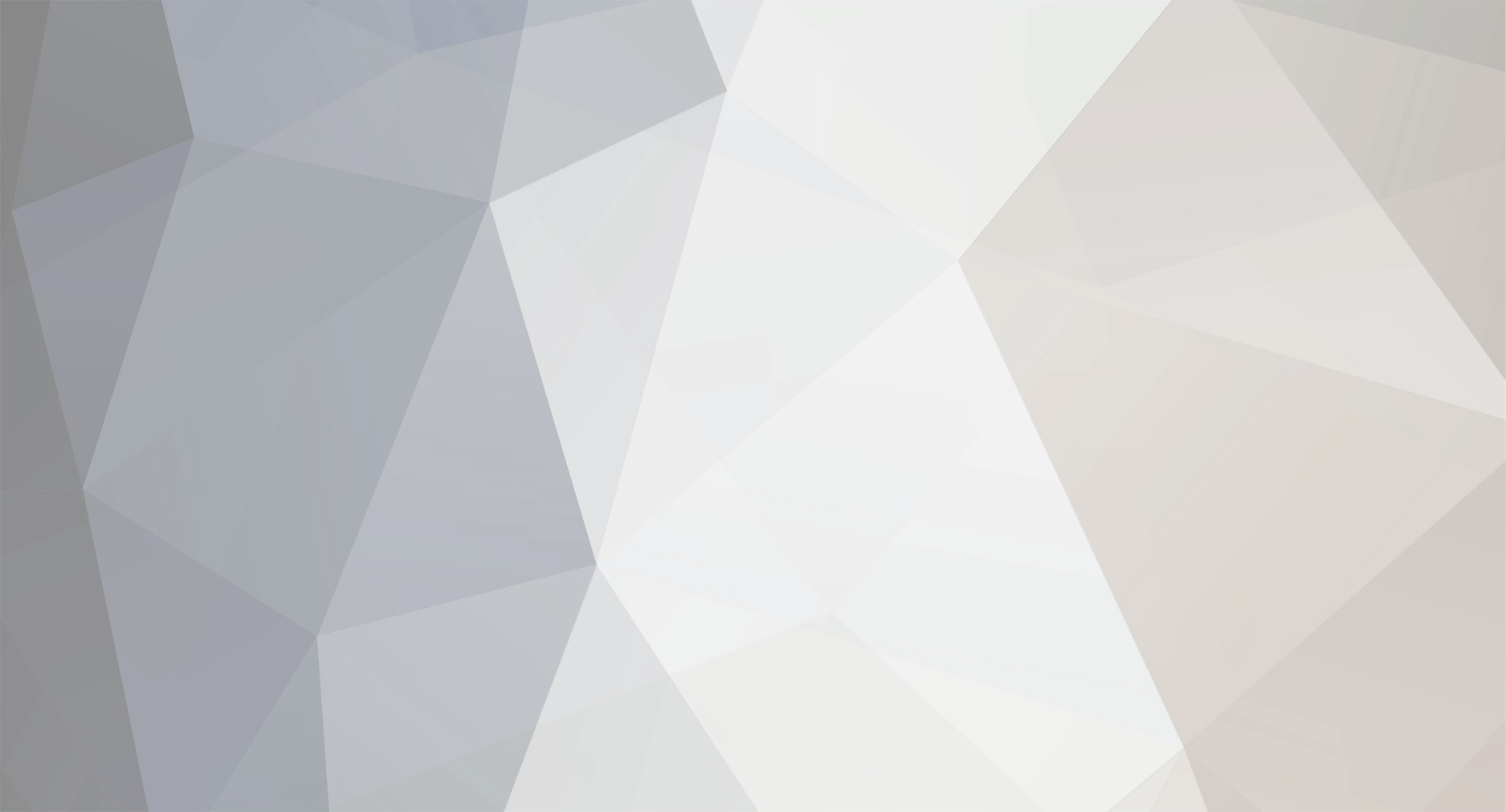
KittenKoder
Members-
Posts
77 -
Joined
-
Last visited
Everything posted by KittenKoder
-
1.12 How do I make my entity or item breathe fire?
KittenKoder replied to TheRPGAdventurer's topic in Modder Support
I don't think it does, but it would be easy to add such a thing. Since the entity has to tick anyway, just add some modifier to the damage that ticks with it. -
1.12 How do I make my entity or item breathe fire?
KittenKoder replied to TheRPGAdventurer's topic in Modder Support
I was talking about using it for the physics logic as it makes sense that there would be a falloff point, unlike the ghast fireballs. It would also need to be dodgeable, would it not? You won't be able to avoid spawning in more entities with something like this, limiting the number of mobs which use it that spawn in at any given point would be a better choice anyway. Another place to check would be Mekanism's flame thrower for the best example of the mechanisms involved. Mekanism has issues, but the flamethrower worked well and used a "spawning in a bunch of entities" method. -
1.12 How do I make my entity or item breathe fire?
KittenKoder replied to TheRPGAdventurer's topic in Modder Support
I would use the arrow logic, as if it was an arrow fired from a bow, but use tiny fires instead of arrows. So I would recommend looking into how the bow and arrows work. -
[Solved] Storing and Retrieving NBT for an Item
KittenKoder replied to shauncjones's topic in Modder Support
I ran into this issue, it was primarily the fact that I didn't have the correct sides or checked the world.isRemote correctly. -
[1.12.2]How does one use Item.getBurnTime on a Block
KittenKoder replied to karrablaster123's topic in Modder Support
I was talking about the penchant for some people to just say "read the docs" when what you mentioned is the case. -
[1.12.2][SOLVED] Issue with update() in tile entity
KittenKoder replied to uncleofbob's topic in Modder Support
From what I see, the problem is that the vanilla engine is not recognizing the modded tickable blocks until they are effected by certain events. The ITickable interface isn't checked until chunk loading, and then added to a special list. But those changes do not seem to take effect immediately after chunk loading for the version I am compiling against either. The only work around I can see is by using scheduleBlockUpdate. If the delay is high enough though, I see no issues that could hurt the mod in any way. -
[1.12.2][SOLVED] Issue with update() in tile entity
KittenKoder replied to uncleofbob's topic in Modder Support
Digging through the Forge and Minecraft sourcecode, registering a tile entity does not automatically register tickers when registering through the registerTileEntity. I find no other references to the list. public static void registerTileEntity(Class<? extends TileEntity> tileEntityClass, String key) { TileEntity.register(key, tileEntityClass); } public static void register(String id, Class <? extends TileEntity > clazz) { REGISTRY.putObject(new ResourceLocation(id), clazz); } public void putObject(K key, V value) { Validate.notNull(key); Validate.notNull(value); this.values = null; if (this.registryObjects.containsKey(key)) { LOGGER.debug("Adding duplicate key '{}' to registry", key); } this.registryObjects.put(key, value); } So far I cannot find when they are registered for the vanilla items. I'm digging into the source code to see if I can find where they are registered as tickables because it isn't happening there. -
[1.12.2] extractItem and insertItem problems for IItemHandler
KittenKoder replied to KittenKoder's topic in Modder Support
I feel like a total moron now. I am trying to transfer an item to the same inventory .... -
The two functions seem to have no replacements and the docs don't point to any. There are no tutorials that show any alternatives for 1.12, all current tutorials still use them. If they are not included, then block states don't work correctly, so am I missing something or are these deprecated just for the sake of deprecating things?
-
I have been working on trying to get inventory items to transfer between things using the new "system" and it just doesn't work no matter what I try. I use the extractItem and nothing changes in the inventory when the simulated variable is set to false. Same with insertItem. If it's an ItemStackHandler instance I can use setStackInSlot in some cases, but not all. For example, this works (inside a block update using scheduled update): if(isdraining && tile.getCurrentFuel() > 0) { tile.consumeFuel(); if(tile.getCurrentFuel() == 0) for(EnumFacing face2 : EnumFacing.values()) this.notifyChange(world, pos, face2); } ItemStackHandler inventory = (ItemStackHandler)tile.getCapability(CapabilityItemHandler.ITEM_HANDLER_CAPABILITY, EnumFacing.UP); if(inventory != null) { ItemStack item = inventory.getStackInSlot(0); Item thing = item.getItem(); int burn = 0; if(thing != null) burn = thing.getItemBurnTime(item); if(!item.isEmpty() && burn != 0) { if(burn < 0) { if(thing instanceof ItemBlock) { Block block = ((ItemBlock)thing).getBlock(); if((block instanceof BlockLog) || (block instanceof BlockPlanks)) burn = 300; else if(block == Blocks.COAL_BLOCK) burn = 16000; } else if(thing == Items.LAVA_BUCKET) burn = 20000; else if(thing == Items.COAL) burn = 1600; else if(thing == Items.BLAZE_ROD) burn = 2400; } if(burn > 0) { if(tile.canConsume(burn)) { tile.increaseFuel(burn); if(item.getItem() == Items.LAVA_BUCKET) { ItemStack nitem = new ItemStack(Items.BUCKET, 1); inventory.setStackInSlot(0, nitem); } else inventory.extractItem(0, 1, false); for(EnumFacing face2 : EnumFacing.values()) this.notifyChange(world, pos, face2); } } } } However in the same update setup, this doesn't work if trying to push a stack into the inventory of a neighboring block. Here's an example with one technique commented out, the other not. Neither technique works: public void updateTick(World world, BlockPos pos, IBlockState state, Random rand) { if(world.isRemote) return; EnumFacing face = state.getValue(FACING); TileEntity tile = world.getTileEntity(pos); TileEntity entity = world.getTileEntity(pos.offset(face)); if((tile instanceof MagitechTileEntityPipe) && entity != null && entity.hasCapability(CapabilityItemHandler.ITEM_HANDLER_CAPABILITY, face.getOpposite())) { System.out.println("Begin Transfer"); ItemStackHandler inventory = (ItemStackHandler)tile.getCapability(CapabilityItemHandler.ITEM_HANDLER_CAPABILITY, EnumFacing.UP); IItemHandler target = tile.getCapability(CapabilityItemHandler.ITEM_HANDLER_CAPABILITY, EnumFacing.UP); boolean done = !(target instanceof ItemStackHandler); for(int i = 0; i < inventory.getSlots() && !done; ++i) { ItemStack test = inventory.extractItem(i, this._speed, true); if(test != null && !test.isEmpty()) { int start = test.getCount(); System.out.println("Got an item " + Integer.toString(start) + " @ " + Integer.toString(i)); test = this.addToInventory(test, (ItemStackHandler)target); int end = test.getCount(); if(end != start) { test = inventory.getStackInSlot(i); if(end > 0) { test = test.copy(); test.setCount(end); inventory.setStackInSlot(i, test); } else inventory.setStackInSlot(i, ItemStack.EMPTY); // inventory.extractItem(i, start - end, false); System.out.println("Item Transfered. " + Integer.toString(end)); tile.markDirty(); entity.markDirty(); done = true; } System.out.println("End Transfer"); } } } world.scheduleBlockUpdate(pos, this, 10, 0); } public ItemStack addToInventory(ItemStack stack, ItemStackHandler target) { int max = target.getSlots(); ItemStack nstack = stack.copy(); int meta = stack.getMetadata(); boolean done = false; for(int i = 0; i < max && !done; ++i) { // nstack = target.insertItem(i, nstack, false); // done = nstack == null || nstack.isEmpty(); ItemStack test = target.getStackInSlot(i); if(test != null && !test.isEmpty()) { Item titem = test.getItem(); int tmeta = test.getMetadata(); if(test.getMaxStackSize() > test.getCount() && titem == nstack.getItem() && meta == tmeta) { int count = Math.min(test.getCount() + nstack.getCount(), test.getMaxStackSize()) - test.getCount(); ItemStack place = test.copy(); place.grow(count); target.setStackInSlot(i, place); System.out.println("Put item into place. " + Integer.toString(i) + " " + place.getItem()); if(count >= nstack.getCount()) { nstack = ItemStack.EMPTY; done = true; } else nstack.shrink(count); } } else { target.setStackInSlot(i, nstack); nstack = ItemStack.EMPTY; done = true; } } return nstack; } In what way would one accomplish transferring items from one block to another using this system?
-
[1.12.2]How does one use Item.getBurnTime on a Block
KittenKoder replied to karrablaster123's topic in Modder Support
The problem is that the javadocs for Minecraft are almost non-existent, and the ones for Forge are very incomplete and lacking in any examples. Not to mention much of the explanations for the methods are vague and don't offer any insight into what you should do for functionality, once in a while we at least see a "don't do this." -
I can't remember how I first learned coding, it's been that long. Back then it was called programming, then it was called software engineering. Today I keep up with forums and tutorials because no one seems to like to create decent docs for their APIs these days.
-
I will confess that I am not use to socializing with humans, but I did not imply "no, your solution is wrong, go away". I needed the containers, which #4 does not explain ergo it did not help. The solution was to subclass the Container class so that there was less added code, thus reducing the amount of bloat to the mod. Though the list is helpful, it does not offer much information as to what to do instead, much less how to avoid writing entirely new systems for doing things. Nothing personal, but it seems that Draco doesn't like to say much and that makes his advice less useful at times. Again, without explaining why or at least offering an explanation of what to do instead, no one else will learn anything. I am guessing that this is why the latest versions of Forge are often treated with disdain.
-
I asked what was wrong with using the class.
-
Why should be implied, should it not? How would you expect someone to learn what their actual mistake was if they don't know why? There are other classes that would cause the same problem, I have discovered these classes only because I figured out why BlockContainer was a bad class. I am developing this mod in the hopes of understanding how Minecraft functions, what makes it tick, so I can develop a game that doesn't make the same mistakes while utilizing concepts that work. Forge may be prematurely deprecating things, but it does correct some issues Minecraft has so knowing how it functions also helps. The mod is a happy side effect of my studies.
-
If you would read my posts you'd realize I didn't want to fix it, I knew how to do that already. I wanted to know why it was happening, I wanted to know the code that was causing it so I could avoid it in the future in case it effects things not yet mentioned by anyone else. The idea of fixing just one thing at a time without knowing why won't prevent future issues.
-
Turning Abandoned Mineshaft generation off in 1.12
KittenKoder replied to kingdadrules's topic in Modder Support
Go into the world generators and see how Minecraft does it. That's your best solution here. The current version of Forge was updated without any documentation and most of the tutorials out there are outdated to the point of breaking things. Specifically in the net.minecraft.world.gen package and subpackages. You'll likely need to add your own world generator and then register that in the list as an option for it to work, you can't override the vanilla one much. -
I knew that part. I was trying to see what the BlockContainer was doing to cause rendering to fail. I had to really dig through the code to figure it out though. The problem I am facing is that being new to modding for Minecraft while also being an old school Java developer, Minecraft is a total mess. Not to mention that the new capability system is not a Java convention, interfaces are still superior to such a thing because of native code implementations. Essentially, before I get too far into the mod itself, I want to know what Minecraft and Forge do and how I can avoid the pitfalls that most modern mods seem to suffer. Since there is little documentation and no tutorials out there I have to drill deep for answers and just hope that someone else could help speed the process along. This particular issue had me baffled until I dug into the TESRs and discovered that the BlockContainer code somehow alters the model data. I need to dig a bit deeper to know why it is being removed completely though, as opposed to having the flaws corrected or compatibility improved. My set of MagitechBlockPartial classes uses true Java conventions to accomplish much of what most of the Block subclasses in Minecraft do without all the mucking about with TESRs and using a minimal number of subclasses. My next goal is to understand what changed in the bounding boxes and how to use the stuff that's already implemented without too much headache. I'm all about reusing the code that's present versus making new stuff, but once in a while I am forced to just work from scratch, as was the case here. That did not help answer my question.
-
Update: It appears that vanilla is still using the BlockContainer class and has a TESR attached to it. The entire reason interfaces were developed in Java was to prevent this mess of code that Forge and Minecraft are showing in 1.12. Ironic that the interfaces are being replaced with a system that will result in massive overhead and serious synchronization problems between Java objects, but hey, I don't plan to go past 1.12 ever anyway.
-
That was done from the start, so it is not the problem. The model wouldn't load at all if that was not already done.
-
Okay, are you not reading anything I posted or just the first few words? I do that, it loads the model, it even renders correctly in hand and in the inventory. But when placed it won't render at all without a custom renderer and even then the UV coordinates are wrong. My question is why and how do I fix it? This is suppose to be a block you place, not something you carry, but when you PLACE it the rendering fails to function as expected. All model/block registering is done through the event system (which lacks in documentation and took a full day just to find the names of some classes for that) and the OBJ loader is made aware of the mod. The registering calls are all shown in the primary class, so what did I miss? What could cause the model to work correctly in hand and inventory but not when placed, as in PLACED AS A BLOCK.
-
Exactly, but it uses a custom renderer that I pieced together by digging through Vanilla and old code, I can't find enough information on the methods to eve know if it's correct. Also I would prefer not to use the custom renderer. public void render(MagitechTileEntityStorageBinTall te, double x, double y, double z, float partialTicks, int destroyStage, float alpha) { Tessellator tessellator = Tessellator.getInstance(); BufferBuilder bufferbuilder = tessellator.getBuffer(); BlockPos pos = te.getPos(); IBlockAccess world = MinecraftForgeClient.getRegionRenderCache(te.getWorld(), pos); IBlockState state = world.getBlockState(pos); // bindTexture(TextureMap.LOCATION_MISSING_TEXTURE); bindTexture(TEXTURE); bufferbuilder.begin(7, DefaultVertexFormats.BLOCK); bufferbuilder.setTranslation((x - (double)pos.getX()) + 0.5, y - (double)pos.getY(), (z - (double)pos.getZ()) + 0.5); IBakedModel model = FMLClientHandler.instance().getClient().getBlockRendererDispatcher().getModelForState(state); FMLClientHandler.instance().getClient().getBlockRendererDispatcher().getBlockModelRenderer().renderModel(world, model, state, pos, bufferbuilder, false); bufferbuilder.setTranslation(0.0D, 0.0D, 0.0D); tessellator.draw(); } As you can see, it's a mess.
-
-
Another huge problem with the new methods is that it's not proper Java.