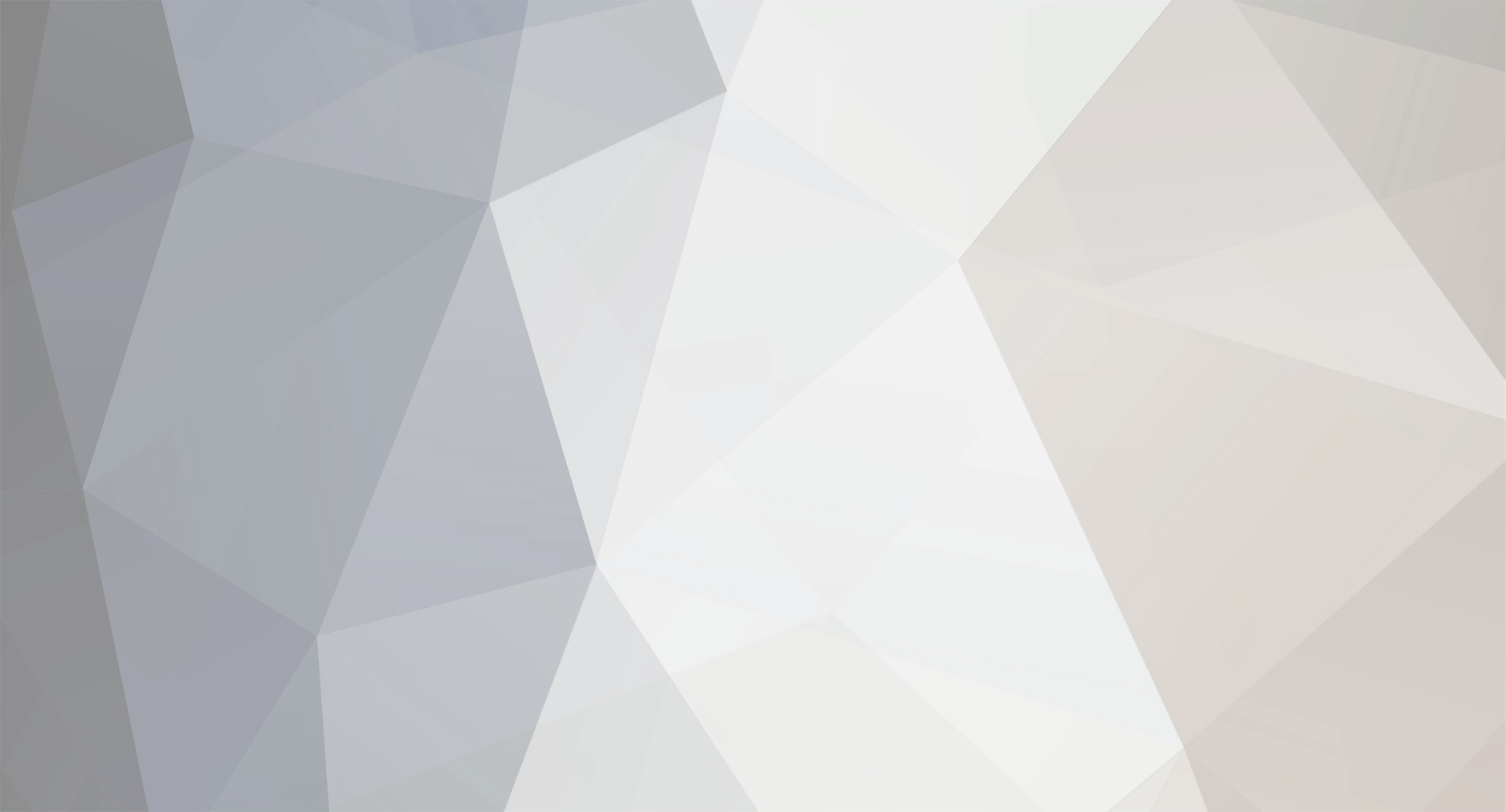
funsize888
Members-
Posts
85 -
Joined
-
Last visited
Everything posted by funsize888
-
Ok, ty will test when back home
-
Sorry I'm new to rendering entity, I'm kinda lost. I have: public class ModEntity implements IRenderFactory{ public static void register(){ createRenderFor(null) } public Render createRenderFor(IRenderFactory renderFactory) { RenderingRegistry.registerEntityRenderingHandler(NukePrimed.class, renderFactory); return null; } } lots of errors but I don't know what goes where
-
Also what should I put for the renderer parameter in: RenderingRegistry.registerEntityRenderingHandler(RenderNukePrimed.class, renderer);
-
should I extend any classes
-
Oh, I don't think I registered it, must've slipped my mind how would I go about doing this?
-
Anybody?
-
I didnt want to open a new thread so ill just continue it on this one. I am having trouble getting the primed nuke to render correctly. It renders as a regular tnt when I light it. Here is the NukePrimed Class: package blocks; import javax.annotation.Nullable; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.MoverType; import net.minecraft.entity.item.EntityTNTPrimed; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.network.datasync.DataParameter; import net.minecraft.network.datasync.DataSerializers; import net.minecraft.network.datasync.EntityDataManager; import net.minecraft.util.EnumParticleTypes; import net.minecraft.world.Explosion; import net.minecraft.world.World; public class NukePrimed extends EntityTNTPrimed { private static final DataParameter<Integer> FUSE = EntityDataManager.<Integer>createKey(EntityTNTPrimed.class, DataSerializers.VARINT); @Nullable private EntityLivingBase tntPlacedBy; /** How long the fuse is */ private int fuse; public NukePrimed(World worldIn) { super(worldIn); this.fuse = 80; this.preventEntitySpawning = true; this.setSize(0.98F, 0.98F); } public NukePrimed(World worldIn, double x, double y, double z, EntityLivingBase igniter) { this(worldIn); this.setPosition(x, y, z); float f = (float)(Math.random() * (Math.PI * 2D)); this.motionX = (double)(-((float)Math.sin((double)f)) * 0.02F); this.motionY = 0.20000000298023224D; this.motionZ = (double)(-((float)Math.cos((double)f)) * 0.02F); this.setFuse(80); this.prevPosX = x; this.prevPosY = y; this.prevPosZ = z; this.tntPlacedBy = igniter; } protected void entityInit() { this.dataManager.register(FUSE, Integer.valueOf(80)); } /** * returns if this entity triggers Block.onEntityWalking on the blocks they walk on. used for spiders and wolves to * prevent them from trampling crops */ protected boolean canTriggerWalking() { return false; } /** * Returns true if other Entities should be prevented from moving through this Entity. */ public boolean canBeCollidedWith() { return !this.isDead; } /** * Called to update the entity's position/logic. */ public void onUpdate() { this.prevPosX = this.posX; this.prevPosY = this.posY; this.prevPosZ = this.posZ; if (!this.hasNoGravity()) { this.motionY -= 0.03999999910593033D; } this.move(MoverType.SELF, this.motionX, this.motionY, this.motionZ); this.motionX *= 0.9800000190734863D; this.motionY *= 0.9800000190734863D; this.motionZ *= 0.9800000190734863D; if (this.onGround) { this.motionX *= 0.699999988079071D; this.motionZ *= 0.699999988079071D; this.motionY *= -0.5D; } --this.fuse; if (this.fuse <= 0) { this.setDead(); if (!this.world.isRemote) { this.explode(); } } else { this.handleWaterMovement(); this.world.spawnParticle(EnumParticleTypes.SMOKE_NORMAL, this.posX, this.posY + 0.5D, this.posZ, 0.0D, 0.0D, 0.0D, new int[0]); } } public Explosion newExplosion(@Nullable Entity entity, double x, double y, double z, float strength, boolean isFlaming, boolean isSmoking) { CustomExplosion explosion = new CustomExplosion(world, entity, x, y, z, strength, isFlaming, isSmoking); if (net.minecraftforge.event.ForgeEventFactory.onExplosionStart(world, explosion)) return explosion; explosion.doExplosionA(); explosion.doExplosionB(true); return explosion; } private void explode() { float f = 100.0F; this.newExplosion(this, this.posX, this.posY, this.posZ, f, false, true); } /** * (abstract) Protected helper method to write subclass entity data to NBT. */ protected void writeEntityToNBT(NBTTagCompound compound) { compound.setShort("Fuse", (short)this.getFuse()); } /** * (abstract) Protected helper method to read subclass entity data from NBT. */ protected void readEntityFromNBT(NBTTagCompound compound) { this.setFuse(compound.getShort("Fuse")); } /** * returns null or the entityliving it was placed or ignited by */ @Nullable public EntityLivingBase getTntPlacedBy() { return this.tntPlacedBy; } public float getEyeHeight() { return 0.0F; } public void setFuse(int fuseIn) { this.dataManager.set(FUSE, Integer.valueOf(fuseIn)); this.fuse = fuseIn; } public void notifyDataManagerChange(DataParameter<?> key) { if (FUSE.equals(key)) { this.fuse = this.getFuseDataManager(); } } /** * Gets the fuse from the data manager */ public int getFuseDataManager() { return ((Integer)this.dataManager.get(FUSE)).intValue(); } public int getFuse() { return this.fuse; } } Here is my NukeRenderClass: package blocks; import init.ModBlocks; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.BlockRendererDispatcher; import net.minecraft.client.renderer.GlStateManager; import net.minecraft.client.renderer.entity.RenderManager; import net.minecraft.client.renderer.entity.RenderTNTPrimed; import net.minecraft.client.renderer.texture.TextureMap; import net.minecraft.util.ResourceLocation; import net.minecraft.util.math.MathHelper; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; import noahsmod.main.MyMod; @SideOnly(Side.CLIENT) public class RenderNukePrimed extends RenderTNTPrimed { public RenderNukePrimed(RenderManager renderManagerIn) { super(renderManagerIn); this.shadowSize = 0.5F; } public void doRender(NukePrimed entity, double x, double y, double z, float entityYaw, float partialTicks) { BlockRendererDispatcher blockrendererdispatcher = Minecraft.getMinecraft().getBlockRendererDispatcher(); GlStateManager.pushMatrix(); GlStateManager.translate((float)x, (float)y + 0.5F, (float)z); if ((float)entity.getFuse() - partialTicks + 1.0F < 10.0F) { float f = 1.0F - ((float)entity.getFuse() - partialTicks + 1.0F) / 10.0F; f = MathHelper.clamp(f, 0.0F, 1.0F); f = f * f; f = f * f; float f1 = 1.0F + f * 0.3F; GlStateManager.scale(f1, f1, f1); } float f2 = (1.0F - ((float)entity.getFuse() - partialTicks + 1.0F) / 100.0F) * 0.8F; this.bindEntityTexture(entity); GlStateManager.rotate(-90.0F, 0.0F, 1.0F, 0.0F); GlStateManager.translate(-0.5F, -0.5F, 0.5F); blockrendererdispatcher.renderBlockBrightness(ModBlocks.nuke.getDefaultState(), entity.getBrightness(partialTicks)); GlStateManager.translate(0.0F, 0.0F, 1.0F); if (this.renderOutlines) { GlStateManager.enableColorMaterial(); GlStateManager.enableOutlineMode(this.getTeamColor(entity)); blockrendererdispatcher.renderBlockBrightness(ModBlocks.nuke.getDefaultState(), 1.0F); GlStateManager.disableOutlineMode(); GlStateManager.disableColorMaterial(); } else if (entity.getFuse() / 5 % 2 == 0) { GlStateManager.disableTexture2D(); GlStateManager.disableLighting(); GlStateManager.enableBlend(); GlStateManager.blendFunc(GlStateManager.SourceFactor.SRC_ALPHA, GlStateManager.DestFactor.DST_ALPHA); GlStateManager.color(1.0F, 1.0F, 1.0F, f2); GlStateManager.doPolygonOffset(-3.0F, -3.0F); GlStateManager.enablePolygonOffset(); blockrendererdispatcher.renderBlockBrightness(ModBlocks.nuke.getDefaultState(), 1.0F); GlStateManager.doPolygonOffset(0.0F, 0.0F); GlStateManager.disablePolygonOffset(); GlStateManager.color(1.0F, 1.0F, 1.0F, 1.0F); GlStateManager.disableBlend(); GlStateManager.enableLighting(); GlStateManager.enableTexture2D(); } GlStateManager.popMatrix(); this.doRender(entity, x, y, z, entityYaw, partialTicks); } protected ResourceLocation getEntityTexture(NukePrimed entity) { return TextureMap.LOCATION_BLOCKS_TEXTURE; } } Any help is appreciated, Thanks!
-
That worked thank you!
-
Here is ModBlocks class: public class ModBlocks { public static Block nuke; public static void init(){ nuke = new Nuke(); } public static void register(){ registerBlock(nuke); } private static void registerBlock(Block block) { GameRegistry.register(nuke); ItemBlock item = new ItemBlock(block); item.setRegistryName(block.getRegistryName()); GameRegistry.register(item); } public static void registerRenders(){ registerRender(nuke); } public static void registerRender(Block block){ ModelLoader.setCustomModelResourceLocation(Item.getItemFromBlock(block), 0, new ModelResourceLocation(block.getRegistryName(), "inventory")); } } Here is ClientProxy: @SideOnly(Side.CLIENT) public class ClientProxy extends CommonProxy{ @Override public void preInit(FMLPreInitializationEvent event) { super.preInit(event); } @Override public void init(FMLInitializationEvent event) { super.init(event); ModBlocks.registerRenders(); } @Override public void postInit(FMLPostInitializationEvent event) { super.postInit(event); // LootTableList.register(new ResourceLocation(MyMod.MODID, "loot_chest")); GameRegistry.registerWorldGenerator(new Creeper_Temple(), 2); } }
-
Which one
-
under registerRender public static void registerRender(Block block){ ModelLoader.setCustomModelResourceLocation(Item.getItemFromBlock(block), 0, new ModelResourceLocation(block.getRegistryName(), "inventory")); } } which I call under ClientProxy
-
Anyone?
-
Still nothing
-
like this?: ModelLoader.setCustomModelResourceLocation(Item.getItemFromBlock(block), 0, new ModelResourceLocation(block.getRegistryName(), "inventory"));
-
So this?: ModelLoader.setCustomModelResourceLocation(Item.getItemFromBlock(block), 0, new ModelResourceLocation((block.getRegistryName()), "inventory"));
-
I get the error: The constructor ResourceLocation(ResourceLocation, String) is undefined. I have: ModelLoader.setCustomModelResourceLocation(Item.getItemFromBlock(block), 0, new ModelResourceLocation(new ResourceLocation(block.getRegistryName(), "inventory"));
-
Nothing in the console, here is the riegister: ModelLoader.setCustomModelResourceLocation(Item.getItemFromBlock(block), 0, new ModelResourceLocation(new ResourceLocation(MyMod.MODID, block.getUnlocalizedName().substring(5)), "inventory"));
-
I did, same result
-
I have: { "parent": "nm:blocks/Nuke" } No errors, but still no texture
-
yes, its at: assets.nm.models.item and this is the file: { "parent": "nm:blocks/Nuke", "display": { "thirdperson": { "rotation": [ 10, -45, 170 ], "translation": [ 0, 1.5, -2.75 ], "scale": [ 0.375, 0.375, 0.375 ] } } }
-
Ok I got that and now it renders on the ground, yay!, but not in my hand or my inventory, I don't get any errors though
-
no I get: [10:16:41] [Client thread/ERROR] [FML]: Exception loading model for variant nm:nuke#explode=false for blockstate "nm:nuke[explode=false]" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model nm:nuke#explode=false with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:260) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:153) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:248) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:155) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:122) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:541) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:387) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_131] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_131] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_131] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_131] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_131] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_131] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_131] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_131] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:78) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1253) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 21 more [10:16:41] [Client thread/ERROR] [FML]: Exception loading model for variant nm:nuke#explode=true for blockstate "nm:nuke[explode=true]" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model nm:nuke#explode=true with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:260) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:153) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:248) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:155) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:122) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:541) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:387) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_131] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_131] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_131] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_131] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_131] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_131] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_131] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_131] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:78) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1253) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 21 more
-
ok now I have: { "variants": { "inventory": { "model": "nm:nuke" } } }
-
Whats wrong with this?: { "variants": { "normal": [ { "model": "nm:nuke" }, { "model": "nm:nuke", "y": 90 }, { "model": "nm:nuke", "y": 180 }, { "model": "nm:nuke", "y": 270 } ] } }