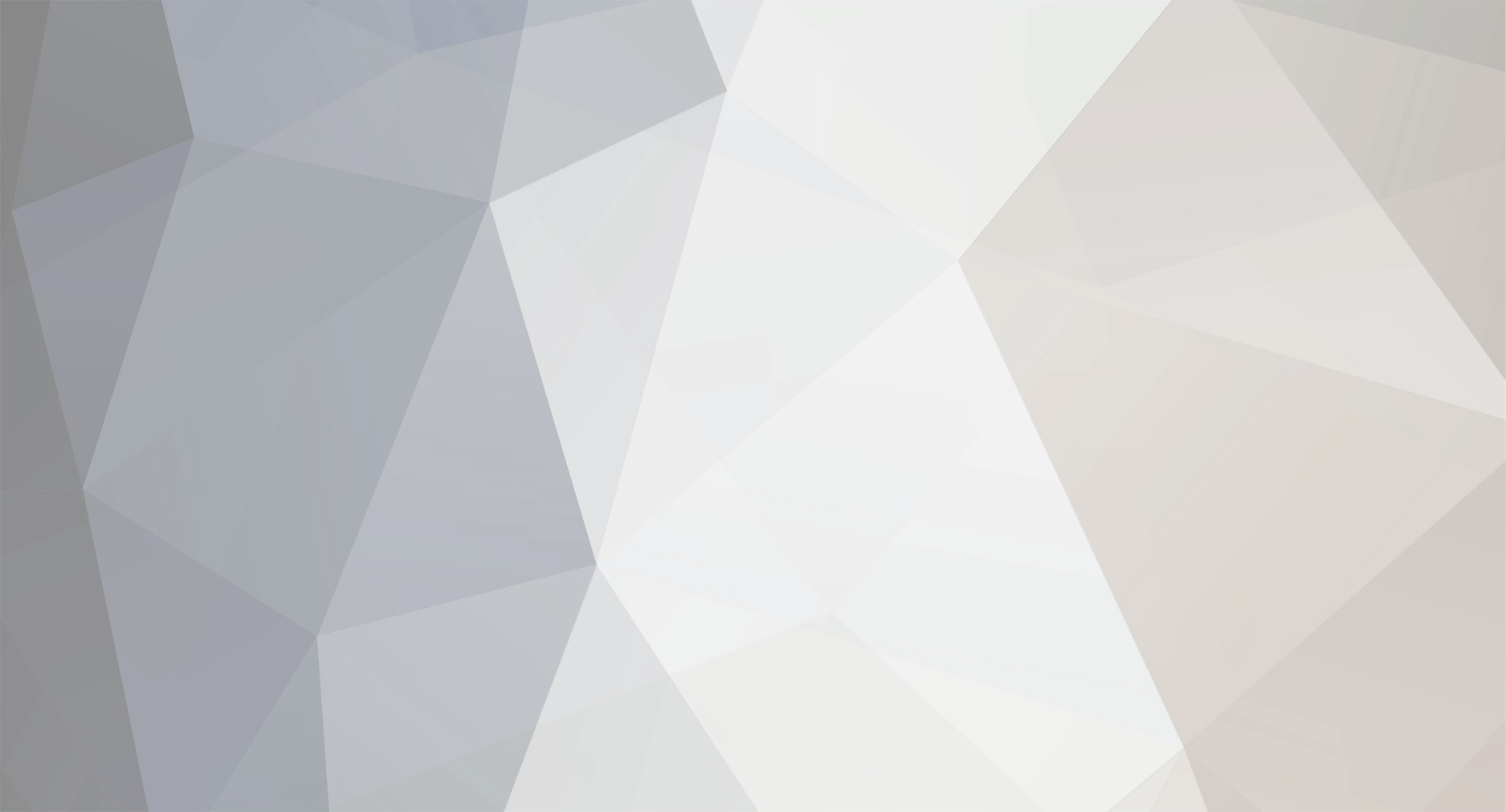
TesterTesting135
Members-
Posts
105 -
Joined
-
Last visited
Everything posted by TesterTesting135
-
Hello, so I've made a cobblestone generator but would like it to auto-eject items. This is my method for doing so: private void sendItems() { if (!outputHandler.getStackInSlot(0).isEmpty()) { for (EnumFacing facing : EnumFacing.VALUES) { TileEntity tileEntity = world.getTileEntity(pos.offset(facing)); if (tileEntity != null && tileEntity.hasCapability(CapabilityItemHandler.ITEM_HANDLER_CAPABILITY, facing.getOpposite())) { IItemHandler handler = tileEntity.getCapability(CapabilityItemHandler.ITEM_HANDLER_CAPABILITY, facing.getOpposite()); if (handler != null) { for (int i = 0; i <= handler.getSlots() ; i++) { handler.insertItem(i, outputHandler.getStackInSlot(0), false); outputHandler.extractItem(0, 64, false); if (outputHandler.getStackInSlot(0).isEmpty()) { break; } } } } } markDirty(); } } but for some reason, it will only insert items to 1 slot of the chest. Any idea why? Thanks.
-
Ok, woops. I didn't remove the first 2 statements. I removed them, and fixed the itemstack now. It works! Thank you so much!
-
Ok, so i fixed that, but I'm still getting "No Key".
-
Ok, so here is my code now: public static final String FREEZE_KEY = "tmfreeze"; @Override public ActionResult<ItemStack> onItemRightClick(World worldIn, EntityPlayer playerIn, EnumHand handIn) { playerIn.setActiveHand(handIn); ItemStack stack = playerIn.getActiveItemStack(); NBTTagCompound nbt; if (!worldIn.isRemote) { if (stack.hasTagCompound()) { nbt = stack.getTagCompound(); } else { nbt = new NBTTagCompound(); } if (nbt.hasKey(FREEZE_KEY)) { nbt.setBoolean(FREEZE_KEY, !nbt.getBoolean(FREEZE_KEY)); } else { nbt.setBoolean(FREEZE_KEY, true); playerIn.sendMessage(new TextComponentString("No Key")); } stack.setTagCompound(nbt); FreezeTime(playerIn, nbt.getBoolean(FREEZE_KEY), ConfigHandler.SWORD_OF_INEVITIBILITY_FREEZE_RANGE); return new ActionResult<>(EnumActionResult.SUCCESS, playerIn.getHeldItem(handIn)); } return super.onItemRightClick(worldIn, playerIn, handIn); } but it's still always true.
-
Ok, thank you
-
Why shouldn't i just return the super?
-
No, what should i return?
-
Ok, thanks for the tip. Any ideas why it's still not working? Thanks.
-
Ok, so i changed the string but for some reason, the hasKey method isn't working. Whenever i right click, it always says it's false. I added a debug line to it, saying "No Key" in chat if there wasn't a key. Here is my code: public static final String FREEZE_KEY = "tmfreeze"; @Override public ActionResult<ItemStack> onItemRightClick(World worldIn, EntityPlayer playerIn, EnumHand handIn) { playerIn.setActiveHand(handIn); ItemStack stack = playerIn.getActiveItemStack(); NBTTagCompound nbt; if (stack.hasTagCompound()) { nbt = stack.getTagCompound(); } else { nbt = new NBTTagCompound(); } if (nbt.hasKey(FREEZE_KEY)) { nbt.setBoolean(FREEZE_KEY, !nbt.getBoolean(FREEZE_KEY)); } else { nbt.setBoolean(FREEZE_KEY, false); playerIn.sendMessage(new TextComponentString("No Key")); } FreezeTime(playerIn, nbt.getBoolean(FREEZE_KEY), ConfigHandler.SWORD_OF_INEVITIBILITY_FREEZE_RANGE); return super.onItemRightClick(worldIn, playerIn, handIn); } Why isn't this working? Thanks.
-
Oh wow! I did not notice that! Thank you so much! As for the mod id, i actually do have a constant, but in the recipe json files and blockstate files you can't really use a constant.
-
Ok, i will change the modid. The only problem is, i'm not sure how to use Intellij to refactor it for all my files. Also, where did I forget an instance of my string? Thanks.
-
Uninstall that current version of java
-
Ok, thanks for the reply. I added this: @Override public ActionResult<ItemStack> onItemRightClick(World worldIn, EntityPlayer playerIn, EnumHand handIn) { playerIn.setActiveHand(handIn); ItemStack stack = playerIn.getActiveItemStack(); NBTTagCompound nbt; if (stack.hasTagCompound()) { nbt = stack.getTagCompound(); } else { nbt = new NBTTagCompound(); } if (nbt.hasKey("tmfreeze")) { nbt.setBoolean("tmfreeze", !nbt.getBoolean("tmfreeze")); } else { nbt.setBoolean("tmfreeze", false); } FreezeTime(playerIn, worldIn, nbt.getBoolean("freeze"), 20); stack.setTagCompound(nbt); return super.onItemRightClick(worldIn, playerIn, handIn); } (The else statement), but it would always set it to false. Any idea why? Thanks.
-
Hello, so I'm trying to make a sword that freezes entities on right click, but i cannot get the toggle working. There is probably a very easy solution to this, but I cannot for the life of me figure it out. Here is the right click method: @Override public ActionResult<ItemStack> onItemRightClick(World worldIn, EntityPlayer playerIn, EnumHand handIn) { playerIn.setActiveHand(handIn); ItemStack stack = playerIn.getActiveItemStack(); NBTTagCompound nbt; if (stack.hasTagCompound()) { nbt = stack.getTagCompound(); } else { nbt = new NBTTagCompound(); } if (nbt.hasKey("freeze")) { nbt.setBoolean("freeze", !nbt.getBoolean("freeze")); } FreezeTime(playerIn, worldIn, nbt.getBoolean("freeze"), 20); stack.setTagCompound(nbt); return super.onItemRightClick(worldIn, playerIn, handIn); } For some reason, instead of toggling "freeze" between true and false, it just stays at false. This code looks like it should work to me, but it doesn't. Please forgive me if this is a stupid mistake. Thanks in advance.
-
So should I use something similar to what the chest does but for all the directions (Pos x, pos z, pos y, neg x, etc)?
-
Oh, ok. So would i make the "multiblock" by doing something that a chest does?
-
Hello, so I've recently made an energy cube and it does everything i want, except for the ability to turn into a multiblock. Bascically i want it to be like ender io's capacitor banks, where they can be any shape as long as they're connected. How would i go about this? Thanks in advance.
-
Alright, i got it all figured out now. Thanks for the help!
-
I got the GUI stuff down and how to send the info to the client, but would I go about actually calculating the RF/T? I've tried storing the current RF in a variable and then 20 seconds later it would subtract that and the current energy stored then divide by 20 for the ticks, but that didn't seem to work.
-
Hello, so I've recently made a energy storage block, and everything is going well except for figuring out RF/T. I want it so that when i hover over the energy bar, it would show the RF/T input. How would I go about this? Thanks in advance!
-
Hello, so I've just switched over to the mappings "stable_39", and I've fixed everything, except for transparency. This is my code: @Override @SideOnly(Side.CLIENT) public BlockRenderLayer getBlockLayer() { return BlockRenderLayer.TRANSLUCENT; } Unfortunately, it doesn't seem like it's there now. What is the correct thing to do now? Thanks in advance. EDIT: This was a really stupid question, i think i fixed it. The correct code if anyone's wondering is: @Override public BlockRenderLayer getRenderLayer() { return BlockRenderLayer.CUTOUT; }
-
Alright, thank you for the reply. I think i'm going to look at some other mods code (Like Furnace Overhaul) and see how they do.
-
1.12.2
-
Hello, so i'm making a furnace here and i would like it to double ores. Unfortunately, i have no idea how to check if the item is actually an ore. I want it to be compatible with other modded ores like aluminum from thermal expansion, etc. How do i do this? Thanks in advance.
-
[SOLVED, BIG MISTAKE] Help with StringUtils
TesterTesting135 replied to TesterTesting135's topic in Modder Support
Oops, sorry, i didn't really clarify. I had actually imported the wrong StringUtils. I had accidentally used Minecraft's StringUtils, but i was supposed to import another one. It was also on episode 10 btw. I didn't realize it started from episode 1.