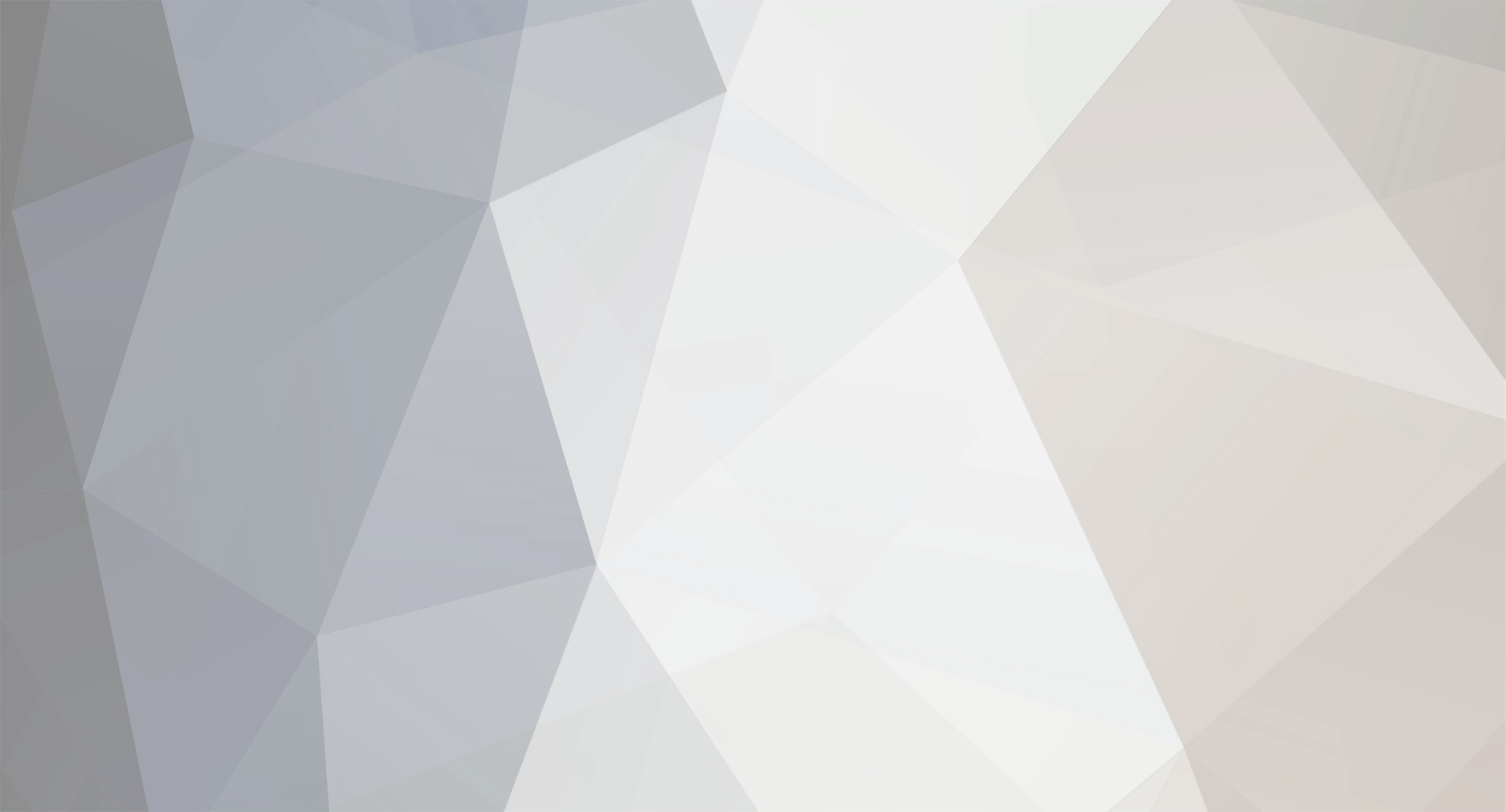
shieldbug1
Forge Modder-
Posts
404 -
Joined
-
Last visited
Everything posted by shieldbug1
-
There is only one World on the client, as diesieben said. So you can just use Minecraft#theWorld.provider.getDimensionName().
-
Problem with opening client in Ecplipse
shieldbug1 replied to FluffyAminal's topic in Support & Bug Reports
Update to the latest forge. -
Checking Whether a Player is Holding a Block
shieldbug1 replied to Adityagupta's topic in Modder Support
Items and Blocks aren't the same thing. Example code public void onPlayerTick(TickEvent.PlayerTick event) { if(event.side.isServer() && event.phase == TickEvent.Phase.START) { ItemStack stack = event.player.getCurrentEquippedItem(); if(stack != null && stack.getItem() != null && Block.getBlockFromItem(stack.getItem()) != null) { Block block = Block.getBlockFromItem(stack.getItem()); } } } -
You almost always have an instance of World available, and World#getCurrentWorldTime is given in ticks. Given that there are 20 ticks/second, to wait 4 seconds you want to wait 80 ticks.
-
You don't need to extend BlockContainer for a tile entity. Just implement ITileEntityProvider.
-
You never initialise your Random instance. Which btw you can use player.getRNG() or something like that, you don't have to instantiate a new instance of Random.
-
If the world is not null I'd assume it would be fine. Anyway, I think a better place to do this is in a PlayerTickEvent. That way you already know the player isn't null and the World object associated with it isn't null either. Then all you'd have to do is surround your code with if(event.side.isClient()) And use event.player rather than Minecraft#thePlayer.
-
Minecraft minecraft = Minecraft.getMinecraft(); if(minecraft.thePlayer != null) { //Code using the player } Minecraft#thePlayer is only valid when the 'player' is in an actual world. RenderTickEvent would be called from the moment the main menu is showing (where there is no 'player').
-
Client for minecraft 1.7.10 wont launch
shieldbug1 replied to stevo323's topic in Support & Bug Reports
Read the EAQ. -
I actually haven't done much rendering at all, but I think this could be because you're not rotating it back after you're done. I could be completely wrong though.
-
It's how to update information for TileEntities. Another way is to use the SimpleNetworkWrapper. You can take a look at pahimar's EE3 code on GitHub for some examples.
-
In your read from NBT you're not doing anything. You have to set the variable, not just get it. this.speed = compound.getInteger("Speed");
-
Why do you feel the need to edit a base class? You most likely don't need to. Forge events and forge hooks offer almost everything you need.
-
Read the EAQ.
-
First bump. I've actually been really busy and haven't gotten any more time to think over this problem, so I would still greatly appreciate any help.
-
You could maybe mess around with the ItemTossEvent, that might work. I'm not sure if ItemExpireEvent gets called when it /expires/ or just whenever the EntityItem dies. Otherwise, you can do what I had to do a while back. Using the WorldTickEvent event, iterate through the event.world.loadedEntityList, if the entity is an instanceof EntityItem, do your checks (handleLavaMovement() is for lava), and if there are whatever other EntityItems of the type you want (EntityItem#getEntityItem and ItemStack#getItem), then remove the entities and spawn the one you want. There probably is a better way to do it, but that's all I can think of right now
-
Well, that works almost perfectly. This is the code that I'm using: @SubscribeEvent public void onMouseClicked(MouseEvent event) { boolean flag = false; int bind = Minecraft.getMinecraft().gameSettings.keyBindAttack.getKeyCode(); /* * Flag is true if the button is also the same as the keyBinding for attacking. */ flag = ((bind == -100) ? (event.button == 0) : ((bind == -99) ? (event.button == 1) : ((bind == -98) ? (event.button == 2) : false))); if(flag && event.buttonstate) { if(Minecraft.getMinecraft().thePlayer != null) { EntityPlayer player = Minecraft.getMinecraft().thePlayer; if(player.getCurrentEquippedItem() != null && player.getCurrentEquippedItem().getItem() instanceof ItemWarHammer) { if(player.attackTime != 0) { event.setCanceled(true); } else { player.attackTime = 20; } } } } } As you can see, it'll work perfectly, unless the keybinding for attack is a key, rather than a mouse click. Now I thought I could use the KeyInputEvent, however that Event isn't cancelable, and from reading documentation on it, it seems that it occurs AFTER vanilla mechanics. Is there any elegant way to do this? I might be able to do something like that from the ClientTick event and constantly checking if the Minecraft#gameSettings.keyBindAttack is being pressed, but then I'd still have to somehow cancel it, and I think that would be rather difficult. As for the potion effects, making it ambient made it see-through enough for me, until I feel like messing with it.
-
I have a class that extends ItemSword. It's supposed to be a 'war-hammer' styled weapon, so I want there to be a longer delay (slower swing and more time in between swings). The visual effect can be easily achieved by applying the mining fatigue effect to the player, and it works perfectly (though, if there was a more elegant way of doing it without the potion effect 'swirls' that would be great), however I haven't managed to find a field I can control to increase the delay between actual hits (if I use the potion effect, the hammer moves slowly, but you can rapidly click it like a sword). Is there a field I can simply change, or am I going to have to hardcode this in somehow? As a side question, since I don't think it requires an enitre thread for itself - What EventBus is it standard to post custom events to - the one where the event you're extending is posted (e.g. extending ItemPickupEvent, posting on FMLCommonHandler#bus()) or posting on an event bus created by you 'dedicated' to your own mod?
-
That's old. Using the new ForgeGradle system, you just need to run the command gradlew build.
-
Read the EAQ.
-
[1.7.10] Rendering a entity model in a tile entity
shieldbug1 replied to Mecblader's topic in Modder Support
I haven't done much rendering or modelling, but I feel like a good place to start would be with the renderer for Mob Spawners. -
Your SidedProxy annotation is on the same field as your Instance annotation. You do not have an instance of ServerProxy annotated with @SidedProxy.
-
http://lmgtfy.com/?q=sidedproxy
-
Alright, thank you very much for the help.
-
The stats sync properly through PlayerLoggedInEvent. They still, however, do not sync when the player is 'Cloned'. Is the Clone event not the appropriate place to sync?