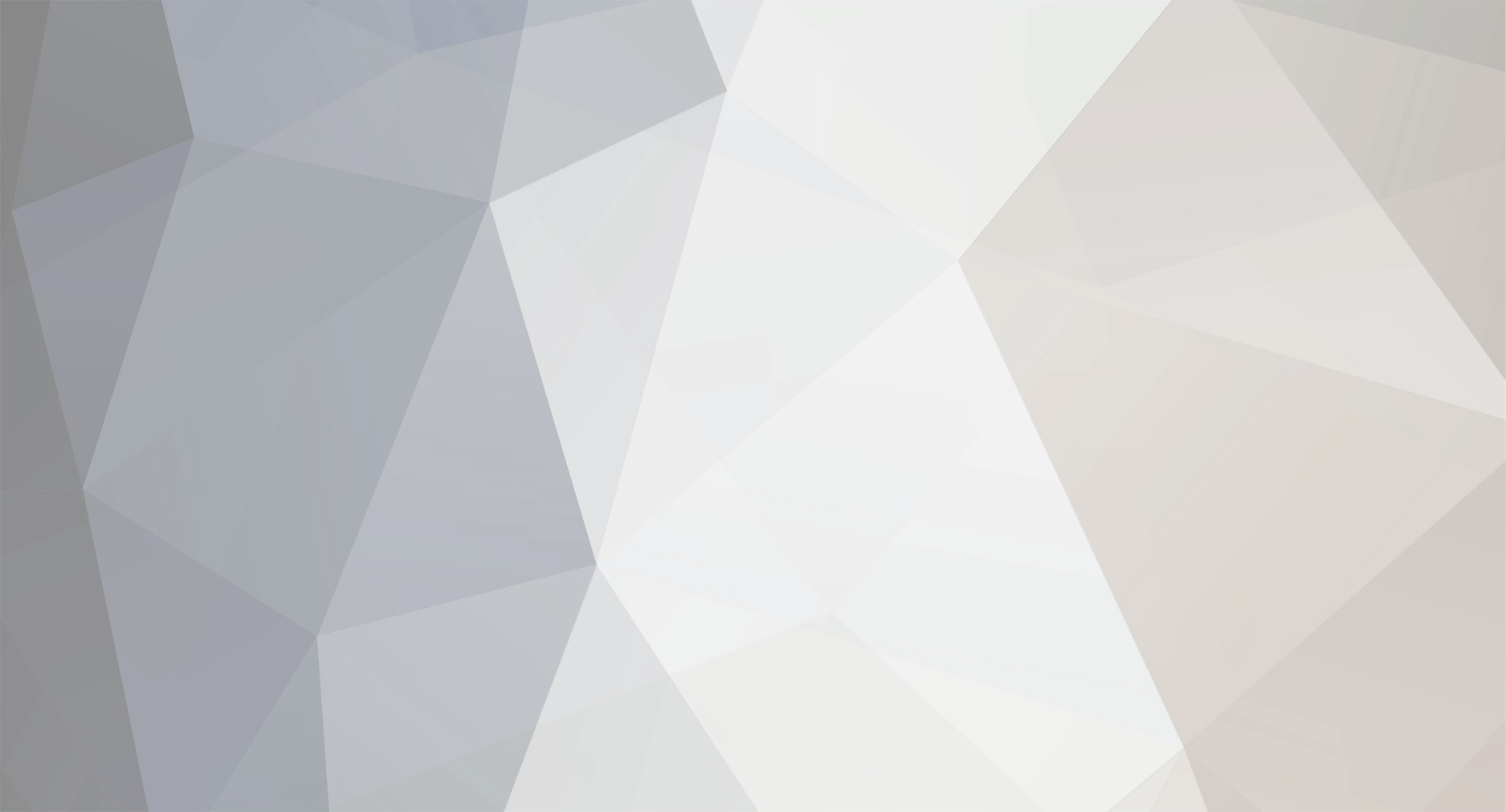
thomask
Members-
Posts
21 -
Joined
-
Last visited
Everything posted by thomask
-
I have a mob that utilizes the PlayerModel, and I'd like it to do the sitting animation (like when a player sits on a pig or in a minecart). How do i do this?
-
Hello, I've created an entity and I would like help giving it a chance to spawn in villages. Also for future reference, how do I spawn my mobs in a certain biome in general?
-
Hello, I'd like some pointers on how to go about creating an entity with the model of a player. I want it to have my custom texture, which is just a normal player skin. I was thinking that for my renderer class, I could extend LivingRenderer because PlayerRenderer does, override getEntityTexture to have the skin i want to use, and then copy over the relevant code from PlayerRenderer, but it doesnt seem like that'll work. As for my model class, should I even make my own? Or can I just use PlayerModel? Any guidance would be appreciated, thank you
-
I'm guessing it's not spawning? Because the arrow is supposed to create an explosion but it's not happening. UPDATE: I've tried using /summon to spawn my entity and my game crashes. Here's what happens: https://pastebin.com/suzV9HSu
-
Hey everybody, I have an entity based off of ArrowEntity that doesn't seem to be spawning. When I trigger onPlayerStoppedUsing it doesnt do anything. It does print the debug message "player stopped using staff" but nothing else I'm 99% sure the issue is with the way I register it because mostly everything was working before I messed with that. I was trying to create my own EntityType as before I was using a constructor that didn't take EntityType as an argument to spawn my entity. Here is how I'm spawning my entity: public class ExplosionStaff extends BowItem { public ExplosionStaff() { super(new Item.Properties().group(ItemGroup.COMBAT)); } @Override public void onPlayerStoppedUsing(ItemStack stack, World worldIn, LivingEntity playerIn, int timeLeft) { ExplosionSpellEntity spell = new ExplosionSpellEntity(RegistryHandler.SPELL_ENTITY.get(), worldIn); int i = this.getUseDuration(stack) - timeLeft; float f = super.getArrowVelocity(i); spell.shoot(playerIn, playerIn.rotationPitch, playerIn.rotationYaw, 0.0F, f * 4.0F, 0.2F); worldIn.addEntity(spell); System.out.println("Debug: player stopped using staff"); } } How I'm registering the entity: public class RegistryHandler { public static final DeferredRegister<Item> ITEMS = new DeferredRegister<>(ForgeRegistries.ITEMS, Megumin.MOD_ID); public static final DeferredRegister<EntityType<?>> ENTITY_TYPES = new DeferredRegister<>(ForgeRegistries.ENTITIES, Megumin.MOD_ID); public static void init() { ITEMS.register(FMLJavaModLoadingContext.get().getModEventBus()); ENTITY_TYPES.register((FMLJavaModLoadingContext.get().getModEventBus())); } // Items public static final RegistryObject<Item> STAFF = ITEMS.register("explosion_staff", ExplosionStaff::new); public static final RegistryObject<EntityType<ExplosionSpellEntity>> SPELL_ENTITY = ENTITY_TYPES.register("spell", () -> EntityType.Builder.<ExplosionSpellEntity>create(ExplosionSpellEntity::new, EntityClassification.MISC) .size(1f, 2f).build(new ResourceLocation(Megumin.MOD_ID, "spell").toString())); } The actual entity: public class ExplosionSpellEntity extends ArrowEntity { public ExplosionSpellEntity(EntityType<? extends ArrowEntity> type, World worldIn) { super(type, worldIn); } public ExplosionSpellEntity(World worldIn, double x, double y, double z) { super(worldIn, x, y, z); } public ExplosionSpellEntity(World worldIn, LivingEntity shooter) { super(worldIn, shooter); } @Override public void onHit(RayTraceResult raytraceResultIn) { System.out.println("Debug: spell entity hit"); this.world.createExplosion(this, this.getPosX(), this.getPosY(), this.getPosZ(), 20, false, Explosion.Mode.DESTROY); this.remove(); } @Override public void tick() { super.tick(); Vec3d vec3d = this.getMotion(); double d3 = vec3d.x; double d4 = vec3d.y; double d0 = vec3d.z; double d5 = this.getPosX() + d3; double d1 = this.getPosY() + d4; double d2 = this.getPosZ() + d0; this.world.addParticle(ParticleTypes.HEART, d5 - d3 * 0.25D, d1 - d4 * 0.25D, d2 - d0 * 0.25D, d3, d4, d0); System.out.println("DEBUG: arrow tick!"); } }
-
Hey, this is how I'm trying to do it so far. @Override public void tick() { super.tick(); this.world.addParticle(ParticleTypes.FLAME, this.getPosX(), this.getPosY(), this.getPosZ(), 0.0, 0.0, 0.0); System.out.println("DEBUG: arrow tick"); } My full class for my arrow: https://pastebin.com/41jCRgYu The particle's aren't showing, but the debug message is printing. Also, I'm not really sure what the particle speed values mean? On AbstractSpawner they're all set to 0 so that's what I did.
-
Send the full error message? And this is the forge installer jar, right?
-
Hey in the recent 1.15.2 mdk versions there is also a README.txt which should help you out. Has instructions for IDEA.
-
Title says it all, I'd like to spawn some vanilla particles (those fire looking ones from spawners) at a certain coordinate. This is for adding a visual effect around an arrow as it flies, so I was thinking I could spawn particles at the arrow's coordinate position at every tick.
-
Projectile that extends off ArrowEntity not shooting
thomask replied to thomask's topic in Modder Support
Thank you for your help as well! -
Projectile that extends off ArrowEntity not shooting
thomask replied to thomask's topic in Modder Support
Thank you so much, it works now. Guess I missed that while reading through BowItem lol -
Projectile that extends off ArrowEntity not shooting
thomask replied to thomask's topic in Modder Support
Hey, I was originally following a tutorial for my mod which registered the bow item like this public class RegistryHandler { public static final DeferredRegister<Item> ITEMS = new DeferredRegister<>(ForgeRegistries.ITEMS, Megumin.MOD_ID); public static void init() { ITEMS.register(FMLJavaModLoadingContext.get().getModEventBus()); } // Items public static final RegistryObject<Item> STAFF = ITEMS.register("explosion_staff", ExplosionStaff::new); } Do i need both of these? When I inserted your code into my main class along with what i already have (above code), I got this error https://pastebin.com/47ag730Q The interesting part seems to be this [23:46:22] [Server-Worker-3/WARN] [ne.mi.re.ForgeRegistry/REGISTRIES]: Registry Item: Override did not have an associated owner object. Name: megumin:explosion_staff Value: air Also, when I delete my registry handler and just use your thing, it's just the same problem as before. The same debug messages print, but no arrow. -
Hey guys, I am trying to make a projectile that extends off of ArrowEntity fire from my item based off of BowEntity. Here is my code for the Bow-based item: public class ExplosionStaff extends BowItem { public ExplosionStaff() { super(new Item.Properties().group(ItemGroup.COMBAT)); } @Override public void onPlayerStoppedUsing(ItemStack stack, World worldIn, LivingEntity playerIn, int timeLeft) { ExplosionSpellEntity spell = new ExplosionSpellEntity(worldIn, playerIn); spell.shoot(playerIn, playerIn.rotationPitch, playerIn.rotationYaw, 0.0F, 50.0F, 0.0F); System.out.println("Debug: player stopped using staff"); } } And here is the code for the arrow-based projectile: public class ExplosionSpellEntity extends ArrowEntity { public ExplosionSpellEntity(EntityType<? extends ArrowEntity> type, World worldIn) { super(type, worldIn); } public ExplosionSpellEntity(World worldIn, double x, double y, double z) { super(worldIn, x, y, z); } public ExplosionSpellEntity(World worldIn, LivingEntity shooter) { super(worldIn, shooter); } @Override public void shoot(Entity shooter, float pitch, float yaw, float p_184547_4_, float velocity, float inaccuracy) { float f = -MathHelper.sin(yaw * ((float)Math.PI / 180F)) * MathHelper.cos(pitch * ((float)Math.PI / 180F)); float f1 = -MathHelper.sin(pitch * ((float)Math.PI / 180F)); float f2 = MathHelper.cos(yaw * ((float)Math.PI / 180F)) * MathHelper.cos(pitch * ((float)Math.PI / 180F)); this.shoot((double)f, (double)f1, (double)f2, velocity, inaccuracy); this.setMotion(this.getMotion().add(shooter.getMotion().x, shooter.onGround ? 0.0D : shooter.getMotion().y, shooter.getMotion().z)); System.out.println("Debug: Shooted!!"); } @Override public void onHit(RayTraceResult raytraceResultIn) { System.out.println("Debug: spell entity hit"); this.world.createExplosion(this, this.getPosX(), this.getPosY(), this.getPosZ(), 20, false, Explosion.Mode.BREAK); } } As you can see, I put various debugging messages here. When I let go of the staff item, it displays the "Shooted!!" and "player stopped using staff" messages, but there is no arrow, so the onHit() debug doesn't print. What do i need to do? I couldn't find any tutorials on how to make projectiles for 1.15.2 so this is just my guess on where to start. I know I have to "register" entities but not sure how to do that. Thank you!
-
Check this out https://mcforge.readthedocs.io/en/latest/
-
Where to get started creating a custom projectile?
thomask replied to thomask's topic in Modder Support
thank you, will try my best -
Where to get started creating a custom projectile?
thomask replied to thomask's topic in Modder Support
yes, so how do i go about creating and using a new projectile entity? -
Where to get started creating a custom projectile?
thomask replied to thomask's topic in Modder Support
i was planning for a projectile that explodes on impact -
Where do i begin making a new type of projectile? I have created an item that extends off of the BowItem class, and I would like it to fire my own type of projectile when onPlayerStoppedUsing. Any advice or tutorial would be appreciated. This is for 1.15.2