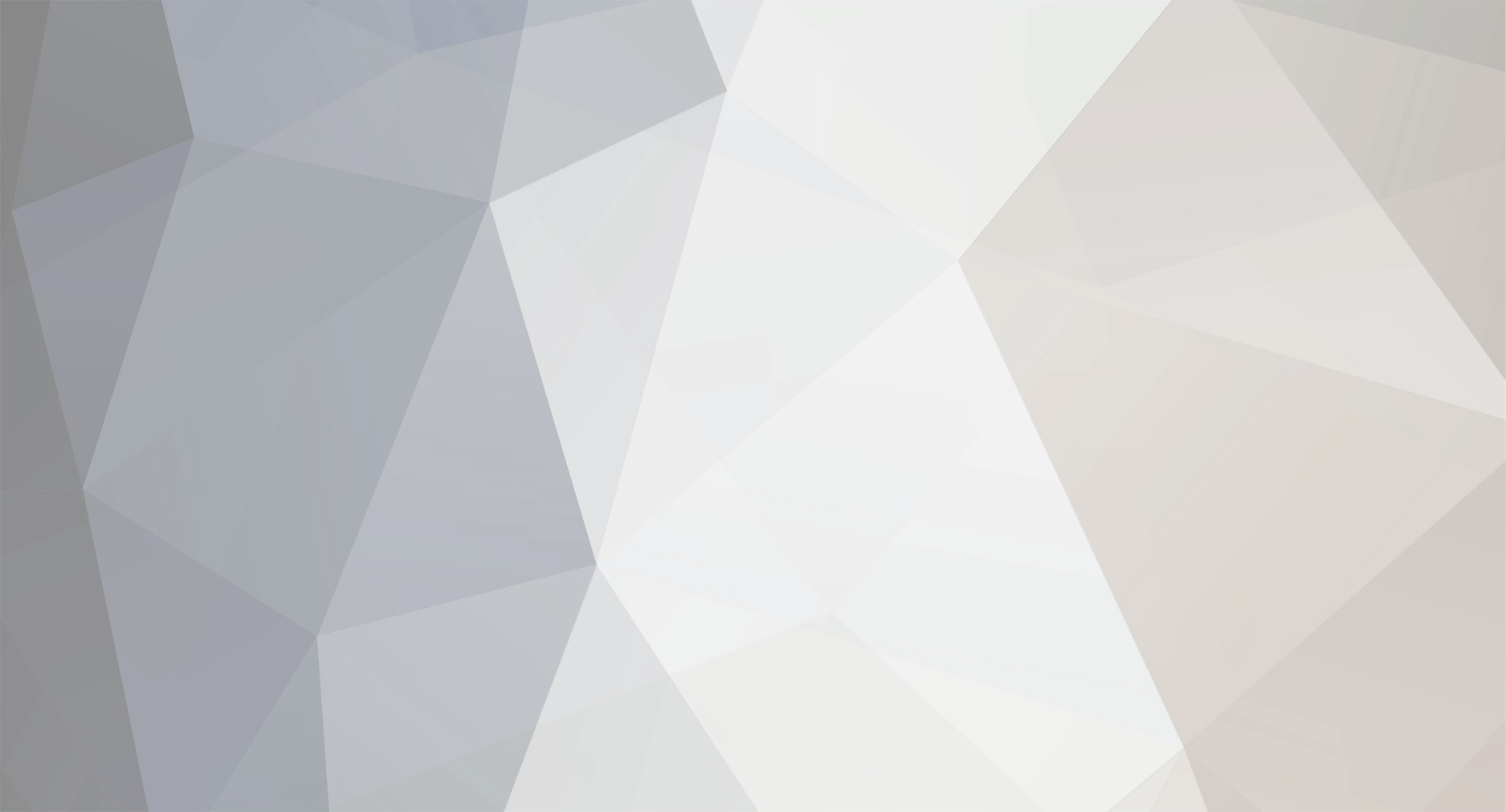
shieldbug1
Forge Modder-
Posts
404 -
Joined
-
Last visited
Everything posted by shieldbug1
-
First of all you're going to want to save a metadata value on the log when placed to determine it's rotation (there are three possible rotations so you would need to use two bits of metadata). Next in your registerIcons method you're gonna have to initialise your different icons. (side, top, and I think you also need sideRotated but I'm not sure). Then you override a method that has both the side and metadata values, and return the right icon based on that. I think that method is called getBlockIcon but I'm not certain. Just have a look around and you'll find it.
-
Do you have a ClientProxy class in the com.crocomet.itemsmod package?
-
Generating Less than one Ore vein per chunk.
shieldbug1 replied to turangur's topic in Modder Support
Look carefully at your for 'loop'. Also if you don't want it to generate in every chunk, just do something like if(random.nexInt(whatever) == 0) somewhere, or something like that. -
If you want your block to grow with bonemeal you need to implement IGrowable.
-
how do i detect when a player breaks a block ?
shieldbug1 replied to Ewe Loon's topic in Modder Support
It's more than two lines if you're an organisation freak like me. I like having different event handlers for different types of events to keep everything organised. -
how do i detect when a player breaks a block ?
shieldbug1 replied to Ewe Loon's topic in Modder Support
Doing it in your main mod file clutters it up and makes it look messy. A lot easier to call proxy.registerEventHandlers(); or whatever you call it. That way if you're registering an event handler only for rendering events, you only do so on the client. You can also split client/server config files like this. I thought most people did this as that's what I've seen from all these available tutorials and I can't see an obvious downside for it. -
how do i detect when a player breaks a block ?
shieldbug1 replied to Ewe Loon's topic in Modder Support
You need to register the class as an EventHandler. If you look at the BreakEvent it is in the minecraftforge package, so you need to register it to the MinecraftForge event bus. That is MinecraftForge.EVENT_BUS.register(INSTANCE_OF_YOUR_EVENT_HANDLER_CLASS); It's common practice to register you event handlers in your common proxy, then in your server/client proxies you override the method, and call the super method. This allows to have a client-only and server-only event handlers (very useful for configuration, rendering, ect.). -
1) Override getIcon(int side, int meta) and return the correct IIcon field for whatever side you want. 2) public static final CreativeTabs YOUR_CREATIVE_TAB = new CreativeTabs(YOUR_MOD_ID) { @Override public Item getTabIconItem() { return YOUR_ITEM; } }; As for 3) I believe you just need register the recipe you want to override as returning nothing, then register your own recipe normally.
-
how do i detect when a player breaks a block ?
shieldbug1 replied to Ewe Loon's topic in Modder Support
BlockEvent.BreakEvent -
[1.7.10][SOLVED]Block not getting replaced on item right click
shieldbug1 replied to The_Fireplace's topic in Modder Support
You're only setting the metadata of the block. Use world.setBlock -
[1.7.2] [SOLVED]Problem with giving items on first join
shieldbug1 replied to RedEnergy's topic in Modder Support
Your getBoolean is always returning false, unless you set it elsewhere. Try using !entitydata.getBoolean("firstJoin") and then set it true. -
[1.7.2][SOLVED] Adding Enchantment Glint to unenchanted Items
shieldbug1 replied to a topic in Modder Support
Override hasEffect(Itemstack itemstack, int pass) in your item class. -
There's two ways of doing this. In your item class there is an addInformation method which contains a List variable. You can then add your own tooltip to the list (as a String). The other way to do this, which (I believe) is the only if you want to add tooltips to vanilla items, is using the ItemToolTipEvent.
-
[1.7.2][unsolved] save mana in world [mana is glitching]
shieldbug1 replied to knokko's topic in Modder Support
The reason it's telling you to make it static is because you're trying to call the field from the class. I suggest you go read up on what static actually means, and the difference between instance fields and class fields. -
[1.7.10] Trying to make an api and a few question about an api.
shieldbug1 replied to drok0920's topic in Modder Support
You use whatever you want to use. There is a lot of debate about what is considered an 'API'. Generally, an API in this case would be an interface that you handle yourself, but it is not limited to it. Example: You have an item that transforms blocks into other blocks. You create an interface called something like ITransformable. Inside this interface you'd have methods like resultBlock() and resultMetadata(), that have return types of Block and int. Other things could include void methods like onTransform() and postTransform(). Then if you or anyone else would like your block to be transformable you make it implement ITransformable, and return the appropriate block and metadata. In your item class you'd check if the block you're clicking is an instance of ITransformable. If it is, you then call onTransform() so you can let the modder do their own functionality, then transform the block with world.setBlock(transformable.resultBlock(), x, y, z) and metadata with world.setBlockMetadata(transformable.resultMetadata(), x, y, z), then call transformable.postTransform() to allow the modder to do any finishing up they want. You want to think an interface out as much as you can, because changing an interface is looked down upon a lot. -
[1.7.2][unsolved] save mana in world [mana is glitching]
shieldbug1 replied to knokko's topic in Modder Support
My understanding of this is that you want to have a 'global' mana variable that is shared between all players, and not exclusive to any one player. I'm not sure, but you could probably save this to the world's NBTTagCompound using NBTTagCompound worldData = worldObj.getWorldInfo().getTagCompound(); or something like that. then just use worldData.setInt(keyName, value); and worldData.getInt(keyName); I have however never used it, and I'm not sure if this is the right NBTTagCompound to be saving to. It would be something along those lines though, I assume. -
[1.7.10] Trying to make an api and a few question about an api.
shieldbug1 replied to drok0920's topic in Modder Support
This is pretty basic java. An interface method cannot have a body. -
I think you want to use the LivingDropsEvent.
-
Problem with addChatMessage sending twice
shieldbug1 replied to TechieCrow's topic in Modder Support
My guess is that it's being called on the server and the client. Potion effects should only be done server side, so you should put those inside if(!world.isRemote) world.isRemote returns true for the client and false for the server. As for the messages, also put them in an if. Since I think addChatMessage only works for the EntityPlayer itself, I don't think it matters whether it's done server side or client side. Just not both. -
It would cause it to spread to blocks further away. That /could/ count as speeding up. I'm not sure how else. There's a method called tickRate you can override I think. Not sure if it's relevant, I've never used it.
-
You are correct. My mistake
-
Uhm... Unless I'm not getting what you're saying... You're /meant/ to lose your items when you die in Minecraft. That's just part of the game...
-
[1.7.2] world.getblock and check room for spawning
shieldbug1 replied to nightscout01's topic in Modder Support
You could do something like this: public Block[] array = {Blocks.grass, whateverOtherBlocks} and then later to check if it's in the array: boolean flag = false; for(Block block : array) { if(world.getBlock(x, y, z) == block) { flag = true; } } if(!flag) { return; } If you're only using the array once, or it's really small, then you could just use the || (or) operator. I'm not sure what you mean by it not working, but it would look something like Block block = world.getBlock(x,y,z); if(block == Blocks.grass || block == anotherBlock || block == anotherBlockAsWell) { //DO WHATEVER } else { return; } -
[1.7.2] looking for event when NetherPortal is Used
shieldbug1 replied to Jacky2611's topic in Modder Support
You don't need to complicate things so much. PlayerChangedDimensionEvent has a toDim integer that I'm pretty sure is the dimension ID. Just check if the dimension ID is -1 (the nether dimension ID) to make sure the player is going to the Nether. No need for flags or block checks.