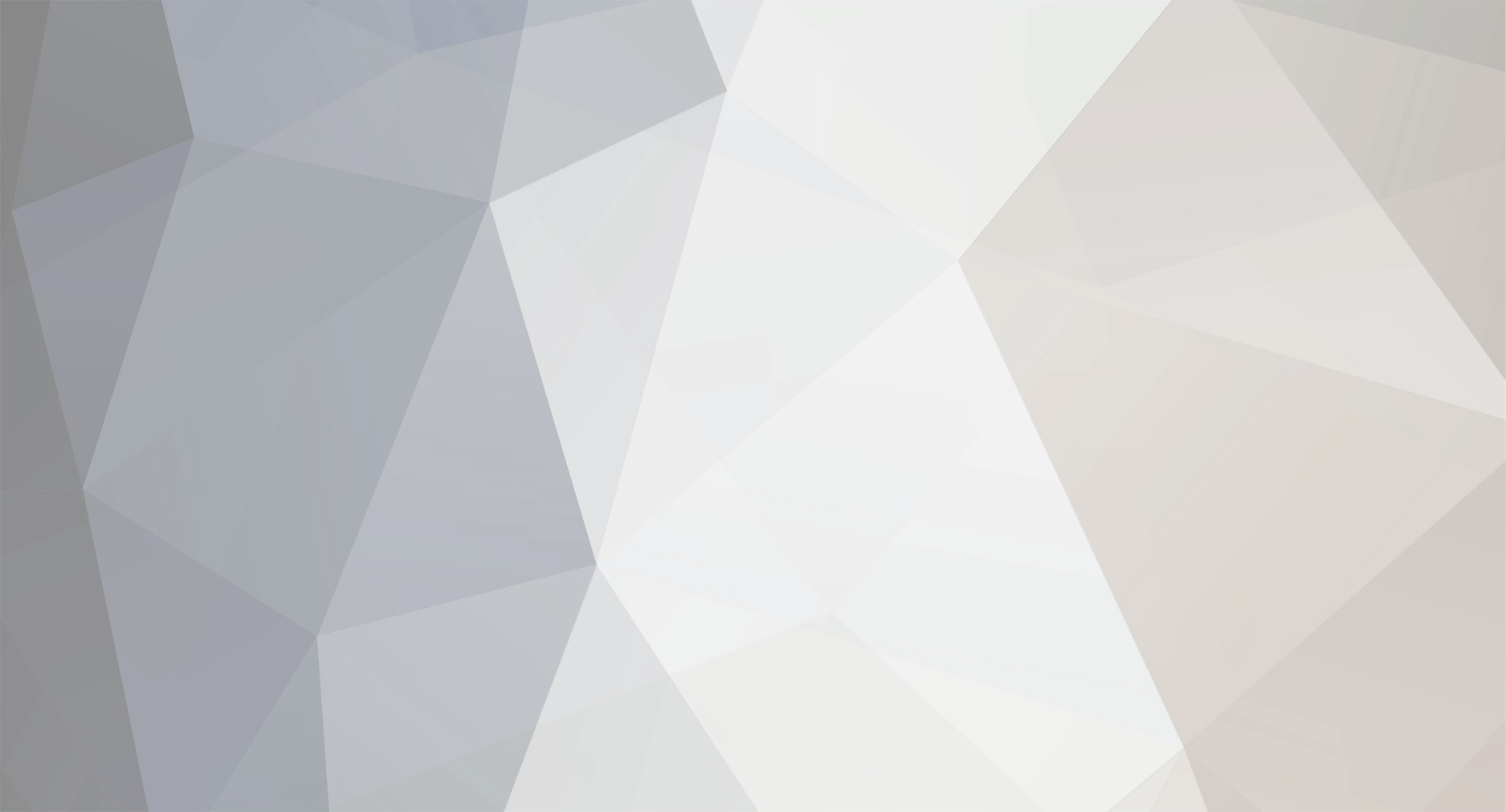
Otep
Members-
Posts
3 -
Joined
-
Last visited
Everything posted by Otep
-
Ok ok, so I made a capability which stores the counter which increases every tick (I used PlayerTickEvent and used the phase thing). So I got that out of the way, thanks for your help @diesieben07 and @vemerion! This is what it looks like btw: public class DelayCapability implements IDelay { private int points; @Override public void setPoints(int points) { this.points = points; } @Override public void subtractPoints(int points) { this.points -= points; } @Override public void addPoints(int points) { this.points += points; } @Override public int getPoints() { return this.points; } } I have another question, more of a logic thing. So let's say I have numerous items that wants to use my tick counter to use it for a delay (like wait for x number of ticks to pass before executing the next line of code) via my capability. How exactly can I make some sort of a function to delay the code using my capability and tick handler? These are my attempts: - Used a for loop to check the number of ticks (this is inside the actual item class) for (set number of times) { if (ticks % 40 == 0) { //do something } } ...But I quickly realized that loops ran faster than ticks so this didn't work (or only ran once) - Used a while loop inside another loop (pretty bad i know) for (set number of times){ while (boolean check){ if (check) { //do something //set check to false } } } ...This is my worst attempt because this crashed the game and hogged the CPU Any idea on how to implement such function in my item classes? Again, thank you guys for your responses, and I hope you'll be patient with me!
-
Thanks for your reply! After learning more about capabilities, I have successfully made my own (although the code is a bit messy haha). Regarding your recommendation, should this: // This code segment determines how many seconds has passed if (tickCounter==20) { //After 1 second do smth } else if (tickCounter==80) { tickCounter=0; // resets the counter back to 0 } tickCounter++; be inside the capability class as a method so the actual tick event would simply look like this: @SubscribeEvent public void delayEvent (net.minecraftforge.event.TickEvent.WorldTickEvent event) { delay.TickCounter(); } Or just simply the global variable? Sorry if these look messy or my English is off, I'm still learning!
-
Good Day! I desperately need some help with coding a general WorldTick handler in my custom mod (magical books). I plan on having multiple items that will use the tick handler after onPlayerStoppedUsing was called. An example of such usage is as follows: An item, when onPlayerStoppedUsing was called, will create a storm that summons lightning bolts that will strike the ground around the player per 20 ticks (which i heard was about one second). This is my main onPlayerStoppedUsing method: @Override public void onPlayerStoppedUsing(ItemStack stack, World worldIn, LivingEntity entityLiving, int timeLeft) { PlayerEntity playerEntity = (PlayerEntity) entityLiving; int a = this.getUseDuration(stack) - timeLeft; if (a >= 0) { //0 because testing purposes ---- this btw determines how long the player held and used the item for if (!worldIn.isRemote()) { //TODO add some stuff here that will determine the area around the player, then pass it to castTome castTome(stack, worldIn, playerEntity); } } } The code above works. This code below is my custom method belonging to a different class (which has the juicy parts): @Override public boolean castTome(ItemStack stack, World world, PlayerEntity playerEntity) { //Sets world rain to true if (!world.getWorldInfo().isRaining()) world.getWorldInfo().setRaining(true); //Summons lightning bolt ((ServerWorld) world).addLightningBolt(new LightningBoltEntity(world, this.posX, this.posY, this.posZ, true)); return true; } The above also works. The last code below is the tick handler I was using before: @SubscribeEvent public void ticker (TickEvent.WorldTickEvent event) { if (tickCounter==20) { //After 1 second do smth } else if (tickCounter==80) { tickCounter=0; // resets the counter back to 0 } tickCounter++; } Is there a way to tell the handler to count ticks and summon lightning every 20 ticks, without placing the actual 'summon lightning' (from the method castTome) code in the tick handler? As I said before, I aim to use this tick handler in almost all of my planned items, and I don't want to create multiple tick handlers which does different things. Thanks in advance!