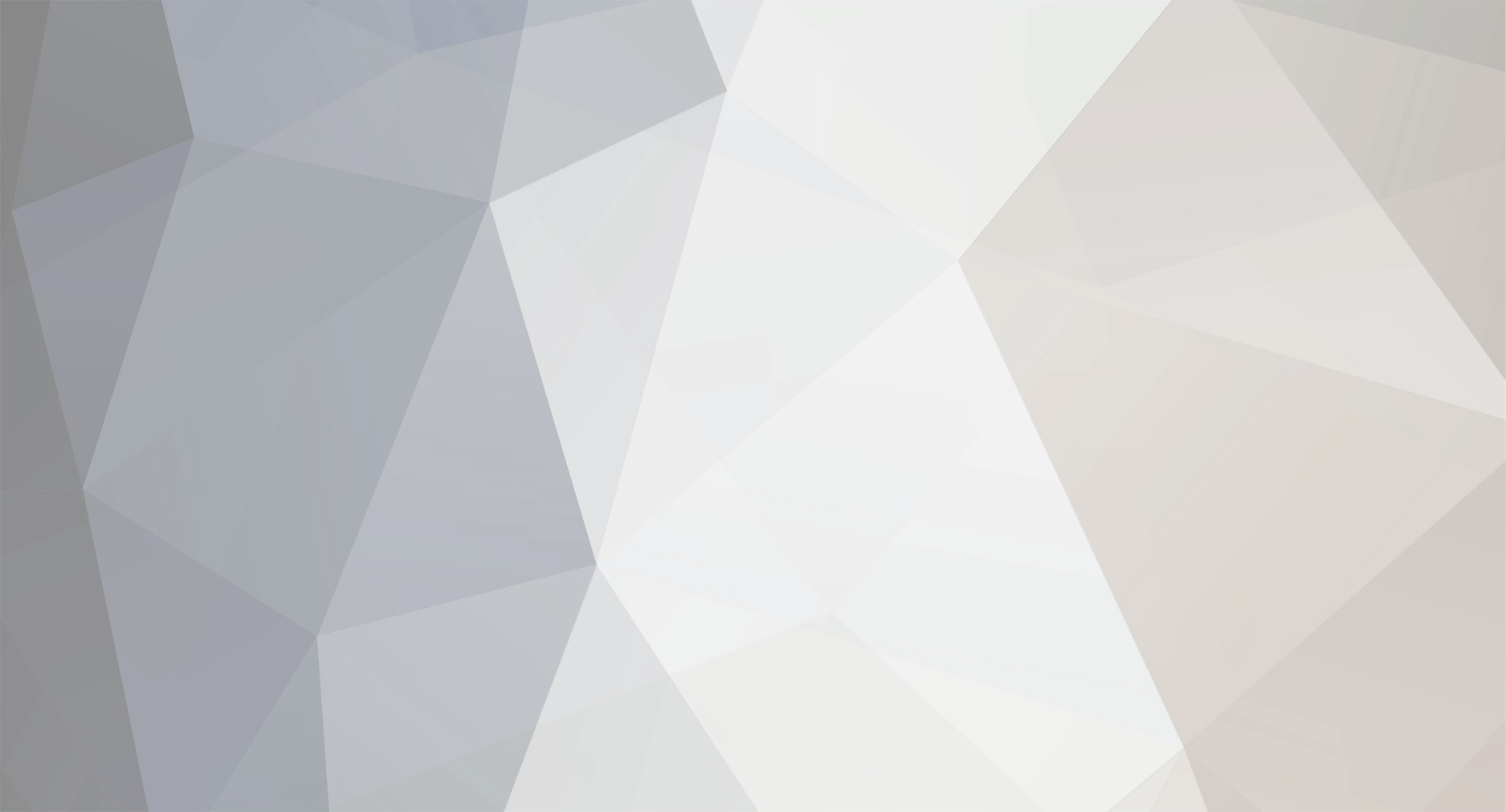
Flawn_
-
Posts
23 -
Joined
-
Last visited
Posts posted by Flawn_
-
-
Hello everyone, I have the following Problem: I have 2 Jars that i add to my build path and it works fine. Also the Debug mode works fine. But when i Compile it in Eclipse the Class wont be found and i become a game crash.
-
Hello everyone,
I want to change the Displayname at the beginning of the game if it is a special name. How do i do it?
-
-
It worked with init at my friend i assume
-
I think in init.
-
func_175180_a in GuiIngame. Just at the top of the bottom you have to call the method. Done
-
Quote
package me.cose.gui.player; import net.minecraft.client.Minecraft; import net.minecraft.client.gui.ScaledResolution; import net.minecraft.client.gui.inventory.GuiInventory; import net.minecraft.client.renderer.GlStateManager; import net.minecraft.client.renderer.InventoryEffectRenderer; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.Container; public abstract class RenderPlayer extends InventoryEffectRenderer { public RenderPlayer(EntityPlayer p_i1094_1_) { super(p_i1094_1_.inventoryContainer); this.allowUserInput = true; } public static void PlayerInTC() { Minecraft mc = Minecraft.getMinecraft(); ScaledResolution var2 = new ScaledResolution(mc, mc.displayWidth, mc.displayHeight); int var3 = var2.getScaledWidth(); int var4 = var2.getScaledHeight(); int ex = var3 / 2 + 195; int ey = var4 / 2 + 117; GuiInventory.drawEntityOnScreen(ex, ey, 30, var3 - 380 - GuiInventory.oldMouseX, var4 - 225 - GuiInventory.oldMouseY, mc.thePlayer); GlStateManager.enableBlend(); } }
PlayerInTC means PlayerinTheCorner
Goodluck
-
Yes it was "GLStateManager.enableBlend()"
Thx Man!
-
No no, I just render the Inventoryplayer again to the left down in Ingame. but when i have a item in the hand i become a blackscreen. Yes like xBox
-
Hello everyone,
when i render the Player in the left Corner ingame and i have a item inhand my screen just becomes black. Why?
-
Why not? I just wanted to ask, because i Find FakePlayer good etc.
-
I ment a functionally Client. Not a mod. When you compile it you have to become a functionally Client jar. Like mcp just with adding Forge, if that works
-
Can you code a Client with forge or use the source within? I want to use the Forge Classes and want to add Mods
-
Yes, but even when i render it in the world it gives me a black screen if i have something in the hand .-.
-
No i ment the Player left above the inv.
-
4 hours ago, diesieben07 said:
You will need to create a fake player to render, if you are not in the world yet there is no player.
But how? Pls say it
-
-
How do you make that?
I would make to it also a Skin
-
Here is the Part where it is called (GuiMainMenu)
public void initGui() { int sx = (int) (width * 0.025); int sy = (int) ( height * 0.25); int ex = width / 2 - 150; EntityLivingBase elb = mc.thePlayer; EntityPlayerSP player = new EntityPlayerSP(); int ey = height / 2 - 150; int swidth = 80 * (int) (width / 427.0); int sheight = 150 * (int) (height / 150.0); updateList = new GuiScrollList(sx, sy, swidth, sheight, 1073741823, "Updates", InfoUpdates.lines, true); GuiInventory.drawEntityOnScreen(ex, ey, 30, (float)(ex) - GuiInventory.oldMouseX, (float)(ey) - GuiInventory.oldMouseY, player); [...] }
-
mc is Minecraft(). Isnt there a possibility that i can render it ? Has somebody a workaround?
-
In GuiMainScreen i gave it so:
EntityLivingBase elb = mc.thePlayer;
[...]
GuiInventory.drawEntityOnScreen(ex, ey, 30, (float)(ex) - GuiInventory.oldMouseX, (float)(ey) - GuiInventory.oldMouseY, elb);
-
Hello everyone,
I want to render the Inventory Player to the main Menu but it makes problems.
Description: Initializing game java.lang.NullPointerException: Initializing game at net.minecraft.client.gui.inventory.GuiInventory.drawEntityOnScreen(GuiInventory.java:103) at net.minecraft.client.gui.GuiMainMenu.initGui(GuiMainMenu.java:213) at net.minecraft.client.gui.GuiScreen.setWorldAndResolution(GuiScreen.java:556) at net.minecraft.client.Minecraft.displayGuiScreen(Minecraft.java:1011) at net.minecraft.client.Minecraft.startGame(Minecraft.java:575) at net.minecraft.client.Minecraft.run(Minecraft.java:402) at net.minecraft.client.main.Main.main(Main.java:114) at Start.main(Start.java:11) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Stacktrace: at net.minecraft.client.gui.inventory.GuiInventory.drawEntityOnScreen(GuiInventory.java:103) at net.minecraft.client.gui.GuiMainMenu.initGui(GuiMainMenu.java:213) at net.minecraft.client.gui.GuiScreen.setWorldAndResolution(GuiScreen.java:556) at net.minecraft.client.Minecraft.displayGuiScreen(Minecraft.java:1011) at net.minecraft.client.Minecraft.startGame(Minecraft.java:575) -- Initialization -- Details: Stacktrace: at net.minecraft.client.Minecraft.run(Minecraft.java:402) at net.minecraft.client.main.Main.main(Main.java:114) at Start.main(Start.java:11) -- System Details -- Details: Minecraft Version: 1.8 Operating System: Mac OS X (x86_64) version 10.12.1 Java Version: 1.8.0_91, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 870970032 bytes (830 MB) / 1038876672 bytes (990 MB) up to 1038876672 bytes (990 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 Launched Version: mcp LWJGL: 2.9.1 OpenGL: Intel(R) Iris(TM) Graphics 6100 GL version 2.1 INTEL-10.20.23, Intel Inc. GL Caps: Using GL 1.3 multitexturing. Using GL 1.3 texture combiners. Using framebuffer objects because ARB_framebuffer_object is supported and separate blending is supported. Shaders are available because OpenGL 2.1 is supported. VBOs are available because OpenGL 1.5 is supported. Using VBOs: No Is Modded: Definitely; Client brand changed to 'Cose' Type: Client (map_client.txt) Resource Packs: [] Current Language: English (US) Profiler Position: N/A (disabled) #@!@# Game crashed! Crash report saved to: #@!@# /Users/nevinpeters/Documents/Cose/jars/./crash-reports/crash-2017-02-05_14.09.16-client.txt AL lib: (EE) alc_cleanup: 1 device not closed
It makes Problems here:
public static void drawEntityOnScreen(int x, int y, int scale, float mouseX, float mouseY, EntityLivingBase entity) { GlStateManager.enableColorMaterial(); GlStateManager.pushMatrix(); GlStateManager.translate((float)x, (float)y, 50.0F); GlStateManager.scale((float)(-scale), (float)scale, (float)scale); GlStateManager.rotate(180.0F, 0.0F, 0.0F, 1.0F); //here float var6 = entity.renderYawOffset; float var7 = entity.rotationYaw; float var8 = entity.rotationPitch; float var9 = entity.prevRotationYawHead; float var10 = entity.rotationYawHead; GlStateManager.rotate(135.0F, 0.0F, 1.0F, 0.0F); RenderHelper.enableStandardItemLighting(); GlStateManager.rotate(-135.0F, 0.0F, 1.0F, 0.0F); GlStateManager.rotate(-((float)Math.atan((double)(mouseY / 40.0F))) * 20.0F, 1.0F, 0.0F, 0.0F); entity.renderYawOffset = (float)Math.atan((double)(mouseX / 40.0F)) * 20.0F; entity.rotationYaw = (float)Math.atan((double)(mouseX / 40.0F)) * 40.0F; entity.rotationPitch = -((float)Math.atan((double)(mouseY / 40.0F))) * 20.0F; entity.rotationYawHead = entity.rotationYaw; entity.prevRotationYawHead = entity.rotationYaw; GlStateManager.translate(0.0F, 0.0F, 0.0F); RenderManager var11 = Minecraft.getMinecraft().getRenderManager(); var11.func_178631_a(180.0F); var11.func_178633_a(false); var11.renderEntityWithPosYaw(entity, 0.0D, 0.0D, 0.0D, 0.0F, 1.0F); var11.func_178633_a(true); entity.renderYawOffset = var6; entity.rotationYaw = var7; entity.rotationPitch = var8; entity.prevRotationYawHead = var9; entity.rotationYawHead = var10; GlStateManager.popMatrix(); RenderHelper.disableStandardItemLighting(); GlStateManager.disableRescaleNormal(); GlStateManager.setActiveTexture(OpenGlHelper.lightmapTexUnit); GlStateManager.func_179090_x(); GlStateManager.setActiveTexture(OpenGlHelper.defaultTexUnit); }
[MCP] [1.8] Jars wont be added at the compiled Client Jar
in Off-topic
Posted
Im in Offtopic, and i wanted to ask it with *MCP* not with Forge. I mod a Client