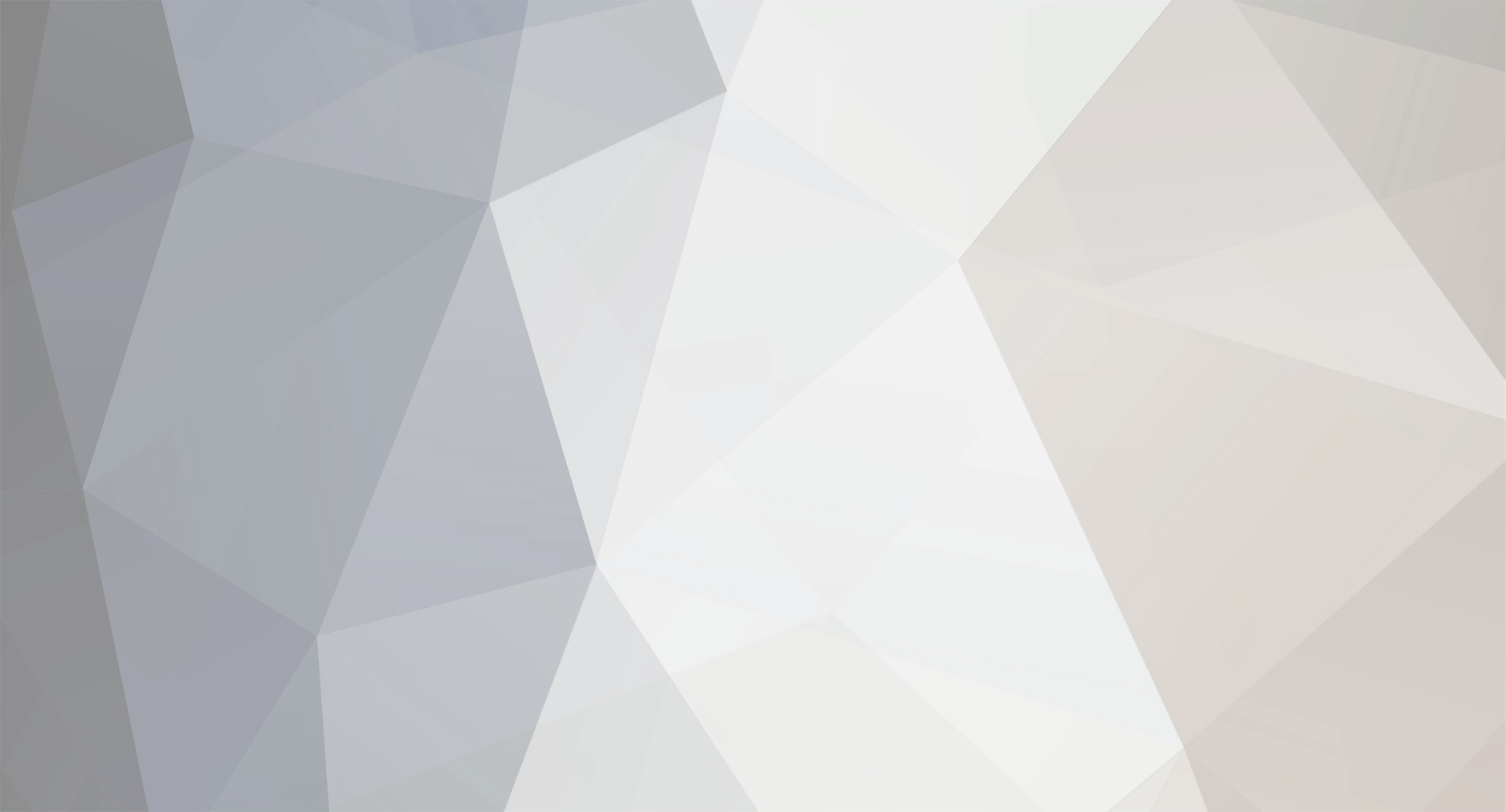
65_7a
Members-
Posts
12 -
Joined
-
Last visited
Everything posted by 65_7a
-
Since it's your own mod, you can use software to decompile the JAR and move your sources to a new mod project.
-
Thanks for responding. Is this the correct way to do it? @SubscribeEvent public static void onStruckByLightning(EntityStruckByLightningEvent e) { if (e.getEntity() instanceof PlayerEntity) { if (((PlayerEntity) e.getEntity()).isHolding(ItemInit.LIGHTNING_AXE.get())) { ItemStack stack = ((PlayerEntity) e.getEntity()).getMainHandItem(); if (stack.hasTag() && stack.getTag().contains(LIGHTNING_IMMUNITY_TAG) && stack.getTag().getLong(LIGHTNING_IMMUNITY_TAG) > e.getEntity().level.getGameTime()) { e.setCanceled(true); } } } } @SubscribeEvent public static void onAttackEntity(AttackEntityEvent e) { if (e.getTarget() instanceof LivingEntity) { PlayerEntity attacker = e.getPlayer(); if (attacker.level.dimension() == World.OVERWORLD && attacker.isHolding(ItemInit.LIGHTNING_AXE.get())) { attacker.getMainHandItem().getCapability(CapabilityEnergy.ENERGY, null).ifPresent(energyStorage -> { if (energyStorage.getEnergyStored() >= 500 && attacker.getAttackStrengthScale(0) >= 1.0F) { CompoundNBT nbt = attacker.getMainHandItem().getOrCreateTag(); nbt.putLong(LIGHTNING_IMMUNITY_TAG, e.getTarget().level.getGameTime() + 20L); LightningBoltEntity lightningBoltEntity = new LightningBoltEntity(EntityType.LIGHTNING_BOLT, e.getTarget().level); lightningBoltEntity.setPos(e.getTarget().getX(), e.getTarget().getY(), e.getTarget().getZ()); e.getTarget().level.addFreshEntity(lightningBoltEntity); e.getTarget().setSecondsOnFire(2); energyStorage.extractEnergy(500, false); attacker.addEffect(new EffectInstance(Effects.FIRE_RESISTANCE, 120, 0, false, false)); } }); } } }
-
I'm creating an item that strikes the target with lightning. I want to grant the attacker with temporary immunity to lightning. The way I plan to do this is setting an NBT tag on the ItemStack when an entity is attacked and removing it after 20 ticks or after the player is struck by lightning. I need help putting some sort of timer after an entity is attacked to set the NBT tag to false. Event Handler class: @Mod.EventBusSubscriber(modid = Main.MODID, bus = Mod.EventBusSubscriber.Bus.FORGE) public class EventHandler { @SubscribeEvent public static void onStruckByLightning(EntityStruckByLightningEvent e) { if (e.getEntity() instanceof PlayerEntity) { if (((PlayerEntity) e.getEntity()).isHolding(ItemInit.LIGHTNING_AXE.get())) { ItemStack stack = ((PlayerEntity) e.getEntity()).getMainHandItem(); if (stack.hasTag() && stack.getTag().contains(LIGHTNING_IMMUNITY_TAG) && stack.getTag().getBoolean(LIGHTNING_IMMUNITY_TAG)) { e.setCanceled(true); stack.getTag().putBoolean(LIGHTNING_IMMUNITY_TAG, false); } } } } @SubscribeEvent public static void onAttackEntity(AttackEntityEvent e) { if (e.getTarget() instanceof LivingEntity) { PlayerEntity attacker = e.getPlayer(); if (attacker.level.dimension() == World.OVERWORLD && attacker.isHolding(ItemInit.LIGHTNING_AXE.get())) { attacker.getMainHandItem().getCapability(CapabilityEnergy.ENERGY, null).ifPresent(energyStorage -> { if (energyStorage.getEnergyStored() >= 500 && attacker.getAttackStrengthScale(0) >= 1.0F) { CompoundNBT nbt = attacker.getMainHandItem().getTag(); if (nbt == null) { nbt = new CompoundNBT(); attacker.getMainHandItem().setTag(nbt); } nbt.putBoolean(LIGHTNING_IMMUNITY_TAG, true); // I want to put some sort of timer here to eventually set this to false. LightningBoltEntity lightningBoltEntity = new LightningBoltEntity(EntityType.LIGHTNING_BOLT, e.getTarget().level); lightningBoltEntity.setPos(e.getTarget().getX(), e.getTarget().getY(), e.getTarget().getZ()); e.getTarget().level.addFreshEntity(lightningBoltEntity); e.getTarget().setSecondsOnFire(2); energyStorage.extractEnergy(500, false); attacker.addEffect(new EffectInstance(Effects.FIRE_RESISTANCE, 120, 0, false, false)); } }); } } } } Other code can be found on my GitHub repo: https://github.com/65-7a/EnergyBasedGear/tree/lightning-axe Thanks for reading.
-
Thanks, that worked!
-
Hi, I've created a new custom inventory and the inventory doesn't seem to accept items from a hopper. I have used this as an example: https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe30_inventory_basic I've looked at Minecraft's ChestTileEntity.java and ChestContainer.java but I still can't figure it out. ExampleChestTileEntity.java: public class ExampleChestTileEntity extends TileEntity implements INamedContainerProvider { public static final int NUMBER_OF_SLOTS = 9; private final ExampleChestContents exampleChestContents; public ExampleChestTileEntity() { super(TileEntityTypeInit.EXAMPLE_CHEST.get()); exampleChestContents = ExampleChestContents.createForTileEntity(NUMBER_OF_SLOTS, this::canPlayerAccessInventory, this::setChanged); exampleChestContents.setOpenInventoryNotificationLambda(() -> playSound(SoundEvents.CHEST_OPEN)); exampleChestContents.setCloseInventoryNotificationLambda(() -> playSound(SoundEvents.CHEST_CLOSE)); } public boolean canPlayerAccessInventory(PlayerEntity player) { if (this.level.getBlockEntity(this.getBlockPos()) != this) return false; // Check if tileentity is still there final double X_CENTRE_OFFSET = 0.5; final double Y_CENTRE_OFFSET = 0.5; final double Z_CENTRE_OFFSET = 0.5; final double MAXIMUM_DISTANCE_SQ = 8.0 * 8.0; return player.distanceToSqr( getBlockPos().getX() + X_CENTRE_OFFSET, getBlockPos().getY() + Y_CENTRE_OFFSET, getBlockPos().getZ() + Z_CENTRE_OFFSET ) < MAXIMUM_DISTANCE_SQ; // Check if player isn't too far away } private static final String INVENTORY_NBT = "contents"; @Override public CompoundNBT save(CompoundNBT parentNBTTagCompound) { super.save(parentNBTTagCompound); // Save position CompoundNBT inventoryNBT = exampleChestContents.serializeNBT(); parentNBTTagCompound.put(INVENTORY_NBT, inventoryNBT); // Save chest contents return parentNBTTagCompound; } @Override public void load(BlockState blockState, CompoundNBT parentNBTTagCompound) { super.load(blockState, parentNBTTagCompound); // Load position CompoundNBT inventoryNBT = parentNBTTagCompound.getCompound(INVENTORY_NBT); exampleChestContents.deserializeNBT(inventoryNBT); // Load chest contents if (exampleChestContents.getContainerSize() != NUMBER_OF_SLOTS) throw new IllegalArgumentException("Corrupted NBT: Number of inventory slots did not match expected."); } @Nullable @Override public SUpdateTileEntityPacket getUpdatePacket() { CompoundNBT nbtTagCompound = new CompoundNBT(); save(nbtTagCompound); int tileEntityType = 42; // arbitrary number return new SUpdateTileEntityPacket(this.getBlockPos(), tileEntityType, nbtTagCompound); } @Override public void onDataPacket(NetworkManager net, SUpdateTileEntityPacket packet) { BlockState blockState = level.getBlockState(getBlockPos()); load(blockState, packet.getTag()); } @Override public CompoundNBT getUpdateTag() { CompoundNBT nbtTagCompound = new CompoundNBT(); save(nbtTagCompound); return nbtTagCompound; } @Override public void handleUpdateTag(BlockState state, CompoundNBT tag) { this.load(state, tag); } public void dropAllContents(World world, BlockPos blockPos) { InventoryHelper.dropContents(world, blockPos, exampleChestContents); } @Override public ITextComponent getDisplayName() { return new TranslationTextComponent("container.callumsmod.example_chest"); } private void playSound(SoundEvent soundEvent) { double x = (double) this.worldPosition.getX() + 0.5D; double y = (double) this.worldPosition.getY() + 0.5D; double z = (double) this.worldPosition.getZ() + 0.5D; this.level.playSound(null, x, y, z, soundEvent, SoundCategory.BLOCKS, 0.5F, this.level.random.nextFloat() * 0.1F + 0.9F); } @Nullable @Override public Container createMenu(int windowID, PlayerInventory playerInventory, PlayerEntity playerEntity) { return ExampleChestContainer.createContainerServerSide(windowID, playerInventory, exampleChestContents); } } Thanks.
-
package com.callumwong.sixsomod.events; import com.callumwong.sixsomod.SixSOMod; import net.minecraft.block.BlockState; import net.minecraft.block.Blocks; import net.minecraft.item.Items; import net.minecraft.util.math.BlockPos; import net.minecraftforge.common.extensions.IForgeBlockState; import net.minecraftforge.event.entity.player.PlayerInteractEvent; import net.minecraftforge.eventbus.api.SubscribeEvent; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventBusSubscriber.Bus; @Mod.EventBusSubscriber(modid = SixSOMod.MOD_ID, bus = Bus.FORGE) public class ClickWaterEvent { @SubscribeEvent public static void clickWaterEvent(PlayerInteractEvent.RightClickBlock event) { BlockPos blockPos = event.getPos().offset(event.getFace()); IForgeBlockState state = event.getWorld().getBlockState(blockPos); if (((BlockState) state).getBlock() == Blocks.WATER) { if(player.getCurrentEquuippedItem() != null){ if(player.getCurrentEquippedItem().getItem() == Items.GLASS_BOTTLE){ } } } } } I am very new to Minecraft Forge, and I am still a beginner at Java. Should I be learning more about Java before I start using Forge?
-
if(player.getCurrentEquuippedItem() != null){ if(player.getCurrentEquippedItem().getItem() == Items.GLASS_BOTTLE){ } } I tried this code. "player" was in red. Does "player" not exist? Is there a new way to access the current equipped item in newer versions?
-
I am very sorry, I don't understand what you mean by "use the player given to me". Do I have to use something other than Minecraft.getInstance().player? Is there something wrong with my code?
-
Thank you for your reply. I have made it so that when you right click a water block, it runs some code. The only thing I need to do now is to check if the player has a specific item in their hand, in my case a glass bottle. I have tried this: if(Minecraft.getInstance().player.getHeldItemMainhand().getItem() == Items.GLASS_BOTTLE) { SixSOMod.LOGGER.info("Right clicked water with glass bottle."); } But nothing was logged when I right clicked water with a glass bottle in my hand.
-
1.14 and above is supported on this forum.
-
I want to make it so when you click on water or a cauldron with a glass bottle, you have a 25% chance of getting an item other than the water bottle. (in my case, a "Salty Water Bottle".) If this is possible, I would like to know how to do this.
-
I want the output of a crafting recipe to be an enchanted item. Is this possible to do, and if so, how would I do it?