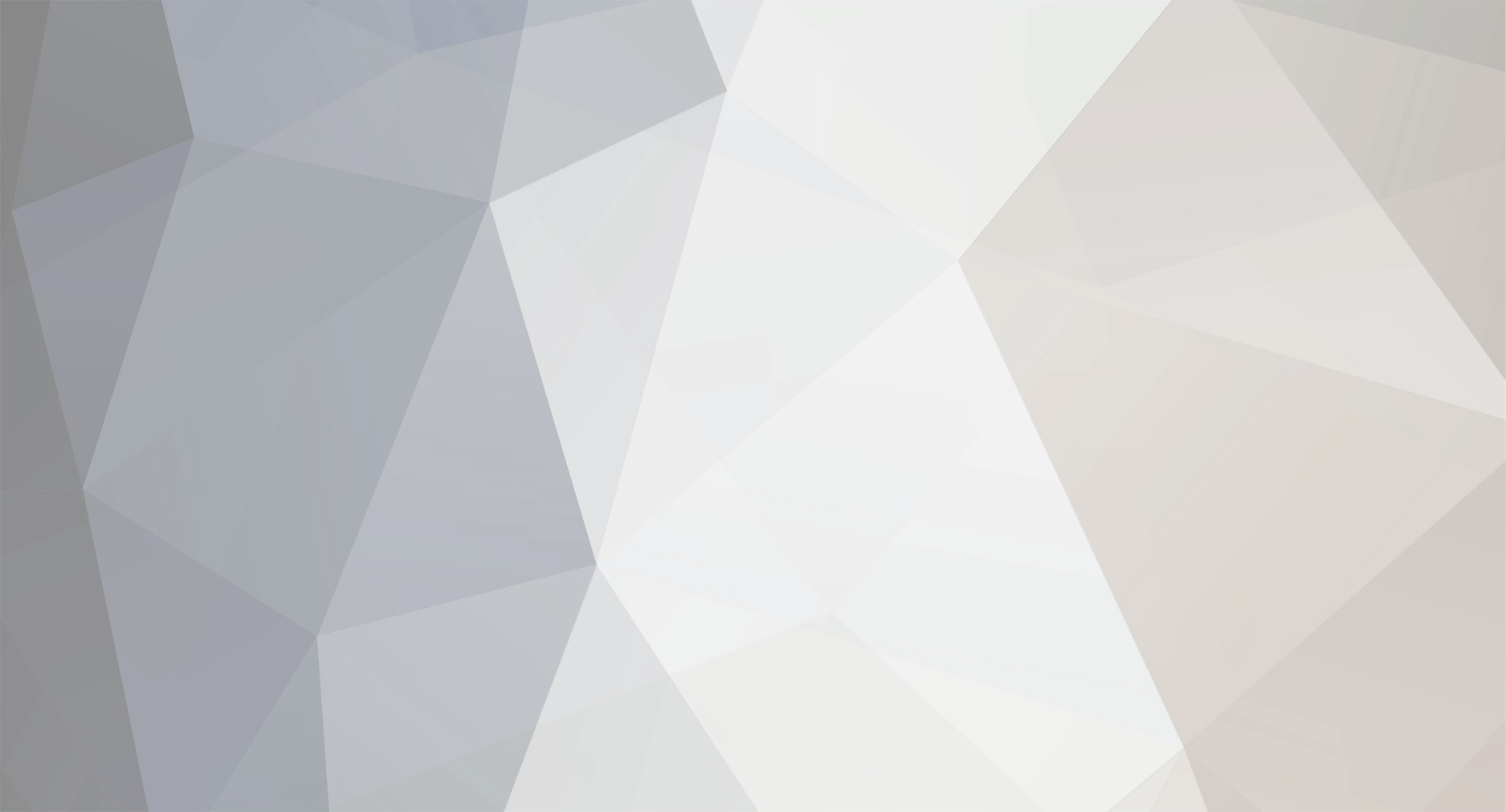
Giga_5
Members-
Posts
24 -
Joined
-
Last visited
Everything posted by Giga_5
-
I have also been told that implementing a biome through JSON can (and should) be done in some cases. How would you register a JSON biome?
-
I am still very new to biomes and world generation as a whole, but i thought i'd give it a shot anyways. My game refuses to acknowledge the biome, what did I do wrong? Biome class: import net.minecraft.entity.EntityClassification; import net.minecraft.entity.EntityType; import net.minecraft.world.biome.*; import net.minecraft.world.gen.GenerationStage; import net.minecraft.world.gen.carver.ConfiguredCarvers; import net.minecraft.world.gen.feature.Features; import net.minecraft.world.gen.feature.structure.StructureFeatures; import net.minecraft.world.gen.surfacebuilders.SurfaceBuilder; public class wastelandBiome { public static Biome wastelandBiomeGen() { BiomeGenerationSettings gen = new BiomeGenerationSettings.Builder() .surfaceBuilder(SurfaceBuilder.DEFAULT.configured(SurfaceBuilder.CONFIG_DESERT)) .addCarver(GenerationStage.Carving.AIR, ConfiguredCarvers.CAVE) .addFeature(GenerationStage.Decoration.LAKES, Features.LAKE_WATER) .addFeature(GenerationStage.Decoration.UNDERGROUND_ORES, Features.ORE_COAL) .addFeature(GenerationStage.Decoration.UNDERGROUND_ORES, Features.ORE_IRON) .addFeature(GenerationStage.Decoration.UNDERGROUND_ORES, Features.ORE_GOLD) .addFeature(GenerationStage.Decoration.UNDERGROUND_ORES, Features.ORE_REDSTONE) .addFeature(GenerationStage.Decoration.UNDERGROUND_ORES, Features.ORE_DIAMOND) .addFeature(GenerationStage.Decoration.UNDERGROUND_ORES, Features.ORE_LAPIS) .addStructureStart(StructureFeatures.MINESHAFT) .addStructureStart(StructureFeatures.STRONGHOLD) .addFeature(GenerationStage.Decoration.LAKES, Features.LAKE_LAVA) .build(); BiomeAmbience ambience = new BiomeAmbience.Builder() .waterColor(0x2e284f) .grassColorOverride(0x665c50) .waterFogColor(0x00d0c17) .fogColor(0x606769) .skyColor(0x3d4142) .build(); MobSpawnInfo mobStuff = new MobSpawnInfo.Builder() .addSpawn(EntityClassification.MONSTER, new MobSpawnInfo.Spawners(EntityType.SPIDER, 100, 4, 8)) .addSpawn(EntityClassification.MONSTER, new MobSpawnInfo.Spawners(EntityType.ZOMBIE, 100, 4, 8)) .addSpawn(EntityClassification.MONSTER, new MobSpawnInfo.Spawners(EntityType.ZOMBIE_VILLAGER, 5, 1, 1)) .addSpawn(EntityClassification.MONSTER, new MobSpawnInfo.Spawners(EntityType.SKELETON, 100, 4, 8)) .addSpawn(EntityClassification.MONSTER, new MobSpawnInfo.Spawners(EntityType.CREEPER, 100, 4, 4)) .addSpawn(EntityClassification.MONSTER, new MobSpawnInfo.Spawners(EntityType.SLIME, 100, 4, 4)) .addSpawn(EntityClassification.MONSTER, new MobSpawnInfo.Spawners(EntityType.ENDERMAN, 10, 1, 4)) .addSpawn(EntityClassification.MONSTER, new MobSpawnInfo.Spawners(EntityType.WITCH, 5, 1, 1)) .build(); return new Biome.Builder() .generationSettings(gen) .specialEffects(ambience) .mobSpawnSettings(mobStuff) .biomeCategory(Biome.Category.PLAINS) .temperatureAdjustment(Biome.TemperatureModifier.NONE) .depth(0.12f) .scale(0.01f) .precipitation(Biome.RainType.RAIN) .temperature(0.5f) .downfall(0.4f) .build(); } } Biome initializer: import mod.giga5.deepmod.holder; import net.minecraft.util.RegistryKey; import net.minecraft.world.biome.Biome; import net.minecraftforge.common.BiomeDictionary; import net.minecraftforge.common.BiomeManager; import net.minecraftforge.eventbus.api.SubscribeEvent; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.event.lifecycle.FMLCommonSetupEvent; import net.minecraftforge.registries.ForgeRegistries; import java.util.Objects; @Mod.EventBusSubscriber(modid = "deepmod", bus = Mod.EventBusSubscriber.Bus.MOD) public class biomeInit { @SubscribeEvent public static void setupBiomes(FMLCommonSetupEvent event) { event.enqueueWork(() -> setupBiome(holder.WASTELAND_BIOME, BiomeManager.BiomeType.DESERT, 100000, BiomeDictionary.Type.OVERWORLD, BiomeDictionary.Type.DEAD, BiomeDictionary.Type.FOREST, BiomeDictionary.Type.HILLS) ); } private static void setupBiome(Biome biome, BiomeManager.BiomeType type, int weight, BiomeDictionary.Type... types) { RegistryKey<Biome> key = RegistryKey.create( ForgeRegistries.Keys.BIOMES, Objects.requireNonNull(ForgeRegistries.BIOMES.getKey(biome)) ); BiomeDictionary.addTypes(key, types); BiomeManager.addBiome(type, new BiomeManager.BiomeEntry(key, weight)); } } Registerer: import mod.giga5.deepmod.biomes.wastelandBiome; import mod.giga5.deepmod.main; import net.minecraft.world.biome.Biome; import net.minecraftforge.eventbus.api.IEventBus; import net.minecraftforge.fml.RegistryObject; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.javafmlmod.FMLJavaModLoadingContext; import net.minecraftforge.registries.DeferredRegister; import net.minecraftforge.registries.ForgeRegistries; @Mod.EventBusSubscriber(modid = "deepmod", bus = Mod.EventBusSubscriber.Bus.MOD) public class deferredregister { public static final DeferredRegister<Biome> BIOMES = DeferredRegister.create(ForgeRegistries.BIOMES, main.MODID); public static final RegistryObject<Biome> WASTELAND_BIOME = BIOMES.register("wasteland_biome", wastelandBiome::wastelandBiomeGen); public static void register() { IEventBus modEventBus = FMLJavaModLoadingContext.get().getModEventBus(); BIOMES.register(modEventBus); } } I am not usually familiar with DeferredRegister, as I usually just use the RegistryEvents. However I was told biomes can be screwed up if done with the standard RegistryEvents, so I went with deferred. thanks!
-
Nevermind, i fixed it
-
Hello! I'm new to oregen, and trying to dip my toes in the water so to speak. My oregen currently doesnt work, and none of my ore spawns in the world. I have tried both CountRange and DepthAverage for the configuration, neither have worked. here is my class to set oregen: public class GenerateCStone { public static void generateCStone() { for(Biome biome : ForgeRegistries.BIOMES) { ConfiguredPlacement<DepthAverageConfig> genPlacementConfig = Placement.COUNT_DEPTH_AVERAGE.configure(new DepthAverageConfig(200, 40, 60)); biome.addFeature(GenerationStage.Decoration.UNDERGROUND_ORES, Feature.ORE .withConfiguration(new OreFeatureConfig(OreFeatureConfig.FillerBlockType.NATURAL_STONE, Storage.CSTONE.getDefaultState(), 7)) .withPlacement(genPlacementConfig)); } } } and here's the registerer to register the generator to the game: @Mod.EventBusSubscriber(modid = Main.MODID, bus = Mod.EventBusSubscriber.Bus.MOD) public class UtilRegister { @SubscribeEvent void setup(FMLCommonSetupEvent e) { DeferredWorkQueue.runLater(GenerateCStone::generateCStone); } } Any help would be appreciated!
-
is this a mappings related issue?
-
20200723-1.16.1, the latest one according to the forge discord bot
-
I am making a custom armor material for my armor but it seems to break no matter what i do. If I enter func_230304f() as a class method, it complains about the lack of getKnockbackResistence(), and vice versa. The error message is also confusing, saying ABTier is not abstract and does not override abstract method getKnockbackResistance() in IArmorMaterial To my knowledge, IArmorMaterial has no method called getKnockbackResistence(). If I include both, It throws a ClassFormatError for duplicate methods on startup. What should I do? Here is the code for my armor item tier: public enum ABTier implements IArmorMaterial { BOMBSHIRT("bombshirt", 80, new int[] {2, 2, 2, 2}, 0, SoundEvents.ITEM_ARMOR_EQUIP_GENERIC, 0.0F, 0.5F, null); private static final int[] MAX_DAMAGE_ARRAY = new int[]{9, 10, 12, 13}; private final String name; private final int maxDamageFactor; private final int[] damageReductionAmountArray; private final int enchantability; private final SoundEvent soundEvent; private final float toughness; private final float knockbackResistance; private final Lazy<Ingredient> repairMaterialLazy; private ABTier(String nameIn, int maxDamageFactorIn, int[] damageReductionAmountArrayIn, int enchantabilityIn, SoundEvent soundEventIn, float toughnessIn, float knockbackResistanceIn, Supplier<Ingredient> repairMaterialSupplier) { this.name = Main.MODID + ":" + nameIn; this.maxDamageFactor = maxDamageFactorIn; this.damageReductionAmountArray = damageReductionAmountArrayIn; this.enchantability = enchantabilityIn; this.soundEvent = soundEventIn; this.toughness = toughnessIn; this.knockbackResistance = knockbackResistanceIn; this.repairMaterialLazy = Lazy.concurrentOf(repairMaterialSupplier); } @Override public int getDurability(EquipmentSlotType slotIn) { return this.maxDamageFactor; } @Override public int getDamageReductionAmount(EquipmentSlotType slotIn) { return this.damageReductionAmountArray[slotIn.getIndex()]; } @Override public int getEnchantability() { return this.enchantability; } @Override public SoundEvent getSoundEvent() { return this.soundEvent; } @Override public Ingredient getRepairMaterial() { return this.repairMaterialLazy.get(); } @OnlyIn(Dist.CLIENT) public String getName() { return this.name; } @Override public float getToughness() { return this.toughness; } public float func_230304_f_() { return this.knockbackResistance; } public float getKnockbackResistance() { return this.knockbackResistance; } }
-
Are there any ways to change a player’s vision, similar to how nightvision/nausea/blindness changes vision? Or are they hard coded? I wanted to create a “sun scorched” effect that increases exposure on a player’s screen, making it extremely bright. Would this be possible?
-
Hello! Novice modder here. I am creating an item that spawns a creeper at the player's location upon player death. It works fine, however I am trying to make it so that the KPS ItemStack is shrank (shrunk?) by 1 each time the player dies, as if the items are being "consumed" by spawning a creeper. How would I go about doing this? Here is my current code: public class KPSEvent { @SubscribeEvent public void onDie(LivingDeathEvent e) { LivingEntity k = e.getEntityLiving(); ItemStack sl = new ItemStack(Holder.KPS); CreeperEntity c = new CreeperEntity(EntityType.CREEPER, k.getEntityWorld()); if(e.getEntity() instanceof PlayerEntity) { if(((PlayerEntity) e.getEntity()).inventory.hasItemStack(sl)) { ((PlayerEntity) e.getEntity()).inventory.deleteStack(sl); c.setPosition(k.getPosX(), k.getPosY()+1, k.getPosZ()); k.getEntityWorld().addEntity(c); } } } }
-
Hello! Sorry if this is a duplicate post, but I couldn't seem to find any other sources. How do you get a player's spawn point? I want to create a food item that will teleport the player to their spawnpoint upon eating it, however I have hit a roadblock when it comes to actually retrieving a player's spawnpoint. Any help would be appreciated!
-
Ahh it worked! thank you for the help. I plan on adding different "types" of enderdust soon to teleport different lengths, I just havent done the NBT stuff yet
-
Hello! RayTracing is still a very new concept to me so this code may be very bad. I am making a mod that adds an enchantment that is supposed to teleport the player where the are looking when holding a certain item, enderdust. Currently it works, however the teleportation is very janky. I get teleported backards, side to side, into blocks, and almost never directly where I am looking. Any help would be appreciated. Code for the teleportation event: public class TeleBootEvent { @SubscribeEvent public void teleBoot(PlayerInteractEvent.RightClickItem event) { PlayerEntity player = event.getPlayer(); Vector3d eyePos = player.getEyePosition(1.0F); BlockRayTraceResult result = player.getEntityWorld().rayTraceBlocks(new RayTraceContext(eyePos, player.getLookVec(), RayTraceContext.BlockMode.COLLIDER, RayTraceContext.FluidMode.NONE, player)); double x = result.getPos().getX(); double y = result.getPos().getY(); double z = result.getPos().getZ(); ItemStack armor = player.getItemStackFromSlot(EquipmentSlotType.FEET); ItemStack enderdust = new ItemStack(ObjectHolderStorage.ENDERDUST); if (EnchantmentHelper.getEnchantmentLevel(ObjectHolderStorage.TELEBLINK, armor) > 0) { if (result.getType() == RayTraceResult.Type.BLOCK) { if (player.getHeldItemMainhand().isItemEqual(enderdust)) { player.setPosition(x, y + 0.5, z); } } } } }
-
World#rayTraceBlocks doesn't seem to still be a thing in 1.16.
-
Hello! This may be a noobish question, but i'm trying to get a better understanding of forge functions. How does one properly implement a raytrace? Say, for instance, I wanted to get the coordinates of a block a player is looking at, outside of the normal "reach" range. How would I go about doing this? Any help would be appriciated
-
[1.16.1] How to apply custom logic to a projectile from an enchanted bow?
Giga_5 replied to Giga_5's topic in Modder Support
do I do this just by registering the bow-based item with the namespace "minecraft:bow"? -
[1.16.1] How to apply custom logic to a projectile from an enchanted bow?
Giga_5 replied to Giga_5's topic in Modder Support
I guess what I’m trying to ask is how do I (if possible) make it so vanilla bows use a different, modified version of onPlayerStoppedUsing instead of the default one? It seems like all of the modifiers that set the arrow’s state from the bow’s enchantment are in there. -
[1.16.1] How to apply custom logic to a projectile from an enchanted bow?
Giga_5 replied to Giga_5's topic in Modder Support
How would i go about overriding the onPlayerStoppedUsing method? -
I'm probably looking in the completely wrong places, but how do bow enchantments change the ArrowEntity fired from the bow, so that they execute logic on impact? (i.e. flame). I've figured out how to apply and use custom enchantments for tools and armor, but bows seem to be strange because they create entities. Can someone point me in the right direction?
-
Hello! I created a custom enchantment that is supposed to work like knockback, but it sends the victim in a random direction at a random velocity depending on the enchantment level. It all works perfectly in a single player environment, but upon hitting a mob with a "Glitch" sword with separate physical client and server, the server crashes with a noSuchMethodError exception. I'm fairly new to modding so I don't fully understand how sides work. Any help would be appreciated. here is the event that executes logic upon a mob being hit with a glitch weapon: public class glitchevent { @SubscribeEvent public void onglitch(AttackEntityEvent e) { Entity target = e.getTarget(); PlayerEntity attacker = e.getPlayer(); int level = EnchantmentHelper.getEnchantmentLevel(ObjectHolderStorage.GLITCH, attacker.getHeldItemMainhand()); Random r = new Random(); int ins = r.nextInt(level + 2); double launchmultiplier = ins; double invertedlaunchmultiplier = ins - ins * ins; int test = r.nextInt(4); World world = e.getPlayer().world; if (!world.isRemote) { switch (test) { case 0: target.setVelocity(launchmultiplier, 0.1D, launchmultiplier); break; case 1: target.setVelocity(invertedlaunchmultiplier, 0.1D, launchmultiplier); break; case 2: target.setVelocity(invertedlaunchmultiplier, 0.1D, invertedlaunchmultiplier); break; case 3: target.setVelocity(launchmultiplier, 0.1D, invertedlaunchmultiplier); break; } } } } and here are the exceptions thrown on the server: [12Aug2020 00:21:21.669] [Server thread/ERROR] [net.minecraftforge.eventbus.EventBus/EVENTBUS]: Exception caught during firing event: net.minecraft.entity.Entity.func_70016_h(DDD)V Index: 1 Listeners: 0: NORMAL 1: ASM: mod.extraenchants.events.glitchevent@2c63f610 onglitch(Lnet/minecraftforge/event/entity/player/AttackEntityEvent;)V java.lang.NoSuchMethodError: net.minecraft.entity.Entity.func_70016_h(DDD)V at mod.extraenchants.events.glitchevent.onglitch(glitchevent.java:36) at net.minecraftforge.eventbus.ASMEventHandler_2_glitchevent_onglitch_AttackEntityEvent.invoke(.dynamic) at net.minecraftforge.eventbus.ASMEventHandler.invoke(ASMEventHandler.java:85) at net.minecraftforge.eventbus.EventBus.post(EventBus.java:297) at net.minecraftforge.common.ForgeHooks.onPlayerAttackTarget(ForgeHooks.java:719) at net.minecraft.entity.player.PlayerEntity.func_71059_n(PlayerEntity.java:1035) at net.minecraft.entity.player.ServerPlayerEntity.func_71059_n(ServerPlayerEntity.java:1280) at net.minecraft.network.play.ServerPlayNetHandler.func_147340_a(ServerPlayNetHandler.java:1127) at net.minecraft.network.play.client.CUseEntityPacket.func_148833_a(SourceFile:74) at net.minecraft.network.play.client.CUseEntityPacket.func_148833_a(SourceFile:13) at net.minecraft.network.PacketThreadUtil.func_225383_a(SourceFile:21) at net.minecraft.util.concurrent.TickDelayedTask.run(SourceFile:18) at net.minecraft.util.concurrent.ThreadTaskExecutor.func_213166_h(SourceFile:144) at net.minecraft.util.concurrent.RecursiveEventLoop.func_213166_h(SourceFile:23) at net.minecraft.server.MinecraftServer.func_213166_h(MinecraftServer.java:735) at net.minecraft.server.MinecraftServer.func_213166_h(MinecraftServer.java:157) at net.minecraft.util.concurrent.ThreadTaskExecutor.func_213168_p(SourceFile:118) at net.minecraft.server.MinecraftServer.func_213205_aW(MinecraftServer.java:718) at net.minecraft.server.MinecraftServer.func_213168_p(MinecraftServer.java:712) at net.minecraft.util.concurrent.ThreadTaskExecutor.func_213160_bf(SourceFile:103) at net.minecraft.server.MinecraftServer.func_213202_o(MinecraftServer.java:697) at net.minecraft.server.MinecraftServer.func_240802_v_(MinecraftServer.java:647) at net.minecraft.server.MinecraftServer.lambda$func_240784_a_$0(MinecraftServer.java:230) at java.base/java.lang.Thread.run(Thread.java:834) [12Aug2020 00:21:21.825] [Server thread/ERROR] [net.minecraft.server.MinecraftServer/]: Encountered an unexpected exception java.lang.NoSuchMethodError: net.minecraft.entity.Entity.func_70016_h(DDD)V at mod.extraenchants.events.glitchevent.onglitch(glitchevent.java:36) ~[?:1.2] at net.minecraftforge.eventbus.ASMEventHandler_2_glitchevent_onglitch_AttackEntityEvent.invoke(.dynamic) ~[?:?] at net.minecraftforge.eventbus.ASMEventHandler.invoke(ASMEventHandler.java:85) ~[eventbus-3.0.3-service.jar:?] at net.minecraftforge.eventbus.EventBus.post(EventBus.java:297) ~[eventbus-3.0.3-service.jar:?] at net.minecraftforge.common.ForgeHooks.onPlayerAttackTarget(ForgeHooks.java:719) ~[?:?] at net.minecraft.entity.player.PlayerEntity.func_71059_n(PlayerEntity.java:1035) ~[?:?] at net.minecraft.entity.player.ServerPlayerEntity.func_71059_n(ServerPlayerEntity.java:1280) ~[?:?] at net.minecraft.network.play.ServerPlayNetHandler.func_147340_a(ServerPlayNetHandler.java:1127) ~[?:?] at net.minecraft.network.play.client.CUseEntityPacket.func_148833_a(SourceFile:74) ~[?:?] at net.minecraft.network.play.client.CUseEntityPacket.func_148833_a(SourceFile:13) ~[?:?] at net.minecraft.network.PacketThreadUtil.func_225383_a(SourceFile:21) ~[?:?] at net.minecraft.util.concurrent.TickDelayedTask.run(SourceFile:18) ~[?:?] at net.minecraft.util.concurrent.ThreadTaskExecutor.func_213166_h(SourceFile:144) ~[?:?] at net.minecraft.util.concurrent.RecursiveEventLoop.func_213166_h(SourceFile:23) ~[?:?] at net.minecraft.server.MinecraftServer.func_213166_h(MinecraftServer.java:735) ~[?:?] at net.minecraft.server.MinecraftServer.func_213166_h(MinecraftServer.java:157) ~[?:?] at net.minecraft.util.concurrent.ThreadTaskExecutor.func_213168_p(SourceFile:118) ~[?:?] at net.minecraft.server.MinecraftServer.func_213205_aW(MinecraftServer.java:718) ~[?:?] at net.minecraft.server.MinecraftServer.func_213168_p(MinecraftServer.java:712) ~[?:?] at net.minecraft.util.concurrent.ThreadTaskExecutor.func_213160_bf(SourceFile:103) ~[?:?] at net.minecraft.server.MinecraftServer.func_213202_o(MinecraftServer.java:697) ~[?:?] at net.minecraft.server.MinecraftServer.func_240802_v_(MinecraftServer.java:647) ~[?:?] at net.minecraft.server.MinecraftServer.lambda$func_240784_a_$0(MinecraftServer.java:230) ~[?:?] at java.lang.Thread.run(Thread.java:834) [?:?]
-
Hello! I know this question is probably asked a lot, but I couldn't find any relevant ones for 1.16. How do you color StringTextComponent lore text without color codes? I have seen the methods mergeStyle(TextFormatting) and applyTextStyle(TextFormatting) thrown around, however neither seems to be a method for StringTextComponent or ITextComponent. I apologize if this is a simple question, i'm new to modding.
-
[1.15.2] How do I change the Description/Lore of an item?
Giga_5 replied to xanderindalzone's topic in Modder Support
sorry to hijack this thread, but where would you call applyTextStyle from? it's not a StringTextComponent method... -
hello, newbie modder here. what type of value does Entity#getArmorInventoryList return? I am trying to make an event that runs custom logic when an arrow strikes an entity with a certain armor piece, and I have it all complete except for the part where the event tests if the entity is wearing the specific armor piece. How do I use getArmorInventoryList to test say, an entity's chestplate?
-
Help with ItemStack values across classes [1.16.1]
Giga_5 replied to Giga_5's topic in Modder Support
Thanks! that solves my problem -
Hello! I'm relatively new to modding and Java in general, but I have the basics down and understand how items/blocks/entities/etc. work. Here I am trying to make an item that takes the item in the player's offhand itemstack and launches it as an entity (that extends from SnowballEntity), and then lands as an item on the ground. It all works except that I cannot find I way for the item entity spawned on the ground at the end to detect what item was initially launched from the player's inventory. Here's my "launcher" item class; public class xplauncher extends Item { public xplauncher(Properties p) { super(p); } public ActionResult<ItemStack> onItemRightClick(World worldIn, PlayerEntity playerIn, Hand handIn) { ItemStack itmsk = playerIn.getHeldItem(handIn); ItemStack stack = playerIn.getHeldItemOffhand(); double lox = playerIn.getPosX(); double loy = playerIn.getPosY(); double loz = playerIn.getPosZ(); xpball ball = new xpball(worldIn, lox, loy, loz); ball.func_234612_a_(playerIn, playerIn.rotationPitch, playerIn.rotationYaw, 4.0F, 1.5F, 1.0F); if(!stack.isEmpty()) { if(!worldIn.isRemote) { ball.setItem(stack); worldIn.addEntity(ball); stack.shrink(1); } } else { System.out.println("Nothing to shoot"); } return ActionResult.func_233538_a_(itmsk, worldIn.isRemote()); } } And here's my "projectile" class; public class xpball extends SnowballEntity { ItemStack it = new ItemStack(); public xpball(World world, double x, double y, double z) { super(world, x, y, z); } protected void onImpact(RayTraceResult rt) { super.onImpact(rt); if (!this.world.isRemote) { this.world.addEntity(new ItemEntity(this.world, this.getPosX(), this.getPosY(), this.getPosZ(), it)); } this.remove(); } } My goal is for the "it" ItemStack in the projectile class to be somehow filled with the value of the "stack" ItemStack from the launcher class. Can anyone help me out? Constructive criticism is happily taken.