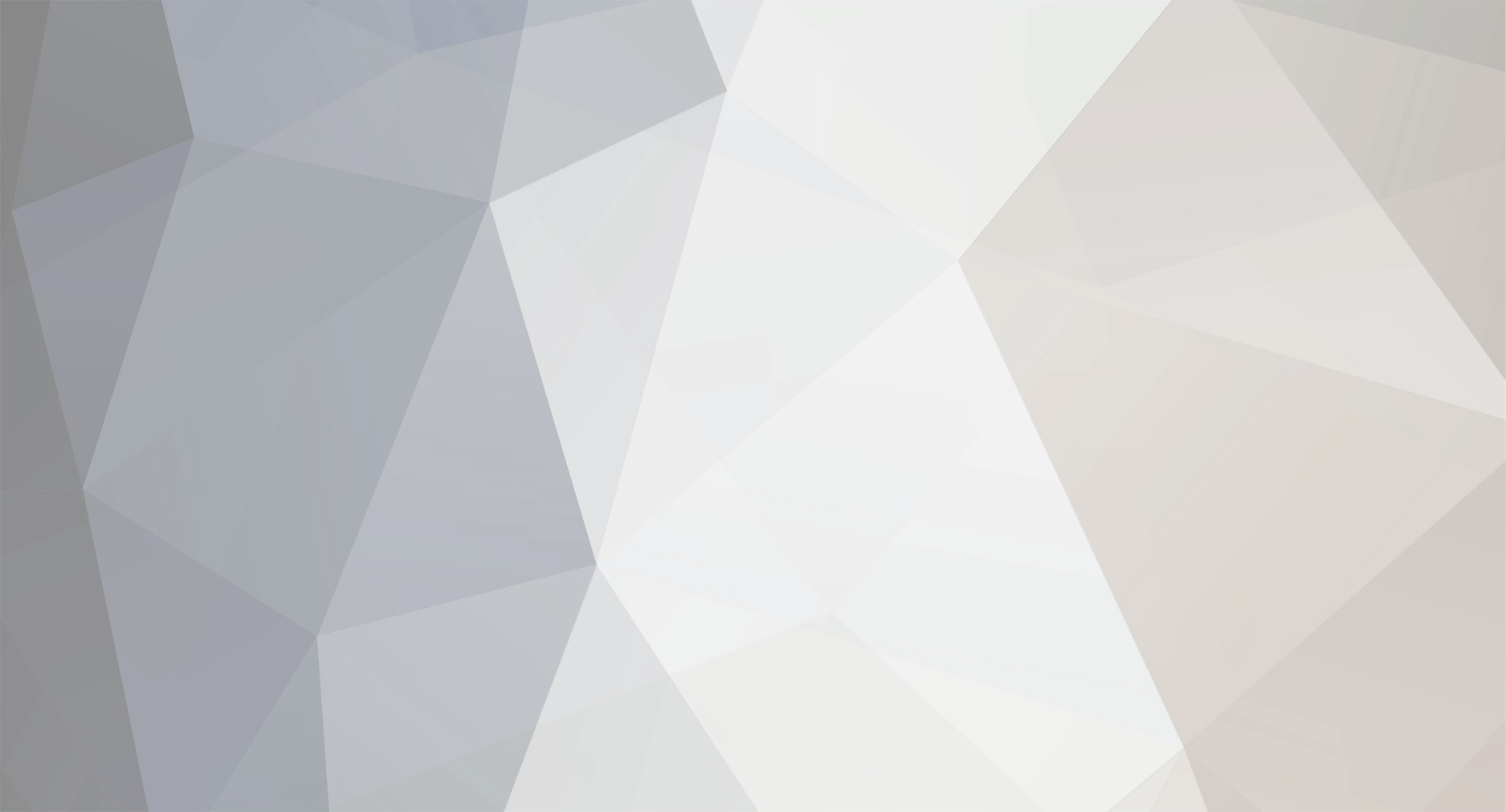
unassigned
Members-
Posts
103 -
Joined
-
Last visited
-
Days Won
1
Everything posted by unassigned
-
Hello, I am trying to register my blocks and items using the new ObjectHolder annotation, however, the fields that should be populated always return null. I've looked through the official documentation, however, I cannot find out what is wrong with my code. Here is my ModBlocks and ModItems classes: Here are the BlockBase, ItemBlockBase, and ItemBase classes: The test item and test block just extend these classes with no extra modifiers. and lastly, here is my event subscriber: and for those interested, here is the error the game throws: Thank you.
-
[1.12.2] Pulling Entities Towards a Block
unassigned replied to unassigned's topic in Modder Support
for(EntityItem item : items) { double moveFactor = 0.5f; double distX = this.pos.getX() - item.posX; double distZ = this.pos.getZ() - item.posZ; double distY = item.posY+1.5D - this.getPos().getY(); double dir = MathHelper.atan2(distZ, distX); double speed = 1f / item.getDistance(this.getPos().getX(), this.getPos().getY(), this.getPos().getZ()) * moveFactor; if(distY<0) { item.motionY += speed; } item.motionX = MathHelper.cos((float)dir) * speed; item.motionZ = MathHelper.sin((float)dir) * speed; } After more testing, this seems to work, however, the items teleport around in random directions. I'm guessing this is a syncing issue, however, I don't know how to actually fix that. Also, would there be any way to stop the items from stacking with each other, if so, how could I achieve it? -
Hello. I have a block that iterates through a 16x16 area for EntityItems, however, I want to add motion towards these entities that 'pull' them towards the said block. I have been trying to do this for quite a while, however, I cannot figure out the math behind it. Currently, I'm finding the relative position of the items from the block and trying to add motion based on this. However, this ultimately failed and I just get items that fly everywhere and look very buggy. Any help would be appreciated. Thanks.
-
The task method worked flawlessly. Thank you very much for your help.
-
What I'm looking for is this: > Spawn in the particle, (its currently an enchantment particle) let it move into its final target position, then decay > after the particle decay (which is about 1.5 seconds) create the explosion at that target position I have everything but the timing down.
-
Oh, I see. So I'd just have to create another tick counter and reset that once task 2 is complete? Or is there a better way to do this?
-
Well, for some reason, I was trying to execute both these tasks at the same rate (5 seconds), when in reality I could just separate the if statements, and make the explosion go off after 6 seconds. However, (maybe this is just my poor understanding of what's actually happening when modulating my TE's ticks) I get a weird phenomenon in which the explosion seems to sync up during the first few calls, but then they become gradually more unsynced for a few more calls, then returns back to being synced again. Here is what I have: if(ticksAlive % 100 == 0) //every 5 seconds { randX = this.pos.getX()+(world.rand.nextInt(8 + 1 + 8) - 8); randZ = this.pos.getZ()+(world.rand.nextInt(8 + 1 + 8) - 8); groundY = (world.getHeight(randX, randZ)); strength = world.rand.nextInt(5 + 1 - 2) + 2; ((WorldServer) world).spawnParticle(EnumParticleTypes.ENCHANTMENT_TABLE, true, randX, groundY, randZ, 1000, 0, 0, 0, 3D); } if(ticksAlive % 120 == 0) //every 6 seconds { world.newExplosion(null, randX, groundY, randZ, strength , false, true); }
-
Hello, I have a tile entity that produces explosions around an area every so often. However, I want to spawn in particles, wait until those particles decay (20ish ticks), then create the explosion. I already have this working, however, I'm not too sure if I should be scheduling tasks, or if there is an another, better way to do this. Here is my current code: public void explosionFX(int x, int y, int z) { ((WorldServer) world).spawnParticle(EnumParticleTypes.ENCHANTMENT_TABLE, true, x, y, z, 100, 0, 0, 0, 3D); Timer timer = new Timer(); timer.schedule(new TimerTask() { @Override public void run() { world.createExplosion(null, x, y, z, 100, false); } }, 1000); //1 second } I could not base this event off tile entity ticks, as this function is already run every 100 ticks. Thanks.
-
[1.12.2] Getting Middle of Chunk from ChunkPos
unassigned replied to unassigned's topic in Modder Support
Oh, I forget that using arithmetic operators is bad when handling chunks. I thought this originally but didn't think it would work when given negative coords (as in, I thought that it would shift the other direction from where the middle would be), but I guess not. for those who are wondering, here is the simple code for this: double x = ((pos.x) << 4) + 8.5; double z = ((pos.z) << 4) + 8.5; Thanks for your help. -
Hello, So I'm trying to spawn in a particle, and eventually, place a block at certain chunk coords, however, I am unable to figure out how I'd actually go about finding the middle of any given chunk. Currently, I have the block coords of the chunk via: int x = (pos.x)*16; int z = (pos.z)*16; int y = world.getHeight(x,z) + 5; which places these coords at the chunk's corner boundary. How would I modify what I have to give me the middle of that chunk? (upon the X,Z axis) Thanks.
-
[1.12.2] Update int within chunk to increase over time
unassigned replied to unassigned's topic in Modder Support
Okay, I have fixed up my code and it is now working fine. I ended up reusing my IVoidHolder and IVoidHolderModifiable so that the list can still be stored and the chunks be accessed under one instance. As well, I used sendToAllTracking to include the dimension it is in when sending the packet. All of these combined allowed me to access the chunks and modify them as I wish. Thank you for your help. -
[1.12.2] Update int within chunk to increase over time
unassigned replied to unassigned's topic in Modder Support
So I'd create another capability with a storage class similar to what I was doing with IVoidHolder? One that just holds that list of ChunkPos and stores to the world? Would I still populate this list using the onChunkLoad / onChunkUnload events? -
[1.12.2] Update int within chunk to increase over time
unassigned replied to unassigned's topic in Modder Support
Okay, I pushed some updated code. -
[1.12.2] Update int within chunk to increase over time
unassigned replied to unassigned's topic in Modder Support
I've been testing for about an hour now, and cannot seem to put a finger on what is happening. I've added a network sync line in the TickEvent when the amount of energy stored is updated. ((VoidEnergy) voidStorage).receiveVoid((world.rand.nextInt(10)+ 1), false); PlentifulUtilities.network.sendToAll(new MessageUpdateVoidValue(voidStorage)); And within my network class, I've added debugs to tell me what messages are being sent. Here is what the debugger shows when the threshold of energy drops below the default value: [11:47:57] [Server thread/INFO] [STDOUT]: [unassigned.plentifulutilities.voidenergy.capability.VoidEnergy:onVoidChanged:114]: sending packet! [11:47:58] [main/INFO] [STDOUT]: [unassigned.plentifulutilities.network.MessageUpdateVoidValue$Handler:lambda$onMessage$0:82]: Pack received and updating! SV: 905 | ticks Sent: 0 [11:47:58] [main/INFO] [STDOUT]: [unassigned.plentifulutilities.network.MessageUpdateVoidValue$Handler:lambda$onMessage$0:82]: Pack received and updating! SV: 980 | ticks Sent: 0 [11:47:58] [main/INFO] [STDOUT]: [unassigned.plentifulutilities.network.MessageUpdateVoidValue$Handler:lambda$onMessage$0:82]: Pack received and updating! SV: 988 | ticks Sent: 0 [11:47:58] [main/INFO] [STDOUT]: [unassigned.plentifulutilities.network.MessageUpdateVoidValue$Handler:lambda$onMessage$0:82]: Pack received and updating! SV: 992 | ticks Sent: 0 [11:47:58] [main/INFO] [STDOUT]: [unassigned.plentifulutilities.network.MessageUpdateVoidValue$Handler:lambda$onMessage$0:82]: Pack received and updating! SV: 1002 | ticks Sent: 0 (do not worry about the ticks sent, I disabled that functionality for now as I try to fix this) As you can see, it properly gets itself back to above 1000, however, the Item that checks this will still display the first value of 905. What is even more interesting is that the Item I have to decrease this value WILL sync up with the client value that is displayed. Here are the two items in question: the 'setter' item: The 'getter' item: What it seems is that the items seem to sync up fine, however, when I try to change the value within the TickEvent, it never syncs, even though it clearly says packets are being sent to the client with the correct data. I pushed another version, if you'd like to see where networking, view the 'network' folder, and for all the void energy related things, view the 'voidenergy' folder. Thank you for your help. -
[1.12.2] Update int within chunk to increase over time
unassigned replied to unassigned's topic in Modder Support
Okay, this worked well I believe, here is the code for the chunk processing: @SubscribeEvent public static void chunkUnload(final ChunkEvent.Unload event) { ChunkPos chunkPos = event.getChunk().getPos(); IVoidStorage voidStorage = getVoidEnergy(event.getChunk()); if(voidStorage == null) return; VOID_CHUNKS_LOADED.remove(chunkPos); } @SubscribeEvent public static void chunkLoad(final ChunkEvent.Load event) { ChunkPos chunkPos = event.getChunk().getPos(); IVoidStorage voidStorage = getVoidEnergy(event.getChunk()); if(voidStorage == null) return; VOID_CHUNKS_LOADED.put(chunkPos, voidStorage); } And here is my new TickEvent: Everything seems to be working fine, other than my values that are checked with the client do no match the values that get output by console here. So obviously, this leads me to think that I'm not sending a packet somewhere, however, I do not know where. I send one during ChunkWatchEvent and every time void related things (ticks/energy) is changed. Here is the WatchEvent: and here is the VoidEnergy class which is the framework behind the whole void system. Thanks for your help. -
[1.12.2] Update int within chunk to increase over time
unassigned replied to unassigned's topic in Modder Support
Okay, so this may or may not be the correct way of doing this, however, I attached an integer onto the capability for keeping track of 'ticks' it has been below the default value. This value is saved within its NBT and is process across the client and server. However, I ran into an issue with the for loop, as it never becomes active, presumably because I'm not correctly trying to access the chunks loaded. Maybe I do not understand the method for getting the currently loaded chunks. I'd like to note I cannot use that HashMap I was using before, as its basically removed from the capability due to moving to serialization. And by 'it never becomes active': I mean that "outside for" is printed at the proper rate, while "within for" is never printed. Again, I have pushed all code to the github if you'd like to see more. -
[1.12.2] Update int within chunk to increase over time
unassigned replied to unassigned's topic in Modder Support
Where would I be keeping this variable stored? Would it be with the capability, or could I do some kind of easier implementation? (ex. would I localize it within the TickEvent?) As well, I've gone through the capability code and tried my best to port this to a serialized capability. All methods that are currently listed as deprecated are going to be removed, however, I am not sure if this is actually correct. Here is the code I have now: And I pushed another version to github if you are interested in other classes. Thanks for your help. -
[1.12.2] Update int within chunk to increase over time
unassigned replied to unassigned's topic in Modder Support
Okay, I'll do this after I get this framework done. Yeah, I can use this map and iterate through the values, however, how would I slow this down to a rate that I would like. In a TE I would normally use the ticks % some-val == 0 to slow, however, I don't see this applicable within the world as a whole. -
[1.12.2] Update int within chunk to increase over time
unassigned replied to unassigned's topic in Modder Support
So I'd get the persistent chunks from the event.world (which I'm presuming gives all currently loaded chunks), get their capability that I've created and then check if its below said default value? Would this require a drastic change in how I'm saving/loading the chunks' 'energy'? As stated, this is derived from an example that I'm trying to learn from. (Choonster). I am aware by me using ChunkDataEvents (which are soon to be deprecated) makes this code obsolete when transitioning to 1.13. So a small list of things that would have to be done in order to keep some kind of compatibility would be nice. As storing things using capabilities like this is still somewhat new to me. Thanks. -
Hello, So I have a capability that stores a value within chunks that can be accessed across items/blocks within my mod. This value, however, I want to increase back to a default value if it has decreased at all. I want this value to increase very slowly, but I don't exactly know how I should do this as efficiently as possible to prevent lag. I'm already subscribed to some events: ChunkDataEvent.Save, ChunkDataEvent.Load, ChunkEvent.Load, ChunkEvent.Unload and ChunkWatchEvent. Here is the capability class that I'm using: All other class references can be found on my github in the folder labeled 'voidenergy'. Thank you.
-
Interestingly, I have added these supers to the methods that need it, and now the item does not stay in the slot; once I put in the item, and close the GUI, it just pops out. The code on github is updated, use these classes: TileEntityBase TileEntityInventoryBase TileEntityVoidAccumualtor ContainerVoidAccumulator Thanks.
-
Alright, I have added that to my holders, thanks!
-
Okay, I've added supers to methods that need it, and I do believe that I am implementing the capabilites via TileEntityBase class: If a TE needs an energy/item capability, you just set the getEnergyStorage/getItemHandler within the class, which reduces the amount of code per TE - which I do within the TE I'm testing (TileEntityVoidAccumulator) I was not aware of the existence of this, so I'd just throw this.inv.deserializeNBT(compound); within the writeNBT; and would I use the serialize method to read or would I need to keep the functionality I have currently. This must somewhat new, as I was not aware of this annotation (most of my modding knowledge is from 1.7.10/1.11). Would this just lead a class, or would this go where it is initialized? Thanks.
-
I do have this in the TileEntityBase that I'm extending. I believe it is not a desync issue as, in the tile that has the inventory, I have: which prints the item properly - and displays correctly when opened and closed, however, once restarted, the client prints: [14:59:46] [main/INFO] [STDOUT]: [unassigned.plentifulutilities.tile.TileEntityVoidAccumulator:update:25]: client? true - item: tile.air while the server does not print anything; probably because the itemstack is considered empty. Here is all of the TileEntityBase code for sending packets and saving other NBT data: Thanks.