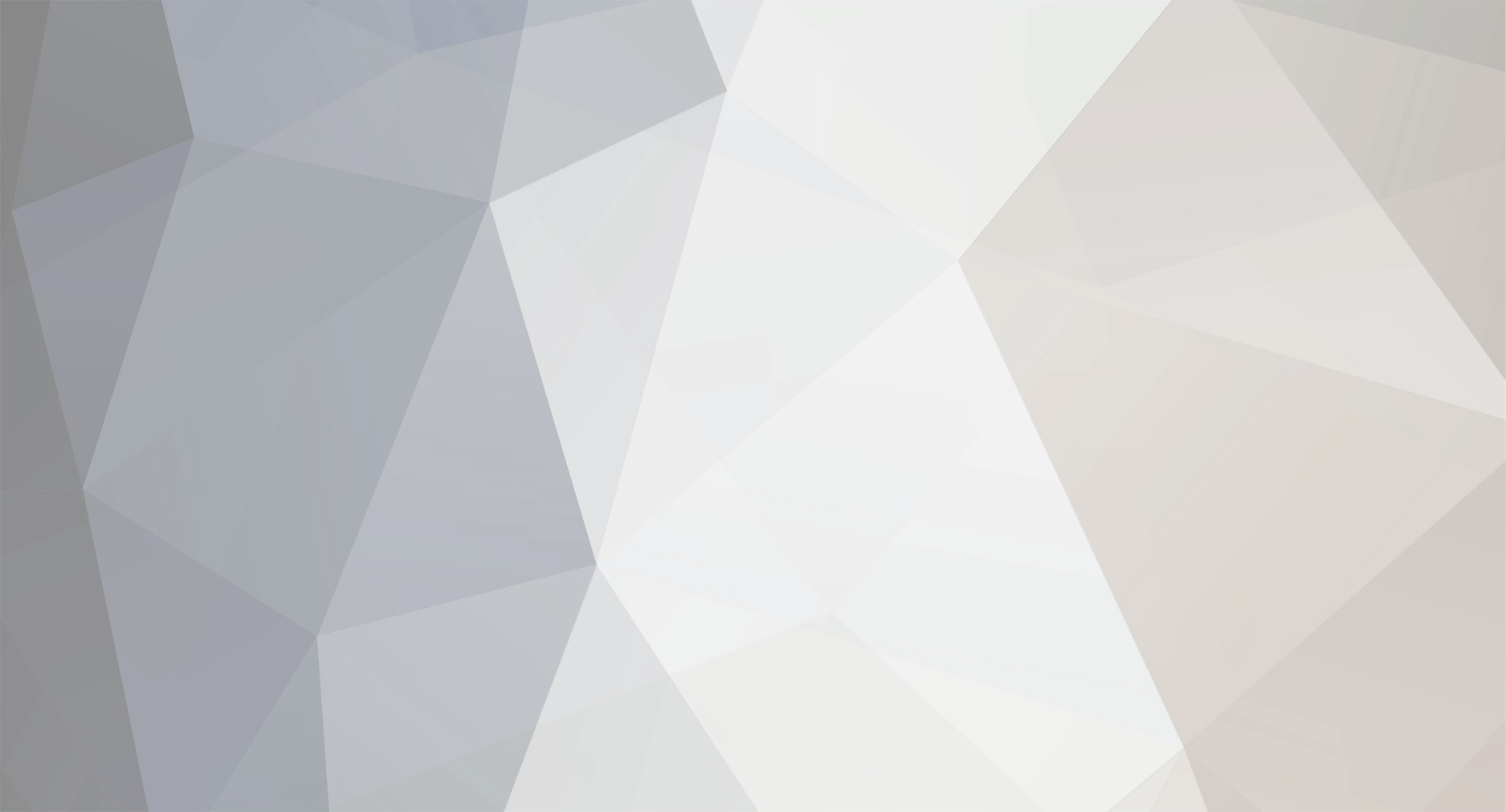
Whompy
Members-
Posts
36 -
Joined
-
Last visited
Everything posted by Whompy
-
Eh I'll just create an entity for each recipe who cares about efficiency ?
-
public class EntitySponge extends EntityVex{ private Item purposeItem = Items.PUMPKIN_PIE; private net.minecraft.block.Block purpose = Blocks.GLASS; private Object time = 1000; private Boolean Item = false; public EntitySponge(World worldIn) { super(worldIn); } public void EntitySpongeadd(World worldIn, Block purpose,Item purposeItem, Object time) { this.time = time; //glass if(this.time == (Object)1000) { this.Item = false; this.purpose = ModBlocks.MAGIC_BLOCK; } //glass if(this.time == (Object)5000) { this.Item = false; this.purposeItem = ModItems.ORB_OF_CHANNELING; } } @Override protected void entityInit() { // TODO Auto-generated method stub } @Override public void readEntityFromNBT(NBTTagCompound compound) { // TODO Auto-generated method stub } @Override public void writeEntityToNBT(NBTTagCompound compound) { // TODO Auto-generated method stub } @Override protected SoundEvent getAmbientSound() { return SoundEvents.BLOCK_BREWING_STAND_BREW; } @Override protected SoundEvent getHurtSound(DamageSource source) { return SoundEvents.BLOCK_FIRE_AMBIENT; } @Override protected SoundEvent getDeathSound() { return SoundEvents.BLOCK_FIRE_EXTINGUISH; } @Override protected ResourceLocation getLootTable() { return LootTableList.EMPTY; } public void onLivingUpdate() { for (int i = 0; i < 1; ++i) { timer((int)this.time); } } public void timer(int time) { try { Thread.sleep(time); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } the thing is that I am trying to make this entity appear to be a different item/block upon being spawned by the FocusBlock with it's own properties such as purposeItem/purposeblock (the item/block it is supposed to create after sitting on top of the custom block) so that it appears to be an item. i resorted to this method because I was struggling with using an actual item to complete my goal. The entity seems to be registering normally,however upon being spawned a null pointer exception comes up on console and the game doesn't crash. How would I go about using different textures for this entity. Would I use another entity per special crafting recipe?
-
Sorry I don't think I was clear, I am trying to render my own untouchable item which will be spawned on top of a block . If I were to say override a block of glass's createEntity/hasCustomEntity functions, wouldn't it also make the entity itself when (obtained through normal means) not be picked up? how would I differentiate between the two possibilities, a boolean? Also, how would I get this to apply to a non modded item's itemEntity. would I need to create another class for modded and nonmodded items? If you know of any example tutorials talking about this or example code, I would appreciate it
-
How am I supposed to render my entity Items. I have tried extending EntityItem and adding my own properties to it. How would I apply this to an item which I am spawning as of now I can't use itemfloating as an ItemEntity public class ItemFloating extends EntityItem{ public int lifespan = -1; private int health; public ItemFloating(World worldIn, double x, double y, double z) { super(worldIn); this.health = 5; this.hoverStart = (float)(Math.random() * Math.PI * 2.0D); this.setSize(0.25F, 0.25F); this.setPosition(x, y, z); this.rotationYaw = (float)(Math.random() * 360.0D); this.motionX = (double)((float)(Math.random() * 0.20000000298023224D - 0.10000000149011612D)); this.motionY = 0.20000000298023224D; this.motionZ = (double)((float)(Math.random() * 0.20000000298023224D - 0.10000000149011612D)); } public ItemFloating(World worldIn, double x, double y, double z, ItemStack stack) { this(worldIn, x, y, z); this.setItem(stack); this.lifespan = (stack.getItem() == null ? -1 : stack.getItem().getEntityLifespan(stack, worldIn)); } @Override public void onUpdate() { cannotPickup(); super.onUpdate(); } } I have not written much else besides my other item block and mob code in case you are wondering.
-
So I attempted to replace that faulty if statement by attempting to get the blockstate of the block I was trying to refer to. if(state.getActualState(worldIn, pos.down()) == ModBlocks.MAGIC_BLOCK.getDefaultState()) {... } that if statement now looks like this However it still doesn't quite get past the if statement I am trying to check if the block bellow the BlockFocus is another specific modded block from my mod any suggestions for how I should go about this?
-
Sorry for having messy code, a good portion of the tile entity related stuff is from earlier testing when I was attempting to store the item on the BlockFocus block in a tlieentity. It didn't quite work out so I left some framework if I managed to figure out how to do it in the future. thx for the advice I will give it another shot
-
Well here is my block code public class BlockFocus extends Block implements IHasModel{ public static AxisAlignedBB BASIC_FOCUS_AABB = new AxisAlignedBB(0D, 0, 0D, 1D, 0.375D, 1D); public BlockFocus(String name, Material materialIn) { super(materialIn); setUnlocalizedName(name); setRegistryName(name); setCreativeTab(CreativeTabs.BUILDING_BLOCKS); ModBlocks.BLOCKS.add(this); ModItems.ITEMS.add(new ItemBlock(this).setRegistryName(this.getRegistryName())); setSoundType(SoundType.WOOD); setHardness(25); setResistance(3000); setHarvestLevel("pickaxe", 0); setLightLevel(0F); } @Override public void registerModels() { Main.proxy.registerItemRenderer(Item.getItemFromBlock(this), 0, "inventory"); } @Override public boolean isOpaqueCube(IBlockState state) { // TODO Auto-generated method stub return false; } @Override public boolean isFullCube(IBlockState state) { // TODO Auto-generated method stub return false; } @Override public AxisAlignedBB getBoundingBox(IBlockState state, IBlockAccess source, BlockPos pos) { return BASIC_FOCUS_AABB; } Item glass = Item.getItemFromBlock(Blocks.GLASS); ItemStack glass2 = new ItemStack(glass); @Override public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumHand hand, EnumFacing facing, float hitX, float hitY, float hitZ) { // TODO Auto-generated method stub if(pos.down() == ModBlocks.MAGIC_BLOCK.getDefaultState()) { if(playerIn.inventory.getCurrentItem().getItem() == glass) { BlockTileEntity.spawnAsEntity(worldIn, pos,glass2); return super.onBlockActivated(worldIn, pos, state, playerIn, hand, facing, hitX, hitY, hitZ); } } return enableStats; } @Override public boolean hasTileEntity(IBlockState state) { // TODO Auto-generated method stub return super.hasTileEntity(state); } @Override public TileEntity createTileEntity(World world, IBlockState state) { // TODO Auto-generated method stub return super.createTileEntity(world, state); } } I planned to then make the item unpickupable and not despawn. Then create an if statement checking if the player is holding shift when right clicking the block to remove the item. BlockTileEntity is another bit of code I wrote. I used autocomplete to find that method, and it does not create any errors in eclispe. According to its description it: "spawns the given ItemStack as an EntityItem into the World at the given position" It doesn't appear to work however public abstract class BlockTileEntity<TE extends TileEntity> extends BlockFocus { public BlockTileEntity(Material material, String name) { super(name, material); } public abstract Class<TE> getTileEntityClass(); public TE getTileEntity(IBlockAccess world, BlockPos pos) { return (TE)world.getTileEntity(pos); } @Override public boolean hasTileEntity(IBlockState state) { return true; } @Nullable @Override public abstract TE createTileEntity(World world, IBlockState state); @Override public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumHand hand, EnumFacing facing, float hitX, float hitY, float hitZ) { // TODO Auto-generated method stub return super.onBlockActivated(worldIn, pos, state, playerIn, hand, facing, hitX, hitY, hitZ); } } for clarification purposes, I am having trouble rendering an item on top of my special block which in the code is made using the class BlockFocus. I would like to make said item act as if it is on a pedestal and for the most part uninterruptible unless someone shift right clicks on the BlockFocus which would remove said item from the pedistal allowing it to despawn and behave like a normal item. Earlier I said I was having right click issues causing the placement of the block to trigger, however I somehow managed to fix that issue. Still need help summoning/rendering an item of choice after the block is right clicked.
-
I tried using this to summon an item when rightclicking it with another item, however it isn't doing anything BlockTileEntity.spawnAsEntity(worldIn, pos,itemstack); I think this may be due to the fact that i have been unable to register my tileentity class called BlockTileEntity. How do I go about this?