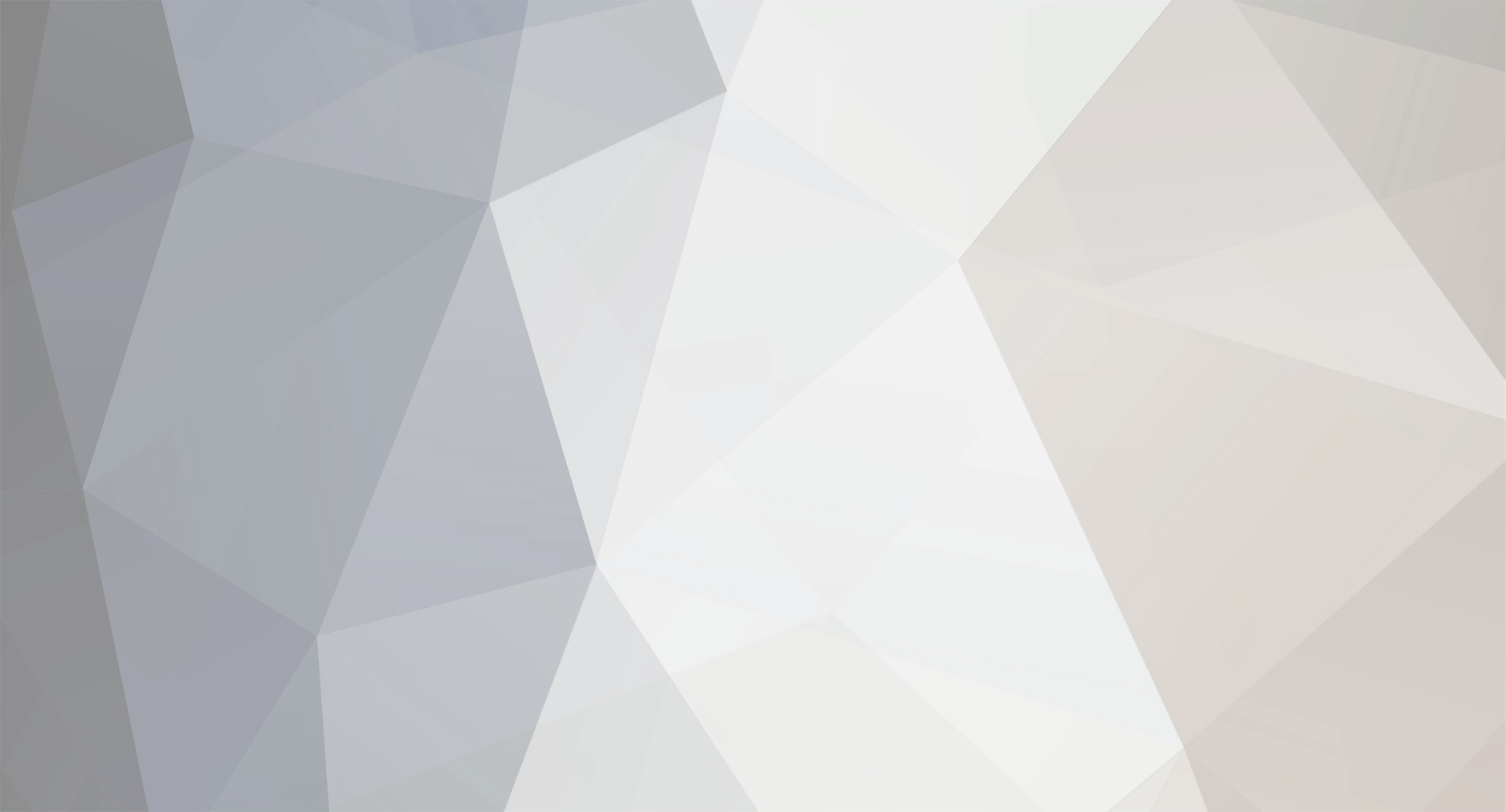
unassigned
Members-
Posts
103 -
Joined
-
Last visited
-
Days Won
1
Everything posted by unassigned
-
Hello, I have an item that I want to have different types of that holds every ingot registered in the game. As in, I want it to have subtypes of every registered ingot that has currently been loaded. However, I do not know how to access this information. I'd also like for this to have configurability, as in, I'd like the user to add (or remove) their own subtype rather easily by modifying a list in config. I know how to add these subtypes, however, I do not know how to automatically them from registered items within forge. Thanks.
-
haha, I was just trying to get a visual, I meant to change this with for loop before posting, but I wanted to see if there was anything better functional wise (ex. more optimized). However, for those interested here is what it looks like now: public boolean matches(ItemStack in, ItemStack infuser1, ItemStack infuser2, ItemStack infuser3, ItemStack infuser4, ItemStack infuser5,ItemStack infuser6,ItemStack infuser7,ItemStack infuser8){ if (!input.apply(in)) return false; List<Ingredient> matches = new ArrayList<>(); ItemStack[] stacks = { infuser1, infuser2, infuser3, infuser4, infuser5, infuser6, infuser7, infuser8 }; Ingredient[] ingredients = { modifier1, modifier2, modifier3, modifier4, modifier5, modifier6, modifier7, modifier8 }; boolean[] unused = { true, true, true, true, true, true, true, true }; for (ItemStack s : stacks) { for(int i = 0; i < ingredients.length; i++) { if(unused[i] && ingredients[i].apply(s)){ matches.add(ingredients[i]); unused[i] = false; } } } return matches.size() == 8; } Thanks.
-
Okay, I went against what you said (even though it's probably better) and ended up switching to use ingredients, made an actual class that handles the items, and it is working now. However, I'd like to post my result to see how this can be improved, as I'm sure by the way it looks, there is a better way. if (!input.apply(in)) return false; List<Ingredient> matches = new ArrayList<>(); ItemStack[] stacks = { infuser1, infuser2, infuser3, infuser4, infuser5, infuser6, infuser7, infuser8 }; boolean[] unused = { true, true, true, true, true, true, true, true }; for (ItemStack s : stacks) { if (unused[0] && modifier1.apply(s)) { matches.add(modifier1); unused[0] = false; } else if (unused[1] && modifier2.apply(s)) { matches.add(modifier2); unused[1] = false; } else if (unused[2] && modifier3.apply(s)) { matches.add(modifier3); unused[2] = false; } else if (unused[3] && modifier4.apply(s)) { matches.add(modifier4); unused[3] = false; } else if (unused[4] && modifier5.apply(s)) { matches.add(modifier2); unused[4] = false; } else if (unused[5] && modifier6.apply(s)) { matches.add(modifier3); unused[5] = false; } else if (unused[6] && modifier7.apply(s)) { matches.add(modifier4); unused[6] = false; } else if (unused[7] && modifier8.apply(s)) { matches.add(modifier4); unused[7] = false; } } return matches.size() == 8; As I said, I went from supplying an array of ItemStack for the ingredients, to just having 8 separate ingredients and checking them.
-
This is considered a shapless recipe, as the order does not matter. (including within the list) Yes, if there is an item that does not belong, the process should be stopped.
-
Well, I have to remove the result, otherwise, it will not know if the duplicate items have been checked, thus 1 item would throw the counter up and return true.
-
Yes, as long as there are 1 or more nether star and 2 or more iron blocks. Here is the recipe being registered
-
Well, they are all 1 in size. How this works is that it gets a list of items from surrounding 'infusers', which can only hold one item. So I need to compare the lists against each other, to make sure each element matches (excluding order). However, as standard collection sorting doesn't work on ItemStacks, I'm stumped.
-
Hello, I have two ItemStack lists of the same length (that CAN contain ItemStack.Empty), and I want to compare them and see if they are the same. This list can carry duplicates of items. I have some base functionality working, however, with my current code, all duplicates within the recipe are accounted for as one. For example, one of my recipes require 6x Iron Blocks, and 1x Nether Star, however, if the list has 2x Iron Blocks and the 1x Nether Star, it will run. Here is an example of the lists I am comparing: Current Items: 1xtile.blockIron@0 1xtile.blockIron@0 1xtile.blockIron@0 0xtile.air@0 1xitem.netherStar@0 1xtile.blockIron@0 1xtile.blockIron@0 1xtile.blockIron@0 Needed Items: 1xitem.netherStar@0 1xtile.blockIron@0 1xtile.blockIron@0 1xtile.blockIron@0 1xtile.blockIron@0 1xtile.blockIron@0 1xtile.blockIron@0 0xtile.air@0 Here is the current comparison code I have: int matches = 0; Iterator<ItemStack> iteratorRecipe = recipeList.iterator(); for(ItemStack ingredient : infusionItems) { while(iteratorRecipe.hasNext()){ ItemStack recipeReqire = iteratorRecipe.next(); if(ItemStack.areItemStackTagsEqual(recipeReqire, ingredient)){ iteratorRecipe.remove(); matches++; } } } And here is the whole method for those who need it: Thanks!
-
Recommended method of storing Entities and TileEntities
unassigned replied to Lord_Lorden's topic in Modder Support
You could have a capability that is saved to the player (or item) that stores the blocks/tiles position and IBlockState (as in a map). For tiles, you'd also have to save its NBT to make sure that everything within it is saved. For entities, you'd have to also have to store what type of entity it is as well as its position. So when the player does x (say destroy a block), get the capability off the player, add the block to the map, and if needed, the NBT, and store it for later. Then, say the player right-clicks a block with some item - grab the capability off the player, access the map, and then iterate through all positions/states (this is also where you'd offset the positions of the blocks relative to the players) and apply needed NBT. Hope this somewhat helps. -
Hello, I have a custom particle that has a custom texture, however, the texture always seems to be missing and just displays as pink/black. Here is the code for the particle: @SideOnly(Side.CLIENT) public class ParticleInfuse extends Particle { public static final ResourceLocation TEXTURE = new ResourceLocation(VoidUtils.MODID, "particles/infuse"); private double[] args; public ParticleInfuse(World world, double x, double y, double z, double xOff, double yOff, double zOff, double... args){ super(world, x+xOff, y+yOff, z+zOff, 0, 0, 0); TextureMap map = Minecraft.getMinecraft().getTextureMapBlocks(); this.setParticleTexture(map.getAtlasSprite(TEXTURE.toString())); System.out.println(TEXTURE.toString()); this.args = args; this.particleMaxAge = 30; //1.5 seconds this.particleScale *= 1; } @Override public void renderParticle(BufferBuilder buffer, Entity entityIn, float partialTicks, float rotationX, float rotationZ, float rotationYZ, float rotationXY, float rotationXZ) { super.renderParticle(buffer, entityIn, partialTicks, rotationX, rotationZ, rotationYZ, rotationXY, rotationXZ); } @Override public void onUpdate() { this.prevPosX = this.posX; this.prevPosY = this.posY; this.prevPosZ = this.posZ; if (this.particleAge++ >= this.particleMaxAge) { this.setExpired(); } if(args.length == 3){ double toX = this.args[0]; double toY = this.args[1]; double toZ = this.args[2]; double moveFactor = 0.125d; this.posX += (toX - this.posX)*moveFactor; this.posY += (toY - this.posY)*moveFactor; this.posZ += (toZ - this.posZ)*moveFactor; } } @Override public int getFXLayer() { return 1; } } Highlighting: In the constructor, I'm using: TextureMap map = Minecraft.getMinecraft().getTextureMapBlocks(); this.setParticleTexture(map.getAtlasSprite(TEXTURE.toString())); to bind the texture, and overriding FX layer to make sure that it will use my custom texture. I've even to go as far as to print the texture location and make sure that the file exists there, but still no luck. Thanks.
-
Hello, I have a TE Special Renderer that displays the item in its inventory, however, the item seems to be rendered with a heavy shadow: I would like the render to look like an Item that is dropped on the ground. Here is the code I'm using to display the item: GlStateManager.pushMatrix(); GlStateManager.disableLighting(); GlStateManager.pushAttrib(); RenderHelper.disableStandardItemLighting(); Minecraft.getMinecraft().getRenderItem().renderItem(stack, ItemCameraTransforms.TransformType.FIXED); RenderHelper.enableStandardItemLighting(); GlStateManager.popAttrib(); GlStateManager.enableLighting(); GlStateManager.popMatrix(); Thanks.
-
Okay, I rearranged my stuff (ex. separating NBT from Syncing) and it seems to be working now. Thanks!
-
My block doesn't actually have a container, nor does it have a GUI. It's a block that stores internally and displays it in the world in a render. I don't think I have to provide a container for this, as nothing is being displayed/interacted with, how I know that these are desynced is from a simple logger within the update function fired on both sides: [16:57:18] [Client thread/INFO] [STDOUT]: [com.github.unassingedxd.voidutils.main.tile.TileVoidInfuser:update:32]: 1xtile.air@0 [16:57:18] [Server thread/INFO] [STDOUT]: [com.github.unassingedxd.voidutils.main.tile.TileVoidInfuser:update:32]: 1xitem.diamond@0 As you can see, the client thread still thinks that there is nothing in it, while the server thread has the correct information being displayed. Yeah, that's what I attempted to separate with my SaveType stuff, to cut down on saving/loading every tick when nothing is actually being done. I think I implemented it correctly, as nothing is being saved atm as nothing is needed synced (within the TileBase). However, on the inventory sided of things, if my 'shouldSyncSlots' is true, then it will send every sync update, however, as you see, its just serializing/deserializing nbt's from the inventory, which I think is what you're getting at. Is there any way to manually send this information without a container? As I still believe that a container isn't necessary for the purposes I have for the block right now. This was my attempt to send that packet within intervals to cut down in network traffic, is there a better way to achieve this? Or do I have the completely wrong idea on sending updates on an interval? Thank you for your time.
-
Hello, I have a tile entity with an Inventory, however, when saving and exiting, then re-entering, the item disappears. As well, the client is never actually aware of the item, its all stored on the server, which makes me believe that there is some kind of desync issue. Here is the my TE base class, which handles capabilities and nbt syncing/saving: And here is the extension of this that adds an inventory: I have stepped through where I dispatch the vanilla packet, and during inventory changed, the function is called and sent to the client, however, nothing actually happens past that, and the item is never synced, thus lost upon restarting. Here is where I'm sending this vanilla packet: Thank you.
-
Hello, I have a tile that 'sucks' in nearby items, however, the motion of the items are super choppy, and seemed off-sycned. Is this a networking issue? If so how would I fix this? and here is the code applying the motion:
-
Hello, I have a 'storage' attached to a chunk via capability, and I want this to update with the rest of Minecraft to modify it. Currently, I'm subscribed to onWorldTick and iterating through a list of chunks that have this storage attached to it, then calling an 'internal update' function to do this modification. This issue with this, however, all values update at the same time, I want them to update separately from each other as their values need to be changed (similar to how TE's work off different ticks). I looked into implementing ITickable, however, the update function is never called, presumably because it does not know my class exists. Is there any way to 'register' my tickable, or is there another way to achieve what I want? Thank you.
-
Okay, so I use: double relX = (this.posX - this.centerBlock.getX()); double relZ = (this.posZ - this.centerBlock.getZ()); double x = Math.round((radius / 2) + (2 * Math.cos(((pi/180)*relX)))+45); double z = Math.round((radius / 2) + (2 * Math.sin(((pi/180)*relZ)))+45); To find the point I want, then apply motion to get there correct? How would I go about applying motion to a certain point, I looked at the Enchantment particles, however, it didn't get me anywhere. Thanks.
-
Maybe I'm just doing this completely wrong, however, I tried adding this to the motion of the particle, but got the same curve. double motX = Math.round((radius / 2) + (2 * Math.cos(((pi/180)*relX)))+90); double motZ = Math.round((radius / 2) + (2 * Math.sin(((pi/180)*relZ)))+90); this.motionX += motX; this.motionZ += motZ; this.move(this.motionX, this.motionY, this.motionZ);
-
I found that stackoverflow, however, I still can't get a proper curve to happen. With my current code, I get a really weird curve that looks like: Here is the current code: double pi = Math.PI; double relX = (this.posX - this.centerBlock.getX()); double relZ = (this.posZ - this.centerBlock.getZ()); this.posX += (int) Math.round((radius / 2) + (2 * Math.cos(((pi/180)*relX) + 90))); this.posZ += (int) Math.round((radius / 2) + (2 * Math.sin(((pi/180)*relZ) + 90))); Thanks.
-
Particle spawning is working, and my particle can have custom behavior. However, the intended behavior I'm trying to get isn't working out so well. The particle is intended to circle around a center block, with a certain radius between it and that block, however, after hours of trying different trigonometric equations, the closest I could get was a curve with these simple 4 lines of code: double relX = (this.posX - this.centerBlock.getX()); double relY = (this.posZ - this.centerBlock.getZ()); this.posX += MathHelper.cos((float)((pi/180) * relX)*20); //20 is a random radius that gave me the best visual this.posZ += MathHelper.sin((float)((pi/180) * relY)*20); The curve I got deviated from the starting position and stopped at a point in which both equations' values reached: xMath: 1.2246469E-16 zMath: 1.2246469E-16 The resulting curve was shaped like an L, nearly representing an exponential line. Now I know the answer is probably some super simple trig, however, I cannot get it to work. Thanks.
-
Thanks, I appreciate this. I'll give it a try and post back on results.
-
Hello, I want to create a particle that goes from its spawn position to a passed in x,z,y. However, I do not have a clue where to go about starting this. I would like the particle to have a custom texture, and custom behavior. Any pointers would be nice. Thank you.
-
[1.12.2] Getting Source of Death from LivingDeathEvent
unassigned replied to unassigned's topic in Modder Support
Oh, yeah. DamageSource#getTrueSource will work. Thanks. -
Hello, I'm trying to spawn in an entity on some mobs death when they are killed by the player, however, I cannot figure out how to check that the player killed the mob and not some other source. I looked into DamageSource, however, it seems that nothing is passed that tells me the source of the damage. Thanks.
-
[1.12.2] ObjectHolder Not Populating Fields
unassigned replied to unassigned's topic in Modder Support
Well, that was definitely the problem. Thanks.