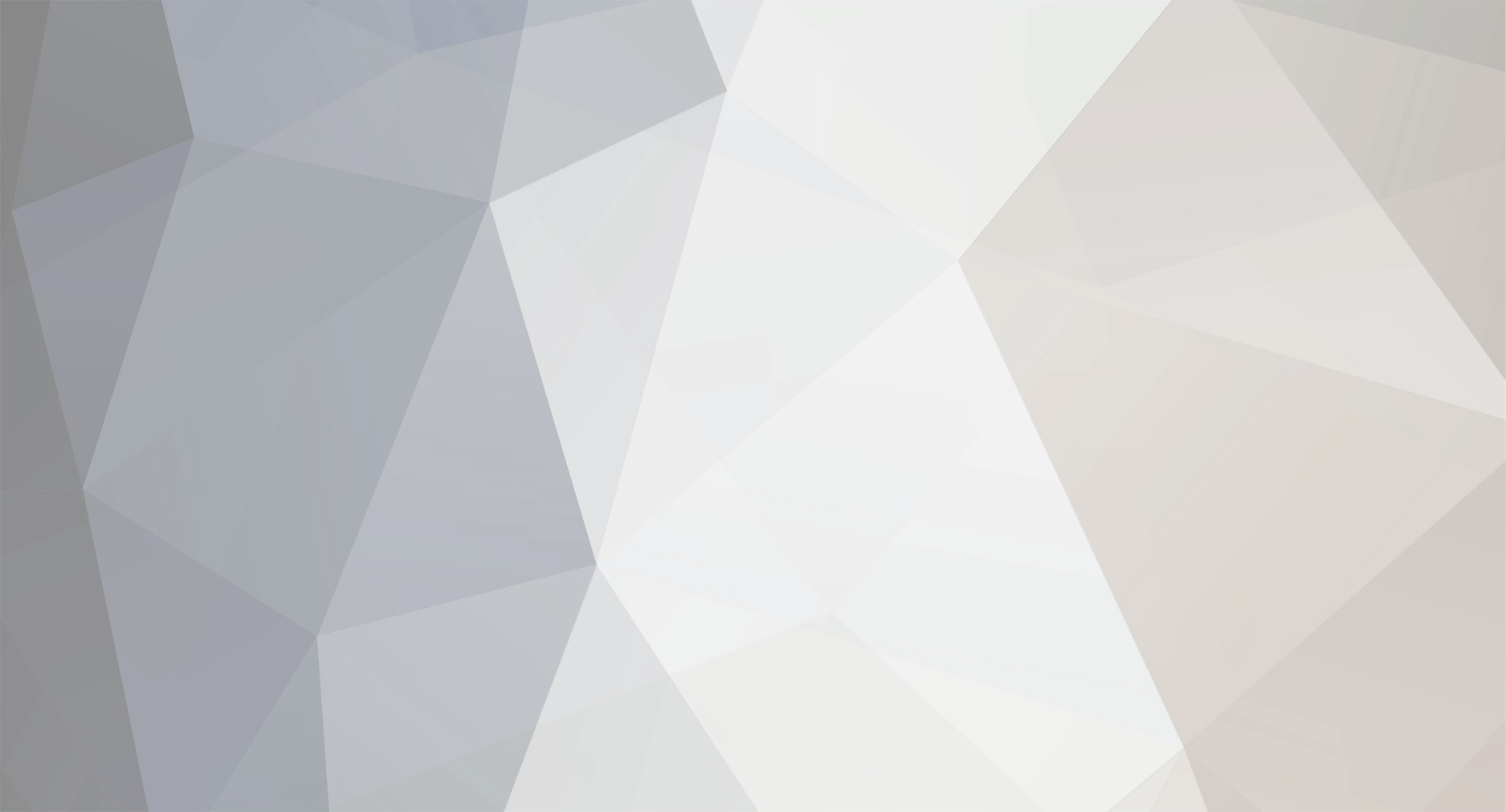
fuzzybat23
Members-
Posts
131 -
Joined
-
Last visited
Everything posted by fuzzybat23
-
I'm not sure if this is possible with the annotation system, but I'm trying to set up a button on the config page that has multiple states. Initially it will read "Break Animation: NONE" Click on it and it will change to "Break Animation: Shrink", then "Break Animation: Down", then "Break Animation: Alpha" and if clicked a fifth time, it reverts back to the initial state of "Break Animation: None" Each state, of course, changing an integer value within the config. NONE = 0 SHRINK = 1 DOWN = 2 ALPHA = 3 Anyone have any ideas how to go about this?
-
Yeah, I realized that about the booleans and fixed it. So now when Minecraft starts up, it creates the config file. I can go into the config screen from the mod options and there are the two buttons, Client and Client2. They have the data entry fields that can be changed. But I noticed a new problem. Every time the game runs, it creates a brand new config file. Also, and I checked this by opening the cfg file before and after changing the fields from the config screen in game, this isn't saving anything new to the config file. If I click on foobar to change it from false to true and click done, it should save csbr.cfg with foobar now obeing true, and it doesn't. Everything I have for my ModConfig.java is here. package com.fuzzybat23.csbr.config; import com.fuzzybat23.csbr.CSBR; import net.minecraftforge.common.config.Config; import net.minecraftforge.common.config.ConfigManager; import net.minecraftforge.fml.client.event.ConfigChangedEvent; import net.minecraftforge.fml.common.Mod; @Config(modid = CSBR.MODID) public class ModConfig { @Config.Comment("Button 1") public static final Client client = new Client(); public static class Client { @Config.Comment("Input field 1") public int var1 = 1; @Config.Comment("Input field 2") public boolean foobar = false; } @Config.Comment("Button 2") public static final Client2 client2 = new Client2(); public static class Client2 { @Config.Comment("Input field 1") public int var1 = 1; @Config.Comment("Input field 2") public boolean foobar = false; } @Mod.EventBusSubscriber(modid = CSBR.MODID) private static class Handler { public static void onConfigChanged(ConfigChangedEvent.OnConfigChangedEvent event) { if (event.getModID().equals(CSBR.MODID)) { ConfigManager.sync(CSBR.MODID, Config.Type.INSTANCE); } } } } I figure that I need to run a check to see if there is a cfg for my mod first, which I believe would use if(ConfigManager.hasConfigForMod(CSBR.MODID)) But I'm not sure exactly where and how to put that in there. On one side of the if else, it'd load the default values creating the cfg. on the other side, it'd use ConfigManager.loadData(data) where data is... of the type ASMDataTable, whatever that is?
-
Now that I have a working config file and config screen, how exactly do you access the values set in the cfg file? Like.. say I'm in a method in my main class, do I call ModConfigClass.Client.var1 or ModConfigClass.Client2.foobar
-
Never mind, I figured it out @Config(modid=YourClass.MODID) public class ModConfigClass { @Config.Comment("Button 1") public static final Client client = new Client(); public static class Client { @Config.Comment("Input field 1") public int var1 = 1; @Config.Comment("Input field 2") public static boolean foobar = false; } @Config.Comment("Button 2") public static final Client2 client2 = new Client2(); public static class Client2 { @Config.Comment("Input field 1") public int var1 = 1; @Config.Comment("Input field 2") public static boolean foobar = false; } @Mod.EventBusSubscriber(modid = YourClass.MODID) private static class Handler { public static void onConfigChanged(ConfigChangedEvent.OnConfigChangedEvent event) { if (event.getModID().equals(YourClass.MODID)) { ConfigManager.sync(YourClass.MODID, Config.Type.INSTANCE); } } } }
-
Ok.. so I did this with the two GenSettings. That put the initial worldGen button leading to two oreAether buttons. public static class Generation { @Config.Comment("Aether Ore generation settings") public final GenSettings oreAether = new GenSettings(1, 80, 128, 4,-1, 1); @Config.Comment("Aether Ore generation settings -- 2") public final GenSettings2 oreAether2 = new GenSettings2(1, 80, 128, 4,-1, 1); } Basically I'm trying to get it to the point where there's x number of buttons on the initial config screen. Click the button and it goes straight to the data entry, not to more buttons leading to the data entry
-
Well, almost anyway I did this: package com.fuzzybat23.csbr.config; import com.fuzzybat23.csbr.CSBR; import net.minecraftforge.common.config.Config; import net.minecraftforge.common.config.ConfigManager; import net.minecraftforge.fml.client.event.ConfigChangedEvent; import net.minecraftforge.fml.common.Mod; /** * Created by V0idWa1k3r on 31-May-17. */ @Config(modid = CSBR.MODID) public class ModConfig { @Config.Comment("Configure AetherWorks's worldgen here") public static final Generation worldGen = new Generation(); public static class Generation { @Config.Comment("Aether Ore generation settings") public final GenSettings oreAether = new GenSettings(1, 80, 128, 4,-1, 1); } public static class GenSettings { @Config.Comment("The amount of times the ore will try to generate in each chunk. Set to less than 1 to turn this into a chance to generate type of value") public float triesPerChunk; @Config.Comment("Minimum Y coordinate for this ore") public int minHeight; @Config.Comment("Maximum Y coordinate for this ore") public int maxHeight; @Config.Comment("The maximum size of the vein") public int veinSize; @Config.Comment("The list of dimension IDs this ore is NOT allowed to generate in") public int[] blacklistDimensions; GenSettings(float triesPerChunk, int minHeight, int maxHeight, int veinSize, int... blacklistDimensions) { this.triesPerChunk = triesPerChunk; this.minHeight = minHeight; this.maxHeight = maxHeight; this.veinSize = veinSize; this.blacklistDimensions = blacklistDimensions; } } @Config.Comment("Configure AetherWorks's worldgen here -- 2") public static final Generation2 worldGen2 = new Generation2(); public static class Generation2 { @Config.Comment("Aether Ore generation settings -- 2") public final GenSettings2 oreAether2 = new GenSettings2(1, 80, 128, 4,-1, 1); } public static class GenSettings2 { @Config.Comment("The amount of times the ore will try to generate in each chunk. Set to less than 1 to turn this into a chance to generate type of value -- 2") public float triesPerChunk2; @Config.Comment("Minimum Y coordinate for this ore -- 2") public int minHeight2; @Config.Comment("Maximum Y coordinate for this ore -- 2") public int maxHeight2; @Config.Comment("The maximum size of the vein -- 2") public int veinSize2; @Config.Comment("The list of dimension IDs this ore is NOT allowed to generate in -- 2") public int[] blacklistDimensions2; GenSettings2(float triesPerChunk2, int minHeight2, int maxHeight2, int veinSize2, int... blacklistDimensions2) { this.triesPerChunk2 = triesPerChunk2; this.minHeight2 = minHeight2; this.maxHeight2 = maxHeight2; this.veinSize2 = veinSize2; this.blacklistDimensions2 = blacklistDimensions2; } } @Mod.EventBusSubscriber(modid = CSBR.MODID) private static class Handler { public static void onConfigChanged(ConfigChangedEvent.OnConfigChangedEvent event) { if (event.getModID().equals(CSBR.MODID)) { ConfigManager.sync(CSBR.MODID, Config.Type.INSTANCE); } } } } Which leads to this in game. worldgen opens oreAether which opens a first set of settings. Then worldgen2 opens oreAether2 which opens the second set of settings. How do I get it so there's the two buttons on the main config screen, click on one and it opens directly to the settings?
-
Damn I was stressing over this part of my mod. Turns out it was actually the easiest part of the whole thing Even figured out the two different pages of settings trick. Thanks guys :>
-
Oh crap, didn't notice that. My brain thinks in first in last out
-
Ahhhh, I think I see. Int... blacklistDimensions ended up with -1, and 1 because they were the only two left and blacklistDimensions is an array, right? I wonder, though, why those values were placed in the order they were in your config file then I'd think that triesPerChunk would be at the top of the list, instead blacklistDimensions is. I'd say that it entered them in reverse order from how they're declared in your code, except that veinSize ends up being the last one entered into the config file.
-
So when you did: @Config.Comment("Minimum Y coordinate for this ore") public int minHeight; It created an entry in the config file with minHeight but with no value set, where if you did: @Config.Comment("Minimum Y coordinate for this ore") public int minHeight = 20; then it would have created the config file with minHeight = 20?
-
So this created the gui for you. How did you create your config file or did this automatically do that, too. And if so, where does it get the default values for the file?
-
Easy or not, I wish someone would make an actual tutorial for it on youtube.
-
I'm working with 1.12. I guess 1.12 doesn't utilize GuiFActory at all anymore, just the @Config?
-
package com.mmyzd.llor; import java.io.File; import java.util.ArrayList; import net.minecraftforge.common.config.Configuration; import net.minecraftforge.common.config.Property; import net.minecraftforge.fml.client.event.ConfigChangedEvent; import net.minecraftforge.fml.common.eventhandler.SubscribeEvent; public class ConfigManager { public Configuration file; public Property useSkyLight; public Property displayMode; public Property chunkRadius; public Property pollingInterval; public ArrayList<String> displayModeName = new ArrayList<String>(); public ArrayList<String> displayModeDesc = new ArrayList<String>(); public ConfigManager(File configDir) { file = new Configuration(new File(configDir, "LLOverlayReloaded.cfg")); displayModeName.add("Standard"); displayModeName.add("Advanced"); displayModeName.add("Minimal"); displayModeDesc.add("Show green (safe) and red (spawnable) areas."); displayModeDesc.add("Show green (safe), red (always spawnable) and yellow (currently safe, but will be spawnable at night) areas."); displayModeDesc.add("Do not show green area. For other areas, use standard mode when not counting sky light, or advanced mode otherwise."); reload(); } @SubscribeEvent public void onConfigurationChanged(ConfigChangedEvent.OnConfigChangedEvent event) { if (event.getModID().equals(LightLevelOverlayReloaded.MODID)) update(); } void reload() { file.load(); String comment = "How to display numbers? (default: 0)"; for (int i = 0; i < displayModeName.size(); i++) { comment += "\n" + String.valueOf(i) + " - "; comment += displayModeName.get(i) + ": "; comment += displayModeDesc.get(i); } useSkyLight = file.get("general", "useSkyLight", false, "If set to true, the sunlight/moonlight will be counted in light level. (default: false)"); displayMode = file.get("general", "displayMode", 0, comment); chunkRadius = file.get("general", "chunkRadius", 3, "The distance (in chunks) of rendering radius. (default: 3)"); pollingInterval = file.get("general", "pollingInterval", 200, "The update interval (in milliseconds) of light level. Farther chunks update less frequently. (default: 200)"); update(); } public void update() { useSkyLight.set(useSkyLight.getBoolean(false)); displayMode.set(Math.min(Math.max(displayMode.getInt(0), 0), displayModeName.size() - 1)); chunkRadius.set(Math.min(Math.max(chunkRadius.getInt(3), 1), 9)); pollingInterval.set(Math.min(Math.max(pollingInterval.getInt(200), 10), 60000)); file.save(); } }
-
Which file in your Git did you initially create and populate the configuration file that your ModConfig.java syncs to? I'd like to compare it to mine. I think I'm slowly getting a grasp on the usage of @Config I'm also wondering how in your main class you initially access your ModConfig to open the Config screen. None of the examples I've seen so far were using @Config, so the structure is quite a bit different. Like.. This one for example, is some code I'm trying to convert to make use of @Config.
-
I don't think I quite understand. I'm still learning this stuff, so I'm not up to snuff on all the terms What exactly do you mean by the fields the classes are mapped to?
-
Let me see if my sleep addled brain got that right. Each time you declared a new public class in your example, that opened up a new category page?
-
I think I see, and I'm not even fully awake yet This is the best and simplest example I've seen yet. You don't know how to setup multiple pages in the Config screen, do you?
-
My main class is: package com.fuzzybat23.csbr; import com.fuzzybat23.csbr.config.ConfigManager; import net.minecraftforge.common.MinecraftForge; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; import java.io.File; @net.minecraftforge.fml.common.Mod(modid = "csbr", version = "1.0", clientSideOnly = true, acceptedMinecraftVersions = "[1.12, 1.13)", guiFactory = "com.fuzzybat23.csbr.config.GuiFactory") public class CSBR { @net.minecraftforge.fml.common.Mod.Instance("CSBR") public static final String MODID = "csbr"; public static CSBR instance; public ConfigManager config; public CSBR() { } @Mod.EventHandler @SideOnly(Side.CLIENT) public void preInit(FMLPreInitializationEvent event) { config = new ConfigManager(event.getModConfigurationDirectory()); } @Mod.EventHandler @SideOnly(Side.CLIENT) public void initialize(FMLInitializationEvent event) { MinecraftForge.EVENT_BUS.register(this); MinecraftForge.EVENT_BUS.register(config); } @Mod.EventHandler @SideOnly(Side.CLIENT) public void load(FMLInitializationEvent event) { } } I can't figure out where that null is coming from
-
Oh, duh 1.12
-
Well, it compiles just fine. I ran the other mod that has the original code and it's config screen opens just fine. Mine doesn't crash when I click on the Config button,but it does throw this: [19:35:13] [main/ERROR] [FML]: There was a critical issue trying to build the config GUI for csbr java.lang.NullPointerException: null at com.fuzzybat23.csbr.config.GuiFactory$ConfigGui.<init>(GuiFactory.java:47) ~[GuiFactory$ConfigGui.class:?] at com.fuzzybat23.csbr.config.GuiFactory.createConfigGui(GuiFactory.java:40) ~[GuiFactory.class:?] at net.minecraftforge.fml.client.GuiModList.actionPerformed(GuiModList.java:301) [GuiModList.class:?] at net.minecraft.client.gui.GuiScreen.mouseClicked(GuiScreen.java:494) [GuiScreen.class:?] at net.minecraftforge.fml.client.GuiModList.mouseClicked(GuiModList.java:205) [GuiModList.class:?] at net.minecraft.client.gui.GuiScreen.handleMouseInput(GuiScreen.java:611) [GuiScreen.class:?] at net.minecraftforge.fml.client.GuiModList.handleMouseInput(GuiModList.java:351) [GuiModList.class:?] at net.minecraft.client.gui.GuiScreen.handleInput(GuiScreen.java:576) [GuiScreen.class:?] at net.minecraft.client.Minecraft.runTick(Minecraft.java:1861) [Minecraft.class:?] at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1171) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:436) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_131] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_131] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_131] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_131] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_131] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_131] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_131] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_131] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?]
-
Actually, mainConfigGuiClass wasn't deleted, it was renamed. It's still in IModGuiFactory, but as createConfigGui. The old -> public Class<? extends GuiScreen> mainConfigGuiClass(); /** * Return a list of the "runtime" categories this mod wishes to populate with * GUI elements. * * Runtime categories are created on demand and organized in a 'lite' tree format. * The parent represents the parent node in the tree. There is one special parent * 'Help' that will always list first, and is generally meant to provide Help type * content for mods. The remaining parents will sort alphabetically, though * this may change if there is a lot of alphabetic abuse. "AAA" is probably never a valid * category parent. * * Runtime configuration itself falls into two flavours: in-game help, which is * generally non interactive except for the text it wishes to show, and client-only * affecting behaviours. This would include things like toggling minimaps, or cheat modes * or anything NOT affecting the behaviour of the server. Please don't abuse this to * change the state of the server in any way, this is intended to behave identically * when the server is local or remote. * * @return the set of options this mod wishes to have available, or empty if none */ The New -> public GuiScreen createConfigGui(GuiScreen parentScreen); /** * Return a list of the "runtime" categories this mod wishes to populate with * GUI elements. * * Runtime categories are created on demand and organized in a 'lite' tree format. * The parent represents the parent node in the tree. There is one special parent * 'Help' that will always list first, and is generally meant to provide Help type * content for mods. The remaining parents will sort alphabetically, though * this may change if there is a lot of alphabetic abuse. "AAA" is probably never a valid * category parent. * * Runtime configuration itself falls into two flavours: in-game help, which is * generally non interactive except for the text it wishes to show, and client-only * affecting behaviours. This would include things like toggling minimaps, or cheat modes * or anything NOT affecting the behaviour of the server. Please don't abuse this to * change the state of the server in any way, this is intended to behave identically * when the server is local or remote. * * @return the set of options this mod wishes to have available, or empty if none */ So get rid of the runtime handler completely, eh? Well, as for the other one which was renamed, not deleted, I redid the code as: @Override public Class<? extends GuiScreen> createConfigGui() { return ConfigGui.class; } It throws the same error though. Should I just erase that line, as well, and delete the GuiModConfig.java file and add it directly into the GuiFactory? package com.fuzzybat23.csbr.config; import java.util.Set; import com.fuzzybat23.csbr.CSBR; import net.minecraft.client.Minecraft; import net.minecraft.client.gui.GuiScreen; import net.minecraftforge.common.config.ConfigElement; import net.minecraftforge.common.config.Configuration; import net.minecraftforge.fml.client.IModGuiFactory; import net.minecraftforge.fml.client.config.GuiConfig; public class GuiFactory implements IModGuiFactory { @Override public void initialize(Minecraft minecraftInstance) { } // @Override // public Class<? extends GuiScreen> createConfigGui() // { // return ConfigGui.class; // } @Override public Set<RuntimeOptionCategoryElement> runtimeGuiCategories() { return null; } @Override public boolean hasConfigGui() { return true; } @Override public GuiScreen createConfigGui(GuiScreen parentScreen) { return new ConfigGui(parentScreen); } public static class ConfigGui extends GuiConfig { public ConfigGui(GuiScreen parent) { super(parent, new ConfigElement(CSBR.instance.config.file.getCategory(Configuration.CATEGORY_GENERAL)).getChildElements(), CSBR.MODID, false, false, GuiConfig.getAbridgedConfigPath(CSBR.instance.config.file.toString())); } } }
-
This snippet of code came from a 1.11 mod I'm trying to use to create a gui config. I think maybe the code was changed between 1.11 and 1.12 as it's telling me it can't me resolved. package com.fuzzybat23.csbr.config; import java.util.Set; import net.minecraft.client.Minecraft; import net.minecraft.client.gui.GuiScreen; import net.minecraftforge.fml.client.IModGuiFactory; public class GuiFactory implements IModGuiFactory { @Override public void initialize(Minecraft minecraftInstance) { } @Override <---- Error 1 public Class<? extends GuiScreen> mainConfigGuiClass() { return GuiModConfig.class; } @Override <---- Error 2 public Set<RuntimeOptionCategoryElement> runtimeGuiCategories() <---- Error 3 { return null; } @Override public RuntimeOptionGuiHandler getHandlerFor(RuntimeOptionCategoryElement element) { return null; } @Override public boolean hasConfigGui() { return true; } @Override public GuiScreen createConfigGui(GuiScreen parentScreen) { return new GuiModConfig(parentScreen); } } Error 1 and Error 2 say "Method does not override method from its superclass." Error 3 is in reference to RuntimeOptionGuiHandler and says "Cannot resolve symbol 'RuntimeOptionGuiHandler' Any one know if this has been changed? This GuiFactory is a direct copy of another person's code that does compile, as far as I know, as his mod has been released with no errors. mainConfigGuiClasss refers to net.minecraftforge.fml.client.config.GuiConfig.java which reads: public GuiConfig(GuiScreen parentScreen, String modID, boolean allRequireWorldRestart, boolean allRequireMcRestart, String title, Class<?>... configClasses) { this(parentScreen, collectConfigElements(configClasses), modID, null, allRequireWorldRestart, allRequireMcRestart, title, null); } and the new GuiModConfig.java in my mod directory reads: package com.fuzzybat23.csbr.config; import com.fuzzybat23.csbr.CSBR; import net.minecraft.client.gui.GuiScreen; import net.minecraftforge.common.config.ConfigElement; import net.minecraftforge.fml.client.config.GuiConfig; public class GuiModConfig extends GuiConfig { public GuiModConfig(GuiScreen parent) { super( parent, new ConfigElement(CSBR.instance.config.file.getCategory("general")).getChildElements(), CSBR.MODID, false, false, GuiConfig.getAbridgedConfigPath(CSBR.instance.config.file.toString()) ); } }
-
I don't suppose anyone knows of any good tutorials on how to build a config gui in Forge? You know, when you go into the main menu, click on mods, scroll down to yours and click on Config button. One that's preferably in english? It'd be nice if Forge put out a template for those.
-
[1.12]Full Screen / Fixed Gui Elements
fuzzybat23 replied to ArmamentHaki's topic in User Submitted Tutorials
Where exactly do you put your png in the file structure, though? Like, I'm going to assume you're using eclipse? Would it go in src/main/resources, where mcmod.info is located?