBlockToAir(pos); } However, how do I write that NBT to the ItemStack?
-
Yeah I was afraid of something like this.. Maybe there is a way to prioritize..? but the EnderIO github isn't update, so I don't know how they handle that..
-
Okay, how do I get the integers from the TE to assign the NBT to the block?
-
No no no.. The Block HAS a TileEntity, which stores 3 values.. I want to access those values and display them on the tooltip.
-
Yes I know blocks and items blocks don't have tile entities, the block itself has one with 3 data numbers... So what I'm asking can I get information from the BLOCK TE and put it into the ITEMBLOCK an tooltip.... ex: public class BlockVoidCrystal extends BlockContainerBase { public BlockVoidCrystal(String name) { super(Material.GLASS, name); } @Override public ItemBlockBase getItemBlock() { return new TheItemBlock(this); } @Nullable @Override public TileEntity createNewTileEntity(World worldIn, int meta) { return new TileEntityVoidCrystal(); } @Override @SideOnly(Side.CLIENT) public boolean shouldSideBeRendered(IBlockState blockState, IBlockAccess worldIn, BlockPos pos, EnumFacing side) { return false; } @Override public boolean isBlockNormalCube(IBlockState blockState) { return false; } @Override public boolean isOpaqueCube(IBlockState blockState) { return false; } public static class TheItemBlock extends ItemBlockBase { public TheItemBlock(Block block){ super(block); this.setHasSubtypes(false); this.setMaxDamage(0); } @Override public String getUnlocalizedName(ItemStack stack){ return this.getUnlocalizedName(); } @Override public int getMetadata(int damage){ return damage; } @Override public void addInformation(ItemStack stack, EntityPlayer player, List<String> list, boolean bool){ list.add(StringUtil.localize("")); } } } How would I access said arbitrary data?..
-
I have a custom ItemBlock which is a inner class to the block. I want to put inside the ItemBlock tooltip some values from the TE.. The TE generates randomly, with randomly assigned values, when you pick it up, I want those values to display in the tooltip.
-
Hey there! So I'm in need of accessing a block's TE so I can get a couple of integers from it.. How would I do this? I have the itemblock already setup, however I do not know how to access that information from the TE.. I know I'll probably have to send a packet with the data in it, but I need to access it first.
-
Hey there! So I have a block, 'tethers' that share energy to nearby TEs that have the Energy Capability.. However I'm having issues actually getting this working, I've changed much code, but with no avail. So I came to post this code here, some methods you should look at is the updateEntity(), receiveEnergy(), extractEnergy().. Currently the forloop of 'energyUsers' is not complete, because I knew that wasn't going to work, I need some insight... public class TileEntityNetworkTethers extends TileEntityBase implements ISharingEnergyHandler, IEnergyDisplay { public CustomEnergyStorage storage = new CustomEnergyStorage(50000, 50, 50); public static final int MAX_SEND = 50; public List<TileEntity> energyUsers = new ArrayList<>(); public TileEntityNetworkTethers() { super("networkTethers"); } @Override public void updateEntity() { super.updateEntity(); if(!world.isRemote) { Iterable<BlockPos> blockPos = BlockPos.getAllInBox(pos.add(-5, -5, -5), pos.add(+5, +5, +5)); for (BlockPos position : blockPos) { TileEntity te = world.getTileEntity(position); if (te != null) { if (!energyUsers.contains(te)) { energyUsers.add(te); } } } if(energyUsers.size() != 0) { for(TileEntity te : energyUsers) { if(te != null) { boolean isProducer = false; int energy = 0; for(EnumFacing facing : EnumFacing.VALUES) { if(isProducer(te, facing)) { energy = extractEnergy(te, facing, MAX_SEND); }else { energy = receiveEnergy(te, facing, MAX_SEND); } } } } } validateList(); if(sendUpdateWithInterval()) { } } } public boolean isProducer(TileEntity te, EnumFacing facing) { if (te.hasCapability(CapabilityEnergy.ENERGY, facing)) { IEnergyStorage cap = te.getCapability(CapabilityEnergy.ENERGY, facing); if (cap != null) { if(cap.canReceive()) { return false; } } } return true; } public int receiveEnergy(TileEntity te, EnumFacing facing, int amount) { if (te.hasCapability(CapabilityEnergy.ENERGY, facing)) { IEnergyStorage cap = te.getCapability(CapabilityEnergy.ENERGY, facing); if (cap != null) { return cap.receiveEnergy(amount, false); } } return 0; } public int extractEnergy(TileEntity te, EnumFacing facing, int amount) { if (te.hasCapability(CapabilityEnergy.ENERGY, facing)) { IEnergyStorage cap = te.getCapability(CapabilityEnergy.ENERGY, facing); if (cap != null) { return cap.extractEnergy(amount, false); } } return 0; } public void validateList() { Iterator<TileEntity> iterator = energyUsers.iterator(); while (iterator.hasNext()) { TileEntity te = iterator.next(); if (te.isInvalid()) { iterator.remove(); } } } @Override public IEnergyStorage getEnergyStorage(EnumFacing facing) { return storage; } @Override public int getEnergyToSplitShare() { return storage.getEnergyStored(); } @Override public boolean doesShareEnergy() { return true; } @Override public EnumFacing[] getEnergyShareSides() { return EnumFacing.VALUES; } @Override public boolean canShareTo(TileEntity tile) { return true; } @Override public CustomEnergyStorage getEnergyStorage() { return storage; } @Override public boolean needsHoldShift() { return false; } } The effect I want to have happen: >Pulls energy from anywhere in the 5x5 space. >With that pulled energy it is buffered within the tether, - Then it goes to either two places: >>Another tether, in which they balance out their energy >>A machine that is requesting power (in need of power) Thanks for your time!
-
Thanks.
-
Hey there! So I'm creating a network that has 'tethers' that send energy to nearby acceptable tiles (5x5) however, it seems I'm getting a concurrent modification execption, I know where the error is happening, but I do not know how to 'avoid' it. Here is the code: public class TileEntityNetworkTethers extends TileEntityBase implements ISharingEnergyHandler { public CustomEnergyStorage storage = new CustomEnergyStorage(50000, 50, 50); public static final int MAX_SEND = 50; public boolean removingFromList = false; public List<TileEntity> energyUsers = new ArrayList<>(); public TileEntityNetworkTethers() { super("networkTethers"); } @Override public void updateEntity() { super.updateEntity(); if(!world.isRemote) { storage.receiveEnergyInternal(2, false); Iterable<BlockPos> blockPos = BlockPos.getAllInBox(pos.add(-5, -5, -5), pos.add(+5, +5, +5)); if(blockPos != null) { for (BlockPos position : blockPos) { TileEntity te = world.getTileEntity(position); if (te != null) { if (!energyUsers.contains(te)) { energyUsers.add(te); } } } } if(energyUsers.size() != 0) { for(TileEntity te : energyUsers) { if(te != null) { System.out.println(removingFromList); for(EnumFacing facing : EnumFacing.VALUES) { handleEnergy(te, facing, MAX_SEND); } } } } validateList(); if(sendUpdateWithInterval()) { } } } public void handleEnergy(TileEntity te, EnumFacing facing, int amount) { if (te.hasCapability(CapabilityEnergy.ENERGY, facing)) { IEnergyStorage cap = te.getCapability(CapabilityEnergy.ENERGY, facing); if (cap != null) { if (cap.canReceive()) { if(storage.getEnergyStored() - amount >= 0) { storage.extractEnergyInternal(amount, false); cap.receiveEnergy(amount, false); } } if(cap.canExtract()) { if(storage.getEnergyStored() + amount <= storage.getMaxEnergyStored()) { this.storage.receiveEnergyInternal(amount, false); cap.extractEnergy(amount, false); } } } } } public void validateList() { if (energyUsers != null && energyUsers.size() != 0) { for (TileEntity te : energyUsers) { if(te != null) { if (world.getTileEntity(te.getPos()) == null) { energyUsers.remove(te); } } } } } @Override public IEnergyStorage getEnergyStorage(EnumFacing facing) { return storage; } @Override public int getEnergyToSplitShare() { return storage.getEnergyStored(); } @Override public boolean doesShareEnergy() { return true; } @Override public EnumFacing[] getEnergyShareSides() { return EnumFacing.VALUES; } @Override public boolean canShareTo(TileEntity tile) { return true; } } Here is the crash: net.minecraft.util.ReportedException: Ticking block entity at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:800) ~[MinecraftServer.class:?] at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:698) ~[MinecraftServer.class:?] at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:156) ~[integratedServer.class:?] at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:547) [MinecraftServer.class:?] at java.lang.Thread.run(Thread.java:745) [?:1.8.0_91] Caused by: java.util.ConcurrentModificationException at java.util.ArrayList$Itr.checkForComodification(ArrayList.java:901) ~[?:1.8.0_91] at java.util.ArrayList$Itr.next(ArrayList.java:851) ~[?:1.8.0_91] at com.lambda.plentifulutilities.block.tile.TileEntityNetworkTethers.validateList(TileEntityNetworkTethers.java:95) ~[TileEntityNetworkTethers.class:?] at com.lambda.plentifulutilities.block.tile.TileEntityNetworkTethers.updateEntity(TileEntityNetworkTethers.java:64) ~[TileEntityNetworkTethers.class:?] at com.lambda.plentifulutilities.block.tile.TileEntityBase.update(TileEntityBase.java:171) ~[TileEntityBase.class:?] at net.minecraft.world.World.updateEntities(World.java:1942) ~[World.class:?] at net.minecraft.world.WorldServer.updateEntities(WorldServer.java:647) ~[WorldServer.class:?] at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:794) ~[MinecraftServer.class:?] ... 4 more [14:53:56] [server thread/ERROR]: This crash report has been saved to: F:\Minecraft Workspace\PlentifulUtilities\PlentifulUtilities\run\.\crash-reports\crash-2017-01-06_14.53.56-server.txt [14:53:56] [server thread/INFO]: Stopping server [14:53:56] [server thread/INFO]: Saving players [14:53:56] [Client thread/INFO] [sTDOUT]: [net.minecraft.init.Bootstrap:printToSYSOUT:600]: ---- Minecraft Crash Report ---- // Uh... Did I do that? Where its happening: public void validateList() { if (energyUsers != null && energyUsers.size() != 0) { for (TileEntity te : energyUsers) { if(te != null) { if (world.getTileEntity(te.getPos()) == null) { energyUsers.remove(te); //--------HERE-------- } } } } } Maybe there is a better way to store a TE other than an list? A map wont work due to them not being able to store duplicate keys. Thanks,
-
Fuck.
-
Hey there! So I have a TileEntityBase class that all my TEs use, it holds all the necessary things for a TE to be correctly synced and created... However, its seems that my all my TEs that extend this do not actually have the 'Energy' capability that is defined. Here is the code, in particular the get/has Capability: @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing){ return this.getCapability(capability, facing) != null; } @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing){ if(capability == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY){ IItemHandler handler = this.getItemHandler(facing); if(handler != null){ return (T)handler; } } else if(capability == CapabilityFluidHandler.FLUID_HANDLER_CAPABILITY){ IFluidHandler tank = this.getFluidHandler(facing); if(tank != null){ return (T)tank; } } else if(capability == CapabilityEnergy.ENERGY){ IEnergyStorage storage = this.getEnergyStorage(facing); if(storage != null){ return (T)storage; } } return super.getCapability(capability, facing); } Here is the entire class if you need it: Here is the TE: public class TileEntityNetworkTethers extends TileEntityBase { public static final int MAX_SEND = 50; public List<TileEntity> energyUsers = new ArrayList<>(); public TileEntityNetworkTethers() { super("networkTethers"); } @Override public void updateEntity() { super.updateEntity(); if(!world.isRemote) { Iterable<BlockPos> blockPos = BlockPos.getAllInBox(pos.add(-5, -5, -5), pos.add(+5, +5, +5)); if(blockPos != null) { for(BlockPos position : blockPos) { TileEntity te = world.getTileEntity(position); if(te != null) { if (!energyUsers.contains(te)) { energyUsers.add(te); } } } } if(energyUsers.size() != 0) { for(TileEntity te : energyUsers) { if(te != null) { for(EnumFacing facing : EnumFacing.VALUES) { giveEnergy(te, facing, MAX_SEND); System.out.println(te.hasCapability(CapabilityEnergy.ENERGY, facing)); } } } } if(sendUpdateWithInterval()) { if(world != null) { validateList(); } } } } public int giveEnergy(TileEntity te, EnumFacing facing, int amount) { if (te.hasCapability(CapabilityEnergy.ENERGY, facing)) { IEnergyStorage cap = te.getCapability(CapabilityEnergy.ENERGY, facing); if (cap != null) { if (cap.canReceive()) { return cap.receiveEnergy(amount, false); } } } return 0; } public static boolean isEnergyTE(TileEntity te) { return (te != null && te.hasCapability(CapabilityEnergy.ENERGY, null)); } public void validateList() { if (energyUsers != null && energyUsers.size() != 0) { for (TileEntity te : energyUsers) { if(te != null) { if (world.getTileEntity(te.getPos()) == null) { energyUsers.remove(te); } } } } } } Thanks for your time
-
Ah, didn't see that! Thanks for that.. Edit: So is there even a way to set meta to a block / state any more? I don't see any methods that actually change the meta of the block.
-
Edit this to your needs, I believe it works. Post back if it doesn't. IBlockState state = world.getBlockState(new BlockPos(pos.getX() + i * , pos.getY() + i * yD, pos.getZ() + i * zD)); Block block = state.getBlock(); int meta = block.getMetaFromState(state); world.setBlockState(new BlockPos(pos.getX() + i * , pos.getY()+ i * yD, pos.getZ() + i * zD), world.getBlockState(pos), meta); The World#setBlockState has 2 methods, one that takes the BlockPos and the new state, and one that takes BlockPos, new state, and 'flags', which has yet to be localized to 'meta' I believe.
-
Do you have a github so I can look at all referencing code?
-
Okay It works! But its way to big in game and in the inventory! Do I have to scale it down in blender, if so what to? how would I edit the inventory model?
-
Yeah should of know that... Thanks for that post, will read up and post back if I have any issues.
-
How are you handling the GUI in the first place? GUI is on the Client (so saying, its always sync'd), so if something isn't syncing right, its gotta be somewhere in your container or TE. Post those.
-
Some code would be nice, to tell us how your doing it in the first place..