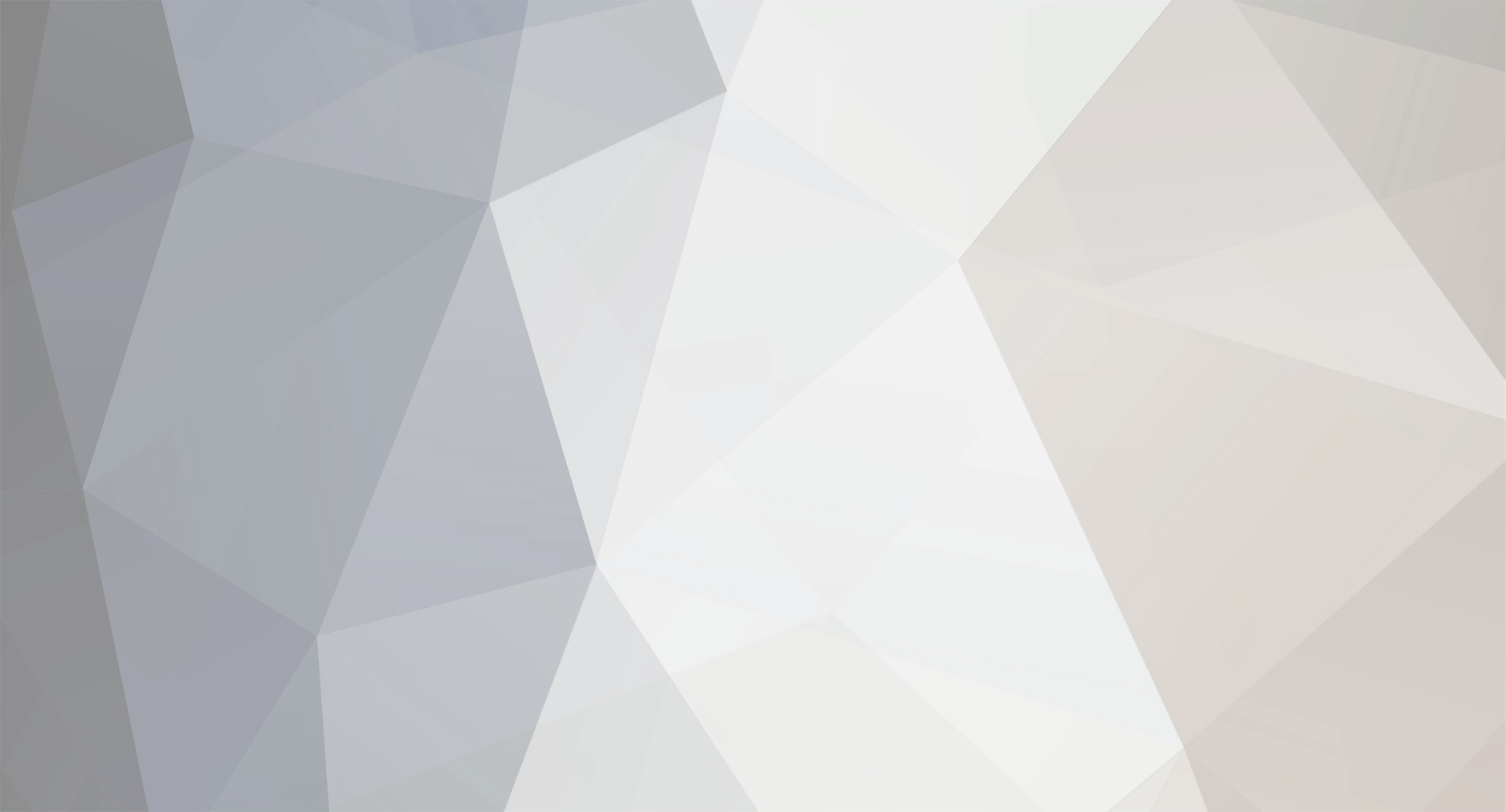
fuzzybat23
Members-
Posts
131 -
Joined
-
Last visited
Everything posted by fuzzybat23
-
I'm doing pretty well learning as I go. I' ma fast learner when it comes to code. Either way I figured it out on my own. I should've been using this all along: HashMap<Integer, DestroyBlockProgress> damagedBlock = (HashMap<Integer, DestroyBlockProgress>) f.get(Minecraft.getMinecraft().renderGlobal); I had the wrong library imported, using Map instead of Hashmap
-
You have to forgive me, I'm kinda learning this as I go, crash course Crash being the keyword here. Heh, anyway.. local variables, So I'd need to declare final static Field f; in my main class, then? public class whatever { final static Field f; private static float getBlockDamage() { try { f = RenderGlobal.class.getDeclaredField("damagedBlocks"); } catch (NoSuchFieldException e) { f = RenderGlobal.class.getDeclaredField("field_72738_E"); } } }
-
I think I may have found the problem, but I'm not sure how to fix it. The version 1.8 code looked like this -> HashMap<Integer, DestroyBlockProgress> map = (HashMap<Integer, DestroyBlockProgress>) f.get(Minecraft.getMinecraft().renderGlobal); I split that into two lines like this when I was rewriting it -> Map<Integer, DestroyBlockProgress> damagedBlocks = Maps.<Integer, DestroyBlockProgress>newHashMap(); f.get(Minecraft.getMinecraft().renderGlobal); I tried combining the two lines like in the original code, but that only threw an error. Map<Integer, DestroyBlockProgress> damagedBlocks = Maps.<Integer, DestroyBlockProgress>newHashMap() f.get(Minecraft.getMinecraft().renderGlobal); Syntax error, insert ";" to complete LocalVariableDeclarationStatement
-
Nope, adding static and final to Field just threw a bunch of errors in my face. static final Field f; <-- Illegal modifier for the variable f; only final is permitted final Field f; try { f = RenderGlobal.class.getDeclaredField("damagedBlocks"); } catch (NoSuchFieldException e) { f = RenderGlobal.class.getDeclaredField("field_72738_E"); <-- The final local variable f may already have been assigned } Though.. with a try, you can't load both the first value and the exception value, right? It's a one or the other, like an If else clause.
-
So instead of Field f; it should read static final Field f; That sound about right?
-
I've even tried this, and it still doesn't seem to return the block damage progress. private static float getBlockDamage(EntityPlayer player, RayTraceResult rtr) { try { Field f; try { f = RenderGlobal.class.getDeclaredField("damagedBlocks"); } catch (NoSuchFieldException e) { f = RenderGlobal.class.getDeclaredField("field_72738_E"); } f.setAccessible(true); Map<Integer, DestroyBlockProgress> damagedBlocks = Maps.<Integer, DestroyBlockProgress>newHashMap(); f.get(Minecraft.getMinecraft().renderGlobal); for (Entry<Integer, DestroyBlockProgress> entry : damagedBlocks.entrySet()) { DestroyBlockProgress destroyblockprogress = entry.getValue(); if (destroyblockprogress.getPosition().equals(rtr.getBlockPos())) { if (destroyblockprogress.getPartialBlockDamage() >= 0 && destroyblockprogress.getPartialBlockDamage() <= 10) { return destroyblockprogress.getPartialBlockDamage() / 10.0F; } } } } catch (Exception e) { e.printStackTrace(); } return 0.0F; }
-
I'm working on a mod that draws the selection box around the targeted block with thicker lines and or multiple colors. That part I have working fine. Now I'm trying to make it shrink the selection box in tandem with how much damage the block is taking. To do that, I'm using code like this: AxisAlignedBB aabb = iblockstate.getSelectedBoundingBox(player.getEntityWorld(), blockpos1).grow(0.0020000000949949026D).offset(-d0, -d1, -d2); float breakProgress = getBlockDamage(player, d0, d1, d2); if(breakAnimation == SHRINK) aabb = aabb.expand(-breakProgress / 2, -breakProgress / 2, -breakProgress / 2); The snag I've hit his how exactly to retrieve the break progress of the targeted block. Does anyone have any ideas how to do this? I've tried adapting the code from RenderGlobal that governs drawing the current break icon on the block being broken, but after loading it up in game, it doesn't seem to do anything at all. Here's what I have so far. package com.fuzzybat23.csbr; import com.fuzzybat23.csbr.proxy.CommonProxy; import com.google.common.collect.Lists; import com.google.common.collect.Maps; import com.google.common.collect.Queues; import com.google.common.collect.Sets; import com.google.gson.JsonSyntaxException; import java.io.IOException; import java.util.Collection; import java.util.Iterator; import java.util.List; import java.util.Map; import java.util.Queue; import java.util.Random; import java.util.Set; import javax.annotation.Nullable; import net.minecraft.block.Block; import net.minecraft.block.BlockChest; import net.minecraft.block.BlockEnderChest; import net.minecraft.block.BlockSign; import net.minecraft.block.BlockSkull; import net.minecraft.block.SoundType; import net.minecraft.block.material.Material; import net.minecraft.block.state.IBlockState; import net.minecraft.client.Minecraft; import net.minecraft.client.audio.ISound; import net.minecraft.client.audio.PositionedSoundRecord; import net.minecraft.client.multiplayer.WorldClient; import net.minecraft.client.particle.Particle; import net.minecraft.client.renderer.ActiveRenderInfo; import net.minecraft.client.renderer.BlockRendererDispatcher; import net.minecraft.client.renderer.BufferBuilder; import net.minecraft.client.renderer.DestroyBlockProgress; import net.minecraft.client.renderer.GlStateManager; import net.minecraft.client.renderer.Tessellator; import net.minecraft.client.renderer.chunk.ChunkRenderDispatcher; import net.minecraft.client.renderer.chunk.CompiledChunk; import net.minecraft.client.renderer.chunk.IRenderChunkFactory; import net.minecraft.client.renderer.chunk.ListChunkFactory; import net.minecraft.client.renderer.chunk.RenderChunk; import net.minecraft.client.renderer.chunk.VboChunkFactory; import net.minecraft.client.renderer.chunk.VisGraph; import net.minecraft.client.renderer.culling.ClippingHelper; import net.minecraft.client.renderer.culling.ClippingHelperImpl; import net.minecraft.client.renderer.culling.Frustum; import net.minecraft.client.renderer.culling.ICamera; import net.minecraft.client.renderer.entity.RenderManager; import net.minecraft.client.renderer.texture.TextureAtlasSprite; import net.minecraft.client.renderer.texture.TextureManager; import net.minecraft.client.renderer.texture.TextureMap; import net.minecraft.client.renderer.tileentity.TileEntityRendererDispatcher; import net.minecraft.client.renderer.vertex.DefaultVertexFormats; import net.minecraft.client.renderer.vertex.VertexBuffer; import net.minecraft.client.renderer.vertex.VertexFormat; import net.minecraft.client.renderer.vertex.VertexFormatElement; import net.minecraft.client.resources.IResourceManager; import net.minecraft.client.resources.IResourceManagerReloadListener; import net.minecraft.client.shader.Framebuffer; import net.minecraft.client.shader.ShaderGroup; import net.minecraft.client.shader.ShaderLinkHelper; import net.minecraft.crash.CrashReport; import net.minecraft.crash.CrashReportCategory; import net.minecraft.crash.ICrashReportDetail; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.init.SoundEvents; import net.minecraft.item.Item; import net.minecraft.item.ItemDye; import net.minecraft.item.ItemRecord; import net.minecraft.tileentity.TileEntity; import net.minecraft.tileentity.TileEntityChest; import net.minecraft.util.BlockRenderLayer; import net.minecraft.util.ClassInheritanceMultiMap; import net.minecraft.util.EnumFacing; import net.minecraft.util.EnumParticleTypes; import net.minecraft.util.ReportedException; import net.minecraft.util.ResourceLocation; import net.minecraft.util.SoundCategory; import net.minecraft.util.SoundEvent; import net.minecraft.util.math.AxisAlignedBB; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.MathHelper; import net.minecraft.util.math.RayTraceResult; import net.minecraft.util.math.Vec3d; import net.minecraft.world.IBlockAccess; import net.minecraft.world.IWorldEventListener; import net.minecraft.world.World; import net.minecraft.world.border.WorldBorder; import net.minecraft.world.chunk.Chunk; import net.minecraftforge.client.event.DrawBlockHighlightEvent; import net.minecraftforge.client.event.MouseEvent; import net.minecraftforge.common.MinecraftForge; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.Mod.Instance; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.common.eventhandler.SubscribeEvent; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; import org.apache.logging.log4j.LogManager; import org.apache.logging.log4j.Logger; import org.lwjgl.opengl.GL11; import org.lwjgl.util.vector.Vector3f; import org.lwjgl.util.vector.Vector4f; @Mod(modid=Reference.Mod_ID, name=Reference.NAME, version=Reference.VERSION, acceptedMinecraftVersions=Reference.ACCEPTED_VERSIONS) public class CSBR { //public class RenderGlobal implements IWorldEventListener, IResourceManagerReloadListener { private static final Logger LOGGER = LogManager.getLogger(); private final Minecraft mc = Minecraft.getMinecraft(); private final Map<Integer, DestroyBlockProgress> damagedBlocks = Maps.<Integer, DestroyBlockProgress>newHashMap(); public static float r = 0.0F; // red public static float g = 0.0F; // green public static float b = 0.0F; // blue public static float a = 0.0F; // alpha public static float br = 0.0F; // blink red public static float bg = 0.0F; // blink green public static float bb = 0.0F; // blink blue public static float ba = 0.0F; // blink alpha public static float bs = 0.0F; // blink speed public static float w = 2.0F; // line width public static int breakAnimation; public static int NONE = 0; public static int SHRINK = 1; public static int DOWN = 2; public static int ALPHA = 3; public static int LASTANIMATION_INDEX = 3; public static boolean disableDepthBuffer; @Instance public static CSBR instance; @SidedProxy(clientSide = Reference.CLIENT_PROXY_CLASS, serverSide = Reference.CLIENT_SERVER_CLASS) public static CommonProxy proxy; @EventHandler public void preInit(FMLPreInitializationEvent event) { proxy.registerTickHandler(); MinecraftForge.EVENT_BUS.register(this); r = 1.0F; a = 0.30F; br = 0.0F; bg = 1.0F; ba = 0.35F; bs = 0.5F; w = 4.0F; breakAnimation = 1; disableDepthBuffer=false; } @EventHandler public void Init(FMLInitializationEvent event) { } @EventHandler public void PostInit(FMLPostInitializationEvent event) { } @SubscribeEvent public void onDrawBlockSelectionBox(DrawBlockHighlightEvent e) { drawSelectionBox(e.getPlayer(), e.getTarget(), e.getSubID(), e.getPartialTicks()); e.setCanceled(true); } /** * Draws the selection box for the player. */ public void drawSelectionBox(EntityPlayer player, RayTraceResult movingObjectPositionIn, int execute, float partialTicks) { if (execute == 0 && movingObjectPositionIn.typeOfHit == RayTraceResult.Type.BLOCK) { if(disableDepthBuffer) { GL11.glDisable(GL11.GL_DEPTH_TEST); } GlStateManager.enableBlend(); GlStateManager.tryBlendFuncSeparate(GlStateManager.SourceFactor.SRC_ALPHA, GlStateManager.DestFactor.ONE_MINUS_SRC_ALPHA, GlStateManager.SourceFactor.ONE, GlStateManager.DestFactor.ZERO); GlStateManager.glLineWidth(w); GlStateManager.disableTexture2D(); GlStateManager.depthMask(false); if (this.mc.objectMouseOver != null && this.mc.objectMouseOver.typeOfHit == RayTraceResult.Type.BLOCK && this.mc.objectMouseOver.getBlockPos() != null ) { BlockPos blockpos1 = this.mc.objectMouseOver.getBlockPos(); IBlockState iblockstate = player.getEntityWorld().getBlockState(blockpos1); double d0 = player.lastTickPosX + (player.posX - player.lastTickPosX) * (double)partialTicks; double d1 = player.lastTickPosY + (player.posY - player.lastTickPosY) * (double)partialTicks; double d2 = player.lastTickPosZ + (player.posZ - player.lastTickPosZ) * (double)partialTicks; AxisAlignedBB aabb = iblockstate.getSelectedBoundingBox(player.getEntityWorld(), blockpos1).grow(0.0020000000949949026D).offset(-d0, -d1, -d2); float breakProgress = getBlockDamage(player, d0, d1, d2); if(breakAnimation == DOWN) aabb = aabb.expand(0.0F, -breakProgress / 2, 0.0F); if(breakAnimation == SHRINK) aabb = aabb.expand(-breakProgress / 2, -breakProgress / 2, -breakProgress / 2); //Draw Boxes drawSelectionBlinkingBox(aabb, br, bg, bb, ba); drawSelectionBoundingBox(aabb, r, g, b, a); } GL11.glEnable(GL11.GL_DEPTH_TEST); GlStateManager.depthMask(true); GlStateManager.enableTexture2D(); GlStateManager.disableBlend(); } } // Draw Block Overlay Mask public static void drawSelectionBlinkingBox(AxisAlignedBB box, float red, float green, float blue, float alpha) { drawMask(box.minX, box.minY, box.minZ, box.maxX, box.maxY, box.maxZ, red, green, blue, alpha); } public static void drawMask(double minX, double minY, double minZ, double maxX, double maxY, double maxZ, float red, float green, float blue, float alpha) { Tessellator t = Tessellator.getInstance(); BufferBuilder b1 = t.getBuffer(); b1.begin(7, DefaultVertexFormats.POSITION_COLOR); if(alpha > 0.0F) { alpha *= (float) Math.abs(Math.sin(Minecraft.getSystemTime() / 100.0D * bs)); drawMask(b1, minX, minY, minZ, maxX, maxY, maxZ, red, green, blue, alpha); t.draw(); } } public static void drawMask(BufferBuilder b1, double minX, double minY, double minZ, double maxX, double maxY, double maxZ, float red, float green, float blue, float alpha) { //up b1.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b1.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); //b1.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); //down b1.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(minX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, maxY, minZ).color(red, green, blue, alpha).endVertex(); //b1.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); //north b1.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); //b1.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); //south b1.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b1.pos(minX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); //b1.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); //east b1.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); //b1.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); //west b1.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b1.pos(minX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b1.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); //b1.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); } // Draw Selection Bounding Box public static void drawSelectionBoundingBox(AxisAlignedBB box, float red, float green, float blue, float alpha) { drawBoundingBox(box.minX, box.minY, box.minZ, box.maxX, box.maxY, box.maxZ, red, green, blue, alpha); } public static void drawBoundingBox(double minX, double minY, double minZ, double maxX, double maxY, double maxZ, float red, float green, float blue, float alpha) { Tessellator t = Tessellator.getInstance(); BufferBuilder b1 = t.getBuffer(); b1.begin(3, DefaultVertexFormats.POSITION_COLOR); drawBoundingBox(b1, minX, minY, minZ, maxX, maxY, maxZ, red, green, blue, alpha); t.draw(); } public static void drawBoundingBox(BufferBuilder b1, double minX, double minY, double minZ, double maxX, double maxY, double maxZ, float red, float green, float blue, float alpha) { b1.pos(minX, minY, minZ).color(red, green, blue, 0.0F).endVertex(); b1.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b1.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b1.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b1.pos(minX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b1.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(minX, maxY, maxZ).color(red, green, blue, 0.0F).endVertex(); b1.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, maxY, maxZ).color(red, green, blue, 0.0F).endVertex(); b1.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, maxY, minZ).color(red, green, blue, 0.0F).endVertex(); b1.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); b1.pos(maxX, minY, minZ).color(red, green, blue, 0.0F).endVertex(); } // Get block damage state private float getBlockDamage(EntityPlayer entityIn, double d0, double d1, double d2) { if (!this.damagedBlocks.isEmpty()) { Iterator<DestroyBlockProgress> iterator = this.damagedBlocks.values().iterator(); while(iterator.hasNext()) { DestroyBlockProgress destroyblockprogress = iterator.next(); BlockPos blockpos = destroyblockprogress.getPosition(); double d3 = (double)blockpos.getX() - d0; double d4 = (double)blockpos.getY() - d1; double d5 = (double)blockpos.getZ() - d2; Block block = entityIn.getEntityWorld().getBlockState(blockpos).getBlock(); TileEntity te = entityIn.getEntityWorld().getTileEntity(blockpos); boolean hasBreak = block instanceof BlockChest || block instanceof BlockEnderChest || block instanceof BlockSign || block instanceof BlockSkull; if (!hasBreak) hasBreak = te != null && te.canRenderBreaking(); if(!hasBreak) { if (d3 * d3 + d4 * d4 + d5 * d5 > 1024.0D) { iterator.remove(); } else { IBlockState iblockstate = entityIn.getEntityWorld().getBlockState(blockpos); if(iblockstate.getMaterial() != Material.AIR) { float f = (float) destroyblockprogress.getPartialBlockDamage(); return f; } } } } } return 0.0F; } }
-
Translating WorldRenderer startDRawingQuads to 1.12
fuzzybat23 replied to fuzzybat23's topic in Modder Support
This ended up being the new code that seems to work, though not underwater. Did I declare mc correctly as Private Final Minecraft mc = Minecraft.getMinecraft();? package com.fuzzybat23.test1; import com.fuzzybat23.test1.proxy.CommonProxy; import com.google.common.collect.Lists; import com.google.common.collect.Maps; import com.google.common.collect.Queues; import com.google.common.collect.Sets; import com.google.gson.JsonSyntaxException; import java.io.IOException; import java.util.Collection; import java.util.Iterator; import java.util.List; import java.util.Map; import java.util.Queue; import java.util.Random; import java.util.Set; import javax.annotation.Nullable; import net.minecraft.block.Block; import net.minecraft.block.BlockChest; import net.minecraft.block.BlockEnderChest; import net.minecraft.block.BlockSign; import net.minecraft.block.BlockSkull; import net.minecraft.block.SoundType; import net.minecraft.block.material.Material; import net.minecraft.block.state.IBlockState; import net.minecraft.client.Minecraft; import net.minecraft.client.audio.ISound; import net.minecraft.client.audio.PositionedSoundRecord; import net.minecraft.client.multiplayer.WorldClient; import net.minecraft.client.particle.Particle; import net.minecraft.client.renderer.ActiveRenderInfo; import net.minecraft.client.renderer.BlockRendererDispatcher; import net.minecraft.client.renderer.BufferBuilder; import net.minecraft.client.renderer.DestroyBlockProgress; import net.minecraft.client.renderer.GlStateManager; import net.minecraft.client.renderer.Tessellator; import net.minecraft.client.renderer.chunk.ChunkRenderDispatcher; import net.minecraft.client.renderer.chunk.CompiledChunk; import net.minecraft.client.renderer.chunk.IRenderChunkFactory; import net.minecraft.client.renderer.chunk.ListChunkFactory; import net.minecraft.client.renderer.chunk.RenderChunk; import net.minecraft.client.renderer.chunk.VboChunkFactory; import net.minecraft.client.renderer.chunk.VisGraph; import net.minecraft.client.renderer.culling.ClippingHelper; import net.minecraft.client.renderer.culling.ClippingHelperImpl; import net.minecraft.client.renderer.culling.Frustum; import net.minecraft.client.renderer.culling.ICamera; import net.minecraft.client.renderer.entity.RenderManager; import net.minecraft.client.renderer.texture.TextureAtlasSprite; import net.minecraft.client.renderer.texture.TextureManager; import net.minecraft.client.renderer.texture.TextureMap; import net.minecraft.client.renderer.tileentity.TileEntityRendererDispatcher; import net.minecraft.client.renderer.vertex.DefaultVertexFormats; import net.minecraft.client.renderer.vertex.VertexBuffer; import net.minecraft.client.renderer.vertex.VertexFormat; import net.minecraft.client.renderer.vertex.VertexFormatElement; import net.minecraft.client.resources.IResourceManager; import net.minecraft.client.resources.IResourceManagerReloadListener; import net.minecraft.client.shader.Framebuffer; import net.minecraft.client.shader.ShaderGroup; import net.minecraft.client.shader.ShaderLinkHelper; import net.minecraft.crash.CrashReport; import net.minecraft.crash.CrashReportCategory; import net.minecraft.crash.ICrashReportDetail; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.init.SoundEvents; import net.minecraft.item.Item; import net.minecraft.item.ItemDye; import net.minecraft.item.ItemRecord; import net.minecraft.tileentity.TileEntity; import net.minecraft.tileentity.TileEntityChest; import net.minecraft.util.BlockRenderLayer; import net.minecraft.util.ClassInheritanceMultiMap; import net.minecraft.util.EnumFacing; import net.minecraft.util.EnumParticleTypes; import net.minecraft.util.ReportedException; import net.minecraft.util.ResourceLocation; import net.minecraft.util.SoundCategory; import net.minecraft.util.SoundEvent; import net.minecraft.util.math.AxisAlignedBB; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.MathHelper; import net.minecraft.util.math.RayTraceResult; import net.minecraft.util.math.Vec3d; import net.minecraft.world.IBlockAccess; import net.minecraft.world.IWorldEventListener; import net.minecraft.world.World; import net.minecraft.world.border.WorldBorder; import net.minecraft.world.chunk.Chunk; import net.minecraftforge.client.event.DrawBlockHighlightEvent; import net.minecraftforge.client.event.MouseEvent; import net.minecraftforge.common.MinecraftForge; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.Mod.Instance; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.common.eventhandler.SubscribeEvent; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; import org.apache.logging.log4j.LogManager; import org.apache.logging.log4j.Logger; import org.lwjgl.opengl.GL11; import org.lwjgl.util.vector.Vector3f; import org.lwjgl.util.vector.Vector4f; @Mod(modid=Reference.Mod_ID, name=Reference.NAME, version=Reference.VERSION, acceptedMinecraftVersions=Reference.ACCEPTED_VERSIONS) public class CSBR { //public class RenderGlobal implements IWorldEventListener, IResourceManagerReloadListener { private static final Logger LOGGER = LogManager.getLogger(); private final Minecraft mc = Minecraft.getMinecraft(); float r = 0.0F; //red float g = 0.0F; //green float b = 0.0F; //blue float a = 0.0F; //alpha float ba = 0.10F; //blink alpha float w = 2.0F; //Line Width public static boolean disableDepthBuffer; public static VertexFormat BLOCK = new VertexFormat(); @Instance public static CSBR instance; @SidedProxy(clientSide = Reference.CLIENT_PROXY_CLASS, serverSide = Reference.CLIENT_SERVER_CLASS) public static CommonProxy proxy; @EventHandler public void preInit(FMLPreInitializationEvent event) { proxy.registerTickHandler(); MinecraftForge.EVENT_BUS.register(this); r = 1.0F; a = 0.30F; ba = 0.05F; w = 4.0F; disableDepthBuffer=false; } @EventHandler public void Init(FMLInitializationEvent event) { } @EventHandler public void PostInit(FMLPostInitializationEvent event) { } @SubscribeEvent public void onDrawBlockSelectionBox(DrawBlockHighlightEvent e) { drawSelectionBox(e.getPlayer(), e.getTarget(), e.getSubID(), e.getPartialTicks()); e.setCanceled(true); } /** * Draws the selection box for the player. */ public void drawSelectionBox(EntityPlayer player, RayTraceResult movingObjectPositionIn, int execute, float partialTicks) { if (execute == 0 && movingObjectPositionIn.typeOfHit == RayTraceResult.Type.BLOCK) { if(disableDepthBuffer) { GL11.glDisable(GL11.GL_DEPTH_TEST); } GlStateManager.enableBlend(); GlStateManager.tryBlendFuncSeparate(GlStateManager.SourceFactor.SRC_ALPHA, GlStateManager.DestFactor.ONE_MINUS_SRC_ALPHA, GlStateManager.SourceFactor.ONE, GlStateManager.DestFactor.ZERO); GlStateManager.glLineWidth(w); GlStateManager.disableTexture2D(); GlStateManager.depthMask(false); if (this.mc.objectMouseOver != null && this.mc.objectMouseOver.typeOfHit == RayTraceResult.Type.BLOCK && this.mc.objectMouseOver.getBlockPos() != null ) { BlockPos blockpos1 = this.mc.objectMouseOver.getBlockPos(); // BlockPos blockpos = movingObjectPositionIn.getBlockPos(); IBlockState iblockstate = player.getEntityWorld().getBlockState(blockpos1); // if (iblockstate.getMaterial() != Material.AIR && player.getEntityWorld().getWorldBorder().contains(blockpos)) // { double d0 = player.lastTickPosX + (player.posX - player.lastTickPosX) * (double)partialTicks; double d1 = player.lastTickPosY + (player.posY - player.lastTickPosY) * (double)partialTicks; double d2 = player.lastTickPosZ + (player.posZ - player.lastTickPosZ) * (double)partialTicks; AxisAlignedBB bb = iblockstate.getSelectedBoundingBox(player.getEntityWorld(), blockpos1).grow(0.0020000000949949026D).offset(-d0, -d1, -d2); //Draw Boxes drawSelectionBoxMask(bb, r, g, b, ba); drawSelectionBoundingBox(bb, r, g, b, a); } GL11.glEnable(GL11.GL_DEPTH_TEST); GlStateManager.depthMask(true); GlStateManager.enableTexture2D(); GlStateManager.disableBlend(); } } public static void drawSelectionBoundingBox(AxisAlignedBB box, float red, float green, float blue, float alpha) { drawBoundingBox(box.minX, box.minY, box.minZ, box.maxX, box.maxY, box.maxZ, red, green, blue, alpha); } public static void drawSelectionBoxMask(AxisAlignedBB box, float red, float green, float blue, float alpha) { drawMask(box.minX, box.minY, box.minZ, box.maxX, box.maxY, box.maxZ, red, green, blue, alpha); } public static void drawBoundingBox(double minX, double minY, double minZ, double maxX, double maxY, double maxZ, float red, float green, float blue, float alpha) { Tessellator t = Tessellator.getInstance(); BufferBuilder b = t.getBuffer(); b.begin(3, DefaultVertexFormats.POSITION_COLOR); drawBoundingBox(b, minX, minY, minZ, maxX, maxY, maxZ, red, green, blue, alpha); t.draw(); } public static void drawMask(double minX, double minY, double minZ, double maxX, double maxY, double maxZ, float red, float green, float blue, float alpha) { Tessellator t = Tessellator.getInstance(); BufferBuilder b = t.getBuffer(); b.begin(7, DefaultVertexFormats.POSITION_COLOR); drawMask(b, minX, minY, minZ, maxX, maxY, maxZ, red, green, blue, alpha); t.draw(); } public static void drawBoundingBox(BufferBuilder b, double minX, double minY, double minZ, double maxX, double maxY, double maxZ, float red, float green, float blue, float alpha) { b.pos(minX, minY, minZ).color(red, green, blue, 0.0F).endVertex(); b.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, maxY, maxZ).color(red, green, blue, 0.0F).endVertex(); b.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, maxZ).color(red, green, blue, 0.0F).endVertex(); b.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, minZ).color(red, green, blue, 0.0F).endVertex(); b.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, minY, minZ).color(red, green, blue, 0.0F).endVertex(); } public static void drawMask(BufferBuilder b, double minX, double minY, double minZ, double maxX, double maxY, double maxZ, float red, float green, float blue, float alpha) { //up b.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); //b.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); //down b.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, minZ).color(red, green, blue, alpha).endVertex(); //b.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); //north b.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); //b.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); //south b.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); //b.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); //east b.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); //b.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); //west b.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); //b.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); } } -
Translating WorldRenderer startDRawingQuads to 1.12
fuzzybat23 replied to fuzzybat23's topic in Modder Support
Meh, I just looked through the entire list of events the eventhandler uses. I don't see anything that could remotely be useful to this other than DrawBlockHighlightEvent, since that is very specific to what I'm doing I'll have to settle with no underwater fanciness until Mojang adds the selection box to underwater viewing. They could at least make it something that can be turned on and off from the settings -
Translating WorldRenderer startDRawingQuads to 1.12
fuzzybat23 replied to fuzzybat23's topic in Modder Support
What about this? BlockPos blockpos1 = this.mc.objectMouseOver.getBlockPos(); Found it in the gui that governs the debug screen here: if (this.mc.objectMouseOver != null && this.mc.objectMouseOver.typeOfHit == RayTraceResult.Type.BLOCK && this.mc.objectMouseOver.getBlockPos() != null) { BlockPos blockpos1 = this.mc.objectMouseOver.getBlockPos(); list.add(String.format("Looking at: %d %d %d", blockpos1.getX(), blockpos1.getY(), blockpos1.getZ())); } -
Translating WorldRenderer startDRawingQuads to 1.12
fuzzybat23 replied to fuzzybat23's topic in Modder Support
I had an idea on that actually. The debug screen. Part of the debug screen is that line that tells you the coordinates of the block you're looking at. Those coordinates are all this mod really needs to function, right? So how does the game get the coordinates that are put up on the debug screen? -
Translating WorldRenderer startDRawingQuads to 1.12
fuzzybat23 replied to fuzzybat23's topic in Modder Support
Ugh, that sounds like a pain in the ass. Shame too, cause the original code, as it was written for 1.8, did draw the box underwater. It called the DrawBlockHighlightEvent as well, I think. Guess they changed that somewhere between 1.8 and now. -
Translating WorldRenderer startDRawingQuads to 1.12
fuzzybat23 replied to fuzzybat23's topic in Modder Support
Just out of idle curiosity, now, you don't happen to know how to make it draw that box when you're underwater, do you? Looking at a block that's in the water, but while your head is above water, draws the bounding box, but when you're completely submerged you get nothing at all. Makes underwater construction so much a bother x.x -
Translating WorldRenderer startDRawingQuads to 1.12
fuzzybat23 replied to fuzzybat23's topic in Modder Support
-
Translating WorldRenderer startDRawingQuads to 1.12
fuzzybat23 replied to fuzzybat23's topic in Modder Support
Oh yeah I was using the original coding. So by quad you mean each face of the cube, right? Just like I have it, up, down, north, south, east and west, but get rid of the duplicate value in each of the directions? -
Translating WorldRenderer startDRawingQuads to 1.12
fuzzybat23 replied to fuzzybat23's topic in Modder Support
It's doing some weird stuff with that criss-crossing in the middle like that. Should I split up each of the six directions into their own separate functions? -
Translating WorldRenderer startDRawingQuads to 1.12
fuzzybat23 replied to fuzzybat23's topic in Modder Support
Ok, making progress It's working, to a degree. But with a weird result, probably due to my poor grasp of vertices This: public static void drawSelectionBoxMask(AxisAlignedBB box, float red, float green, float blue, float alpha) { drawMask(box.minX, box.minY, box.minZ, box.maxX, box.maxY, box.maxZ, red, green, blue, alpha); } public static void drawMask(double minX, double minY, double minZ, double maxX, double maxY, double maxZ, float red, float green, float blue, float alpha) { Tessellator tessellator = Tessellator.getInstance(); BufferBuilder bufferbuilder = tessellator.getBuffer(); bufferbuilder.begin(7, DefaultVertexFormats.POSITION_COLOR); drawMask(bufferbuilder, minX, minY, minZ, maxX, maxY, maxZ, red, green, blue, alpha); tessellator.draw(); } public static void drawMask(BufferBuilder b, double minX, double minY, double minZ, double maxX, double maxY, double maxZ, float red, float green, float blue, float alpha) { //up b.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); //down b.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); //north b.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); //south b.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); //east b.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); //west b.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); b.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); } ends up spitting out something that looks like this: -
Translating WorldRenderer startDRawingQuads to 1.12
fuzzybat23 replied to fuzzybat23's topic in Modder Support
Yeah, I just plugged in the 7 to my original code and it came out looking pretty weird. I'm gonna have to play with the vertices to get them right. I've always sucked at math and geometry -
Translating WorldRenderer startDRawingQuads to 1.12
fuzzybat23 replied to fuzzybat23's topic in Modder Support
It's funny, just as your thing blipped up a reply, I glanced up and saw this already in my code for drawing the bounding box. bufferbuilder.begin(3, DefaultVertexFormats.POSITION_COLOR); So to start, I'd want something similar. public static void whatever(double minX, double minY, double minZ, double maxX, double maxY, double maxZ, float red, float green, float blue, float alpha) { Tessellator t = Tessellator.getInstance(); BufferBuilder b = t.getBuffer(); b.begin(7, DefaultVertexFormats.POSITION_COLOR); drawMask(bufferbuilder, minX, minY, minZ, maxX, maxY, maxZ, red, green, blue, alpha); t.draw(); } public static void drawMask(BufferBuilder buffer, double minX, double minY, double minZ, double maxX, double maxY, double maxZ, float red, float green, float blue, float alpha) { buffer.pos(minX, minY, minZ).color(red, green, blue, 0.0F).endVertex(); buffer.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); buffer.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); buffer.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); buffer.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); buffer.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); buffer.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); buffer.pos(maxX, maxY, minZ).color(red, green, blue, alpha).endVertex(); buffer.pos(maxX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); buffer.pos(minX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); buffer.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); buffer.pos(minX, maxY, maxZ).color(red, green, blue, 0.0F).endVertex(); buffer.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); buffer.pos(maxX, maxY, maxZ).color(red, green, blue, 0.0F).endVertex(); buffer.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); buffer.pos(maxX, maxY, minZ).color(red, green, blue, 0.0F).endVertex(); buffer.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); buffer.pos(maxX, minY, minZ).color(red, green, blue, 0.0F).endVertex(); } Is that about right? -
Translating WorldRenderer startDRawingQuads to 1.12
fuzzybat23 replied to fuzzybat23's topic in Modder Support
Ok, I already have texture2d disabled in the function that ultimately calls my drawboundingbox function. if(disableDepthBuffer) { GL11.glDisable(GL11.GL_DEPTH_TEST); } GlStateManager.enableBlend(); GlStateManager.tryBlendFuncSeparate(GlStateManager.SourceFactor.SRC_ALPHA, GlStateManager.DestFactor.ONE_MINUS_SRC_ALPHA, GlStateManager.SourceFactor.ONE, GlStateManager.DestFactor.ZERO); GlStateManager.glLineWidth(2.0F); GlStateManager.disableTexture2D(); GlStateManager.depthMask(false); How do I use this POSITION_COLOR now? I'm still learning this stuff as I go. I know I've seen position_Color somewhere recently, but for the life of me I can't remember where. -
Translating WorldRenderer startDRawingQuads to 1.12
fuzzybat23 replied to fuzzybat23's topic in Modder Support
So in the place of start drawing quads, I'd probably want: buffer.begin(7, BLOCK); To draw a block which I would apply said color and alpha channel to? -
I have an old mod that use to apply a mask to blocks the cursor is aimed at, instead of just drawing the outlined bounding box. See attached picture for to get the idea of what I'm trying to explain. Anyhoo, this is how the bounding box is actually drawn in 1.12; public static void drawSelectionBoundingBox(AxisAlignedBB box, float red, float green, float blue, float alpha) { drawBoundingBox(box.minX, box.minY, box.minZ, box.maxX, box.maxY, box.maxZ, red, green, blue, alpha); } public static void drawBoundingBox(double minX, double minY, double minZ, double maxX, double maxY, double maxZ, float red, float green, float blue, float alpha) { Tessellator tessellator = Tessellator.getInstance(); BufferBuilder bufferbuilder = tessellator.getBuffer(); bufferbuilder.begin(3, DefaultVertexFormats.POSITION_COLOR); drawBoundingBox(bufferbuilder, minX, minY, minZ, maxX, maxY, maxZ, red, green, blue, alpha); tessellator.draw(); } public static void drawBoundingBox(BufferBuilder buffer, double minX, double minY, double minZ, double maxX, double maxY, double maxZ, float red, float green, float blue, float alpha) { buffer.pos(minX, minY, minZ).color(red, green, blue, 0.0F).endVertex(); buffer.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); buffer.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); buffer.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); buffer.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); buffer.pos(minX, minY, minZ).color(red, green, blue, alpha).endVertex(); buffer.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); buffer.pos(maxX, maxY, minZ).color(red, green, blue, alpha).endVertex(); buffer.pos(maxX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); buffer.pos(minX, maxY, maxZ).color(red, green, blue, alpha).endVertex(); buffer.pos(minX, maxY, minZ).color(red, green, blue, alpha).endVertex(); buffer.pos(minX, maxY, maxZ).color(red, green, blue, 0.0F).endVertex(); buffer.pos(minX, minY, maxZ).color(red, green, blue, alpha).endVertex(); buffer.pos(maxX, maxY, maxZ).color(red, green, blue, 0.0F).endVertex(); buffer.pos(maxX, minY, maxZ).color(red, green, blue, alpha).endVertex(); buffer.pos(maxX, maxY, minZ).color(red, green, blue, 0.0F).endVertex(); buffer.pos(maxX, minY, minZ).color(red, green, blue, alpha).endVertex(); buffer.pos(maxX, minY, minZ).color(red, green, blue, 0.0F).endVertex(); } And this is the old way this was drawn back in 1.8, I believe: private static void drawBlinkingBlock(AxisAlignedBB par1AxisAlignedBB, float alpha) { Tessellator tessellator = Tessellator.getInstance(); if (alpha > 0.0F) { if (getBlinkSpeed() > 0 && CSB.breakAnimation != ALPHA) alpha *= (float) Math.abs(Math.sin(Minecraft.getSystemTime() / 100.0D * getBlinkSpeed())); GL11.glColor4f(getRed(), getGreen(), getBlue(), alpha); renderDown(par1AxisAlignedBB); renderUp(par1AxisAlignedBB); renderNorth(par1AxisAlignedBB); renderSouth(par1AxisAlignedBB); renderWest(par1AxisAlignedBB); renderEast(par1AxisAlignedBB); } } public static void renderUp(AxisAlignedBB par1AxisAlignedBB) { Tessellator tessellator = Tessellator.getInstance(); WorldRenderer worldrenderer = tessellator.getWorldRenderer(); worldrenderer.startDrawingQuads(); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.minY, par1AxisAlignedBB.minZ); worldrenderer.addVertex(par1AxisAlignedBB.maxX, par1AxisAlignedBB.minY, par1AxisAlignedBB.minZ); worldrenderer.addVertex(par1AxisAlignedBB.maxX, par1AxisAlignedBB.minY, par1AxisAlignedBB.maxZ); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.minY, par1AxisAlignedBB.maxZ); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.minY, par1AxisAlignedBB.minZ); tessellator.draw(); } public static void renderDown(AxisAlignedBB par1AxisAlignedBB) { Tessellator tessellator = Tessellator.getInstance(); WorldRenderer worldrenderer = tessellator.getWorldRenderer(); worldrenderer.startDrawingQuads(); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.maxY, par1AxisAlignedBB.minZ); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.maxY, par1AxisAlignedBB.maxZ); worldrenderer.addVertex(par1AxisAlignedBB.maxX, par1AxisAlignedBB.maxY, par1AxisAlignedBB.maxZ); worldrenderer.addVertex(par1AxisAlignedBB.maxX, par1AxisAlignedBB.maxY, par1AxisAlignedBB.minZ); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.maxY, par1AxisAlignedBB.minZ); tessellator.draw(); } public static void renderNorth(AxisAlignedBB par1AxisAlignedBB) { Tessellator tessellator = Tessellator.getInstance(); WorldRenderer worldrenderer = tessellator.getWorldRenderer(); worldrenderer.startDrawingQuads(); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.minY, par1AxisAlignedBB.minZ); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.maxY, par1AxisAlignedBB.minZ); worldrenderer.addVertex(par1AxisAlignedBB.maxX, par1AxisAlignedBB.maxY, par1AxisAlignedBB.minZ); worldrenderer.addVertex(par1AxisAlignedBB.maxX, par1AxisAlignedBB.minY, par1AxisAlignedBB.minZ); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.minY, par1AxisAlignedBB.minZ); tessellator.draw(); } public static void renderSouth(AxisAlignedBB par1AxisAlignedBB) { Tessellator tessellator = Tessellator.getInstance(); WorldRenderer worldrenderer = tessellator.getWorldRenderer(); worldrenderer.startDrawingQuads(); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.minY, par1AxisAlignedBB.maxZ); worldrenderer.addVertex(par1AxisAlignedBB.maxX, par1AxisAlignedBB.minY, par1AxisAlignedBB.maxZ); worldrenderer.addVertex(par1AxisAlignedBB.maxX, par1AxisAlignedBB.maxY, par1AxisAlignedBB.maxZ); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.maxY, par1AxisAlignedBB.maxZ); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.minY, par1AxisAlignedBB.maxZ); tessellator.draw(); } public static void renderWest(AxisAlignedBB par1AxisAlignedBB) { Tessellator tessellator = Tessellator.getInstance(); WorldRenderer worldrenderer = tessellator.getWorldRenderer(); worldrenderer.startDrawingQuads(); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.minY, par1AxisAlignedBB.minZ); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.minY, par1AxisAlignedBB.maxZ); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.maxY, par1AxisAlignedBB.maxZ); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.maxY, par1AxisAlignedBB.minZ); worldrenderer.addVertex(par1AxisAlignedBB.minX, par1AxisAlignedBB.minY, par1AxisAlignedBB.minZ); tessellator.draw(); } public static void renderEast(AxisAlignedBB par1AxisAlignedBB) { Tessellator tessellator = Tessellator.getInstance(); WorldRenderer worldrenderer = tessellator.getWorldRenderer(); worldrenderer.startDrawingQuads(); worldrenderer.addVertex(par1AxisAlignedBB.maxX, par1AxisAlignedBB.minY, par1AxisAlignedBB.minZ); worldrenderer.addVertex(par1AxisAlignedBB.maxX, par1AxisAlignedBB.maxY, par1AxisAlignedBB.minZ); worldrenderer.addVertex(par1AxisAlignedBB.maxX, par1AxisAlignedBB.maxY, par1AxisAlignedBB.maxZ); worldrenderer.addVertex(par1AxisAlignedBB.maxX, par1AxisAlignedBB.minY, par1AxisAlignedBB.maxZ); worldrenderer.addVertex(par1AxisAlignedBB.maxX, par1AxisAlignedBB.minY, par1AxisAlignedBB.minZ); tessellator.draw(); } getBlinkSpeed(), getRed(), getGreen(), and getBlue() are calls to retrieve the float values from a config file. For this post, assume Blink Speed is 0, Red is 1.0F, Green is 0.0F and Blue is 0.0F, basically meaning I'm trying to achieve what is in the picture, only with a solid red bounding box and the inside mask has an alpha value of 0.5F
-
Null pointer exception keeps getting thrown calling IBlockState
fuzzybat23 replied to fuzzybat23's topic in Modder Support
I'm trying to revitalize the Custom Selection Box mod from the ground up. Found the source in RenderGlobal, now I can butcher the original code to get the gui working how I want. The original coder stopped work on it in vs 1.3, then someone picked it up and updated it to 1.8 and he's not touched it since. Now that I have it functioning at least a little, it mots me way closer than I was before. Thanks :> Now I need to figure out why the game doesn't draw the selection box when you're under water -
Null pointer exception keeps getting thrown calling IBlockState
fuzzybat23 replied to fuzzybat23's topic in Modder Support
Already did. I changed out every instance of this.world with player.getEntityWorld() Game ran with no crash and the bounding box is white, as I have it set in its color code portion. :> -
Null pointer exception keeps getting thrown calling IBlockState
fuzzybat23 replied to fuzzybat23's topic in Modder Support
Something along the lines of this? IBlockState iblockstate = player.getEntityWorld().getBlockState(blockpos);