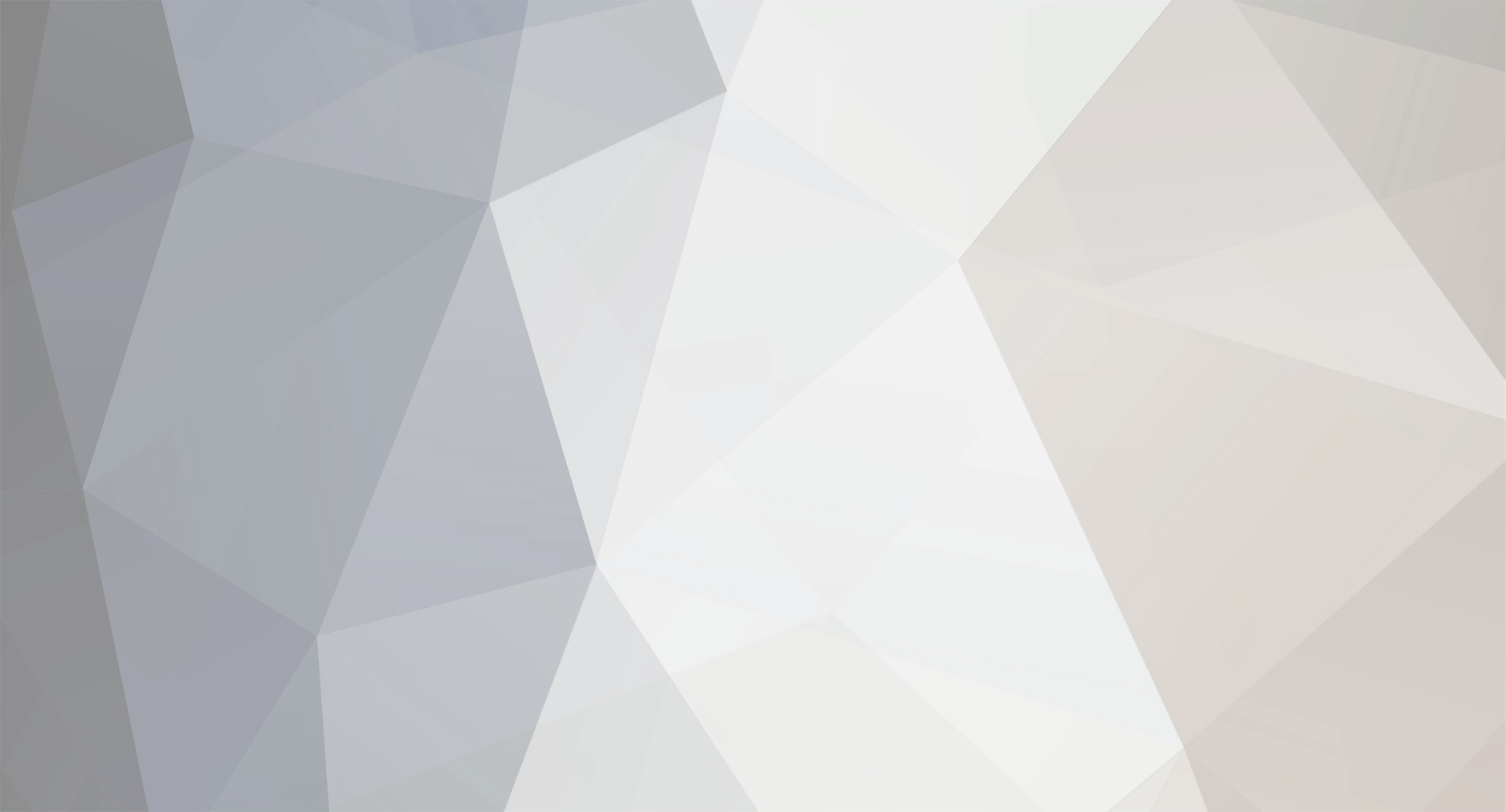
Meldexun
Members-
Posts
184 -
Joined
-
Last visited
Everything posted by Meldexun
-
Yes I don't need to save the data. But how can I stop it from saving NBT data. This is my provider class: public class StructureDataProvider implements ICapabilitySerializable<NBTBase> { @CapabilityInject(IStructureData.class) public static final Capability<IStructureData> STRUCTURE_DATA = null; private IStructureData instance = STRUCTURE_DATA.getDefaultInstance(); @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing) { return capability == STRUCTURE_DATA; } @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { return capability == STRUCTURE_DATA ? STRUCTURE_DATA.<T> cast(this.instance) : null; } @Override public NBTBase serializeNBT() { return STRUCTURE_DATA.getStorage().writeNBT(STRUCTURE_DATA, this.instance, null); } @Override public void deserializeNBT(NBTBase nbt) { STRUCTURE_DATA.getStorage().readNBT(STRUCTURE_DATA, this.instance, null, nbt); } } and this is my storage class: public class StructureDataStorage implements IStorage<IStructureData> { @Override public NBTBase writeNBT(Capability<IStructureData> capability, IStructureData instance, EnumFacing side) { return new NBTTagInt(0); } @Override public void readNBT(Capability<IStructureData> capability, IStructureData instance, EnumFacing side, NBTBase nbt) { } } What i mean is that it now saves a NBTTagInt which is pointless because i don't use/need it. But I have to return a NBTBase. Can i change something to avoid this unnecessary data saving.
-
I just need to save the 2 BlockPos on server side temporarily. But is this really the only/best way?
-
When registering an instance of your event handler with MinecraftForge.EVENT_BUS.register() then you have to use non static events handlers. Or you can pass the complete event handler class. You can also automatically register your event handler by annotating your event handler class with @Mod.EventBusSubscriber. You can read more about it here: https://mcforge.readthedocs.io/en/latest/events/intro/
-
[1.11.2] Trying to filter entity attack targets
Meldexun replied to Triphion's topic in Modder Support
You could make a for loop and check for every entity in the targetList if it is an ally or a player and then add it to your friendlyList. -
Hi there, I want to save 2 BlockPos for a player. They don't need to be saved when leaving and reentering the world. I thought of adding a Capability to the player but then i have to save something as NBT. Is there an easier way to save 2 BlockPos for a player? Thanks for the help.
-
I just meant for the model and rotation part
-
It's just a testing mod. I just wanted to see if my model works.
-
Yes and i thought i got everything right. I looked a bit in the web and now i also overwrite this method: @Override public IBlockState getStateForPlacement(World world, BlockPos pos, EnumFacing facing, float hitX, float hitY, float hitZ, int meta, EntityLivingBase placer, EnumHand hand) { return this.getDefaultState().withProperty(FACING, placer.getHorizontalFacing()); } and it seems to work fine. Feel free to correct me if I'm making trash
-
Hello there, I created a block and i want that it rotates when you place it in the world. Like the stairs in Minecraft. But my block isn't rotating in the direction i look. So here is my block class: public class BaseBlock extends BlockHorizontal { public BaseBlock(String name) { super(Material.ROCK); setUnlocalizedName(name); setRegistryName(CrystalicVoid.modid, name); setCreativeTab(CrystalicVoid.tabCrystalicVoid); setDefaultState(this.blockState.getBaseState().withProperty(FACING, EnumFacing.NORTH)); } @Override public boolean isOpaqueCube(IBlockState state) { return false; } @Override public boolean isFullCube(IBlockState state) { return false; } @Override protected BlockStateContainer createBlockState() { return new BlockStateContainer(this, FACING); } @Override public IBlockState getStateFromMeta(int meta) { return this.getDefaultState().withProperty(FACING, EnumFacing.getHorizontal(meta)); } @Override public int getMetaFromState(IBlockState state) { return state.getValue(FACING).getHorizontalIndex(); } } and my blockstate: { "variants": { "facing=north": {"model": "crystalic_void:body_back_legs"}, "facing=east": {"model": "crystalic_void:body_back_legs", "y": 90}, "facing=south": {"model": "crystalic_void:body_back_legs", "y": 180}, "facing=west": {"model": "crystalic_void:body_back_leg", "y": 270} } } and my model: { "credit": "Made with Blockbench", "textures": { "0": "blocks/quartz_block_side", "particle": "blocks/quartz_block_side" }, "elements": [ { "name": "legs", "from": [8.1, 14.7, 21.1], "to": [14.1, 30.7, 27.1], "faces": { "north": {"uv": [0, 0, 6, 16], "texture": "#0"}, "east": {"uv": [0, 0, 6, 16], "texture": "#0"}, "south": {"uv": [0, 0, 6, 16], "texture": "#0"}, "west": {"uv": [0, 0, 6, 16], "texture": "#0"}, "up": {"uv": [0, 0, 6, 6], "texture": "#0"}, "down": {"uv": [0, 0, 6, 6], "texture": "#0"} } }, { "name": "legs", "from": [2, 0, 21.1], "to": [8, 16, 27.1], "rotation": {"angle": -22.5, "axis": "z", "origin": [8, 0, 24]}, "faces": { "north": {"uv": [0, 0, 6, 16], "texture": "#0"}, "east": {"uv": [0, 0, 6, 16], "texture": "#0"}, "south": {"uv": [0, 0, 6, 16], "texture": "#0"}, "west": {"uv": [0, 0, 6, 16], "texture": "#0"}, "up": {"uv": [0, 0, 6, 6], "rotation": 270, "texture": "#0"}, "down": {"uv": [0, 0, 6, 6], "rotation": 90, "texture": "#0"} } }, { "name": "legs", "from": [14, 16, 4.9], "to": [20, 32, 10.9], "rotation": {"angle": 22.5, "axis": "z", "origin": [20, 16, 8]}, "faces": { "north": {"uv": [0, 0, 6, 16], "texture": "#0"}, "east": {"uv": [0, 0, 6, 16], "texture": "#0"}, "south": {"uv": [0, 0, 6, 16], "texture": "#0"}, "west": {"uv": [0, 0, 6, 16], "texture": "#0"}, "up": {"uv": [0, 0, 6, 6], "rotation": 270, "texture": "#0"}, "down": {"uv": [0, 0, 6, 6], "rotation": 90, "texture": "#0"} } }, { "name": "legs", "from": [14, 0, 4.9], "to": [20, 16, 10.9], "faces": { "north": {"uv": [0, 0, 6, 16], "texture": "#0"}, "east": {"uv": [0, 0, 6, 16], "texture": "#0"}, "south": {"uv": [0, 0, 6, 16], "texture": "#0"}, "west": {"uv": [0, 0, 6, 16], "texture": "#0"}, "up": {"uv": [0, 0, 6, 6], "texture": "#0"}, "down": {"uv": [0, 0, 6, 6], "texture": "#0"} } } ] }
-
I'm sorry but I lost some files. So I can't send you any example.
-
So here are my full classes. @EventBusSubscriber public class InitBiome { public static Biome kelpForest; @SubscribeEvent public static void registerBiome(RegistryEvent.Register<Biome> event) { kelpForest = new KelpForest().setRegistryName(BetterDiving.modid, "Kelp Forest"); event.getRegistry().register(kelpForest); BiomeDictionary.addTypes(kelpForest, Type.OCEAN, Type.WATER); BiomeManager.addBiome(BiomeType.WARM, new BiomeEntry(kelpForest, 10)); } } And my Biome class public class KelpForest extends Biome { public KelpForest() { super(new BiomeProperties("Kelp Forest").setBaseHeight(-1.5f).setHeightVariation(0.05f).setWaterColor(8257405).setBaseBiome("ocean")); this.spawnableCreatureList.clear(); topBlock = Blocks.SAND.getStateFromMeta(0); fillerBlock = Blocks.SAND.getStateFromMeta(0); this.decorator.clayGen = null; this.decorator.sandGen = null; this.decorator.gravelGen = null; this.decorator.dirtGen = null; this.decorator.gravelOreGen = null; this.decorator.graniteGen = null; this.decorator.dioriteGen = null; this.decorator.andesiteGen = null; this.decorator.flowerGen = null; this.decorator.mushroomBrownGen = null; this.decorator.mushroomRedGen = null; this.decorator.bigMushroomGen = null; this.decorator.reedGen = null; this.decorator.cactusGen = null; this.decorator.waterlilyGen = null; this.decorator.waterlilyPerChunk = 0; this.decorator.treesPerChunk = 0; this.decorator.extraTreeChance = 0; this.decorator.flowersPerChunk = 0; this.decorator.grassPerChunk = 0; this.decorator.deadBushPerChunk = 0; this.decorator.mushroomsPerChunk = 0; this.decorator.reedsPerChunk = 0; this.decorator.cactiPerChunk = 0; this.decorator.gravelPatchesPerChunk = 0; this.decorator.sandPatchesPerChunk = 0; this.decorator.clayPerChunk = 0; this.decorator.bigMushroomsPerChunk = 0; this.decorator.generateFalls = false; } @Override public Biome.TempCategory getTempCategory() { return Biome.TempCategory.OCEAN; } @Override public BiomeDecorator getModdedBiomeDecorator(BiomeDecorator original) { return new DecoratorKelpForest(); } }
-
Ok. I will post my code tomorrow. It's to late for me now.
-
I'm registering my biome correctly. So it is spawning as a ocean biome with water and so on. But Minecraft generates Biome Types (warm,...) and if i use this: BiomeManager.addBiome(BiomeType.WARM, new BiomeEntry(kelpForest, 20)); When a warm biome type generates my biome has a chance to appear. But I want my biome to spawn when a ocean biome is generated and not when a warm biome is generated.
-
Ok i found out that minecraft spawns Biome Types (Warm, Cold, Icy or Desert). So with this when minecraft is spawning a Warm biome my biome has a chance to be generated. BiomeManager.addBiome(BiomeType.WARM, new BiomeEntry(kelpForest, 20)); But there is no Biome Type Ocean. Does nobody know how I can register and generate it as a Ocean biome?
-
I was also thinking of adding a check if my mod is loaded on the server too. But a hacker could more easily write his own mod than hack into mine.
-
My mod changes vanilla movement under water so you don't sink automatically and you swim in the looking direction. But since movement is always handeled client side I noticed that my mod can easily be used to cheat on a PVP server, because the mod has only to be installed on client. Can I change something so that it can't be used as a cheat engine? Also it seems very, very simple to create a speed enhancing mod which only makes the player 1-2% faster. That would be hard to track from a server be still gives you an advantage.
-
Yes I'm using @EventBusSubscriber. But I didn't know that I can specify a Side there. Will do that right know. Thank you
-
I heard that I shouldn't use @SideOnly. So that was my way to register the item models: @SideOnly(Side.CLIENT) @SubscribeEvent public static void registerRenders(ModelRegistryEvent event) { registerRender(item1, 0); //... } private static void registerRenderMeta(Item item, int meta) { ModelLoader.setCustomModelResourceLocation(item, meta, new ModelResourceLocation(item.getRegistryName(), "inventory")); } I changed it and now I have this: @SubscribeEvent public static void registerRenders(ModelRegistryEvent event) { BetterDiving.proxy.registerItemRender(item1, 0); } And in my client proxy the registerItemRender looks like this: @Override public void registerItemRender(Item item, int meta) { ModelLoader.setCustomModelResourceLocation(item, meta, new ModelResourceLocation(item.getRegistryName(), "inventory")); } In my server proxy this method does nothing. So am I registering the models the correct way now?
-
[Solved] Crashing Before the Game Loads up [1.12.2]
Meldexun replied to Interplay's topic in Modder Support
I'm not totally sure but I think you should annotate your onModelRegister with @SideOnly(Side.CLIENT). (I'm wrong. Stick with the post of diesieben07) But I know another thing. Your oxygen mask has a custom model and to use it you are overriding the getArmorModel method in your class. But you are creating a new model every tick inside this method with: ModelOxygenMask model1 = new ModelOxygenMask(); You should create your model in your client proxy. -
I have created a new Biome and now I want to make it spawn with a ocean biome around it. Or at least next to my biome should be a ocean biome. So this is what I'm doing when registering my biome: @SubscribeEvent public static void registerBiome(RegistryEvent.Register<Biome> event) { event.getRegistry().register(kelpForest); BiomeDictionary.addTypes(kelpForest, Type.OCEAN); BiomeManager.addBiome(BiomeType.WARM, new BiomeEntry(kelpForest, 20)); BiomeManager.addSpawnBiome(kelpForest); } But it seems that it just randomly spawns.
-
[1.12.2] Item glint and durabilitybar prolem
Meldexun replied to SaltStation's topic in Modder Support
I think you have to create a capability which is added to your itemStack. Documentation -
I created a custom armor and a custom model. To show my model I'm overwriting the getArmorModel() method in my custom armor class. But where should I create the object of my custom model which is returned by the getArmorModel() method? Currently I'm creating it in my ClientProxy: public class ClientProxy implements IProxy { public static CustomModel model1; @Override public void init() { model1 = new CustomModel(1.0f); } }
-
So I just create a method in my Client proxy which is than called by the MessageHandler. @Override public IMessage onMessage(MessageCapabilitySync messageCapabilitySync, MessageContext ctx) { ClientProxy.message1(messageCapabilitySync, ctx); return null; }
-
I now check in my MessageHandler if the message is recieved client side and it seems to work. @Override public IMessage onMessage(MessageCapabilitySync messageCapabilitySync, MessageContext ctx) { if (ctx.side.isClient()) { //do some stuff } return null; } I tried that before but then it was crashing all the time. Thats why I used the proxy to register my message. I also noticed that most people name it IProxy. Maybe I will change it too.
-
Ok. That makes sense. I will try to do that. It's just the name of the interface. I have just followed a tutorial for modding beginning and there it was named common proxy. I'm not doing something in this interface.