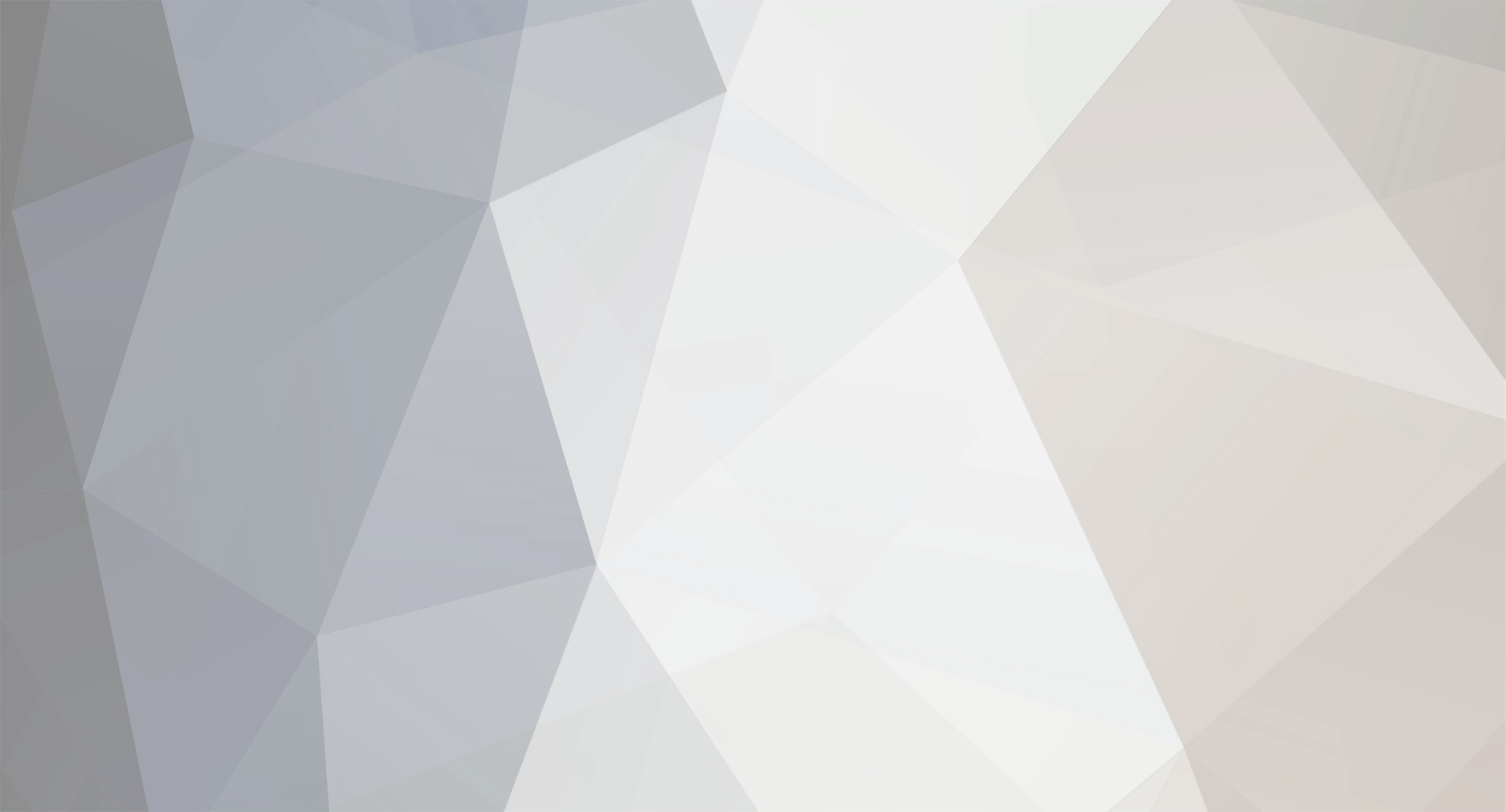
Keitaro
Members-
Posts
38 -
Joined
-
Last visited
Everything posted by Keitaro
-
Since the IRenderFactory just provides a renderer, you can use lambda expressions. Like this: RenderingRegistry.registerEntityRenderingHandler(EntityRed.class,(RenderManager manager) -> new RenderRed(manager)); It's still one line per Entity, but i think it doesn't get shorter.
-
That exception is unrelated, it just tells you you couldn't log in, since you normally don't use an account inside your development setup. I think there is a way to use your mojang account, but it's not really needed. If you have code depending on names you can just add -username NICK to your run configuration to test that stuff. Your crash should have another reason.
-
Ah, you have your models and textures folders on the same level as the assets folder, not inside assets/modid.
-
For 1.12 the model folders are called blocks and items and not block and item. My bad, was wrong.
-
[SOLVED] [1.12.2] Rendering custom layers on players
Keitaro replied to FlashHUN's topic in Modder Support
Looking at the image, the translation seems to be fine. You just have to GlStateManager.scalef(0.f,2f,0f) to increase the height of the overlay. Maybe you have to translate it down more after that, can't remember if you count from the top or the bottom. It would look steched, so maybe you can just replace the texture with a square in that instance. But not to burst your bubble, if someone has a custom Skin which has bigger eyes from the beginning or something like that, your overlay will be out of place anyway. -
[SOLVED] [1.12.2] Rendering custom layers on players
Keitaro replied to FlashHUN's topic in Modder Support
But that's the clients player. He needs to know the player who send the package. I used the ctx.getServerHandler().player for my CAPABILITY, but since it was nothing visual, i am not sure if that's the right one. -
[SOLVED] [1.12.2] Rendering custom layers on players
Keitaro replied to FlashHUN's topic in Modder Support
Not for every player. But let's say 'Alice' changes her 'mana' to 'true' and 'Bob' is tracking her, so 'Bob' gets a package with the data 'true'. Since 'Bob's client is the one receiving the package 'Minecraft.getMinecraft().player' is 'Bob' and not 'Alice'. So 'Bob's client sets the 'mana' of 'Bob' to 'true' and not of 'Alice'. IIRC i got the player out of the MessageContext the last time i used this and not from the Minecraft instance. -
Just DON'T use instances of your blocks, items and entities when you register them. Use ObjectHolder and their RegistryName. For example, i would use it like this: public class EntityInit { @ObjectHolder(Reference.MOD_ID + ":entity_white_face") public static EntityType<EntityWhiteFace> WHITE_FACE; public static void register(IForgeRegistry<EntityType<?>> registry) { registry.register(EntityType.Builder.create(EntityWhiteFace.class, EntityWhiteFace::new).build(Reference.MOD_ID + ":entity_white_face").setRegistryName(Reference.MOD_ID, "entity_white_face")); } } And just call that register function. @SubscribeEvent public static void registerEntities(final RegistryEvent.Register<EntityType<?>> event) { EntityInit.register(event.getRegistry()); } For another entity you just add a ObjectHolder and copy&change the registry.register call. Not sure if that's best practice or not, but so i have all my blocks, items and entites inside one class. PS: I think @ObjectHolder(Reference.MOD_ID + ":entity_white_face") public static final EntityType<EntityWhiteFace> WHITE_FACE = null; is the recommended definition.
-
[SOLVED] [1.12.2] Rendering custom layers on players
Keitaro replied to FlashHUN's topic in Modder Support
If i am not totally mistaken and handleManaB is the Package all your Clients receive, than you set the Dojutsu state for the players themself every time somebody changes it and they never know about the original player who changed it. Somebody correct me if i am wrong. -
Item name and texture won't work correctly [SOLVED]
Keitaro replied to Dr.Nickenstein's topic in Modder Support
Sometimes you have to refresh the folders within eclipse, otherwise the files don't get recognized. I don't use eclipse, but i think it's just a rightclick on the folder/package and there should be refresh or something. Intelij calls it sync. The name is set inside your language file, for english it should be assets/yourmodid/lang/en_US.lang, just add item.(name of the ore)_ingot.name=Display name. -
I lock at https://files.minecraftforge.net/maven/de/oceanlabs/mcp/mcp_snapshot/ for the latest version. The log should tell you the urls gradle tries to download from and you can check with the ones of that page. Last time i had a problem i just changed the date and forgot to change the minecraft version as well.
-
how to reduce the damage you receive if you have an item in inventory
Keitaro replied to Ragnar's topic in Modder Support
You can subscribe to the LivingHurtEvent and check the players inventory. Something like this should work: @SubscribeEvent public static void onPLayerHurtEvent(LivingHurtEvent event) { Entity entity = event.getEntity(); if (entity instanceof EntityPlayer) { EntityPlayer player = (EntityPlayer) entity; float damage = event.getAmount(); for (ItemStack stack : player.inventory.mainInventory) { if(stack.getItem() instanceof ItemAppleGold){ damage = damage/2; } } } } This should reduce the damage by half if you got a golden apple in your inventory. -
I'm working on a chest with a scrollbar and visually it work's fine, but after i scroll the inventory, all slots have the index 0 if clicked. I think my idea of how to update the inventory is wrong or i am missing something. I update the slots of my container, based on a rowOffset for the inventory. This is the function of the container: private void updateSlots() { if(this.te.hasCapability(CapabilityItemHandler.ITEM_HANDLER_CAPABILITY, null)) { IItemHandler inventory = te.getCapability(CapabilityItemHandler.ITEM_HANDLER_CAPABILITY, null); int slotOffsetPlayer = 4 * 9; // Update own slots for (int row = 0; row < CHEST_ROWS; ++row) { for (int col = 0; col < CHEST_COLUMNS; ++col) { int x = CHEST_OFFSET_X + col * 18; int y = CHEST_OFFSET_Y + row * 18; this.inventorySlots.set(slotOffsetPlayer + (col + row * CHEST_COLUMNS), new SlotItemHandler(inventory, col + (row + rowOffset) * CHEST_COLUMNS, x, y)); } } } } Like i said the content get's displayed correctly, but whichever slot i click, i always interact with the slot with index 0. I changed the order in which the slots are added to the container from Chest, Player, Hotbar to Hotbar, Player, Chest and now i can still interact with the player's inventory and hotbar. But all slots i changed are inaccessible. In my first attempt i used a second inventory, which held the visible inventory and synced it back and forth with the whole inventory. But i didn't use capabilities for this, and switched after i read that by using the ItemHandlerCapability i do not have to sync the client manually with the server via packets. Because of that i rewrote the whole container and tried it first without the second inventory. Am i just missing something after i update the slots or do i still need the second temporary inventory?