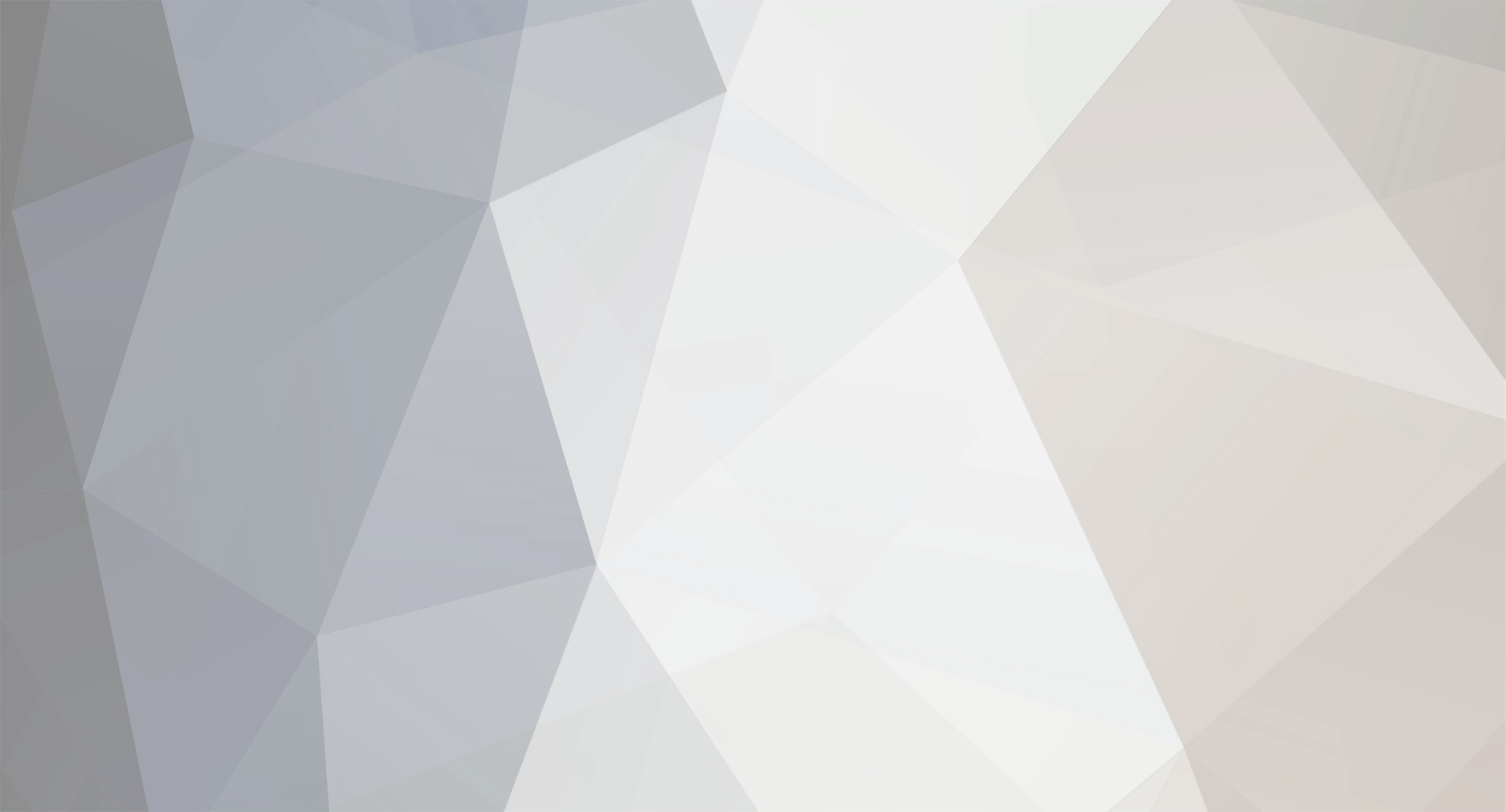
uncleofbob
-
Posts
21 -
Joined
-
Last visited
Posts posted by uncleofbob
-
-
Hi again! It's working now! It was all related to how I used the position wrong.
Somehow update() ran when the block was placed in certain quadrants related to the first block i placed. This made me change the way I get my Tile entity pos.
Now I just access "this.getPos()" in methods that need it. The onLoad() wasn't necessary either.
And yes, I scrapped the scheduleBlockUpdate workaround!
Again, thank you all for replies and using your time on my problem!
-
1 hour ago, Draco18s said:
Why the hell are you storing your TE's position? TEs already do this, that's what getPos() does.
Because Im obviously a n00b ?.
-
1
-
-
Can it be ? Have I solved my problem ?!
I've added the following method to my tile entity, and update() hasn't failed yet!
@Override public void onLoad() { posB = this.getPos(); }
This code changes the way I get the BlockPos, which i used to get from a set method in tile entity i called from onBlockPlacedBy.
-
7 hours ago, jabelar said:
Well it is a "cheat" in the sense that ITickable should not need any such silliness. Think about it -- a furnace happily cooks something as an ITickable and any animated tile entity is happily updated every tick. You should really find the root cause of the problem. But if it works I guess use it. Something is still wrong with your implementation in my opinion though and it might bite you later.
When I get deeper in to modding, I'll probably be able to find the root cause of my problem. Now i just want to continue my mod development.
Thank you all for help !
-
1
-
-
--------------------------------------------
Not a solution!
---------------------------------------------
So I've done like @KittenKodersuggested:
Is this cheat ?
@Override public void onBlockPlacedBy(World worldIn, BlockPos pos, IBlockState state, EntityLivingBase placer, ItemStack stack) { TileEntity tileentity = worldIn.getTileEntity(pos); if (tileentity instanceof JdwOSDigger) { ((JdwOSDigger)tileentity).setPos(pos); worldIn.scheduleBlockUpdate(pos, this, 20, 1); // schedule first update } } @Override public void updateTick(World worldIn, BlockPos pos, IBlockState state, Random rand) { if(!worldIn.isRemote) { TileEntity tileentity = worldIn.getTileEntity(pos); if (tileentity instanceof JdwOSDigger){ ((JdwOSDigger)tileentity).jdwUpdate(); //call jdwupDate in tile entity worldIn.scheduleBlockUpdate(pos, this, 20, 1); //schedule next update. } } }
-
1
-
-
I've added "-clean" at the first line in eclipse.ini. This wipes out some eclipse runtime cache which seem to cause the problem.I'll put the thread to [SOLVED] when I'm 100% sure. -
I've now checked the lists: "tickableTileEntities" and "loadedTileEntityList" while right clicking on block. In combination I ran the Chunk.getTileEntityMap() to see if its found in the chunk. The number (id?) after the tile entity also changes when I destroy the block and place a new, which I assume states that it detects a new instance of my JdwOSDigger tile entity.
[12:52:18] [..onBlockActivated:86]: from Chunk: {BlockPos{x=-125, y=66, z=224}=com.jolge.derpworld.tileentity.JdwOSDigger@af23ab1}
[12:52:18] [.....onBlockActivated:90]: loadedTileEntityList[21]: com.jolge.derpworld.tileentity.JdwOSDigger@af23ab1
[12:52:18] [....onBlockActivated:97]: tickableTileEntities[21]: com.jolge.derpworld.tileentity.JdwOSDigger@af23ab1
[12:52:33] [....onBlockActivated:86]: from Chunk: {BlockPos{x=-125, y=66, z=224}=com.jolge.derpworld.tileentity.JdwOSDigger@2979b61f}[12:52:33] [....onBlockActivated:90]: loadedTileEntityList[21]: com.jolge.derpworld.tileentity.JdwOSDigger@2979b61f
[12:52:33] [....onBlockActivated:97]: tickableTileEntities[21]: com.jolge.derpworld.tileentity.JdwOSDigger@2979b61f
I'll import my mod to a new eclipse environment later, to see if it helps.
-
And again..
There must be something wrong with my Modding environment.
I restarted the game 10 times to verify that update() worked..
Only to find out it stopped working after a while...
-
1
-
-
[IGNORE THIS POST!!]]
Finally! It seems i've found the issue in my code.@diesieben07 was right stating that the tile entity didn't register properlySe comments below// I think the registered tile entity excluded ITickable when writing <?extends TileEntity> public static void registerTileEntity(final Class<?extends TileEntity> tileEntityClass, final String name) { GameRegistry.registerTileEntity(tileEntityClass, name); } // when I removed it, it seems to run as I want. I assume it just takes whatever the class extends and implements. public static void registerTileEntity(final Class tileEntityClass, final String name) { GameRegistry.registerTileEntity(tileEntityClass, name); }
-
1
-
-
Thx ! So its schedualBlockUpdate in onBlockAdded, and override the updateTick method? Currently Im testing with every 20 ticks, but it may even be slower. Thx again!
-
Now I register the Block, ItemBlock and TileEntity directly in RegistryHandler. Update() still won't run.
Guess I'll have to dig deeper !
-
Could it be related to my tile entity class? Maybe the instantiation of the tile entity ?
-
Now I'm doing it like this:
@EventHandler public void preinit(FMLPreInitializationEvent event) { GameRegistry.registerTileEntity(JdwOSDigger.class, Reference.MODID + "oilsanddigger"); }
-
My bad! Seems when i refresh or do some changes to the block class it works the first time I start a new world.
hmm..
-
Found the problem!Forgot to Override Block#updateTick in the block classTHx for all responses!EDIT: MY BAD NO FIX!
-
I sort of copied the way its done in TestMod3:
Changed to preInit now, but still no update() running.
-
Hi!
A Quick (2 sec) look at the vanilla code:
EntityAIFIndEntityNearest.class
EntityAIFindEntityNearestPlayer.class
-
Thanks for the quick replies! I think the tile entity gets registered. I can call methods from onBlockActivated which works fine.
Is there something wrong with the tile entity constructor maybe?
My RegistryHandler.class : Should I register right after Blocks instead?
package com.jolge.derpworld.util; import com.jolge.derpworld.init.BlockInit; import com.jolge.derpworld.init.ItemInit; import com.jolge.derpworld.tileentity.JdwOSDigger; import net.minecraft.block.Block; import net.minecraft.item.Item; import net.minecraft.tileentity.TileEntity; import net.minecraftforge.client.event.ModelRegistryEvent; import net.minecraftforge.event.RegistryEvent; import net.minecraftforge.fml.common.Mod.EventBusSubscriber; import net.minecraftforge.fml.common.eventhandler.SubscribeEvent; import net.minecraftforge.fml.common.registry.GameRegistry; import net.minecraftforge.registries.IForgeRegistry; @EventBusSubscriber public class RegistryHandler { @SubscribeEvent public static void onBlockRegister(RegistryEvent.Register<Block> event) { event.getRegistry().registerAll(BlockInit.BLOCKS.toArray(new Block[0])); } @SubscribeEvent public static void onItemRegister(RegistryEvent.Register<Item> event) { event.getRegistry().registerAll(ItemInit.ITEMS.toArray(new Item[0])); registerTileEntities(); } @SubscribeEvent public static void onModelRegister(ModelRegistryEvent event) { for(Item item : ItemInit.ITEMS) { if(item instanceof IHasModel) { ((IHasModel)item).registerModels(); } } for(Block block : BlockInit.BLOCKS) { if(block instanceof IHasModel) { ((IHasModel)block).registerModels(); } } } public static void registerTileEntities() { GameRegistry.registerTileEntity(JdwOSDigger.class, Reference.MODID + "oil_sand_digger"); System.out.println("Registered: oil_sand_digger"); }
-
I have done some tests with the NBT methods. update() still doesn't seem to run.
Should NBT be the case when I create a new world and update() doesn't work?
public void readFromNBT(NBTTagCompound compound) { super.readFromNBT(compound); //his.note = compound.getByte("note"); this.isActivated = compound.getBoolean("Status"); this.tickCounter = compound.getInteger("count"); this.index = compound.getInteger("indexz"); } public NBTTagCompound writeToNBT(NBTTagCompound compound) { super.writeToNBT(compound); //compound.setByte(); compound.setInteger("indexz", this.index); compound.setInteger("count", this.tickCounter); compound.setBoolean("Status", this.isActivated); return compound; }
-
-------------------------------------------------------------------------------------------------------------------
SOLVED:
Long story short, I messed up accessing the Tile Entity Pos for each instance.
The solution was to scrap the setPos() method (which I first of all should have renamed), and use this.getPos() whenever I needed the Tile Entity pos.
I already knew about getPos() but it seems I implemented it wrong, cause it always returned BlockPos(0,0,0).
In Block I removed everything inside onBlockPlacedBy
In Tile Entity I removed the setPos() method and the posB field.
And as example I now use: mapQuarry(5, this.getPos())
---------------------------------------------------------------------------------------------------------------------
Hi!
I'm very new to modding in Minecraft. I got stuck when changing from calling TE-method from the Block's "onBlockActivated", to using update() instead.
The goal is to "world.destroyBlock" at BlockPos specified in AREA<BlockPos> every 20 ticks. This worked if I just called a TE-method from onBlockActivated and looped through AREA (although this happens instantly).
I'm obviously missing something, as update() runs pretty randomly when i restart the game.
I'll be happy if i just get the world.destroyBlock to start removing blocks in update().
Block class:
package com.jolge.derpworld.block; import java.util.Random; import com.jolge.derpworld.DerpWorld; import com.jolge.derpworld.init.BlockInit; import com.jolge.derpworld.init.ItemInit; import com.jolge.derpworld.tileentity.JdwOSDigger; import com.jolge.derpworld.util.IHasModel; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.block.state.IBlockState; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.Item; import net.minecraft.item.ItemBlock; import net.minecraft.item.ItemStack; import net.minecraft.stats.StatList; import net.minecraft.tileentity.TileEntity; import net.minecraft.tileentity.TileEntityFurnace; import net.minecraft.util.EnumFacing; import net.minecraft.util.EnumHand; import net.minecraft.util.math.BlockPos; import net.minecraft.world.World; public class JdwBlockBitumen extends Block implements IHasModel{ public JdwBlockBitumen(String name, Material material) { super(material); setUnlocalizedName(name); setRegistryName(name); setCreativeTab(CreativeTabs.MISC); setHarvestLevel("pick_axe", 1); BlockInit.BLOCKS.add(this); ItemInit.ITEMS.add(new ItemBlock(this).setRegistryName(this.getRegistryName())); } @Override public boolean hasTileEntity(IBlockState state) { return true; } @Override public TileEntity createTileEntity(final World worldIn, final IBlockState state) { return new JdwOSDigger(); } @Override public void onBlockAdded(World worldIn, BlockPos pos, IBlockState state) { } @Override public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumHand hand, EnumFacing facing, float hitX, float hitY, float hitZ) { if (!worldIn.isRemote ) { final JdwOSDigger tileEntity = (JdwOSDigger) worldIn.getTileEntity(pos); if(tileEntity != null) { tileEntity.toggleActive(); } } return true; } @Override public void onBlockPlacedBy(World worldIn, BlockPos pos, IBlockState state, EntityLivingBase placer, ItemStack stack) { TileEntity tileentity = worldIn.getTileEntity(pos); if (tileentity instanceof JdwOSDigger) { ((JdwOSDigger)tileentity).setPos(pos); } } @Override public void registerModels() { DerpWorld.proxy.registerItemRenderer(Item.getItemFromBlock(this), 0, "inventory"); } }
Tile Entity class:
package com.jolge.derpworld.tileentity; import java.util.ArrayList; import java.util.List; import java.util.Random; import net.minecraft.block.Block; import net.minecraft.block.BlockDirt; import net.minecraft.block.BlockGrass; import net.minecraft.block.state.IBlockState; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.ITickable; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.MathHelper; import net.minecraft.world.World; import scala.actors.threadpool.Arrays; public class JdwOSDigger extends TileEntity implements ITickable{ public boolean isActivated; public BlockPos posB; public List<BlockPos> AREA = new ArrayList<BlockPos>(); public int radi = 0; public int index = 0; public int tickCounter = 0; public JdwOSDigger() { super(); } public void setPos(BlockPos pos) { this.posB = pos; } @Override public void update() { if (!world.isRemote){ if(isActivated) { tickCounter++; if (tickCounter==20) { world.destroyBlock(AREA.get(index), false); System.out.println("Destroyed block at: " + AREA.get(index) ); index++; tickCounter = 0; } } } } public void toggleActive() { if (this.isActivated) { this.isActivated = false; } else { this.isActivated = true; mapQuarry(5, this.posB); } } public void mapQuarry (int radi, BlockPos pos) { List<BlockPos> l = new ArrayList<BlockPos>(); int[] squares = new int[radi]; BlockPos qC = null; //Quadrant Center int bx, by, bz = 0; for(int k = 0; k<4; k++) { switch(k){ case 0: qC = pos.add(radi+1, 0, radi+1); //+X+Z = NorthEast = 1 break; case 1: qC = pos.add(-(radi+1), 0, radi+1); //-X+Z = NorthWest = 2 break; case 2: qC = pos.add(radi+1, 0, -(radi+1)); //+X-Z = SouthEast = 3 break; case 3: qC = pos.add(-(radi+1), 0, -(radi+1)); //-X-Z = SouthWest = 4 break; } for(int e = 0; e<radi/2; e++) { for(int i = -radi+e; i <= radi-e; i++) { for (int j = -radi+e; j<=radi-e; j++) { bx = qC.getX() + i; bz = qC.getZ() + j; by = qC.getY() - 1 - e; BlockPos pos2 = new BlockPos( bx , by, bz ); l.add(pos2); } } } } AREA.addAll(l); } }
[1.12.2][SOLVED] Issue with update() in tile entity
in Modder Support
Posted
I know the names of block and tile entity class is a bit odd. And my use of variables could be less. It will be changed.
Block:
Tile Entity: