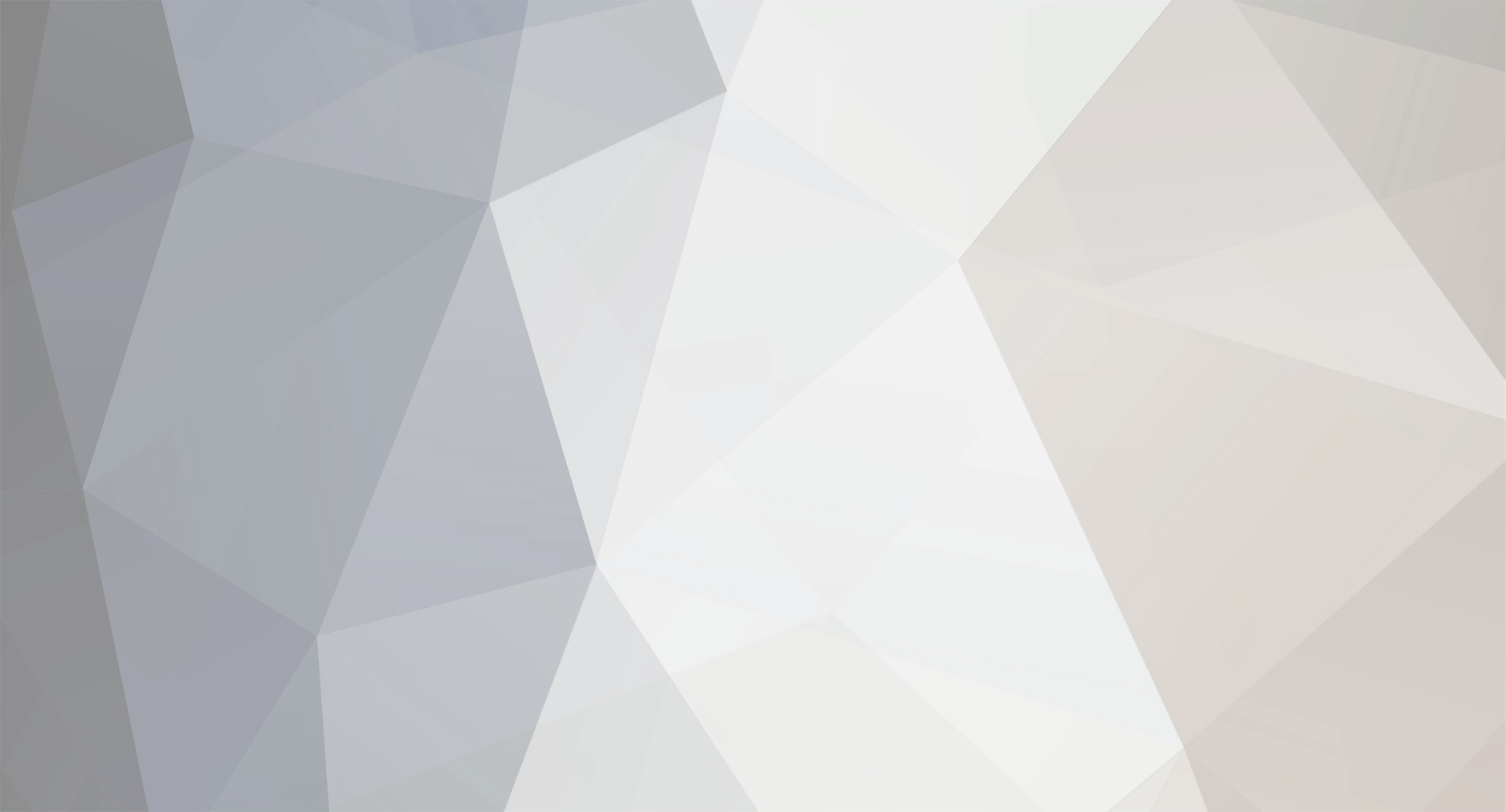
skip999
Members-
Posts
140 -
Joined
-
Last visited
Everything posted by skip999
-
Okay so I got the sound to play in the OnRightClickEvent handler. However, I cannot get it to play in the LivingHurtEvent handler. I have confirmed the sound itself plays correctly. However, it will not play, and I can't figure out why. I am out of ideas on how to get it to work, so I would appreciate some help. Here is the code for the handler methods: public static void takeDamageWithArmor(LivingHurtEvent event) { ItemStack[] ARMOR_PIECES = new ItemStack[] { new ItemStack(DeferredRegisters.COMPOSITE_ARMOR_PIECES.get(EquipmentSlotType.HEAD).get()), new ItemStack(DeferredRegisters.COMPOSITE_ARMOR_PIECES.get(EquipmentSlotType.CHEST).get()), new ItemStack(DeferredRegisters.COMPOSITE_ARMOR_PIECES.get(EquipmentSlotType.LEGS).get()), new ItemStack(DeferredRegisters.COMPOSITE_ARMOR_PIECES.get(EquipmentSlotType.FEET).get()) }; List<ItemStack> armorPieces = new ArrayList<>(); event.getEntityLiving().getArmorInventoryList().forEach(armorPieces::add); if(ItemStack.areItemsEqualIgnoreDurability(armorPieces.get(0), ARMOR_PIECES[3]) && ItemStack.areItemsEqualIgnoreDurability(armorPieces.get(1), ARMOR_PIECES[2]) && ItemStack.areItemsEqualIgnoreDurability(armorPieces.get(2), ARMOR_PIECES[1]) && ItemStack.areItemsEqualIgnoreDurability(armorPieces.get(3), ARMOR_PIECES[0]) ) { if(event.getAmount() <= NO_DAMAGE_DEALT) { if(!event.getSource().equals(DamageSource.OUT_OF_WORLD)) { event.setCanceled(true); } } ItemStack stack = armorPieces.get(2); stack.getCapability(CapabilityCeramicPlate.CERAMIC_PLATE_HOLDER_CAPABILITY).ifPresent(h ->{ if(event.getAmount() >= LETHAL_DAMAGE_AMOUNT && h.getPlateCount() > 0) { event.getEntityLiving().getEntityWorld().playSound( event.getEntityLiving().chunkCoordX, event.getEntityLiving().chunkCoordY, event.getEntityLiving().chunkCoordZ, SoundRegister.SOUND_CERAMICPLATEBREAKING.get(), SoundCategory.PLAYERS, 1, 1, false); event.setAmount((float) Math.sqrt(event.getAmount())); h.setPlateCount(h.getPlateCount() - 1); } }); } } public static void addPlateToArmor(RightClickItem event) { if(ItemStack.areItemsEqualIgnoreDurability(event.getItemStack(), new ItemStack(DeferredRegisters.SUBTYPEITEM_MAPPINGS.get(SubtypeCeramic.plate)))){ List<ItemStack> armorPieces = new ArrayList<>(); event.getEntityLiving().getArmorInventoryList().forEach(armorPieces::add); ItemStack chestplate = armorPieces.get(2); if(ItemStack.areItemsEqualIgnoreDurability(chestplate, new ItemStack(DeferredRegisters.COMPOSITE_ARMOR_PIECES.get(EquipmentSlotType.CHEST).get()))) { chestplate.getCapability(CapabilityCeramicPlate.CERAMIC_PLATE_HOLDER_CAPABILITY).ifPresent(h -> { if(h.getPlateCount() < 2) { event.getEntityLiving().getEntityWorld().playSound( event.getEntityLiving().chunkCoordX, event.getEntityLiving().chunkCoordY, event.getEntityLiving().chunkCoordZ, SoundRegister.SOUND_CERAMICPLATEADDED.get(), SoundCategory.PLAYERS, 1, 1, false); h.setPlateCount(h.getPlateCount() + 1); event.getItemStack().shrink(1); } }); } } }
-
Come to think of it, I couldn't find any tutorials on the topic for 1.16. If someone could point me to one, I would appreciate it?
-
Hello, I have been working on some custom armor, and have tried implementing a custom render model for it. I have followed the documentation and other examples as best I can, and with moderate sucess. However, I am having problems still. 1. Every armor piece renders the whole armor set. I'm not sure why this is happening as I handle that here: https://github.com/skiprocks999/Electrodynamics-JEI-Integration/blob/14e902979cdfcdb41910b5b6e67d2879fef0c12a/src/main/java/electrodynamics/common/player/armor/types/composite/CompositeArmorItem.java#L76 2. The armor is not moving with the player like it should. I have set it to, but it doesn't still. However, I feel this may be due to problem 1. https://github.com/skiprocks999/Electrodynamics-JEI-Integration/blob/14e902979cdfcdb41910b5b6e67d2879fef0c12a/src/main/java/electrodynamics/common/player/armor/types/composite/CompositeArmorModel.java#L18 I'm not quite sure what I'm doing wrong, so I would appreciate any help that you can give me. Thanks!
-
Well I got the potato part right thanks!
-
I tried doing the getWorld() option and it still is refusing to play. I have put a link to the code here on my github. Am I being a potato? https://github.com/skiprocks999/Electrodynamics-JEI-Integration/blob/2dda66479361c3739b69264e6c6942c1640c5db7/src/main/java/electrodynamics/api/capability/compositearmor/CapabilityCeramicPlateHandler.java#L63 The multiple playSound() calls are there due to my testing. I feel I should point that out.
-
So I would need to call something along the lines of event.getEntity().getWorld().playSound() (I know that's not the exact method call but I'm not at my computer rn) Would this not play it for everyone in the world regardless of location like the wither spawn sound? I want this to be a localized sound.
-
It's an EntityHurtEvent, and I have the entity (which in this case is the player) emit a sound using the .playSound() method. However according to the documentation this will only be heard by other players on a server. I want the sound to be heard by the player as well in single player.
-
I'm not in front of my computer right now, but I take it there is a Minecraft.getInstance().isSinglePlayer() call of some kind you can do to check.
-
Will that return null if it's a server world?
-
I was taking a look at that. How would I get an instance of PlayerClientEntity in my EventHandler? I tried casting and checking the instance of the LivingEntity that the event passes in, but that didn't work.
-
I decided to keep things simple and just do an RightClickEvent for it. As far as a custom render model is concerned, how would I go about implementing it. I know Urban mentioned BipedArmorLayer, but how would I go about ensuring my overrides are called in rendering?
-
I got it to work I was just being a potato and not understanding how listeners work.
-
Okay so I have created the Capability. My question is now how to attach it to an item when a new item is created. Would I use the ItemEvent?
-
Hello, I am trying to have an event play a custom sound when it fires. Specifically, it is a LivingHurtEvent. Calling the playSound() method of the event plays the sound on the server. This works fine, however the sound is not played in singleplayer as a result. What would I need to do in order to handle the case in singleplayer?
-
I can see the confusion here. What I am essentially trying to add is a "ceramic plate" system to the armor. When the player takes a certain amount of damage, a plate is used to reduce it. I need the armor piece to be able to "store" these plates as a value. However, this value would be unique to each piece of armor. I tried using the class constant method you mentioned, but doing that has the value appear across all pieces of that armor. I know NBT is the way to do this, but I don't know how to implement it.
-
Hello, I am trying to create a set of custom armor that has a value that is unique to each chestplate. I know that in order to do this, I need to assign an NBT tag to the armor. However, I am not quite sure how to do this. I essentially need this value to be stored like durability. If anyone could help me out here I would appreciate it.
-
What method would I need to override for the custom repairing. I have modified the getIsRepairable() method, now i just need to handle the item consumption and variable increment.
-
Well what I am trying to do is essentially implement a sort of "ceramic plate" function with the armor. If the player wearing it is in a full suit of it, has a plate in the armor, and is dealt lethal damage (i.e. greater than 20), then the plate "breaks" and the damage is reduced significantly. Otherwise, it behaves like normal armor.
-
What I want to have happen is that when the player is wearing my armor and recieves a certain amount of damage in it, then the amount of damage dealt is decreased. What I am concerned about happening is that this will occur along with the vanilla process that handles damage to players in armor, undoing what I want to do. Does that make more sense?
-
Would it be possible to override the vanilla event that handles damage with armor with my event? The problem I am concerned about is that both events will fire at the same time, nullifying what I am trying to do.
-
I was able to get the even working fairly easily. However, I can't seem to find how to get the amount of damage the attacking source deals. Looking around in eclipse for suggested method calls, I can't find a method that returns what I assume would be an integer representing this. Assuming an LivingDamangeEvent name "event", I assume it looks something like event.getSource().getDamageAmount(), but that option doesn't exist according to eclipse. What do I need to call to get this value?
-
Hello, I am attempting to create some custom armor, and I have a few questions. 1. I want to modify the way the game calculates the damage dealt to the player when wearing the armor. Essentially, I want to be able to check if the damage dealt is greater than 20 (or "Lethal" if that's an option ideally). I don't know which method I need to override in order to make this possible. 2. I want to be able to craft the armor with an item and "add" that item to the armor. Essentially when the armor is crafted with the item, the item is consumed and it's count in the armor increases. Ideally, I would like this done through the chestplate. How would I go about doing this? 3. I would like to do a custom render model for the armor. I have no idea how to do this as I have never done it before (think draconic evo armor model). I would appreciate any and all help that you can give me. Thanks in advance!
-
Hello, I am wrapping up some work on some custom recipe classes that I just made, and I have a small annoyance with the latest.log I was hoping I could get some help on. Essentially, everything loads and works perfectly. However, the log will display this (I have attached the full log for more details) for all my custom recipes: I am not sure why it is doing this. I can only assume it's when Vanilla scans and forms its recipe categories, but I don't know how to fix it. Thanks for the help! latest.log
-
Hello, My question is simple: is there an easy way to convert from an instance of IInventory to an instance of IItemHandlerModifiable? The mod I am working with is very special, so it does not have an implementation of ItemStackHandler. Thanks in advance!
-
I finally tracked down the error. It actually was coming from another class entirely. At least I know what the issue is being caused by now. As a quick question, can anyone point me to a list of fluid tags please. I can't seem to find one.