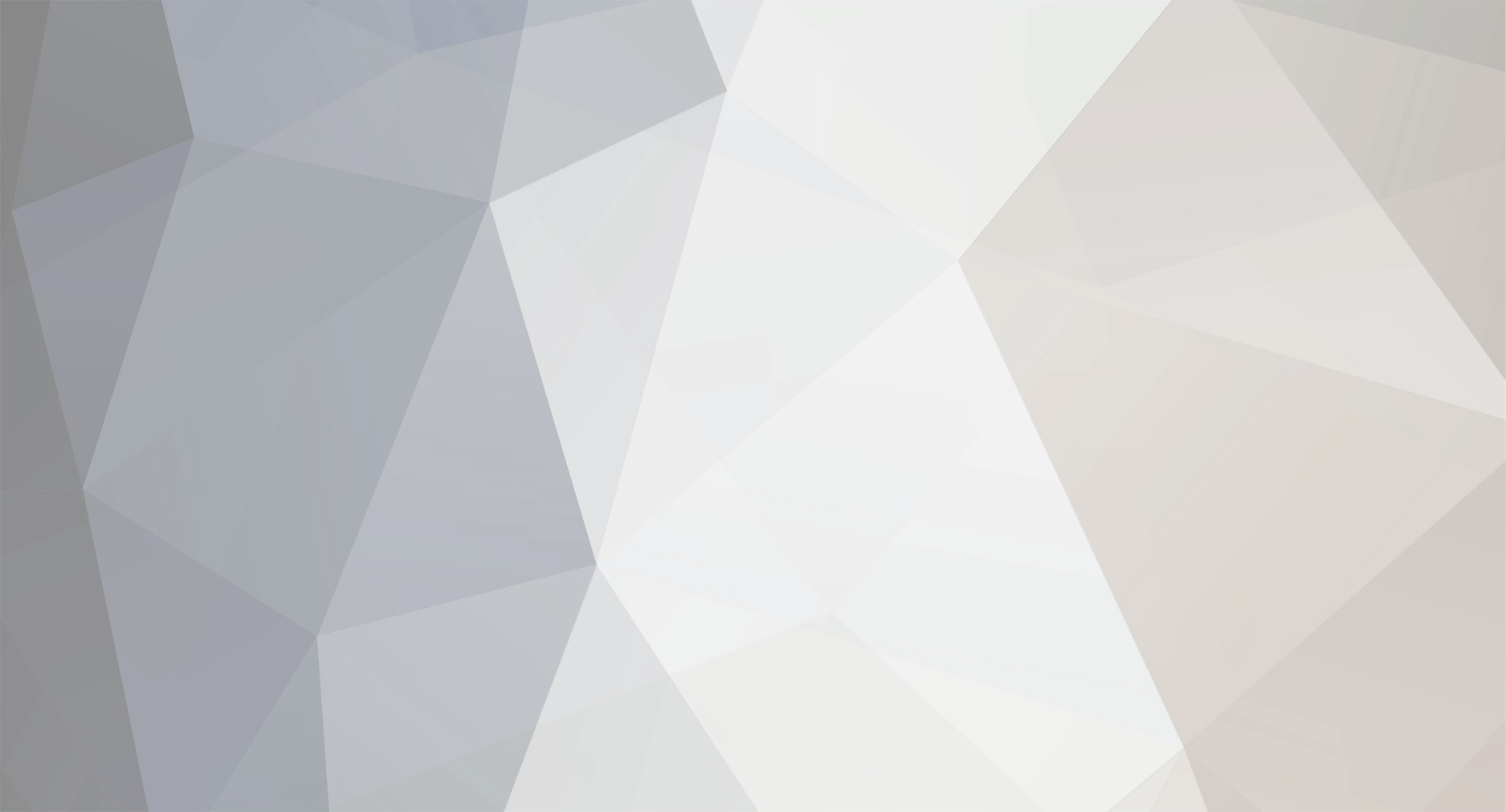
lisilew
Members-
Posts
56 -
Joined
-
Last visited
Everything posted by lisilew
-
How can it be done? Is it based on packets or can I simply import each other as library in IDE?
-
Can data be transferred between mod and plugin? For example, can plugin use items and enchantments made by mod? If I have economy plugin, can mod read and write amount of money a player has?
-
I am not sure when is checking sides appropriate or not. When I face problems like methods being called twice or some data from entity being not available, putting !world.isRemote usually solves them. So I have quite a lot of !world.isRemote checks and I felt like I was doing something wrong. This is what the documentation says and I am confused about game logic. What exactly is game logic and how do I determine if my operation is game logic and thus should run in logical server? And what kinds of operations should run in logical client?
-
Setters are also used by Capability.IStorage. Should readNBT method from Capability.IStorage also send packet?
-
Sorry for bringing this old topic to life. When I update the capability in getCapability().ifPresent(), do I have to update local capability and send packet or is just sending packet to PacketDistributor.TRACKING_ENTITY enough? entity.getCapability(Capabilities.CAPABILITY).ifPresent(capability -> { if (!capability.flag) { capability.flag = true; PacketHandler.INSTANCE.send(PacketDistributor.TRACKING_ENTITY.with(() -> entity), new Packet(entity.getEntityId(), true)); } }); In other words, is the third line, capability.flag = true;, needed?
-
I am using Blockbench. However, I cannot get position correctly. I use skin and add cubes to parts. Do I have to use modded entity? Because using position values from Blockbench places cubes differently on Minecraft. Also, the first layer has size of 1 and the second layer has size of 0.5. How should I apply these values on Blockbench?
-
I want to add wings on both sides of boots. So far, I have learned that my model needs to extend BipedModel and use addChild to attach ModelRenderer to parts. And my item needs to extend ArmorItem and override getArmorTexture and getArmorModel. What I do not understand is how to follow vanilla armor models. Vanilla armor model has to layers with size of 0.5 and 1. I have extended the first layer to 64 by 64 to include textures for wings. How should my model class look to attach wings on both sides of boots without creating two ModelRenderers? And how should my item class look to use two layers? I have spent hours to find some tutorials but they were all either recoloring vanilla textures or adding one model on head. Any examples of custom armor models on top of vanilla armor model would be really appreciated. Also, which program does everyone use when modelling?
-
The class where onStartTracking is located is annotated with @Mod.EventBusSubscriber(modid = "examplemod"). Unless events are annotated with @OnlyIn(Dist.CLIENT), I listen to events in that class. PacketDistributor.PLAYER.with requires a supplier of ServerPlayerEntity. I casted event.getPlayer() to ServerPlayerEntity. Is this safe or should I divide the current class into client and server events? I am still quite confused about sides and in which sides events are fired.
-
Oh, so when the packet is sent to PacketDistributor.TRACKING_ENTITY, the packet is sent from server to players, thus, handled by clients and Minecraft.getInstance().world is safe to access.
-
Then, do I need different packet that uses Minecraft.getInstance().player instead of ServerPlayerEntity or just change declaration type to PlayerEntity?
-
public class MyPacket { private int id; private boolean flag; public MyPacket(int id, boolean flag) { this.id = id; this.flag = flag; } public MyPacket(PacketBuffer packetBuffer) { id = packetBuffer.readInt(); flag = packetBuffer.readBoolean(); } public void encode(PacketBuffer packetBuffer) { packetBuffer.writeInt(id); packetBuffer.writeBoolean(flag); } public void handle(Supplier<NetworkEvent.Context> supplier) { NetworkEvent.Contxext context = supplier.get(); context.enqueueWork(() -> { ServerPlayerEntity player = context.getSender(); if (player != null) { Entity entity = player.world.getEntityByID(id); if (entity != null) { entity.getCapability(Capabilities.MY_CAPABILITY).ifPresent(capability -> { capability.setFlag(flag); }); } } }); context.setPacketHandled(true); } Above is the packet that is sent using PacketHandler.INSTANCE.send(PacketDistributor.TRACKING_ENTITY.with(() -> entity), new MyPacket(entity.getEntityId(), true)); when the capability changes from initially false to true under a condition. @SubscribeEvent public static void onStartTracking(PlayerEvent.StartTracking event) { event.getTarget().getCapability(Capabilities.MY_CAPABILITY).ifPresent(capability -> { PacketHandler.INSTANCE.send(PacketDistributor.PLAYER.with(event.getPlayer()), new MyPacket(capability.getEntityId(), capability.getFlag())); }) } Is this correct way to synchronize the capability?
-
I apologize if this question is redundant and annoys you. I just started to understand capability system so I want to make everything clear. When you say PlayerEvent.StartTracking will be fired to send modified data from untracked entities, do you mean I have to do something when PlayerEvent.StartTracking is fired or Forge's capability system does the job of taking care of capability modification on untracked entities as long as I use PacketDistributor.TRACKING_ENTITY?
-
Thanks. This solved the issue. I might as well just extend AbstractArrowEntity to remove code duplication. Anyway, once again, thank you iamacatlolol, Animefan8888 and diesieben07 for taking your time and helping me out.
-
createArrow is called when I have CompoundArrowItem in my inventory whether I am in survival or creative mode. From enabling hitbox, I noticed that the entity is actually not spawned even though createArrow is called. Other mechanics like finding ammo from inventory and consuming ammo work.
-
What is the purpose of customeArrow? BowItem uses createArrow on ArrowItem to create AbstractArrowEntity. Then, it calls customeArrow(abstractArrowEntity). If I override that, arrow loses texture. If I do not, arrow uses vanilla texture. I assume that I am not correctly understanding what customeArrow is supposed to do.
-
When I use summon command, getEntityTexture is called and CompoundArrowEntity is summoned with my texture.
-
Constructor is called by RenderingRegistry.register but getEntityTexture is never called.
-
public class EntityTypes { private static final DeferredRegister<EntityType<?>> ENTITY_TYPES = new DeferredRegister<>(ForgeRegistries.ENTITIES, ExampleMod.ID); public static final RegistryObject<EntityType<CompoundArrowEntity>> COMPOUND_ARROW = register("compound_arrow", EntityType.Builder.<CompoundArrowEntity>create((p_create_1_, p_create_2_) -> new CompoundArrowEntity(p_create_2_), EntityClassification.MISC).size((float) 0.5, (float) 0.5)); private static <T extends Entity> RegistryObject<EntityType<T>> register(String name, EntityType.Builder<T> builder) { return ENTITY_TYPES.register(name, () -> builder.build(new ResourceLocation(ExampleMod.ID, name).toString())); } public static void register(IEventBus bus) { ENTITY_TYPES.register(bus); } } public class CompoundBowItem extends BowItem { public CompoundBowItem(Properties builder) { super(builder); } @Override public Predicate<ItemStack> getInventoryAmmoPredicate() { return getAmmoPredicate(); } @Override public Predicate<ItemStack> getAmmoPredicate() { return itemStack -> itemStack.getItem() instanceof CompoundArrowItem; } @Override public AbstractArrowEntity customeArrow(AbstractArrowEntity arrow) { return new CompoundArrowEntity(arrow.world); } } public class CompoundArrowItem extends ArrowItem { public CompoundArrowItem(Properties builder) { super(builder); } @Override public AbstractArrowEntity createArrow(World worldIn, ItemStack stack, LivingEntity shooter) { return new CompoundArrowEntity(worldIn); } } public class CompoundArrowEntity extends ArrowEntity { public CompoundArrowEntity(World world) { super(EntityTypes.COMPOUND_ARROW.get(), world); } @Override protected ItemStack getArrowStack() { return new ItemStack(Items.COMPOUND_ARROW.get()); } @Override public IPacket<?> createSpawnPacket() { return NetworkHooks.getEntitySpawningPacket(this); } } I have updated classes like above. I added customeArrow in CompoundBowItem and now debugger says constructor of CompoundArrowEntity is called. However, now it does not even have vanilla texture and only shooting sound is played.
-
Added breakpoints to all three constructors and it seems like it is not called. Then I am guessing that my arrow should extend AbstractArrowEntity as I cannot override two constructors from ArrowEntity with my arrow type.
-
I have set breakpoint on getEntityTexture and also put System.out.println but it seems like method is never called.
-
If I send packet using PacketDistributor.TRACKING_ENTITY whenever capability changes, will players who were not tracking that entity at that moment get updated when they starts tracking that entity? I am also going to add different capability to players so can you elaborate more on this or are you talking about PlayerEvent.Clone?
-
Can storing players in the capability be replaced with // Send to all players tracking this chunk INSTANCE.send(PacketDistributor.TRACKING_CHUNK.with(chunk), new MyMessage()); ?
-
@Override public IPacket<?> createSpawnPacket() { return NetworkHooks.getEntitySpawningPacket(this); } I did override it but it still uses vanilla arrow texture. Nothing related to CompoundArrowEntity was logged so I assume the issue is not about invalid resources. Do I need anything else other than PNG file under textures/entity/projectiles for EntityRenderer?
-
I have a capability that is attached to monsters. When I sync the capability, which events should I listen to? I was thinking LivingSpawnEvent for the first case and PlayerEvent.StartTracking for the third case. Are those two events enough or are there any events should I listen to other than those two?
-
This is how I registered the renderer and I think I do not have any issues with it. private void doClientStuff(final FMLClientSetupEvent event) { // do something that can only be done on the client LOGGER.info("Got game settings {}", event.getMinecraftSupplier().get().gameSettings); RenderingRegistry.registerEntityRenderingHandler(EntityTypes.COMPOUND_ARROW.get(), CompoundArrowRenderer::new); }